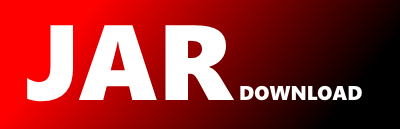
org.integratedmodelling.api.runtime.ISession Maven / Gradle / Ivy
package org.integratedmodelling.api.runtime;
import java.io.Closeable;
import java.util.List;
import org.integratedmodelling.api.auth.IUser;
import org.integratedmodelling.api.network.INetwork;
import org.integratedmodelling.exceptions.KlabException;
/**
* A session is the context for any observation, and needs to exist before observations can be made. Sessions
* are created by IEngine.createSession().
*
* @author Ferd
*
*/
public interface ISession extends Closeable {
/**
* @author Ferd A session listener reports crucial observation events.
*/
public static interface Listener {
/**
* Called at each observation event that creates or modifies a context.
*
* @param context
* @param isNew
*/
void contextEvent(IContext context, boolean isNew);
/**
* Called at each event that starts or modifies a task.
*
* @param task
* @param isNew
*/
void taskEvent(ITask task, boolean isNew);
}
/**
* Each session has a string ID.
*
* @return the session id.
*/
String getId();
/**
* Each session belongs to a user.
*
* @return the user the session belongs to.
*/
IUser getUser();
/**
* Observe a subject generator to create a context. Further observations must be made directly in the
* subjects resulting from this.
*
* The context is created by the task returned. If using directly and not using the notification bus, call
* task.finish() to wait until the context is computed and returned.
*
* Optional extents may be passed to force the final subject's scale to adapt to them. For example we may
* pass a geographical region which a passed forcing extent may turn into a grid of a given resolution, or
* we could pass a temporal context to adopt when it wasn't originally there.
*
* @param directObserver either a direct observer or a fully qualified name that can be resolved to one.
* @param options any optional modifiers for the context of observation. These can be: partially specified
* extents (to force interpretation of incomplete time/space to specified topologies);
* namespaces or strings (to be matched to scenarios to activate); or collections thereof.
*
* @return the observation task, already started, that will create the context and the subject in the
* engine.
*
* @throws KlabException
*/
ITask observe(Object observable, Object... options) throws KlabException;
/**
* Return the contexts observed so far. Note that sessions are free to remove contexts if storage gets too
* large.
*
* @return all the contexts observed in this session.
*/
List getContexts();
/**
* Add listeners to be notified of what happens.
*
* @param listener
*/
void addListener(Listener listener);
/**
* An exclusive session can change the engine's internal environment, i.e. load, update and delete
* knowledge.
*
* @return true if user has locking rights on the engine.
*/
boolean isExclusive();
/**
* If the user has exclusive privileges, she may request a lock to perform modifications on the engine's
* knowledge. The lock should be released as soon as possible.
*
* @return true if the request has succeeded.
*/
boolean requestLock();
/**
* Release a previously acquired lock.
*
* @return true if there was a lock to release.
*/
boolean releaseLock();
/**
* Sessions may be reopenable if asked.
*
* @return whether this session can be reopened by the same user after close.
*/
boolean isReopenable();
/**
* Each client session has its own view of the network, so when a session is active we should use this one
* and not the one in the Engine, whose view is that of the user owning the engine and not necessarily the
* one connected to the session.
*
* @return the view of the network for the session user. Never null.
*/
INetwork getNetwork();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy