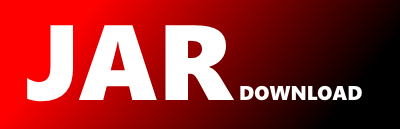
org.integratedmodelling.engine.geospace.functions.SPACE Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa - integratedmodelling.org - any
* other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite, meant to enable
* modular, collaborative, integrated development of interoperable data and model
* components. For details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or modify it under the terms
* of the Affero General Public License Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful, but without any
* warranty; without even the implied warranty of merchantability or fitness for a
* particular purpose. See the Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 59 Temple Place - Suite
* 330, Boston, MA 02111-1307, USA. The license is also available at:
* https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.geospace.functions;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Map;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IExpression;
import org.integratedmodelling.api.modelling.IFunctionCall;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.api.services.annotations.Prototype;
import org.integratedmodelling.common.kim.expr.CodeExpression;
import org.integratedmodelling.common.vocabulary.NS;
import org.integratedmodelling.engine.geospace.coverage.CoverageFactory;
import org.integratedmodelling.engine.geospace.coverage.vector.VectorCoverage;
import org.integratedmodelling.engine.geospace.extents.Grid;
import org.integratedmodelling.engine.geospace.extents.SpaceExtent;
import org.integratedmodelling.engine.geospace.literals.ShapeValue;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabValidationException;
@Prototype(id = "space", args = {
"# shape",
Prototype.TEXT,
"# projection",
Prototype.TEXT,
"# features",
Prototype.TEXT,
"# grid",
Prototype.TEXT,
"# vector-file",
Prototype.TEXT,
"# service",
Prototype.TEXT,
"# shape-id",
Prototype.TEXT }, returnTypes = { NS.SPACE_DOMAIN })
public class SPACE extends CodeExpression implements IExpression {
@Override
public Object eval(Map parameters, IMonitor monitor, IConcept... context)
throws KlabException {
ShapeValue shape = null;
String crs = "EPSG:4326";
double resolution = -1.0;
VectorCoverage vectors = null;
boolean forceGrid = false;
if (parameters.containsKey("projection")) {
crs = parameters.get("projection").toString();
}
/*
* shape is explicit (shape) or relative to a corner or center
*/
if (parameters.containsKey("shape")) {
if (parameters.get("shape") instanceof ShapeValue) {
shape = (ShapeValue) parameters.get("shape");
} else {
shape = new ShapeValue(parameters.get("shape").toString());
}
// TODO: Why do we transform shape here? It is transformed also in Grid.java
// line
// shape = shape.transform(Geospace.get().getDefaultCRS());
}
if (parameters.containsKey("grid")) {
if (parameters.get("grid") == null
|| parameters.get("grid").equals(IFunctionCall.NULL_PARAMETER)) {
forceGrid = true;
} else if (parameters.get("grid") instanceof Integer) {
resolution = ((Integer) (parameters.get("grid"))).doubleValue();
} else {
resolution = Grid.parseResolution(parameters.get("grid").toString());
}
}
if (parameters.containsKey("features")) {
URL url;
try {
url = new URL(parameters.get("features").toString());
} catch (MalformedURLException e) {
throw new KlabValidationException(e);
}
vectors = (VectorCoverage) CoverageFactory.readVector(url, null, null, null, null);
}
Grid grid = null;
if (shape != null && resolution > 0.0) {
grid = new Grid(shape, resolution);
}
SpaceExtent ret = null;
/*
* TODO handle forceGrid
*/
if (grid != null) {
ret = new SpaceExtent(grid);
} else if (vectors != null) {
ret = new SpaceExtent(vectors);
if (shape != null) {
ret.set(shape, true);
}
} else if (shape != null) {
ret = new SpaceExtent(shape);
} else if (resolution > 0.0) {
ret = new SpaceExtent(resolution);
} else {
ret = new SpaceExtent();
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy