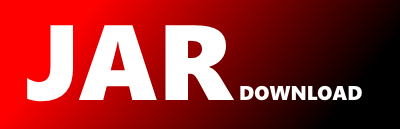
org.integratedmodelling.kmodeler.viewer.ViewController Maven / Gradle / Ivy
The newest version!
package org.integratedmodelling.kmodeler.viewer;
import org.integratedmodelling.api.network.API;
import org.integratedmodelling.api.runtime.ISession;
import org.integratedmodelling.common.beans.requests.ViewerCommand;
import org.integratedmodelling.common.beans.requests.ViewerNotification;
import org.integratedmodelling.common.model.runtime.Session;
import org.integratedmodelling.exceptions.KlabAuthorizationException;
import org.integratedmodelling.exceptions.KlabException;
import org.integratedmodelling.kmodeler.components.SessionManager;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.messaging.handler.annotation.MessageMapping;
import org.springframework.messaging.simp.SimpMessagingTemplate;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
/**
* @author ferdinando.villa
*
*/
@RestController
public class ViewController {
@Autowired
private SimpMessagingTemplate webSocket;
@Autowired
private SessionManager sessionManager;
/**
* REST endpoint for the client to send requests to the viewer.
* @param request
* @param headers
* @throws KlabException
*/
@RequestMapping(value = API.VIEW, method = RequestMethod.PUT)
public void send(@RequestBody ViewerCommand request, @RequestHeader HttpHeaders headers)
throws KlabException {
ISession session = sessionManager.getSession(headers.get(API.AUTHENTICATION_HEADER));
if (session == null) {
throw new KlabAuthorizationException("viewer: session authentication failed");
}
sendViewerCommand(session, request);
}
/**
* Sends messages to Javascript.
*
* @param session
* @param command
*/
public void sendViewerCommand(ISession session, ViewerCommand command) {
webSocket.convertAndSend("/session/" + session.getId(), command);
}
/**
* This gets messages sent to /klab/viewer from the javascript
* side of the dataviewer. In addition to consuming these ourselves,
* we relay some UI actions (such as clicking on the map) to the
* client, using send() in the session monitor.
*
* @param message
*/
@MessageMapping(API.VIEWER_ENDPOINT)
public void handleTask(ViewerNotification message) {
ISession session = sessionManager.getSession(message.getSessionId());
switch (message.getCommand()) {
case "on-click":
case "object-drop":
case "tool-drop":
case "on-view-change":
case "shape-added":
((Session)session).getMonitor().send(message);
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy