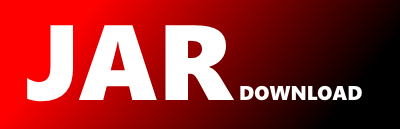
org.integratedmodelling.kim.kim.impl.ObserverImpl Maven / Gradle / Ivy
/**
* generated by Xtext 2.9.2
*/
package org.integratedmodelling.kim.kim.impl;
import java.util.Collection;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
import org.eclipse.emf.ecore.util.EObjectContainmentEList;
import org.eclipse.emf.ecore.util.InternalEList;
import org.integratedmodelling.kim.kim.Classification;
import org.integratedmodelling.kim.kim.ConceptDeclaration;
import org.integratedmodelling.kim.kim.Contextualization;
import org.integratedmodelling.kim.kim.Currency;
import org.integratedmodelling.kim.kim.Dependency;
import org.integratedmodelling.kim.kim.Function;
import org.integratedmodelling.kim.kim.KimPackage;
import org.integratedmodelling.kim.kim.LookupFunction;
import org.integratedmodelling.kim.kim.NUMBER;
import org.integratedmodelling.kim.kim.Observable;
import org.integratedmodelling.kim.kim.Observer;
import org.integratedmodelling.kim.kim.TraitDef;
import org.integratedmodelling.kim.kim.Unit;
/**
*
* An implementation of the model object 'Observer'.
*
*
* The following features are implemented:
*
*
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getObservable Observable}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getUnit Unit}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getTrait Trait}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getDiscretization Discretization}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getAccessor Accessor}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getLookup Lookup}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getDependencies Dependencies}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getContextualizers Contextualizers}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getConcept Concept}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#isDerived Derived}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getFrom From}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getTo To}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#isInteger Integer}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getOther Other}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getType Type}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getDistributionUnit Distribution Unit}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getCurrency Currency}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#isDiscretizer Discretizer}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getTraits Traits}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getClassification Classification}
* - {@link org.integratedmodelling.kim.kim.impl.ObserverImpl#getMetadataProperty Metadata Property}
*
*
* @generated
*/
public class ObserverImpl extends MinimalEObjectImpl.Container implements Observer
{
/**
* The cached value of the '{@link #getObservable() Observable}' containment reference.
*
*
* @see #getObservable()
* @generated
* @ordered
*/
protected Observable observable;
/**
* The cached value of the '{@link #getUnit() Unit}' containment reference.
*
*
* @see #getUnit()
* @generated
* @ordered
*/
protected Unit unit;
/**
* The cached value of the '{@link #getTrait() Trait}' containment reference.
*
*
* @see #getTrait()
* @generated
* @ordered
*/
protected TraitDef trait;
/**
* The cached value of the '{@link #getDiscretization() Discretization}' containment reference.
*
*
* @see #getDiscretization()
* @generated
* @ordered
*/
protected Classification discretization;
/**
* The cached value of the '{@link #getAccessor() Accessor}' containment reference.
*
*
* @see #getAccessor()
* @generated
* @ordered
*/
protected Function accessor;
/**
* The cached value of the '{@link #getLookup() Lookup}' containment reference.
*
*
* @see #getLookup()
* @generated
* @ordered
*/
protected LookupFunction lookup;
/**
* The cached value of the '{@link #getDependencies() Dependencies}' containment reference list.
*
*
* @see #getDependencies()
* @generated
* @ordered
*/
protected EList dependencies;
/**
* The cached value of the '{@link #getContextualizers() Contextualizers}' containment reference list.
*
*
* @see #getContextualizers()
* @generated
* @ordered
*/
protected EList contextualizers;
/**
* The cached value of the '{@link #getConcept() Concept}' containment reference.
*
*
* @see #getConcept()
* @generated
* @ordered
*/
protected ConceptDeclaration concept;
/**
* The default value of the '{@link #isDerived() Derived}' attribute.
*
*
* @see #isDerived()
* @generated
* @ordered
*/
protected static final boolean DERIVED_EDEFAULT = false;
/**
* The cached value of the '{@link #isDerived() Derived}' attribute.
*
*
* @see #isDerived()
* @generated
* @ordered
*/
protected boolean derived = DERIVED_EDEFAULT;
/**
* The cached value of the '{@link #getFrom() From}' containment reference.
*
*
* @see #getFrom()
* @generated
* @ordered
*/
protected NUMBER from;
/**
* The cached value of the '{@link #getTo() To}' containment reference.
*
*
* @see #getTo()
* @generated
* @ordered
*/
protected NUMBER to;
/**
* The default value of the '{@link #isInteger() Integer}' attribute.
*
*
* @see #isInteger()
* @generated
* @ordered
*/
protected static final boolean INTEGER_EDEFAULT = false;
/**
* The cached value of the '{@link #isInteger() Integer}' attribute.
*
*
* @see #isInteger()
* @generated
* @ordered
*/
protected boolean integer = INTEGER_EDEFAULT;
/**
* The cached value of the '{@link #getOther() Other}' containment reference.
*
*
* @see #getOther()
* @generated
* @ordered
*/
protected ConceptDeclaration other;
/**
* The default value of the '{@link #getType() Type}' attribute.
*
*
* @see #getType()
* @generated
* @ordered
*/
protected static final String TYPE_EDEFAULT = null;
/**
* The cached value of the '{@link #getType() Type}' attribute.
*
*
* @see #getType()
* @generated
* @ordered
*/
protected String type = TYPE_EDEFAULT;
/**
* The cached value of the '{@link #getDistributionUnit() Distribution Unit}' containment reference.
*
*
* @see #getDistributionUnit()
* @generated
* @ordered
*/
protected Unit distributionUnit;
/**
* The cached value of the '{@link #getCurrency() Currency}' containment reference.
*
*
* @see #getCurrency()
* @generated
* @ordered
*/
protected Currency currency;
/**
* The default value of the '{@link #isDiscretizer() Discretizer}' attribute.
*
*
* @see #isDiscretizer()
* @generated
* @ordered
*/
protected static final boolean DISCRETIZER_EDEFAULT = false;
/**
* The cached value of the '{@link #isDiscretizer() Discretizer}' attribute.
*
*
* @see #isDiscretizer()
* @generated
* @ordered
*/
protected boolean discretizer = DISCRETIZER_EDEFAULT;
/**
* The cached value of the '{@link #getTraits() Traits}' containment reference list.
*
*
* @see #getTraits()
* @generated
* @ordered
*/
protected EList traits;
/**
* The cached value of the '{@link #getClassification() Classification}' containment reference.
*
*
* @see #getClassification()
* @generated
* @ordered
*/
protected Classification classification;
/**
* The default value of the '{@link #getMetadataProperty() Metadata Property}' attribute.
*
*
* @see #getMetadataProperty()
* @generated
* @ordered
*/
protected static final String METADATA_PROPERTY_EDEFAULT = null;
/**
* The cached value of the '{@link #getMetadataProperty() Metadata Property}' attribute.
*
*
* @see #getMetadataProperty()
* @generated
* @ordered
*/
protected String metadataProperty = METADATA_PROPERTY_EDEFAULT;
/**
*
*
* @generated
*/
protected ObserverImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass()
{
return KimPackage.Literals.OBSERVER;
}
/**
*
*
* @generated
*/
public Observable getObservable()
{
return observable;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetObservable(Observable newObservable, NotificationChain msgs)
{
Observable oldObservable = observable;
observable = newObservable;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__OBSERVABLE, oldObservable, newObservable);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setObservable(Observable newObservable)
{
if (newObservable != observable)
{
NotificationChain msgs = null;
if (observable != null)
msgs = ((InternalEObject)observable).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__OBSERVABLE, null, msgs);
if (newObservable != null)
msgs = ((InternalEObject)newObservable).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__OBSERVABLE, null, msgs);
msgs = basicSetObservable(newObservable, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__OBSERVABLE, newObservable, newObservable));
}
/**
*
*
* @generated
*/
public Unit getUnit()
{
return unit;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetUnit(Unit newUnit, NotificationChain msgs)
{
Unit oldUnit = unit;
unit = newUnit;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__UNIT, oldUnit, newUnit);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setUnit(Unit newUnit)
{
if (newUnit != unit)
{
NotificationChain msgs = null;
if (unit != null)
msgs = ((InternalEObject)unit).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__UNIT, null, msgs);
if (newUnit != null)
msgs = ((InternalEObject)newUnit).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__UNIT, null, msgs);
msgs = basicSetUnit(newUnit, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__UNIT, newUnit, newUnit));
}
/**
*
*
* @generated
*/
public TraitDef getTrait()
{
return trait;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetTrait(TraitDef newTrait, NotificationChain msgs)
{
TraitDef oldTrait = trait;
trait = newTrait;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__TRAIT, oldTrait, newTrait);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setTrait(TraitDef newTrait)
{
if (newTrait != trait)
{
NotificationChain msgs = null;
if (trait != null)
msgs = ((InternalEObject)trait).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__TRAIT, null, msgs);
if (newTrait != null)
msgs = ((InternalEObject)newTrait).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__TRAIT, null, msgs);
msgs = basicSetTrait(newTrait, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__TRAIT, newTrait, newTrait));
}
/**
*
*
* @generated
*/
public Classification getDiscretization()
{
return discretization;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetDiscretization(Classification newDiscretization, NotificationChain msgs)
{
Classification oldDiscretization = discretization;
discretization = newDiscretization;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DISCRETIZATION, oldDiscretization, newDiscretization);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setDiscretization(Classification newDiscretization)
{
if (newDiscretization != discretization)
{
NotificationChain msgs = null;
if (discretization != null)
msgs = ((InternalEObject)discretization).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__DISCRETIZATION, null, msgs);
if (newDiscretization != null)
msgs = ((InternalEObject)newDiscretization).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__DISCRETIZATION, null, msgs);
msgs = basicSetDiscretization(newDiscretization, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DISCRETIZATION, newDiscretization, newDiscretization));
}
/**
*
*
* @generated
*/
public Function getAccessor()
{
return accessor;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetAccessor(Function newAccessor, NotificationChain msgs)
{
Function oldAccessor = accessor;
accessor = newAccessor;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__ACCESSOR, oldAccessor, newAccessor);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setAccessor(Function newAccessor)
{
if (newAccessor != accessor)
{
NotificationChain msgs = null;
if (accessor != null)
msgs = ((InternalEObject)accessor).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__ACCESSOR, null, msgs);
if (newAccessor != null)
msgs = ((InternalEObject)newAccessor).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__ACCESSOR, null, msgs);
msgs = basicSetAccessor(newAccessor, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__ACCESSOR, newAccessor, newAccessor));
}
/**
*
*
* @generated
*/
public LookupFunction getLookup()
{
return lookup;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetLookup(LookupFunction newLookup, NotificationChain msgs)
{
LookupFunction oldLookup = lookup;
lookup = newLookup;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__LOOKUP, oldLookup, newLookup);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setLookup(LookupFunction newLookup)
{
if (newLookup != lookup)
{
NotificationChain msgs = null;
if (lookup != null)
msgs = ((InternalEObject)lookup).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__LOOKUP, null, msgs);
if (newLookup != null)
msgs = ((InternalEObject)newLookup).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__LOOKUP, null, msgs);
msgs = basicSetLookup(newLookup, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__LOOKUP, newLookup, newLookup));
}
/**
*
*
* @generated
*/
public EList getDependencies()
{
if (dependencies == null)
{
dependencies = new EObjectContainmentEList(Dependency.class, this, KimPackage.OBSERVER__DEPENDENCIES);
}
return dependencies;
}
/**
*
*
* @generated
*/
public EList getContextualizers()
{
if (contextualizers == null)
{
contextualizers = new EObjectContainmentEList(Contextualization.class, this, KimPackage.OBSERVER__CONTEXTUALIZERS);
}
return contextualizers;
}
/**
*
*
* @generated
*/
public ConceptDeclaration getConcept()
{
return concept;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetConcept(ConceptDeclaration newConcept, NotificationChain msgs)
{
ConceptDeclaration oldConcept = concept;
concept = newConcept;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CONCEPT, oldConcept, newConcept);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setConcept(ConceptDeclaration newConcept)
{
if (newConcept != concept)
{
NotificationChain msgs = null;
if (concept != null)
msgs = ((InternalEObject)concept).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CONCEPT, null, msgs);
if (newConcept != null)
msgs = ((InternalEObject)newConcept).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CONCEPT, null, msgs);
msgs = basicSetConcept(newConcept, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CONCEPT, newConcept, newConcept));
}
/**
*
*
* @generated
*/
public boolean isDerived()
{
return derived;
}
/**
*
*
* @generated
*/
public void setDerived(boolean newDerived)
{
boolean oldDerived = derived;
derived = newDerived;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DERIVED, oldDerived, derived));
}
/**
*
*
* @generated
*/
public NUMBER getFrom()
{
return from;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetFrom(NUMBER newFrom, NotificationChain msgs)
{
NUMBER oldFrom = from;
from = newFrom;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__FROM, oldFrom, newFrom);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setFrom(NUMBER newFrom)
{
if (newFrom != from)
{
NotificationChain msgs = null;
if (from != null)
msgs = ((InternalEObject)from).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__FROM, null, msgs);
if (newFrom != null)
msgs = ((InternalEObject)newFrom).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__FROM, null, msgs);
msgs = basicSetFrom(newFrom, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__FROM, newFrom, newFrom));
}
/**
*
*
* @generated
*/
public NUMBER getTo()
{
return to;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetTo(NUMBER newTo, NotificationChain msgs)
{
NUMBER oldTo = to;
to = newTo;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__TO, oldTo, newTo);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setTo(NUMBER newTo)
{
if (newTo != to)
{
NotificationChain msgs = null;
if (to != null)
msgs = ((InternalEObject)to).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__TO, null, msgs);
if (newTo != null)
msgs = ((InternalEObject)newTo).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__TO, null, msgs);
msgs = basicSetTo(newTo, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__TO, newTo, newTo));
}
/**
*
*
* @generated
*/
public boolean isInteger()
{
return integer;
}
/**
*
*
* @generated
*/
public void setInteger(boolean newInteger)
{
boolean oldInteger = integer;
integer = newInteger;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__INTEGER, oldInteger, integer));
}
/**
*
*
* @generated
*/
public ConceptDeclaration getOther()
{
return other;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetOther(ConceptDeclaration newOther, NotificationChain msgs)
{
ConceptDeclaration oldOther = other;
other = newOther;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__OTHER, oldOther, newOther);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setOther(ConceptDeclaration newOther)
{
if (newOther != other)
{
NotificationChain msgs = null;
if (other != null)
msgs = ((InternalEObject)other).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__OTHER, null, msgs);
if (newOther != null)
msgs = ((InternalEObject)newOther).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__OTHER, null, msgs);
msgs = basicSetOther(newOther, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__OTHER, newOther, newOther));
}
/**
*
*
* @generated
*/
public String getType()
{
return type;
}
/**
*
*
* @generated
*/
public void setType(String newType)
{
String oldType = type;
type = newType;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__TYPE, oldType, type));
}
/**
*
*
* @generated
*/
public Unit getDistributionUnit()
{
return distributionUnit;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetDistributionUnit(Unit newDistributionUnit, NotificationChain msgs)
{
Unit oldDistributionUnit = distributionUnit;
distributionUnit = newDistributionUnit;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DISTRIBUTION_UNIT, oldDistributionUnit, newDistributionUnit);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setDistributionUnit(Unit newDistributionUnit)
{
if (newDistributionUnit != distributionUnit)
{
NotificationChain msgs = null;
if (distributionUnit != null)
msgs = ((InternalEObject)distributionUnit).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__DISTRIBUTION_UNIT, null, msgs);
if (newDistributionUnit != null)
msgs = ((InternalEObject)newDistributionUnit).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__DISTRIBUTION_UNIT, null, msgs);
msgs = basicSetDistributionUnit(newDistributionUnit, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DISTRIBUTION_UNIT, newDistributionUnit, newDistributionUnit));
}
/**
*
*
* @generated
*/
public Currency getCurrency()
{
return currency;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetCurrency(Currency newCurrency, NotificationChain msgs)
{
Currency oldCurrency = currency;
currency = newCurrency;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CURRENCY, oldCurrency, newCurrency);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setCurrency(Currency newCurrency)
{
if (newCurrency != currency)
{
NotificationChain msgs = null;
if (currency != null)
msgs = ((InternalEObject)currency).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CURRENCY, null, msgs);
if (newCurrency != null)
msgs = ((InternalEObject)newCurrency).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CURRENCY, null, msgs);
msgs = basicSetCurrency(newCurrency, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CURRENCY, newCurrency, newCurrency));
}
/**
*
*
* @generated
*/
public boolean isDiscretizer()
{
return discretizer;
}
/**
*
*
* @generated
*/
public void setDiscretizer(boolean newDiscretizer)
{
boolean oldDiscretizer = discretizer;
discretizer = newDiscretizer;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__DISCRETIZER, oldDiscretizer, discretizer));
}
/**
*
*
* @generated
*/
public EList getTraits()
{
if (traits == null)
{
traits = new EObjectContainmentEList(TraitDef.class, this, KimPackage.OBSERVER__TRAITS);
}
return traits;
}
/**
*
*
* @generated
*/
public Classification getClassification()
{
return classification;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetClassification(Classification newClassification, NotificationChain msgs)
{
Classification oldClassification = classification;
classification = newClassification;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CLASSIFICATION, oldClassification, newClassification);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setClassification(Classification newClassification)
{
if (newClassification != classification)
{
NotificationChain msgs = null;
if (classification != null)
msgs = ((InternalEObject)classification).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CLASSIFICATION, null, msgs);
if (newClassification != null)
msgs = ((InternalEObject)newClassification).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.OBSERVER__CLASSIFICATION, null, msgs);
msgs = basicSetClassification(newClassification, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__CLASSIFICATION, newClassification, newClassification));
}
/**
*
*
* @generated
*/
public String getMetadataProperty()
{
return metadataProperty;
}
/**
*
*
* @generated
*/
public void setMetadataProperty(String newMetadataProperty)
{
String oldMetadataProperty = metadataProperty;
metadataProperty = newMetadataProperty;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.OBSERVER__METADATA_PROPERTY, oldMetadataProperty, metadataProperty));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs)
{
switch (featureID)
{
case KimPackage.OBSERVER__OBSERVABLE:
return basicSetObservable(null, msgs);
case KimPackage.OBSERVER__UNIT:
return basicSetUnit(null, msgs);
case KimPackage.OBSERVER__TRAIT:
return basicSetTrait(null, msgs);
case KimPackage.OBSERVER__DISCRETIZATION:
return basicSetDiscretization(null, msgs);
case KimPackage.OBSERVER__ACCESSOR:
return basicSetAccessor(null, msgs);
case KimPackage.OBSERVER__LOOKUP:
return basicSetLookup(null, msgs);
case KimPackage.OBSERVER__DEPENDENCIES:
return ((InternalEList>)getDependencies()).basicRemove(otherEnd, msgs);
case KimPackage.OBSERVER__CONTEXTUALIZERS:
return ((InternalEList>)getContextualizers()).basicRemove(otherEnd, msgs);
case KimPackage.OBSERVER__CONCEPT:
return basicSetConcept(null, msgs);
case KimPackage.OBSERVER__FROM:
return basicSetFrom(null, msgs);
case KimPackage.OBSERVER__TO:
return basicSetTo(null, msgs);
case KimPackage.OBSERVER__OTHER:
return basicSetOther(null, msgs);
case KimPackage.OBSERVER__DISTRIBUTION_UNIT:
return basicSetDistributionUnit(null, msgs);
case KimPackage.OBSERVER__CURRENCY:
return basicSetCurrency(null, msgs);
case KimPackage.OBSERVER__TRAITS:
return ((InternalEList>)getTraits()).basicRemove(otherEnd, msgs);
case KimPackage.OBSERVER__CLASSIFICATION:
return basicSetClassification(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case KimPackage.OBSERVER__OBSERVABLE:
return getObservable();
case KimPackage.OBSERVER__UNIT:
return getUnit();
case KimPackage.OBSERVER__TRAIT:
return getTrait();
case KimPackage.OBSERVER__DISCRETIZATION:
return getDiscretization();
case KimPackage.OBSERVER__ACCESSOR:
return getAccessor();
case KimPackage.OBSERVER__LOOKUP:
return getLookup();
case KimPackage.OBSERVER__DEPENDENCIES:
return getDependencies();
case KimPackage.OBSERVER__CONTEXTUALIZERS:
return getContextualizers();
case KimPackage.OBSERVER__CONCEPT:
return getConcept();
case KimPackage.OBSERVER__DERIVED:
return isDerived();
case KimPackage.OBSERVER__FROM:
return getFrom();
case KimPackage.OBSERVER__TO:
return getTo();
case KimPackage.OBSERVER__INTEGER:
return isInteger();
case KimPackage.OBSERVER__OTHER:
return getOther();
case KimPackage.OBSERVER__TYPE:
return getType();
case KimPackage.OBSERVER__DISTRIBUTION_UNIT:
return getDistributionUnit();
case KimPackage.OBSERVER__CURRENCY:
return getCurrency();
case KimPackage.OBSERVER__DISCRETIZER:
return isDiscretizer();
case KimPackage.OBSERVER__TRAITS:
return getTraits();
case KimPackage.OBSERVER__CLASSIFICATION:
return getClassification();
case KimPackage.OBSERVER__METADATA_PROPERTY:
return getMetadataProperty();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@SuppressWarnings("unchecked")
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case KimPackage.OBSERVER__OBSERVABLE:
setObservable((Observable)newValue);
return;
case KimPackage.OBSERVER__UNIT:
setUnit((Unit)newValue);
return;
case KimPackage.OBSERVER__TRAIT:
setTrait((TraitDef)newValue);
return;
case KimPackage.OBSERVER__DISCRETIZATION:
setDiscretization((Classification)newValue);
return;
case KimPackage.OBSERVER__ACCESSOR:
setAccessor((Function)newValue);
return;
case KimPackage.OBSERVER__LOOKUP:
setLookup((LookupFunction)newValue);
return;
case KimPackage.OBSERVER__DEPENDENCIES:
getDependencies().clear();
getDependencies().addAll((Collection extends Dependency>)newValue);
return;
case KimPackage.OBSERVER__CONTEXTUALIZERS:
getContextualizers().clear();
getContextualizers().addAll((Collection extends Contextualization>)newValue);
return;
case KimPackage.OBSERVER__CONCEPT:
setConcept((ConceptDeclaration)newValue);
return;
case KimPackage.OBSERVER__DERIVED:
setDerived((Boolean)newValue);
return;
case KimPackage.OBSERVER__FROM:
setFrom((NUMBER)newValue);
return;
case KimPackage.OBSERVER__TO:
setTo((NUMBER)newValue);
return;
case KimPackage.OBSERVER__INTEGER:
setInteger((Boolean)newValue);
return;
case KimPackage.OBSERVER__OTHER:
setOther((ConceptDeclaration)newValue);
return;
case KimPackage.OBSERVER__TYPE:
setType((String)newValue);
return;
case KimPackage.OBSERVER__DISTRIBUTION_UNIT:
setDistributionUnit((Unit)newValue);
return;
case KimPackage.OBSERVER__CURRENCY:
setCurrency((Currency)newValue);
return;
case KimPackage.OBSERVER__DISCRETIZER:
setDiscretizer((Boolean)newValue);
return;
case KimPackage.OBSERVER__TRAITS:
getTraits().clear();
getTraits().addAll((Collection extends TraitDef>)newValue);
return;
case KimPackage.OBSERVER__CLASSIFICATION:
setClassification((Classification)newValue);
return;
case KimPackage.OBSERVER__METADATA_PROPERTY:
setMetadataProperty((String)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case KimPackage.OBSERVER__OBSERVABLE:
setObservable((Observable)null);
return;
case KimPackage.OBSERVER__UNIT:
setUnit((Unit)null);
return;
case KimPackage.OBSERVER__TRAIT:
setTrait((TraitDef)null);
return;
case KimPackage.OBSERVER__DISCRETIZATION:
setDiscretization((Classification)null);
return;
case KimPackage.OBSERVER__ACCESSOR:
setAccessor((Function)null);
return;
case KimPackage.OBSERVER__LOOKUP:
setLookup((LookupFunction)null);
return;
case KimPackage.OBSERVER__DEPENDENCIES:
getDependencies().clear();
return;
case KimPackage.OBSERVER__CONTEXTUALIZERS:
getContextualizers().clear();
return;
case KimPackage.OBSERVER__CONCEPT:
setConcept((ConceptDeclaration)null);
return;
case KimPackage.OBSERVER__DERIVED:
setDerived(DERIVED_EDEFAULT);
return;
case KimPackage.OBSERVER__FROM:
setFrom((NUMBER)null);
return;
case KimPackage.OBSERVER__TO:
setTo((NUMBER)null);
return;
case KimPackage.OBSERVER__INTEGER:
setInteger(INTEGER_EDEFAULT);
return;
case KimPackage.OBSERVER__OTHER:
setOther((ConceptDeclaration)null);
return;
case KimPackage.OBSERVER__TYPE:
setType(TYPE_EDEFAULT);
return;
case KimPackage.OBSERVER__DISTRIBUTION_UNIT:
setDistributionUnit((Unit)null);
return;
case KimPackage.OBSERVER__CURRENCY:
setCurrency((Currency)null);
return;
case KimPackage.OBSERVER__DISCRETIZER:
setDiscretizer(DISCRETIZER_EDEFAULT);
return;
case KimPackage.OBSERVER__TRAITS:
getTraits().clear();
return;
case KimPackage.OBSERVER__CLASSIFICATION:
setClassification((Classification)null);
return;
case KimPackage.OBSERVER__METADATA_PROPERTY:
setMetadataProperty(METADATA_PROPERTY_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case KimPackage.OBSERVER__OBSERVABLE:
return observable != null;
case KimPackage.OBSERVER__UNIT:
return unit != null;
case KimPackage.OBSERVER__TRAIT:
return trait != null;
case KimPackage.OBSERVER__DISCRETIZATION:
return discretization != null;
case KimPackage.OBSERVER__ACCESSOR:
return accessor != null;
case KimPackage.OBSERVER__LOOKUP:
return lookup != null;
case KimPackage.OBSERVER__DEPENDENCIES:
return dependencies != null && !dependencies.isEmpty();
case KimPackage.OBSERVER__CONTEXTUALIZERS:
return contextualizers != null && !contextualizers.isEmpty();
case KimPackage.OBSERVER__CONCEPT:
return concept != null;
case KimPackage.OBSERVER__DERIVED:
return derived != DERIVED_EDEFAULT;
case KimPackage.OBSERVER__FROM:
return from != null;
case KimPackage.OBSERVER__TO:
return to != null;
case KimPackage.OBSERVER__INTEGER:
return integer != INTEGER_EDEFAULT;
case KimPackage.OBSERVER__OTHER:
return other != null;
case KimPackage.OBSERVER__TYPE:
return TYPE_EDEFAULT == null ? type != null : !TYPE_EDEFAULT.equals(type);
case KimPackage.OBSERVER__DISTRIBUTION_UNIT:
return distributionUnit != null;
case KimPackage.OBSERVER__CURRENCY:
return currency != null;
case KimPackage.OBSERVER__DISCRETIZER:
return discretizer != DISCRETIZER_EDEFAULT;
case KimPackage.OBSERVER__TRAITS:
return traits != null && !traits.isEmpty();
case KimPackage.OBSERVER__CLASSIFICATION:
return classification != null;
case KimPackage.OBSERVER__METADATA_PROPERTY:
return METADATA_PROPERTY_EDEFAULT == null ? metadataProperty != null : !METADATA_PROPERTY_EDEFAULT.equals(metadataProperty);
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (derived: ");
result.append(derived);
result.append(", integer: ");
result.append(integer);
result.append(", type: ");
result.append(type);
result.append(", discretizer: ");
result.append(discretizer);
result.append(", metadataProperty: ");
result.append(metadataProperty);
result.append(')');
return result.toString();
}
} //ObserverImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy