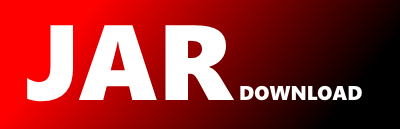
org.integratedmodelling.api.runtime.ITask Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright (C) 2007, 2014:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.api.runtime;
import org.integratedmodelling.api.modelling.resolution.IDataflow;
import org.integratedmodelling.api.modelling.resolution.IResolution;
/**
* A ITask is the process that makes an atomic observation in a context.
* A task is returned by IContext.observe() immediately; it will return
* a result when finished. If synchronous behavior is desired, finish() will
* return when the computation is finished, and return the context so that
* other observations can be called on it (context.observe().finish().observe()).
*
* A task may create its own context. This is because we may not know the observable
* the moment the task is created: all tasks should update the model space before
* resolving the observable. So the first task will have no context associated until
* finished.
*
* @author ferdinando.villa
*
*/
public interface ITask {
/**
* We use our own enum to allow discriminating between finished OK, finished
* in error, and user-interrupted. Thread.State just uses FINISHED for all.
*
* @author ferdinando.villa
*
*/
public static enum Status {
QUEUED,
RUNNING,
INITIALIZING,
FINISHED,
ERROR,
INTERRUPTED
}
/**
* Peek current status and return it.
* @return the status at the time of request.
*/
Status getStatus();
/**
* Task have an ID that's unique within a session. getId() would be a better
* name, but at the server side we want tasks to be threads, and those have
* a conflicting getId() that we don't want to mess with.
*
* @return the task ID.
*/
String getTaskId();
/**
* Task description. Should be short and readable as it's shown to
* non-technical users in UIs.
*
* @return a readable description of what the task is doing.
*/
String getDescription();
/**
* Interrupt task at the first chance. Task cannot be resumed
* after this. Interruption is not guaranteed, depending on
* engine implementation.
*
*/
void interrupt();
/**
* Return the context this task belongs to. Never null, although
* the context may be empty.
*
* @return the context this task is running in.
*/
IContext getContext();
/**
* Wait until end of task and return the context after the
* task has completed (null in case of failure or interruption).
*
* @return the context after termination of the task.
*/
IContext finish();
/**
* Return the start time. If the task is waiting, return when we started waiting.
*
* @return the start time of the task, at engine side.
*/
long getStartTime();
/**
* Return the end time. This is only not zero if the task status is finished.
*
* @return the time of termination, or 0 if the task is still running.
*/
long getEndTime();
/**
* @return the dataflow if any
*/
IDataflow getDataflow();
/**
*
* @return the resolution graph if any
*/
IResolution getResolution();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy