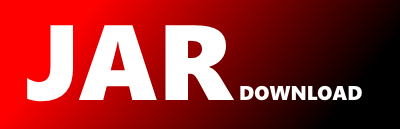
org.integratedmodelling.engine.visualization.VideoMedia Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (C) 2007, 2015:
*
* - Ferdinando Villa
* - integratedmodelling.org
* - any other authors listed in @author annotations
*
* All rights reserved. This file is part of the k.LAB software suite,
* meant to enable modular, collaborative, integrated
* development of interoperable data and model components. For
* details, see http://integratedmodelling.org.
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Affero General Public License
* Version 3 or any later version.
*
* This program is distributed in the hope that it will be useful,
* but without any warranty; without even the implied warranty of
* merchantability or fitness for a particular purpose. See the
* Affero General Public License for more details.
*
* You should have received a copy of the Affero General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* The license is also available at: https://www.gnu.org/licenses/agpl.html
*******************************************************************************/
package org.integratedmodelling.engine.visualization;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.util.Map;
import javax.activation.MimeType;
import javax.imageio.stream.FileImageOutputStream;
import javax.imageio.stream.ImageOutputStream;
import org.integratedmodelling.api.modelling.IObservation;
import org.integratedmodelling.api.modelling.IScale;
import org.integratedmodelling.api.modelling.IState;
import org.integratedmodelling.api.modelling.visualization.IColormap;
import org.integratedmodelling.api.modelling.visualization.IFileMedia;
import org.integratedmodelling.api.modelling.visualization.IImageViewport;
import org.integratedmodelling.api.modelling.visualization.ILegend;
import org.integratedmodelling.api.modelling.visualization.IMedia;
import org.integratedmodelling.api.modelling.visualization.IViewport;
import org.integratedmodelling.api.monitoring.IMonitor;
import org.integratedmodelling.api.space.ISpatialExtent;
import org.integratedmodelling.api.time.ITemporalExtent;
import org.integratedmodelling.common.space.SpaceLocator;
import org.integratedmodelling.common.time.TimeLocator;
import org.integratedmodelling.common.utils.image.GifSequenceWriter;
import org.integratedmodelling.common.utils.image.ImageUtil;
import org.integratedmodelling.common.visualization.Viewport;
import org.integratedmodelling.engine.geospace.extents.Grid;
import org.integratedmodelling.engine.geospace.extents.SpaceExtent;
import org.integratedmodelling.engine.geospace.interfaces.IGridMask;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabIOException;
import org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
public class VideoMedia implements IFileMedia {
public static final int COVERAGE_MAP = 0;
public static final int EARTH_IMAGE_BACKGROUND = 1;
public static final int CONTOUR_MAP = 2;
private double SMOOTH_FACTOR = 1.8;
IImageViewport _viewport;
IObservation _observation;
IScale.Index _index;
private IColormap _colormap;
// private StateClass _type;
private ILegend _legend;
private IMonitor _monitor;
private boolean _addDate = true;
/*
* true if no-data appear in the data.
*/
boolean _hasNull = false;
int _mapType = COVERAGE_MAP;
private MimeType _mtype;
private DateTimeFormatter dateFormat = DateTimeFormat.forPattern("dd/MM/YYYY hh:mm");
/**
* TODO add back media type when we're more flexible.
*
* @param observation
* @param viewport
* @param options
*/
public VideoMedia(IObservation observation, IViewport viewport, Map options) {
_viewport = (IImageViewport) viewport;
if (_viewport == null) {
_viewport = new Viewport(600, 600);
}
_observation = observation;
if (options != null) {
if (options.containsKey("image-type")) {
String tp = options.get("image-type").toString();
if (tp.equals("coverage")) {
_mapType = COVERAGE_MAP;
} else if (tp.equals("contour")) {
_mapType = CONTOUR_MAP;
}
}
}
}
// @Override
// public MimeType getMIMEType() {
// return _mtype;
// }
@Override
public ILegend getLegend() {
return _legend;
}
@Override
public File getFile() throws KlabException {
ISpatialExtent space = _observation.getScale().getSpace();
ITemporalExtent time = _observation.getScale().getTime();
File ret = null;
if (_observation instanceof IState) {
if (space != null && time != null) {
IColormap colormap = VisualizationFactory.getColormap((IState) _observation);
GifSequenceWriter writer = null;
ImageOutputStream output = null;
try {
/*
* TODO this one will not loop and have 200ms between frames. Make these parameters.
*/
ret = File.createTempFile("vid", "gif");
output = new FileImageOutputStream(ret);
for (int i = 0; i < time.getMultiplicity()
- 3 /* FIXME NEEDS CHECK - NOT ALL STATES ARE FLUSHED */; i++) {
BufferedImage map = (BufferedImage) makeMap(space, VisualizationFactory
.getDisplayData((IState) _observation, _observation
.getScale()
.getIndex(TimeLocator.get(i), SpaceLocator.all()), true), colormap);
if (_addDate) {
// ugh
ITemporalExtent text = time.getExtent(i);
String timestamp = new DateTime(text.getStart().getMillis()).toString(dateFormat);
Graphics g = map.getGraphics();
g.setFont(g.getFont().deriveFont(10f));
g.drawString(timestamp, map.getWidth() - 90, map.getHeight() - 5);
BufferedImage newmap = new BufferedImage(map.getWidth(), map
.getHeight(), BufferedImage.TYPE_BYTE_INDEXED);
newmap.getGraphics().drawImage(map, 0, 0, null);
g.dispose();
map = newmap;
}
if (writer == null) {
writer = new GifSequenceWriter(output, map.getType(), 333, false);
}
writer.writeToSequence(map);
}
} catch (Exception e) {
throw new KlabIOException(e);
} finally {
try {
if (writer != null) {
writer.close();
}
if (output != null) {
output.close();
}
} catch (Exception e) {
// aaargh
throw new KlabIOException(e);
}
}
}
}
return ret;
}
@Override
public IMedia scale(IViewport viewport) {
return null;
}
public Image makeMap(ISpatialExtent spaceExt, int[] data, IColormap colormap) throws KlabException {
if (data == null) {
return null;
}
int n = 0;
for (int z : data) {
if (z > 0) {
n++;
}
}
if (spaceExt instanceof SpaceExtent && ((SpaceExtent) spaceExt).isGrid()) {
Grid space = ((SpaceExtent) spaceExt).getGrid();
IGridMask mask = space.getActivationLayer();
int[] xy = _viewport.getSizeFor(space.getXCells(), space.getYCells());
return ImageUtil
.createImage(ImageUtil.upsideDown(data, space.getXCells()), space
.getXCells(), xy[0], xy[1], colormap);
}
return null;
}
@Override
public String getMediaType() {
return _mtype.toString();
}
@Override
public ByteArrayInputStream getStream() throws KlabException {
// FileOutputStream os = new FileOutputStream();
// try {
// ImageIO.write((RenderedImage) getImage(),"png", os);
// } catch (IOException e) {
// throw new KlabIOException(e);
// }
// return new ByteArrayInputStream(os.toByteArray());
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy