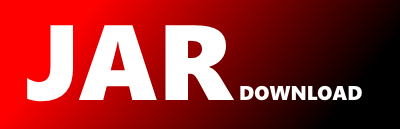
org.integratedmodelling.engine.modelling.resolver.ResolutionStrategy Maven / Gradle / Ivy
///*******************************************************************************
// * Copyright (C) 2007, 2015:
// *
// * - Ferdinando Villa - integratedmodelling.org - any
// * other authors listed in @author annotations
// *
// * All rights reserved. This file is part of the k.LAB software suite, meant to enable
// * modular, collaborative, integrated development of interoperable data and model
// * components. For details, see http://integratedmodelling.org.
// *
// * This program is free software; you can redistribute it and/or modify it under the terms
// * of the Affero General Public License Version 3 or any later version.
// *
// * This program is distributed in the hope that it will be useful, but without any
// * warranty; without even the implied warranty of merchantability or fitness for a
// * particular purpose. See the Affero General Public License for more details.
// *
// * You should have received a copy of the Affero General Public License along with this
// * program; if not, write to the Free Software Foundation, Inc., 59 Temple Place - Suite
// * 330, Boston, MA 02111-1307, USA. The license is also available at:
// * https://www.gnu.org/licenses/agpl.html
// *******************************************************************************/
//package org.integratedmodelling.engine.modelling.resolver;
//
//import java.util.ArrayList;
//import java.util.Collections;
//import java.util.Iterator;
//import java.util.List;
//
//import org.integratedmodelling.api.knowledge.IConcept;
//import org.integratedmodelling.api.modelling.IActiveDirectObservation;
//import org.integratedmodelling.api.modelling.IActiveProcess;
//import org.integratedmodelling.api.modelling.IActiveSubject;
//import org.integratedmodelling.api.modelling.ICoverage;
//import org.integratedmodelling.api.modelling.IDirectObservation;
//import org.integratedmodelling.api.modelling.IModel;
//import org.integratedmodelling.api.modelling.IObservable;
//import org.integratedmodelling.api.modelling.IRelationship;
//import org.integratedmodelling.api.modelling.IScale;
//import org.integratedmodelling.api.modelling.ISubject;
//import org.integratedmodelling.api.modelling.resolution.IDataflow;
//import org.integratedmodelling.api.modelling.resolution.IResolution;
//import org.integratedmodelling.api.modelling.resolution.IResolutionScope;
//import org.integratedmodelling.api.modelling.resolution.IResolutionStrategy;
//import org.integratedmodelling.api.modelling.scheduling.ITransition;
//import org.integratedmodelling.api.monitoring.IMonitor;
//import org.integratedmodelling.api.monitoring.Messages;
//import org.integratedmodelling.api.provenance.IProvenance;
//import org.integratedmodelling.common.configuration.KLAB;
//import org.integratedmodelling.common.interfaces.actuators.IDirectActuator;
//import org.integratedmodelling.common.interfaces.actuators.IEventActuator;
//import org.integratedmodelling.common.interfaces.actuators.IProcessActuator;
//import org.integratedmodelling.common.interfaces.actuators.ISubjectActuator;
//import org.integratedmodelling.common.kim.KIMModel;
//import org.integratedmodelling.common.vocabulary.NS;
//import org.integratedmodelling.common.vocabulary.Observable;
//import org.integratedmodelling.common.vocabulary.ObservationMetadata;
//import org.integratedmodelling.engine.modelling.kbox.ObservationKbox;
//import org.integratedmodelling.engine.modelling.runtime.Context;
//import org.integratedmodelling.engine.modelling.runtime.DirectObservation;
//import org.integratedmodelling.engine.modelling.runtime.Process;
//import org.integratedmodelling.engine.modelling.runtime.Subject;
//import org.integratedmodelling.exceptions.KlabException;
//
//public class ResolutionStrategy implements IResolutionStrategy {
//
// List steps = new ArrayList();
// ICoverage coverage;
// DirectObservation subject;
// ISubject contextSubject;
// List scenarios;
// IScale scale;
// IProvenance.Action cause;
//
// public static class ResolutionStep implements Step {
//
// IResolution provenance;
// IDataflow workflow;
// IModel model;
// IDirectActuator> accessor;
//
// // if this isn't null, this step will be a database search for subjects of
// // this type in the scale/scenario combination of interest.
// IConcept subjectType;
// /*
// * if this isn't null, it's a trait that must be added to the main observable of
// * any direct observation created in this step. Only applicable for model and
// * subject steps.
// */
// IConcept interpretAs;
//
// public ResolutionStep(IResolution provenance, IDataflow workflow) {
// this.provenance = provenance;
// this.workflow = workflow;
// }
//
// public ResolutionStep(IModel model, IConcept interpretAs) {
// this.model = model;
// this.interpretAs = interpretAs;
// }
//
// public ResolutionStep(IConcept subjectType, IConcept interpretAs) {
// this.subjectType = subjectType;
// this.interpretAs = interpretAs;
// }
//
// @Override
// public IModel getModel() {
// return model;
// }
//
// @Override
// public IResolution getResolutionGraph() {
// return provenance;
// }
//
// @Override
// public IDataflow getWorkflow() {
// return workflow;
// }
//
// }
//
// public ResolutionStrategy(IDirectObservation subject) {
// this.subject = (DirectObservation) subject;
// }
//
// @Override
// public int getStepCount() {
// return this.steps.size();
// }
//
// @Override
// public ICoverage getCoverage() {
// return this.coverage;
// }
//
// public void addStep(IResolution provenance, IDataflow workflow) {
// this.steps.add(new ResolutionStep(provenance, workflow));
// }
//
// public void addStep(IModel model, IConcept interpretAs) {
// this.steps.add(new ResolutionStep(model, interpretAs));
// }
//
// public void addStep(IConcept subjectType, IConcept interpretAs) {
// this.steps.add(new ResolutionStep(subjectType, interpretAs));
// }
//
// @Override
// public Iterator iterator() {
// return this.steps.iterator();
// }
//
// @Override
// public Step getStep(int i) {
// return this.steps.get(i);
// }
//
// public void setCause(IProvenance.Action cause) {
// this.cause = cause;
// }
//
// @Override
// public boolean execute(ITransition transition, IResolutionScope context) throws KlabException {
//
// IMonitor smonitor = ((Subject) this.subject).getMonitor();
//
// /*
// * TODO use provenance (metadata should be OK now, need to add processes).
// */
//
// for (Step rs : this.steps) {
//
// ResolutionStep s = (ResolutionStep) rs;
//
// /*
// * subject dependencies are only handled at initialization; afterwards it's
// * the processes and subject's tasks to create them.
// */
// if (s.subjectType != null && transition == null) {
//
// int objs = 0;
// for (ObservationMetadata sg : ObservationKbox.get()
// .query(Collections.singleton(s.subjectType), this.scale, this.scenarios, false)) {
//
// ISubject subj = (ISubject) KLAB.MFACTORY
// .createSubject(sg.getSubjectObserver(((ResolutionScope) context)
// .getMonitor()), this.subject, smonitor);
// ((DirectObservation) subj).setContextSubject(this.subject);
// if (((Subject) subj)
// .initialize(this.subject.getResolutionScope(), /* FIXME! */ null, smonitor)
// .isEmpty()) {
// ((Subject) this.subject).getMonitor().warn("cannot resolve dependent subject "
// + subj.getName());
// } else {
// ((Subject) subj).setObservable(((Observable) subj.getObservable())
// .withInterpretingTrait(s.interpretAs));
// // FIXME! need a relationship - must be found or specified. Then
// // both the
// // subject and the relationship must be resolved.
// ((ISubject) this.subject).getStructure()
// .link((ISubject) this.subject, subj, (IRelationship) null);
// }
// objs++;
// }
//
// /*
// * TODO record collection and initialization w/their provenance
// */
//
// KLAB.info(objs + " objects of type " + s.subjectType + " retrieved from network search");
//
// }
//
// // if there is a workflow, run it for the current transition.
// if (s.getWorkflow() != null) {
// if (!s.getWorkflow().run(transition)) {
// return false;
// }
// }
//
// if (s.getModel() != null) {
//
// // if there is an object source and we're initializing, create and
// // initialize objects
// if (transition == null && ((KIMModel) s.model).hasObjectSource()) {
//
//// int n = 0;
//// for (IActiveSubject subj : SubjectFactory
//// .createSubjects(s.model, s.model
//// .getObjectSource(((Subject) this.subject)
//// .getMonitor()), this.scale, (ISubject) this.subject, smonitor)) {
////
//// // add it to establish relationship, then resolve it
//// // FIXME! need a relationship - must be found or specified. Then
//// // both the
//// // subject and the relationship must be resolved.
//// ((ISubject) this.subject).getStructure()
//// .link((ISubject) this.subject, subj, (IRelationship) null);
////
//// ((Context) this.subject.getContext()).addDelta(subj);
////
//// Resolver resolver = new Resolver(this.subject
//// .getResolutionScope(), /* FIXME */ null);
//// if (resolver.resolve(subj, smonitor).isEmpty()) {
//// smonitor.warn("cannot resolve dependent subject " + subj.getName());
//// } else {
////
//// ((Subject) subj).setObservable(((Observable) subj.getObservable())
//// .withInterpretingTrait(s.interpretAs));
//// /*
//// * TODO schedule?
//// */
//// ((Subject) subj).execActions(transition);
////
//// }
//// n++;
//// }
////
//// /*
//// * TODO record collection and initializations w/their provenance
//// */
////
//// smonitor.info("created " + n + " " + s.model.getObservable().getType()
//// + " subjects", Messages.INFOCLASS_MODEL);
// }
//
// if (transition == null && s.accessor == null) {
// s.accessor = (IDirectActuator>) Dataflow.getDirectActuator(s.model, context, smonitor);
// }
//
// // if there is an accessor, run it
// if (s.accessor != null) {
//
// if (transition == null) {
//
// s.accessor.notifyModel(s.model);
// for (IObservable observable : s.model.getObservables()) {
// if (NS.isQuality(observable) || NS.isTrait(observable)) {
// s.accessor.notifyExpectedOutput(observable, observable
// .getObserver(), observable.getFormalName());
// }
// }
//
// /*
// * if the model is a process model, create the process and insert
// * it in the observation schedule.
// */
// if (s.accessor instanceof IProcessActuator) {
// /*
// * TODO use subject factory and an annotation mechanism like
// * for subjects to select the implementation.
// */
// IActiveProcess process = new Process(s.model, this.subject, (IProcessActuator) s.accessor, smonitor);
// ((IProcessActuator) s.accessor)
// .initialize(process, this.subject, context, smonitor);
// ((Process) process).setObservable(((Observable) process.getObservable())
// .withInterpretingTrait(s.interpretAs));
// this.subject.addProcess(process);
//
// } else if (s.accessor instanceof IEventActuator) {
//
// /*
// * TODO event models should have a chance to create and
// * process an event at transitions only, initialization should
// * not be passed anything.
// */
//
// } else {
// this.subject = (Subject) ((ISubjectActuator) s.accessor)
// .initialize((IActiveSubject) this.subject, (IActiveDirectObservation) this.contextSubject, context, smonitor);
// }
//
// /*
// * if this was a subject model, the accessor may have created
// * subjects, which we need to resolve in the main subject's
// * context. TODO this must resolve and initialize both subjects
// * and relationships. CHECK is the subject being inserted in the
// * observation graph?
// */
// if (NS.isThing(s.model.getObservable())) {
// for (ISubject subj : ((ISubject) this.subject).getSubjects()) {
//
// if (!((Subject) subj).isInitialized()) {
// ((Subject) subj).setContextSubject(this.subject);
// if (((Subject) subj)
// .initialize(this.subject
// .getResolutionScope(), /* FIXME! */ null, smonitor)
// .isEmpty()) {
// smonitor.warn("cannot resolve dependent subject "
// + subj.getName());
// } else {
//
// ((Subject) subj).setObservable(((Observable) subj.getObservable())
// .withInterpretingTrait(s.interpretAs));
//
// /*
// * TODO schedule?
// */
//
// ((Subject) subj).execActions(transition);
//
// }
// }
// }
// }
//
// }
//
// /*
// * run actions if any
// */
// ((Subject) this.subject).execActions(transition);
//
// }
// }
// }
//
// return false;
//
// }
//
// public void merge(ResolutionStrategy strategy) {
// for (Step s : strategy) {
// this.steps.add(s);
// }
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy