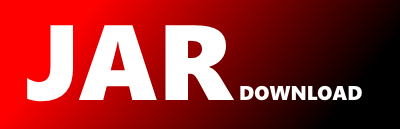
org.integratedmodelling.kim.kim.impl.StateImpl Maven / Gradle / Ivy
/**
* generated by Xtext 2.9.2
*/
package org.integratedmodelling.kim.kim.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
import org.integratedmodelling.kim.kim.Function;
import org.integratedmodelling.kim.kim.KimPackage;
import org.integratedmodelling.kim.kim.Literal;
import org.integratedmodelling.kim.kim.ObservationGenerator;
import org.integratedmodelling.kim.kim.ObserveStatement;
import org.integratedmodelling.kim.kim.State;
/**
*
* An implementation of the model object 'State'.
*
*
* The following features are implemented:
*
*
* - {@link org.integratedmodelling.kim.kim.impl.StateImpl#getLiteral Literal}
* - {@link org.integratedmodelling.kim.kim.impl.StateImpl#getFunction Function}
* - {@link org.integratedmodelling.kim.kim.impl.StateImpl#getObserver Observer}
* - {@link org.integratedmodelling.kim.kim.impl.StateImpl#getObservation Observation}
*
*
* @generated
*/
public class StateImpl extends MinimalEObjectImpl.Container implements State
{
/**
* The cached value of the '{@link #getLiteral() Literal}' containment reference.
*
*
* @see #getLiteral()
* @generated
* @ordered
*/
protected Literal literal;
/**
* The cached value of the '{@link #getFunction() Function}' containment reference.
*
*
* @see #getFunction()
* @generated
* @ordered
*/
protected Function function;
/**
* The cached value of the '{@link #getObserver() Observer}' containment reference.
*
*
* @see #getObserver()
* @generated
* @ordered
*/
protected ObservationGenerator observer;
/**
* The cached value of the '{@link #getObservation() Observation}' containment reference.
*
*
* @see #getObservation()
* @generated
* @ordered
*/
protected ObserveStatement observation;
/**
*
*
* @generated
*/
protected StateImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass()
{
return KimPackage.Literals.STATE;
}
/**
*
*
* @generated
*/
public Literal getLiteral()
{
return literal;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetLiteral(Literal newLiteral, NotificationChain msgs)
{
Literal oldLiteral = literal;
literal = newLiteral;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.STATE__LITERAL, oldLiteral, newLiteral);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setLiteral(Literal newLiteral)
{
if (newLiteral != literal)
{
NotificationChain msgs = null;
if (literal != null)
msgs = ((InternalEObject)literal).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__LITERAL, null, msgs);
if (newLiteral != null)
msgs = ((InternalEObject)newLiteral).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__LITERAL, null, msgs);
msgs = basicSetLiteral(newLiteral, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.STATE__LITERAL, newLiteral, newLiteral));
}
/**
*
*
* @generated
*/
public Function getFunction()
{
return function;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetFunction(Function newFunction, NotificationChain msgs)
{
Function oldFunction = function;
function = newFunction;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.STATE__FUNCTION, oldFunction, newFunction);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setFunction(Function newFunction)
{
if (newFunction != function)
{
NotificationChain msgs = null;
if (function != null)
msgs = ((InternalEObject)function).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__FUNCTION, null, msgs);
if (newFunction != null)
msgs = ((InternalEObject)newFunction).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__FUNCTION, null, msgs);
msgs = basicSetFunction(newFunction, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.STATE__FUNCTION, newFunction, newFunction));
}
/**
*
*
* @generated
*/
public ObservationGenerator getObserver()
{
return observer;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetObserver(ObservationGenerator newObserver, NotificationChain msgs)
{
ObservationGenerator oldObserver = observer;
observer = newObserver;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.STATE__OBSERVER, oldObserver, newObserver);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setObserver(ObservationGenerator newObserver)
{
if (newObserver != observer)
{
NotificationChain msgs = null;
if (observer != null)
msgs = ((InternalEObject)observer).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__OBSERVER, null, msgs);
if (newObserver != null)
msgs = ((InternalEObject)newObserver).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__OBSERVER, null, msgs);
msgs = basicSetObserver(newObserver, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.STATE__OBSERVER, newObserver, newObserver));
}
/**
*
*
* @generated
*/
public ObserveStatement getObservation()
{
return observation;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetObservation(ObserveStatement newObservation, NotificationChain msgs)
{
ObserveStatement oldObservation = observation;
observation = newObservation;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, KimPackage.STATE__OBSERVATION, oldObservation, newObservation);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setObservation(ObserveStatement newObservation)
{
if (newObservation != observation)
{
NotificationChain msgs = null;
if (observation != null)
msgs = ((InternalEObject)observation).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__OBSERVATION, null, msgs);
if (newObservation != null)
msgs = ((InternalEObject)newObservation).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - KimPackage.STATE__OBSERVATION, null, msgs);
msgs = basicSetObservation(newObservation, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, KimPackage.STATE__OBSERVATION, newObservation, newObservation));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs)
{
switch (featureID)
{
case KimPackage.STATE__LITERAL:
return basicSetLiteral(null, msgs);
case KimPackage.STATE__FUNCTION:
return basicSetFunction(null, msgs);
case KimPackage.STATE__OBSERVER:
return basicSetObserver(null, msgs);
case KimPackage.STATE__OBSERVATION:
return basicSetObservation(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case KimPackage.STATE__LITERAL:
return getLiteral();
case KimPackage.STATE__FUNCTION:
return getFunction();
case KimPackage.STATE__OBSERVER:
return getObserver();
case KimPackage.STATE__OBSERVATION:
return getObservation();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case KimPackage.STATE__LITERAL:
setLiteral((Literal)newValue);
return;
case KimPackage.STATE__FUNCTION:
setFunction((Function)newValue);
return;
case KimPackage.STATE__OBSERVER:
setObserver((ObservationGenerator)newValue);
return;
case KimPackage.STATE__OBSERVATION:
setObservation((ObserveStatement)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case KimPackage.STATE__LITERAL:
setLiteral((Literal)null);
return;
case KimPackage.STATE__FUNCTION:
setFunction((Function)null);
return;
case KimPackage.STATE__OBSERVER:
setObserver((ObservationGenerator)null);
return;
case KimPackage.STATE__OBSERVATION:
setObservation((ObserveStatement)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case KimPackage.STATE__LITERAL:
return literal != null;
case KimPackage.STATE__FUNCTION:
return function != null;
case KimPackage.STATE__OBSERVER:
return observer != null;
case KimPackage.STATE__OBSERVATION:
return observation != null;
}
return super.eIsSet(featureID);
}
} //StateImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy