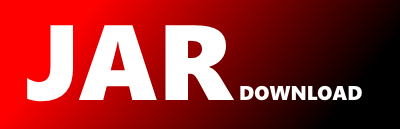
org.integratedmodelling.engine.geospace.functions.WCS Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.geospace.functions;
import java.io.File;
import java.util.Map;
import org.integratedmodelling.api.data.ITable;
import org.integratedmodelling.api.data.ITableSet;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IExpression;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.api.services.annotations.Prototype;
import org.integratedmodelling.common.data.TableFactory;
import org.integratedmodelling.common.kim.expr.CodeExpression;
import org.integratedmodelling.common.utils.URLUtils;
import org.integratedmodelling.common.vocabulary.NS;
import org.integratedmodelling.engine.geospace.datasources.WCSGridDataSource;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabIOException;
import org.integratedmodelling.exceptions.KlabValidationException;
@Prototype(
id = "wcs",
args = {
"# service",
Prototype.TEXT,
"# id",
Prototype.TEXT,
"# no-data",
Prototype.FLOAT,
"# lookup-table",
Prototype.TEXT,
"# table-sheet",
Prototype.TEXT,
"# match-column", // if missing, use value as linear index
Prototype.TEXT,
"# output-column",
Prototype.TEXT,
"# urn",
Prototype.TEXT },
returnTypes = { NS.DATASOURCE })
public class WCS extends CodeExpression implements IExpression {
@Override
public Object eval(Map parameters, IMonitor monitor, IConcept... context)
throws KlabException {
String id = null;
String service = null;
File table = null;
String sheet = null;
String inputcol = null;
String outputcol = null;
Map, ?> mapping = null;
boolean available = true;
if (parameters.containsKey("urn")) {
/*
* leave the service blank, this will signal to the datasource that we're using a URN that
* we'll resolve when we read the data.
*/
id = parameters.get("urn").toString();
// Datarecord dr = DataAssetResolver.getDatarecord(parameters.get("urn").toString(), monitor);
// if (dr != null) {
//
// service = dr.getAttribute(IDataAsset.KEY_URL);
//
// id = dr.getAttribute(IDataAsset.KEY_PUBLISHED_DATAID);
// /*
// * patch to avoid having to republish every individual file, or Luke having
// * to do work.
// */ if (id == null) {
// id = dr.getAttribute(IDataAsset.KEY_DATAID);
// }
//
// if (dr.getAttribute(IDataAsset.KEY_NAMESPACE) != null
// && !dr.getAttribute(IDataAsset.KEY_NAMESPACE).isEmpty()) {
// id = dr.getAttribute(IDataAsset.KEY_NAMESPACE) + ":" + id;
// }
// }
} else {
Object serv = parameters.get("service");
if (serv instanceof String) {
service = serv.toString();
} else if (serv instanceof String[]) {
String[] ss = (String[]) serv;
for (String s : ss) {
if (URLUtils.ping(s)) {
service = s;
break;
}
}
}
// ensure we don't proceed if service is null
id = service == null ? null : parameters.get("id").toString();
}
if (id == null)
return null;
double noData = Double.NaN;
/*
* TODO support a list of nodata values
*/
if (parameters.containsKey("no-data")) {
noData = Double.parseDouble(parameters.get("no-data").toString());
}
if (parameters.containsKey("lookup-table")) {
table = new File(getProject().getLoadPath() + File.separator
+ parameters.get("lookup-table").toString());
if (!parameters.containsKey("table-sheet") || !parameters.containsKey("match-column")
|| !parameters.containsKey("output-column")) {
throw new KlabValidationException("wcs: must specify 'table-sheet', 'match-column' and 'output-column' parameters along with 'lookup-table'");
}
sheet = parameters.get("table-sheet").toString();
inputcol = parameters.get("match-column").toString();
outputcol = parameters.get("output-column").toString();
/*
* retrieve/cache the MAPPING and feed it to the data source. When that's done,
* we can just let the GC dispose of the tableset.
*/
ITableSet tableset = TableFactory.open(table);
if (tableset == null) {
throw new KlabIOException("lookup table: file " + table
+ " is not a recognized table format");
}
ITable _table = tableset.getTable(sheet);
if (_table == null) {
throw new KlabIOException("lookup table: table " + sheet
+ " not found in imported file");
}
mapping = _table.getMapping(inputcol, outputcol);
if (mapping == null) {
throw new KlabIOException("lookup table: mapping " + inputcol + " -> " + outputcol
+ " cannot be established: check column names");
}
}
/*
* service == null says that id is a URN for deferred resolution
*/
WCSGridDataSource ret = new WCSGridDataSource(service, id, new double[] { noData }, available);
if (mapping != null) {
ret.setValueMapping(mapping);
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy