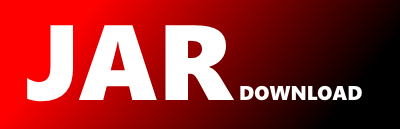
org.integratedmodelling.kim.kim.impl.ActionImpl Maven / Gradle / Ivy
/**
* generated by Xtext 2.9.2
*/
package org.integratedmodelling.kim.kim.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
import org.integratedmodelling.kim.kim.Action;
import org.integratedmodelling.kim.kim.Condition;
import org.integratedmodelling.kim.kim.KimPackage;
import org.integratedmodelling.kim.kim.Value;
/**
*
* An implementation of the model object 'Action'.
*
*
* The following features are implemented:
*
*
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isChange Change}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isSet Set}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#getChanged Changed}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#getValue Value}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#getExtension Extension}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#getCondition Condition}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isIntegrate Integrate}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isDo Do}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isMove Move}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#getWhere Where}
* - {@link org.integratedmodelling.kim.kim.impl.ActionImpl#isAway Away}
*
*
* @generated
*/
public class ActionImpl extends MinimalEObjectImpl.Container implements Action
{
/**
* The default value of the '{@link #isChange() Change}' attribute.
*
*
* @see #isChange()
* @generated
* @ordered
*/
protected static final boolean CHANGE_EDEFAULT = false;
/**
* The cached value of the '{@link #isChange() Change}' attribute.
*
*
* @see #isChange()
* @generated
* @ordered
*/
protected boolean change = CHANGE_EDEFAULT;
/**
* The default value of the '{@link #isSet() Set}' attribute.
*
*
* @see #isSet()
* @generated
* @ordered
*/
protected static final boolean SET_EDEFAULT = false;
/**
* The cached value of the '{@link #isSet() Set}' attribute.
*
*
* @see #isSet()
* @generated
* @ordered
*/
protected boolean set = SET_EDEFAULT;
/**
* The default value of the '{@link #getChanged() Changed}' attribute.
*
*
* @see #getChanged()
* @generated
* @ordered
*/
protected static final String CHANGED_EDEFAULT = null;
/**
* The cached value of the '{@link #getChanged() Changed}' attribute.
*
*
* @see #getChanged()
* @generated
* @ordered
*/
protected String changed = CHANGED_EDEFAULT;
/**
* The cached value of the '{@link #getValue() Value}' containment reference.
*
*
* @see #getValue()
* @generated
* @ordered
*/
protected Value value;
/**
* The default value of the '{@link #getExtension() Extension}' attribute.
*
*
* @see #getExtension()
* @generated
* @ordered
*/
protected static final String EXTENSION_EDEFAULT = null;
/**
* The cached value of the '{@link #getExtension() Extension}' attribute.
*
*
* @see #getExtension()
* @generated
* @ordered
*/
protected String extension = EXTENSION_EDEFAULT;
/**
* The cached value of the '{@link #getCondition() Condition}' containment reference.
*
*
* @see #getCondition()
* @generated
* @ordered
*/
protected Condition condition;
/**
* The default value of the '{@link #isIntegrate() Integrate}' attribute.
*
*
* @see #isIntegrate()
* @generated
* @ordered
*/
protected static final boolean INTEGRATE_EDEFAULT = false;
/**
* The cached value of the '{@link #isIntegrate() Integrate}' attribute.
*
*
* @see #isIntegrate()
* @generated
* @ordered
*/
protected boolean integrate = INTEGRATE_EDEFAULT;
/**
* The default value of the '{@link #isDo() Do}' attribute.
*
*
* @see #isDo()
* @generated
* @ordered
*/
protected static final boolean DO_EDEFAULT = false;
/**
* The cached value of the '{@link #isDo() Do}' attribute.
*
*
* @see #isDo()
* @generated
* @ordered
*/
protected boolean do_ = DO_EDEFAULT;
/**
* The default value of the '{@link #isMove() Move}' attribute.
*
*
* @see #isMove()
* @generated
* @ordered
*/
protected static final boolean MOVE_EDEFAULT = false;
/**
* The cached value of the '{@link #isMove() Move}' attribute.
*
*
* @see #isMove()
* @generated
* @ordered
*/
protected boolean move = MOVE_EDEFAULT;
/**
* The cached value of the '{@link #getWhere() Where}' containment reference.
*
*
* @see #getWhere()
* @generated
* @ordered
*/
protected Value where;
/**
* The default value of the '{@link #isAway() Away}' attribute.
*
*
* @see #isAway()
* @generated
* @ordered
*/
protected static final boolean AWAY_EDEFAULT = false;
/**
* The cached value of the '{@link #isAway() Away}' attribute.
*
*
* @see #isAway()
* @generated
* @ordered
*/
protected boolean away = AWAY_EDEFAULT;
/**
*
*
* @generated
*/
protected ActionImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass()
{
return KimPackage.Literals.ACTION;
}
/**
*
*
* @generated
*/
public boolean isChange()
{
return change;
}
/**
*
*
* @generated
*/
public void setChange(boolean newChange)
{
boolean oldChange = change;
change = newChange;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__CHANGE, oldChange, change));
}
/**
*
*
* @generated
*/
public boolean isSet()
{
return set;
}
/**
*
*
* @generated
*/
public void setSet(boolean newSet)
{
boolean oldSet = set;
set = newSet;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__SET, oldSet, set));
}
/**
*
*
* @generated
*/
public String getChanged()
{
return changed;
}
/**
*
*
* @generated
*/
public void setChanged(String newChanged)
{
String oldChanged = changed;
changed = newChanged;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__CHANGED, oldChanged, changed));
}
/**
*
*
* @generated
*/
public Value getValue()
{
return value;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetValue(Value newValue, NotificationChain msgs)
{
Value oldValue = value;
value = newValue;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__VALUE, oldValue, newValue);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setValue(Value newValue)
{
if (newValue != value)
{
NotificationChain msgs = null;
if (value != null)
msgs = ((InternalEObject)value).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__VALUE, null, msgs);
if (newValue != null)
msgs = ((InternalEObject)newValue).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__VALUE, null, msgs);
msgs = basicSetValue(newValue, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__VALUE, newValue, newValue));
}
/**
*
*
* @generated
*/
public String getExtension()
{
return extension;
}
/**
*
*
* @generated
*/
public void setExtension(String newExtension)
{
String oldExtension = extension;
extension = newExtension;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__EXTENSION, oldExtension, extension));
}
/**
*
*
* @generated
*/
public Condition getCondition()
{
return condition;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetCondition(Condition newCondition, NotificationChain msgs)
{
Condition oldCondition = condition;
condition = newCondition;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__CONDITION, oldCondition, newCondition);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setCondition(Condition newCondition)
{
if (newCondition != condition)
{
NotificationChain msgs = null;
if (condition != null)
msgs = ((InternalEObject)condition).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__CONDITION, null, msgs);
if (newCondition != null)
msgs = ((InternalEObject)newCondition).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__CONDITION, null, msgs);
msgs = basicSetCondition(newCondition, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__CONDITION, newCondition, newCondition));
}
/**
*
*
* @generated
*/
public boolean isIntegrate()
{
return integrate;
}
/**
*
*
* @generated
*/
public void setIntegrate(boolean newIntegrate)
{
boolean oldIntegrate = integrate;
integrate = newIntegrate;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__INTEGRATE, oldIntegrate, integrate));
}
/**
*
*
* @generated
*/
public boolean isDo()
{
return do_;
}
/**
*
*
* @generated
*/
public void setDo(boolean newDo)
{
boolean oldDo = do_;
do_ = newDo;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__DO, oldDo, do_));
}
/**
*
*
* @generated
*/
public boolean isMove()
{
return move;
}
/**
*
*
* @generated
*/
public void setMove(boolean newMove)
{
boolean oldMove = move;
move = newMove;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__MOVE, oldMove, move));
}
/**
*
*
* @generated
*/
public Value getWhere()
{
return where;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetWhere(Value newWhere, NotificationChain msgs)
{
Value oldWhere = where;
where = newWhere;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__WHERE, oldWhere, newWhere);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setWhere(Value newWhere)
{
if (newWhere != where)
{
NotificationChain msgs = null;
if (where != null)
msgs = ((InternalEObject)where).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__WHERE, null, msgs);
if (newWhere != null)
msgs = ((InternalEObject)newWhere).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.ACTION__WHERE, null, msgs);
msgs = basicSetWhere(newWhere, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__WHERE, newWhere, newWhere));
}
/**
*
*
* @generated
*/
public boolean isAway()
{
return away;
}
/**
*
*
* @generated
*/
public void setAway(boolean newAway)
{
boolean oldAway = away;
away = newAway;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.ACTION__AWAY, oldAway, away));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs)
{
switch (featureID)
{
case KimPackage.ACTION__VALUE:
return basicSetValue(null, msgs);
case KimPackage.ACTION__CONDITION:
return basicSetCondition(null, msgs);
case KimPackage.ACTION__WHERE:
return basicSetWhere(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case KimPackage.ACTION__CHANGE:
return isChange();
case KimPackage.ACTION__SET:
return isSet();
case KimPackage.ACTION__CHANGED:
return getChanged();
case KimPackage.ACTION__VALUE:
return getValue();
case KimPackage.ACTION__EXTENSION:
return getExtension();
case KimPackage.ACTION__CONDITION:
return getCondition();
case KimPackage.ACTION__INTEGRATE:
return isIntegrate();
case KimPackage.ACTION__DO:
return isDo();
case KimPackage.ACTION__MOVE:
return isMove();
case KimPackage.ACTION__WHERE:
return getWhere();
case KimPackage.ACTION__AWAY:
return isAway();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case KimPackage.ACTION__CHANGE:
setChange((Boolean)newValue);
return;
case KimPackage.ACTION__SET:
setSet((Boolean)newValue);
return;
case KimPackage.ACTION__CHANGED:
setChanged((String)newValue);
return;
case KimPackage.ACTION__VALUE:
setValue((Value)newValue);
return;
case KimPackage.ACTION__EXTENSION:
setExtension((String)newValue);
return;
case KimPackage.ACTION__CONDITION:
setCondition((Condition)newValue);
return;
case KimPackage.ACTION__INTEGRATE:
setIntegrate((Boolean)newValue);
return;
case KimPackage.ACTION__DO:
setDo((Boolean)newValue);
return;
case KimPackage.ACTION__MOVE:
setMove((Boolean)newValue);
return;
case KimPackage.ACTION__WHERE:
setWhere((Value)newValue);
return;
case KimPackage.ACTION__AWAY:
setAway((Boolean)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case KimPackage.ACTION__CHANGE:
setChange(CHANGE_EDEFAULT);
return;
case KimPackage.ACTION__SET:
setSet(SET_EDEFAULT);
return;
case KimPackage.ACTION__CHANGED:
setChanged(CHANGED_EDEFAULT);
return;
case KimPackage.ACTION__VALUE:
setValue((Value)null);
return;
case KimPackage.ACTION__EXTENSION:
setExtension(EXTENSION_EDEFAULT);
return;
case KimPackage.ACTION__CONDITION:
setCondition((Condition)null);
return;
case KimPackage.ACTION__INTEGRATE:
setIntegrate(INTEGRATE_EDEFAULT);
return;
case KimPackage.ACTION__DO:
setDo(DO_EDEFAULT);
return;
case KimPackage.ACTION__MOVE:
setMove(MOVE_EDEFAULT);
return;
case KimPackage.ACTION__WHERE:
setWhere((Value)null);
return;
case KimPackage.ACTION__AWAY:
setAway(AWAY_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case KimPackage.ACTION__CHANGE:
return change != CHANGE_EDEFAULT;
case KimPackage.ACTION__SET:
return set != SET_EDEFAULT;
case KimPackage.ACTION__CHANGED:
return CHANGED_EDEFAULT == null ? changed != null : !CHANGED_EDEFAULT.equals(changed);
case KimPackage.ACTION__VALUE:
return value != null;
case KimPackage.ACTION__EXTENSION:
return EXTENSION_EDEFAULT == null ? extension != null : !EXTENSION_EDEFAULT.equals(extension);
case KimPackage.ACTION__CONDITION:
return condition != null;
case KimPackage.ACTION__INTEGRATE:
return integrate != INTEGRATE_EDEFAULT;
case KimPackage.ACTION__DO:
return do_ != DO_EDEFAULT;
case KimPackage.ACTION__MOVE:
return move != MOVE_EDEFAULT;
case KimPackage.ACTION__WHERE:
return where != null;
case KimPackage.ACTION__AWAY:
return away != AWAY_EDEFAULT;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (change: ");
result.append(change);
result.append(", set: ");
result.append(set);
result.append(", changed: ");
result.append(changed);
result.append(", extension: ");
result.append(extension);
result.append(", integrate: ");
result.append(integrate);
result.append(", do: ");
result.append(do_);
result.append(", move: ");
result.append(move);
result.append(", away: ");
result.append(away);
result.append(')');
return result.toString();
}
} //ActionImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy