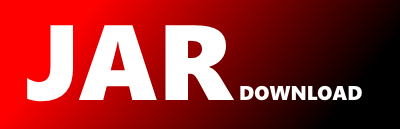
org.integratedmodelling.api.project.IProject Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.project;
import java.io.File;
import java.util.Collection;
import java.util.List;
import java.util.Properties;
import org.integratedmodelling.Version;
import org.integratedmodelling.api.lang.IParsingScope;
import org.integratedmodelling.api.modelling.INamespace;
import org.integratedmodelling.exceptions.KlabException;
/**
* k.LAB projects are plug-in packages that provide knowledge resources and data assets.
*
* @author Ferd
*/
public interface IProject {
/**
* Projects have a name. The project name is a key, cannot be the same in different projects,
* and it must be unque in the set of project and components.
*
* @return the project name
*/
public String getId();
/**
* Find a resource file or directory in the plugin. Extract it to a scratch
* area if necessary so it is a File that can be copied and
* used locally. Expected to be read only. Return null if not found.
*
* @param resource
* @return a file for the passed resource name, or null.
*/
public File findResource(String resource);
/**
* Installation directory. It needs to be non-null even if the plugin doesn't have resources.
*
* @return the project directory on the filesystem.
*/
public File getLoadPath();
/**
* Also must not return null.
*
* @return properties for the project, from META-INF/klab.properties.
*/
public Properties getProperties();
/**
* Projects are mandatorily versioned.
* @return version from klab.properties.
*/
public Version getVersion();
/**
* Return all the namespaces defined in the project.
*
* @return namespace list
*/
public Collection getNamespaces();
/**
* Source folders are scanned at startup and monitored during development. The location of
* source folders is in the SOURCE_FOLDER_PROPERTY of the thinklab properties. There is
* one source folder per project.
*
* @return the path of the source directory, relative to the project workspace.
*/
public String getSourceRelativePath();
/**
* Find the resource file that defines the passed namespace. If not
* found, return null unless returnIfAbsent is true.
*
* @param namespace
* @param returnIfAbsent if true, return the file where the namespace SHOULD be even if
* it does not exist.
* @return the file where the named namespace lives, or null.
*/
public File findResourceForNamespace(String namespace, boolean returnIfAbsent);
/**
* Find a namespace either in this project or in any of the
* prerequisites, using the context to load it if necessary. Return
* null if nothing is found.
* TODO move to ProjectManager
* @param namespace
* @param context
* @return the loaded namespace from this or another project in the project manager.
* @throws KlabException for errors during load
*/
public INamespace findNamespaceForImport(String namespace, IParsingScope context)
throws KlabException;
/**
* Get all projects we depend on. These should be ordered in load order.
*
* @return the set of project we depend on.
* @throws KlabException
*/
public abstract List getPrerequisites() throws KlabException;
/**
* Return when this was last modified, so that we can load efficiently. This should
* return the modification time of the newest resource.
*
* @return the last time anything in this project was modified.
*/
public long getLastModificationTime();
/**
* Return true if this provides a definition for the named namespace. Must be
* able to answer before loading anything.
*
* @param namespaceId
* @return true if the namespace id passed is provided by this project.
*/
boolean providesNamespace(String namespaceId);
/**
* Return the name of each top-level resource folder in the project that
* is not one of the automatically managed ones.
*
* @return names of all folders not recognized as "system" folders.
*/
public abstract List getUserResourceFolders();
/**
* Return true if any of the resources in the project has caused compilation errors. If
* this is true, the project should not be used.
*
* @return true if loading caused any errors from k.IM or binary components.
*/
public boolean hasErrors();
/**
* Get any error messages raised when loading the project.
*
* @return
*/
public List getErrors();
/**
*
* @return true if loading caused any warnings from k.IM or binary components.
*/
public boolean hasWarnings();
/**
* Return all the script files in the project. Those should be in their own directory.
*
* @return any script files in the project (partly unimplemented)
*/
public Collection getScripts();
/**
*
* @param resource
* @return return the namespace corresponding to a file.
*/
INamespace findNamespaceForResource(File resource);
/**
* A project that is closed is invisible to the project manager. The setting is
* permanent and persists across sessions and engines until open(true) is passed.
*
* @param open
*/
void open(boolean open);
/**
* Report the open/close status of this project.
*
* @return whether the project is open.
*/
boolean isOpen();
/**
* If true, the project has been downloaded and synchronized from a remote
* location at authentication. For this reason it should be considered read-only
* and not shown in editors.
*
* @return true if project is not in the main user workspace.
*/
boolean isRemote();
/**
* If the project is remote, this returns the ID of the originating network
* node.
*
* @return the node id. Returns null if isRemote() is false.
*/
String getOriginatingNodeId();
/**
* Worldview status is indicated by a thinklab.worldview = true property. Only one worldview
* is permitted and a worldview must exist.
*
* @return if the project is a worldview.
*/
boolean isWorldview();
/**
* Set the project version and persist it.
*
* @param version
* @throws KlabException if any error happened during persistence (e.g. read-only project).
*/
void setVersion(Version version) throws KlabException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy