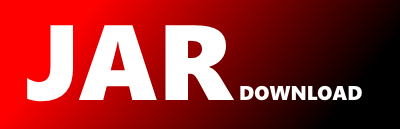
org.integratedmodelling.kim.kim.impl.ContextualizationImpl Maven / Gradle / Ivy
/**
* generated by Xtext 2.9.2
*/
package org.integratedmodelling.kim.kim.impl;
import java.util.Collection;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
import org.eclipse.emf.ecore.util.EObjectContainmentEList;
import org.eclipse.emf.ecore.util.InternalEList;
import org.integratedmodelling.kim.kim.Action;
import org.integratedmodelling.kim.kim.ConceptDeclaration;
import org.integratedmodelling.kim.kim.Contextualization;
import org.integratedmodelling.kim.kim.FunctionOrID;
import org.integratedmodelling.kim.kim.KimPackage;
/**
*
* An implementation of the model object 'Contextualization'.
*
*
* The following features are implemented:
*
*
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#isIntegrated Integrated}
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#getDomain Domain}
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#getActions Actions}
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#isInitialization Initialization}
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#isResolution Resolution}
* - {@link org.integratedmodelling.kim.kim.impl.ContextualizationImpl#getEvent Event}
*
*
* @generated
*/
public class ContextualizationImpl extends MinimalEObjectImpl.Container implements Contextualization
{
/**
* The default value of the '{@link #isIntegrated() Integrated}' attribute.
*
*
* @see #isIntegrated()
* @generated
* @ordered
*/
protected static final boolean INTEGRATED_EDEFAULT = false;
/**
* The cached value of the '{@link #isIntegrated() Integrated}' attribute.
*
*
* @see #isIntegrated()
* @generated
* @ordered
*/
protected boolean integrated = INTEGRATED_EDEFAULT;
/**
* The cached value of the '{@link #getDomain() Domain}' containment reference list.
*
*
* @see #getDomain()
* @generated
* @ordered
*/
protected EList domain;
/**
* The cached value of the '{@link #getActions() Actions}' containment reference list.
*
*
* @see #getActions()
* @generated
* @ordered
*/
protected EList actions;
/**
* The default value of the '{@link #isInitialization() Initialization}' attribute.
*
*
* @see #isInitialization()
* @generated
* @ordered
*/
protected static final boolean INITIALIZATION_EDEFAULT = false;
/**
* The cached value of the '{@link #isInitialization() Initialization}' attribute.
*
*
* @see #isInitialization()
* @generated
* @ordered
*/
protected boolean initialization = INITIALIZATION_EDEFAULT;
/**
* The default value of the '{@link #isResolution() Resolution}' attribute.
*
*
* @see #isResolution()
* @generated
* @ordered
*/
protected static final boolean RESOLUTION_EDEFAULT = false;
/**
* The cached value of the '{@link #isResolution() Resolution}' attribute.
*
*
* @see #isResolution()
* @generated
* @ordered
*/
protected boolean resolution = RESOLUTION_EDEFAULT;
/**
* The cached value of the '{@link #getEvent() Event}' containment reference.
*
*
* @see #getEvent()
* @generated
* @ordered
*/
protected ConceptDeclaration event;
/**
*
*
* @generated
*/
protected ContextualizationImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass()
{
return KimPackage.Literals.CONTEXTUALIZATION;
}
/**
*
*
* @generated
*/
public boolean isIntegrated()
{
return integrated;
}
/**
*
*
* @generated
*/
public void setIntegrated(boolean newIntegrated)
{
boolean oldIntegrated = integrated;
integrated = newIntegrated;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.CONTEXTUALIZATION__INTEGRATED, oldIntegrated, integrated));
}
/**
*
*
* @generated
*/
public EList getDomain()
{
if (domain == null)
{
domain = new EObjectContainmentEList(FunctionOrID.class, this, KimPackage.CONTEXTUALIZATION__DOMAIN);
}
return domain;
}
/**
*
*
* @generated
*/
public EList getActions()
{
if (actions == null)
{
actions = new EObjectContainmentEList(Action.class, this, KimPackage.CONTEXTUALIZATION__ACTIONS);
}
return actions;
}
/**
*
*
* @generated
*/
public boolean isInitialization()
{
return initialization;
}
/**
*
*
* @generated
*/
public void setInitialization(boolean newInitialization)
{
boolean oldInitialization = initialization;
initialization = newInitialization;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.CONTEXTUALIZATION__INITIALIZATION, oldInitialization, initialization));
}
/**
*
*
* @generated
*/
public boolean isResolution()
{
return resolution;
}
/**
*
*
* @generated
*/
public void setResolution(boolean newResolution)
{
boolean oldResolution = resolution;
resolution = newResolution;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.CONTEXTUALIZATION__RESOLUTION, oldResolution, resolution));
}
/**
*
*
* @generated
*/
public ConceptDeclaration getEvent()
{
return event;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetEvent(ConceptDeclaration newEvent, NotificationChain msgs)
{
ConceptDeclaration oldEvent = event;
event = newEvent;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.CONTEXTUALIZATION__EVENT, oldEvent, newEvent);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setEvent(ConceptDeclaration newEvent)
{
if (newEvent != event)
{
NotificationChain msgs = null;
if (event != null)
msgs = ((InternalEObject)event).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.CONTEXTUALIZATION__EVENT, null, msgs);
if (newEvent != null)
msgs = ((InternalEObject)newEvent).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.CONTEXTUALIZATION__EVENT, null, msgs);
msgs = basicSetEvent(newEvent, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.CONTEXTUALIZATION__EVENT, newEvent, newEvent));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs)
{
switch (featureID)
{
case KimPackage.CONTEXTUALIZATION__DOMAIN:
return ((InternalEList>)getDomain()).basicRemove(otherEnd, msgs);
case KimPackage.CONTEXTUALIZATION__ACTIONS:
return ((InternalEList>)getActions()).basicRemove(otherEnd, msgs);
case KimPackage.CONTEXTUALIZATION__EVENT:
return basicSetEvent(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case KimPackage.CONTEXTUALIZATION__INTEGRATED:
return isIntegrated();
case KimPackage.CONTEXTUALIZATION__DOMAIN:
return getDomain();
case KimPackage.CONTEXTUALIZATION__ACTIONS:
return getActions();
case KimPackage.CONTEXTUALIZATION__INITIALIZATION:
return isInitialization();
case KimPackage.CONTEXTUALIZATION__RESOLUTION:
return isResolution();
case KimPackage.CONTEXTUALIZATION__EVENT:
return getEvent();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case KimPackage.CONTEXTUALIZATION__INTEGRATED:
setIntegrated((Boolean)newValue);
return;
case KimPackage.CONTEXTUALIZATION__DOMAIN:
getDomain().clear();
getDomain().addAll((Collection extends FunctionOrID>)newValue);
return;
case KimPackage.CONTEXTUALIZATION__ACTIONS:
getActions().clear();
getActions().addAll((Collection extends Action>)newValue);
return;
case KimPackage.CONTEXTUALIZATION__INITIALIZATION:
setInitialization((Boolean)newValue);
return;
case KimPackage.CONTEXTUALIZATION__RESOLUTION:
setResolution((Boolean)newValue);
return;
case KimPackage.CONTEXTUALIZATION__EVENT:
setEvent((ConceptDeclaration)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case KimPackage.CONTEXTUALIZATION__INTEGRATED:
setIntegrated(INTEGRATED_EDEFAULT);
return;
case KimPackage.CONTEXTUALIZATION__DOMAIN:
getDomain().clear();
return;
case KimPackage.CONTEXTUALIZATION__ACTIONS:
getActions().clear();
return;
case KimPackage.CONTEXTUALIZATION__INITIALIZATION:
setInitialization(INITIALIZATION_EDEFAULT);
return;
case KimPackage.CONTEXTUALIZATION__RESOLUTION:
setResolution(RESOLUTION_EDEFAULT);
return;
case KimPackage.CONTEXTUALIZATION__EVENT:
setEvent((ConceptDeclaration)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case KimPackage.CONTEXTUALIZATION__INTEGRATED:
return integrated != INTEGRATED_EDEFAULT;
case KimPackage.CONTEXTUALIZATION__DOMAIN:
return domain != null && !domain.isEmpty();
case KimPackage.CONTEXTUALIZATION__ACTIONS:
return actions != null && !actions.isEmpty();
case KimPackage.CONTEXTUALIZATION__INITIALIZATION:
return initialization != INITIALIZATION_EDEFAULT;
case KimPackage.CONTEXTUALIZATION__RESOLUTION:
return resolution != RESOLUTION_EDEFAULT;
case KimPackage.CONTEXTUALIZATION__EVENT:
return event != null;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (integrated: ");
result.append(integrated);
result.append(", initialization: ");
result.append(initialization);
result.append(", resolution: ");
result.append(resolution);
result.append(')');
return result.toString();
}
} //ContextualizationImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy