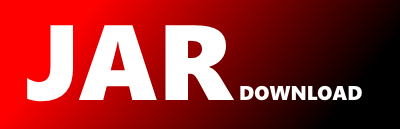
org.integratedmodelling.api.network.INetwork Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.network;
import java.util.Collection;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.api.services.IPrototype;
/**
* Each server builds a network by a mutual certificate sharing process. The hand-shaking between two connected
* nodes notifies capabilities, components and permissions. A network is a maximally connected, undirected
* graph of INodes.
*
* Users authenticate on one node only, and the nodes do not share authentication information. The various
* capabilities are allowed based only on groups, which are shared across the network.
*
* Embedded servers CONNECT to a network through their primary server if allowed, but are not part of it; only
* publicly reachable ones are.
*
* @author ferdinando.villa
*
*/
public interface INetwork {
/**
* A listener for network events. Is notified at on/offline (meaning depends on which
* kind of network) and when nodes go online or offline.
*
* @author Ferd
*
*/
public interface Listener {
/**
*
*/
void online();
/**
*
*/
void offline();
/**
* @param node
*/
void nodeOnline(INode node);
/**
* @param node
*/
void nodeOffline(INode node);
}
/**
* A poor man's map/reduce: this gets passed to broadcast() which will
* choose the nodes based on the output of acceptNode(), perform the
* executeCall() action on each node in independent threads, then merge()
* the result of all operations that did not fail and return.
*
* @author Ferd
* @param type of result for atomic node operation
* @param type of result for merge operation
*
*/
public static interface DistributedOperation {
/**
* Selects nodes that execute() will be broadcast to.
* @param node
* @return true if node provides the required functionalities.
*/
boolean acceptNode(INode node);
/**
* Executes the remote call that returns the individual node results.
* @param node
* @return results of the call
*/
T1 executeCall(INode node);
/**
* Merge results of all successful executeCall() into the final result
* of the distributed operation.
*
* @param results
* @return merged result returned by broadcast()
*/
T2 merge(Collection results);
}
/**
* Return all the nodes in a network.
*
* @return all nodes known to the network.
*/
Collection getNodes();
/**
* Get the node with a given name.
* @param s
* @return the named node or null.
*/
INode getNode(String s);
/**
* Networks are dynamic as nodes may go up and down in time. This should return true when the
* network has changed, and may be called by a thread any time. After checking for changes, it
* should reset the status to unchanged until another event happens.
*
* @return true if node configuration has changed since last called.
*/
boolean hasChanged();
/**
* The URL for the current instance if public, or that of the primary server if
* personal. Client networks return the URL of the personal engine.
*
* @return the URL. Never null.
*/
String getUrl();
/**
* Find the prototype for a service published by any connected node. Return null if not
* found.
*
* @param id
* @return a service prototype, or null.
*/
IPrototype findPrototype(String id);
/**
* Change the network status between online and offline and perform any actions
* necessary to implementing the change.
*
* @param active
*/
void activate(boolean active);
/**
* True if we are authenticated and connected.
*
* @return true if online.
*/
boolean isOnline();
/**
* Return all the nodes that provide a named resource. Pass a project id,
* component id, project or component (to check if anything else provides it) or a
* IResourceConfiguration.StaticResource constant..
*
* @param s
* @return true if any node in the network provides the named resource.
*/
Collection getNodesProviding(Object s);
/**
* Add a listener to monitor events.
*
* @param listener
*/
void addListener(Listener listener);
/**
* Call this one to "map" an operation on all matching nodes, then return
* the results of a "reduce" step on the results.
*
* @param operation
* @param monitor
* @return result of reduce over invoked map
*/
T2 broadcast(DistributedOperation operation, IMonitor monitor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy