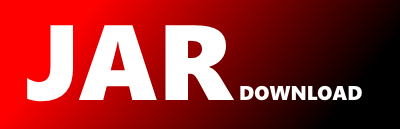
org.integratedmodelling.engine.geospace.gis.SextanteOperations Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.geospace.gis;
import java.awt.geom.Point2D;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.modelling.IActiveDirectObservation;
import org.integratedmodelling.api.modelling.IActiveSubject;
import org.integratedmodelling.api.modelling.IObservable;
import org.integratedmodelling.api.modelling.IScale;
import org.integratedmodelling.api.modelling.IState;
import org.integratedmodelling.api.modelling.ISubject;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.api.space.IGrid;
import org.integratedmodelling.api.space.ISpatialExtent;
import org.integratedmodelling.common.space.IGeometricShape;
import org.integratedmodelling.common.states.States;
import org.integratedmodelling.engine.geospace.coverage.raster.RasterCoverage;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabIOException;
import com.vividsolutions.jts.geom.Point;
import es.unex.sextante.core.ITaskMonitor;
import es.unex.sextante.core.OutputFactory;
import es.unex.sextante.core.OutputObjectsSet;
import es.unex.sextante.core.ParametersSet;
import es.unex.sextante.dataObjects.IRasterLayer;
import es.unex.sextante.dataObjects.IVectorLayer;
import es.unex.sextante.geotools.GTOutputFactory;
import es.unex.sextante.geotools.GTRasterLayer;
import es.unex.sextante.lighting.viewshed.ApproximatedViewshedAlgorithm;
import es.unex.sextante.lighting.visibility.VisibilityAlgorithm;
import es.unex.sextante.morphometry.aspect.AspectAlgorithm;
import es.unex.sextante.outputs.Output;
public abstract class SextanteOperations {
public static IState getRasterViewshed(IState elevation, IActiveDirectObservation context, ISubject viewpoint, IObservable observable, IMonitor monitor)
throws KlabException {
IState ret = null;
VisibilityAlgorithm alg = new VisibilityAlgorithm();
ParametersSet parms = alg.getParameters();
IRasterLayer dem = getInputAsRaster(elevation);
Point zio = ((IGeometricShape) (viewpoint.getScale().getSpace().getShape())).getGeometry()
.getCentroid();
// Env.logger.info("VS " + zio);
// what a lovely API.
Point2D point = new Point2D.Double(zio.getCoordinate().x, zio.getCoordinate().y);
try {
parms.getParameter(VisibilityAlgorithm.DEM).setParameterValue(dem);
parms.getParameter(VisibilityAlgorithm.POINT).setParameterValue(point);
parms.getParameter(VisibilityAlgorithm.METHOD).setParameterValue("Visibility");
parms.getParameter(VisibilityAlgorithm.RADIUS).setParameterValue(0.0);
parms.getParameter(VisibilityAlgorithm.HEIGHTOBS)
.setParameterValue(1.00/* average standing person - makes a big difference. */);
parms.getParameter(VisibilityAlgorithm.HEIGHT).setParameterValue(10 /* boh */);
OutputFactory outputFactory = new GTOutputFactory();
if (alg.execute(getTaskMonitor(monitor), outputFactory)) {
OutputObjectsSet outputs = alg.getOutputObjects();
Output computed = outputs.getOutput(VisibilityAlgorithm.RESULT);
IRasterLayer layer = (IRasterLayer) computed.getOutputObject();
if (elevation.isTemporallyDistributed()) {
ret = getStateFromRaster(observable, context, layer);
} else {
ret = getStaticStateFromRaster(observable, context, layer);
}
}
} catch (Exception e) {
monitor.error(e);
}
return ret;
}
/* this just does not work. */
public static IVectorLayer getViewshed(IState elevation, ISubject viewpoint, IMonitor monitor)
throws KlabException {
IVectorLayer ret = null;
ApproximatedViewshedAlgorithm alg = new ApproximatedViewshedAlgorithm();
ParametersSet parms = alg.getParameters();
IRasterLayer dem = getInputAsRaster(elevation);
Point zio = ((IGeometricShape) (viewpoint.getScale().getSpace().getShape())).getGeometry()
.getCentroid();
// what a lovely API.
Point2D point = new Point2D.Double(zio.getCoordinate().x, zio.getCoordinate().y);
try {
parms.getParameter(ApproximatedViewshedAlgorithm.DEM).setParameterValue(dem);
parms.getParameter(ApproximatedViewshedAlgorithm.POINT).setParameterValue(point);
parms.getParameter(ApproximatedViewshedAlgorithm.HEIGHTOBS).setParameterValue(0);
parms.getParameter(ApproximatedViewshedAlgorithm.HEIGHT).setParameterValue(0);
OutputFactory outputFactory = new GTOutputFactory();
if (alg.execute(getTaskMonitor(monitor), outputFactory)) {
OutputObjectsSet outputs = alg.getOutputObjects();
Output computed = outputs.getOutput(ApproximatedViewshedAlgorithm.RESULT);
ret = (IVectorLayer) computed.getOutputObject();
}
} catch (Exception e) {
monitor.error(e);
}
return ret;
}
public static IState getAspect(IState elevation, IObservable output, IActiveSubject context, int method, IMonitor monitor)
throws KlabException {
AspectAlgorithm alg = new AspectAlgorithm();
ParametersSet parms = alg.getParameters();
/*
* units based on the output observer
*/
int unit = AspectAlgorithm.UNITS_DEGREES;
IRasterLayer dem = getInputAsRaster(elevation);
try {
parms.getParameter(AspectAlgorithm.UNITS).setParameterValue(new Integer(unit));
parms.getParameter(AspectAlgorithm.METHOD).setParameterValue(method);
parms.getParameter(AspectAlgorithm.DEM).setParameterValue(dem);
OutputFactory outputFactory = new GTOutputFactory();
alg.execute(getTaskMonitor(monitor), outputFactory);
OutputObjectsSet outputs = alg.getOutputObjects();
Output computed = outputs.getOutput(AspectAlgorithm.ASPECT);
IState aspect = null;
/*
* result is only dynamic if elevation is.
*/
if (elevation.isTemporallyDistributed()) {
aspect = getStateFromRaster(output, context, (IRasterLayer) computed.getOutputObject());
} else {
aspect = getStaticStateFromRaster(output, context, (IRasterLayer) computed.getOutputObject());
}
return aspect;
} catch (Exception e) {
monitor.error(e);
}
return null;
}
public static ITaskMonitor getTaskMonitor(IMonitor monitor) {
return new GISOperations.TaskMonitor(monitor);
}
/**
* Build a coverage from the numeric transposition of the
* passed state.
* @param state
* @return raster layer
* @throws KlabException
*/
public static IRasterLayer getInputAsRaster(IState state) throws KlabException {
if (state == null)
return null;
GTRasterLayer ret = null;
RasterCoverage coverage = new RasterCoverage(state);
ret = new GTRasterLayer();
ret.create(coverage.getCoverage());
return ret;
}
public static IRasterLayer getRaster(IState state, IMonitor monitor) {
GTRasterLayer ret = null;
try {
RasterCoverage coverage = new RasterCoverage(state);
ret = new GTRasterLayer();
ret.create(coverage.getCoverage());
} catch (KlabException e) {
monitor.error("error building a raster representation of state "
+ state.getObservable().getType());
return null;
}
return ret;
}
/*
* build a feature collection from all the subjects of the passed
* type.
*/
protected IVectorLayer getInputAsVector(IConcept observable) {
/*
* TODO
*/
return null;
}
/**
* Create or get a state from a subject and put the passed raster in the states corresponding to the passed
* transition.
*
* @param observable
* @param subject
* @param layer
* @param locators
* @return state
* @throws KlabException
*/
public static IState getStateFromRaster(IObservable observable, IActiveDirectObservation subject, IRasterLayer layer, IScale.Locator... locators)
throws KlabException {
GTRasterLayer l = (GTRasterLayer) layer;
ISpatialExtent ext = subject.getScale().getSpace();
if (ext == null || ext.getGrid() == null) {
throw new KlabIOException("cannot return a raster state from a non-grid spatial extent");
}
IState ret = subject.getState(observable);
IGrid grid = ext.getGrid();
/*
* FIXME this is a bit of a pain and should be clarified at the API level in IScale.
*/
Iterable index = null;
if (locators == null || locators.length == 0) {
index = subject.getScale().getIndex(IScale.Locator.INITIALIZATION);
} else {
index = subject.getScale().getIndex(locators);
}
for (int n : index) {
int spaceOffset = subject.getScale().getExtentOffset(ext, n);
int[] xy = grid.getXYOffsets(spaceOffset);
States.set(ret, l.getCellValueAsDouble(xy[0], xy[1]), n);
}
return ret;
}
/**
* Create or get a state from a subject and put the passed raster in the states corresponding to the passed
* transition.
*
* @param observable
* @param subject
* @param layer
* @param locators
* @return state
* @throws KlabException
*/
public static IState getStaticStateFromRaster(IObservable observable, IActiveDirectObservation subject, IRasterLayer layer, IScale.Locator... locators)
throws KlabException {
GTRasterLayer l = (GTRasterLayer) layer;
ISpatialExtent ext = subject.getScale().getSpace();
if (ext == null || ext.getGrid() == null) {
throw new KlabIOException("cannot return a raster state from a non-grid spatial extent");
}
IState ret = subject.getStaticState(observable);
IGrid grid = ext.getGrid();
/*
* FIXME this is a bit of a pain and should be clarified at the API level in IScale.
*/
Iterable index = null;
if (locators == null || locators.length == 0) {
index = subject.getScale().getIndex(IScale.Locator.INITIALIZATION);
} else {
index = subject.getScale().getIndex(locators);
}
for (int n : index) {
int spaceOffset = subject.getScale().getExtentOffset(ext, n);
int[] xy = grid.getXYOffsets(spaceOffset);
States.set(ret, l.getCellValueAsDouble(xy[0], xy[1]), n);
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy