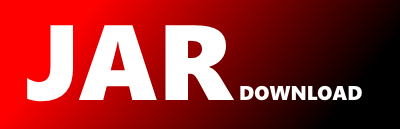
org.integratedmodelling.lang.LogicalConnector Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2014:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.lang;
import org.integratedmodelling.exceptions.KlabValidationException;
/**
* A logical connector, representing one of the possible four connectors (union (or), intersection (and),
* exclusion (not), disjunction (xor)).
* A bit complicated as a wrapper for an int, but it solves several problems related to string conversions and
* equality. Private constructors force use of parseLogicalConnector to obtain one of the four possible static
* instances. Because only the static members are used, == can be used to check for equality, although equals()
* can be also used with both integers, LogicalConnectors and Strings. toString() will work properly.
*
* @author Ferdinando Villa
*/
public class LogicalConnector {
static public final int _UNION = 0;
static public final int _INTERSECTION = 1;
static public final int _EXCLUSION = 2;
static public final int _DISJOINT_UNION = 3;
static public LogicalConnector UNION = new LogicalConnector(_UNION);
static public LogicalConnector INTERSECTION = new LogicalConnector(_INTERSECTION);
static public LogicalConnector EXCLUSION = new LogicalConnector(_EXCLUSION);
static public LogicalConnector DISJOINT_UNION = new LogicalConnector(_DISJOINT_UNION);
/**
* The value of a connector. Use this one in switch statements, or use equality
* with static connector members in if statements.
*/
public int value;
/**
* Checks if string is a valid representation of a logical connector.
* @param s a string
* @return true if string represents a connector.
*/
public static boolean isLogicalConnector(String s) {
// TODO
boolean ret = true;
try {
parseLogicalConnector(s);
} catch (KlabValidationException e) {
ret = false;
}
return ret;
}
/**
* Parses string into connector and returns result. Will never allocate new connectors, but only
* return the static instance corresponding to the string.
* @param s A string representing a logical connector.
* @return a LogicalConnector
* @throws KlabValidationException if string is silly.
*/
public static LogicalConnector parseLogicalConnector(String s) throws KlabValidationException {
LogicalConnector value = null;
s = s.trim().toLowerCase();
// TODO support formal notation quantifiers too
if (s.equals("and") || s.equals("intersection"))
value = INTERSECTION;
else if (s.equals("or") || s.equals("union"))
value = UNION;
else if (s.equals("not") || s.equals("exclusion"))
value = EXCLUSION;
else if (s.equals("xor") || s.equals("disjoint-union"))
value = DISJOINT_UNION;
else
throw new KlabValidationException(s + " is not a valid logical connector");
return value;
}
private LogicalConnector(int i) {
value = i;
}
/**
*
* @param c
* @return true if same
*/
public boolean equals(LogicalConnector c) {
return c.value == value;
}
public boolean equals(int c) {
return value == c;
}
public boolean equals(String s) throws KlabValidationException {
return value == parseLogicalConnector(s).value;
}
@Override
public String toString() {
String ret = "";
switch (value) {
case _UNION:
ret = "or";
break;
case _INTERSECTION:
ret = "and";
break;
case _EXCLUSION:
ret = "not";
break;
case _DISJOINT_UNION:
ret = "xor";
break;
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy