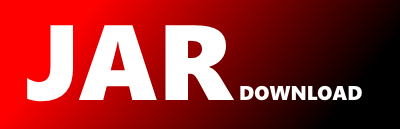
org.integratedmodelling.engine.modelling.StateFactory Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa - integratedmodelling.org - any
* other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite, meant to enable
* modular, collaborative, integrated development of interoperable data and model
* components. For details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or modify it under the terms
* of the Affero General Public License Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful, but without any
* warranty; without even the implied warranty of merchantability or fitness for a
* particular purpose. See the Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 59 Temple Place - Suite
* 330, Boston, MA 02111-1307, USA. The license is also available at:
* https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.modelling;
import java.util.HashMap;
import org.integratedmodelling.api.modelling.IExtent;
import org.integratedmodelling.api.modelling.IScale;
import org.integratedmodelling.api.modelling.IState;
import org.integratedmodelling.common.states.State;
import org.integratedmodelling.engine.modelling.runtime.Scale;
/**
* For now, this will only work on IObjectState, because those are the states which can
* store values for objects. No other states would reasonably need to be generated by a
* factory.
*
* NOTE: new states are created with a null observer because it should not be necessary.
* see comment on IState.getObserver()
*
* @author luke
*
*/
public class StateFactory {
private static final StateFactory instance = new StateFactory();
private abstract class StateGenerator {
abstract State generateFrom(IState original, IScale newScale);
}
/*
* FIXME this should have become unnecessary now that all the polimorphism is
* encapsulated within State and there is only one State class in common. Check with
* Luke when everything is stable.
*/
private final HashMap, StateGenerator> stateGenerators = new HashMap, StateGenerator>() {
// default
// serial
// ID
private static final long serialVersionUID = 1L;
{
put(State.class, new StateGenerator() {
@Override
// FIXME
// see
// what
// to
// do
// with
// dynamic
// state
State generateFrom(IState original, IScale newScale) {
State result = new State(original
.getObservable(), newScale, true, /*
* FIXME not sure this is
* used; for now no
* probabilistic outcome is
* possible
*/ false, ((State) original)
.getContextObservation());
return result;
}
});
// put(ConstObjectState.class,
// new
// StateGenerator()
// {
// @Override
// ConstObjectState
// generateFrom(IState
// original,
// IScale
// newScale)
// {
// ConstObjectState
// result =
// new
// ConstObjectState(original
// .getObservable(),
// newScale,
// null,
// null);
// return
// result;
// }
// });
}
};
public static IState getEmptyClone(IState original, IScale targetScale) {
StateGenerator generator = instance.stateGenerators.get(original.getClass());
// TODO remove this when we can be more flexible.
// it's too early to use the dynamic-state-creation; we're currently locked into
// returning ObjectState
// objects
generator = instance.stateGenerators.get(State.class);
IScale newScale = new Scale(new IExtent[] { targetScale.getSpace() });
IState result = generator.generateFrom(original, newScale);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy