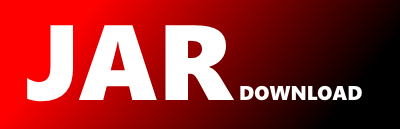
org.integratedmodelling.api.modelling.IExtent Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.modelling;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IProperty;
import org.integratedmodelling.api.modelling.IScale.Locator;
import org.integratedmodelling.collections.Pair;
import org.integratedmodelling.exceptions.KlabException;
/**
* An Extent describes the topology of the observable
* it's linked to. Conceptual models are capable of producing unions or intersections
* of extents.
*
* Extents must be conceptualizable and the result of conceptualizing
* them must be an observation describing the extent. They are also expected
* to implement equals() and hash() in a fast way (e.g. by using signatures).
*
* @author Ferdinando Villa
*
*/
public abstract interface IExtent extends IState, ITopology {
// return values for locate()
public static int INAPPROPRIATE_LOCATOR = -2;
public static int GENERIC_LOCATOR = -1;
/**
* Return the main concept for the topological class represented by this extent. It should
* be the same concept for all the different kinds of extents representing the same domain,
* i.e. geospace:Space, which should be an ancestor to the observable of this
* extent. It will be used to ensure that no two extents of the same domain concept
* appear in a context. The context implementation is expected to try to merge extents
* that share the same domain concept even if their observables are not the same.
*
* TODO remove and use getType()/getObservable()
*
* @return the domain concept for the extent
*/
public abstract IConcept getDomainConcept();
// /**
// * Get the property that should link this extent to a ITopologicallyComparable object
// * describing the extent of its coverage.
// * @deprecated obscure, if needed put inside
// * @return cov
// */
// @Deprecated
// public IProperty getCoverageProperty();
/**
* Collapse the multiplicity and return the extent that represents
* the full extent of our topology in one single state. This extent may
* not necessarily be of the same class.
*
* @return a new extent with getValueCount() == 1.
*/
public IExtent collapse();
/**
* Return the n-th state of the ordered topology as a new extent with one
* state.
*
* @param stateIndex
* @return a new extent with getValueCount() == 1.
*/
public IExtent getExtent(int stateIndex);
/**
* Return the single-valued topological value that represents the total extent covered, ignoring
* the subdivisions.
*
* @return the full, 1-dim extent.
*/
public ITopologicallyComparable> getExtent();
/**
* True if the i-th state of the topology correspond to a concrete subdivision where
* observations can be made. Determines the status of "data" vs. "no-data"
* for the state of an observation defined over this extent.
*
* @param stateIndex
* @return whether there is an observable world at the given location.
*/
public abstract boolean isCovered(int stateIndex);
/**
* Return an extent of the same domainConcept that represents the merge
* of the two. If force is false, this operation should just complete
* the semantics - e.g. add a shape to a grid or such - and complain
* if things are incompatible.
*
* If force is true, return an extent that is capable of representing the passed one through
* the "lens" of our semantics. Return null if the operation is legal but
* it results in no context, throw an exception if we don't know what to
* do with the passed context.
*
*
* @param extent
* @param force
* @return the merged extent
* @throws KlabException
*/
public IExtent merge(IExtent extent, boolean force) throws KlabException;
/**
* Try to cover the extent with the passed object and return the resulting
* coverage object and the proportion of the total extent covered by it.
*
* FIXME put inside, remove from API
* @param obj
* @return cov
* @throws KlabException
*/
public Pair, Double> checkCoverage(ITopologicallyComparable> obj)
throws KlabException;
/**
* True if the extent is completely specified and usable. Extents may
* be partially specified to constrain observation to specific representations
* or scales.
*
* @return true if consistent
*/
public boolean isConsistent();
/**
* Return true if this extent covers nothing.
* @return true if empty
*/
public abstract boolean isEmpty();
/**
* Each extent may have > 1 inner dimensions. If so, the linear offset (0 .. getMultiplicity())
* will be mapped to them according to their size and order. This one returns the full
* internal dimensionality. If the extent is one-dimensional, it will
* return new int[] { getMultiplicity() }
.
*
* @return dimension sizes
*/
public abstract int[] getDimensionSizes();
/**
* Translate a linear offset into the offsets for each dimension. If the dimension is 1,
* return the offset itself.
*
* @param linearOffset
* @param rowFirst if true, endeavor to return offset in the order that most closely
* resembles row-first ordering wrt euclidean x,y,z (default).
* @return dimension offsets
*/
int[] getDimensionOffsets(int linearOffset, boolean rowFirst);
/**
* Use the passed object to locate an unambiguous extent within the topology. If
* an extent can be located based on the passed object, return the index of the extent.
* If the locator is not usable by this extent, return IExtent.INAPPROPRIATE_LOCATOR. If
* the locator selects the whole extent, return GENERIC_LOCATOR. Passing a locator that
* does not fit the extent should be admitted. Do not throw errors but return INAPPROPRIATE_LOCATOR
* in that case.
*
* @param locator
* @return the offset corresponding to the locator
*/
int locate(Locator locator);
/**
* Get a state mediator to the passed extent. If extent is incompatible return null; if
* no mediation is needed, return an identity mediator, which all implementations should
* provide. Do not throw exceptions (unchecked exception only if called improperly).
*
* @param extent the foreign extent to mediate to.
* @param observable the observable - mediators will want to know it to establish the aggregation strategy.
* @param trait a data reduction trait to interpret the mediated values (may be null).
* @return the configured mediator or null
*/
public IState.Mediator getMediator(IExtent extent, IObservableSemantics observable, IConcept trait);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy