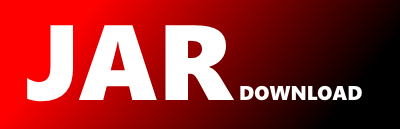
org.integratedmodelling.kmodeler.controller.ObservationKboxController Maven / Gradle / Ivy
The newest version!
package org.integratedmodelling.kmodeler.controller;
import java.io.File;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.integratedmodelling.api.configuration.IResourceConfiguration.StaticResource;
import org.integratedmodelling.api.modelling.IDirectObserver;
import org.integratedmodelling.api.modelling.INamespace;
import org.integratedmodelling.api.modelling.IObservableSemantics;
import org.integratedmodelling.api.modelling.IScale;
import org.integratedmodelling.api.network.API;
import org.integratedmodelling.api.runtime.ISession;
import org.integratedmodelling.common.beans.Observation;
import org.integratedmodelling.common.beans.ObservationData;
import org.integratedmodelling.common.beans.requests.DeployRequest;
import org.integratedmodelling.common.beans.requests.ObservationQuery;
import org.integratedmodelling.common.beans.responses.ObservationQueryResponse;
import org.integratedmodelling.common.beans.responses.Observations;
import org.integratedmodelling.common.configuration.KLAB;
import org.integratedmodelling.common.kim.KIMNamespace;
import org.integratedmodelling.common.utils.MiscUtilities;
import org.integratedmodelling.common.vocabulary.ObservableSemantics;
import org.integratedmodelling.engine.ModelFactory;
import org.integratedmodelling.engine.geospace.coverage.vector.VectorCoverage;
import org.integratedmodelling.engine.modelling.kbox.ObservationKbox;
import org.integratedmodelling.engine.modelling.runtime.Scale;
import org.integratedmodelling.exceptions.KlabAuthorizationException;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.exceptions.KlabResourceNotFoundException;
import org.integratedmodelling.exceptions.KlabUnsupportedOperationException;
import org.integratedmodelling.kmodeler.components.SessionManager;
import org.integratedmodelling.kserver.controller.KServerController;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
/**
* Engine implementation, using session authentication and broadcasting to all
* visible nodes.
*
* @author ferdinando.villa
*
*/
@RestController
public class ObservationKboxController {
@Autowired
SessionManager sessionManager;
@RequestMapping(value = API.QUERY_OBSERVATIONS, method = RequestMethod.POST)
public @ResponseBody ObservationQueryResponse query(@RequestBody ObservationQuery query,
@RequestHeader HttpHeaders headers, HttpServletRequest request) {
return null;
}
@RequestMapping(value = API.RETRIEVE_OBSERVATION, method = RequestMethod.GET)
public @ResponseBody Observation retrieve(@PathVariable String id, @RequestHeader HttpHeaders headers,
HttpServletRequest request) {
return null;
}
/**
* For now importing observations is local only - full support of remote
* requires archiving potentially multiple files, and it's more of a node
* thing than an engine thing. The supported workflow for now should be
* local import into engine, and if requested, remote upload to node from
* engine, passing finished and validated observations through the same or a
* different endpoint rather than an import file.
*
* @param data
* @param headers
* @param request
* @return
* @throws KlabException
*/
@RequestMapping(value = API.IMPORT_OBSERVATIONS, method = RequestMethod.POST)
public @ResponseBody Observations importObservations(@RequestBody DeployRequest data,
@RequestHeader HttpHeaders headers, HttpServletRequest request) throws KlabException {
if (!(KServerController.isLocalIp(request) || KServerController.isAdmin(headers))) {
throw new KlabAuthorizationException("no authorization for local deployment");
}
// String id = data.getId();
File outfile = new File(data.getPath());
if (!outfile.exists()) {
throw new KlabResourceNotFoundException("import file " + outfile + " not found at engine side");
}
List result = null;
if (MiscUtilities.getFileExtension(outfile.toString()).equals("shp")) {
result = VectorCoverage.readFeatures(outfile);
} /* TODO other formats - maybe pluginize one day if justified */ else {
throw new KlabUnsupportedOperationException("don't know how to import observations from file " + outfile);
}
Observations ret = new Observations();
if (result != null) {
ret.getObservations().addAll(result);
}
return ret;
}
/**
* Submit observation. Return a unique URN for it.
*
* TODO should take complex observations with children, states and state values.
*
* @param data
* @param headers
* @param request
* @throws KlabException
*/
@RequestMapping(value = API.SUBMIT_OBSERVATION, method = RequestMethod.POST)
public @ResponseBody String submitObservation(@RequestBody Observation data, @RequestHeader HttpHeaders headers,
HttpServletRequest request) throws KlabException {
ISession session = sessionManager.getSession(headers.get(API.AUTHENTICATION_HEADER));
if (session == null) {
throw new KlabAuthorizationException("observe: session authentication failed");
}
/*
* user must be allowed to submit to this node
*/
if (!KLAB.ENGINE.getResourceConfiguration().isAuthorized(StaticResource.OBSERVATION_SUBMIT, session.getUser(),
request.getRemoteHost())) {
throw new KlabAuthorizationException("user " + session.getUser() + " is not authorized to submit observations");
}
/*
* TODO must become much more comprehensive, with states, sub-objects etc.
*/
IScale scale = KLAB.MFACTORY.adapt(data.getScale(), Scale.class);
IObservableSemantics observable = KLAB.MFACTORY.adapt(data.getObservable(), ObservableSemantics.class);
String id = data.getId();
INamespace localNamespace = KLAB.MMANAGER.getLocalNamespace();
IDirectObserver observer = ModelFactory.createDirectObserver(observable.getType(), id, localNamespace,
null, scale);
((KIMNamespace) localNamespace).addModelObject(observer);
long oid = ObservationKbox.get().store(observer);
ObservationKbox.get().reindexLocalObservations();
return ObservationKbox.get().getUrnForStoredObservation(oid, session.getUser());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy