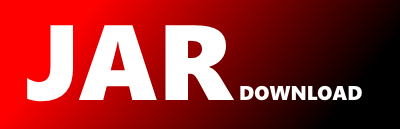
org.integratedmodelling.engine.cache.CacheUtils Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
//package org.integratedmodelling.engine.cache;
//
//import java.awt.image.BufferedImage;
//import java.io.ByteArrayInputStream;
//import java.io.ByteArrayOutputStream;
//import java.io.IOException;
//import java.io.InputStream;
//import java.util.Map;
//
//import javax.imageio.ImageIO;
//
//import org.apache.jcs.JCS;
//import org.apache.jcs.access.exception.CacheException;
//import org.integratedmodelling.api.auth.IUser;
//import org.integratedmodelling.auth.data.Datarecord;
//import org.integratedmodelling.auth.rest.RESTUser;
//import org.integratedmodelling.common.configuration.Env;
//import org.integratedmodelling.engine.Thinklab;
//import org.integratedmodelling.exceptions.ThinklabException;
//import org.integratedmodelling.exceptions.ThinklabIOException;
//
//public class CacheUtils {
//
// static JCS _dataCache;
// static JCS _imageCache;
// static JCS _wcsCache;
// static JCS _wfsCache;
//
// static JCS getDataCache() throws ThinklabException {
//
// if (_dataCache == null) {
// try {
// _dataCache = JCS.getInstance("data");
// } catch (CacheException e) {
// throw new ThinklabIOException(e);
// }
// }
// return _dataCache;
// }
//
// /**
// * Retrieves a BufferedImage from the passed cache; return null if not there.
// * @throws ThinklabIOException
// *
// * @throws ImageNotFoundException
// * @throws CacheException
// * @throws IOException
// */
// public static BufferedImage getImage(String key, JCS imageCache) throws ThinklabIOException {
// BufferedImage img = null;
// byte[] imageAsBytes = (byte[]) imageCache.get(key);
// if (imageAsBytes != null) {
// // Convert the byte array back to the bufferedimage
// InputStream in = new ByteArrayInputStream(imageAsBytes);
// try {
// img = javax.imageio.ImageIO.read(in);
// } catch (IOException e) {
// throw new ThinklabIOException(e);
// }
// }
// return img;
// }
//
// /**
// * Save passed image to cache with given key. If it's already there, just return it.
// * @throws ThinklabIOException
// *
// * @throws ImageNotFoundException
// * @throws CacheException
// * @throws IOException
// */
// public static BufferedImage loadImage(String key, BufferedImage img, JCS imageCache)
// throws ThinklabIOException {
// try {
// if (img != null) {
// // Convert the image to bytes, BufferedImages cannot be put into the cache
// ByteArrayOutputStream baos = new ByteArrayOutputStream();
// ImageIO.write(img, "png", baos);
// byte[] bytesOut = baos.toByteArray();
// imageCache.put(key, bytesOut);
// }
// } catch (Exception e) {
// throw new ThinklabIOException(e);
// }
// return img;
// }
//
// /**
// * If data record is in cache, return it; otherwise ask it to primary server and store it.
// *
// * @param urn
// * @return
// */
// public static Datarecord getDatarecord(String urn, IUser user) {
//
// JCS dataCache = null;
// try {
// dataCache = getDataCache();
// } catch (ThinklabException e) {
// // continue without cache
// }
//
// Datarecord ret = null;
// if (dataCache != null) {
//
// Map, ?> map = (Map, ?>) dataCache.get(urn);
// if (map != null) {
// ret = new Datarecord(map);
// } else if (user != null && user.getServerURL() != null) {
//
// ret = ((RESTUser) user).getAuthClient().getDatasource(urn);
//
// } else if (!Env.NETWORK.isPersonal()) {
//
// /*
// * public engine can get the asset if authorized
// */
// // ret = RESTHelper.getAsset(urn, Env.NETWORK, Datarecord.class);
// }
//
// if (map == null && ret != null) {
// try {
// dataCache.put(urn, ret.adapt());
// } catch (CacheException e) {
// Thinklab.logger().warn("unexpected error saving " + urn + " to data cache");
// }
// }
// }
//
// return ret;
// }
// }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy