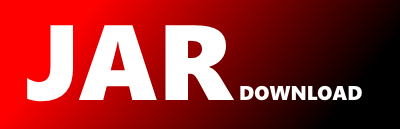
org.integratedmodelling.api.modelling.IModel Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa - integratedmodelling.org - any
* other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite, meant to enable
* modular, collaborative, integrated development of interoperable data and model
* components. For details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or modify it under the terms
* of the Affero General Public License Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful, but without any
* warranty; without even the implied warranty of merchantability or fitness for a
* particular purpose. See the Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 59 Temple Place - Suite
* 330, Boston, MA 02111-1307, USA. The license is also available at:
* https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.modelling;
import java.util.Collection;
import java.util.List;
import org.integratedmodelling.api.knowledge.IProperty;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.collections.Pair;
import org.integratedmodelling.exceptions.KlabException;
/**
* A Model, i.e. a semantic annotation. When unresolved (not linking to states), it is a
* query that uses observers to produce its result observation. It must have at least one
* observable. If it has more, they must have been given observation semantics through
* other models in the same namespace.
*
* Models may be unresolved (extensional, i.e. they leave their observable specified only
* at a semantic level) or resolved (intensional, i.e. they can build their observations
* as they are and provide observation semantics for it).
*
*/
public interface IModel extends IObservingObject {
/**
* Return the semantics of all observables we are observing. The first in the list is
* the actual observable and must exist; the others are expected side-effects of
* observing the first, which must be connected to semantics (i.e. they are specified
* along with observers in the language, or have correspondent, unambiguous models in
* the same namespace).
*
* Secondary observables must be qualities even in agent models.
*
* @return all the observables in order of declaration.
*/
public List getObservables();
/**
* Return a newly created datasource initialized to use the passed monitor.
*
* @param monitor
*
* @return a new datasource if the model has the specifications for one, or null.
* @throws KlabException
*/
public IDataSource getDatasource(IMonitor monitor) throws KlabException;
/**
* Models that were given an inline value instead of a datasource will return it here.
*
* @return the inline value (model 20 as ...) if any.
*/
public Object getInlineValue();
/**
* Return a newly created object source initialized to use the passed monitor.
*
* @param monitor
*
* @return a new object source if the model has one specified in it, or null.
* @throws KlabException
*/
public abstract IObjectSource getObjectSource(IMonitor monitor) throws KlabException;
/**
* This will only be called in models that produce objects (isReificationModel() ==
* true) and have defined observers for attributes of the objects produced. It is used
* to create de-reifying data models by "painting" the object attributes over the
* context, ignoring the identity of the objects. For each attribute name returned,
* the method getAttributeObserver() must return a valid observer. Some of the
* attributes may be internally generated: for example, it is always possible to infer
* 'presence of' an object from an observation of the object itself.
*
* @return the list of dereified attributes with their observers
*/
public Collection> getAttributeObservers();
/**
* In reification models, return any attributes that must be matched to metadata of
* the resulting objects (such as name or label), along with the metadata property
* they represent.
*
* @return metadata from the dereification list
*/
public Collection> getAttributeMetadata();
/**
* Return the observer that made this observation and provides the full observation
* semantics for it. Data models have one observer, which may be a
* IConditionalObserver switching to others according to context. Object models (agent
* models) have no observer, so this returns null and their isReificationModel()
* method returns true.
*
* @return the observer in quality models, null in others.
*/
public IObserver getObserver();
/**
* Return true if this model can be computed on its own and has associated data.
* Normally this amounts to having a data/object source or an instantiator with
* getDependencies().size() == 0, but implementations may provide faster ways to
* inquire (e.g. without creating the datasource).
*
* Should really be named isIntensional() but let's stop the good terminology at
* reification to keep the API readable by the non-philosopher.
*
* @return true if model is a leaf in a dependency tree.
*/
public boolean isResolved();
/**
* True if the model is an instantiator, i.e. produces objects ('model each' in k.IM).
* If it is not, it's an 'explanatory' model, which explains/computes an existing
* observation by providing a narrative for its observation, rather than producing new
* observations.
*
* @return true if model creates direct observations
*/
public abstract boolean isInstantiator();
/**
* True if the model is expected to contextually specialize an abstract concept. Such
* models are used when a dependency, trait or role are abstract when resolved; if so,
* the model will make the necessary observation and return the most appropriate
* concrete concept to the resolver, which will then proceed in the contextualization.
*
* @return
*/
public abstract boolean isSpecializer();
/**
* Called by the resolver before a model is used so that it has a chance to verify
* runtime availability before being chosen for resolution.
*
* @return true if everything is OK for computation
*/
public boolean isAvailable();
/**
* Archetype models produce observations that are only expected to provide archetypes
* for the intended observables, i.e. have no pretension of completeness over the
* context. Such could be, for example, an object generator that knows that not all
* objects can be observed, or a quality observer that only sees a few points in a
* space. In this case, implementations may choose to use other models to fill in
* gaps, or lacking those, use the archetype model to learn how to observe the
* observable in full.
*
* @return true if model produces archetypes.
*/
public boolean isArchetype();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy