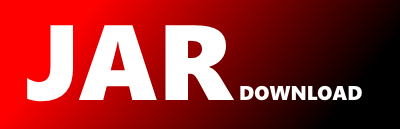
org.integratedmodelling.engine.time.extents.IrregularTemporalGrid Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.time.extents;
import java.util.Iterator;
import org.integratedmodelling.api.knowledge.IConcept;
import org.integratedmodelling.api.knowledge.IProperty;
import org.integratedmodelling.api.metadata.IMetadata;
import org.integratedmodelling.api.modelling.IExtent;
import org.integratedmodelling.api.modelling.IObservable;
import org.integratedmodelling.api.modelling.IObserver;
import org.integratedmodelling.api.modelling.IScale;
import org.integratedmodelling.api.modelling.IScale.Index;
import org.integratedmodelling.api.modelling.IScale.Locator;
import org.integratedmodelling.api.modelling.ITopologicallyComparable;
import org.integratedmodelling.api.modelling.storage.IStorage;
import org.integratedmodelling.api.space.ISpatialExtent;
import org.integratedmodelling.api.time.ITemporalExtent;
import org.integratedmodelling.api.time.ITimeDuration;
import org.integratedmodelling.api.time.ITimeInstant;
import org.integratedmodelling.api.time.ITimePeriod;
import org.integratedmodelling.collections.Pair;
import org.integratedmodelling.common.model.runtime.Observation;
import org.integratedmodelling.engine.time.Time;
import org.integratedmodelling.exceptions.KlabException;
/**
* @author Ferdinando Villa
*
*/
public class IrregularTemporalGrid extends Observation implements ITemporalExtent {
@Override
public Object getValue(int offset) {
// TODO Auto-generated method stub
return null;
}
@Override
public int[] getDimensionSizes() {
return new int[] { (int) getMultiplicity() };
}
@Override
public int[] getDimensionOffsets(int linearOffset, boolean rowFirst) {
return new int[] { linearOffset };
}
@Override
public long getValueCount() {
// TODO Auto-generated method stub
return 0;
}
@Override
public boolean isSpatiallyDistributed() {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean isTemporallyDistributed() {
// TODO Auto-generated method stub
return false;
}
@Override
public long getMultiplicity() {
// TODO Auto-generated method stub
return 0L;
}
@Override
public ITemporalExtent intersection(IExtent other) {
// TODO Auto-generated method stub
return null;
}
@Override
public IExtent union(IExtent other) {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean contains(IExtent o) throws KlabException {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean overlaps(IExtent o) throws KlabException {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean intersects(IExtent o) throws KlabException {
// TODO Auto-generated method stub
return false;
}
@Override
public ITimePeriod collapse() {
// TODO Auto-generated method stub
return null;
}
@Override
public ITemporalExtent getExtent(int stateIndex) {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean isCovered(int stateIndex) {
// TODO Auto-generated method stub
return false;
}
// @Override
// public boolean isDiscontinuous() throws ThinklabException {
// // TODO Auto-generated method stub
// return false;
// }
@Override
public IConcept getDomainConcept() {
return Time.TIME_DOMAIN;
}
@Override
public IObserver getObserver() {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean isTemporal() {
// TODO Auto-generated method stub
return true;
}
@Override
public boolean isSpatial() {
// TODO Auto-generated method stub
return false;
}
@Override
public ISpatialExtent getSpace() {
return null;
}
@Override
public ITemporalExtent getTime() {
return this;
}
@Override
public boolean isConsistent() {
// TODO Auto-generated method stub
return false;
}
@Override
public IProperty getCoverageProperty() {
// TODO Auto-generated method stub
return null;
}
@Override
public IExtent merge(IExtent extent, boolean force) throws KlabException {
// TODO Auto-generated method stub
return null;
}
@Override
public Pair, Double> checkCoverage(ITopologicallyComparable> obj) {
// TODO Auto-generated method stub
return null;
}
@Override
public ITopologicallyComparable union(ITopologicallyComparable> other)
throws KlabException {
// TODO Auto-generated method stub
return null;
}
@Override
public ITopologicallyComparable intersection(ITopologicallyComparable> other)
throws KlabException {
// TODO Auto-generated method stub
return null;
}
@Override
public double getCoveredExtent() {
// TODO Auto-generated method stub
return 0;
}
@Override
public boolean isEmpty() {
// TODO Auto-generated method stub
return false;
}
@Override
public ITimeInstant getStart() {
// TODO Auto-generated method stub
return null;
}
@Override
public ITimeInstant getEnd() {
// TODO Auto-generated method stub
return null;
}
@Override
public IScale getScale() {
// TODO Auto-generated method stub
return null;
}
//
// @Override
// public BitSet getMask() {
// // TODO Auto-generated method stub
// return null;
// }
@Override
public ITemporalExtent getExtent() {
// TODO Auto-generated method stub
return null;
}
@Override
public ITimeDuration getStep() {
// TODO Auto-generated method stub
return null;
}
@Override
public IStorage> getStorage() {
// TODO Auto-generated method stub
return null;
}
@Override
public Iterator> iterator(Index index) {
// TODO Auto-generated method stub
return null;
}
@Override
public IMetadata getMetadata() {
// TODO Auto-generated method stub
return null;
}
@Override
public IObservable getObservable() {
// TODO Auto-generated method stub
return null;
}
// @Override
// public IConcept getDirectType() {
// // TODO Auto-generated method stub
// return null;
// }
//
// @Override
// public INamespace getNamespace() {
// // TODO Auto-generated method stub
// return null;
// }
//
// @Override
// public boolean is(Object other) {
// // TODO Auto-generated method stub
// return false;
// }
@Override
public int locate(Locator locator) {
if (locator == null)
return 0;
// TODO locate transition or time period/instant
return -1;
}
@Override
public Mediator getMediator(IExtent extent, IObservable observable, IConcept trait) {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean isConstant() {
return getMultiplicity() == 1;
}
@Override
public boolean isDynamic() {
return getMultiplicity() > 1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy