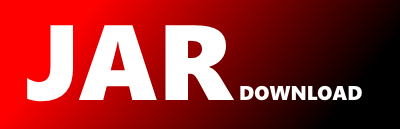
org.integratedmodelling.engine.KnowledgeManager Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collection;
import org.integratedmodelling.api.factories.IKnowledgeFactory;
import org.integratedmodelling.api.knowledge.IAuthority;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IKnowledge;
import org.integratedmodelling.api.knowledge.IKnowledgeIndex;
import org.integratedmodelling.api.knowledge.IOntology;
import org.integratedmodelling.api.knowledge.IProperty;
import org.integratedmodelling.api.modelling.INamespace;
import org.integratedmodelling.api.ui.IBookmarkManager;
import org.integratedmodelling.common.authority.AuthorityFactory;
import org.integratedmodelling.common.configuration.KLAB;
import org.integratedmodelling.common.interfaces.OWLManager;
import org.integratedmodelling.common.kim.KIMModelManager;
import org.integratedmodelling.common.owl.OWL;
import org.integratedmodelling.common.owl.Ontology;
import org.integratedmodelling.common.vocabulary.NS;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabIOException;
import org.integratedmodelling.exceptions.KlabResourceNotFoundException;
/**
* Main knowledge manager functionalities.
*
* TODO make it a single class in common; give it search, bookmark and documentation
* facilities.
*
* @author Ferd
*
*/
public class KnowledgeManager implements IKnowledgeFactory, OWLManager {
private OWL manager;
protected KnowledgeManager() {
}
public void initialize() throws KlabException {
try {
manager = new OWL(KLAB.CONFIG.getDataPath("knowledge"));
} catch (Exception e) {
throw new KlabIOException(e);
}
/*
* declare namespaces for each core ontology
*/
int nOnt = 0;
for (INamespace o : manager.getNamespaces()) {
((KIMModelManager) (KLAB.MMANAGER)).addNamespace(o);
nOnt++;
}
KLAB.info("loaded " + nOnt + " core ontologies");
/*
* register common XSD types
*/
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#Name", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#Literal", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#anyURI", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchemaPlainLiteral", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#decimal", requireConcept(NS.DOUBLE));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#boolean", requireConcept(NS.BOOLEAN));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#language", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#integer", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#int", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#negativeInteger", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#nonNegativeInteger", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#long", requireConcept(NS.LONG));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#float", requireConcept(NS.FLOAT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#real", requireConcept(NS.DOUBLE));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#double", requireConcept(NS.DOUBLE));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#string", requireConcept(NS.TEXT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#shape", requireConcept(NS.POLYGON));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#float", requireConcept(NS.FLOAT));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#short", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#unsignedShort", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#unsignedInt", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#unsignedByte", requireConcept(NS.INTEGER));
manager.registerDatatypeMapping("http://www.w3.org/2001/XMLSchema#unsignedLong", requireConcept(NS.LONG));
manager.registerDatatypeMapping("http://www.integratedmodelling.org/ks/geospace/geospace.owl#line", requireConcept(NS.LINE));
manager.registerDatatypeMapping("http://www.integratedmodelling.org/ks/geospace/geospace.owl#polygon", requireConcept(NS.POLYGON));
manager.registerDatatypeMapping("http://www.integratedmodelling.org/ks/geospace/geospace.owl#point", requireConcept(NS.POINT));
}
@Override
public IProperty getProperty(String prop) {
return manager.getProperty(prop);
}
@Override
public IConcept getConcept(String conc) {
return manager.getConcept(conc);
}
@Override
public IKnowledge getKnowledge(String k) {
IKnowledge ret = getConcept(k);
if (ret == null) {
ret = getProperty(k);
}
return ret;
}
public void shutdown() {
}
public IConcept requireConcept(String id) throws KlabException {
IConcept ret = getConcept(id);
if (ret == null) {
throw new KlabResourceNotFoundException("concept " + id + " is unknown");
}
return ret;
}
public IProperty requireProperty(String id) throws KlabException {
IProperty ret = getProperty(id);
if (ret == null) {
throw new KlabResourceNotFoundException("property " + id + " is unknown");
}
return ret;
}
@Override
public IOntology refreshOntology(URL url, String name) throws KlabException {
return manager.refreshOntology(url, name);
}
@Override
public boolean releaseOntology(String s) {
if (manager.getOntology(s) == null) {
return false;
}
manager.releaseOntology(getOntology(s));
return true;
}
@Override
public void releaseAllOntologies() {
manager.clear();
}
@Override
public IOntology getOntology(String ontName) {
return manager.getOntology(ontName);
}
@Override
public Collection getOntologies(boolean includeInternal) {
return manager.getOntologies(includeInternal);
}
@Override
public IOntology createOntology(String id, String ontologyPrefix) throws KlabException {
return manager.requireOntology(id, ontologyPrefix);
}
@Override
public Collection getRootConcepts() {
ArrayList ret = new ArrayList();
for (IOntology onto : getOntologies(true)) {
for (IConcept c : onto.getConcepts()) {
Collection pp = c.getParents();
if (pp.size() == 0 || (pp.size() == 1 && pp.iterator().next().is(getRootConcept())))
ret.add(c);
}
}
return ret;
}
@Override
public Collection getConcepts() {
ArrayList ret = new ArrayList();
for (IOntology onto : getOntologies(true)) {
for (IConcept c : onto.getConcepts()) {
ret.add(c);
}
}
return ret;
}
@Override
public IConcept getRootConcept() {
return manager.getRootConcept();
}
@Override
public IConcept getNothing() {
return manager.getNothing();
}
@Override
public File exportOntology(String ontologyId) throws KlabException {
IOntology ontology = getOntology(ontologyId);
if (ontology == null)
return null;
if (((Ontology) ontology).getResourceUrl() != null) {
try {
URL url = new URL(((Ontology) ontology).getResourceUrl());
if (url.getProtocol().startsWith("file")) {
return new File(url.getFile());
}
} catch (MalformedURLException e) {
// just move on
}
}
File ret;
try {
ret = File.createTempFile("ont", "owl");
} catch (IOException e) {
throw new KlabIOException(e);
}
if (!ontology.write(ret))
return null;
return ret;
}
// public void extractCoreOntologies(File tf) throws ThinklabIOException {
// manager.extractCoreOntologies(tf);
// }
@Override
public OWL getOWLManager() {
return manager;
}
@Override
public INamespace getCoreNamespace(String ns) {
return manager.getNamespace(ns);
}
public IOntology requireOntology(String id, String ontologyNamespacePrefix) {
return manager.requireOntology(id, ontologyNamespacePrefix);
}
@Override
public IAuthority getAuthority(String id) {
return AuthorityFactory.get().getAuthority(id);
}
@Override
public IOntology requireOntology(String id) {
return requireOntology(id, "http://integratedmodelling.org/ks");
}
@Override
public IKnowledgeIndex getIndex() {
// TODO Auto-generated method stub
return null;
}
@Override
public IBookmarkManager getBookmarkManager() {
// TODO Auto-generated method stub
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy