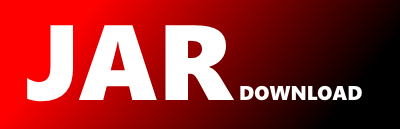
org.integratedmodelling.api.modelling.IObservableSemantics Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.modelling;
import org.integratedmodelling.api.factories.IModelManager;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IKnowledge;
import org.integratedmodelling.api.knowledge.ISemantic;
/**
* For tightness and efficiency, we define a specialized concept that describes the
* semantics of the observed
* incarnation of another concept. It provides specialized and efficient access to the
* restrictions that
* define the observation type and the inherent subject type (if any). If there is a model
* associated with the
* observation (anywhere except in a resolved IState) the IObservable provides access to
* that as well.
* If getSemantics() is called, the observable concepts reside in the observation
* namespace returned by
* {@link IModelManager#getObservationNamespace()}.
*
* @author Ferd
*/
public interface IObservableSemantics extends ISemantic {
/**
* An observable can be used to "explain" something already instantiated (or a state,
* which isn't
* really instantiated) or to instantiate its type, for subsequent explanation. The
* latter only
* applies to direct observables. We keep this distinction (corresponding to the
* presence of the
* 'each' keyword in the language) here for simplicity. Two observables must have the
* same action
* as well as the same observable in order to be equal.
*
* @author Ferd
*/
enum Action {
EXPLAIN,
INSTANTIATE
}
/**
* Return the action context in which this observable is employed. If INSTANTIATE, we
* are using it to
* create instances of the direct observable it describes. Otherwise we are applying
* it to an existing
* observation to compute its states.
*
* @return the context of existence of this observable
*/
Action getAction();
/**
* Return the model we're using to interpret the observable. This is only defined in
* model observables,
* and will return null in IStates.
*
* @return the model that's observing this, if any.
*/
IModel getModel();
/**
* Return the observer that made this observation. It will normally return
* getModel().getObserver(), but
* will be defined in IStates where getModel() returns null, and will return null when
* getModel() returns
* a subject model. To investigate the semantics of the observation,
* getObservationType() should be used
* instead of getObserver(), as that will be available in every situation, including
* past
* serialization/deserialization.
*
* @return the observer that's observing this, if any.
*/
IObserver getObserver();
/**
* Return the type of the observation that the un-observed type is restricted with.
* E.g. this may return
* NS.RANKING if we're looking at a numeric way of observing the observable. For
* subject observations, it
* will return NS.DIRECT_OBSERVATION. This will never return null.
*
* @return the type of observation being used.
*/
IConcept getObservationType();
/**
* An observable is always given a formal name that it can be referred to with. In
* models, this is the
* formal name specified by the modeler. If no formal name is specified, a sensible
* and decent-looking
* default should be provided.
*
* @return a name with which to refer to this
*/
String getFormalName();
/**
* Check identity of all concept known in both objects. All common concept must be
* what expected, no
* concepts must be not in common. For traits, we check that the trait we contain, if
* any, is
* inherited by the passed observable.
*
* @param observable
* @return true if the passed observable is semantically consistent with this.
*/
boolean is(IObservableSemantics observable);
/**
* Name of either the main type or the delegate type, if any.
*
* @return the best local name to be used
*/
String getLocalName();
/**
* Check identity of the main type only. Implies identity of the delegate type, which
* is forced to be a
* subclass of it.
*
* @param concept
* @return true if the passed knowledge is semantically consistent with this
*/
boolean is(IKnowledge concept);
/**
* Check identity of observation and observed type. Good to inquire whether e.g. we're
* quantifying or
* classifying.
*
* @param observationType
* @param observedType
* @return true in case of consistency
*/
boolean is(IConcept observationType, IConcept observedType);
/**
* Check identity of all types except trait.
*
* @param observationType
* @param observedType
* @param inherentToType
* @return true in case of consistency
*/
boolean is(IConcept observationType, IConcept observedType, IConcept inherentToType);
/**
* Check identity of all types. Like passing the correspondent observable.
*
* @param observationType
* @param observedType
* @param inherentToType
* @param traitType
* @return true in case of consistency
*/
boolean is(IConcept observationType, IConcept observedType, IConcept inherentToType, IConcept traitType);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy