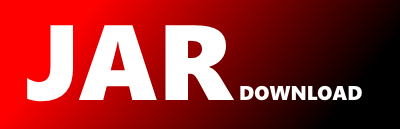
org.integratedmodelling.kim.services.KimGrammarAccess Maven / Gradle / Ivy
/*
* generated by Xtext 2.9.2
*/
package org.integratedmodelling.kim.services;
import com.google.inject.Inject;
import com.google.inject.Singleton;
import java.util.List;
import org.eclipse.xtext.Action;
import org.eclipse.xtext.Alternatives;
import org.eclipse.xtext.Assignment;
import org.eclipse.xtext.EnumLiteralDeclaration;
import org.eclipse.xtext.EnumRule;
import org.eclipse.xtext.Grammar;
import org.eclipse.xtext.GrammarUtil;
import org.eclipse.xtext.Group;
import org.eclipse.xtext.Keyword;
import org.eclipse.xtext.ParserRule;
import org.eclipse.xtext.RuleCall;
import org.eclipse.xtext.TerminalRule;
import org.eclipse.xtext.UnorderedGroup;
import org.eclipse.xtext.common.services.TerminalsGrammarAccess;
import org.eclipse.xtext.service.AbstractElementFinder.AbstractEnumRuleElementFinder;
import org.eclipse.xtext.service.AbstractElementFinder.AbstractGrammarElementFinder;
import org.eclipse.xtext.service.GrammarProvider;
@Singleton
public class KimGrammarAccess extends AbstractGrammarElementFinder {
public class ModelElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Model");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cNamespaceAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cNamespaceNamespaceParserRuleCall_0_0 = (RuleCall)cNamespaceAssignment_0.eContents().get(0);
private final Assignment cStatementsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cStatementsStatementParserRuleCall_1_0 = (RuleCall)cStatementsAssignment_1.eContents().get(0);
//Model:
// namespace=Namespace statements+=Statement*;
@Override public ParserRule getRule() { return rule; }
//namespace=Namespace statements+=Statement*
public Group getGroup() { return cGroup; }
//namespace=Namespace
public Assignment getNamespaceAssignment_0() { return cNamespaceAssignment_0; }
//Namespace
public RuleCall getNamespaceNamespaceParserRuleCall_0_0() { return cNamespaceNamespaceParserRuleCall_0_0; }
//statements+=Statement*
public Assignment getStatementsAssignment_1() { return cStatementsAssignment_1; }
//Statement
public RuleCall getStatementsStatementParserRuleCall_1_0() { return cStatementsStatementParserRuleCall_1_0; }
}
public class ValidIDElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ValidID");
private final RuleCall cIDHTerminalRuleCall = (RuleCall)rule.eContents().get(1);
//ValidID:
// IDH / * (=> ('-'|':'|'.') IDH)* * /;
@Override public ParserRule getRule() { return rule; }
//IDH
public RuleCall getIDHTerminalRuleCall() { return cIDHTerminalRuleCall; }
}
public class MetadataElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Metadata");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cMetadataAction_0 = (Action)cGroup.eContents().get(0);
private final Keyword cLeftCurlyBracketKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Group cGroup_2 = (Group)cGroup.eContents().get(2);
private final Assignment cIdsAssignment_2_0 = (Assignment)cGroup_2.eContents().get(0);
private final RuleCall cIdsValidIDParserRuleCall_2_0_0 = (RuleCall)cIdsAssignment_2_0.eContents().get(0);
private final Assignment cValuesAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final Alternatives cValuesAlternatives_2_1_0 = (Alternatives)cValuesAssignment_2_1.eContents().get(0);
private final RuleCall cValuesLiteralOrIDParserRuleCall_2_1_0_0 = (RuleCall)cValuesAlternatives_2_1_0.eContents().get(0);
private final RuleCall cValuesMetadataParserRuleCall_2_1_0_1 = (RuleCall)cValuesAlternatives_2_1_0.eContents().get(1);
private final RuleCall cValuesListParserRuleCall_2_1_0_2 = (RuleCall)cValuesAlternatives_2_1_0.eContents().get(2);
private final Keyword cRightCurlyBracketKeyword_3 = (Keyword)cGroup.eContents().get(3);
//Metadata:
// {Metadata} '{' (ids+=ValidID values+=(LiteralOrID | Metadata | List))* '}';
@Override public ParserRule getRule() { return rule; }
//{Metadata} '{' (ids+=ValidID values+=(LiteralOrID | Metadata | List))* '}'
public Group getGroup() { return cGroup; }
//{Metadata}
public Action getMetadataAction_0() { return cMetadataAction_0; }
//'{'
public Keyword getLeftCurlyBracketKeyword_1() { return cLeftCurlyBracketKeyword_1; }
//(ids+=ValidID values+=(LiteralOrID | Metadata | List))*
public Group getGroup_2() { return cGroup_2; }
//ids+=ValidID
public Assignment getIdsAssignment_2_0() { return cIdsAssignment_2_0; }
//ValidID
public RuleCall getIdsValidIDParserRuleCall_2_0_0() { return cIdsValidIDParserRuleCall_2_0_0; }
//values+=(LiteralOrID | Metadata | List)
public Assignment getValuesAssignment_2_1() { return cValuesAssignment_2_1; }
//(LiteralOrID | Metadata | List)
public Alternatives getValuesAlternatives_2_1_0() { return cValuesAlternatives_2_1_0; }
//LiteralOrID
public RuleCall getValuesLiteralOrIDParserRuleCall_2_1_0_0() { return cValuesLiteralOrIDParserRuleCall_2_1_0_0; }
//Metadata
public RuleCall getValuesMetadataParserRuleCall_2_1_0_1() { return cValuesMetadataParserRuleCall_2_1_0_1; }
//List
public RuleCall getValuesListParserRuleCall_2_1_0_2() { return cValuesListParserRuleCall_2_1_0_2; }
//'}'
public Keyword getRightCurlyBracketKeyword_3() { return cRightCurlyBracketKeyword_3; }
}
public class NUMBERElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.NUMBER");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cIntAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cIntINTTerminalRuleCall_0_0 = (RuleCall)cIntAssignment_0.eContents().get(0);
private final Assignment cSintAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cSintSINTTerminalRuleCall_1_0 = (RuleCall)cSintAssignment_1.eContents().get(0);
private final Assignment cFloatAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final RuleCall cFloatGNUMBERTerminalRuleCall_2_0 = (RuleCall)cFloatAssignment_2.eContents().get(0);
//NUMBER:
// int=INT | sint=SINT | float=GNUMBER;
@Override public ParserRule getRule() { return rule; }
//int=INT | sint=SINT | float=GNUMBER
public Alternatives getAlternatives() { return cAlternatives; }
//int=INT
public Assignment getIntAssignment_0() { return cIntAssignment_0; }
//INT
public RuleCall getIntINTTerminalRuleCall_0_0() { return cIntINTTerminalRuleCall_0_0; }
//sint=SINT
public Assignment getSintAssignment_1() { return cSintAssignment_1; }
//SINT
public RuleCall getSintSINTTerminalRuleCall_1_0() { return cSintSINTTerminalRuleCall_1_0; }
//float=GNUMBER
public Assignment getFloatAssignment_2() { return cFloatAssignment_2; }
//GNUMBER
public RuleCall getFloatGNUMBERTerminalRuleCall_2_0() { return cFloatGNUMBERTerminalRuleCall_2_0; }
}
public class ListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.List");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cListAction_0 = (Action)cGroup.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cContentsAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final Alternatives cContentsAlternatives_2_0 = (Alternatives)cContentsAssignment_2.eContents().get(0);
private final RuleCall cContentsLiteralOrIDOrListKWParserRuleCall_2_0_0 = (RuleCall)cContentsAlternatives_2_0.eContents().get(0);
private final RuleCall cContentsListParserRuleCall_2_0_1 = (RuleCall)cContentsAlternatives_2_0.eContents().get(1);
private final Keyword cRightParenthesisKeyword_3 = (Keyword)cGroup.eContents().get(3);
//List:
// {List} '(' contents+=(LiteralOrIDOrListKW | List)* ')';
@Override public ParserRule getRule() { return rule; }
//{List} '(' contents+=(LiteralOrIDOrListKW | List)* ')'
public Group getGroup() { return cGroup; }
//{List}
public Action getListAction_0() { return cListAction_0; }
//'('
public Keyword getLeftParenthesisKeyword_1() { return cLeftParenthesisKeyword_1; }
//contents+=(LiteralOrIDOrListKW | List)*
public Assignment getContentsAssignment_2() { return cContentsAssignment_2; }
//(LiteralOrIDOrListKW | List)
public Alternatives getContentsAlternatives_2_0() { return cContentsAlternatives_2_0; }
//LiteralOrIDOrListKW
public RuleCall getContentsLiteralOrIDOrListKWParserRuleCall_2_0_0() { return cContentsLiteralOrIDOrListKWParserRuleCall_2_0_0; }
//List
public RuleCall getContentsListParserRuleCall_2_0_1() { return cContentsListParserRuleCall_2_0_1; }
//')'
public Keyword getRightParenthesisKeyword_3() { return cRightParenthesisKeyword_3; }
}
public class LiteralElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Literal");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cNumberAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cNumberNUMBERParserRuleCall_0_0 = (RuleCall)cNumberAssignment_0.eContents().get(0);
private final Assignment cStringAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cStringSTRINGTerminalRuleCall_1_0 = (RuleCall)cStringAssignment_1.eContents().get(0);
private final Assignment cBooleanAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final Alternatives cBooleanAlternatives_2_0 = (Alternatives)cBooleanAssignment_2.eContents().get(0);
private final Keyword cBooleanTrueKeyword_2_0_0 = (Keyword)cBooleanAlternatives_2_0.eContents().get(0);
private final Keyword cBooleanFalseKeyword_2_0_1 = (Keyword)cBooleanAlternatives_2_0.eContents().get(1);
//Literal:
// number=NUMBER | string=STRING | boolean=('true' | 'false');
@Override public ParserRule getRule() { return rule; }
//number=NUMBER | string=STRING | boolean=('true' | 'false')
public Alternatives getAlternatives() { return cAlternatives; }
//number=NUMBER
public Assignment getNumberAssignment_0() { return cNumberAssignment_0; }
//NUMBER
public RuleCall getNumberNUMBERParserRuleCall_0_0() { return cNumberNUMBERParserRuleCall_0_0; }
//string=STRING
public Assignment getStringAssignment_1() { return cStringAssignment_1; }
//STRING
public RuleCall getStringSTRINGTerminalRuleCall_1_0() { return cStringSTRINGTerminalRuleCall_1_0; }
//boolean=('true' | 'false')
public Assignment getBooleanAssignment_2() { return cBooleanAssignment_2; }
//('true' | 'false')
public Alternatives getBooleanAlternatives_2_0() { return cBooleanAlternatives_2_0; }
//'true'
public Keyword getBooleanTrueKeyword_2_0_0() { return cBooleanTrueKeyword_2_0_0; }
//'false'
public Keyword getBooleanFalseKeyword_2_0_1() { return cBooleanFalseKeyword_2_0_1; }
}
public class LiteralOrIDOrListKWElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.LiteralOrIDOrListKW");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cNumberAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cNumberNUMBERParserRuleCall_0_0 = (RuleCall)cNumberAssignment_0.eContents().get(0);
private final Assignment cStringAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cStringSTRINGTerminalRuleCall_1_0 = (RuleCall)cStringAssignment_1.eContents().get(0);
private final Assignment cBooleanAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final Alternatives cBooleanAlternatives_2_0 = (Alternatives)cBooleanAssignment_2.eContents().get(0);
private final Keyword cBooleanTrueKeyword_2_0_0 = (Keyword)cBooleanAlternatives_2_0.eContents().get(0);
private final Keyword cBooleanFalseKeyword_2_0_1 = (Keyword)cBooleanAlternatives_2_0.eContents().get(1);
private final Group cGroup_3 = (Group)cAlternatives.eContents().get(3);
private final Assignment cFromAssignment_3_0 = (Assignment)cGroup_3.eContents().get(0);
private final RuleCall cFromNUMBERParserRuleCall_3_0_0 = (RuleCall)cFromAssignment_3_0.eContents().get(0);
private final Keyword cToKeyword_3_1 = (Keyword)cGroup_3.eContents().get(1);
private final Assignment cToAssignment_3_2 = (Assignment)cGroup_3.eContents().get(2);
private final RuleCall cToNUMBERParserRuleCall_3_2_0 = (RuleCall)cToAssignment_3_2.eContents().get(0);
private final Assignment cIdAssignment_4 = (Assignment)cAlternatives.eContents().get(4);
private final RuleCall cIdValidIDParserRuleCall_4_0 = (RuleCall)cIdAssignment_4.eContents().get(0);
private final Assignment cIdAssignment_5 = (Assignment)cAlternatives.eContents().get(5);
private final Keyword cIdAsKeyword_5_0 = (Keyword)cIdAssignment_5.eContents().get(0);
private final Assignment cCommaAssignment_6 = (Assignment)cAlternatives.eContents().get(6);
private final Keyword cCommaCommaKeyword_6_0 = (Keyword)cCommaAssignment_6.eContents().get(0);
//LiteralOrIDOrListKW Literal:
// number=NUMBER | string=STRING | boolean=('true' | 'false') | from=NUMBER 'to' to=NUMBER | id=ValidID | id='as' |
// comma?=','
@Override public ParserRule getRule() { return rule; }
//number=NUMBER | string=STRING | boolean=('true' | 'false') | from=NUMBER 'to' to=NUMBER | id=ValidID | id='as' |
//comma?=','
public Alternatives getAlternatives() { return cAlternatives; }
//number=NUMBER
public Assignment getNumberAssignment_0() { return cNumberAssignment_0; }
//NUMBER
public RuleCall getNumberNUMBERParserRuleCall_0_0() { return cNumberNUMBERParserRuleCall_0_0; }
//string=STRING
public Assignment getStringAssignment_1() { return cStringAssignment_1; }
//STRING
public RuleCall getStringSTRINGTerminalRuleCall_1_0() { return cStringSTRINGTerminalRuleCall_1_0; }
//boolean=('true' | 'false')
public Assignment getBooleanAssignment_2() { return cBooleanAssignment_2; }
//('true' | 'false')
public Alternatives getBooleanAlternatives_2_0() { return cBooleanAlternatives_2_0; }
//'true'
public Keyword getBooleanTrueKeyword_2_0_0() { return cBooleanTrueKeyword_2_0_0; }
//'false'
public Keyword getBooleanFalseKeyword_2_0_1() { return cBooleanFalseKeyword_2_0_1; }
//from=NUMBER 'to' to=NUMBER
public Group getGroup_3() { return cGroup_3; }
//from=NUMBER
public Assignment getFromAssignment_3_0() { return cFromAssignment_3_0; }
//NUMBER
public RuleCall getFromNUMBERParserRuleCall_3_0_0() { return cFromNUMBERParserRuleCall_3_0_0; }
//'to'
public Keyword getToKeyword_3_1() { return cToKeyword_3_1; }
//to=NUMBER
public Assignment getToAssignment_3_2() { return cToAssignment_3_2; }
//NUMBER
public RuleCall getToNUMBERParserRuleCall_3_2_0() { return cToNUMBERParserRuleCall_3_2_0; }
//id=ValidID
public Assignment getIdAssignment_4() { return cIdAssignment_4; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_4_0() { return cIdValidIDParserRuleCall_4_0; }
//id='as'
public Assignment getIdAssignment_5() { return cIdAssignment_5; }
//'as'
public Keyword getIdAsKeyword_5_0() { return cIdAsKeyword_5_0; }
//comma?=','
public Assignment getCommaAssignment_6() { return cCommaAssignment_6; }
//','
public Keyword getCommaCommaKeyword_6_0() { return cCommaCommaKeyword_6_0; }
}
public class LiteralOrIDElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.LiteralOrID");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cNumberAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cNumberNUMBERParserRuleCall_0_0 = (RuleCall)cNumberAssignment_0.eContents().get(0);
private final Assignment cStringAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cStringSTRINGTerminalRuleCall_1_0 = (RuleCall)cStringAssignment_1.eContents().get(0);
private final Assignment cBooleanAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final Alternatives cBooleanAlternatives_2_0 = (Alternatives)cBooleanAssignment_2.eContents().get(0);
private final Keyword cBooleanTrueKeyword_2_0_0 = (Keyword)cBooleanAlternatives_2_0.eContents().get(0);
private final Keyword cBooleanFalseKeyword_2_0_1 = (Keyword)cBooleanAlternatives_2_0.eContents().get(1);
private final Assignment cIdAssignment_3 = (Assignment)cAlternatives.eContents().get(3);
private final RuleCall cIdValidIDParserRuleCall_3_0 = (RuleCall)cIdAssignment_3.eContents().get(0);
//LiteralOrID Literal:
// number=NUMBER | string=STRING | boolean=('true' | 'false') | id=ValidID
@Override public ParserRule getRule() { return rule; }
//number=NUMBER | string=STRING | boolean=('true' | 'false') | id=ValidID
public Alternatives getAlternatives() { return cAlternatives; }
//number=NUMBER
public Assignment getNumberAssignment_0() { return cNumberAssignment_0; }
//NUMBER
public RuleCall getNumberNUMBERParserRuleCall_0_0() { return cNumberNUMBERParserRuleCall_0_0; }
//string=STRING
public Assignment getStringAssignment_1() { return cStringAssignment_1; }
//STRING
public RuleCall getStringSTRINGTerminalRuleCall_1_0() { return cStringSTRINGTerminalRuleCall_1_0; }
//boolean=('true' | 'false')
public Assignment getBooleanAssignment_2() { return cBooleanAssignment_2; }
//('true' | 'false')
public Alternatives getBooleanAlternatives_2_0() { return cBooleanAlternatives_2_0; }
//'true'
public Keyword getBooleanTrueKeyword_2_0_0() { return cBooleanTrueKeyword_2_0_0; }
//'false'
public Keyword getBooleanFalseKeyword_2_0_1() { return cBooleanFalseKeyword_2_0_1; }
//id=ValidID
public Assignment getIdAssignment_3() { return cIdAssignment_3; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_3_0() { return cIdValidIDParserRuleCall_3_0; }
}
public class REL_OPERATORElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.REL_OPERATOR");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cGtAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final Keyword cGtGreaterThanSignKeyword_0_0 = (Keyword)cGtAssignment_0.eContents().get(0);
private final Assignment cLtAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final Keyword cLtLessThanSignKeyword_1_0 = (Keyword)cLtAssignment_1.eContents().get(0);
private final Assignment cEqAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final Keyword cEqEqualsSignKeyword_2_0 = (Keyword)cEqAssignment_2.eContents().get(0);
private final Assignment cNeAssignment_3 = (Assignment)cAlternatives.eContents().get(3);
private final Keyword cNeExclamationMarkEqualsSignKeyword_3_0 = (Keyword)cNeAssignment_3.eContents().get(0);
private final Assignment cLeAssignment_4 = (Assignment)cAlternatives.eContents().get(4);
private final Keyword cLeLessThanSignEqualsSignKeyword_4_0 = (Keyword)cLeAssignment_4.eContents().get(0);
private final Assignment cGeAssignment_5 = (Assignment)cAlternatives.eContents().get(5);
private final Keyword cGeGreaterThanSignEqualsSignKeyword_5_0 = (Keyword)cGeAssignment_5.eContents().get(0);
/// *
// * enums
// * / REL_OPERATOR:
// gt?='>' | lt?='<' | eq?='=' | ne?='!=' | le?='<=' | ge?='>=';
@Override public ParserRule getRule() { return rule; }
//gt?='>' | lt?='<' | eq?='=' | ne?='!=' | le?='<=' | ge?='>='
public Alternatives getAlternatives() { return cAlternatives; }
//gt?='>'
public Assignment getGtAssignment_0() { return cGtAssignment_0; }
//'>'
public Keyword getGtGreaterThanSignKeyword_0_0() { return cGtGreaterThanSignKeyword_0_0; }
//lt?='<'
public Assignment getLtAssignment_1() { return cLtAssignment_1; }
//'<'
public Keyword getLtLessThanSignKeyword_1_0() { return cLtLessThanSignKeyword_1_0; }
//eq?='='
public Assignment getEqAssignment_2() { return cEqAssignment_2; }
//'='
public Keyword getEqEqualsSignKeyword_2_0() { return cEqEqualsSignKeyword_2_0; }
//ne?='!='
public Assignment getNeAssignment_3() { return cNeAssignment_3; }
//'!='
public Keyword getNeExclamationMarkEqualsSignKeyword_3_0() { return cNeExclamationMarkEqualsSignKeyword_3_0; }
//le?='<='
public Assignment getLeAssignment_4() { return cLeAssignment_4; }
//'<='
public Keyword getLeLessThanSignEqualsSignKeyword_4_0() { return cLeLessThanSignEqualsSignKeyword_4_0; }
//ge?='>='
public Assignment getGeAssignment_5() { return cGeAssignment_5; }
//'>='
public Keyword getGeGreaterThanSignEqualsSignKeyword_5_0() { return cGeGreaterThanSignEqualsSignKeyword_5_0; }
}
public class KeyValuePairElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.KeyValuePair");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cKeyAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cKeyValidIDParserRuleCall_0_0 = (RuleCall)cKeyAssignment_0.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Assignment cInteractiveAssignment_1_0 = (Assignment)cAlternatives_1.eContents().get(0);
private final Keyword cInteractiveQuestionMarkEqualsSignKeyword_1_0_0 = (Keyword)cInteractiveAssignment_1_0.eContents().get(0);
private final Keyword cEqualsSignKeyword_1_1 = (Keyword)cAlternatives_1.eContents().get(1);
private final Assignment cValueAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cValueValueParserRuleCall_2_0 = (RuleCall)cValueAssignment_2.eContents().get(0);
//KeyValuePair:
// key=ValidID (interactive?='?=' | '=') value=Value;
@Override public ParserRule getRule() { return rule; }
//key=ValidID (interactive?='?=' | '=') value=Value
public Group getGroup() { return cGroup; }
//key=ValidID
public Assignment getKeyAssignment_0() { return cKeyAssignment_0; }
//ValidID
public RuleCall getKeyValidIDParserRuleCall_0_0() { return cKeyValidIDParserRuleCall_0_0; }
//(interactive?='?=' | '=')
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//interactive?='?='
public Assignment getInteractiveAssignment_1_0() { return cInteractiveAssignment_1_0; }
//'?='
public Keyword getInteractiveQuestionMarkEqualsSignKeyword_1_0_0() { return cInteractiveQuestionMarkEqualsSignKeyword_1_0_0; }
//'='
public Keyword getEqualsSignKeyword_1_1() { return cEqualsSignKeyword_1_1; }
//value=Value
public Assignment getValueAssignment_2() { return cValueAssignment_2; }
//Value
public RuleCall getValueValueParserRuleCall_2_0() { return cValueValueParserRuleCall_2_0; }
}
public class ParameterListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ParameterList");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cSingleValueAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cSingleValueValueParserRuleCall_0_0 = (RuleCall)cSingleValueAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cPairsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final RuleCall cPairsKeyValuePairParserRuleCall_1_0_0 = (RuleCall)cPairsAssignment_1_0.eContents().get(0);
private final Group cGroup_1_1 = (Group)cGroup_1.eContents().get(1);
private final Keyword cCommaKeyword_1_1_0 = (Keyword)cGroup_1_1.eContents().get(0);
private final Assignment cPairsAssignment_1_1_1 = (Assignment)cGroup_1_1.eContents().get(1);
private final RuleCall cPairsKeyValuePairParserRuleCall_1_1_1_0 = (RuleCall)cPairsAssignment_1_1_1.eContents().get(0);
//ParameterList:
// singleValue=Value | pairs+=KeyValuePair (=> ',' pairs+=KeyValuePair)*;
@Override public ParserRule getRule() { return rule; }
//singleValue=Value | pairs+=KeyValuePair (=> ',' pairs+=KeyValuePair)*
public Alternatives getAlternatives() { return cAlternatives; }
//singleValue=Value
public Assignment getSingleValueAssignment_0() { return cSingleValueAssignment_0; }
//Value
public RuleCall getSingleValueValueParserRuleCall_0_0() { return cSingleValueValueParserRuleCall_0_0; }
//pairs+=KeyValuePair (=> ',' pairs+=KeyValuePair)*
public Group getGroup_1() { return cGroup_1; }
//pairs+=KeyValuePair
public Assignment getPairsAssignment_1_0() { return cPairsAssignment_1_0; }
//KeyValuePair
public RuleCall getPairsKeyValuePairParserRuleCall_1_0_0() { return cPairsKeyValuePairParserRuleCall_1_0_0; }
//(=> ',' pairs+=KeyValuePair)*
public Group getGroup_1_1() { return cGroup_1_1; }
//=> ','
public Keyword getCommaKeyword_1_1_0() { return cCommaKeyword_1_1_0; }
//pairs+=KeyValuePair
public Assignment getPairsAssignment_1_1_1() { return cPairsAssignment_1_1_1; }
//KeyValuePair
public RuleCall getPairsKeyValuePairParserRuleCall_1_1_1_0() { return cPairsKeyValuePairParserRuleCall_1_1_1_0; }
}
public class UnitElementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.UnitElement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cIdAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cIdValidIDParserRuleCall_0_0 = (RuleCall)cIdAssignment_0.eContents().get(0);
private final Assignment cNumAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cNumNUMBERParserRuleCall_1_0 = (RuleCall)cNumAssignment_1.eContents().get(0);
private final Group cGroup_2 = (Group)cAlternatives.eContents().get(2);
private final Keyword cLeftParenthesisKeyword_2_0 = (Keyword)cGroup_2.eContents().get(0);
private final Assignment cUnitAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cUnitUnitParserRuleCall_2_1_0 = (RuleCall)cUnitAssignment_2_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_2_2 = (Keyword)cGroup_2.eContents().get(2);
/// *
// * ---------------------------------------
// * Unit of measurement re: isr-108, parseable by Java
// * TODO implement expression syntax and provide validator
// * ---------------------------------------
// * /
//UnitElement:
// id=ValidID | num=NUMBER | '(' unit=Unit ')';
@Override public ParserRule getRule() { return rule; }
//id=ValidID | num=NUMBER | '(' unit=Unit ')'
public Alternatives getAlternatives() { return cAlternatives; }
//id=ValidID
public Assignment getIdAssignment_0() { return cIdAssignment_0; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_0_0() { return cIdValidIDParserRuleCall_0_0; }
//num=NUMBER
public Assignment getNumAssignment_1() { return cNumAssignment_1; }
//NUMBER
public RuleCall getNumNUMBERParserRuleCall_1_0() { return cNumNUMBERParserRuleCall_1_0; }
//'(' unit=Unit ')'
public Group getGroup_2() { return cGroup_2; }
//'('
public Keyword getLeftParenthesisKeyword_2_0() { return cLeftParenthesisKeyword_2_0; }
//unit=Unit
public Assignment getUnitAssignment_2_1() { return cUnitAssignment_2_1; }
//Unit
public RuleCall getUnitUnitParserRuleCall_2_1_0() { return cUnitUnitParserRuleCall_2_1_0; }
//')'
public Keyword getRightParenthesisKeyword_2_2() { return cRightParenthesisKeyword_2_2; }
}
public class UnitElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Unit");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Action cUnitAction_0 = (Action)cGroup.eContents().get(0);
private final Assignment cRootAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cRootUnitElementParserRuleCall_1_0 = (RuleCall)cRootAssignment_1.eContents().get(0);
private final Group cGroup_2 = (Group)cGroup.eContents().get(2);
private final Group cGroup_2_0 = (Group)cGroup_2.eContents().get(0);
private final Assignment cConnectorsAssignment_2_0_0 = (Assignment)cGroup_2_0.eContents().get(0);
private final RuleCall cConnectorsUnitOpEnumRuleCall_2_0_0_0 = (RuleCall)cConnectorsAssignment_2_0_0.eContents().get(0);
private final Assignment cUnitsAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cUnitsUnitElementParserRuleCall_2_1_0 = (RuleCall)cUnitsAssignment_2_1.eContents().get(0);
//Unit:
// {Unit} root=UnitElement? (=> (connectors+=UnitOp) units+=UnitElement)*;
@Override public ParserRule getRule() { return rule; }
//{Unit} root=UnitElement? (=> (connectors+=UnitOp) units+=UnitElement)*
public Group getGroup() { return cGroup; }
//{Unit}
public Action getUnitAction_0() { return cUnitAction_0; }
//root=UnitElement?
public Assignment getRootAssignment_1() { return cRootAssignment_1; }
//UnitElement
public RuleCall getRootUnitElementParserRuleCall_1_0() { return cRootUnitElementParserRuleCall_1_0; }
//(=> (connectors+=UnitOp) units+=UnitElement)*
public Group getGroup_2() { return cGroup_2; }
//=> (connectors+=UnitOp)
public Group getGroup_2_0() { return cGroup_2_0; }
//connectors+=UnitOp
public Assignment getConnectorsAssignment_2_0_0() { return cConnectorsAssignment_2_0_0; }
//UnitOp
public RuleCall getConnectorsUnitOpEnumRuleCall_2_0_0_0() { return cConnectorsUnitOpEnumRuleCall_2_0_0_0; }
//units+=UnitElement
public Assignment getUnitsAssignment_2_1() { return cUnitsAssignment_2_1; }
//UnitElement
public RuleCall getUnitsUnitElementParserRuleCall_2_1_0() { return cUnitsUnitElementParserRuleCall_2_1_0; }
}
public class CurrencyElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Currency");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cIdAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final RuleCall cIdIDTerminalRuleCall_0_0_0 = (RuleCall)cIdAssignment_0_0.eContents().get(0);
private final Group cGroup_0_1 = (Group)cGroup_0.eContents().get(1);
private final Keyword cCommercialAtKeyword_0_1_0 = (Keyword)cGroup_0_1.eContents().get(0);
private final Assignment cYearAssignment_0_1_1 = (Assignment)cGroup_0_1.eContents().get(1);
private final RuleCall cYearINTTerminalRuleCall_0_1_1_0 = (RuleCall)cYearAssignment_0_1_1.eContents().get(0);
private final Assignment cConceptAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cConceptValidIDParserRuleCall_1_0 = (RuleCall)cConceptAssignment_1.eContents().get(0);
//Currency:
// id=ID ('@' year=INT) | concept=ValidID;
@Override public ParserRule getRule() { return rule; }
//id=ID ('@' year=INT) | concept=ValidID
public Alternatives getAlternatives() { return cAlternatives; }
//id=ID ('@' year=INT)
public Group getGroup_0() { return cGroup_0; }
//id=ID
public Assignment getIdAssignment_0_0() { return cIdAssignment_0_0; }
//ID
public RuleCall getIdIDTerminalRuleCall_0_0_0() { return cIdIDTerminalRuleCall_0_0_0; }
//('@' year=INT)
public Group getGroup_0_1() { return cGroup_0_1; }
//'@'
public Keyword getCommercialAtKeyword_0_1_0() { return cCommercialAtKeyword_0_1_0; }
//year=INT
public Assignment getYearAssignment_0_1_1() { return cYearAssignment_0_1_1; }
//INT
public RuleCall getYearINTTerminalRuleCall_0_1_1_0() { return cYearINTTerminalRuleCall_0_1_1_0; }
//concept=ValidID
public Assignment getConceptAssignment_1() { return cConceptAssignment_1; }
//ValidID
public RuleCall getConceptValidIDParserRuleCall_1_0() { return cConceptValidIDParserRuleCall_1_0; }
}
public class ValueElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Value");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cLiteralAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cLiteralLiteralParserRuleCall_0_0 = (RuleCall)cLiteralAssignment_0.eContents().get(0);
private final Assignment cFunctionAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cFunctionFunctionParserRuleCall_1_0 = (RuleCall)cFunctionAssignment_1.eContents().get(0);
private final Assignment cExprAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final RuleCall cExprEXPRTerminalRuleCall_2_0 = (RuleCall)cExprAssignment_2.eContents().get(0);
private final Assignment cIdAssignment_3 = (Assignment)cAlternatives.eContents().get(3);
private final RuleCall cIdValidIDParserRuleCall_3_0 = (RuleCall)cIdAssignment_3.eContents().get(0);
private final Assignment cListAssignment_4 = (Assignment)cAlternatives.eContents().get(4);
private final RuleCall cListListParserRuleCall_4_0 = (RuleCall)cListAssignment_4.eContents().get(0);
private final Assignment cNullAssignment_5 = (Assignment)cAlternatives.eContents().get(5);
private final Keyword cNullUnknownKeyword_5_0 = (Keyword)cNullAssignment_5.eContents().get(0);
//Value:
// literal=Literal | function=Function | expr=EXPR | id=ValidID | list=List | null?='unknown';
@Override public ParserRule getRule() { return rule; }
//literal=Literal | function=Function | expr=EXPR | id=ValidID | list=List | null?='unknown'
public Alternatives getAlternatives() { return cAlternatives; }
//literal=Literal
public Assignment getLiteralAssignment_0() { return cLiteralAssignment_0; }
//Literal
public RuleCall getLiteralLiteralParserRuleCall_0_0() { return cLiteralLiteralParserRuleCall_0_0; }
//function=Function
public Assignment getFunctionAssignment_1() { return cFunctionAssignment_1; }
//Function
public RuleCall getFunctionFunctionParserRuleCall_1_0() { return cFunctionFunctionParserRuleCall_1_0; }
//expr=EXPR
public Assignment getExprAssignment_2() { return cExprAssignment_2; }
//EXPR
public RuleCall getExprEXPRTerminalRuleCall_2_0() { return cExprEXPRTerminalRuleCall_2_0; }
//id=ValidID
public Assignment getIdAssignment_3() { return cIdAssignment_3; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_3_0() { return cIdValidIDParserRuleCall_3_0; }
//list=List
public Assignment getListAssignment_4() { return cListAssignment_4; }
//List
public RuleCall getListListParserRuleCall_4_0() { return cListListParserRuleCall_4_0; }
//null?='unknown'
public Assignment getNullAssignment_5() { return cNullAssignment_5; }
//'unknown'
public Keyword getNullUnknownKeyword_5_0() { return cNullUnknownKeyword_5_0; }
}
public class CONCEPTElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.CONCEPT");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Keyword cQualityKeyword_0 = (Keyword)cAlternatives.eContents().get(0);
private final Keyword cClassKeyword_1 = (Keyword)cAlternatives.eContents().get(1);
private final Keyword cQuantityKeyword_2 = (Keyword)cAlternatives.eContents().get(2);
private final Keyword cConfigurationKeyword_3 = (Keyword)cAlternatives.eContents().get(3);
private final RuleCall cEXTENSIVE_PROPERTYParserRuleCall_4 = (RuleCall)cAlternatives.eContents().get(4);
private final RuleCall cINTENSIVE_PROPERTYParserRuleCall_5 = (RuleCall)cAlternatives.eContents().get(5);
private final RuleCall cAGENT_TYPEParserRuleCall_6 = (RuleCall)cAlternatives.eContents().get(6);
private final RuleCall cTRAITParserRuleCall_7 = (RuleCall)cAlternatives.eContents().get(7);
/// **
// * Carefully selected keywords to define a concept, which enable automatic definition of
// * fairly complex semantics and validation against upper ontologies. For now implement
// * physical properties, value concepts and basic agent types re: DOLCE types.
// *
// * A similar thing could be done for properties
// *
// * This is a lot of keywords, although it's probably good to protect these names from
// * being used as identifiers in anything.
// * /
//CONCEPT:
// 'quality' | 'class' | 'quantity' | 'configuration' | EXTENSIVE_PROPERTY | INTENSIVE_PROPERTY | AGENT_TYPE | TRAIT;
@Override public ParserRule getRule() { return rule; }
//'quality' | 'class' | 'quantity' | 'configuration' | EXTENSIVE_PROPERTY | INTENSIVE_PROPERTY | AGENT_TYPE | TRAIT
public Alternatives getAlternatives() { return cAlternatives; }
//'quality'
public Keyword getQualityKeyword_0() { return cQualityKeyword_0; }
//'class'
public Keyword getClassKeyword_1() { return cClassKeyword_1; }
//'quantity'
public Keyword getQuantityKeyword_2() { return cQuantityKeyword_2; }
//'configuration'
public Keyword getConfigurationKeyword_3() { return cConfigurationKeyword_3; }
//EXTENSIVE_PROPERTY
public RuleCall getEXTENSIVE_PROPERTYParserRuleCall_4() { return cEXTENSIVE_PROPERTYParserRuleCall_4; }
//INTENSIVE_PROPERTY
public RuleCall getINTENSIVE_PROPERTYParserRuleCall_5() { return cINTENSIVE_PROPERTYParserRuleCall_5; }
//AGENT_TYPE
public RuleCall getAGENT_TYPEParserRuleCall_6() { return cAGENT_TYPEParserRuleCall_6; }
//TRAIT
public RuleCall getTRAITParserRuleCall_7() { return cTRAITParserRuleCall_7; }
}
public class TRAITElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.TRAIT");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Keyword cOrderingKeyword_0 = (Keyword)cAlternatives.eContents().get(0);
private final Keyword cAttributeKeyword_1 = (Keyword)cAlternatives.eContents().get(1);
private final Keyword cIdentityKeyword_2 = (Keyword)cAlternatives.eContents().get(2);
private final Keyword cRoleKeyword_3 = (Keyword)cAlternatives.eContents().get(3);
private final Keyword cRealmKeyword_4 = (Keyword)cAlternatives.eContents().get(4);
private final Keyword cDomainKeyword_5 = (Keyword)cAlternatives.eContents().get(5);
//TRAIT:
// 'ordering' | 'attribute' | 'identity' | 'role' | 'realm' | 'domain';
@Override public ParserRule getRule() { return rule; }
//'ordering' | 'attribute' | 'identity' | 'role' | 'realm' | 'domain'
public Alternatives getAlternatives() { return cAlternatives; }
//'ordering'
public Keyword getOrderingKeyword_0() { return cOrderingKeyword_0; }
//'attribute'
public Keyword getAttributeKeyword_1() { return cAttributeKeyword_1; }
//'identity'
public Keyword getIdentityKeyword_2() { return cIdentityKeyword_2; }
//'role'
public Keyword getRoleKeyword_3() { return cRoleKeyword_3; }
//'realm'
public Keyword getRealmKeyword_4() { return cRealmKeyword_4; }
//'domain'
public Keyword getDomainKeyword_5() { return cDomainKeyword_5; }
}
public class EXTENSIVE_PROPERTYElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.EXTENSIVE_PROPERTY");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Keyword cEnergyKeyword_0 = (Keyword)cAlternatives.eContents().get(0);
private final Keyword cEntropyKeyword_1 = (Keyword)cAlternatives.eContents().get(1);
private final Keyword cLengthKeyword_2 = (Keyword)cAlternatives.eContents().get(2);
private final Keyword cMassKeyword_3 = (Keyword)cAlternatives.eContents().get(3);
private final Keyword cVolumeKeyword_4 = (Keyword)cAlternatives.eContents().get(4);
private final Keyword cWeightKeyword_5 = (Keyword)cAlternatives.eContents().get(5);
private final Keyword cMoneyKeyword_6 = (Keyword)cAlternatives.eContents().get(6);
private final Keyword cDurationKeyword_7 = (Keyword)cAlternatives.eContents().get(7);
private final Keyword cAreaKeyword_8 = (Keyword)cAlternatives.eContents().get(8);
//EXTENSIVE_PROPERTY:
// 'energy' | 'entropy' | 'length' | 'mass' | 'volume' | 'weight' | 'money' | 'duration' | 'area';
@Override public ParserRule getRule() { return rule; }
//'energy' | 'entropy' | 'length' | 'mass' | 'volume' | 'weight' | 'money' | 'duration' | 'area'
public Alternatives getAlternatives() { return cAlternatives; }
//'energy'
public Keyword getEnergyKeyword_0() { return cEnergyKeyword_0; }
//'entropy'
public Keyword getEntropyKeyword_1() { return cEntropyKeyword_1; }
//'length'
public Keyword getLengthKeyword_2() { return cLengthKeyword_2; }
//'mass'
public Keyword getMassKeyword_3() { return cMassKeyword_3; }
//'volume'
public Keyword getVolumeKeyword_4() { return cVolumeKeyword_4; }
//'weight'
public Keyword getWeightKeyword_5() { return cWeightKeyword_5; }
//'money'
public Keyword getMoneyKeyword_6() { return cMoneyKeyword_6; }
//'duration'
public Keyword getDurationKeyword_7() { return cDurationKeyword_7; }
//'area'
public Keyword getAreaKeyword_8() { return cAreaKeyword_8; }
}
public class INTENSIVE_PROPERTYElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.INTENSIVE_PROPERTY");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Keyword cAccelerationKeyword_0 = (Keyword)cAlternatives.eContents().get(0);
private final Keyword cPriorityKeyword_1 = (Keyword)cAlternatives.eContents().get(1);
private final Keyword cElectricPotentialKeyword_2 = (Keyword)cAlternatives.eContents().get(2);
private final Keyword cChargeKeyword_3 = (Keyword)cAlternatives.eContents().get(3);
private final Keyword cResistanceKeyword_4 = (Keyword)cAlternatives.eContents().get(4);
private final Keyword cResistivityKeyword_5 = (Keyword)cAlternatives.eContents().get(5);
private final Keyword cPressureKeyword_6 = (Keyword)cAlternatives.eContents().get(6);
private final Keyword cAngleKeyword_7 = (Keyword)cAlternatives.eContents().get(7);
private final Keyword cVelocityKeyword_8 = (Keyword)cAlternatives.eContents().get(8);
private final Keyword cTemperatureKeyword_9 = (Keyword)cAlternatives.eContents().get(9);
private final Keyword cViscosityKeyword_10 = (Keyword)cAlternatives.eContents().get(10);
//INTENSIVE_PROPERTY:
// 'acceleration' | 'priority' | 'electric-potential' | 'charge' | 'resistance' | 'resistivity' | 'pressure' | 'angle' |
// 'velocity' | 'temperature' | 'viscosity';
@Override public ParserRule getRule() { return rule; }
//'acceleration' | 'priority' | 'electric-potential' | 'charge' | 'resistance' | 'resistivity' | 'pressure' | 'angle' |
//'velocity' | 'temperature' | 'viscosity'
public Alternatives getAlternatives() { return cAlternatives; }
//'acceleration'
public Keyword getAccelerationKeyword_0() { return cAccelerationKeyword_0; }
//'priority'
public Keyword getPriorityKeyword_1() { return cPriorityKeyword_1; }
//'electric-potential'
public Keyword getElectricPotentialKeyword_2() { return cElectricPotentialKeyword_2; }
//'charge'
public Keyword getChargeKeyword_3() { return cChargeKeyword_3; }
//'resistance'
public Keyword getResistanceKeyword_4() { return cResistanceKeyword_4; }
//'resistivity'
public Keyword getResistivityKeyword_5() { return cResistivityKeyword_5; }
//'pressure'
public Keyword getPressureKeyword_6() { return cPressureKeyword_6; }
//'angle'
public Keyword getAngleKeyword_7() { return cAngleKeyword_7; }
//'velocity'
public Keyword getVelocityKeyword_8() { return cVelocityKeyword_8; }
//'temperature'
public Keyword getTemperatureKeyword_9() { return cTemperatureKeyword_9; }
//'viscosity'
public Keyword getViscosityKeyword_10() { return cViscosityKeyword_10; }
}
public class AGENT_TYPEElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.AGENT_TYPE");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Keyword cThingKeyword_0 = (Keyword)cAlternatives.eContents().get(0);
private final Keyword cProcessKeyword_1 = (Keyword)cAlternatives.eContents().get(1);
private final Keyword cAgentKeyword_2 = (Keyword)cAlternatives.eContents().get(2);
private final Keyword cEventKeyword_3 = (Keyword)cAlternatives.eContents().get(3);
//AGENT_TYPE:
// 'thing' | 'process' | 'agent' | 'event';
@Override public ParserRule getRule() { return rule; }
//'thing' | 'process' | 'agent' | 'event'
public Alternatives getAlternatives() { return cAlternatives; }
//'thing'
public Keyword getThingKeyword_0() { return cThingKeyword_0; }
//'process'
public Keyword getProcessKeyword_1() { return cProcessKeyword_1; }
//'agent'
public Keyword getAgentKeyword_2() { return cAgentKeyword_2; }
//'event'
public Keyword getEventKeyword_3() { return cEventKeyword_3; }
}
public class ConceptStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ConceptStatement");
private final UnorderedGroup cUnorderedGroup = (UnorderedGroup)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cUnorderedGroup.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_0_0 = (UnorderedGroup)cGroup_0.eContents().get(0);
private final Assignment cAbstractAssignment_0_0_0 = (Assignment)cUnorderedGroup_0_0.eContents().get(0);
private final Keyword cAbstractAbstractKeyword_0_0_0_0 = (Keyword)cAbstractAssignment_0_0_0.eContents().get(0);
private final Assignment cDeniableAssignment_0_0_1 = (Assignment)cUnorderedGroup_0_0.eContents().get(1);
private final Keyword cDeniableDeniableKeyword_0_0_1_0 = (Keyword)cDeniableAssignment_0_0_1.eContents().get(0);
private final Assignment cSpecifierAssignment_0_0_2 = (Assignment)cUnorderedGroup_0_0.eContents().get(2);
private final Alternatives cSpecifierAlternatives_0_0_2_0 = (Alternatives)cSpecifierAssignment_0_0_2.eContents().get(0);
private final Keyword cSpecifierDeliberativeKeyword_0_0_2_0_0 = (Keyword)cSpecifierAlternatives_0_0_2_0.eContents().get(0);
private final Keyword cSpecifierInteractiveKeyword_0_0_2_0_1 = (Keyword)cSpecifierAlternatives_0_0_2_0.eContents().get(1);
private final Keyword cSpecifierReactiveKeyword_0_0_2_0_2 = (Keyword)cSpecifierAlternatives_0_0_2_0.eContents().get(2);
private final Keyword cSpecifierSubjectiveKeyword_0_0_2_0_3 = (Keyword)cSpecifierAlternatives_0_0_2_0.eContents().get(3);
private final Keyword cSpecifierObjectiveKeyword_0_0_2_0_4 = (Keyword)cSpecifierAlternatives_0_0_2_0.eContents().get(4);
private final Assignment cConceptAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final RuleCall cConceptCONCEPTParserRuleCall_0_1_0 = (RuleCall)cConceptAssignment_0_1.eContents().get(0);
private final Alternatives cAlternatives_0_2 = (Alternatives)cGroup_0.eContents().get(2);
private final Assignment cRootAssignment_0_2_0 = (Assignment)cAlternatives_0_2.eContents().get(0);
private final Keyword cRootRootKeyword_0_2_0_0 = (Keyword)cRootAssignment_0_2_0.eContents().get(0);
private final Group cGroup_0_2_1 = (Group)cAlternatives_0_2.eContents().get(1);
private final Group cGroup_0_2_1_0 = (Group)cGroup_0_2_1.eContents().get(0);
private final Assignment cDeclarationAssignment_0_2_1_0_0 = (Assignment)cGroup_0_2_1_0.eContents().get(0);
private final RuleCall cDeclarationValidIDParserRuleCall_0_2_1_0_0_0 = (RuleCall)cDeclarationAssignment_0_2_1_0_0.eContents().get(0);
private final Group cGroup_0_2_1_0_1 = (Group)cGroup_0_2_1_0.eContents().get(1);
private final Keyword cIdentifiedKeyword_0_2_1_0_1_0 = (Keyword)cGroup_0_2_1_0_1.eContents().get(0);
private final Keyword cAsKeyword_0_2_1_0_1_1 = (Keyword)cGroup_0_2_1_0_1.eContents().get(1);
private final Assignment cIdentifierAssignment_0_2_1_0_1_2 = (Assignment)cGroup_0_2_1_0_1.eContents().get(2);
private final RuleCall cIdentifierSTRINGTerminalRuleCall_0_2_1_0_1_2_0 = (RuleCall)cIdentifierAssignment_0_2_1_0_1_2.eContents().get(0);
private final Keyword cByKeyword_0_2_1_0_1_3 = (Keyword)cGroup_0_2_1_0_1.eContents().get(3);
private final Assignment cAuthorityAssignment_0_2_1_0_1_4 = (Assignment)cGroup_0_2_1_0_1.eContents().get(4);
private final RuleCall cAuthorityValidIDParserRuleCall_0_2_1_0_1_4_0 = (RuleCall)cAuthorityAssignment_0_2_1_0_1_4.eContents().get(0);
private final Group cGroup_0_2_1_1 = (Group)cGroup_0_2_1.eContents().get(1);
private final Keyword cNamedKeyword_0_2_1_1_0 = (Keyword)cGroup_0_2_1_1.eContents().get(0);
private final Assignment cNameAssignment_0_2_1_1_1 = (Assignment)cGroup_0_2_1_1.eContents().get(1);
private final Alternatives cNameAlternatives_0_2_1_1_1_0 = (Alternatives)cNameAssignment_0_2_1_1_1.eContents().get(0);
private final RuleCall cNameValidIDParserRuleCall_0_2_1_1_1_0_0 = (RuleCall)cNameAlternatives_0_2_1_1_1_0.eContents().get(0);
private final RuleCall cNameSTRINGTerminalRuleCall_0_2_1_1_1_0_1 = (RuleCall)cNameAlternatives_0_2_1_1_1_0.eContents().get(1);
private final Assignment cDocstringAssignment_0_3 = (Assignment)cGroup_0.eContents().get(3);
private final RuleCall cDocstringSTRINGTerminalRuleCall_0_3_0 = (RuleCall)cDocstringAssignment_0_3.eContents().get(0);
private final Group cGroup_1 = (Group)cUnorderedGroup.eContents().get(1);
private final Alternatives cAlternatives_1_0 = (Alternatives)cGroup_1.eContents().get(0);
private final Keyword cIsKeyword_1_0_0 = (Keyword)cAlternatives_1_0.eContents().get(0);
private final Assignment cExtensionAssignment_1_0_1 = (Assignment)cAlternatives_1_0.eContents().get(1);
private final Keyword cExtensionExtendsKeyword_1_0_1_0 = (Keyword)cExtensionAssignment_1_0_1.eContents().get(0);
private final Alternatives cAlternatives_1_1 = (Alternatives)cGroup_1.eContents().get(1);
private final Assignment cNothingAssignment_1_1_0 = (Assignment)cAlternatives_1_1.eContents().get(0);
private final Keyword cNothingNothingKeyword_1_1_0_0 = (Keyword)cNothingAssignment_1_1_0.eContents().get(0);
private final Assignment cParentsAssignment_1_1_1 = (Assignment)cAlternatives_1_1.eContents().get(1);
private final RuleCall cParentsConceptListParserRuleCall_1_1_1_0 = (RuleCall)cParentsAssignment_1_1_1.eContents().get(0);
private final Alternatives cAlternatives_2 = (Alternatives)cUnorderedGroup.eContents().get(2);
private final Group cGroup_2_0 = (Group)cAlternatives_2.eContents().get(0);
private final Keyword cExposesKeyword_2_0_0 = (Keyword)cGroup_2_0.eContents().get(0);
private final Assignment cContextualizedTraitsAssignment_2_0_1 = (Assignment)cGroup_2_0.eContents().get(1);
private final RuleCall cContextualizedTraitsDerivedConceptListParserRuleCall_2_0_1_0 = (RuleCall)cContextualizedTraitsAssignment_2_0_1.eContents().get(0);
private final Group cGroup_2_1 = (Group)cAlternatives_2.eContents().get(1);
private final Assignment cSpecificAssignment_2_1_0 = (Assignment)cGroup_2_1.eContents().get(0);
private final Keyword cSpecificWithKeyword_2_1_0_0 = (Keyword)cSpecificAssignment_2_1_0.eContents().get(0);
private final Assignment cContextualizedTraitsAssignment_2_1_1 = (Assignment)cGroup_2_1.eContents().get(1);
private final RuleCall cContextualizedTraitsNegatableDerivedConceptListParserRuleCall_2_1_1_0 = (RuleCall)cContextualizedTraitsAssignment_2_1_1.eContents().get(0);
private final Group cGroup_3 = (Group)cUnorderedGroup.eContents().get(3);
private final Keyword cRequiresKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cRequirementAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cRequirementIdentityRequirementListParserRuleCall_3_1_0 = (RuleCall)cRequirementAssignment_3_1.eContents().get(0);
private final Group cGroup_4 = (Group)cUnorderedGroup.eContents().get(4);
private final Keyword cDescribesKeyword_4_0 = (Keyword)cGroup_4.eContents().get(0);
private final Assignment cDescribedQualityAssignment_4_1 = (Assignment)cGroup_4.eContents().get(1);
private final RuleCall cDescribedQualityDerivedConceptDeclarationParserRuleCall_4_1_0 = (RuleCall)cDescribedQualityAssignment_4_1.eContents().get(0);
private final Group cGroup_5 = (Group)cUnorderedGroup.eContents().get(5);
private final Keyword cInheritsKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Assignment cActuallyInheritedTraitsAssignment_5_1 = (Assignment)cGroup_5.eContents().get(1);
private final RuleCall cActuallyInheritedTraitsConceptListParserRuleCall_5_1_0 = (RuleCall)cActuallyInheritedTraitsAssignment_5_1.eContents().get(0);
private final Group cGroup_6 = (Group)cUnorderedGroup.eContents().get(6);
private final Keyword cHasKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Keyword cRoleKeyword_6_1 = (Keyword)cGroup_6.eContents().get(1);
private final Assignment cRolesAssignment_6_2 = (Assignment)cGroup_6.eContents().get(2);
private final RuleCall cRolesConceptListParserRuleCall_6_2_0 = (RuleCall)cRolesAssignment_6_2.eContents().get(0);
private final Group cGroup_6_3 = (Group)cGroup_6.eContents().get(3);
private final Keyword cForKeyword_6_3_0 = (Keyword)cGroup_6_3.eContents().get(0);
private final Assignment cTargetObservableAssignment_6_3_1 = (Assignment)cGroup_6_3.eContents().get(1);
private final RuleCall cTargetObservableConceptListParserRuleCall_6_3_1_0 = (RuleCall)cTargetObservableAssignment_6_3_1.eContents().get(0);
private final Keyword cInKeyword_6_4 = (Keyword)cGroup_6.eContents().get(4);
private final Assignment cRestrictedObservableAssignment_6_5 = (Assignment)cGroup_6.eContents().get(5);
private final RuleCall cRestrictedObservableConceptListParserRuleCall_6_5_0 = (RuleCall)cRestrictedObservableAssignment_6_5.eContents().get(0);
private final Group cGroup_7 = (Group)cUnorderedGroup.eContents().get(7);
private final Keyword cConfersKeyword_7_0 = (Keyword)cGroup_7.eContents().get(0);
private final Assignment cConferredTraitsAssignment_7_1 = (Assignment)cGroup_7.eContents().get(1);
private final RuleCall cConferredTraitsConceptListParserRuleCall_7_1_0 = (RuleCall)cConferredTraitsAssignment_7_1.eContents().get(0);
private final Group cGroup_7_2 = (Group)cGroup_7.eContents().get(2);
private final Keyword cToKeyword_7_2_0 = (Keyword)cGroup_7_2.eContents().get(0);
private final Assignment cConferredTargetsAssignment_7_2_1 = (Assignment)cGroup_7_2.eContents().get(1);
private final RuleCall cConferredTargetsConceptListParserRuleCall_7_2_1_0 = (RuleCall)cConferredTargetsAssignment_7_2_1.eContents().get(0);
private final Group cGroup_8 = (Group)cUnorderedGroup.eContents().get(8);
private final Keyword cCreatesKeyword_8_0 = (Keyword)cGroup_8.eContents().get(0);
private final Assignment cCreatesAssignment_8_1 = (Assignment)cGroup_8.eContents().get(1);
private final RuleCall cCreatesConceptListParserRuleCall_8_1_0 = (RuleCall)cCreatesAssignment_8_1.eContents().get(0);
private final Group cGroup_9 = (Group)cUnorderedGroup.eContents().get(9);
private final Keyword cAppliesKeyword_9_0 = (Keyword)cGroup_9.eContents().get(0);
private final Keyword cToKeyword_9_1 = (Keyword)cGroup_9.eContents().get(1);
private final Assignment cTraitTargetsAssignment_9_2 = (Assignment)cGroup_9.eContents().get(2);
private final RuleCall cTraitTargetsConceptListParserRuleCall_9_2_0 = (RuleCall)cTraitTargetsAssignment_9_2.eContents().get(0);
private final Group cGroup_10 = (Group)cUnorderedGroup.eContents().get(10);
private final Keyword cAffectsKeyword_10_0 = (Keyword)cGroup_10.eContents().get(0);
private final Assignment cQualitiesAffectedAssignment_10_1 = (Assignment)cGroup_10.eContents().get(1);
private final RuleCall cQualitiesAffectedConceptListParserRuleCall_10_1_0 = (RuleCall)cQualitiesAffectedAssignment_10_1.eContents().get(0);
private final Group cGroup_11 = (Group)cUnorderedGroup.eContents().get(11);
private final Keyword cEqualsKeyword_11_0 = (Keyword)cGroup_11.eContents().get(0);
private final Assignment cEquivalencesAssignment_11_1 = (Assignment)cGroup_11.eContents().get(1);
private final RuleCall cEquivalencesConceptListParserRuleCall_11_1_0 = (RuleCall)cEquivalencesAssignment_11_1.eContents().get(0);
private final Group cGroup_12 = (Group)cUnorderedGroup.eContents().get(12);
private final Keyword cHasKeyword_12_0 = (Keyword)cGroup_12.eContents().get(0);
private final Assignment cDisjointAssignment_12_1 = (Assignment)cGroup_12.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_12_1_0 = (Keyword)cDisjointAssignment_12_1.eContents().get(0);
private final Keyword cChildrenKeyword_12_2 = (Keyword)cGroup_12.eContents().get(2);
private final Assignment cChildrenAssignment_12_3 = (Assignment)cGroup_12.eContents().get(3);
private final RuleCall cChildrenConceptListParserRuleCall_12_3_0 = (RuleCall)cChildrenAssignment_12_3.eContents().get(0);
private final Assignment cRestrictionsAssignment_13 = (Assignment)cUnorderedGroup.eContents().get(13);
private final RuleCall cRestrictionsRestrictionStatementParserRuleCall_13_0 = (RuleCall)cRestrictionsAssignment_13.eContents().get(0);
private final Group cGroup_14 = (Group)cUnorderedGroup.eContents().get(14);
private final Keyword cWithKeyword_14_0 = (Keyword)cGroup_14.eContents().get(0);
private final Keyword cMetadataKeyword_14_1 = (Keyword)cGroup_14.eContents().get(1);
private final Assignment cMetadataAssignment_14_2 = (Assignment)cGroup_14.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_14_2_0 = (RuleCall)cMetadataAssignment_14_2.eContents().get(0);
/// *
// * ------------------------------------------------------------------------------------------
// * Ontology language - basically a more intuitive and imperative OW2L/Manchester syntax. Does not
// * cover all of OWL2 yet, but should be plenty for modeling.
// *
// * deniable is for traits only. If a trait is deniable, 'not Trait' is a trait (of not being Trait).
// * if not deniable, 'not Trait' means being any of the other concrete traits; if there is only one
// * concrete trait it's an error. If there are two, it means being the other.
// * ------------------------------------------------------------------------------------------
// * /
//ConceptStatement:
// (abstract?='abstract'? & deniable?='deniable'? & specifier=('deliberative' | 'interactive' | 'reactive' |
// 'subjective' | 'objective')?) concept=CONCEPT (root?='root' | (declaration=ValidID ('identified' 'as'
// identifier=STRING 'by' authority=ValidID)?) ('named' name=(ValidID | STRING))?) docstring=STRING? & (('is' |
// extension?='extends') (nothing?='nothing' | parents=ConceptList))? & ('exposes'
// contextualizedTraits=DerivedConceptList | specific?='with' contextualizedTraits=NegatableDerivedConceptList)? &
// ('requires' requirement=IdentityRequirementList)? & ('describes' describedQuality=DerivedConceptDeclaration)? &
// ('inherits' actuallyInheritedTraits=ConceptList)? & ('has' 'role' roles=ConceptList ('for'
// targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('confers' conferredTraits=ConceptList ('to'
// conferredTargets=ConceptList)?)? & ('creates' creates=ConceptList)? & ('applies' 'to' traitTargets=ConceptList)? &
// ('affects' qualitiesAffected=ConceptList)? & ('equals' equivalences=ConceptList)? & ('has' disjoint?='disjoint'?
// 'children' children=ConceptList)? & restrictions+=RestrictionStatement* & ('with' 'metadata' metadata=Metadata)?;
@Override public ParserRule getRule() { return rule; }
//(abstract?='abstract'? & deniable?='deniable'? & specifier=('deliberative' | 'interactive' | 'reactive' | 'subjective' |
//'objective')?) concept=CONCEPT (root?='root' | (declaration=ValidID ('identified' 'as' identifier=STRING 'by'
//authority=ValidID)?) ('named' name=(ValidID | STRING))?) docstring=STRING? & (('is' | extension?='extends')
//(nothing?='nothing' | parents=ConceptList))? & ('exposes' contextualizedTraits=DerivedConceptList | specific?='with'
//contextualizedTraits=NegatableDerivedConceptList)? & ('requires' requirement=IdentityRequirementList)? & ('describes'
//describedQuality=DerivedConceptDeclaration)? & ('inherits' actuallyInheritedTraits=ConceptList)? & ('has' 'role'
//roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('confers'
//conferredTraits=ConceptList ('to' conferredTargets=ConceptList)?)? & ('creates' creates=ConceptList)? & ('applies'
//'to' traitTargets=ConceptList)? & ('affects' qualitiesAffected=ConceptList)? & ('equals' equivalences=ConceptList)? &
//('has' disjoint?='disjoint'? 'children' children=ConceptList)? & restrictions+=RestrictionStatement* & ('with'
//'metadata' metadata=Metadata)?
public UnorderedGroup getUnorderedGroup() { return cUnorderedGroup; }
//(abstract?='abstract'? & deniable?='deniable'? & specifier=('deliberative' | 'interactive' | 'reactive' | 'subjective' |
//'objective')?) concept=CONCEPT (root?='root' | (declaration=ValidID ('identified' 'as' identifier=STRING 'by'
//authority=ValidID)?) ('named' name=(ValidID | STRING))?) docstring=STRING?
public Group getGroup_0() { return cGroup_0; }
//(abstract?='abstract'? & deniable?='deniable'? & specifier=('deliberative' | 'interactive' | 'reactive' | 'subjective' |
//'objective')?)
public UnorderedGroup getUnorderedGroup_0_0() { return cUnorderedGroup_0_0; }
//abstract?='abstract'?
public Assignment getAbstractAssignment_0_0_0() { return cAbstractAssignment_0_0_0; }
//'abstract'
public Keyword getAbstractAbstractKeyword_0_0_0_0() { return cAbstractAbstractKeyword_0_0_0_0; }
//deniable?='deniable'?
public Assignment getDeniableAssignment_0_0_1() { return cDeniableAssignment_0_0_1; }
//'deniable'
public Keyword getDeniableDeniableKeyword_0_0_1_0() { return cDeniableDeniableKeyword_0_0_1_0; }
//specifier=('deliberative' | 'interactive' | 'reactive' | 'subjective' | 'objective')?
public Assignment getSpecifierAssignment_0_0_2() { return cSpecifierAssignment_0_0_2; }
//('deliberative' | 'interactive' | 'reactive' | 'subjective' | 'objective')
public Alternatives getSpecifierAlternatives_0_0_2_0() { return cSpecifierAlternatives_0_0_2_0; }
//'deliberative'
public Keyword getSpecifierDeliberativeKeyword_0_0_2_0_0() { return cSpecifierDeliberativeKeyword_0_0_2_0_0; }
//'interactive'
public Keyword getSpecifierInteractiveKeyword_0_0_2_0_1() { return cSpecifierInteractiveKeyword_0_0_2_0_1; }
//'reactive'
public Keyword getSpecifierReactiveKeyword_0_0_2_0_2() { return cSpecifierReactiveKeyword_0_0_2_0_2; }
//'subjective'
public Keyword getSpecifierSubjectiveKeyword_0_0_2_0_3() { return cSpecifierSubjectiveKeyword_0_0_2_0_3; }
//'objective'
public Keyword getSpecifierObjectiveKeyword_0_0_2_0_4() { return cSpecifierObjectiveKeyword_0_0_2_0_4; }
/// *
// * root only allowed after 'domain' and once in a domain project.
// * 'identified as' should simply declare an ALIAS for an authority concept. With that a user can declare and name
// * an authority concept, which does not carry the name beyond the usage in namespaces. This guarantees interoperability
// * across applications.
// *
// * Concept name is stored in rdfs:label annotation but is indexed by the ontologies and it
// * can be used as an alias in concept retrieval. In a future release we should enable languages
// * (named X in en, Y in es) and allow "jargon files" for external translation. Namespaces should
// * be able to state their language and tune on one of these (and only one) while the "official"
// * label (without 'in') remains available to all.
// *
// * /
//concept=CONCEPT
public Assignment getConceptAssignment_0_1() { return cConceptAssignment_0_1; }
//CONCEPT
public RuleCall getConceptCONCEPTParserRuleCall_0_1_0() { return cConceptCONCEPTParserRuleCall_0_1_0; }
//(root?='root' | (declaration=ValidID ('identified' 'as' identifier=STRING 'by' authority=ValidID)?) ('named'
//name=(ValidID | STRING))?)
public Alternatives getAlternatives_0_2() { return cAlternatives_0_2; }
//root?='root'
public Assignment getRootAssignment_0_2_0() { return cRootAssignment_0_2_0; }
//'root'
public Keyword getRootRootKeyword_0_2_0_0() { return cRootRootKeyword_0_2_0_0; }
//(declaration=ValidID ('identified' 'as' identifier=STRING 'by' authority=ValidID)?) ('named' name=(ValidID | STRING))?
public Group getGroup_0_2_1() { return cGroup_0_2_1; }
//(declaration=ValidID ('identified' 'as' identifier=STRING 'by' authority=ValidID)?)
public Group getGroup_0_2_1_0() { return cGroup_0_2_1_0; }
//declaration=ValidID
public Assignment getDeclarationAssignment_0_2_1_0_0() { return cDeclarationAssignment_0_2_1_0_0; }
//ValidID
public RuleCall getDeclarationValidIDParserRuleCall_0_2_1_0_0_0() { return cDeclarationValidIDParserRuleCall_0_2_1_0_0_0; }
//('identified' 'as' identifier=STRING 'by' authority=ValidID)?
public Group getGroup_0_2_1_0_1() { return cGroup_0_2_1_0_1; }
//'identified'
public Keyword getIdentifiedKeyword_0_2_1_0_1_0() { return cIdentifiedKeyword_0_2_1_0_1_0; }
//'as'
public Keyword getAsKeyword_0_2_1_0_1_1() { return cAsKeyword_0_2_1_0_1_1; }
//identifier=STRING
public Assignment getIdentifierAssignment_0_2_1_0_1_2() { return cIdentifierAssignment_0_2_1_0_1_2; }
//STRING
public RuleCall getIdentifierSTRINGTerminalRuleCall_0_2_1_0_1_2_0() { return cIdentifierSTRINGTerminalRuleCall_0_2_1_0_1_2_0; }
//'by'
public Keyword getByKeyword_0_2_1_0_1_3() { return cByKeyword_0_2_1_0_1_3; }
//authority=ValidID
public Assignment getAuthorityAssignment_0_2_1_0_1_4() { return cAuthorityAssignment_0_2_1_0_1_4; }
//ValidID
public RuleCall getAuthorityValidIDParserRuleCall_0_2_1_0_1_4_0() { return cAuthorityValidIDParserRuleCall_0_2_1_0_1_4_0; }
//('named' name=(ValidID | STRING))?
public Group getGroup_0_2_1_1() { return cGroup_0_2_1_1; }
//'named'
public Keyword getNamedKeyword_0_2_1_1_0() { return cNamedKeyword_0_2_1_1_0; }
//name=(ValidID | STRING)
public Assignment getNameAssignment_0_2_1_1_1() { return cNameAssignment_0_2_1_1_1; }
//(ValidID | STRING)
public Alternatives getNameAlternatives_0_2_1_1_1_0() { return cNameAlternatives_0_2_1_1_1_0; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_0_2_1_1_1_0_0() { return cNameValidIDParserRuleCall_0_2_1_1_1_0_0; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_0_2_1_1_1_0_1() { return cNameSTRINGTerminalRuleCall_0_2_1_1_1_0_1; }
//docstring=STRING?
public Assignment getDocstringAssignment_0_3() { return cDocstringAssignment_0_3; }
//STRING
public RuleCall getDocstringSTRINGTerminalRuleCall_0_3_0() { return cDocstringSTRINGTerminalRuleCall_0_3_0; }
//(('is' | extension?='extends') (nothing?='nothing' | parents=ConceptList))?
public Group getGroup_1() { return cGroup_1; }
//('is' | extension?='extends')
public Alternatives getAlternatives_1_0() { return cAlternatives_1_0; }
//'is'
public Keyword getIsKeyword_1_0_0() { return cIsKeyword_1_0_0; }
//extension?='extends'
public Assignment getExtensionAssignment_1_0_1() { return cExtensionAssignment_1_0_1; }
//'extends'
public Keyword getExtensionExtendsKeyword_1_0_1_0() { return cExtensionExtendsKeyword_1_0_1_0; }
//(nothing?='nothing' | parents=ConceptList)
public Alternatives getAlternatives_1_1() { return cAlternatives_1_1; }
//nothing?='nothing'
public Assignment getNothingAssignment_1_1_0() { return cNothingAssignment_1_1_0; }
//'nothing'
public Keyword getNothingNothingKeyword_1_1_0_0() { return cNothingNothingKeyword_1_1_0_0; }
//parents=ConceptList
public Assignment getParentsAssignment_1_1_1() { return cParentsAssignment_1_1_1; }
//ConceptList
public RuleCall getParentsConceptListParserRuleCall_1_1_1_0() { return cParentsConceptListParserRuleCall_1_1_1_0; }
//('exposes' contextualizedTraits=DerivedConceptList | specific?='with' contextualizedTraits=NegatableDerivedConceptList)?
public Alternatives getAlternatives_2() { return cAlternatives_2; }
//'exposes' contextualizedTraits=DerivedConceptList
public Group getGroup_2_0() { return cGroup_2_0; }
//'exposes'
public Keyword getExposesKeyword_2_0_0() { return cExposesKeyword_2_0_0; }
//contextualizedTraits=DerivedConceptList
public Assignment getContextualizedTraitsAssignment_2_0_1() { return cContextualizedTraitsAssignment_2_0_1; }
//DerivedConceptList
public RuleCall getContextualizedTraitsDerivedConceptListParserRuleCall_2_0_1_0() { return cContextualizedTraitsDerivedConceptListParserRuleCall_2_0_1_0; }
//specific?='with' contextualizedTraits=NegatableDerivedConceptList
public Group getGroup_2_1() { return cGroup_2_1; }
//specific?='with'
public Assignment getSpecificAssignment_2_1_0() { return cSpecificAssignment_2_1_0; }
//'with'
public Keyword getSpecificWithKeyword_2_1_0_0() { return cSpecificWithKeyword_2_1_0_0; }
//contextualizedTraits=NegatableDerivedConceptList
public Assignment getContextualizedTraitsAssignment_2_1_1() { return cContextualizedTraitsAssignment_2_1_1; }
//NegatableDerivedConceptList
public RuleCall getContextualizedTraitsNegatableDerivedConceptListParserRuleCall_2_1_1_0() { return cContextualizedTraitsNegatableDerivedConceptListParserRuleCall_2_1_1_0; }
//('requires' requirement=IdentityRequirementList)?
public Group getGroup_3() { return cGroup_3; }
//'requires'
public Keyword getRequiresKeyword_3_0() { return cRequiresKeyword_3_0; }
//requirement=IdentityRequirementList
public Assignment getRequirementAssignment_3_1() { return cRequirementAssignment_3_1; }
//IdentityRequirementList
public RuleCall getRequirementIdentityRequirementListParserRuleCall_3_1_0() { return cRequirementIdentityRequirementListParserRuleCall_3_1_0; }
//('describes' describedQuality=DerivedConceptDeclaration)?
public Group getGroup_4() { return cGroup_4; }
//'describes'
public Keyword getDescribesKeyword_4_0() { return cDescribesKeyword_4_0; }
//describedQuality=DerivedConceptDeclaration
public Assignment getDescribedQualityAssignment_4_1() { return cDescribedQualityAssignment_4_1; }
//DerivedConceptDeclaration
public RuleCall getDescribedQualityDerivedConceptDeclarationParserRuleCall_4_1_0() { return cDescribedQualityDerivedConceptDeclarationParserRuleCall_4_1_0; }
//('inherits' actuallyInheritedTraits=ConceptList)?
public Group getGroup_5() { return cGroup_5; }
//'inherits'
public Keyword getInheritsKeyword_5_0() { return cInheritsKeyword_5_0; }
//actuallyInheritedTraits=ConceptList
public Assignment getActuallyInheritedTraitsAssignment_5_1() { return cActuallyInheritedTraitsAssignment_5_1; }
//ConceptList
public RuleCall getActuallyInheritedTraitsConceptListParserRuleCall_5_1_0() { return cActuallyInheritedTraitsConceptListParserRuleCall_5_1_0; }
//('has' 'role' roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)?
public Group getGroup_6() { return cGroup_6; }
//'has'
public Keyword getHasKeyword_6_0() { return cHasKeyword_6_0; }
//'role'
public Keyword getRoleKeyword_6_1() { return cRoleKeyword_6_1; }
//roles=ConceptList
public Assignment getRolesAssignment_6_2() { return cRolesAssignment_6_2; }
//ConceptList
public RuleCall getRolesConceptListParserRuleCall_6_2_0() { return cRolesConceptListParserRuleCall_6_2_0; }
//('for' targetObservable=ConceptList)?
public Group getGroup_6_3() { return cGroup_6_3; }
//'for'
public Keyword getForKeyword_6_3_0() { return cForKeyword_6_3_0; }
//targetObservable=ConceptList
public Assignment getTargetObservableAssignment_6_3_1() { return cTargetObservableAssignment_6_3_1; }
//ConceptList
public RuleCall getTargetObservableConceptListParserRuleCall_6_3_1_0() { return cTargetObservableConceptListParserRuleCall_6_3_1_0; }
//'in'
public Keyword getInKeyword_6_4() { return cInKeyword_6_4; }
//restrictedObservable=ConceptList
public Assignment getRestrictedObservableAssignment_6_5() { return cRestrictedObservableAssignment_6_5; }
//ConceptList
public RuleCall getRestrictedObservableConceptListParserRuleCall_6_5_0() { return cRestrictedObservableConceptListParserRuleCall_6_5_0; }
//('confers' conferredTraits=ConceptList ('to' conferredTargets=ConceptList)?)?
public Group getGroup_7() { return cGroup_7; }
//'confers'
public Keyword getConfersKeyword_7_0() { return cConfersKeyword_7_0; }
//conferredTraits=ConceptList
public Assignment getConferredTraitsAssignment_7_1() { return cConferredTraitsAssignment_7_1; }
//ConceptList
public RuleCall getConferredTraitsConceptListParserRuleCall_7_1_0() { return cConferredTraitsConceptListParserRuleCall_7_1_0; }
//('to' conferredTargets=ConceptList)?
public Group getGroup_7_2() { return cGroup_7_2; }
//'to'
public Keyword getToKeyword_7_2_0() { return cToKeyword_7_2_0; }
//conferredTargets=ConceptList
public Assignment getConferredTargetsAssignment_7_2_1() { return cConferredTargetsAssignment_7_2_1; }
//ConceptList
public RuleCall getConferredTargetsConceptListParserRuleCall_7_2_1_0() { return cConferredTargetsConceptListParserRuleCall_7_2_1_0; }
//('creates' creates=ConceptList)?
public Group getGroup_8() { return cGroup_8; }
//'creates'
public Keyword getCreatesKeyword_8_0() { return cCreatesKeyword_8_0; }
//creates=ConceptList
public Assignment getCreatesAssignment_8_1() { return cCreatesAssignment_8_1; }
//ConceptList
public RuleCall getCreatesConceptListParserRuleCall_8_1_0() { return cCreatesConceptListParserRuleCall_8_1_0; }
//('applies' 'to' traitTargets=ConceptList)?
public Group getGroup_9() { return cGroup_9; }
//'applies'
public Keyword getAppliesKeyword_9_0() { return cAppliesKeyword_9_0; }
//'to'
public Keyword getToKeyword_9_1() { return cToKeyword_9_1; }
//traitTargets=ConceptList
public Assignment getTraitTargetsAssignment_9_2() { return cTraitTargetsAssignment_9_2; }
//ConceptList
public RuleCall getTraitTargetsConceptListParserRuleCall_9_2_0() { return cTraitTargetsConceptListParserRuleCall_9_2_0; }
//('affects' qualitiesAffected=ConceptList)?
public Group getGroup_10() { return cGroup_10; }
//'affects'
public Keyword getAffectsKeyword_10_0() { return cAffectsKeyword_10_0; }
//qualitiesAffected=ConceptList
public Assignment getQualitiesAffectedAssignment_10_1() { return cQualitiesAffectedAssignment_10_1; }
//ConceptList
public RuleCall getQualitiesAffectedConceptListParserRuleCall_10_1_0() { return cQualitiesAffectedConceptListParserRuleCall_10_1_0; }
//('equals' equivalences=ConceptList)?
public Group getGroup_11() { return cGroup_11; }
//'equals'
public Keyword getEqualsKeyword_11_0() { return cEqualsKeyword_11_0; }
//equivalences=ConceptList
public Assignment getEquivalencesAssignment_11_1() { return cEquivalencesAssignment_11_1; }
//ConceptList
public RuleCall getEquivalencesConceptListParserRuleCall_11_1_0() { return cEquivalencesConceptListParserRuleCall_11_1_0; }
//('has' disjoint?='disjoint'? 'children' children=ConceptList)?
public Group getGroup_12() { return cGroup_12; }
//'has'
public Keyword getHasKeyword_12_0() { return cHasKeyword_12_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_12_1() { return cDisjointAssignment_12_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_12_1_0() { return cDisjointDisjointKeyword_12_1_0; }
//'children'
public Keyword getChildrenKeyword_12_2() { return cChildrenKeyword_12_2; }
//children=ConceptList
public Assignment getChildrenAssignment_12_3() { return cChildrenAssignment_12_3; }
//ConceptList
public RuleCall getChildrenConceptListParserRuleCall_12_3_0() { return cChildrenConceptListParserRuleCall_12_3_0; }
//restrictions+=RestrictionStatement*
public Assignment getRestrictionsAssignment_13() { return cRestrictionsAssignment_13; }
//RestrictionStatement
public RuleCall getRestrictionsRestrictionStatementParserRuleCall_13_0() { return cRestrictionsRestrictionStatementParserRuleCall_13_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_14() { return cGroup_14; }
//'with'
public Keyword getWithKeyword_14_0() { return cWithKeyword_14_0; }
//'metadata'
public Keyword getMetadataKeyword_14_1() { return cMetadataKeyword_14_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_14_2() { return cMetadataAssignment_14_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_14_2_0() { return cMetadataMetadataParserRuleCall_14_2_0; }
}
public class ContextualRedeclarationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ContextualRedeclaration");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cRedeclarationAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final Keyword cRedeclarationConceptKeyword_0_0 = (Keyword)cRedeclarationAssignment_0.eContents().get(0);
private final Assignment cRedeclaredAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cRedeclaredConceptDeclarationParserRuleCall_1_0 = (RuleCall)cRedeclaredAssignment_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_2 = (UnorderedGroup)cGroup.eContents().get(2);
private final Group cGroup_2_0 = (Group)cUnorderedGroup_2.eContents().get(0);
private final Keyword cHasKeyword_2_0_0 = (Keyword)cGroup_2_0.eContents().get(0);
private final Keyword cRoleKeyword_2_0_1 = (Keyword)cGroup_2_0.eContents().get(1);
private final Assignment cRolesAssignment_2_0_2 = (Assignment)cGroup_2_0.eContents().get(2);
private final RuleCall cRolesConceptListParserRuleCall_2_0_2_0 = (RuleCall)cRolesAssignment_2_0_2.eContents().get(0);
private final Group cGroup_2_0_3 = (Group)cGroup_2_0.eContents().get(3);
private final Keyword cForKeyword_2_0_3_0 = (Keyword)cGroup_2_0_3.eContents().get(0);
private final Assignment cTargetObservableAssignment_2_0_3_1 = (Assignment)cGroup_2_0_3.eContents().get(1);
private final RuleCall cTargetObservableConceptListParserRuleCall_2_0_3_1_0 = (RuleCall)cTargetObservableAssignment_2_0_3_1.eContents().get(0);
private final Keyword cInKeyword_2_0_4 = (Keyword)cGroup_2_0.eContents().get(4);
private final Assignment cRestrictedObservableAssignment_2_0_5 = (Assignment)cGroup_2_0.eContents().get(5);
private final RuleCall cRestrictedObservableConceptListParserRuleCall_2_0_5_0 = (RuleCall)cRestrictedObservableAssignment_2_0_5.eContents().get(0);
private final Group cGroup_2_1 = (Group)cUnorderedGroup_2.eContents().get(1);
private final Keyword cHasKeyword_2_1_0 = (Keyword)cGroup_2_1.eContents().get(0);
private final Assignment cDisjointAssignment_2_1_1 = (Assignment)cGroup_2_1.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_2_1_1_0 = (Keyword)cDisjointAssignment_2_1_1.eContents().get(0);
private final Keyword cChildrenKeyword_2_1_2 = (Keyword)cGroup_2_1.eContents().get(2);
private final Assignment cChildrenAssignment_2_1_3 = (Assignment)cGroup_2_1.eContents().get(3);
private final RuleCall cChildrenConceptListParserRuleCall_2_1_3_0 = (RuleCall)cChildrenAssignment_2_1_3.eContents().get(0);
/// *
// * 'concept' is a reference to a concept that must have been seen before. Can only add children and scoped roles.
// * /
//ContextualRedeclaration ConceptStatement:
// redeclaration?='concept' redeclared=ConceptDeclaration (('has' 'role' roles=ConceptList ('for'
// targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('has' disjoint?='disjoint'? 'children'
// children=ConceptList)?)
@Override public ParserRule getRule() { return rule; }
//redeclaration?='concept' redeclared=ConceptDeclaration (('has' 'role' roles=ConceptList ('for'
//targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('has' disjoint?='disjoint'? 'children'
//children=ConceptList)?)
public Group getGroup() { return cGroup; }
//redeclaration?='concept'
public Assignment getRedeclarationAssignment_0() { return cRedeclarationAssignment_0; }
//'concept'
public Keyword getRedeclarationConceptKeyword_0_0() { return cRedeclarationConceptKeyword_0_0; }
//redeclared=ConceptDeclaration
public Assignment getRedeclaredAssignment_1() { return cRedeclaredAssignment_1; }
//ConceptDeclaration
public RuleCall getRedeclaredConceptDeclarationParserRuleCall_1_0() { return cRedeclaredConceptDeclarationParserRuleCall_1_0; }
//(('has' 'role' roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('has'
//disjoint?='disjoint'? 'children' children=ConceptList)?)
public UnorderedGroup getUnorderedGroup_2() { return cUnorderedGroup_2; }
//('has' 'role' roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)?
public Group getGroup_2_0() { return cGroup_2_0; }
//'has'
public Keyword getHasKeyword_2_0_0() { return cHasKeyword_2_0_0; }
//'role'
public Keyword getRoleKeyword_2_0_1() { return cRoleKeyword_2_0_1; }
//roles=ConceptList
public Assignment getRolesAssignment_2_0_2() { return cRolesAssignment_2_0_2; }
//ConceptList
public RuleCall getRolesConceptListParserRuleCall_2_0_2_0() { return cRolesConceptListParserRuleCall_2_0_2_0; }
//('for' targetObservable=ConceptList)?
public Group getGroup_2_0_3() { return cGroup_2_0_3; }
//'for'
public Keyword getForKeyword_2_0_3_0() { return cForKeyword_2_0_3_0; }
//targetObservable=ConceptList
public Assignment getTargetObservableAssignment_2_0_3_1() { return cTargetObservableAssignment_2_0_3_1; }
//ConceptList
public RuleCall getTargetObservableConceptListParserRuleCall_2_0_3_1_0() { return cTargetObservableConceptListParserRuleCall_2_0_3_1_0; }
//'in'
public Keyword getInKeyword_2_0_4() { return cInKeyword_2_0_4; }
//restrictedObservable=ConceptList
public Assignment getRestrictedObservableAssignment_2_0_5() { return cRestrictedObservableAssignment_2_0_5; }
//ConceptList
public RuleCall getRestrictedObservableConceptListParserRuleCall_2_0_5_0() { return cRestrictedObservableConceptListParserRuleCall_2_0_5_0; }
//('has' disjoint?='disjoint'? 'children' children=ConceptList)?
public Group getGroup_2_1() { return cGroup_2_1; }
//'has'
public Keyword getHasKeyword_2_1_0() { return cHasKeyword_2_1_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_2_1_1() { return cDisjointAssignment_2_1_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_2_1_1_0() { return cDisjointDisjointKeyword_2_1_1_0; }
//'children'
public Keyword getChildrenKeyword_2_1_2() { return cChildrenKeyword_2_1_2; }
//children=ConceptList
public Assignment getChildrenAssignment_2_1_3() { return cChildrenAssignment_2_1_3; }
//ConceptList
public RuleCall getChildrenConceptListParserRuleCall_2_1_3_0() { return cChildrenConceptListParserRuleCall_2_1_3_0; }
}
public class SubConceptStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.SubConceptStatement");
private final UnorderedGroup cUnorderedGroup = (UnorderedGroup)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cUnorderedGroup.eContents().get(0);
private final Assignment cAnnotationsAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final RuleCall cAnnotationsAnnotationParserRuleCall_0_0_0 = (RuleCall)cAnnotationsAssignment_0_0.eContents().get(0);
private final Assignment cAbstractAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final Keyword cAbstractAbstractKeyword_0_1_0 = (Keyword)cAbstractAssignment_0_1.eContents().get(0);
private final Group cGroup_0_2 = (Group)cGroup_0.eContents().get(2);
private final Assignment cDeclarationAssignment_0_2_0 = (Assignment)cGroup_0_2.eContents().get(0);
private final RuleCall cDeclarationValidIDParserRuleCall_0_2_0_0 = (RuleCall)cDeclarationAssignment_0_2_0.eContents().get(0);
private final Group cGroup_0_2_1 = (Group)cGroup_0_2.eContents().get(1);
private final Keyword cIdentifiedKeyword_0_2_1_0 = (Keyword)cGroup_0_2_1.eContents().get(0);
private final Keyword cAsKeyword_0_2_1_1 = (Keyword)cGroup_0_2_1.eContents().get(1);
private final Assignment cIdentifierAssignment_0_2_1_2 = (Assignment)cGroup_0_2_1.eContents().get(2);
private final RuleCall cIdentifierSTRINGTerminalRuleCall_0_2_1_2_0 = (RuleCall)cIdentifierAssignment_0_2_1_2.eContents().get(0);
private final Keyword cByKeyword_0_2_1_3 = (Keyword)cGroup_0_2_1.eContents().get(3);
private final Assignment cAuthorityAssignment_0_2_1_4 = (Assignment)cGroup_0_2_1.eContents().get(4);
private final RuleCall cAuthorityValidIDParserRuleCall_0_2_1_4_0 = (RuleCall)cAuthorityAssignment_0_2_1_4.eContents().get(0);
private final Group cGroup_0_2_2 = (Group)cGroup_0_2.eContents().get(2);
private final Keyword cNamedKeyword_0_2_2_0 = (Keyword)cGroup_0_2_2.eContents().get(0);
private final Assignment cNameAssignment_0_2_2_1 = (Assignment)cGroup_0_2_2.eContents().get(1);
private final Alternatives cNameAlternatives_0_2_2_1_0 = (Alternatives)cNameAssignment_0_2_2_1.eContents().get(0);
private final RuleCall cNameValidIDParserRuleCall_0_2_2_1_0_0 = (RuleCall)cNameAlternatives_0_2_2_1_0.eContents().get(0);
private final RuleCall cNameSTRINGTerminalRuleCall_0_2_2_1_0_1 = (RuleCall)cNameAlternatives_0_2_2_1_0.eContents().get(1);
private final Assignment cDocstringAssignment_0_3 = (Assignment)cGroup_0.eContents().get(3);
private final RuleCall cDocstringSTRINGTerminalRuleCall_0_3_0 = (RuleCall)cDocstringAssignment_0_3.eContents().get(0);
private final Group cGroup_1 = (Group)cUnorderedGroup.eContents().get(1);
private final Keyword cIsKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cParentsAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cParentsConceptListParserRuleCall_1_1_0 = (RuleCall)cParentsAssignment_1_1.eContents().get(0);
private final Group cGroup_2 = (Group)cUnorderedGroup.eContents().get(2);
private final Keyword cRequiresKeyword_2_0 = (Keyword)cGroup_2.eContents().get(0);
private final Assignment cRequirementAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cRequirementIdentityRequirementListParserRuleCall_2_1_0 = (RuleCall)cRequirementAssignment_2_1.eContents().get(0);
private final Group cGroup_3 = (Group)cUnorderedGroup.eContents().get(3);
private final Keyword cInheritsKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cActuallyInheritedTraitsAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cActuallyInheritedTraitsConceptListParserRuleCall_3_1_0 = (RuleCall)cActuallyInheritedTraitsAssignment_3_1.eContents().get(0);
private final Group cGroup_4 = (Group)cUnorderedGroup.eContents().get(4);
private final Keyword cHasKeyword_4_0 = (Keyword)cGroup_4.eContents().get(0);
private final Keyword cRoleKeyword_4_1 = (Keyword)cGroup_4.eContents().get(1);
private final Assignment cRolesAssignment_4_2 = (Assignment)cGroup_4.eContents().get(2);
private final RuleCall cRolesConceptListParserRuleCall_4_2_0 = (RuleCall)cRolesAssignment_4_2.eContents().get(0);
private final Group cGroup_4_3 = (Group)cGroup_4.eContents().get(3);
private final Keyword cForKeyword_4_3_0 = (Keyword)cGroup_4_3.eContents().get(0);
private final Assignment cTargetObservableAssignment_4_3_1 = (Assignment)cGroup_4_3.eContents().get(1);
private final RuleCall cTargetObservableConceptListParserRuleCall_4_3_1_0 = (RuleCall)cTargetObservableAssignment_4_3_1.eContents().get(0);
private final Keyword cInKeyword_4_4 = (Keyword)cGroup_4.eContents().get(4);
private final Assignment cRestrictedObservableAssignment_4_5 = (Assignment)cGroup_4.eContents().get(5);
private final RuleCall cRestrictedObservableConceptListParserRuleCall_4_5_0 = (RuleCall)cRestrictedObservableAssignment_4_5.eContents().get(0);
private final Group cGroup_5 = (Group)cUnorderedGroup.eContents().get(5);
private final Keyword cAffectsKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Assignment cQualitiesAffectedAssignment_5_1 = (Assignment)cGroup_5.eContents().get(1);
private final RuleCall cQualitiesAffectedDerivedConceptListParserRuleCall_5_1_0 = (RuleCall)cQualitiesAffectedAssignment_5_1.eContents().get(0);
private final Group cGroup_6 = (Group)cUnorderedGroup.eContents().get(6);
private final Keyword cCreatesKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Assignment cCreatesAssignment_6_1 = (Assignment)cGroup_6.eContents().get(1);
private final RuleCall cCreatesConceptListParserRuleCall_6_1_0 = (RuleCall)cCreatesAssignment_6_1.eContents().get(0);
private final Group cGroup_7 = (Group)cUnorderedGroup.eContents().get(7);
private final Keyword cAppliesKeyword_7_0 = (Keyword)cGroup_7.eContents().get(0);
private final Keyword cToKeyword_7_1 = (Keyword)cGroup_7.eContents().get(1);
private final Assignment cTraitTargetsAssignment_7_2 = (Assignment)cGroup_7.eContents().get(2);
private final RuleCall cTraitTargetsConceptListParserRuleCall_7_2_0 = (RuleCall)cTraitTargetsAssignment_7_2.eContents().get(0);
private final Group cGroup_8 = (Group)cUnorderedGroup.eContents().get(8);
private final Keyword cConfersKeyword_8_0 = (Keyword)cGroup_8.eContents().get(0);
private final Assignment cConferredTraitsAssignment_8_1 = (Assignment)cGroup_8.eContents().get(1);
private final RuleCall cConferredTraitsConceptListParserRuleCall_8_1_0 = (RuleCall)cConferredTraitsAssignment_8_1.eContents().get(0);
private final Group cGroup_8_2 = (Group)cGroup_8.eContents().get(2);
private final Keyword cToKeyword_8_2_0 = (Keyword)cGroup_8_2.eContents().get(0);
private final Assignment cTargetTypeAssignment_8_2_1 = (Assignment)cGroup_8_2.eContents().get(1);
private final Alternatives cTargetTypeAlternatives_8_2_1_0 = (Alternatives)cTargetTypeAssignment_8_2_1.eContents().get(0);
private final Keyword cTargetTypeSourceKeyword_8_2_1_0_0 = (Keyword)cTargetTypeAlternatives_8_2_1_0.eContents().get(0);
private final Keyword cTargetTypeTargetKeyword_8_2_1_0_1 = (Keyword)cTargetTypeAlternatives_8_2_1_0.eContents().get(1);
private final Assignment cConferredTargetsAssignment_8_2_2 = (Assignment)cGroup_8_2.eContents().get(2);
private final RuleCall cConferredTargetsConceptListParserRuleCall_8_2_2_0 = (RuleCall)cConferredTargetsAssignment_8_2_2.eContents().get(0);
private final Group cGroup_9 = (Group)cUnorderedGroup.eContents().get(9);
private final Keyword cEqualsKeyword_9_0 = (Keyword)cGroup_9.eContents().get(0);
private final Assignment cEquivalencesAssignment_9_1 = (Assignment)cGroup_9.eContents().get(1);
private final RuleCall cEquivalencesConceptListParserRuleCall_9_1_0 = (RuleCall)cEquivalencesAssignment_9_1.eContents().get(0);
private final Assignment cRestrictionsAssignment_10 = (Assignment)cUnorderedGroup.eContents().get(10);
private final RuleCall cRestrictionsRestrictionStatementParserRuleCall_10_0 = (RuleCall)cRestrictionsAssignment_10.eContents().get(0);
private final Group cGroup_11 = (Group)cUnorderedGroup.eContents().get(11);
private final Keyword cHasKeyword_11_0 = (Keyword)cGroup_11.eContents().get(0);
private final Assignment cDisjointAssignment_11_1 = (Assignment)cGroup_11.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_11_1_0 = (Keyword)cDisjointAssignment_11_1.eContents().get(0);
private final Keyword cChildrenKeyword_11_2 = (Keyword)cGroup_11.eContents().get(2);
private final Assignment cChildrenAssignment_11_3 = (Assignment)cGroup_11.eContents().get(3);
private final RuleCall cChildrenConceptListParserRuleCall_11_3_0 = (RuleCall)cChildrenAssignment_11_3.eContents().get(0);
private final Group cGroup_12 = (Group)cUnorderedGroup.eContents().get(12);
private final Keyword cWithKeyword_12_0 = (Keyword)cGroup_12.eContents().get(0);
private final Keyword cMetadataKeyword_12_1 = (Keyword)cGroup_12.eContents().get(1);
private final Assignment cMetadataAssignment_12_2 = (Assignment)cGroup_12.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_12_2_0 = (RuleCall)cMetadataAssignment_12_2.eContents().get(0);
/// *
// * used for child definitions so that subconcept can be defined without having
// * to repeat the concept keyword.
// * /
//SubConceptStatement ConceptStatement:
// annotations+=Annotation* abstract?='abstract'? (declaration=ValidID ('identified' 'as' identifier=STRING 'by'
// authority=ValidID)? ('named' name=(ValidID | STRING))?) docstring=STRING? & ('is' parents=ConceptList)? & ('requires'
// requirement=IdentityRequirementList)? & ('inherits' actuallyInheritedTraits=ConceptList)? & ('has' 'role'
// roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('affects'
// qualitiesAffected=DerivedConceptList)? & ('creates' creates=ConceptList)? & ('applies' 'to'
// traitTargets=ConceptList)? & ('confers' conferredTraits=ConceptList ('to' targetType=('source' | 'target')?
// conferredTargets=ConceptList?)?)? & ('equals' equivalences=ConceptList)? & restrictions+=RestrictionStatement* &
// ('has' disjoint?='disjoint'? 'children' children=ConceptList)? & ('with' 'metadata' metadata=Metadata)?
@Override public ParserRule getRule() { return rule; }
//annotations+=Annotation* abstract?='abstract'? (declaration=ValidID ('identified' 'as' identifier=STRING 'by'
//authority=ValidID)? ('named' name=(ValidID | STRING))?) docstring=STRING? & ('is' parents=ConceptList)? & ('requires'
//requirement=IdentityRequirementList)? & ('inherits' actuallyInheritedTraits=ConceptList)? & ('has' 'role'
//roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)? & ('affects'
//qualitiesAffected=DerivedConceptList)? & ('creates' creates=ConceptList)? & ('applies' 'to' traitTargets=ConceptList)?
//& ('confers' conferredTraits=ConceptList ('to' targetType=('source' | 'target')? conferredTargets=ConceptList?)?)? &
//('equals' equivalences=ConceptList)? & restrictions+=RestrictionStatement* & ('has' disjoint?='disjoint'? 'children'
//children=ConceptList)? & ('with' 'metadata' metadata=Metadata)?
public UnorderedGroup getUnorderedGroup() { return cUnorderedGroup; }
//annotations+=Annotation* abstract?='abstract'? (declaration=ValidID ('identified' 'as' identifier=STRING 'by'
//authority=ValidID)? ('named' name=(ValidID | STRING))?) docstring=STRING?
public Group getGroup_0() { return cGroup_0; }
//annotations+=Annotation*
public Assignment getAnnotationsAssignment_0_0() { return cAnnotationsAssignment_0_0; }
//Annotation
public RuleCall getAnnotationsAnnotationParserRuleCall_0_0_0() { return cAnnotationsAnnotationParserRuleCall_0_0_0; }
//abstract?='abstract'?
public Assignment getAbstractAssignment_0_1() { return cAbstractAssignment_0_1; }
//'abstract'
public Keyword getAbstractAbstractKeyword_0_1_0() { return cAbstractAbstractKeyword_0_1_0; }
//(declaration=ValidID ('identified' 'as' identifier=STRING 'by' authority=ValidID)? ('named' name=(ValidID | STRING))?)
public Group getGroup_0_2() { return cGroup_0_2; }
//declaration=ValidID
public Assignment getDeclarationAssignment_0_2_0() { return cDeclarationAssignment_0_2_0; }
//ValidID
public RuleCall getDeclarationValidIDParserRuleCall_0_2_0_0() { return cDeclarationValidIDParserRuleCall_0_2_0_0; }
//('identified' 'as' identifier=STRING 'by' authority=ValidID)?
public Group getGroup_0_2_1() { return cGroup_0_2_1; }
//'identified'
public Keyword getIdentifiedKeyword_0_2_1_0() { return cIdentifiedKeyword_0_2_1_0; }
//'as'
public Keyword getAsKeyword_0_2_1_1() { return cAsKeyword_0_2_1_1; }
//identifier=STRING
public Assignment getIdentifierAssignment_0_2_1_2() { return cIdentifierAssignment_0_2_1_2; }
//STRING
public RuleCall getIdentifierSTRINGTerminalRuleCall_0_2_1_2_0() { return cIdentifierSTRINGTerminalRuleCall_0_2_1_2_0; }
//'by'
public Keyword getByKeyword_0_2_1_3() { return cByKeyword_0_2_1_3; }
//authority=ValidID
public Assignment getAuthorityAssignment_0_2_1_4() { return cAuthorityAssignment_0_2_1_4; }
//ValidID
public RuleCall getAuthorityValidIDParserRuleCall_0_2_1_4_0() { return cAuthorityValidIDParserRuleCall_0_2_1_4_0; }
//('named' name=(ValidID | STRING))?
public Group getGroup_0_2_2() { return cGroup_0_2_2; }
//'named'
public Keyword getNamedKeyword_0_2_2_0() { return cNamedKeyword_0_2_2_0; }
//name=(ValidID | STRING)
public Assignment getNameAssignment_0_2_2_1() { return cNameAssignment_0_2_2_1; }
//(ValidID | STRING)
public Alternatives getNameAlternatives_0_2_2_1_0() { return cNameAlternatives_0_2_2_1_0; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_0_2_2_1_0_0() { return cNameValidIDParserRuleCall_0_2_2_1_0_0; }
//STRING
public RuleCall getNameSTRINGTerminalRuleCall_0_2_2_1_0_1() { return cNameSTRINGTerminalRuleCall_0_2_2_1_0_1; }
//docstring=STRING?
public Assignment getDocstringAssignment_0_3() { return cDocstringAssignment_0_3; }
//STRING
public RuleCall getDocstringSTRINGTerminalRuleCall_0_3_0() { return cDocstringSTRINGTerminalRuleCall_0_3_0; }
//('is' parents=ConceptList)?
public Group getGroup_1() { return cGroup_1; }
//'is'
public Keyword getIsKeyword_1_0() { return cIsKeyword_1_0; }
//parents=ConceptList
public Assignment getParentsAssignment_1_1() { return cParentsAssignment_1_1; }
//ConceptList
public RuleCall getParentsConceptListParserRuleCall_1_1_0() { return cParentsConceptListParserRuleCall_1_1_0; }
//('requires' requirement=IdentityRequirementList)?
public Group getGroup_2() { return cGroup_2; }
//'requires'
public Keyword getRequiresKeyword_2_0() { return cRequiresKeyword_2_0; }
//requirement=IdentityRequirementList
public Assignment getRequirementAssignment_2_1() { return cRequirementAssignment_2_1; }
//IdentityRequirementList
public RuleCall getRequirementIdentityRequirementListParserRuleCall_2_1_0() { return cRequirementIdentityRequirementListParserRuleCall_2_1_0; }
//('inherits' actuallyInheritedTraits=ConceptList)?
public Group getGroup_3() { return cGroup_3; }
//'inherits'
public Keyword getInheritsKeyword_3_0() { return cInheritsKeyword_3_0; }
//actuallyInheritedTraits=ConceptList
public Assignment getActuallyInheritedTraitsAssignment_3_1() { return cActuallyInheritedTraitsAssignment_3_1; }
//ConceptList
public RuleCall getActuallyInheritedTraitsConceptListParserRuleCall_3_1_0() { return cActuallyInheritedTraitsConceptListParserRuleCall_3_1_0; }
//('has' 'role' roles=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList)?
public Group getGroup_4() { return cGroup_4; }
//'has'
public Keyword getHasKeyword_4_0() { return cHasKeyword_4_0; }
//'role'
public Keyword getRoleKeyword_4_1() { return cRoleKeyword_4_1; }
//roles=ConceptList
public Assignment getRolesAssignment_4_2() { return cRolesAssignment_4_2; }
//ConceptList
public RuleCall getRolesConceptListParserRuleCall_4_2_0() { return cRolesConceptListParserRuleCall_4_2_0; }
//('for' targetObservable=ConceptList)?
public Group getGroup_4_3() { return cGroup_4_3; }
//'for'
public Keyword getForKeyword_4_3_0() { return cForKeyword_4_3_0; }
//targetObservable=ConceptList
public Assignment getTargetObservableAssignment_4_3_1() { return cTargetObservableAssignment_4_3_1; }
//ConceptList
public RuleCall getTargetObservableConceptListParserRuleCall_4_3_1_0() { return cTargetObservableConceptListParserRuleCall_4_3_1_0; }
//'in'
public Keyword getInKeyword_4_4() { return cInKeyword_4_4; }
//restrictedObservable=ConceptList
public Assignment getRestrictedObservableAssignment_4_5() { return cRestrictedObservableAssignment_4_5; }
//ConceptList
public RuleCall getRestrictedObservableConceptListParserRuleCall_4_5_0() { return cRestrictedObservableConceptListParserRuleCall_4_5_0; }
//('affects' qualitiesAffected=DerivedConceptList)?
public Group getGroup_5() { return cGroup_5; }
//'affects'
public Keyword getAffectsKeyword_5_0() { return cAffectsKeyword_5_0; }
//qualitiesAffected=DerivedConceptList
public Assignment getQualitiesAffectedAssignment_5_1() { return cQualitiesAffectedAssignment_5_1; }
//DerivedConceptList
public RuleCall getQualitiesAffectedDerivedConceptListParserRuleCall_5_1_0() { return cQualitiesAffectedDerivedConceptListParserRuleCall_5_1_0; }
//('creates' creates=ConceptList)?
public Group getGroup_6() { return cGroup_6; }
//'creates'
public Keyword getCreatesKeyword_6_0() { return cCreatesKeyword_6_0; }
//creates=ConceptList
public Assignment getCreatesAssignment_6_1() { return cCreatesAssignment_6_1; }
//ConceptList
public RuleCall getCreatesConceptListParserRuleCall_6_1_0() { return cCreatesConceptListParserRuleCall_6_1_0; }
//('applies' 'to' traitTargets=ConceptList)?
public Group getGroup_7() { return cGroup_7; }
//'applies'
public Keyword getAppliesKeyword_7_0() { return cAppliesKeyword_7_0; }
//'to'
public Keyword getToKeyword_7_1() { return cToKeyword_7_1; }
//traitTargets=ConceptList
public Assignment getTraitTargetsAssignment_7_2() { return cTraitTargetsAssignment_7_2; }
//ConceptList
public RuleCall getTraitTargetsConceptListParserRuleCall_7_2_0() { return cTraitTargetsConceptListParserRuleCall_7_2_0; }
//('confers' conferredTraits=ConceptList ('to' targetType=('source' | 'target')? conferredTargets=ConceptList?)?)?
public Group getGroup_8() { return cGroup_8; }
//'confers'
public Keyword getConfersKeyword_8_0() { return cConfersKeyword_8_0; }
//conferredTraits=ConceptList
public Assignment getConferredTraitsAssignment_8_1() { return cConferredTraitsAssignment_8_1; }
//ConceptList
public RuleCall getConferredTraitsConceptListParserRuleCall_8_1_0() { return cConferredTraitsConceptListParserRuleCall_8_1_0; }
//('to' targetType=('source' | 'target')? conferredTargets=ConceptList?)?
public Group getGroup_8_2() { return cGroup_8_2; }
//'to'
public Keyword getToKeyword_8_2_0() { return cToKeyword_8_2_0; }
//targetType=('source' | 'target')?
public Assignment getTargetTypeAssignment_8_2_1() { return cTargetTypeAssignment_8_2_1; }
//('source' | 'target')
public Alternatives getTargetTypeAlternatives_8_2_1_0() { return cTargetTypeAlternatives_8_2_1_0; }
//'source'
public Keyword getTargetTypeSourceKeyword_8_2_1_0_0() { return cTargetTypeSourceKeyword_8_2_1_0_0; }
//'target'
public Keyword getTargetTypeTargetKeyword_8_2_1_0_1() { return cTargetTypeTargetKeyword_8_2_1_0_1; }
//conferredTargets=ConceptList?
public Assignment getConferredTargetsAssignment_8_2_2() { return cConferredTargetsAssignment_8_2_2; }
//ConceptList
public RuleCall getConferredTargetsConceptListParserRuleCall_8_2_2_0() { return cConferredTargetsConceptListParserRuleCall_8_2_2_0; }
//('equals' equivalences=ConceptList)?
public Group getGroup_9() { return cGroup_9; }
//'equals'
public Keyword getEqualsKeyword_9_0() { return cEqualsKeyword_9_0; }
//equivalences=ConceptList
public Assignment getEquivalencesAssignment_9_1() { return cEquivalencesAssignment_9_1; }
//ConceptList
public RuleCall getEquivalencesConceptListParserRuleCall_9_1_0() { return cEquivalencesConceptListParserRuleCall_9_1_0; }
//restrictions+=RestrictionStatement*
public Assignment getRestrictionsAssignment_10() { return cRestrictionsAssignment_10; }
//RestrictionStatement
public RuleCall getRestrictionsRestrictionStatementParserRuleCall_10_0() { return cRestrictionsRestrictionStatementParserRuleCall_10_0; }
//('has' disjoint?='disjoint'? 'children' children=ConceptList)?
public Group getGroup_11() { return cGroup_11; }
//'has'
public Keyword getHasKeyword_11_0() { return cHasKeyword_11_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_11_1() { return cDisjointAssignment_11_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_11_1_0() { return cDisjointDisjointKeyword_11_1_0; }
//'children'
public Keyword getChildrenKeyword_11_2() { return cChildrenKeyword_11_2; }
//children=ConceptList
public Assignment getChildrenAssignment_11_3() { return cChildrenAssignment_11_3; }
//ConceptList
public RuleCall getChildrenConceptListParserRuleCall_11_3_0() { return cChildrenConceptListParserRuleCall_11_3_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_12() { return cGroup_12; }
//'with'
public Keyword getWithKeyword_12_0() { return cWithKeyword_12_0; }
//'metadata'
public Keyword getMetadataKeyword_12_1() { return cMetadataKeyword_12_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_12_2() { return cMetadataAssignment_12_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_12_2_0() { return cMetadataMetadataParserRuleCall_12_2_0; }
}
public class IdentityRequirementListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.IdentityRequirementList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cRequirementAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cRequirementIdentityRequirementParserRuleCall_0_0 = (RuleCall)cRequirementAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cRequirementAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cRequirementIdentityRequirementParserRuleCall_1_1_0 = (RuleCall)cRequirementAssignment_1_1.eContents().get(0);
//IdentityRequirementList:
// requirement+=IdentityRequirement (',' requirement+=IdentityRequirement)*;
@Override public ParserRule getRule() { return rule; }
//requirement+=IdentityRequirement (',' requirement+=IdentityRequirement)*
public Group getGroup() { return cGroup; }
//requirement+=IdentityRequirement
public Assignment getRequirementAssignment_0() { return cRequirementAssignment_0; }
//IdentityRequirement
public RuleCall getRequirementIdentityRequirementParserRuleCall_0_0() { return cRequirementIdentityRequirementParserRuleCall_0_0; }
//(',' requirement+=IdentityRequirement)*
public Group getGroup_1() { return cGroup_1; }
//','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//requirement+=IdentityRequirement
public Assignment getRequirementAssignment_1_1() { return cRequirementAssignment_1_1; }
//IdentityRequirement
public RuleCall getRequirementIdentityRequirementParserRuleCall_1_1_0() { return cRequirementIdentityRequirementParserRuleCall_1_1_0; }
}
public class IdentityRequirementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.IdentityRequirement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Keyword cIdentityKeyword_0_0 = (Keyword)cAlternatives_0.eContents().get(0);
private final Keyword cAttributeKeyword_0_1 = (Keyword)cAlternatives_0.eContents().get(1);
private final Keyword cRealmKeyword_0_2 = (Keyword)cAlternatives_0.eContents().get(2);
private final Keyword cExtentKeyword_0_3 = (Keyword)cAlternatives_0.eContents().get(3);
private final Keyword cAuthorityKeyword_0_4 = (Keyword)cAlternatives_0.eContents().get(4);
private final Assignment cIdentityAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cIdentityConceptListParserRuleCall_1_0 = (RuleCall)cIdentityAssignment_1.eContents().get(0);
//IdentityRequirement:
// ('identity' | 'attribute' | 'realm' | 'extent' | 'authority') identity=ConceptList;
@Override public ParserRule getRule() { return rule; }
//('identity' | 'attribute' | 'realm' | 'extent' | 'authority') identity=ConceptList
public Group getGroup() { return cGroup; }
//('identity' | 'attribute' | 'realm' | 'extent' | 'authority')
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//'identity'
public Keyword getIdentityKeyword_0_0() { return cIdentityKeyword_0_0; }
//'attribute'
public Keyword getAttributeKeyword_0_1() { return cAttributeKeyword_0_1; }
//'realm'
public Keyword getRealmKeyword_0_2() { return cRealmKeyword_0_2; }
//'extent'
public Keyword getExtentKeyword_0_3() { return cExtentKeyword_0_3; }
//'authority'
public Keyword getAuthorityKeyword_0_4() { return cAuthorityKeyword_0_4; }
//identity=ConceptList
public Assignment getIdentityAssignment_1() { return cIdentityAssignment_1; }
//ConceptList
public RuleCall getIdentityConceptListParserRuleCall_1_0() { return cIdentityConceptListParserRuleCall_1_0; }
}
public class ConceptIdentifierElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ConceptIdentifier");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cNegatedAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final Alternatives cNegatedAlternatives_0_0 = (Alternatives)cNegatedAssignment_0.eContents().get(0);
private final Keyword cNegatedNoKeyword_0_0_0 = (Keyword)cNegatedAlternatives_0_0.eContents().get(0);
private final Keyword cNegatedNotKeyword_0_0_1 = (Keyword)cNegatedAlternatives_0_0.eContents().get(1);
private final Assignment cIdAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cIdValidIDParserRuleCall_1_0 = (RuleCall)cIdAssignment_1.eContents().get(0);
//ConceptIdentifier:
// negated?=('no' | 'not')? id=ValidID;
@Override public ParserRule getRule() { return rule; }
//negated?=('no' | 'not')? id=ValidID
public Group getGroup() { return cGroup; }
//negated?=('no' | 'not')?
public Assignment getNegatedAssignment_0() { return cNegatedAssignment_0; }
//('no' | 'not')
public Alternatives getNegatedAlternatives_0_0() { return cNegatedAlternatives_0_0; }
//'no'
public Keyword getNegatedNoKeyword_0_0_0() { return cNegatedNoKeyword_0_0_0; }
//'not'
public Keyword getNegatedNotKeyword_0_0_1() { return cNegatedNotKeyword_0_0_1; }
//id=ValidID
public Assignment getIdAssignment_1() { return cIdAssignment_1; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_1_0() { return cIdValidIDParserRuleCall_1_0; }
}
public class DerivedConceptIdentifierElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.DerivedConceptIdentifier");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cPresenceAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final Keyword cPresencePresenceKeyword_0_0_0 = (Keyword)cPresenceAssignment_0_0.eContents().get(0);
private final Keyword cOfKeyword_0_1 = (Keyword)cGroup_0.eContents().get(1);
private final Assignment cIdAssignment_0_2 = (Assignment)cGroup_0.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_0_2_0 = (RuleCall)cIdAssignment_0_2.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cCountAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Keyword cCountCountKeyword_1_0_0 = (Keyword)cCountAssignment_1_0.eContents().get(0);
private final Keyword cOfKeyword_1_1 = (Keyword)cGroup_1.eContents().get(1);
private final Assignment cIdAssignment_1_2 = (Assignment)cGroup_1.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_1_2_0 = (RuleCall)cIdAssignment_1_2.eContents().get(0);
private final Group cGroup_2 = (Group)cAlternatives.eContents().get(2);
private final Assignment cDistanceAssignment_2_0 = (Assignment)cGroup_2.eContents().get(0);
private final Keyword cDistanceDistanceKeyword_2_0_0 = (Keyword)cDistanceAssignment_2_0.eContents().get(0);
private final Alternatives cAlternatives_2_1 = (Alternatives)cGroup_2.eContents().get(1);
private final Keyword cFromKeyword_2_1_0 = (Keyword)cAlternatives_2_1.eContents().get(0);
private final Keyword cToKeyword_2_1_1 = (Keyword)cAlternatives_2_1.eContents().get(1);
private final Assignment cIdAssignment_2_2 = (Assignment)cGroup_2.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_2_2_0 = (RuleCall)cIdAssignment_2_2.eContents().get(0);
private final Group cGroup_3 = (Group)cAlternatives.eContents().get(3);
private final Assignment cProbabilityAssignment_3_0 = (Assignment)cGroup_3.eContents().get(0);
private final Keyword cProbabilityProbabilityKeyword_3_0_0 = (Keyword)cProbabilityAssignment_3_0.eContents().get(0);
private final Keyword cOfKeyword_3_1 = (Keyword)cGroup_3.eContents().get(1);
private final Assignment cIdAssignment_3_2 = (Assignment)cGroup_3.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_3_2_0 = (RuleCall)cIdAssignment_3_2.eContents().get(0);
private final Group cGroup_4 = (Group)cAlternatives.eContents().get(4);
private final Assignment cUncertaintyAssignment_4_0 = (Assignment)cGroup_4.eContents().get(0);
private final Keyword cUncertaintyUncertaintyKeyword_4_0_0 = (Keyword)cUncertaintyAssignment_4_0.eContents().get(0);
private final Keyword cOfKeyword_4_1 = (Keyword)cGroup_4.eContents().get(1);
private final Assignment cIdAssignment_4_2 = (Assignment)cGroup_4.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_4_2_0 = (RuleCall)cIdAssignment_4_2.eContents().get(0);
private final Group cGroup_5 = (Group)cAlternatives.eContents().get(5);
private final Assignment cProportionAssignment_5_0 = (Assignment)cGroup_5.eContents().get(0);
private final Alternatives cProportionAlternatives_5_0_0 = (Alternatives)cProportionAssignment_5_0.eContents().get(0);
private final Keyword cProportionProportionKeyword_5_0_0_0 = (Keyword)cProportionAlternatives_5_0_0.eContents().get(0);
private final Keyword cProportionPercentageKeyword_5_0_0_1 = (Keyword)cProportionAlternatives_5_0_0.eContents().get(1);
private final Keyword cOfKeyword_5_1 = (Keyword)cGroup_5.eContents().get(1);
private final Assignment cIdAssignment_5_2 = (Assignment)cGroup_5.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_5_2_0 = (RuleCall)cIdAssignment_5_2.eContents().get(0);
private final Keyword cInKeyword_5_3 = (Keyword)cGroup_5.eContents().get(3);
private final Assignment cId2Assignment_5_4 = (Assignment)cGroup_5.eContents().get(4);
private final RuleCall cId2ValidIDParserRuleCall_5_4_0 = (RuleCall)cId2Assignment_5_4.eContents().get(0);
private final Group cGroup_6 = (Group)cAlternatives.eContents().get(6);
private final Assignment cRatioAssignment_6_0 = (Assignment)cGroup_6.eContents().get(0);
private final Keyword cRatioRatioKeyword_6_0_0 = (Keyword)cRatioAssignment_6_0.eContents().get(0);
private final Keyword cOfKeyword_6_1 = (Keyword)cGroup_6.eContents().get(1);
private final Assignment cIdAssignment_6_2 = (Assignment)cGroup_6.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_6_2_0 = (RuleCall)cIdAssignment_6_2.eContents().get(0);
private final Keyword cToKeyword_6_3 = (Keyword)cGroup_6.eContents().get(3);
private final Assignment cId2Assignment_6_4 = (Assignment)cGroup_6.eContents().get(4);
private final RuleCall cId2ValidIDParserRuleCall_6_4_0 = (RuleCall)cId2Assignment_6_4.eContents().get(0);
private final Group cGroup_7 = (Group)cAlternatives.eContents().get(7);
private final Assignment cValueAssignment_7_0 = (Assignment)cGroup_7.eContents().get(0);
private final Keyword cValueValueKeyword_7_0_0 = (Keyword)cValueAssignment_7_0.eContents().get(0);
private final Keyword cOfKeyword_7_1 = (Keyword)cGroup_7.eContents().get(1);
private final Assignment cIdAssignment_7_2 = (Assignment)cGroup_7.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_7_2_0 = (RuleCall)cIdAssignment_7_2.eContents().get(0);
private final Keyword cOverKeyword_7_3 = (Keyword)cGroup_7.eContents().get(3);
private final Assignment cId2Assignment_7_4 = (Assignment)cGroup_7.eContents().get(4);
private final RuleCall cId2ValidIDParserRuleCall_7_4_0 = (RuleCall)cId2Assignment_7_4.eContents().get(0);
private final Assignment cIdAssignment_8 = (Assignment)cAlternatives.eContents().get(8);
private final RuleCall cIdValidIDParserRuleCall_8_0 = (RuleCall)cIdAssignment_8.eContents().get(0);
/// **
// * Referring to derived concepts created by built-in observations.
// * / DerivedConceptIdentifier ConceptIdentifier:
// presence?='presence' 'of' id=ValidID | count?='count' 'of' id=ValidID | distance?='distance' ('from' | 'to')
// id=ValidID | probability?='probability' 'of' id=ValidID | uncertainty?='uncertainty' 'of' id=ValidID |
// proportion?=('proportion' | 'percentage') 'of' id=ValidID 'in' id2=ValidID | ratio?='ratio' 'of' id=ValidID 'to'
// id2=ValidID | value?='value' 'of' id=ValidID 'over' id2=ValidID | id=ValidID
@Override public ParserRule getRule() { return rule; }
//presence?='presence' 'of' id=ValidID | count?='count' 'of' id=ValidID | distance?='distance' ('from' | 'to') id=ValidID
//| probability?='probability' 'of' id=ValidID | uncertainty?='uncertainty' 'of' id=ValidID | proportion?=('proportion'
//| 'percentage') 'of' id=ValidID 'in' id2=ValidID | ratio?='ratio' 'of' id=ValidID 'to' id2=ValidID | value?='value'
//'of' id=ValidID 'over' id2=ValidID | id=ValidID
public Alternatives getAlternatives() { return cAlternatives; }
//presence?='presence' 'of' id=ValidID
public Group getGroup_0() { return cGroup_0; }
//presence?='presence'
public Assignment getPresenceAssignment_0_0() { return cPresenceAssignment_0_0; }
//'presence'
public Keyword getPresencePresenceKeyword_0_0_0() { return cPresencePresenceKeyword_0_0_0; }
//'of'
public Keyword getOfKeyword_0_1() { return cOfKeyword_0_1; }
//id=ValidID
public Assignment getIdAssignment_0_2() { return cIdAssignment_0_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_0_2_0() { return cIdValidIDParserRuleCall_0_2_0; }
//count?='count' 'of' id=ValidID
public Group getGroup_1() { return cGroup_1; }
//count?='count'
public Assignment getCountAssignment_1_0() { return cCountAssignment_1_0; }
//'count'
public Keyword getCountCountKeyword_1_0_0() { return cCountCountKeyword_1_0_0; }
//'of'
public Keyword getOfKeyword_1_1() { return cOfKeyword_1_1; }
//id=ValidID
public Assignment getIdAssignment_1_2() { return cIdAssignment_1_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_1_2_0() { return cIdValidIDParserRuleCall_1_2_0; }
//distance?='distance' ('from' | 'to') id=ValidID
public Group getGroup_2() { return cGroup_2; }
//distance?='distance'
public Assignment getDistanceAssignment_2_0() { return cDistanceAssignment_2_0; }
//'distance'
public Keyword getDistanceDistanceKeyword_2_0_0() { return cDistanceDistanceKeyword_2_0_0; }
//('from' | 'to')
public Alternatives getAlternatives_2_1() { return cAlternatives_2_1; }
//'from'
public Keyword getFromKeyword_2_1_0() { return cFromKeyword_2_1_0; }
//'to'
public Keyword getToKeyword_2_1_1() { return cToKeyword_2_1_1; }
//id=ValidID
public Assignment getIdAssignment_2_2() { return cIdAssignment_2_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_2_2_0() { return cIdValidIDParserRuleCall_2_2_0; }
//probability?='probability' 'of' id=ValidID
public Group getGroup_3() { return cGroup_3; }
//probability?='probability'
public Assignment getProbabilityAssignment_3_0() { return cProbabilityAssignment_3_0; }
//'probability'
public Keyword getProbabilityProbabilityKeyword_3_0_0() { return cProbabilityProbabilityKeyword_3_0_0; }
//'of'
public Keyword getOfKeyword_3_1() { return cOfKeyword_3_1; }
//id=ValidID
public Assignment getIdAssignment_3_2() { return cIdAssignment_3_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_3_2_0() { return cIdValidIDParserRuleCall_3_2_0; }
//uncertainty?='uncertainty' 'of' id=ValidID
public Group getGroup_4() { return cGroup_4; }
//uncertainty?='uncertainty'
public Assignment getUncertaintyAssignment_4_0() { return cUncertaintyAssignment_4_0; }
//'uncertainty'
public Keyword getUncertaintyUncertaintyKeyword_4_0_0() { return cUncertaintyUncertaintyKeyword_4_0_0; }
//'of'
public Keyword getOfKeyword_4_1() { return cOfKeyword_4_1; }
//id=ValidID
public Assignment getIdAssignment_4_2() { return cIdAssignment_4_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_4_2_0() { return cIdValidIDParserRuleCall_4_2_0; }
//proportion?=('proportion' | 'percentage') 'of' id=ValidID 'in' id2=ValidID
public Group getGroup_5() { return cGroup_5; }
//proportion?=('proportion' | 'percentage')
public Assignment getProportionAssignment_5_0() { return cProportionAssignment_5_0; }
//('proportion' | 'percentage')
public Alternatives getProportionAlternatives_5_0_0() { return cProportionAlternatives_5_0_0; }
//'proportion'
public Keyword getProportionProportionKeyword_5_0_0_0() { return cProportionProportionKeyword_5_0_0_0; }
//'percentage'
public Keyword getProportionPercentageKeyword_5_0_0_1() { return cProportionPercentageKeyword_5_0_0_1; }
//'of'
public Keyword getOfKeyword_5_1() { return cOfKeyword_5_1; }
//id=ValidID
public Assignment getIdAssignment_5_2() { return cIdAssignment_5_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_5_2_0() { return cIdValidIDParserRuleCall_5_2_0; }
//'in'
public Keyword getInKeyword_5_3() { return cInKeyword_5_3; }
//id2=ValidID
public Assignment getId2Assignment_5_4() { return cId2Assignment_5_4; }
//ValidID
public RuleCall getId2ValidIDParserRuleCall_5_4_0() { return cId2ValidIDParserRuleCall_5_4_0; }
//ratio?='ratio' 'of' id=ValidID 'to' id2=ValidID
public Group getGroup_6() { return cGroup_6; }
//ratio?='ratio'
public Assignment getRatioAssignment_6_0() { return cRatioAssignment_6_0; }
//'ratio'
public Keyword getRatioRatioKeyword_6_0_0() { return cRatioRatioKeyword_6_0_0; }
//'of'
public Keyword getOfKeyword_6_1() { return cOfKeyword_6_1; }
//id=ValidID
public Assignment getIdAssignment_6_2() { return cIdAssignment_6_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_6_2_0() { return cIdValidIDParserRuleCall_6_2_0; }
//'to'
public Keyword getToKeyword_6_3() { return cToKeyword_6_3; }
//id2=ValidID
public Assignment getId2Assignment_6_4() { return cId2Assignment_6_4; }
//ValidID
public RuleCall getId2ValidIDParserRuleCall_6_4_0() { return cId2ValidIDParserRuleCall_6_4_0; }
//value?='value' 'of' id=ValidID 'over' id2=ValidID
public Group getGroup_7() { return cGroup_7; }
//value?='value'
public Assignment getValueAssignment_7_0() { return cValueAssignment_7_0; }
//'value'
public Keyword getValueValueKeyword_7_0_0() { return cValueValueKeyword_7_0_0; }
//'of'
public Keyword getOfKeyword_7_1() { return cOfKeyword_7_1; }
//id=ValidID
public Assignment getIdAssignment_7_2() { return cIdAssignment_7_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_7_2_0() { return cIdValidIDParserRuleCall_7_2_0; }
//'over'
public Keyword getOverKeyword_7_3() { return cOverKeyword_7_3; }
//id2=ValidID
public Assignment getId2Assignment_7_4() { return cId2Assignment_7_4; }
//ValidID
public RuleCall getId2ValidIDParserRuleCall_7_4_0() { return cId2ValidIDParserRuleCall_7_4_0; }
//id=ValidID
public Assignment getIdAssignment_8() { return cIdAssignment_8; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_8_0() { return cIdValidIDParserRuleCall_8_0; }
}
public class ConceptDeclarationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ConceptDeclaration");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cParentAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final Keyword cParentParentKeyword_0_0 = (Keyword)cParentAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cIdsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final RuleCall cIdsConceptIdentifierParserRuleCall_1_0_0 = (RuleCall)cIdsAssignment_1_0.eContents().get(0);
private final Assignment cIdsAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cIdsConceptIdentifierParserRuleCall_1_1_0 = (RuleCall)cIdsAssignment_1_1.eContents().get(0);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Keyword cOfKeyword_1_2_0 = (Keyword)cGroup_1_2.eContents().get(0);
private final Assignment cInherentAssignment_1_2_1 = (Assignment)cGroup_1_2.eContents().get(1);
private final RuleCall cInherentConceptIdentifierParserRuleCall_1_2_1_0 = (RuleCall)cInherentAssignment_1_2_1.eContents().get(0);
private final Assignment cOuterContextAssignment_1_3 = (Assignment)cGroup_1.eContents().get(3);
private final RuleCall cOuterContextOuterContextParserRuleCall_1_3_0 = (RuleCall)cOuterContextAssignment_1_3.eContents().get(0);
private final Group cGroup_1_4 = (Group)cGroup_1.eContents().get(4);
private final Keyword cDownKeyword_1_4_0 = (Keyword)cGroup_1_4.eContents().get(0);
private final Keyword cToKeyword_1_4_1 = (Keyword)cGroup_1_4.eContents().get(1);
private final Assignment cDownToAssignment_1_4_2 = (Assignment)cGroup_1_4.eContents().get(2);
private final RuleCall cDownToValidIDParserRuleCall_1_4_2_0 = (RuleCall)cDownToAssignment_1_4_2.eContents().get(0);
/// *
// * concept with optional traits. Accepts 'parent' only in child lists for orderings
// * and 'down to' only in 'exposes' statements for concrete classes.
// * /
//ConceptDeclaration:
// parent?='parent' | ids+=ConceptIdentifier => ids+=ConceptIdentifier* ('of' inherent+=ConceptIdentifier*)?
// outerContext=OuterContext? ('down' 'to' downTo=ValidID)?;
@Override public ParserRule getRule() { return rule; }
//parent?='parent' | ids+=ConceptIdentifier => ids+=ConceptIdentifier* ('of' inherent+=ConceptIdentifier*)?
//outerContext=OuterContext? ('down' 'to' downTo=ValidID)?
public Alternatives getAlternatives() { return cAlternatives; }
//parent?='parent'
public Assignment getParentAssignment_0() { return cParentAssignment_0; }
//'parent'
public Keyword getParentParentKeyword_0_0() { return cParentParentKeyword_0_0; }
//ids+=ConceptIdentifier => ids+=ConceptIdentifier* ('of' inherent+=ConceptIdentifier*)? outerContext=OuterContext?
//('down' 'to' downTo=ValidID)?
public Group getGroup_1() { return cGroup_1; }
//ids+=ConceptIdentifier
public Assignment getIdsAssignment_1_0() { return cIdsAssignment_1_0; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_1_0_0() { return cIdsConceptIdentifierParserRuleCall_1_0_0; }
//=> ids+=ConceptIdentifier*
public Assignment getIdsAssignment_1_1() { return cIdsAssignment_1_1; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_1_1_0() { return cIdsConceptIdentifierParserRuleCall_1_1_0; }
//('of' inherent+=ConceptIdentifier*)?
public Group getGroup_1_2() { return cGroup_1_2; }
//'of'
public Keyword getOfKeyword_1_2_0() { return cOfKeyword_1_2_0; }
//inherent+=ConceptIdentifier*
public Assignment getInherentAssignment_1_2_1() { return cInherentAssignment_1_2_1; }
//ConceptIdentifier
public RuleCall getInherentConceptIdentifierParserRuleCall_1_2_1_0() { return cInherentConceptIdentifierParserRuleCall_1_2_1_0; }
//outerContext=OuterContext?
public Assignment getOuterContextAssignment_1_3() { return cOuterContextAssignment_1_3; }
//OuterContext
public RuleCall getOuterContextOuterContextParserRuleCall_1_3_0() { return cOuterContextOuterContextParserRuleCall_1_3_0; }
//('down' 'to' downTo=ValidID)?
public Group getGroup_1_4() { return cGroup_1_4; }
//'down'
public Keyword getDownKeyword_1_4_0() { return cDownKeyword_1_4_0; }
//'to'
public Keyword getToKeyword_1_4_1() { return cToKeyword_1_4_1; }
//downTo=ValidID
public Assignment getDownToAssignment_1_4_2() { return cDownToAssignment_1_4_2; }
//ValidID
public RuleCall getDownToValidIDParserRuleCall_1_4_2_0() { return cDownToValidIDParserRuleCall_1_4_2_0; }
}
public class DerivedConceptDeclarationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.DerivedConceptDeclaration");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cParentAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final Keyword cParentParentKeyword_0_0 = (Keyword)cParentAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cIdsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final RuleCall cIdsDerivedConceptIdentifierParserRuleCall_1_0_0 = (RuleCall)cIdsAssignment_1_0.eContents().get(0);
private final Assignment cIdsAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cIdsDerivedConceptIdentifierParserRuleCall_1_1_0 = (RuleCall)cIdsAssignment_1_1.eContents().get(0);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Keyword cOfKeyword_1_2_0 = (Keyword)cGroup_1_2.eContents().get(0);
private final Assignment cInherentAssignment_1_2_1 = (Assignment)cGroup_1_2.eContents().get(1);
private final RuleCall cInherentConceptIdentifierParserRuleCall_1_2_1_0 = (RuleCall)cInherentAssignment_1_2_1.eContents().get(0);
private final Assignment cOuterContextAssignment_1_3 = (Assignment)cGroup_1.eContents().get(3);
private final RuleCall cOuterContextOuterContextParserRuleCall_1_3_0 = (RuleCall)cOuterContextAssignment_1_3.eContents().get(0);
private final Group cGroup_1_4 = (Group)cGroup_1.eContents().get(4);
private final Keyword cDownKeyword_1_4_0 = (Keyword)cGroup_1_4.eContents().get(0);
private final Keyword cToKeyword_1_4_1 = (Keyword)cGroup_1_4.eContents().get(1);
private final Assignment cDownToAssignment_1_4_2 = (Assignment)cGroup_1_4.eContents().get(2);
private final RuleCall cDownToValidIDParserRuleCall_1_4_2_0 = (RuleCall)cDownToAssignment_1_4_2.eContents().get(0);
/// *
// * concept with optional traits. Accepts 'parent' only in child lists for orderings
// * and 'down to' only in 'exposes' statements for concrete classes.
// * /
//DerivedConceptDeclaration ConceptDeclaration:
// parent?='parent' | ids+=DerivedConceptIdentifier => ids+=DerivedConceptIdentifier* ('of'
// inherent+=ConceptIdentifier*)? outerContext=OuterContext? ('down' 'to' downTo=ValidID)?
@Override public ParserRule getRule() { return rule; }
//parent?='parent' | ids+=DerivedConceptIdentifier => ids+=DerivedConceptIdentifier* ('of' inherent+=ConceptIdentifier*)?
//outerContext=OuterContext? ('down' 'to' downTo=ValidID)?
public Alternatives getAlternatives() { return cAlternatives; }
//parent?='parent'
public Assignment getParentAssignment_0() { return cParentAssignment_0; }
//'parent'
public Keyword getParentParentKeyword_0_0() { return cParentParentKeyword_0_0; }
//ids+=DerivedConceptIdentifier => ids+=DerivedConceptIdentifier* ('of' inherent+=ConceptIdentifier*)?
//outerContext=OuterContext? ('down' 'to' downTo=ValidID)?
public Group getGroup_1() { return cGroup_1; }
//ids+=DerivedConceptIdentifier
public Assignment getIdsAssignment_1_0() { return cIdsAssignment_1_0; }
//DerivedConceptIdentifier
public RuleCall getIdsDerivedConceptIdentifierParserRuleCall_1_0_0() { return cIdsDerivedConceptIdentifierParserRuleCall_1_0_0; }
//=> ids+=DerivedConceptIdentifier*
public Assignment getIdsAssignment_1_1() { return cIdsAssignment_1_1; }
//DerivedConceptIdentifier
public RuleCall getIdsDerivedConceptIdentifierParserRuleCall_1_1_0() { return cIdsDerivedConceptIdentifierParserRuleCall_1_1_0; }
//('of' inherent+=ConceptIdentifier*)?
public Group getGroup_1_2() { return cGroup_1_2; }
//'of'
public Keyword getOfKeyword_1_2_0() { return cOfKeyword_1_2_0; }
//inherent+=ConceptIdentifier*
public Assignment getInherentAssignment_1_2_1() { return cInherentAssignment_1_2_1; }
//ConceptIdentifier
public RuleCall getInherentConceptIdentifierParserRuleCall_1_2_1_0() { return cInherentConceptIdentifierParserRuleCall_1_2_1_0; }
//outerContext=OuterContext?
public Assignment getOuterContextAssignment_1_3() { return cOuterContextAssignment_1_3; }
//OuterContext
public RuleCall getOuterContextOuterContextParserRuleCall_1_3_0() { return cOuterContextOuterContextParserRuleCall_1_3_0; }
//('down' 'to' downTo=ValidID)?
public Group getGroup_1_4() { return cGroup_1_4; }
//'down'
public Keyword getDownKeyword_1_4_0() { return cDownKeyword_1_4_0; }
//'to'
public Keyword getToKeyword_1_4_1() { return cToKeyword_1_4_1; }
//downTo=ValidID
public Assignment getDownToAssignment_1_4_2() { return cDownToAssignment_1_4_2; }
//ValidID
public RuleCall getDownToValidIDParserRuleCall_1_4_2_0() { return cDownToValidIDParserRuleCall_1_4_2_0; }
}
public class NegatableConceptDeclarationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.NegatableConceptDeclaration");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cNegatedAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final Alternatives cNegatedAlternatives_0_0_0 = (Alternatives)cNegatedAssignment_0_0.eContents().get(0);
private final Keyword cNegatedNoKeyword_0_0_0_0 = (Keyword)cNegatedAlternatives_0_0_0.eContents().get(0);
private final Keyword cNegatedNotKeyword_0_0_0_1 = (Keyword)cNegatedAlternatives_0_0_0.eContents().get(1);
private final Assignment cUnknownAssignment_0_1 = (Assignment)cAlternatives_0.eContents().get(1);
private final Keyword cUnknownUnknownKeyword_0_1_0 = (Keyword)cUnknownAssignment_0_1.eContents().get(0);
private final Assignment cAllAssignment_0_2 = (Assignment)cAlternatives_0.eContents().get(2);
private final Keyword cAllAllKeyword_0_2_0 = (Keyword)cAllAssignment_0_2.eContents().get(0);
private final Assignment cIdsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cIdsConceptIdentifierParserRuleCall_1_0 = (RuleCall)cIdsAssignment_1.eContents().get(0);
private final Assignment cIdsAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cIdsConceptIdentifierParserRuleCall_2_0 = (RuleCall)cIdsAssignment_2.eContents().get(0);
private final Group cGroup_3 = (Group)cGroup.eContents().get(3);
private final Keyword cOfKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cInherentAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cInherentConceptIdentifierParserRuleCall_3_1_0 = (RuleCall)cInherentAssignment_3_1.eContents().get(0);
private final Assignment cOuterContextAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final RuleCall cOuterContextOuterContextParserRuleCall_4_0 = (RuleCall)cOuterContextAssignment_4.eContents().get(0);
/// **
// * Same with possible negation in front.
// * / NegatableConceptDeclaration ConceptDeclaration:
// (negated?=('no' | 'not') | unknown?='unknown' | all?='all')? ids+=ConceptIdentifier => ids+=ConceptIdentifier* ('of'
// inherent+=ConceptIdentifier*)? outerContext=OuterContext?
@Override public ParserRule getRule() { return rule; }
//(negated?=('no' | 'not') | unknown?='unknown' | all?='all')? ids+=ConceptIdentifier => ids+=ConceptIdentifier* ('of'
//inherent+=ConceptIdentifier*)? outerContext=OuterContext?
public Group getGroup() { return cGroup; }
//(negated?=('no' | 'not') | unknown?='unknown' | all?='all')?
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//negated?=('no' | 'not')
public Assignment getNegatedAssignment_0_0() { return cNegatedAssignment_0_0; }
//('no' | 'not')
public Alternatives getNegatedAlternatives_0_0_0() { return cNegatedAlternatives_0_0_0; }
//'no'
public Keyword getNegatedNoKeyword_0_0_0_0() { return cNegatedNoKeyword_0_0_0_0; }
//'not'
public Keyword getNegatedNotKeyword_0_0_0_1() { return cNegatedNotKeyword_0_0_0_1; }
//unknown?='unknown'
public Assignment getUnknownAssignment_0_1() { return cUnknownAssignment_0_1; }
//'unknown'
public Keyword getUnknownUnknownKeyword_0_1_0() { return cUnknownUnknownKeyword_0_1_0; }
//all?='all'
public Assignment getAllAssignment_0_2() { return cAllAssignment_0_2; }
//'all'
public Keyword getAllAllKeyword_0_2_0() { return cAllAllKeyword_0_2_0; }
//ids+=ConceptIdentifier
public Assignment getIdsAssignment_1() { return cIdsAssignment_1; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_1_0() { return cIdsConceptIdentifierParserRuleCall_1_0; }
//=> ids+=ConceptIdentifier*
public Assignment getIdsAssignment_2() { return cIdsAssignment_2; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_2_0() { return cIdsConceptIdentifierParserRuleCall_2_0; }
//('of' inherent+=ConceptIdentifier*)?
public Group getGroup_3() { return cGroup_3; }
//'of'
public Keyword getOfKeyword_3_0() { return cOfKeyword_3_0; }
//inherent+=ConceptIdentifier*
public Assignment getInherentAssignment_3_1() { return cInherentAssignment_3_1; }
//ConceptIdentifier
public RuleCall getInherentConceptIdentifierParserRuleCall_3_1_0() { return cInherentConceptIdentifierParserRuleCall_3_1_0; }
//outerContext=OuterContext?
public Assignment getOuterContextAssignment_4() { return cOuterContextAssignment_4; }
//OuterContext
public RuleCall getOuterContextOuterContextParserRuleCall_4_0() { return cOuterContextOuterContextParserRuleCall_4_0; }
}
public class NegatableDerivedConceptDeclarationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.NegatableDerivedConceptDeclaration");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cNegatedAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final Alternatives cNegatedAlternatives_0_0_0 = (Alternatives)cNegatedAssignment_0_0.eContents().get(0);
private final Keyword cNegatedNoKeyword_0_0_0_0 = (Keyword)cNegatedAlternatives_0_0_0.eContents().get(0);
private final Keyword cNegatedNotKeyword_0_0_0_1 = (Keyword)cNegatedAlternatives_0_0_0.eContents().get(1);
private final Assignment cUnknownAssignment_0_1 = (Assignment)cAlternatives_0.eContents().get(1);
private final Keyword cUnknownUnknownKeyword_0_1_0 = (Keyword)cUnknownAssignment_0_1.eContents().get(0);
private final Assignment cAllAssignment_0_2 = (Assignment)cAlternatives_0.eContents().get(2);
private final Keyword cAllAllKeyword_0_2_0 = (Keyword)cAllAssignment_0_2.eContents().get(0);
private final Assignment cIdsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cIdsDerivedConceptIdentifierParserRuleCall_1_0 = (RuleCall)cIdsAssignment_1.eContents().get(0);
private final Assignment cIdsAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cIdsDerivedConceptIdentifierParserRuleCall_2_0 = (RuleCall)cIdsAssignment_2.eContents().get(0);
private final Group cGroup_3 = (Group)cGroup.eContents().get(3);
private final Keyword cOfKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cInherentAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cInherentConceptIdentifierParserRuleCall_3_1_0 = (RuleCall)cInherentAssignment_3_1.eContents().get(0);
private final Assignment cOuterContextAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final RuleCall cOuterContextOuterContextParserRuleCall_4_0 = (RuleCall)cOuterContextAssignment_4.eContents().get(0);
/// *
// * Same with compound qualities.
// * / NegatableDerivedConceptDeclaration ConceptDeclaration:
// (negated?=('no' | 'not') | unknown?='unknown' | all?='all')? ids+=DerivedConceptIdentifier =>
// ids+=DerivedConceptIdentifier* ('of' inherent+=ConceptIdentifier*)? outerContext=OuterContext?
@Override public ParserRule getRule() { return rule; }
//(negated?=('no' | 'not') | unknown?='unknown' | all?='all')? ids+=DerivedConceptIdentifier =>
//ids+=DerivedConceptIdentifier* ('of' inherent+=ConceptIdentifier*)? outerContext=OuterContext?
public Group getGroup() { return cGroup; }
//(negated?=('no' | 'not') | unknown?='unknown' | all?='all')?
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//negated?=('no' | 'not')
public Assignment getNegatedAssignment_0_0() { return cNegatedAssignment_0_0; }
//('no' | 'not')
public Alternatives getNegatedAlternatives_0_0_0() { return cNegatedAlternatives_0_0_0; }
//'no'
public Keyword getNegatedNoKeyword_0_0_0_0() { return cNegatedNoKeyword_0_0_0_0; }
//'not'
public Keyword getNegatedNotKeyword_0_0_0_1() { return cNegatedNotKeyword_0_0_0_1; }
//unknown?='unknown'
public Assignment getUnknownAssignment_0_1() { return cUnknownAssignment_0_1; }
//'unknown'
public Keyword getUnknownUnknownKeyword_0_1_0() { return cUnknownUnknownKeyword_0_1_0; }
//all?='all'
public Assignment getAllAssignment_0_2() { return cAllAssignment_0_2; }
//'all'
public Keyword getAllAllKeyword_0_2_0() { return cAllAllKeyword_0_2_0; }
//ids+=DerivedConceptIdentifier
public Assignment getIdsAssignment_1() { return cIdsAssignment_1; }
//DerivedConceptIdentifier
public RuleCall getIdsDerivedConceptIdentifierParserRuleCall_1_0() { return cIdsDerivedConceptIdentifierParserRuleCall_1_0; }
//=> ids+=DerivedConceptIdentifier*
public Assignment getIdsAssignment_2() { return cIdsAssignment_2; }
//DerivedConceptIdentifier
public RuleCall getIdsDerivedConceptIdentifierParserRuleCall_2_0() { return cIdsDerivedConceptIdentifierParserRuleCall_2_0; }
//('of' inherent+=ConceptIdentifier*)?
public Group getGroup_3() { return cGroup_3; }
//'of'
public Keyword getOfKeyword_3_0() { return cOfKeyword_3_0; }
//inherent+=ConceptIdentifier*
public Assignment getInherentAssignment_3_1() { return cInherentAssignment_3_1; }
//ConceptIdentifier
public RuleCall getInherentConceptIdentifierParserRuleCall_3_1_0() { return cInherentConceptIdentifierParserRuleCall_3_1_0; }
//outerContext=OuterContext?
public Assignment getOuterContextAssignment_4() { return cOuterContextAssignment_4; }
//OuterContext
public RuleCall getOuterContextOuterContextParserRuleCall_4_0() { return cOuterContextOuterContextParserRuleCall_4_0; }
}
public class OuterContextElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.OuterContext");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cWithinKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Assignment cIdsAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cIdsConceptIdentifierParserRuleCall_1_0 = (RuleCall)cIdsAssignment_1.eContents().get(0);
private final Assignment cIdsAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cIdsConceptIdentifierParserRuleCall_2_0 = (RuleCall)cIdsAssignment_2.eContents().get(0);
//OuterContext:
// 'within' ids+=ConceptIdentifier => ids+=ConceptIdentifier*;
@Override public ParserRule getRule() { return rule; }
//'within' ids+=ConceptIdentifier => ids+=ConceptIdentifier*
public Group getGroup() { return cGroup; }
//'within'
public Keyword getWithinKeyword_0() { return cWithinKeyword_0; }
//ids+=ConceptIdentifier
public Assignment getIdsAssignment_1() { return cIdsAssignment_1; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_1_0() { return cIdsConceptIdentifierParserRuleCall_1_0; }
//=> ids+=ConceptIdentifier*
public Assignment getIdsAssignment_2() { return cIdsAssignment_2; }
//ConceptIdentifier
public RuleCall getIdsConceptIdentifierParserRuleCall_2_0() { return cIdsConceptIdentifierParserRuleCall_2_0; }
}
public class RestrictionStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.RestrictionStatement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cRelTypeAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final Alternatives cRelTypeAlternatives_0_0_0 = (Alternatives)cRelTypeAssignment_0_0.eContents().get(0);
private final Keyword cRelTypeUsesKeyword_0_0_0_0 = (Keyword)cRelTypeAlternatives_0_0_0.eContents().get(0);
private final Keyword cRelTypeHasKeyword_0_0_0_1 = (Keyword)cRelTypeAlternatives_0_0_0.eContents().get(1);
private final Keyword cRelTypeContainsKeyword_0_0_0_2 = (Keyword)cRelTypeAlternatives_0_0_0.eContents().get(2);
private final Assignment cRelDefsAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final RuleCall cRelDefsRestrictionDefinitionListParserRuleCall_0_1_0 = (RuleCall)cRelDefsAssignment_0_1.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Keyword cUsesKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Keyword cAuthorityKeyword_1_1 = (Keyword)cGroup_1.eContents().get(1);
private final Assignment cAuthoritiesAssignment_1_2 = (Assignment)cGroup_1.eContents().get(2);
private final RuleCall cAuthoritiesValidIDParserRuleCall_1_2_0 = (RuleCall)cAuthoritiesAssignment_1_2.eContents().get(0);
private final Group cGroup_1_3 = (Group)cGroup_1.eContents().get(3);
private final Keyword cCommaKeyword_1_3_0 = (Keyword)cGroup_1_3.eContents().get(0);
private final Assignment cAuthoritiesAssignment_1_3_1 = (Assignment)cGroup_1_3.eContents().get(1);
private final RuleCall cAuthoritiesValidIDParserRuleCall_1_3_1_0 = (RuleCall)cAuthoritiesAssignment_1_3_1.eContents().get(0);
private final Group cGroup_2 = (Group)cAlternatives.eContents().get(2);
private final Keyword cUsesKeyword_2_0 = (Keyword)cGroup_2.eContents().get(0);
private final Assignment cValueAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cValueLiteralParserRuleCall_2_1_0 = (RuleCall)cValueAssignment_2_1.eContents().get(0);
private final Assignment cLiteralAssignment_2_2 = (Assignment)cGroup_2.eContents().get(2);
private final Keyword cLiteralForKeyword_2_2_0 = (Keyword)cLiteralAssignment_2_2.eContents().get(0);
private final Alternatives cAlternatives_2_3 = (Alternatives)cGroup_2.eContents().get(3);
private final Assignment cSubjectAssignment_2_3_0 = (Assignment)cAlternatives_2_3.eContents().get(0);
private final RuleCall cSubjectValidIDParserRuleCall_2_3_0_0 = (RuleCall)cSubjectAssignment_2_3_0.eContents().get(0);
private final Group cGroup_2_3_1 = (Group)cAlternatives_2_3.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_2_3_1_0 = (Keyword)cGroup_2_3_1.eContents().get(0);
private final Assignment cStatementAssignment_2_3_1_1 = (Assignment)cGroup_2_3_1.eContents().get(1);
private final RuleCall cStatementPropertyStatementParserRuleCall_2_3_1_1_0 = (RuleCall)cStatementAssignment_2_3_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_2_3_1_2 = (Keyword)cGroup_2_3_1.eContents().get(2);
/// *
// * Ultra-simple restriction statements:
// *
// * uses [only] CCC [for PPP]; // PPP can be a property or a concept
// * uses at [least|most] 1 CCC [for PPP]
// * uses exactly 1 CCC [for PPP]
// * uses 120 for PPP // data property
// *
// * uses = has; requires = functional has; contains = part-of
// *
// * if PPP is a concept (much more intuitive), must be restricting a known one and hasPPP is created (or used).
// *
// * Also hosts the 'uses authority' restriction which is only accepted by domains.
// *
// * /
//RestrictionStatement:
// relType=('uses' | 'has' | 'contains') relDefs=RestrictionDefinitionList | 'uses' 'authority' authorities+=ValidID (=>
// ',' authorities+=ValidID)* | 'uses' value=Literal literal?='for' (subject=ValidID | '(' statement=PropertyStatement
// ')');
@Override public ParserRule getRule() { return rule; }
//relType=('uses' | 'has' | 'contains') relDefs=RestrictionDefinitionList | 'uses' 'authority' authorities+=ValidID (=>
//',' authorities+=ValidID)* | 'uses' value=Literal literal?='for' (subject=ValidID | '(' statement=PropertyStatement
//')')
public Alternatives getAlternatives() { return cAlternatives; }
//relType=('uses' | 'has' | 'contains') relDefs=RestrictionDefinitionList
public Group getGroup_0() { return cGroup_0; }
//relType=('uses' | 'has' | 'contains')
public Assignment getRelTypeAssignment_0_0() { return cRelTypeAssignment_0_0; }
//('uses' | 'has' | 'contains')
public Alternatives getRelTypeAlternatives_0_0_0() { return cRelTypeAlternatives_0_0_0; }
//'uses'
public Keyword getRelTypeUsesKeyword_0_0_0_0() { return cRelTypeUsesKeyword_0_0_0_0; }
//'has'
public Keyword getRelTypeHasKeyword_0_0_0_1() { return cRelTypeHasKeyword_0_0_0_1; }
//'contains'
public Keyword getRelTypeContainsKeyword_0_0_0_2() { return cRelTypeContainsKeyword_0_0_0_2; }
//relDefs=RestrictionDefinitionList
public Assignment getRelDefsAssignment_0_1() { return cRelDefsAssignment_0_1; }
//RestrictionDefinitionList
public RuleCall getRelDefsRestrictionDefinitionListParserRuleCall_0_1_0() { return cRelDefsRestrictionDefinitionListParserRuleCall_0_1_0; }
//'uses' 'authority' authorities+=ValidID (=> ',' authorities+=ValidID)*
public Group getGroup_1() { return cGroup_1; }
//'uses'
public Keyword getUsesKeyword_1_0() { return cUsesKeyword_1_0; }
//'authority'
public Keyword getAuthorityKeyword_1_1() { return cAuthorityKeyword_1_1; }
//authorities+=ValidID
public Assignment getAuthoritiesAssignment_1_2() { return cAuthoritiesAssignment_1_2; }
//ValidID
public RuleCall getAuthoritiesValidIDParserRuleCall_1_2_0() { return cAuthoritiesValidIDParserRuleCall_1_2_0; }
//(=> ',' authorities+=ValidID)*
public Group getGroup_1_3() { return cGroup_1_3; }
//=> ','
public Keyword getCommaKeyword_1_3_0() { return cCommaKeyword_1_3_0; }
//authorities+=ValidID
public Assignment getAuthoritiesAssignment_1_3_1() { return cAuthoritiesAssignment_1_3_1; }
//ValidID
public RuleCall getAuthoritiesValidIDParserRuleCall_1_3_1_0() { return cAuthoritiesValidIDParserRuleCall_1_3_1_0; }
//'uses' value=Literal literal?='for' (subject=ValidID | '(' statement=PropertyStatement ')')
public Group getGroup_2() { return cGroup_2; }
//'uses'
public Keyword getUsesKeyword_2_0() { return cUsesKeyword_2_0; }
//value=Literal
public Assignment getValueAssignment_2_1() { return cValueAssignment_2_1; }
//Literal
public RuleCall getValueLiteralParserRuleCall_2_1_0() { return cValueLiteralParserRuleCall_2_1_0; }
//literal?='for'
public Assignment getLiteralAssignment_2_2() { return cLiteralAssignment_2_2; }
//'for'
public Keyword getLiteralForKeyword_2_2_0() { return cLiteralForKeyword_2_2_0; }
//(subject=ValidID | '(' statement=PropertyStatement ')')
public Alternatives getAlternatives_2_3() { return cAlternatives_2_3; }
//subject=ValidID
public Assignment getSubjectAssignment_2_3_0() { return cSubjectAssignment_2_3_0; }
//ValidID
public RuleCall getSubjectValidIDParserRuleCall_2_3_0_0() { return cSubjectValidIDParserRuleCall_2_3_0_0; }
//'(' statement=PropertyStatement ')'
public Group getGroup_2_3_1() { return cGroup_2_3_1; }
//'('
public Keyword getLeftParenthesisKeyword_2_3_1_0() { return cLeftParenthesisKeyword_2_3_1_0; }
//statement=PropertyStatement
public Assignment getStatementAssignment_2_3_1_1() { return cStatementAssignment_2_3_1_1; }
//PropertyStatement
public RuleCall getStatementPropertyStatementParserRuleCall_2_3_1_1_0() { return cStatementPropertyStatementParserRuleCall_2_3_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_2_3_1_2() { return cRightParenthesisKeyword_2_3_1_2; }
}
public class RestrictionDefinitionListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.RestrictionDefinitionList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDefinitionsAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDefinitionsRestrictionDefinitionParserRuleCall_0_0 = (RuleCall)cDefinitionsAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cDefinitionsRestrictionDefinitionParserRuleCall_1_1_0 = (RuleCall)cDefinitionsAssignment_1_1.eContents().get(0);
//RestrictionDefinitionList:
// definitions+=RestrictionDefinition (',' definitions+=RestrictionDefinition)*;
@Override public ParserRule getRule() { return rule; }
//definitions+=RestrictionDefinition (',' definitions+=RestrictionDefinition)*
public Group getGroup() { return cGroup; }
//definitions+=RestrictionDefinition
public Assignment getDefinitionsAssignment_0() { return cDefinitionsAssignment_0; }
//RestrictionDefinition
public RuleCall getDefinitionsRestrictionDefinitionParserRuleCall_0_0() { return cDefinitionsRestrictionDefinitionParserRuleCall_0_0; }
//(',' definitions+=RestrictionDefinition)*
public Group getGroup_1() { return cGroup_1; }
//','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//definitions+=RestrictionDefinition
public Assignment getDefinitionsAssignment_1_1() { return cDefinitionsAssignment_1_1; }
//RestrictionDefinition
public RuleCall getDefinitionsRestrictionDefinitionParserRuleCall_1_1_0() { return cDefinitionsRestrictionDefinitionParserRuleCall_1_1_0; }
}
public class RestrictionDefinitionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.RestrictionDefinition");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cOnlyAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final Keyword cOnlyOnlyKeyword_0_0_0 = (Keyword)cOnlyAssignment_0_0.eContents().get(0);
private final Assignment cNoneAssignment_0_1 = (Assignment)cAlternatives_0.eContents().get(1);
private final Keyword cNoneNoKeyword_0_1_0 = (Keyword)cNoneAssignment_0_1.eContents().get(0);
private final Group cGroup_0_2 = (Group)cAlternatives_0.eContents().get(2);
private final Alternatives cAlternatives_0_2_0 = (Alternatives)cGroup_0_2.eContents().get(0);
private final Assignment cExactlyAssignment_0_2_0_0 = (Assignment)cAlternatives_0_2_0.eContents().get(0);
private final Keyword cExactlyExactlyKeyword_0_2_0_0_0 = (Keyword)cExactlyAssignment_0_2_0_0.eContents().get(0);
private final Group cGroup_0_2_0_1 = (Group)cAlternatives_0_2_0.eContents().get(1);
private final Keyword cAtKeyword_0_2_0_1_0 = (Keyword)cGroup_0_2_0_1.eContents().get(0);
private final Assignment cAtLeastAssignment_0_2_0_1_1 = (Assignment)cGroup_0_2_0_1.eContents().get(1);
private final Keyword cAtLeastLeastKeyword_0_2_0_1_1_0 = (Keyword)cAtLeastAssignment_0_2_0_1_1.eContents().get(0);
private final Group cGroup_0_2_0_2 = (Group)cAlternatives_0_2_0.eContents().get(2);
private final Keyword cAtKeyword_0_2_0_2_0 = (Keyword)cGroup_0_2_0_2.eContents().get(0);
private final Assignment cAtMostAssignment_0_2_0_2_1 = (Assignment)cGroup_0_2_0_2.eContents().get(1);
private final Keyword cAtMostMostKeyword_0_2_0_2_1_0 = (Keyword)cAtMostAssignment_0_2_0_2_1.eContents().get(0);
private final Assignment cHowmanyAssignment_0_2_1 = (Assignment)cGroup_0_2.eContents().get(1);
private final RuleCall cHowmanyNUMBERParserRuleCall_0_2_1_0 = (RuleCall)cHowmanyAssignment_0_2_1.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Assignment cSourceAssignment_1_0 = (Assignment)cAlternatives_1.eContents().get(0);
private final RuleCall cSourceValidIDParserRuleCall_1_0_0 = (RuleCall)cSourceAssignment_1_0.eContents().get(0);
private final Assignment cDataTypeAssignment_1_1 = (Assignment)cAlternatives_1.eContents().get(1);
private final RuleCall cDataTypeDataTypeEnumRuleCall_1_1_0 = (RuleCall)cDataTypeAssignment_1_1.eContents().get(0);
private final Group cGroup_2 = (Group)cGroup.eContents().get(2);
private final Keyword cInheritingKeyword_2_0 = (Keyword)cGroup_2.eContents().get(0);
private final Assignment cTraitTypeAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cTraitTypeValidIDParserRuleCall_2_1_0 = (RuleCall)cTraitTypeAssignment_2_1.eContents().get(0);
private final Group cGroup_3 = (Group)cGroup.eContents().get(3);
private final Alternatives cAlternatives_3_0 = (Alternatives)cGroup_3.eContents().get(0);
private final Keyword cForKeyword_3_0_0 = (Keyword)cAlternatives_3_0.eContents().get(0);
private final Keyword cAsKeyword_3_0_1 = (Keyword)cAlternatives_3_0.eContents().get(1);
private final Assignment cSubjectAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cSubjectValidIDParserRuleCall_3_1_0 = (RuleCall)cSubjectAssignment_3_1.eContents().get(0);
//RestrictionDefinition:
// (only?='only' | none?='no' | (exactly?='exactly' | 'at' atLeast?='least' | 'at' atMost?='most') howmany=NUMBER)?
// (source=ValidID | dataType=DataType) ('inheriting' traitType=ValidID)? (('for' | 'as') subject=ValidID)?;
@Override public ParserRule getRule() { return rule; }
//(only?='only' | none?='no' | (exactly?='exactly' | 'at' atLeast?='least' | 'at' atMost?='most') howmany=NUMBER)?
//(source=ValidID | dataType=DataType) ('inheriting' traitType=ValidID)? (('for' | 'as') subject=ValidID)?
public Group getGroup() { return cGroup; }
//(only?='only' | none?='no' | (exactly?='exactly' | 'at' atLeast?='least' | 'at' atMost?='most') howmany=NUMBER)?
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//only?='only'
public Assignment getOnlyAssignment_0_0() { return cOnlyAssignment_0_0; }
//'only'
public Keyword getOnlyOnlyKeyword_0_0_0() { return cOnlyOnlyKeyword_0_0_0; }
//none?='no'
public Assignment getNoneAssignment_0_1() { return cNoneAssignment_0_1; }
//'no'
public Keyword getNoneNoKeyword_0_1_0() { return cNoneNoKeyword_0_1_0; }
//(exactly?='exactly' | 'at' atLeast?='least' | 'at' atMost?='most') howmany=NUMBER
public Group getGroup_0_2() { return cGroup_0_2; }
//(exactly?='exactly' | 'at' atLeast?='least' | 'at' atMost?='most')
public Alternatives getAlternatives_0_2_0() { return cAlternatives_0_2_0; }
//exactly?='exactly'
public Assignment getExactlyAssignment_0_2_0_0() { return cExactlyAssignment_0_2_0_0; }
//'exactly'
public Keyword getExactlyExactlyKeyword_0_2_0_0_0() { return cExactlyExactlyKeyword_0_2_0_0_0; }
//'at' atLeast?='least'
public Group getGroup_0_2_0_1() { return cGroup_0_2_0_1; }
//'at'
public Keyword getAtKeyword_0_2_0_1_0() { return cAtKeyword_0_2_0_1_0; }
//atLeast?='least'
public Assignment getAtLeastAssignment_0_2_0_1_1() { return cAtLeastAssignment_0_2_0_1_1; }
//'least'
public Keyword getAtLeastLeastKeyword_0_2_0_1_1_0() { return cAtLeastLeastKeyword_0_2_0_1_1_0; }
//'at' atMost?='most'
public Group getGroup_0_2_0_2() { return cGroup_0_2_0_2; }
//'at'
public Keyword getAtKeyword_0_2_0_2_0() { return cAtKeyword_0_2_0_2_0; }
//atMost?='most'
public Assignment getAtMostAssignment_0_2_0_2_1() { return cAtMostAssignment_0_2_0_2_1; }
//'most'
public Keyword getAtMostMostKeyword_0_2_0_2_1_0() { return cAtMostMostKeyword_0_2_0_2_1_0; }
//howmany=NUMBER
public Assignment getHowmanyAssignment_0_2_1() { return cHowmanyAssignment_0_2_1; }
//NUMBER
public RuleCall getHowmanyNUMBERParserRuleCall_0_2_1_0() { return cHowmanyNUMBERParserRuleCall_0_2_1_0; }
//(source=ValidID | dataType=DataType)
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//source=ValidID
public Assignment getSourceAssignment_1_0() { return cSourceAssignment_1_0; }
//ValidID
public RuleCall getSourceValidIDParserRuleCall_1_0_0() { return cSourceValidIDParserRuleCall_1_0_0; }
//dataType=DataType
public Assignment getDataTypeAssignment_1_1() { return cDataTypeAssignment_1_1; }
//DataType
public RuleCall getDataTypeDataTypeEnumRuleCall_1_1_0() { return cDataTypeDataTypeEnumRuleCall_1_1_0; }
//('inheriting' traitType=ValidID)?
public Group getGroup_2() { return cGroup_2; }
//'inheriting'
public Keyword getInheritingKeyword_2_0() { return cInheritingKeyword_2_0; }
//traitType=ValidID
public Assignment getTraitTypeAssignment_2_1() { return cTraitTypeAssignment_2_1; }
//ValidID
public RuleCall getTraitTypeValidIDParserRuleCall_2_1_0() { return cTraitTypeValidIDParserRuleCall_2_1_0; }
//(('for' | 'as') subject=ValidID)?
public Group getGroup_3() { return cGroup_3; }
//('for' | 'as')
public Alternatives getAlternatives_3_0() { return cAlternatives_3_0; }
//'for'
public Keyword getForKeyword_3_0_0() { return cForKeyword_3_0_0; }
//'as'
public Keyword getAsKeyword_3_0_1() { return cAsKeyword_3_0_1; }
//subject=ValidID
public Assignment getSubjectAssignment_3_1() { return cSubjectAssignment_3_1; }
//ValidID
public RuleCall getSubjectValidIDParserRuleCall_3_1_0() { return cSubjectValidIDParserRuleCall_3_1_0; }
}
public class PropertyStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.PropertyStatement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cAbstractAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final Keyword cAbstractAbstractKeyword_0_0_0 = (Keyword)cAbstractAssignment_0_0.eContents().get(0);
private final Assignment cModifiersAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final RuleCall cModifiersModifierListParserRuleCall_0_1_0 = (RuleCall)cModifiersAssignment_0_1.eContents().get(0);
private final Keyword cRelationshipKeyword_0_2 = (Keyword)cGroup_0.eContents().get(2);
private final Assignment cIdAssignment_0_3 = (Assignment)cGroup_0.eContents().get(3);
private final RuleCall cIdValidIDParserRuleCall_0_3_0 = (RuleCall)cIdAssignment_0_3.eContents().get(0);
private final Assignment cDocstringAssignment_0_4 = (Assignment)cGroup_0.eContents().get(4);
private final RuleCall cDocstringSTRINGTerminalRuleCall_0_4_0 = (RuleCall)cDocstringAssignment_0_4.eContents().get(0);
private final Group cGroup_0_5 = (Group)cGroup_0.eContents().get(5);
private final Keyword cIsKeyword_0_5_0 = (Keyword)cGroup_0_5.eContents().get(0);
private final Assignment cParentsAssignment_0_5_1 = (Assignment)cGroup_0_5.eContents().get(1);
private final RuleCall cParentsPropertyListParserRuleCall_0_5_1_0 = (RuleCall)cParentsAssignment_0_5_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_0_6 = (UnorderedGroup)cGroup_0.eContents().get(6);
private final Alternatives cAlternatives_0_6_0 = (Alternatives)cUnorderedGroup_0_6.eContents().get(0);
private final Group cGroup_0_6_0_0 = (Group)cAlternatives_0_6_0.eContents().get(0);
private final Keyword cLinksKeyword_0_6_0_0_0 = (Keyword)cGroup_0_6_0_0.eContents().get(0);
private final Assignment cDomainAssignment_0_6_0_0_1 = (Assignment)cGroup_0_6_0_0.eContents().get(1);
private final RuleCall cDomainConceptListParserRuleCall_0_6_0_0_1_0 = (RuleCall)cDomainAssignment_0_6_0_0_1.eContents().get(0);
private final Keyword cToKeyword_0_6_0_0_2 = (Keyword)cGroup_0_6_0_0.eContents().get(2);
private final Assignment cRangeAssignment_0_6_0_0_3 = (Assignment)cGroup_0_6_0_0.eContents().get(3);
private final RuleCall cRangeConceptListParserRuleCall_0_6_0_0_3_0 = (RuleCall)cRangeAssignment_0_6_0_0_3.eContents().get(0);
private final Group cGroup_0_6_0_1 = (Group)cAlternatives_0_6_0.eContents().get(1);
private final Keyword cAppliesKeyword_0_6_0_1_0 = (Keyword)cGroup_0_6_0_1.eContents().get(0);
private final Keyword cToKeyword_0_6_0_1_1 = (Keyword)cGroup_0_6_0_1.eContents().get(1);
private final Assignment cDomainAssignment_0_6_0_1_2 = (Assignment)cGroup_0_6_0_1.eContents().get(2);
private final RuleCall cDomainConceptListParserRuleCall_0_6_0_1_2_0 = (RuleCall)cDomainAssignment_0_6_0_1_2.eContents().get(0);
private final Alternatives cAlternatives_0_6_0_1_3 = (Alternatives)cGroup_0_6_0_1.eContents().get(3);
private final Group cGroup_0_6_0_1_3_0 = (Group)cAlternatives_0_6_0_1_3.eContents().get(0);
private final Keyword cWithKeyword_0_6_0_1_3_0_0 = (Keyword)cGroup_0_6_0_1_3_0.eContents().get(0);
private final Keyword cRangeKeyword_0_6_0_1_3_0_1 = (Keyword)cGroup_0_6_0_1_3_0.eContents().get(1);
private final Assignment cRangeAssignment_0_6_0_1_3_0_2 = (Assignment)cGroup_0_6_0_1_3_0.eContents().get(2);
private final RuleCall cRangeConceptListParserRuleCall_0_6_0_1_3_0_2_0 = (RuleCall)cRangeAssignment_0_6_0_1_3_0_2.eContents().get(0);
private final Group cGroup_0_6_0_1_3_1 = (Group)cAlternatives_0_6_0_1_3.eContents().get(1);
private final Keyword cWithKeyword_0_6_0_1_3_1_0 = (Keyword)cGroup_0_6_0_1_3_1.eContents().get(0);
private final Assignment cDataAssignment_0_6_0_1_3_1_1 = (Assignment)cGroup_0_6_0_1_3_1.eContents().get(1);
private final Keyword cDataTypeKeyword_0_6_0_1_3_1_1_0 = (Keyword)cDataAssignment_0_6_0_1_3_1_1.eContents().get(0);
private final Assignment cDataTypeAssignment_0_6_0_1_3_1_2 = (Assignment)cGroup_0_6_0_1_3_1.eContents().get(2);
private final RuleCall cDataTypeDataTypeEnumRuleCall_0_6_0_1_3_1_2_0 = (RuleCall)cDataTypeAssignment_0_6_0_1_3_1_2.eContents().get(0);
private final Group cGroup_0_6_1 = (Group)cUnorderedGroup_0_6.eContents().get(1);
private final Keyword cRequiresKeyword_0_6_1_0 = (Keyword)cGroup_0_6_1.eContents().get(0);
private final Assignment cRequirementAssignment_0_6_1_1 = (Assignment)cGroup_0_6_1.eContents().get(1);
private final RuleCall cRequirementIdentityRequirementListParserRuleCall_0_6_1_1_0 = (RuleCall)cRequirementAssignment_0_6_1_1.eContents().get(0);
private final Group cGroup_0_6_2 = (Group)cUnorderedGroup_0_6.eContents().get(2);
private final Keyword cInheritsKeyword_0_6_2_0 = (Keyword)cGroup_0_6_2.eContents().get(0);
private final Assignment cActuallyInheritedTraitsAssignment_0_6_2_1 = (Assignment)cGroup_0_6_2.eContents().get(1);
private final RuleCall cActuallyInheritedTraitsConceptListParserRuleCall_0_6_2_1_0 = (RuleCall)cActuallyInheritedTraitsAssignment_0_6_2_1.eContents().get(0);
private final Group cGroup_0_6_3 = (Group)cUnorderedGroup_0_6.eContents().get(3);
private final Keyword cHasKeyword_0_6_3_0 = (Keyword)cGroup_0_6_3.eContents().get(0);
private final Assignment cDisjointAssignment_0_6_3_1 = (Assignment)cGroup_0_6_3.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_0_6_3_1_0 = (Keyword)cDisjointAssignment_0_6_3_1.eContents().get(0);
private final Keyword cChildrenKeyword_0_6_3_2 = (Keyword)cGroup_0_6_3.eContents().get(2);
private final Assignment cChildrenAssignment_0_6_3_3 = (Assignment)cGroup_0_6_3.eContents().get(3);
private final RuleCall cChildrenPropertyListParserRuleCall_0_6_3_3_0 = (RuleCall)cChildrenAssignment_0_6_3_3.eContents().get(0);
private final Group cGroup_0_6_4 = (Group)cUnorderedGroup_0_6.eContents().get(4);
private final Keyword cInverseKeyword_0_6_4_0 = (Keyword)cGroup_0_6_4.eContents().get(0);
private final Keyword cOfKeyword_0_6_4_1 = (Keyword)cGroup_0_6_4.eContents().get(1);
private final Assignment cInverseAssignment_0_6_4_2 = (Assignment)cGroup_0_6_4.eContents().get(2);
private final RuleCall cInverseValidIDParserRuleCall_0_6_4_2_0 = (RuleCall)cInverseAssignment_0_6_4_2.eContents().get(0);
private final Group cGroup_0_7 = (Group)cGroup_0.eContents().get(7);
private final Keyword cWithKeyword_0_7_0 = (Keyword)cGroup_0_7.eContents().get(0);
private final Keyword cMetadataKeyword_0_7_1 = (Keyword)cGroup_0_7.eContents().get(1);
private final Assignment cMetadataAssignment_0_7_2 = (Assignment)cGroup_0_7.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_0_7_2_0 = (RuleCall)cMetadataAssignment_0_7_2.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cAbstractAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Keyword cAbstractAbstractKeyword_1_0_0 = (Keyword)cAbstractAssignment_1_0.eContents().get(0);
private final Assignment cAnnotationAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final Keyword cAnnotationAnnotationKeyword_1_1_0 = (Keyword)cAnnotationAssignment_1_1.eContents().get(0);
private final Assignment cIdAssignment_1_2 = (Assignment)cGroup_1.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_1_2_0 = (RuleCall)cIdAssignment_1_2.eContents().get(0);
private final Group cGroup_1_3 = (Group)cGroup_1.eContents().get(3);
private final Keyword cIsKeyword_1_3_0 = (Keyword)cGroup_1_3.eContents().get(0);
private final Assignment cParentsAssignment_1_3_1 = (Assignment)cGroup_1_3.eContents().get(1);
private final RuleCall cParentsPropertyListParserRuleCall_1_3_1_0 = (RuleCall)cParentsAssignment_1_3_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_1_4 = (UnorderedGroup)cGroup_1.eContents().get(4);
private final Group cGroup_1_4_0 = (Group)cUnorderedGroup_1_4.eContents().get(0);
private final Keyword cWithKeyword_1_4_0_0 = (Keyword)cGroup_1_4_0.eContents().get(0);
private final Keyword cTypeKeyword_1_4_0_1 = (Keyword)cGroup_1_4_0.eContents().get(1);
private final Assignment cDataTypeAssignment_1_4_0_2 = (Assignment)cGroup_1_4_0.eContents().get(2);
private final RuleCall cDataTypeDataTypeEnumRuleCall_1_4_0_2_0 = (RuleCall)cDataTypeAssignment_1_4_0_2.eContents().get(0);
private final Group cGroup_1_4_1 = (Group)cUnorderedGroup_1_4.eContents().get(1);
private final Keyword cHasKeyword_1_4_1_0 = (Keyword)cGroup_1_4_1.eContents().get(0);
private final Assignment cDisjointAssignment_1_4_1_1 = (Assignment)cGroup_1_4_1.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_1_4_1_1_0 = (Keyword)cDisjointAssignment_1_4_1_1.eContents().get(0);
private final Keyword cChildrenKeyword_1_4_1_2 = (Keyword)cGroup_1_4_1.eContents().get(2);
private final Assignment cChildrenAssignment_1_4_1_3 = (Assignment)cGroup_1_4_1.eContents().get(3);
private final RuleCall cChildrenPropertyListParserRuleCall_1_4_1_3_0 = (RuleCall)cChildrenAssignment_1_4_1_3.eContents().get(0);
private final Group cGroup_1_4_2 = (Group)cUnorderedGroup_1_4.eContents().get(2);
private final Keyword cInverseKeyword_1_4_2_0 = (Keyword)cGroup_1_4_2.eContents().get(0);
private final Keyword cOfKeyword_1_4_2_1 = (Keyword)cGroup_1_4_2.eContents().get(1);
private final Assignment cInverseAssignment_1_4_2_2 = (Assignment)cGroup_1_4_2.eContents().get(2);
private final RuleCall cInverseValidIDParserRuleCall_1_4_2_2_0 = (RuleCall)cInverseAssignment_1_4_2_2.eContents().get(0);
private final Group cGroup_1_5 = (Group)cGroup_1.eContents().get(5);
private final Keyword cWithKeyword_1_5_0 = (Keyword)cGroup_1_5.eContents().get(0);
private final Keyword cMetadataKeyword_1_5_1 = (Keyword)cGroup_1_5.eContents().get(1);
private final Assignment cMetadataAssignment_1_5_2 = (Assignment)cGroup_1_5.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_1_5_2_0 = (RuleCall)cMetadataAssignment_1_5_2.eContents().get(0);
/// *
// * data property are distinguished from object properties by having a type instead
// * of a range. So the minimum property spec is of an object property pointing to owl:Thing.
// * Properties can be chained like concepts - no repetition of keyword and qualifiers for
// * subsequent ones.
// *
// * /
//PropertyStatement:
// abstract?='abstract'? modifiers=ModifierList? 'relationship' id=ValidID docstring=STRING? ('is'
// parents=PropertyList)? (('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList
// ('with' 'range' range=ConceptList | 'with' data?='type' dataType=DataType)?)? & ('requires'
// requirement=IdentityRequirementList)? & ('inherits' actuallyInheritedTraits=ConceptList)? & ('has'
// disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse' 'of' inverse=ValidID)?) ('with' 'metadata'
// metadata=Metadata)? | abstract?='abstract'? annotation?='annotation' id=ValidID ('is' parents=PropertyList)? (('with'
// 'type' dataType=DataType)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse' 'of'
// inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)?;
@Override public ParserRule getRule() { return rule; }
//abstract?='abstract'? modifiers=ModifierList? 'relationship' id=ValidID docstring=STRING? ('is' parents=PropertyList)?
//(('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range'
//range=ConceptList | 'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? &
//('inherits' actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? &
//('inverse' 'of' inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)? | abstract?='abstract'?
//annotation?='annotation' id=ValidID ('is' parents=PropertyList)? (('with' 'type' dataType=DataType)? & ('has'
//disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse' 'of' inverse=ValidID)?) ('with' 'metadata'
//metadata=Metadata)?
public Alternatives getAlternatives() { return cAlternatives; }
//abstract?='abstract'? modifiers=ModifierList? 'relationship' id=ValidID docstring=STRING? ('is' parents=PropertyList)?
//(('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range'
//range=ConceptList | 'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? &
//('inherits' actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? &
//('inverse' 'of' inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)?
public Group getGroup_0() { return cGroup_0; }
//abstract?='abstract'?
public Assignment getAbstractAssignment_0_0() { return cAbstractAssignment_0_0; }
//'abstract'
public Keyword getAbstractAbstractKeyword_0_0_0() { return cAbstractAbstractKeyword_0_0_0; }
//modifiers=ModifierList?
public Assignment getModifiersAssignment_0_1() { return cModifiersAssignment_0_1; }
//ModifierList
public RuleCall getModifiersModifierListParserRuleCall_0_1_0() { return cModifiersModifierListParserRuleCall_0_1_0; }
//'relationship'
public Keyword getRelationshipKeyword_0_2() { return cRelationshipKeyword_0_2; }
//id=ValidID
public Assignment getIdAssignment_0_3() { return cIdAssignment_0_3; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_0_3_0() { return cIdValidIDParserRuleCall_0_3_0; }
//docstring=STRING?
public Assignment getDocstringAssignment_0_4() { return cDocstringAssignment_0_4; }
//STRING
public RuleCall getDocstringSTRINGTerminalRuleCall_0_4_0() { return cDocstringSTRINGTerminalRuleCall_0_4_0; }
//('is' parents=PropertyList)?
public Group getGroup_0_5() { return cGroup_0_5; }
//'is'
public Keyword getIsKeyword_0_5_0() { return cIsKeyword_0_5_0; }
//parents=PropertyList
public Assignment getParentsAssignment_0_5_1() { return cParentsAssignment_0_5_1; }
//PropertyList
public RuleCall getParentsPropertyListParserRuleCall_0_5_1_0() { return cParentsPropertyListParserRuleCall_0_5_1_0; }
//(('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range'
//range=ConceptList | 'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? &
//('inherits' actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? &
//('inverse' 'of' inverse=ValidID)?)
public UnorderedGroup getUnorderedGroup_0_6() { return cUnorderedGroup_0_6; }
//('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList
//| 'with' data?='type' dataType=DataType)?)?
public Alternatives getAlternatives_0_6_0() { return cAlternatives_0_6_0; }
//'links' domain=ConceptList 'to' range=ConceptList
public Group getGroup_0_6_0_0() { return cGroup_0_6_0_0; }
//'links'
public Keyword getLinksKeyword_0_6_0_0_0() { return cLinksKeyword_0_6_0_0_0; }
//domain=ConceptList
public Assignment getDomainAssignment_0_6_0_0_1() { return cDomainAssignment_0_6_0_0_1; }
//ConceptList
public RuleCall getDomainConceptListParserRuleCall_0_6_0_0_1_0() { return cDomainConceptListParserRuleCall_0_6_0_0_1_0; }
//'to'
public Keyword getToKeyword_0_6_0_0_2() { return cToKeyword_0_6_0_0_2; }
//range=ConceptList
public Assignment getRangeAssignment_0_6_0_0_3() { return cRangeAssignment_0_6_0_0_3; }
//ConceptList
public RuleCall getRangeConceptListParserRuleCall_0_6_0_0_3_0() { return cRangeConceptListParserRuleCall_0_6_0_0_3_0; }
//'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList | 'with' data?='type' dataType=DataType)?
public Group getGroup_0_6_0_1() { return cGroup_0_6_0_1; }
//'applies'
public Keyword getAppliesKeyword_0_6_0_1_0() { return cAppliesKeyword_0_6_0_1_0; }
//'to'
public Keyword getToKeyword_0_6_0_1_1() { return cToKeyword_0_6_0_1_1; }
//domain=ConceptList
public Assignment getDomainAssignment_0_6_0_1_2() { return cDomainAssignment_0_6_0_1_2; }
//ConceptList
public RuleCall getDomainConceptListParserRuleCall_0_6_0_1_2_0() { return cDomainConceptListParserRuleCall_0_6_0_1_2_0; }
//('with' 'range' range=ConceptList | 'with' data?='type' dataType=DataType)?
public Alternatives getAlternatives_0_6_0_1_3() { return cAlternatives_0_6_0_1_3; }
//'with' 'range' range=ConceptList
public Group getGroup_0_6_0_1_3_0() { return cGroup_0_6_0_1_3_0; }
//'with'
public Keyword getWithKeyword_0_6_0_1_3_0_0() { return cWithKeyword_0_6_0_1_3_0_0; }
//'range'
public Keyword getRangeKeyword_0_6_0_1_3_0_1() { return cRangeKeyword_0_6_0_1_3_0_1; }
//range=ConceptList
public Assignment getRangeAssignment_0_6_0_1_3_0_2() { return cRangeAssignment_0_6_0_1_3_0_2; }
//ConceptList
public RuleCall getRangeConceptListParserRuleCall_0_6_0_1_3_0_2_0() { return cRangeConceptListParserRuleCall_0_6_0_1_3_0_2_0; }
//'with' data?='type' dataType=DataType
public Group getGroup_0_6_0_1_3_1() { return cGroup_0_6_0_1_3_1; }
//'with'
public Keyword getWithKeyword_0_6_0_1_3_1_0() { return cWithKeyword_0_6_0_1_3_1_0; }
//data?='type'
public Assignment getDataAssignment_0_6_0_1_3_1_1() { return cDataAssignment_0_6_0_1_3_1_1; }
//'type'
public Keyword getDataTypeKeyword_0_6_0_1_3_1_1_0() { return cDataTypeKeyword_0_6_0_1_3_1_1_0; }
//dataType=DataType
public Assignment getDataTypeAssignment_0_6_0_1_3_1_2() { return cDataTypeAssignment_0_6_0_1_3_1_2; }
//DataType
public RuleCall getDataTypeDataTypeEnumRuleCall_0_6_0_1_3_1_2_0() { return cDataTypeDataTypeEnumRuleCall_0_6_0_1_3_1_2_0; }
//('requires' requirement=IdentityRequirementList)?
public Group getGroup_0_6_1() { return cGroup_0_6_1; }
//'requires'
public Keyword getRequiresKeyword_0_6_1_0() { return cRequiresKeyword_0_6_1_0; }
//requirement=IdentityRequirementList
public Assignment getRequirementAssignment_0_6_1_1() { return cRequirementAssignment_0_6_1_1; }
//IdentityRequirementList
public RuleCall getRequirementIdentityRequirementListParserRuleCall_0_6_1_1_0() { return cRequirementIdentityRequirementListParserRuleCall_0_6_1_1_0; }
//('inherits' actuallyInheritedTraits=ConceptList)?
public Group getGroup_0_6_2() { return cGroup_0_6_2; }
//'inherits'
public Keyword getInheritsKeyword_0_6_2_0() { return cInheritsKeyword_0_6_2_0; }
//actuallyInheritedTraits=ConceptList
public Assignment getActuallyInheritedTraitsAssignment_0_6_2_1() { return cActuallyInheritedTraitsAssignment_0_6_2_1; }
//ConceptList
public RuleCall getActuallyInheritedTraitsConceptListParserRuleCall_0_6_2_1_0() { return cActuallyInheritedTraitsConceptListParserRuleCall_0_6_2_1_0; }
//('has' disjoint?='disjoint'? 'children' children=PropertyList)?
public Group getGroup_0_6_3() { return cGroup_0_6_3; }
//'has'
public Keyword getHasKeyword_0_6_3_0() { return cHasKeyword_0_6_3_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_0_6_3_1() { return cDisjointAssignment_0_6_3_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_0_6_3_1_0() { return cDisjointDisjointKeyword_0_6_3_1_0; }
//'children'
public Keyword getChildrenKeyword_0_6_3_2() { return cChildrenKeyword_0_6_3_2; }
//children=PropertyList
public Assignment getChildrenAssignment_0_6_3_3() { return cChildrenAssignment_0_6_3_3; }
//PropertyList
public RuleCall getChildrenPropertyListParserRuleCall_0_6_3_3_0() { return cChildrenPropertyListParserRuleCall_0_6_3_3_0; }
//('inverse' 'of' inverse=ValidID)?
public Group getGroup_0_6_4() { return cGroup_0_6_4; }
//'inverse'
public Keyword getInverseKeyword_0_6_4_0() { return cInverseKeyword_0_6_4_0; }
//'of'
public Keyword getOfKeyword_0_6_4_1() { return cOfKeyword_0_6_4_1; }
//inverse=ValidID
public Assignment getInverseAssignment_0_6_4_2() { return cInverseAssignment_0_6_4_2; }
//ValidID
public RuleCall getInverseValidIDParserRuleCall_0_6_4_2_0() { return cInverseValidIDParserRuleCall_0_6_4_2_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_0_7() { return cGroup_0_7; }
//'with'
public Keyword getWithKeyword_0_7_0() { return cWithKeyword_0_7_0; }
//'metadata'
public Keyword getMetadataKeyword_0_7_1() { return cMetadataKeyword_0_7_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_0_7_2() { return cMetadataAssignment_0_7_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_0_7_2_0() { return cMetadataMetadataParserRuleCall_0_7_2_0; }
//abstract?='abstract'? annotation?='annotation' id=ValidID ('is' parents=PropertyList)? (('with' 'type'
//dataType=DataType)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse' 'of'
//inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)?
public Group getGroup_1() { return cGroup_1; }
//abstract?='abstract'?
public Assignment getAbstractAssignment_1_0() { return cAbstractAssignment_1_0; }
//'abstract'
public Keyword getAbstractAbstractKeyword_1_0_0() { return cAbstractAbstractKeyword_1_0_0; }
//annotation?='annotation'
public Assignment getAnnotationAssignment_1_1() { return cAnnotationAssignment_1_1; }
//'annotation'
public Keyword getAnnotationAnnotationKeyword_1_1_0() { return cAnnotationAnnotationKeyword_1_1_0; }
//id=ValidID
public Assignment getIdAssignment_1_2() { return cIdAssignment_1_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_1_2_0() { return cIdValidIDParserRuleCall_1_2_0; }
//('is' parents=PropertyList)?
public Group getGroup_1_3() { return cGroup_1_3; }
//'is'
public Keyword getIsKeyword_1_3_0() { return cIsKeyword_1_3_0; }
//parents=PropertyList
public Assignment getParentsAssignment_1_3_1() { return cParentsAssignment_1_3_1; }
//PropertyList
public RuleCall getParentsPropertyListParserRuleCall_1_3_1_0() { return cParentsPropertyListParserRuleCall_1_3_1_0; }
//(('with' 'type' dataType=DataType)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse' 'of'
//inverse=ValidID)?)
public UnorderedGroup getUnorderedGroup_1_4() { return cUnorderedGroup_1_4; }
//('with' 'type' dataType=DataType)?
public Group getGroup_1_4_0() { return cGroup_1_4_0; }
//'with'
public Keyword getWithKeyword_1_4_0_0() { return cWithKeyword_1_4_0_0; }
//'type'
public Keyword getTypeKeyword_1_4_0_1() { return cTypeKeyword_1_4_0_1; }
//dataType=DataType
public Assignment getDataTypeAssignment_1_4_0_2() { return cDataTypeAssignment_1_4_0_2; }
//DataType
public RuleCall getDataTypeDataTypeEnumRuleCall_1_4_0_2_0() { return cDataTypeDataTypeEnumRuleCall_1_4_0_2_0; }
//('has' disjoint?='disjoint'? 'children' children=PropertyList)?
public Group getGroup_1_4_1() { return cGroup_1_4_1; }
//'has'
public Keyword getHasKeyword_1_4_1_0() { return cHasKeyword_1_4_1_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_1_4_1_1() { return cDisjointAssignment_1_4_1_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_1_4_1_1_0() { return cDisjointDisjointKeyword_1_4_1_1_0; }
//'children'
public Keyword getChildrenKeyword_1_4_1_2() { return cChildrenKeyword_1_4_1_2; }
//children=PropertyList
public Assignment getChildrenAssignment_1_4_1_3() { return cChildrenAssignment_1_4_1_3; }
//PropertyList
public RuleCall getChildrenPropertyListParserRuleCall_1_4_1_3_0() { return cChildrenPropertyListParserRuleCall_1_4_1_3_0; }
//('inverse' 'of' inverse=ValidID)?
public Group getGroup_1_4_2() { return cGroup_1_4_2; }
//'inverse'
public Keyword getInverseKeyword_1_4_2_0() { return cInverseKeyword_1_4_2_0; }
//'of'
public Keyword getOfKeyword_1_4_2_1() { return cOfKeyword_1_4_2_1; }
//inverse=ValidID
public Assignment getInverseAssignment_1_4_2_2() { return cInverseAssignment_1_4_2_2; }
//ValidID
public RuleCall getInverseValidIDParserRuleCall_1_4_2_2_0() { return cInverseValidIDParserRuleCall_1_4_2_2_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_1_5() { return cGroup_1_5; }
//'with'
public Keyword getWithKeyword_1_5_0() { return cWithKeyword_1_5_0; }
//'metadata'
public Keyword getMetadataKeyword_1_5_1() { return cMetadataKeyword_1_5_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_1_5_2() { return cMetadataAssignment_1_5_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_1_5_2_0() { return cMetadataMetadataParserRuleCall_1_5_2_0; }
}
public class SubPropertyStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.SubPropertyStatement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cAnnotationsAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cAnnotationsAnnotationParserRuleCall_0_0 = (RuleCall)cAnnotationsAssignment_0.eContents().get(0);
private final Assignment cAbstractAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final Keyword cAbstractAbstractKeyword_1_0 = (Keyword)cAbstractAssignment_1.eContents().get(0);
private final Assignment cIdAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_2_0 = (RuleCall)cIdAssignment_2.eContents().get(0);
private final Assignment cDocstringAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cDocstringSTRINGTerminalRuleCall_3_0 = (RuleCall)cDocstringAssignment_3.eContents().get(0);
private final Group cGroup_4 = (Group)cGroup.eContents().get(4);
private final Keyword cIsKeyword_4_0 = (Keyword)cGroup_4.eContents().get(0);
private final Assignment cParentsAssignment_4_1 = (Assignment)cGroup_4.eContents().get(1);
private final RuleCall cParentsPropertyListParserRuleCall_4_1_0 = (RuleCall)cParentsAssignment_4_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_5 = (UnorderedGroup)cGroup.eContents().get(5);
private final Alternatives cAlternatives_5_0 = (Alternatives)cUnorderedGroup_5.eContents().get(0);
private final Group cGroup_5_0_0 = (Group)cAlternatives_5_0.eContents().get(0);
private final Keyword cLinksKeyword_5_0_0_0 = (Keyword)cGroup_5_0_0.eContents().get(0);
private final Assignment cDomainAssignment_5_0_0_1 = (Assignment)cGroup_5_0_0.eContents().get(1);
private final RuleCall cDomainConceptListParserRuleCall_5_0_0_1_0 = (RuleCall)cDomainAssignment_5_0_0_1.eContents().get(0);
private final Keyword cToKeyword_5_0_0_2 = (Keyword)cGroup_5_0_0.eContents().get(2);
private final Assignment cRangeAssignment_5_0_0_3 = (Assignment)cGroup_5_0_0.eContents().get(3);
private final RuleCall cRangeConceptListParserRuleCall_5_0_0_3_0 = (RuleCall)cRangeAssignment_5_0_0_3.eContents().get(0);
private final Group cGroup_5_0_1 = (Group)cAlternatives_5_0.eContents().get(1);
private final Keyword cAppliesKeyword_5_0_1_0 = (Keyword)cGroup_5_0_1.eContents().get(0);
private final Keyword cToKeyword_5_0_1_1 = (Keyword)cGroup_5_0_1.eContents().get(1);
private final Assignment cDomainAssignment_5_0_1_2 = (Assignment)cGroup_5_0_1.eContents().get(2);
private final RuleCall cDomainConceptListParserRuleCall_5_0_1_2_0 = (RuleCall)cDomainAssignment_5_0_1_2.eContents().get(0);
private final Alternatives cAlternatives_5_0_1_3 = (Alternatives)cGroup_5_0_1.eContents().get(3);
private final Group cGroup_5_0_1_3_0 = (Group)cAlternatives_5_0_1_3.eContents().get(0);
private final Keyword cWithKeyword_5_0_1_3_0_0 = (Keyword)cGroup_5_0_1_3_0.eContents().get(0);
private final Keyword cRangeKeyword_5_0_1_3_0_1 = (Keyword)cGroup_5_0_1_3_0.eContents().get(1);
private final Assignment cRangeAssignment_5_0_1_3_0_2 = (Assignment)cGroup_5_0_1_3_0.eContents().get(2);
private final RuleCall cRangeConceptListParserRuleCall_5_0_1_3_0_2_0 = (RuleCall)cRangeAssignment_5_0_1_3_0_2.eContents().get(0);
private final Group cGroup_5_0_1_3_1 = (Group)cAlternatives_5_0_1_3.eContents().get(1);
private final Keyword cWithKeyword_5_0_1_3_1_0 = (Keyword)cGroup_5_0_1_3_1.eContents().get(0);
private final Assignment cDataAssignment_5_0_1_3_1_1 = (Assignment)cGroup_5_0_1_3_1.eContents().get(1);
private final Keyword cDataTypeKeyword_5_0_1_3_1_1_0 = (Keyword)cDataAssignment_5_0_1_3_1_1.eContents().get(0);
private final Assignment cDataTypeAssignment_5_0_1_3_1_2 = (Assignment)cGroup_5_0_1_3_1.eContents().get(2);
private final RuleCall cDataTypeDataTypeEnumRuleCall_5_0_1_3_1_2_0 = (RuleCall)cDataTypeAssignment_5_0_1_3_1_2.eContents().get(0);
private final Group cGroup_5_1 = (Group)cUnorderedGroup_5.eContents().get(1);
private final Keyword cRequiresKeyword_5_1_0 = (Keyword)cGroup_5_1.eContents().get(0);
private final Assignment cRequirementAssignment_5_1_1 = (Assignment)cGroup_5_1.eContents().get(1);
private final RuleCall cRequirementIdentityRequirementListParserRuleCall_5_1_1_0 = (RuleCall)cRequirementAssignment_5_1_1.eContents().get(0);
private final Group cGroup_5_2 = (Group)cUnorderedGroup_5.eContents().get(2);
private final Keyword cInheritsKeyword_5_2_0 = (Keyword)cGroup_5_2.eContents().get(0);
private final Assignment cActuallyInheritedTraitsAssignment_5_2_1 = (Assignment)cGroup_5_2.eContents().get(1);
private final RuleCall cActuallyInheritedTraitsConceptListParserRuleCall_5_2_1_0 = (RuleCall)cActuallyInheritedTraitsAssignment_5_2_1.eContents().get(0);
private final Group cGroup_5_3 = (Group)cUnorderedGroup_5.eContents().get(3);
private final Keyword cHasKeyword_5_3_0 = (Keyword)cGroup_5_3.eContents().get(0);
private final Assignment cDisjointAssignment_5_3_1 = (Assignment)cGroup_5_3.eContents().get(1);
private final Keyword cDisjointDisjointKeyword_5_3_1_0 = (Keyword)cDisjointAssignment_5_3_1.eContents().get(0);
private final Keyword cChildrenKeyword_5_3_2 = (Keyword)cGroup_5_3.eContents().get(2);
private final Assignment cChildrenAssignment_5_3_3 = (Assignment)cGroup_5_3.eContents().get(3);
private final RuleCall cChildrenPropertyListParserRuleCall_5_3_3_0 = (RuleCall)cChildrenAssignment_5_3_3.eContents().get(0);
private final Group cGroup_5_4 = (Group)cUnorderedGroup_5.eContents().get(4);
private final Keyword cInverseKeyword_5_4_0 = (Keyword)cGroup_5_4.eContents().get(0);
private final Keyword cOfKeyword_5_4_1 = (Keyword)cGroup_5_4.eContents().get(1);
private final Assignment cInverseAssignment_5_4_2 = (Assignment)cGroup_5_4.eContents().get(2);
private final RuleCall cInverseValidIDParserRuleCall_5_4_2_0 = (RuleCall)cInverseAssignment_5_4_2.eContents().get(0);
private final Group cGroup_6 = (Group)cGroup.eContents().get(6);
private final Keyword cWithKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Keyword cMetadataKeyword_6_1 = (Keyword)cGroup_6.eContents().get(1);
private final Assignment cMetadataAssignment_6_2 = (Assignment)cGroup_6.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_6_2_0 = (RuleCall)cMetadataAssignment_6_2.eContents().get(0);
//SubPropertyStatement PropertyStatement:
// annotations+=Annotation* abstract?='abstract'? id=ValidID docstring=STRING? ('is' parents=PropertyList)? (('links'
// domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList |
// 'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? & ('inherits'
// actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse'
// 'of' inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)?
@Override public ParserRule getRule() { return rule; }
//annotations+=Annotation* abstract?='abstract'? id=ValidID docstring=STRING? ('is' parents=PropertyList)? (('links'
//domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList |
//'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? & ('inherits'
//actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? & ('inverse'
//'of' inverse=ValidID)?) ('with' 'metadata' metadata=Metadata)?
public Group getGroup() { return cGroup; }
//annotations+=Annotation*
public Assignment getAnnotationsAssignment_0() { return cAnnotationsAssignment_0; }
//Annotation
public RuleCall getAnnotationsAnnotationParserRuleCall_0_0() { return cAnnotationsAnnotationParserRuleCall_0_0; }
//abstract?='abstract'?
public Assignment getAbstractAssignment_1() { return cAbstractAssignment_1; }
//'abstract'
public Keyword getAbstractAbstractKeyword_1_0() { return cAbstractAbstractKeyword_1_0; }
//id=ValidID
public Assignment getIdAssignment_2() { return cIdAssignment_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_2_0() { return cIdValidIDParserRuleCall_2_0; }
//docstring=STRING?
public Assignment getDocstringAssignment_3() { return cDocstringAssignment_3; }
//STRING
public RuleCall getDocstringSTRINGTerminalRuleCall_3_0() { return cDocstringSTRINGTerminalRuleCall_3_0; }
//('is' parents=PropertyList)?
public Group getGroup_4() { return cGroup_4; }
//'is'
public Keyword getIsKeyword_4_0() { return cIsKeyword_4_0; }
//parents=PropertyList
public Assignment getParentsAssignment_4_1() { return cParentsAssignment_4_1; }
//PropertyList
public RuleCall getParentsPropertyListParserRuleCall_4_1_0() { return cParentsPropertyListParserRuleCall_4_1_0; }
//(('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range'
//range=ConceptList | 'with' data?='type' dataType=DataType)?)? & ('requires' requirement=IdentityRequirementList)? &
//('inherits' actuallyInheritedTraits=ConceptList)? & ('has' disjoint?='disjoint'? 'children' children=PropertyList)? &
//('inverse' 'of' inverse=ValidID)?)
public UnorderedGroup getUnorderedGroup_5() { return cUnorderedGroup_5; }
//('links' domain=ConceptList 'to' range=ConceptList | 'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList
//| 'with' data?='type' dataType=DataType)?)?
public Alternatives getAlternatives_5_0() { return cAlternatives_5_0; }
//'links' domain=ConceptList 'to' range=ConceptList
public Group getGroup_5_0_0() { return cGroup_5_0_0; }
//'links'
public Keyword getLinksKeyword_5_0_0_0() { return cLinksKeyword_5_0_0_0; }
//domain=ConceptList
public Assignment getDomainAssignment_5_0_0_1() { return cDomainAssignment_5_0_0_1; }
//ConceptList
public RuleCall getDomainConceptListParserRuleCall_5_0_0_1_0() { return cDomainConceptListParserRuleCall_5_0_0_1_0; }
//'to'
public Keyword getToKeyword_5_0_0_2() { return cToKeyword_5_0_0_2; }
//range=ConceptList
public Assignment getRangeAssignment_5_0_0_3() { return cRangeAssignment_5_0_0_3; }
//ConceptList
public RuleCall getRangeConceptListParserRuleCall_5_0_0_3_0() { return cRangeConceptListParserRuleCall_5_0_0_3_0; }
//'applies' 'to' domain=ConceptList ('with' 'range' range=ConceptList | 'with' data?='type' dataType=DataType)?
public Group getGroup_5_0_1() { return cGroup_5_0_1; }
//'applies'
public Keyword getAppliesKeyword_5_0_1_0() { return cAppliesKeyword_5_0_1_0; }
//'to'
public Keyword getToKeyword_5_0_1_1() { return cToKeyword_5_0_1_1; }
//domain=ConceptList
public Assignment getDomainAssignment_5_0_1_2() { return cDomainAssignment_5_0_1_2; }
//ConceptList
public RuleCall getDomainConceptListParserRuleCall_5_0_1_2_0() { return cDomainConceptListParserRuleCall_5_0_1_2_0; }
//('with' 'range' range=ConceptList | 'with' data?='type' dataType=DataType)?
public Alternatives getAlternatives_5_0_1_3() { return cAlternatives_5_0_1_3; }
//'with' 'range' range=ConceptList
public Group getGroup_5_0_1_3_0() { return cGroup_5_0_1_3_0; }
//'with'
public Keyword getWithKeyword_5_0_1_3_0_0() { return cWithKeyword_5_0_1_3_0_0; }
//'range'
public Keyword getRangeKeyword_5_0_1_3_0_1() { return cRangeKeyword_5_0_1_3_0_1; }
//range=ConceptList
public Assignment getRangeAssignment_5_0_1_3_0_2() { return cRangeAssignment_5_0_1_3_0_2; }
//ConceptList
public RuleCall getRangeConceptListParserRuleCall_5_0_1_3_0_2_0() { return cRangeConceptListParserRuleCall_5_0_1_3_0_2_0; }
//'with' data?='type' dataType=DataType
public Group getGroup_5_0_1_3_1() { return cGroup_5_0_1_3_1; }
//'with'
public Keyword getWithKeyword_5_0_1_3_1_0() { return cWithKeyword_5_0_1_3_1_0; }
//data?='type'
public Assignment getDataAssignment_5_0_1_3_1_1() { return cDataAssignment_5_0_1_3_1_1; }
//'type'
public Keyword getDataTypeKeyword_5_0_1_3_1_1_0() { return cDataTypeKeyword_5_0_1_3_1_1_0; }
//dataType=DataType
public Assignment getDataTypeAssignment_5_0_1_3_1_2() { return cDataTypeAssignment_5_0_1_3_1_2; }
//DataType
public RuleCall getDataTypeDataTypeEnumRuleCall_5_0_1_3_1_2_0() { return cDataTypeDataTypeEnumRuleCall_5_0_1_3_1_2_0; }
//('requires' requirement=IdentityRequirementList)?
public Group getGroup_5_1() { return cGroup_5_1; }
//'requires'
public Keyword getRequiresKeyword_5_1_0() { return cRequiresKeyword_5_1_0; }
//requirement=IdentityRequirementList
public Assignment getRequirementAssignment_5_1_1() { return cRequirementAssignment_5_1_1; }
//IdentityRequirementList
public RuleCall getRequirementIdentityRequirementListParserRuleCall_5_1_1_0() { return cRequirementIdentityRequirementListParserRuleCall_5_1_1_0; }
//('inherits' actuallyInheritedTraits=ConceptList)?
public Group getGroup_5_2() { return cGroup_5_2; }
//'inherits'
public Keyword getInheritsKeyword_5_2_0() { return cInheritsKeyword_5_2_0; }
//actuallyInheritedTraits=ConceptList
public Assignment getActuallyInheritedTraitsAssignment_5_2_1() { return cActuallyInheritedTraitsAssignment_5_2_1; }
//ConceptList
public RuleCall getActuallyInheritedTraitsConceptListParserRuleCall_5_2_1_0() { return cActuallyInheritedTraitsConceptListParserRuleCall_5_2_1_0; }
//('has' disjoint?='disjoint'? 'children' children=PropertyList)?
public Group getGroup_5_3() { return cGroup_5_3; }
//'has'
public Keyword getHasKeyword_5_3_0() { return cHasKeyword_5_3_0; }
//disjoint?='disjoint'?
public Assignment getDisjointAssignment_5_3_1() { return cDisjointAssignment_5_3_1; }
//'disjoint'
public Keyword getDisjointDisjointKeyword_5_3_1_0() { return cDisjointDisjointKeyword_5_3_1_0; }
//'children'
public Keyword getChildrenKeyword_5_3_2() { return cChildrenKeyword_5_3_2; }
//children=PropertyList
public Assignment getChildrenAssignment_5_3_3() { return cChildrenAssignment_5_3_3; }
//PropertyList
public RuleCall getChildrenPropertyListParserRuleCall_5_3_3_0() { return cChildrenPropertyListParserRuleCall_5_3_3_0; }
//('inverse' 'of' inverse=ValidID)?
public Group getGroup_5_4() { return cGroup_5_4; }
//'inverse'
public Keyword getInverseKeyword_5_4_0() { return cInverseKeyword_5_4_0; }
//'of'
public Keyword getOfKeyword_5_4_1() { return cOfKeyword_5_4_1; }
//inverse=ValidID
public Assignment getInverseAssignment_5_4_2() { return cInverseAssignment_5_4_2; }
//ValidID
public RuleCall getInverseValidIDParserRuleCall_5_4_2_0() { return cInverseValidIDParserRuleCall_5_4_2_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_6() { return cGroup_6; }
//'with'
public Keyword getWithKeyword_6_0() { return cWithKeyword_6_0; }
//'metadata'
public Keyword getMetadataKeyword_6_1() { return cMetadataKeyword_6_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_6_2() { return cMetadataAssignment_6_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_6_2_0() { return cMetadataMetadataParserRuleCall_6_2_0; }
}
public class ConceptListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ConceptList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cConceptAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final RuleCall cConceptConceptDeclarationParserRuleCall_0_0_0 = (RuleCall)cConceptAssignment_0_0.eContents().get(0);
private final Group cGroup_0_1 = (Group)cAlternatives_0.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_0_1_0 = (Keyword)cGroup_0_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_0_1_1 = (Assignment)cGroup_0_1.eContents().get(1);
private final RuleCall cDefinitionsSubConceptStatementParserRuleCall_0_1_1_0 = (RuleCall)cDefinitionsAssignment_0_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_0_1_2 = (Keyword)cGroup_0_1.eContents().get(2);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Assignment cConjunctionsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Alternatives cConjunctionsAlternatives_1_0_0 = (Alternatives)cConjunctionsAssignment_1_0.eContents().get(0);
private final Keyword cConjunctionsCommaKeyword_1_0_0_0 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(0);
private final Keyword cConjunctionsVerticalLineKeyword_1_0_0_1 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(1);
private final Alternatives cAlternatives_1_1 = (Alternatives)cGroup_1.eContents().get(1);
private final Assignment cConceptAssignment_1_1_0 = (Assignment)cAlternatives_1_1.eContents().get(0);
private final RuleCall cConceptConceptDeclarationParserRuleCall_1_1_0_0 = (RuleCall)cConceptAssignment_1_1_0.eContents().get(0);
private final Group cGroup_1_1_1 = (Group)cAlternatives_1_1.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_1_1_1_0 = (Keyword)cGroup_1_1_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_1_1_1_1 = (Assignment)cGroup_1_1_1.eContents().get(1);
private final RuleCall cDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0 = (RuleCall)cDefinitionsAssignment_1_1_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_1_1_1_2 = (Keyword)cGroup_1_1_1.eContents().get(2);
/// **
// * TODO needs either a comma or a | for intersection/union
// * / ConceptList:
// (concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')') (conjunctions+=(',' | '|')
// (concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*;
@Override public ParserRule getRule() { return rule; }
//(concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')') (conjunctions+=(',' | '|')
//(concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*
public Group getGroup() { return cGroup; }
//(concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')')
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//concept+=ConceptDeclaration
public Assignment getConceptAssignment_0_0() { return cConceptAssignment_0_0; }
//ConceptDeclaration
public RuleCall getConceptConceptDeclarationParserRuleCall_0_0_0() { return cConceptConceptDeclarationParserRuleCall_0_0_0; }
//'(' definitions+=SubConceptStatement ')'
public Group getGroup_0_1() { return cGroup_0_1; }
//'('
public Keyword getLeftParenthesisKeyword_0_1_0() { return cLeftParenthesisKeyword_0_1_0; }
//definitions+=SubConceptStatement
public Assignment getDefinitionsAssignment_0_1_1() { return cDefinitionsAssignment_0_1_1; }
//SubConceptStatement
public RuleCall getDefinitionsSubConceptStatementParserRuleCall_0_1_1_0() { return cDefinitionsSubConceptStatementParserRuleCall_0_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_0_1_2() { return cRightParenthesisKeyword_0_1_2; }
//(conjunctions+=(',' | '|') (concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*
public Group getGroup_1() { return cGroup_1; }
//conjunctions+=(',' | '|')
public Assignment getConjunctionsAssignment_1_0() { return cConjunctionsAssignment_1_0; }
//(',' | '|')
public Alternatives getConjunctionsAlternatives_1_0_0() { return cConjunctionsAlternatives_1_0_0; }
//','
public Keyword getConjunctionsCommaKeyword_1_0_0_0() { return cConjunctionsCommaKeyword_1_0_0_0; }
//'|'
public Keyword getConjunctionsVerticalLineKeyword_1_0_0_1() { return cConjunctionsVerticalLineKeyword_1_0_0_1; }
//(concept+=ConceptDeclaration | '(' definitions+=SubConceptStatement ')')
public Alternatives getAlternatives_1_1() { return cAlternatives_1_1; }
//concept+=ConceptDeclaration
public Assignment getConceptAssignment_1_1_0() { return cConceptAssignment_1_1_0; }
//ConceptDeclaration
public RuleCall getConceptConceptDeclarationParserRuleCall_1_1_0_0() { return cConceptConceptDeclarationParserRuleCall_1_1_0_0; }
//'(' definitions+=SubConceptStatement ')'
public Group getGroup_1_1_1() { return cGroup_1_1_1; }
//'('
public Keyword getLeftParenthesisKeyword_1_1_1_0() { return cLeftParenthesisKeyword_1_1_1_0; }
//definitions+=SubConceptStatement
public Assignment getDefinitionsAssignment_1_1_1_1() { return cDefinitionsAssignment_1_1_1_1; }
//SubConceptStatement
public RuleCall getDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0() { return cDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_1_1_1_2() { return cRightParenthesisKeyword_1_1_1_2; }
}
public class DerivedConceptListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.DerivedConceptList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cConceptAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final RuleCall cConceptDerivedConceptDeclarationParserRuleCall_0_0_0 = (RuleCall)cConceptAssignment_0_0.eContents().get(0);
private final Group cGroup_0_1 = (Group)cAlternatives_0.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_0_1_0 = (Keyword)cGroup_0_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_0_1_1 = (Assignment)cGroup_0_1.eContents().get(1);
private final RuleCall cDefinitionsSubConceptStatementParserRuleCall_0_1_1_0 = (RuleCall)cDefinitionsAssignment_0_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_0_1_2 = (Keyword)cGroup_0_1.eContents().get(2);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Assignment cConjunctionsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Alternatives cConjunctionsAlternatives_1_0_0 = (Alternatives)cConjunctionsAssignment_1_0.eContents().get(0);
private final Keyword cConjunctionsCommaKeyword_1_0_0_0 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(0);
private final Keyword cConjunctionsVerticalLineKeyword_1_0_0_1 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(1);
private final Alternatives cAlternatives_1_1 = (Alternatives)cGroup_1.eContents().get(1);
private final Assignment cConceptAssignment_1_1_0 = (Assignment)cAlternatives_1_1.eContents().get(0);
private final RuleCall cConceptDerivedConceptDeclarationParserRuleCall_1_1_0_0 = (RuleCall)cConceptAssignment_1_1_0.eContents().get(0);
private final Group cGroup_1_1_1 = (Group)cAlternatives_1_1.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_1_1_1_0 = (Keyword)cGroup_1_1_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_1_1_1_1 = (Assignment)cGroup_1_1_1.eContents().get(1);
private final RuleCall cDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0 = (RuleCall)cDefinitionsAssignment_1_1_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_1_1_1_2 = (Keyword)cGroup_1_1_1.eContents().get(2);
//DerivedConceptList ConceptList:
// (concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')') (conjunctions+=(',' | '|')
// (concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*
@Override public ParserRule getRule() { return rule; }
//(concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')') (conjunctions+=(',' | '|')
//(concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*
public Group getGroup() { return cGroup; }
//(concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')')
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//concept+=DerivedConceptDeclaration
public Assignment getConceptAssignment_0_0() { return cConceptAssignment_0_0; }
//DerivedConceptDeclaration
public RuleCall getConceptDerivedConceptDeclarationParserRuleCall_0_0_0() { return cConceptDerivedConceptDeclarationParserRuleCall_0_0_0; }
//'(' definitions+=SubConceptStatement ')'
public Group getGroup_0_1() { return cGroup_0_1; }
//'('
public Keyword getLeftParenthesisKeyword_0_1_0() { return cLeftParenthesisKeyword_0_1_0; }
//definitions+=SubConceptStatement
public Assignment getDefinitionsAssignment_0_1_1() { return cDefinitionsAssignment_0_1_1; }
//SubConceptStatement
public RuleCall getDefinitionsSubConceptStatementParserRuleCall_0_1_1_0() { return cDefinitionsSubConceptStatementParserRuleCall_0_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_0_1_2() { return cRightParenthesisKeyword_0_1_2; }
//(conjunctions+=(',' | '|') (concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')'))*
public Group getGroup_1() { return cGroup_1; }
//conjunctions+=(',' | '|')
public Assignment getConjunctionsAssignment_1_0() { return cConjunctionsAssignment_1_0; }
//(',' | '|')
public Alternatives getConjunctionsAlternatives_1_0_0() { return cConjunctionsAlternatives_1_0_0; }
//','
public Keyword getConjunctionsCommaKeyword_1_0_0_0() { return cConjunctionsCommaKeyword_1_0_0_0; }
//'|'
public Keyword getConjunctionsVerticalLineKeyword_1_0_0_1() { return cConjunctionsVerticalLineKeyword_1_0_0_1; }
//(concept+=DerivedConceptDeclaration | '(' definitions+=SubConceptStatement ')')
public Alternatives getAlternatives_1_1() { return cAlternatives_1_1; }
//concept+=DerivedConceptDeclaration
public Assignment getConceptAssignment_1_1_0() { return cConceptAssignment_1_1_0; }
//DerivedConceptDeclaration
public RuleCall getConceptDerivedConceptDeclarationParserRuleCall_1_1_0_0() { return cConceptDerivedConceptDeclarationParserRuleCall_1_1_0_0; }
//'(' definitions+=SubConceptStatement ')'
public Group getGroup_1_1_1() { return cGroup_1_1_1; }
//'('
public Keyword getLeftParenthesisKeyword_1_1_1_0() { return cLeftParenthesisKeyword_1_1_1_0; }
//definitions+=SubConceptStatement
public Assignment getDefinitionsAssignment_1_1_1_1() { return cDefinitionsAssignment_1_1_1_1; }
//SubConceptStatement
public RuleCall getDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0() { return cDefinitionsSubConceptStatementParserRuleCall_1_1_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_1_1_1_2() { return cRightParenthesisKeyword_1_1_1_2; }
}
public class NegatableConceptListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.NegatableConceptList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cConceptAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cConceptNegatableConceptDeclarationParserRuleCall_0_0 = (RuleCall)cConceptAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cConceptAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cConceptNegatableConceptDeclarationParserRuleCall_1_1_0 = (RuleCall)cConceptAssignment_1_1.eContents().get(0);
//NegatableConceptList ConceptList:
// concept+=NegatableConceptDeclaration (=> ',' concept+=NegatableConceptDeclaration)*
@Override public ParserRule getRule() { return rule; }
//concept+=NegatableConceptDeclaration (=> ',' concept+=NegatableConceptDeclaration)*
public Group getGroup() { return cGroup; }
//concept+=NegatableConceptDeclaration
public Assignment getConceptAssignment_0() { return cConceptAssignment_0; }
//NegatableConceptDeclaration
public RuleCall getConceptNegatableConceptDeclarationParserRuleCall_0_0() { return cConceptNegatableConceptDeclarationParserRuleCall_0_0; }
//(=> ',' concept+=NegatableConceptDeclaration)*
public Group getGroup_1() { return cGroup_1; }
//=> ','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//concept+=NegatableConceptDeclaration
public Assignment getConceptAssignment_1_1() { return cConceptAssignment_1_1; }
//NegatableConceptDeclaration
public RuleCall getConceptNegatableConceptDeclarationParserRuleCall_1_1_0() { return cConceptNegatableConceptDeclarationParserRuleCall_1_1_0; }
}
public class NegatableDerivedConceptListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.NegatableDerivedConceptList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cConceptAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cConceptNegatableDerivedConceptDeclarationParserRuleCall_0_0 = (RuleCall)cConceptAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cConceptAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cConceptNegatableDerivedConceptDeclarationParserRuleCall_1_1_0 = (RuleCall)cConceptAssignment_1_1.eContents().get(0);
//NegatableDerivedConceptList ConceptList:
// concept+=NegatableDerivedConceptDeclaration (=> ',' concept+=NegatableDerivedConceptDeclaration)*
@Override public ParserRule getRule() { return rule; }
//concept+=NegatableDerivedConceptDeclaration (=> ',' concept+=NegatableDerivedConceptDeclaration)*
public Group getGroup() { return cGroup; }
//concept+=NegatableDerivedConceptDeclaration
public Assignment getConceptAssignment_0() { return cConceptAssignment_0; }
//NegatableDerivedConceptDeclaration
public RuleCall getConceptNegatableDerivedConceptDeclarationParserRuleCall_0_0() { return cConceptNegatableDerivedConceptDeclarationParserRuleCall_0_0; }
//(=> ',' concept+=NegatableDerivedConceptDeclaration)*
public Group getGroup_1() { return cGroup_1; }
//=> ','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//concept+=NegatableDerivedConceptDeclaration
public Assignment getConceptAssignment_1_1() { return cConceptAssignment_1_1; }
//NegatableDerivedConceptDeclaration
public RuleCall getConceptNegatableDerivedConceptDeclarationParserRuleCall_1_1_0() { return cConceptNegatableDerivedConceptDeclarationParserRuleCall_1_1_0; }
}
public class PropertyListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.PropertyList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final Assignment cPropertyAssignment_0_0 = (Assignment)cAlternatives_0.eContents().get(0);
private final RuleCall cPropertyValidIDParserRuleCall_0_0_0 = (RuleCall)cPropertyAssignment_0_0.eContents().get(0);
private final Group cGroup_0_1 = (Group)cAlternatives_0.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_0_1_0 = (Keyword)cGroup_0_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_0_1_1 = (Assignment)cGroup_0_1.eContents().get(1);
private final RuleCall cDefinitionsSubPropertyStatementParserRuleCall_0_1_1_0 = (RuleCall)cDefinitionsAssignment_0_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_0_1_2 = (Keyword)cGroup_0_1.eContents().get(2);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Assignment cConjunctionsAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Alternatives cConjunctionsAlternatives_1_0_0 = (Alternatives)cConjunctionsAssignment_1_0.eContents().get(0);
private final Keyword cConjunctionsCommaKeyword_1_0_0_0 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(0);
private final Keyword cConjunctionsVerticalLineKeyword_1_0_0_1 = (Keyword)cConjunctionsAlternatives_1_0_0.eContents().get(1);
private final Alternatives cAlternatives_1_1 = (Alternatives)cGroup_1.eContents().get(1);
private final Assignment cPropertyAssignment_1_1_0 = (Assignment)cAlternatives_1_1.eContents().get(0);
private final RuleCall cPropertyValidIDParserRuleCall_1_1_0_0 = (RuleCall)cPropertyAssignment_1_1_0.eContents().get(0);
private final Group cGroup_1_1_1 = (Group)cAlternatives_1_1.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_1_1_1_0 = (Keyword)cGroup_1_1_1.eContents().get(0);
private final Assignment cDefinitionsAssignment_1_1_1_1 = (Assignment)cGroup_1_1_1.eContents().get(1);
private final RuleCall cDefinitionsSubPropertyStatementParserRuleCall_1_1_1_1_0 = (RuleCall)cDefinitionsAssignment_1_1_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_1_1_1_2 = (Keyword)cGroup_1_1_1.eContents().get(2);
//PropertyList:
// (property+=ValidID | '(' definitions+=SubPropertyStatement ')') (conjunctions+=(',' | '|') (property+=ValidID | '('
// definitions+=SubPropertyStatement ')'))*;
@Override public ParserRule getRule() { return rule; }
//(property+=ValidID | '(' definitions+=SubPropertyStatement ')') (conjunctions+=(',' | '|') (property+=ValidID | '('
//definitions+=SubPropertyStatement ')'))*
public Group getGroup() { return cGroup; }
//(property+=ValidID | '(' definitions+=SubPropertyStatement ')')
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//property+=ValidID
public Assignment getPropertyAssignment_0_0() { return cPropertyAssignment_0_0; }
//ValidID
public RuleCall getPropertyValidIDParserRuleCall_0_0_0() { return cPropertyValidIDParserRuleCall_0_0_0; }
//'(' definitions+=SubPropertyStatement ')'
public Group getGroup_0_1() { return cGroup_0_1; }
//'('
public Keyword getLeftParenthesisKeyword_0_1_0() { return cLeftParenthesisKeyword_0_1_0; }
//definitions+=SubPropertyStatement
public Assignment getDefinitionsAssignment_0_1_1() { return cDefinitionsAssignment_0_1_1; }
//SubPropertyStatement
public RuleCall getDefinitionsSubPropertyStatementParserRuleCall_0_1_1_0() { return cDefinitionsSubPropertyStatementParserRuleCall_0_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_0_1_2() { return cRightParenthesisKeyword_0_1_2; }
//(conjunctions+=(',' | '|') (property+=ValidID | '(' definitions+=SubPropertyStatement ')'))*
public Group getGroup_1() { return cGroup_1; }
//conjunctions+=(',' | '|')
public Assignment getConjunctionsAssignment_1_0() { return cConjunctionsAssignment_1_0; }
//(',' | '|')
public Alternatives getConjunctionsAlternatives_1_0_0() { return cConjunctionsAlternatives_1_0_0; }
//','
public Keyword getConjunctionsCommaKeyword_1_0_0_0() { return cConjunctionsCommaKeyword_1_0_0_0; }
//'|'
public Keyword getConjunctionsVerticalLineKeyword_1_0_0_1() { return cConjunctionsVerticalLineKeyword_1_0_0_1; }
//(property+=ValidID | '(' definitions+=SubPropertyStatement ')')
public Alternatives getAlternatives_1_1() { return cAlternatives_1_1; }
//property+=ValidID
public Assignment getPropertyAssignment_1_1_0() { return cPropertyAssignment_1_1_0; }
//ValidID
public RuleCall getPropertyValidIDParserRuleCall_1_1_0_0() { return cPropertyValidIDParserRuleCall_1_1_0_0; }
//'(' definitions+=SubPropertyStatement ')'
public Group getGroup_1_1_1() { return cGroup_1_1_1; }
//'('
public Keyword getLeftParenthesisKeyword_1_1_1_0() { return cLeftParenthesisKeyword_1_1_1_0; }
//definitions+=SubPropertyStatement
public Assignment getDefinitionsAssignment_1_1_1_1() { return cDefinitionsAssignment_1_1_1_1; }
//SubPropertyStatement
public RuleCall getDefinitionsSubPropertyStatementParserRuleCall_1_1_1_1_0() { return cDefinitionsSubPropertyStatementParserRuleCall_1_1_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_1_1_1_2() { return cRightParenthesisKeyword_1_1_1_2; }
}
public class ModifierListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ModifierList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cModifierAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cModifierPropertyModifierEnumRuleCall_0_0 = (RuleCall)cModifierAssignment_0.eContents().get(0);
private final Assignment cModifierAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cModifierPropertyModifierEnumRuleCall_1_0 = (RuleCall)cModifierAssignment_1.eContents().get(0);
//ModifierList:
// modifier+=PropertyModifier modifier+=PropertyModifier*;
@Override public ParserRule getRule() { return rule; }
//modifier+=PropertyModifier modifier+=PropertyModifier*
public Group getGroup() { return cGroup; }
//modifier+=PropertyModifier
public Assignment getModifierAssignment_0() { return cModifierAssignment_0; }
//PropertyModifier
public RuleCall getModifierPropertyModifierEnumRuleCall_0_0() { return cModifierPropertyModifierEnumRuleCall_0_0; }
//modifier+=PropertyModifier*
public Assignment getModifierAssignment_1() { return cModifierAssignment_1; }
//PropertyModifier
public RuleCall getModifierPropertyModifierEnumRuleCall_1_0() { return cModifierPropertyModifierEnumRuleCall_1_0; }
}
public class AnnotationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Annotation");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cIdAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cIdANNOTATION_IDTerminalRuleCall_0_0 = (RuleCall)cIdAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cLeftParenthesisKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cParametersAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cParametersParameterListParserRuleCall_1_1_0 = (RuleCall)cParametersAssignment_1_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_1_2 = (Keyword)cGroup_1.eContents().get(2);
//Annotation:
// id=ANNOTATION_ID ('(' parameters=ParameterList? ')')?;
@Override public ParserRule getRule() { return rule; }
//id=ANNOTATION_ID ('(' parameters=ParameterList? ')')?
public Group getGroup() { return cGroup; }
//id=ANNOTATION_ID
public Assignment getIdAssignment_0() { return cIdAssignment_0; }
//ANNOTATION_ID
public RuleCall getIdANNOTATION_IDTerminalRuleCall_0_0() { return cIdANNOTATION_IDTerminalRuleCall_0_0; }
//('(' parameters=ParameterList? ')')?
public Group getGroup_1() { return cGroup_1; }
//'('
public Keyword getLeftParenthesisKeyword_1_0() { return cLeftParenthesisKeyword_1_0; }
//parameters=ParameterList?
public Assignment getParametersAssignment_1_1() { return cParametersAssignment_1_1; }
//ParameterList
public RuleCall getParametersParameterListParserRuleCall_1_1_0() { return cParametersParameterListParserRuleCall_1_1_0; }
//')'
public Keyword getRightParenthesisKeyword_1_2() { return cRightParenthesisKeyword_1_2; }
}
public class QualifiedNameListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.QualifiedNameList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cNamesAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cNamesValidIDParserRuleCall_0_0 = (RuleCall)cNamesAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cNamesAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cNamesValidIDParserRuleCall_1_1_0 = (RuleCall)cNamesAssignment_1_1.eContents().get(0);
//QualifiedNameList:
// names+=ValidID (=> ',' names+=ValidID)*;
@Override public ParserRule getRule() { return rule; }
//names+=ValidID (=> ',' names+=ValidID)*
public Group getGroup() { return cGroup; }
//names+=ValidID
public Assignment getNamesAssignment_0() { return cNamesAssignment_0; }
//ValidID
public RuleCall getNamesValidIDParserRuleCall_0_0() { return cNamesValidIDParserRuleCall_0_0; }
//(=> ',' names+=ValidID)*
public Group getGroup_1() { return cGroup_1; }
//=> ','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//names+=ValidID
public Assignment getNamesAssignment_1_1() { return cNamesAssignment_1_1; }
//ValidID
public RuleCall getNamesValidIDParserRuleCall_1_1_0() { return cNamesValidIDParserRuleCall_1_1_0; }
}
public class FunctionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Function");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cIdAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cIdValidIDParserRuleCall_0_0 = (RuleCall)cIdAssignment_0.eContents().get(0);
private final Keyword cLeftParenthesisKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cParametersAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cParametersParameterListParserRuleCall_2_0 = (RuleCall)cParametersAssignment_2.eContents().get(0);
private final Keyword cRightParenthesisKeyword_3 = (Keyword)cGroup.eContents().get(3);
//Function:
// id=ValidID '(' parameters=ParameterList? ')';
@Override public ParserRule getRule() { return rule; }
//id=ValidID '(' parameters=ParameterList? ')'
public Group getGroup() { return cGroup; }
//id=ValidID
public Assignment getIdAssignment_0() { return cIdAssignment_0; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_0_0() { return cIdValidIDParserRuleCall_0_0; }
//'('
public Keyword getLeftParenthesisKeyword_1() { return cLeftParenthesisKeyword_1; }
//parameters=ParameterList?
public Assignment getParametersAssignment_2() { return cParametersAssignment_2; }
//ParameterList
public RuleCall getParametersParameterListParserRuleCall_2_0() { return cParametersParameterListParserRuleCall_2_0; }
//')'
public Keyword getRightParenthesisKeyword_3() { return cRightParenthesisKeyword_3; }
}
public class ContextualizationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Contextualization");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cIntegratedAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final Keyword cIntegratedAggregatedKeyword_0_0_0 = (Keyword)cIntegratedAssignment_0_0.eContents().get(0);
private final Keyword cOverKeyword_0_1 = (Keyword)cGroup_0.eContents().get(1);
private final Assignment cDomainAssignment_0_2 = (Assignment)cGroup_0.eContents().get(2);
private final RuleCall cDomainFunctionOrIDParserRuleCall_0_2_0 = (RuleCall)cDomainAssignment_0_2.eContents().get(0);
private final Group cGroup_0_3 = (Group)cGroup_0.eContents().get(3);
private final Keyword cCommaKeyword_0_3_0 = (Keyword)cGroup_0_3.eContents().get(0);
private final Assignment cDomainAssignment_0_3_1 = (Assignment)cGroup_0_3.eContents().get(1);
private final RuleCall cDomainFunctionOrIDParserRuleCall_0_3_1_0 = (RuleCall)cDomainAssignment_0_3_1.eContents().get(0);
private final Group cGroup_0_4 = (Group)cGroup_0.eContents().get(4);
private final Assignment cActionsAssignment_0_4_0 = (Assignment)cGroup_0_4.eContents().get(0);
private final RuleCall cActionsActionParserRuleCall_0_4_0_0 = (RuleCall)cActionsAssignment_0_4_0.eContents().get(0);
private final Group cGroup_0_4_1 = (Group)cGroup_0_4.eContents().get(1);
private final Keyword cCommaKeyword_0_4_1_0 = (Keyword)cGroup_0_4_1.eContents().get(0);
private final Assignment cActionsAssignment_0_4_1_1 = (Assignment)cGroup_0_4_1.eContents().get(1);
private final RuleCall cActionsActionParserRuleCall_0_4_1_1_0 = (RuleCall)cActionsAssignment_0_4_1_1.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Keyword cOnKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Alternatives cAlternatives_1_1 = (Alternatives)cGroup_1.eContents().get(1);
private final Assignment cInitializationAssignment_1_1_0 = (Assignment)cAlternatives_1_1.eContents().get(0);
private final Keyword cInitializationDefinitionKeyword_1_1_0_0 = (Keyword)cInitializationAssignment_1_1_0.eContents().get(0);
private final Assignment cResolutionAssignment_1_1_1 = (Assignment)cAlternatives_1_1.eContents().get(1);
private final Keyword cResolutionResolutionKeyword_1_1_1_0 = (Keyword)cResolutionAssignment_1_1_1.eContents().get(0);
private final Assignment cEventAssignment_1_1_2 = (Assignment)cAlternatives_1_1.eContents().get(2);
private final RuleCall cEventConceptDeclarationParserRuleCall_1_1_2_0 = (RuleCall)cEventAssignment_1_1_2.eContents().get(0);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Assignment cActionsAssignment_1_2_0 = (Assignment)cGroup_1_2.eContents().get(0);
private final RuleCall cActionsActionParserRuleCall_1_2_0_0 = (RuleCall)cActionsAssignment_1_2_0.eContents().get(0);
private final Group cGroup_1_2_1 = (Group)cGroup_1_2.eContents().get(1);
private final Keyword cCommaKeyword_1_2_1_0 = (Keyword)cGroup_1_2_1.eContents().get(0);
private final Assignment cActionsAssignment_1_2_1_1 = (Assignment)cGroup_1_2_1.eContents().get(1);
private final RuleCall cActionsActionParserRuleCall_1_2_1_1_0 = (RuleCall)cActionsAssignment_1_2_1_1.eContents().get(0);
//Contextualization:
// integrated?='aggregated'? 'over' domain+=FunctionOrID (=> ',' domain+=FunctionOrID)* (actions+=Action (=> ','
// actions+=Action)*)? | 'on' (initialization?='definition' | resolution?='resolution' | event=ConceptDeclaration)
// (actions+=Action (=> ',' actions+=Action)*)?;
@Override public ParserRule getRule() { return rule; }
//integrated?='aggregated'? 'over' domain+=FunctionOrID (=> ',' domain+=FunctionOrID)* (actions+=Action (=> ','
//actions+=Action)*)? | 'on' (initialization?='definition' | resolution?='resolution' | event=ConceptDeclaration)
//(actions+=Action (=> ',' actions+=Action)*)?
public Alternatives getAlternatives() { return cAlternatives; }
//integrated?='aggregated'? 'over' domain+=FunctionOrID (=> ',' domain+=FunctionOrID)* (actions+=Action (=> ','
//actions+=Action)*)?
public Group getGroup_0() { return cGroup_0; }
//integrated?='aggregated'?
public Assignment getIntegratedAssignment_0_0() { return cIntegratedAssignment_0_0; }
//'aggregated'
public Keyword getIntegratedAggregatedKeyword_0_0_0() { return cIntegratedAggregatedKeyword_0_0_0; }
//'over'
public Keyword getOverKeyword_0_1() { return cOverKeyword_0_1; }
//domain+=FunctionOrID
public Assignment getDomainAssignment_0_2() { return cDomainAssignment_0_2; }
//FunctionOrID
public RuleCall getDomainFunctionOrIDParserRuleCall_0_2_0() { return cDomainFunctionOrIDParserRuleCall_0_2_0; }
//(=> ',' domain+=FunctionOrID)*
public Group getGroup_0_3() { return cGroup_0_3; }
//=> ','
public Keyword getCommaKeyword_0_3_0() { return cCommaKeyword_0_3_0; }
//domain+=FunctionOrID
public Assignment getDomainAssignment_0_3_1() { return cDomainAssignment_0_3_1; }
//FunctionOrID
public RuleCall getDomainFunctionOrIDParserRuleCall_0_3_1_0() { return cDomainFunctionOrIDParserRuleCall_0_3_1_0; }
//(actions+=Action (=> ',' actions+=Action)*)?
public Group getGroup_0_4() { return cGroup_0_4; }
//actions+=Action
public Assignment getActionsAssignment_0_4_0() { return cActionsAssignment_0_4_0; }
//Action
public RuleCall getActionsActionParserRuleCall_0_4_0_0() { return cActionsActionParserRuleCall_0_4_0_0; }
//(=> ',' actions+=Action)*
public Group getGroup_0_4_1() { return cGroup_0_4_1; }
//=> ','
public Keyword getCommaKeyword_0_4_1_0() { return cCommaKeyword_0_4_1_0; }
//actions+=Action
public Assignment getActionsAssignment_0_4_1_1() { return cActionsAssignment_0_4_1_1; }
//Action
public RuleCall getActionsActionParserRuleCall_0_4_1_1_0() { return cActionsActionParserRuleCall_0_4_1_1_0; }
//'on' (initialization?='definition' | resolution?='resolution' | event=ConceptDeclaration) (actions+=Action (=> ','
//actions+=Action)*)?
public Group getGroup_1() { return cGroup_1; }
//'on'
public Keyword getOnKeyword_1_0() { return cOnKeyword_1_0; }
//(initialization?='definition' | resolution?='resolution' | event=ConceptDeclaration)
public Alternatives getAlternatives_1_1() { return cAlternatives_1_1; }
//initialization?='definition'
public Assignment getInitializationAssignment_1_1_0() { return cInitializationAssignment_1_1_0; }
//'definition'
public Keyword getInitializationDefinitionKeyword_1_1_0_0() { return cInitializationDefinitionKeyword_1_1_0_0; }
//resolution?='resolution'
public Assignment getResolutionAssignment_1_1_1() { return cResolutionAssignment_1_1_1; }
//'resolution'
public Keyword getResolutionResolutionKeyword_1_1_1_0() { return cResolutionResolutionKeyword_1_1_1_0; }
//event=ConceptDeclaration
public Assignment getEventAssignment_1_1_2() { return cEventAssignment_1_1_2; }
//ConceptDeclaration
public RuleCall getEventConceptDeclarationParserRuleCall_1_1_2_0() { return cEventConceptDeclarationParserRuleCall_1_1_2_0; }
//(actions+=Action (=> ',' actions+=Action)*)?
public Group getGroup_1_2() { return cGroup_1_2; }
//actions+=Action
public Assignment getActionsAssignment_1_2_0() { return cActionsAssignment_1_2_0; }
//Action
public RuleCall getActionsActionParserRuleCall_1_2_0_0() { return cActionsActionParserRuleCall_1_2_0_0; }
//(=> ',' actions+=Action)*
public Group getGroup_1_2_1() { return cGroup_1_2_1; }
//=> ','
public Keyword getCommaKeyword_1_2_1_0() { return cCommaKeyword_1_2_1_0; }
//actions+=Action
public Assignment getActionsAssignment_1_2_1_1() { return cActionsAssignment_1_2_1_1; }
//Action
public RuleCall getActionsActionParserRuleCall_1_2_1_1_0() { return cActionsActionParserRuleCall_1_2_1_1_0; }
}
public class FunctionOrIDElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.FunctionOrID");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cFunctionAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cFunctionFunctionParserRuleCall_0_0 = (RuleCall)cFunctionAssignment_0.eContents().get(0);
private final Assignment cFunctionIdAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cFunctionIdValidIDParserRuleCall_1_0 = (RuleCall)cFunctionIdAssignment_1.eContents().get(0);
//FunctionOrID:
// function=Function | functionId=ValidID;
@Override public ParserRule getRule() { return rule; }
//function=Function | functionId=ValidID
public Alternatives getAlternatives() { return cAlternatives; }
//function=Function
public Assignment getFunctionAssignment_0() { return cFunctionAssignment_0; }
//Function
public RuleCall getFunctionFunctionParserRuleCall_0_0() { return cFunctionFunctionParserRuleCall_0_0; }
//functionId=ValidID
public Assignment getFunctionIdAssignment_1() { return cFunctionIdAssignment_1; }
//ValidID
public RuleCall getFunctionIdValidIDParserRuleCall_1_0() { return cFunctionIdValidIDParserRuleCall_1_0; }
}
public class ActionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Action");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Alternatives cAlternatives_0_0 = (Alternatives)cGroup_0.eContents().get(0);
private final Assignment cChangeAssignment_0_0_0 = (Assignment)cAlternatives_0_0.eContents().get(0);
private final Keyword cChangeChangeKeyword_0_0_0_0 = (Keyword)cChangeAssignment_0_0_0.eContents().get(0);
private final Assignment cSetAssignment_0_0_1 = (Assignment)cAlternatives_0_0.eContents().get(1);
private final Keyword cSetSetKeyword_0_0_1_0 = (Keyword)cSetAssignment_0_0_1.eContents().get(0);
private final Assignment cChangedAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final RuleCall cChangedValidIDParserRuleCall_0_1_0 = (RuleCall)cChangedAssignment_0_1.eContents().get(0);
private final Keyword cToKeyword_0_2 = (Keyword)cGroup_0.eContents().get(2);
private final Assignment cValueAssignment_0_3 = (Assignment)cGroup_0.eContents().get(3);
private final RuleCall cValueValueParserRuleCall_0_3_0 = (RuleCall)cValueAssignment_0_3.eContents().get(0);
private final Group cGroup_0_4 = (Group)cGroup_0.eContents().get(4);
private final Keyword cUsingKeyword_0_4_0 = (Keyword)cGroup_0_4.eContents().get(0);
private final Assignment cExtensionAssignment_0_4_1 = (Assignment)cGroup_0_4.eContents().get(1);
private final RuleCall cExtensionValidIDParserRuleCall_0_4_1_0 = (RuleCall)cExtensionAssignment_0_4_1.eContents().get(0);
private final Assignment cConditionAssignment_0_5 = (Assignment)cGroup_0.eContents().get(5);
private final RuleCall cConditionConditionParserRuleCall_0_5_0 = (RuleCall)cConditionAssignment_0_5.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cIntegrateAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final Keyword cIntegrateIntegrateKeyword_1_0_0 = (Keyword)cIntegrateAssignment_1_0.eContents().get(0);
private final Assignment cChangedAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cChangedValidIDParserRuleCall_1_1_0 = (RuleCall)cChangedAssignment_1_1.eContents().get(0);
private final Keyword cAsKeyword_1_2 = (Keyword)cGroup_1.eContents().get(2);
private final Assignment cValueAssignment_1_3 = (Assignment)cGroup_1.eContents().get(3);
private final RuleCall cValueValueParserRuleCall_1_3_0 = (RuleCall)cValueAssignment_1_3.eContents().get(0);
private final Group cGroup_1_4 = (Group)cGroup_1.eContents().get(4);
private final Keyword cUsingKeyword_1_4_0 = (Keyword)cGroup_1_4.eContents().get(0);
private final Assignment cExtensionAssignment_1_4_1 = (Assignment)cGroup_1_4.eContents().get(1);
private final RuleCall cExtensionValidIDParserRuleCall_1_4_1_0 = (RuleCall)cExtensionAssignment_1_4_1.eContents().get(0);
private final Assignment cConditionAssignment_1_5 = (Assignment)cGroup_1.eContents().get(5);
private final RuleCall cConditionConditionParserRuleCall_1_5_0 = (RuleCall)cConditionAssignment_1_5.eContents().get(0);
private final Group cGroup_2 = (Group)cAlternatives.eContents().get(2);
private final Assignment cDoAssignment_2_0 = (Assignment)cGroup_2.eContents().get(0);
private final Keyword cDoDoKeyword_2_0_0 = (Keyword)cDoAssignment_2_0.eContents().get(0);
private final Assignment cValueAssignment_2_1 = (Assignment)cGroup_2.eContents().get(1);
private final RuleCall cValueValueParserRuleCall_2_1_0 = (RuleCall)cValueAssignment_2_1.eContents().get(0);
private final Group cGroup_2_2 = (Group)cGroup_2.eContents().get(2);
private final Keyword cUsingKeyword_2_2_0 = (Keyword)cGroup_2_2.eContents().get(0);
private final Assignment cExtensionAssignment_2_2_1 = (Assignment)cGroup_2_2.eContents().get(1);
private final RuleCall cExtensionValidIDParserRuleCall_2_2_1_0 = (RuleCall)cExtensionAssignment_2_2_1.eContents().get(0);
private final Assignment cConditionAssignment_2_3 = (Assignment)cGroup_2.eContents().get(3);
private final RuleCall cConditionConditionParserRuleCall_2_3_0 = (RuleCall)cConditionAssignment_2_3.eContents().get(0);
private final Group cGroup_3 = (Group)cAlternatives.eContents().get(3);
private final Assignment cMoveAssignment_3_0 = (Assignment)cGroup_3.eContents().get(0);
private final Keyword cMoveMoveKeyword_3_0_0 = (Keyword)cMoveAssignment_3_0.eContents().get(0);
private final Alternatives cAlternatives_3_1 = (Alternatives)cGroup_3.eContents().get(1);
private final Assignment cWhereAssignment_3_1_0 = (Assignment)cAlternatives_3_1.eContents().get(0);
private final RuleCall cWhereValueParserRuleCall_3_1_0_0 = (RuleCall)cWhereAssignment_3_1_0.eContents().get(0);
private final Assignment cAwayAssignment_3_1_1 = (Assignment)cAlternatives_3_1.eContents().get(1);
private final Keyword cAwayAwayKeyword_3_1_1_0 = (Keyword)cAwayAssignment_3_1_1.eContents().get(0);
private final Assignment cConditionAssignment_3_2 = (Assignment)cGroup_3.eContents().get(2);
private final RuleCall cConditionConditionParserRuleCall_3_2_0 = (RuleCall)cConditionAssignment_3_2.eContents().get(0);
//Action:
// (change?='change' | set?='set') changed=ValidID? 'to' value=Value ('using' extension=ValidID)? =>
// condition=Condition? | integrate?='integrate' changed=ValidID? 'as' value=Value ('using' extension=ValidID)? =>
// condition=Condition? | do?='do' value=Value ('using' extension=ValidID)? => condition=Condition? | move?='move'
// (where=Value | away?='away') => condition=Condition?;
@Override public ParserRule getRule() { return rule; }
//(change?='change' | set?='set') changed=ValidID? 'to' value=Value ('using' extension=ValidID)? => condition=Condition? |
//integrate?='integrate' changed=ValidID? 'as' value=Value ('using' extension=ValidID)? => condition=Condition? |
//do?='do' value=Value ('using' extension=ValidID)? => condition=Condition? | move?='move' (where=Value | away?='away')
//=> condition=Condition?
public Alternatives getAlternatives() { return cAlternatives; }
//(change?='change' | set?='set') changed=ValidID? 'to' value=Value ('using' extension=ValidID)? => condition=Condition?
public Group getGroup_0() { return cGroup_0; }
//(change?='change' | set?='set')
public Alternatives getAlternatives_0_0() { return cAlternatives_0_0; }
//change?='change'
public Assignment getChangeAssignment_0_0_0() { return cChangeAssignment_0_0_0; }
//'change'
public Keyword getChangeChangeKeyword_0_0_0_0() { return cChangeChangeKeyword_0_0_0_0; }
//set?='set'
public Assignment getSetAssignment_0_0_1() { return cSetAssignment_0_0_1; }
//'set'
public Keyword getSetSetKeyword_0_0_1_0() { return cSetSetKeyword_0_0_1_0; }
//changed=ValidID?
public Assignment getChangedAssignment_0_1() { return cChangedAssignment_0_1; }
//ValidID
public RuleCall getChangedValidIDParserRuleCall_0_1_0() { return cChangedValidIDParserRuleCall_0_1_0; }
//'to'
public Keyword getToKeyword_0_2() { return cToKeyword_0_2; }
//value=Value
public Assignment getValueAssignment_0_3() { return cValueAssignment_0_3; }
//Value
public RuleCall getValueValueParserRuleCall_0_3_0() { return cValueValueParserRuleCall_0_3_0; }
//('using' extension=ValidID)?
public Group getGroup_0_4() { return cGroup_0_4; }
//'using'
public Keyword getUsingKeyword_0_4_0() { return cUsingKeyword_0_4_0; }
//extension=ValidID
public Assignment getExtensionAssignment_0_4_1() { return cExtensionAssignment_0_4_1; }
//ValidID
public RuleCall getExtensionValidIDParserRuleCall_0_4_1_0() { return cExtensionValidIDParserRuleCall_0_4_1_0; }
//=> condition=Condition?
public Assignment getConditionAssignment_0_5() { return cConditionAssignment_0_5; }
//Condition
public RuleCall getConditionConditionParserRuleCall_0_5_0() { return cConditionConditionParserRuleCall_0_5_0; }
//integrate?='integrate' changed=ValidID? 'as' value=Value ('using' extension=ValidID)? => condition=Condition?
public Group getGroup_1() { return cGroup_1; }
//integrate?='integrate'
public Assignment getIntegrateAssignment_1_0() { return cIntegrateAssignment_1_0; }
//'integrate'
public Keyword getIntegrateIntegrateKeyword_1_0_0() { return cIntegrateIntegrateKeyword_1_0_0; }
//changed=ValidID?
public Assignment getChangedAssignment_1_1() { return cChangedAssignment_1_1; }
//ValidID
public RuleCall getChangedValidIDParserRuleCall_1_1_0() { return cChangedValidIDParserRuleCall_1_1_0; }
//'as'
public Keyword getAsKeyword_1_2() { return cAsKeyword_1_2; }
//value=Value
public Assignment getValueAssignment_1_3() { return cValueAssignment_1_3; }
//Value
public RuleCall getValueValueParserRuleCall_1_3_0() { return cValueValueParserRuleCall_1_3_0; }
//('using' extension=ValidID)?
public Group getGroup_1_4() { return cGroup_1_4; }
//'using'
public Keyword getUsingKeyword_1_4_0() { return cUsingKeyword_1_4_0; }
//extension=ValidID
public Assignment getExtensionAssignment_1_4_1() { return cExtensionAssignment_1_4_1; }
//ValidID
public RuleCall getExtensionValidIDParserRuleCall_1_4_1_0() { return cExtensionValidIDParserRuleCall_1_4_1_0; }
//=> condition=Condition?
public Assignment getConditionAssignment_1_5() { return cConditionAssignment_1_5; }
//Condition
public RuleCall getConditionConditionParserRuleCall_1_5_0() { return cConditionConditionParserRuleCall_1_5_0; }
//do?='do' value=Value ('using' extension=ValidID)? => condition=Condition?
public Group getGroup_2() { return cGroup_2; }
//do?='do'
public Assignment getDoAssignment_2_0() { return cDoAssignment_2_0; }
//'do'
public Keyword getDoDoKeyword_2_0_0() { return cDoDoKeyword_2_0_0; }
//value=Value
public Assignment getValueAssignment_2_1() { return cValueAssignment_2_1; }
//Value
public RuleCall getValueValueParserRuleCall_2_1_0() { return cValueValueParserRuleCall_2_1_0; }
//('using' extension=ValidID)?
public Group getGroup_2_2() { return cGroup_2_2; }
//'using'
public Keyword getUsingKeyword_2_2_0() { return cUsingKeyword_2_2_0; }
//extension=ValidID
public Assignment getExtensionAssignment_2_2_1() { return cExtensionAssignment_2_2_1; }
//ValidID
public RuleCall getExtensionValidIDParserRuleCall_2_2_1_0() { return cExtensionValidIDParserRuleCall_2_2_1_0; }
//=> condition=Condition?
public Assignment getConditionAssignment_2_3() { return cConditionAssignment_2_3; }
//Condition
public RuleCall getConditionConditionParserRuleCall_2_3_0() { return cConditionConditionParserRuleCall_2_3_0; }
/// *
// * 'move away' sounds a lot nicer than 'die'
// * / move?='move' (where=Value | away?='away') => condition=Condition?
public Group getGroup_3() { return cGroup_3; }
/// *
// * 'move away' sounds a lot nicer than 'die'
// * / move?='move'
public Assignment getMoveAssignment_3_0() { return cMoveAssignment_3_0; }
//'move'
public Keyword getMoveMoveKeyword_3_0_0() { return cMoveMoveKeyword_3_0_0; }
//(where=Value | away?='away')
public Alternatives getAlternatives_3_1() { return cAlternatives_3_1; }
//where=Value
public Assignment getWhereAssignment_3_1_0() { return cWhereAssignment_3_1_0; }
//Value
public RuleCall getWhereValueParserRuleCall_3_1_0_0() { return cWhereValueParserRuleCall_3_1_0_0; }
//away?='away'
public Assignment getAwayAssignment_3_1_1() { return cAwayAssignment_3_1_1; }
//'away'
public Keyword getAwayAwayKeyword_3_1_1_0() { return cAwayAwayKeyword_3_1_1_0; }
//=> condition=Condition?
public Assignment getConditionAssignment_3_2() { return cConditionAssignment_3_2; }
//Condition
public RuleCall getConditionConditionParserRuleCall_3_2_0() { return cConditionConditionParserRuleCall_3_2_0; }
}
public class NamespaceElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Namespace");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cAnnotationsAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cAnnotationsAnnotationParserRuleCall_0_0 = (RuleCall)cAnnotationsAssignment_0.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_1 = (UnorderedGroup)cGroup.eContents().get(1);
private final Assignment cPrivateAssignment_1_0 = (Assignment)cUnorderedGroup_1.eContents().get(0);
private final Keyword cPrivatePrivateKeyword_1_0_0 = (Keyword)cPrivateAssignment_1_0.eContents().get(0);
private final Assignment cInactiveAssignment_1_1 = (Assignment)cUnorderedGroup_1.eContents().get(1);
private final Keyword cInactiveVoidKeyword_1_1_0 = (Keyword)cInactiveAssignment_1_1.eContents().get(0);
private final Alternatives cAlternatives_2 = (Alternatives)cGroup.eContents().get(2);
private final Keyword cNamespaceKeyword_2_0 = (Keyword)cAlternatives_2.eContents().get(0);
private final Assignment cScenarioAssignment_2_1 = (Assignment)cAlternatives_2.eContents().get(1);
private final Keyword cScenarioScenarioKeyword_2_1_0 = (Keyword)cScenarioAssignment_2_1.eContents().get(0);
private final Assignment cNameAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cNameValidIDParserRuleCall_3_0 = (RuleCall)cNameAssignment_3.eContents().get(0);
private final Assignment cDocstringAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final RuleCall cDocstringSTRINGTerminalRuleCall_4_0 = (RuleCall)cDocstringAssignment_4.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_5 = (UnorderedGroup)cGroup.eContents().get(5);
private final Group cGroup_5_0 = (Group)cUnorderedGroup_5.eContents().get(0);
private final Keyword cUsingKeyword_5_0_0 = (Keyword)cGroup_5_0.eContents().get(0);
private final Assignment cImportListAssignment_5_0_1 = (Assignment)cGroup_5_0.eContents().get(1);
private final RuleCall cImportListImportListParserRuleCall_5_0_1_0 = (RuleCall)cImportListAssignment_5_0_1.eContents().get(0);
private final Group cGroup_5_1 = (Group)cUnorderedGroup_5.eContents().get(1);
private final Keyword cCoveringKeyword_5_1_0 = (Keyword)cGroup_5_1.eContents().get(0);
private final Assignment cCoverageListAssignment_5_1_1 = (Assignment)cGroup_5_1.eContents().get(1);
private final RuleCall cCoverageListCoverageListParserRuleCall_5_1_1_0 = (RuleCall)cCoverageListAssignment_5_1_1.eContents().get(0);
private final Group cGroup_5_2 = (Group)cUnorderedGroup_5.eContents().get(2);
private final Keyword cExportsKeyword_5_2_0 = (Keyword)cGroup_5_2.eContents().get(0);
private final Assignment cExportListAssignment_5_2_1 = (Assignment)cGroup_5_2.eContents().get(1);
private final RuleCall cExportListValidIDParserRuleCall_5_2_1_0 = (RuleCall)cExportListAssignment_5_2_1.eContents().get(0);
private final Group cGroup_5_2_2 = (Group)cGroup_5_2.eContents().get(2);
private final Keyword cCommaKeyword_5_2_2_0 = (Keyword)cGroup_5_2_2.eContents().get(0);
private final Assignment cExportListAssignment_5_2_2_1 = (Assignment)cGroup_5_2_2.eContents().get(1);
private final RuleCall cExportListValidIDParserRuleCall_5_2_2_1_0 = (RuleCall)cExportListAssignment_5_2_2_1.eContents().get(0);
private final Group cGroup_5_3 = (Group)cUnorderedGroup_5.eContents().get(3);
private final Keyword cInKeyword_5_3_0 = (Keyword)cGroup_5_3.eContents().get(0);
private final Keyword cDomainKeyword_5_3_1 = (Keyword)cGroup_5_3.eContents().get(1);
private final Alternatives cAlternatives_5_3_2 = (Alternatives)cGroup_5_3.eContents().get(2);
private final Assignment cRootDomainAssignment_5_3_2_0 = (Assignment)cAlternatives_5_3_2.eContents().get(0);
private final Keyword cRootDomainRootKeyword_5_3_2_0_0 = (Keyword)cRootDomainAssignment_5_3_2_0.eContents().get(0);
private final Assignment cDomainConceptAssignment_5_3_2_1 = (Assignment)cAlternatives_5_3_2.eContents().get(1);
private final RuleCall cDomainConceptValidIDParserRuleCall_5_3_2_1_0 = (RuleCall)cDomainConceptAssignment_5_3_2_1.eContents().get(0);
private final Group cGroup_5_4 = (Group)cUnorderedGroup_5.eContents().get(4);
private final Keyword cDisjointKeyword_5_4_0 = (Keyword)cGroup_5_4.eContents().get(0);
private final Keyword cWithKeyword_5_4_1 = (Keyword)cGroup_5_4.eContents().get(1);
private final Assignment cDisjointNamespacesAssignment_5_4_2 = (Assignment)cGroup_5_4.eContents().get(2);
private final RuleCall cDisjointNamespacesQualifiedNameListParserRuleCall_5_4_2_0 = (RuleCall)cDisjointNamespacesAssignment_5_4_2.eContents().get(0);
private final Group cGroup_5_5 = (Group)cUnorderedGroup_5.eContents().get(5);
private final Keyword cVersionKeyword_5_5_0 = (Keyword)cGroup_5_5.eContents().get(0);
private final Assignment cVersionAssignment_5_5_1 = (Assignment)cGroup_5_5.eContents().get(1);
private final RuleCall cVersionValidIDParserRuleCall_5_5_1_0 = (RuleCall)cVersionAssignment_5_5_1.eContents().get(0);
private final Group cGroup_5_6 = (Group)cUnorderedGroup_5.eContents().get(6);
private final Keyword cResolveKeyword_5_6_0 = (Keyword)cGroup_5_6.eContents().get(0);
private final Group cGroup_5_6_1 = (Group)cGroup_5_6.eContents().get(1);
private final Keyword cFromKeyword_5_6_1_0 = (Keyword)cGroup_5_6_1.eContents().get(0);
private final Assignment cLookupNamespaceAssignment_5_6_1_1 = (Assignment)cGroup_5_6_1.eContents().get(1);
private final RuleCall cLookupNamespaceValidIDParserRuleCall_5_6_1_1_0 = (RuleCall)cLookupNamespaceAssignment_5_6_1_1.eContents().get(0);
private final Group cGroup_5_6_2 = (Group)cGroup_5_6.eContents().get(2);
private final Keyword cOutsideKeyword_5_6_2_0 = (Keyword)cGroup_5_6_2.eContents().get(0);
private final Assignment cBlacklistNamespaceAssignment_5_6_2_1 = (Assignment)cGroup_5_6_2.eContents().get(1);
private final RuleCall cBlacklistNamespaceValidIDParserRuleCall_5_6_2_1_0 = (RuleCall)cBlacklistNamespaceAssignment_5_6_2_1.eContents().get(0);
private final Group cGroup_5_6_3 = (Group)cGroup_5_6.eContents().get(3);
private final Keyword cUsingKeyword_5_6_3_0 = (Keyword)cGroup_5_6_3.eContents().get(0);
private final Assignment cWeightsAssignment_5_6_3_1 = (Assignment)cGroup_5_6_3.eContents().get(1);
private final RuleCall cWeightsMetadataParserRuleCall_5_6_3_1_0 = (RuleCall)cWeightsAssignment_5_6_3_1.eContents().get(0);
private final Group cGroup_5_7 = (Group)cUnorderedGroup_5.eContents().get(7);
private final Keyword cTrainKeyword_5_7_0 = (Keyword)cGroup_5_7.eContents().get(0);
private final Keyword cInKeyword_5_7_1 = (Keyword)cGroup_5_7.eContents().get(1);
private final Assignment cTrainingNamespaceAssignment_5_7_2 = (Assignment)cGroup_5_7.eContents().get(2);
private final RuleCall cTrainingNamespaceValidIDParserRuleCall_5_7_2_0 = (RuleCall)cTrainingNamespaceAssignment_5_7_2.eContents().get(0);
private final Group cGroup_6 = (Group)cGroup.eContents().get(6);
private final Keyword cWithKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Keyword cMetadataKeyword_6_1 = (Keyword)cGroup_6.eContents().get(1);
private final Assignment cMetadataAssignment_6_2 = (Assignment)cGroup_6.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_6_2_0 = (RuleCall)cMetadataAssignment_6_2.eContents().get(0);
private final Keyword cSemicolonKeyword_7 = (Keyword)cGroup.eContents().get(7);
/// *
// * Namespace - entry point of all files. Only interactive session may start without this statement.
// * A namespace may be a scenario - if so, nothing changes except its models will never be used from
// * the DB unless the scenario is being computed (should be 'observe ... in scenario ....).
// * /
//Namespace:
// annotations+=Annotation* (private?='private'? & inactive?='void'?) ('namespace' | scenario?='scenario') name=ValidID
// docstring=STRING? (('using' importList=ImportList)? & ('covering' coverageList=CoverageList)? & ('exports'
// exportList+=ValidID (=> ',' exportList+=ValidID)*)? & ('in' 'domain' (rootDomain?='root' | domainConcept=ValidID))? &
// ('disjoint' 'with' disjointNamespaces=QualifiedNameList)? & ('version' version=ValidID)? & ('resolve' ('from'
// lookupNamespace+=ValidID*)? ('outside' blacklistNamespace+=ValidID*)? ('using' weights=Metadata)?)? & ('train' 'in'
// trainingNamespace+=ValidID*)?) ('with' 'metadata' metadata=Metadata)? ';';
@Override public ParserRule getRule() { return rule; }
//annotations+=Annotation* (private?='private'? & inactive?='void'?) ('namespace' | scenario?='scenario') name=ValidID
//docstring=STRING? (('using' importList=ImportList)? & ('covering' coverageList=CoverageList)? & ('exports'
//exportList+=ValidID (=> ',' exportList+=ValidID)*)? & ('in' 'domain' (rootDomain?='root' | domainConcept=ValidID))? &
//('disjoint' 'with' disjointNamespaces=QualifiedNameList)? & ('version' version=ValidID)? & ('resolve' ('from'
//lookupNamespace+=ValidID*)? ('outside' blacklistNamespace+=ValidID*)? ('using' weights=Metadata)?)? & ('train' 'in'
//trainingNamespace+=ValidID*)?) ('with' 'metadata' metadata=Metadata)? ';'
public Group getGroup() { return cGroup; }
//annotations+=Annotation*
public Assignment getAnnotationsAssignment_0() { return cAnnotationsAssignment_0; }
//Annotation
public RuleCall getAnnotationsAnnotationParserRuleCall_0_0() { return cAnnotationsAnnotationParserRuleCall_0_0; }
//(private?='private'? & inactive?='void'?)
public UnorderedGroup getUnorderedGroup_1() { return cUnorderedGroup_1; }
//private?='private'?
public Assignment getPrivateAssignment_1_0() { return cPrivateAssignment_1_0; }
//'private'
public Keyword getPrivatePrivateKeyword_1_0_0() { return cPrivatePrivateKeyword_1_0_0; }
//inactive?='void'?
public Assignment getInactiveAssignment_1_1() { return cInactiveAssignment_1_1; }
//'void'
public Keyword getInactiveVoidKeyword_1_1_0() { return cInactiveVoidKeyword_1_1_0; }
//('namespace' | scenario?='scenario')
public Alternatives getAlternatives_2() { return cAlternatives_2; }
//'namespace'
public Keyword getNamespaceKeyword_2_0() { return cNamespaceKeyword_2_0; }
//scenario?='scenario'
public Assignment getScenarioAssignment_2_1() { return cScenarioAssignment_2_1; }
//'scenario'
public Keyword getScenarioScenarioKeyword_2_1_0() { return cScenarioScenarioKeyword_2_1_0; }
//name=ValidID
public Assignment getNameAssignment_3() { return cNameAssignment_3; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_3_0() { return cNameValidIDParserRuleCall_3_0; }
//docstring=STRING?
public Assignment getDocstringAssignment_4() { return cDocstringAssignment_4; }
//STRING
public RuleCall getDocstringSTRINGTerminalRuleCall_4_0() { return cDocstringSTRINGTerminalRuleCall_4_0; }
//(('using' importList=ImportList)? & ('covering' coverageList=CoverageList)? & ('exports' exportList+=ValidID (=> ','
//exportList+=ValidID)*)? & ('in' 'domain' (rootDomain?='root' | domainConcept=ValidID))? & ('disjoint' 'with'
//disjointNamespaces=QualifiedNameList)? & ('version' version=ValidID)? & ('resolve' ('from' lookupNamespace+=ValidID*)?
//('outside' blacklistNamespace+=ValidID*)? ('using' weights=Metadata)?)? & ('train' 'in' trainingNamespace+=ValidID*)?)
public UnorderedGroup getUnorderedGroup_5() { return cUnorderedGroup_5; }
//('using' importList=ImportList)?
public Group getGroup_5_0() { return cGroup_5_0; }
//'using'
public Keyword getUsingKeyword_5_0_0() { return cUsingKeyword_5_0_0; }
//importList=ImportList
public Assignment getImportListAssignment_5_0_1() { return cImportListAssignment_5_0_1; }
//ImportList
public RuleCall getImportListImportListParserRuleCall_5_0_1_0() { return cImportListImportListParserRuleCall_5_0_1_0; }
//('covering' coverageList=CoverageList)?
public Group getGroup_5_1() { return cGroup_5_1; }
//'covering'
public Keyword getCoveringKeyword_5_1_0() { return cCoveringKeyword_5_1_0; }
//coverageList=CoverageList
public Assignment getCoverageListAssignment_5_1_1() { return cCoverageListAssignment_5_1_1; }
//CoverageList
public RuleCall getCoverageListCoverageListParserRuleCall_5_1_1_0() { return cCoverageListCoverageListParserRuleCall_5_1_1_0; }
//('exports' exportList+=ValidID (=> ',' exportList+=ValidID)*)?
public Group getGroup_5_2() { return cGroup_5_2; }
//'exports'
public Keyword getExportsKeyword_5_2_0() { return cExportsKeyword_5_2_0; }
//exportList+=ValidID
public Assignment getExportListAssignment_5_2_1() { return cExportListAssignment_5_2_1; }
//ValidID
public RuleCall getExportListValidIDParserRuleCall_5_2_1_0() { return cExportListValidIDParserRuleCall_5_2_1_0; }
//(=> ',' exportList+=ValidID)*
public Group getGroup_5_2_2() { return cGroup_5_2_2; }
//=> ','
public Keyword getCommaKeyword_5_2_2_0() { return cCommaKeyword_5_2_2_0; }
//exportList+=ValidID
public Assignment getExportListAssignment_5_2_2_1() { return cExportListAssignment_5_2_2_1; }
//ValidID
public RuleCall getExportListValidIDParserRuleCall_5_2_2_1_0() { return cExportListValidIDParserRuleCall_5_2_2_1_0; }
//('in' 'domain' (rootDomain?='root' | domainConcept=ValidID))?
public Group getGroup_5_3() { return cGroup_5_3; }
//'in'
public Keyword getInKeyword_5_3_0() { return cInKeyword_5_3_0; }
//'domain'
public Keyword getDomainKeyword_5_3_1() { return cDomainKeyword_5_3_1; }
//(rootDomain?='root' | domainConcept=ValidID)
public Alternatives getAlternatives_5_3_2() { return cAlternatives_5_3_2; }
//rootDomain?='root'
public Assignment getRootDomainAssignment_5_3_2_0() { return cRootDomainAssignment_5_3_2_0; }
//'root'
public Keyword getRootDomainRootKeyword_5_3_2_0_0() { return cRootDomainRootKeyword_5_3_2_0_0; }
//domainConcept=ValidID
public Assignment getDomainConceptAssignment_5_3_2_1() { return cDomainConceptAssignment_5_3_2_1; }
//ValidID
public RuleCall getDomainConceptValidIDParserRuleCall_5_3_2_1_0() { return cDomainConceptValidIDParserRuleCall_5_3_2_1_0; }
//('disjoint' 'with' disjointNamespaces=QualifiedNameList)?
public Group getGroup_5_4() { return cGroup_5_4; }
//'disjoint'
public Keyword getDisjointKeyword_5_4_0() { return cDisjointKeyword_5_4_0; }
//'with'
public Keyword getWithKeyword_5_4_1() { return cWithKeyword_5_4_1; }
//disjointNamespaces=QualifiedNameList
public Assignment getDisjointNamespacesAssignment_5_4_2() { return cDisjointNamespacesAssignment_5_4_2; }
//QualifiedNameList
public RuleCall getDisjointNamespacesQualifiedNameListParserRuleCall_5_4_2_0() { return cDisjointNamespacesQualifiedNameListParserRuleCall_5_4_2_0; }
//('version' version=ValidID)?
public Group getGroup_5_5() { return cGroup_5_5; }
//'version'
public Keyword getVersionKeyword_5_5_0() { return cVersionKeyword_5_5_0; }
//version=ValidID
public Assignment getVersionAssignment_5_5_1() { return cVersionAssignment_5_5_1; }
//ValidID
public RuleCall getVersionValidIDParserRuleCall_5_5_1_0() { return cVersionValidIDParserRuleCall_5_5_1_0; }
//('resolve' ('from' lookupNamespace+=ValidID*)? ('outside' blacklistNamespace+=ValidID*)? ('using' weights=Metadata)?)?
public Group getGroup_5_6() { return cGroup_5_6; }
//'resolve'
public Keyword getResolveKeyword_5_6_0() { return cResolveKeyword_5_6_0; }
//('from' lookupNamespace+=ValidID*)?
public Group getGroup_5_6_1() { return cGroup_5_6_1; }
//'from'
public Keyword getFromKeyword_5_6_1_0() { return cFromKeyword_5_6_1_0; }
//lookupNamespace+=ValidID*
public Assignment getLookupNamespaceAssignment_5_6_1_1() { return cLookupNamespaceAssignment_5_6_1_1; }
//ValidID
public RuleCall getLookupNamespaceValidIDParserRuleCall_5_6_1_1_0() { return cLookupNamespaceValidIDParserRuleCall_5_6_1_1_0; }
//('outside' blacklistNamespace+=ValidID*)?
public Group getGroup_5_6_2() { return cGroup_5_6_2; }
//'outside'
public Keyword getOutsideKeyword_5_6_2_0() { return cOutsideKeyword_5_6_2_0; }
//blacklistNamespace+=ValidID*
public Assignment getBlacklistNamespaceAssignment_5_6_2_1() { return cBlacklistNamespaceAssignment_5_6_2_1; }
//ValidID
public RuleCall getBlacklistNamespaceValidIDParserRuleCall_5_6_2_1_0() { return cBlacklistNamespaceValidIDParserRuleCall_5_6_2_1_0; }
//('using' weights=Metadata)?
public Group getGroup_5_6_3() { return cGroup_5_6_3; }
//'using'
public Keyword getUsingKeyword_5_6_3_0() { return cUsingKeyword_5_6_3_0; }
//weights=Metadata
public Assignment getWeightsAssignment_5_6_3_1() { return cWeightsAssignment_5_6_3_1; }
//Metadata
public RuleCall getWeightsMetadataParserRuleCall_5_6_3_1_0() { return cWeightsMetadataParserRuleCall_5_6_3_1_0; }
//('train' 'in' trainingNamespace+=ValidID*)?
public Group getGroup_5_7() { return cGroup_5_7; }
//'train'
public Keyword getTrainKeyword_5_7_0() { return cTrainKeyword_5_7_0; }
//'in'
public Keyword getInKeyword_5_7_1() { return cInKeyword_5_7_1; }
//trainingNamespace+=ValidID*
public Assignment getTrainingNamespaceAssignment_5_7_2() { return cTrainingNamespaceAssignment_5_7_2; }
//ValidID
public RuleCall getTrainingNamespaceValidIDParserRuleCall_5_7_2_0() { return cTrainingNamespaceValidIDParserRuleCall_5_7_2_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_6() { return cGroup_6; }
//'with'
public Keyword getWithKeyword_6_0() { return cWithKeyword_6_0; }
//'metadata'
public Keyword getMetadataKeyword_6_1() { return cMetadataKeyword_6_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_6_2() { return cMetadataAssignment_6_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_6_2_0() { return cMetadataMetadataParserRuleCall_6_2_0; }
//';'
public Keyword getSemicolonKeyword_7() { return cSemicolonKeyword_7; }
}
public class CoverageListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.CoverageList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cCoverageAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cCoverageCoverageParserRuleCall_0_0 = (RuleCall)cCoverageAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cCoverageAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cCoverageCoverageParserRuleCall_1_1_0 = (RuleCall)cCoverageAssignment_1_1.eContents().get(0);
//CoverageList:
// coverage+=Coverage (=> ',' coverage+=Coverage)*;
@Override public ParserRule getRule() { return rule; }
//coverage+=Coverage (=> ',' coverage+=Coverage)*
public Group getGroup() { return cGroup; }
//coverage+=Coverage
public Assignment getCoverageAssignment_0() { return cCoverageAssignment_0; }
//Coverage
public RuleCall getCoverageCoverageParserRuleCall_0_0() { return cCoverageCoverageParserRuleCall_0_0; }
//(=> ',' coverage+=Coverage)*
public Group getGroup_1() { return cGroup_1; }
//=> ','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//coverage+=Coverage
public Assignment getCoverageAssignment_1_1() { return cCoverageAssignment_1_1; }
//Coverage
public RuleCall getCoverageCoverageParserRuleCall_1_1_0() { return cCoverageCoverageParserRuleCall_1_1_0; }
}
public class CoverageElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Coverage");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cIdAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cIdValidIDParserRuleCall_0_0 = (RuleCall)cIdAssignment_0.eContents().get(0);
private final Assignment cFunctionAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cFunctionFunctionParserRuleCall_1_0 = (RuleCall)cFunctionAssignment_1.eContents().get(0);
//Coverage:
// id=ValidID | function=Function;
@Override public ParserRule getRule() { return rule; }
//id=ValidID | function=Function
public Alternatives getAlternatives() { return cAlternatives; }
//id=ValidID
public Assignment getIdAssignment_0() { return cIdAssignment_0; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_0_0() { return cIdValidIDParserRuleCall_0_0; }
//function=Function
public Assignment getFunctionAssignment_1() { return cFunctionAssignment_1; }
//Function
public RuleCall getFunctionFunctionParserRuleCall_1_0() { return cFunctionFunctionParserRuleCall_1_0; }
}
public class ImportListElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ImportList");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cImportsAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cImportsImportParserRuleCall_0_0 = (RuleCall)cImportsAssignment_0.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cCommaKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cImportsAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cImportsImportParserRuleCall_1_1_0 = (RuleCall)cImportsAssignment_1_1.eContents().get(0);
//ImportList:
// imports+=Import (=> ',' imports+=Import)*;
@Override public ParserRule getRule() { return rule; }
//imports+=Import (=> ',' imports+=Import)*
public Group getGroup() { return cGroup; }
//imports+=Import
public Assignment getImportsAssignment_0() { return cImportsAssignment_0; }
//Import
public RuleCall getImportsImportParserRuleCall_0_0() { return cImportsImportParserRuleCall_0_0; }
//(=> ',' imports+=Import)*
public Group getGroup_1() { return cGroup_1; }
//=> ','
public Keyword getCommaKeyword_1_0() { return cCommaKeyword_1_0; }
//imports+=Import
public Assignment getImportsAssignment_1_1() { return cImportsAssignment_1_1; }
//Import
public RuleCall getImportsImportParserRuleCall_1_1_0() { return cImportsImportParserRuleCall_1_1_0; }
}
public class ImportElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Import");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cGroup.eContents().get(0);
private final Alternatives cAlternatives_0_0 = (Alternatives)cGroup_0.eContents().get(0);
private final Assignment cImportsAssignment_0_0_0 = (Assignment)cAlternatives_0_0.eContents().get(0);
private final RuleCall cImportsListParserRuleCall_0_0_0_0 = (RuleCall)cImportsAssignment_0_0_0.eContents().get(0);
private final Assignment cStarAssignment_0_0_1 = (Assignment)cAlternatives_0_0.eContents().get(1);
private final Keyword cStarAsteriskKeyword_0_0_1_0 = (Keyword)cStarAssignment_0_0_1.eContents().get(0);
private final Keyword cFromKeyword_0_1 = (Keyword)cGroup_0.eContents().get(1);
private final Assignment cImportedAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cImportedValidIDParserRuleCall_1_0 = (RuleCall)cImportedAssignment_1.eContents().get(0);
//Import:
// ((imports=List | star?='*') 'from')? imported=ValidID;
@Override public ParserRule getRule() { return rule; }
//((imports=List | star?='*') 'from')? imported=ValidID
public Group getGroup() { return cGroup; }
//((imports=List | star?='*') 'from')?
public Group getGroup_0() { return cGroup_0; }
//(imports=List | star?='*')
public Alternatives getAlternatives_0_0() { return cAlternatives_0_0; }
//imports=List
public Assignment getImportsAssignment_0_0_0() { return cImportsAssignment_0_0_0; }
//List
public RuleCall getImportsListParserRuleCall_0_0_0_0() { return cImportsListParserRuleCall_0_0_0_0; }
//star?='*'
public Assignment getStarAssignment_0_0_1() { return cStarAssignment_0_0_1; }
//'*'
public Keyword getStarAsteriskKeyword_0_0_1_0() { return cStarAsteriskKeyword_0_0_1_0; }
//'from'
public Keyword getFromKeyword_0_1() { return cFromKeyword_0_1; }
//imported=ValidID
public Assignment getImportedAssignment_1() { return cImportedAssignment_1; }
//ValidID
public RuleCall getImportedValidIDParserRuleCall_1_0() { return cImportedValidIDParserRuleCall_1_0; }
}
public class ModelObservableAdditionalElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ModelObservableAdditional");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cDeclarationAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final RuleCall cDeclarationConceptDeclarationParserRuleCall_0_0_0 = (RuleCall)cDeclarationAssignment_0_0.eContents().get(0);
private final Assignment cOptionalAssignment_0_1 = (Assignment)cGroup_0.eContents().get(1);
private final Keyword cOptionalOptionalKeyword_0_1_0 = (Keyword)cOptionalAssignment_0_1.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Assignment cInlineModelAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final RuleCall cInlineModelInlineModelParserRuleCall_1_0_0 = (RuleCall)cInlineModelAssignment_1_0.eContents().get(0);
private final Assignment cOptionalAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final Keyword cOptionalOptionalKeyword_1_1_0 = (Keyword)cOptionalAssignment_1_1.eContents().get(0);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Keyword cNamedKeyword_1_2_0 = (Keyword)cGroup_1_2.eContents().get(0);
private final Assignment cObservableNameAssignment_1_2_1 = (Assignment)cGroup_1_2.eContents().get(1);
private final RuleCall cObservableNameValidIDParserRuleCall_1_2_1_0 = (RuleCall)cObservableNameAssignment_1_2_1.eContents().get(0);
//ModelObservableAdditional ModelObservable:
// declaration=ConceptDeclaration optional?='optional'? / * ) * / | inlineModel=InlineModel optional?='optional'? ('named'
// observableName=ValidID)
@Override public ParserRule getRule() { return rule; }
/// * concept ID. The 'using' clause can only be used from the second observable on (the first 'uses' the model itself) * /
//declaration=ConceptDeclaration optional?='optional'? / * ) * / | inlineModel=InlineModel optional?='optional'? ('named'
//observableName=ValidID)
public Alternatives getAlternatives() { return cAlternatives; }
/// * concept ID. The 'using' clause can only be used from the second observable on (the first 'uses' the model itself) * /
//declaration=ConceptDeclaration optional?='optional'?
public Group getGroup_0() { return cGroup_0; }
/// * concept ID. The 'using' clause can only be used from the second observable on (the first 'uses' the model itself) * /
//declaration=ConceptDeclaration
public Assignment getDeclarationAssignment_0_0() { return cDeclarationAssignment_0_0; }
//ConceptDeclaration
public RuleCall getDeclarationConceptDeclarationParserRuleCall_0_0_0() { return cDeclarationConceptDeclarationParserRuleCall_0_0_0; }
//optional?='optional'?
public Assignment getOptionalAssignment_0_1() { return cOptionalAssignment_0_1; }
//'optional'
public Keyword getOptionalOptionalKeyword_0_1_0() { return cOptionalOptionalKeyword_0_1_0; }
/// * inline (simple) model - in parentheses without 'model' keyword, named for output name, only from the second observable on. * /
//inlineModel=InlineModel optional?='optional'? ('named' observableName=ValidID)
public Group getGroup_1() { return cGroup_1; }
/// * inline (simple) model - in parentheses without 'model' keyword, named for output name, only from the second observable on. * /
//inlineModel=InlineModel
public Assignment getInlineModelAssignment_1_0() { return cInlineModelAssignment_1_0; }
//InlineModel
public RuleCall getInlineModelInlineModelParserRuleCall_1_0_0() { return cInlineModelInlineModelParserRuleCall_1_0_0; }
//optional?='optional'?
public Assignment getOptionalAssignment_1_1() { return cOptionalAssignment_1_1; }
//'optional'
public Keyword getOptionalOptionalKeyword_1_1_0() { return cOptionalOptionalKeyword_1_1_0; }
//('named' observableName=ValidID)
public Group getGroup_1_2() { return cGroup_1_2; }
//'named'
public Keyword getNamedKeyword_1_2_0() { return cNamedKeyword_1_2_0; }
//observableName=ValidID
public Assignment getObservableNameAssignment_1_2_1() { return cObservableNameAssignment_1_2_1; }
//ValidID
public RuleCall getObservableNameValidIDParserRuleCall_1_2_1_0() { return cObservableNameValidIDParserRuleCall_1_2_1_0; }
}
public class ModelObservableElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ModelObservable");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cFunctionAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cFunctionFunctionParserRuleCall_0_0 = (RuleCall)cFunctionAssignment_0.eContents().get(0);
private final Assignment cDeclarationAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cDeclarationConceptDeclarationParserRuleCall_1_0 = (RuleCall)cDeclarationAssignment_1.eContents().get(0);
private final Assignment cLiteralAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final RuleCall cLiteralLiteralParserRuleCall_2_0 = (RuleCall)cLiteralAssignment_2.eContents().get(0);
private final Group cGroup_3 = (Group)cAlternatives.eContents().get(3);
private final Keyword cLeftParenthesisKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cConceptStatementAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cConceptStatementConceptStatementParserRuleCall_3_1_0 = (RuleCall)cConceptStatementAssignment_3_1.eContents().get(0);
private final Keyword cRightParenthesisKeyword_3_2 = (Keyword)cGroup_3.eContents().get(2);
//ModelObservable:
// function=Function | declaration=ConceptDeclaration | literal=Literal | / * inlined concept statement * / '('
// conceptStatement=ConceptStatement ')';
@Override public ParserRule getRule() { return rule; }
/// * datasource or function generating an observer * / function=Function | declaration=ConceptDeclaration | literal=Literal
//| / * inlined concept statement * / '(' conceptStatement=ConceptStatement ')'
public Alternatives getAlternatives() { return cAlternatives; }
/// * datasource or function generating an observer * / function=Function
public Assignment getFunctionAssignment_0() { return cFunctionAssignment_0; }
//Function
public RuleCall getFunctionFunctionParserRuleCall_0_0() { return cFunctionFunctionParserRuleCall_0_0; }
/// * concept ID. The 'using' clause can only be used from the second observable on (the first 'uses' the model itself) * /
//declaration=ConceptDeclaration
public Assignment getDeclarationAssignment_1() { return cDeclarationAssignment_1; }
//ConceptDeclaration
public RuleCall getDeclarationConceptDeclarationParserRuleCall_1_0() { return cDeclarationConceptDeclarationParserRuleCall_1_0; }
/// * annotate an inline number or concept as a localized observation of something. * / literal=Literal
public Assignment getLiteralAssignment_2() { return cLiteralAssignment_2; }
//Literal
public RuleCall getLiteralLiteralParserRuleCall_2_0() { return cLiteralLiteralParserRuleCall_2_0; }
/// * inlined concept statement * / '(' conceptStatement=ConceptStatement ')'
public Group getGroup_3() { return cGroup_3; }
/// * inlined concept statement * / '('
public Keyword getLeftParenthesisKeyword_3_0() { return cLeftParenthesisKeyword_3_0; }
//conceptStatement=ConceptStatement
public Assignment getConceptStatementAssignment_3_1() { return cConceptStatementAssignment_3_1; }
//ConceptStatement
public RuleCall getConceptStatementConceptStatementParserRuleCall_3_1_0() { return cConceptStatementConceptStatementParserRuleCall_3_1_0; }
//')'
public Keyword getRightParenthesisKeyword_3_2() { return cRightParenthesisKeyword_3_2; }
}
public class StatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Statement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cAnnotationsAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cAnnotationsAnnotationParserRuleCall_0_0 = (RuleCall)cAnnotationsAssignment_0.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Group cGroup_1_0 = (Group)cAlternatives_1.eContents().get(0);
private final Assignment cConceptAssignment_1_0_0 = (Assignment)cGroup_1_0.eContents().get(0);
private final RuleCall cConceptConceptStatementParserRuleCall_1_0_0_0 = (RuleCall)cConceptAssignment_1_0_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_0_1 = (Keyword)cGroup_1_0.eContents().get(1);
private final Group cGroup_1_1 = (Group)cAlternatives_1.eContents().get(1);
private final Assignment cConceptAssignment_1_1_0 = (Assignment)cGroup_1_1.eContents().get(0);
private final RuleCall cConceptContextualRedeclarationParserRuleCall_1_1_0_0 = (RuleCall)cConceptAssignment_1_1_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_1_1 = (Keyword)cGroup_1_1.eContents().get(1);
private final Group cGroup_1_2 = (Group)cAlternatives_1.eContents().get(2);
private final Assignment cContextualizationAssignment_1_2_0 = (Assignment)cGroup_1_2.eContents().get(0);
private final RuleCall cContextualizationContextualizeStatementParserRuleCall_1_2_0_0 = (RuleCall)cContextualizationAssignment_1_2_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_2_1 = (Keyword)cGroup_1_2.eContents().get(1);
private final Group cGroup_1_3 = (Group)cAlternatives_1.eContents().get(3);
private final Assignment cPropertyAssignment_1_3_0 = (Assignment)cGroup_1_3.eContents().get(0);
private final RuleCall cPropertyPropertyStatementParserRuleCall_1_3_0_0 = (RuleCall)cPropertyAssignment_1_3_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_3_1 = (Keyword)cGroup_1_3.eContents().get(1);
private final Group cGroup_1_4 = (Group)cAlternatives_1.eContents().get(4);
private final Assignment cModelAssignment_1_4_0 = (Assignment)cGroup_1_4.eContents().get(0);
private final RuleCall cModelModelStatementParserRuleCall_1_4_0_0 = (RuleCall)cModelAssignment_1_4_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_4_1 = (Keyword)cGroup_1_4.eContents().get(1);
private final Group cGroup_1_5 = (Group)cAlternatives_1.eContents().get(5);
private final Assignment cObserveAssignment_1_5_0 = (Assignment)cGroup_1_5.eContents().get(0);
private final RuleCall cObserveObserveStatementParserRuleCall_1_5_0_0 = (RuleCall)cObserveAssignment_1_5_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_5_1 = (Keyword)cGroup_1_5.eContents().get(1);
private final Group cGroup_1_6 = (Group)cAlternatives_1.eContents().get(6);
private final Assignment cDefineAssignment_1_6_0 = (Assignment)cGroup_1_6.eContents().get(0);
private final RuleCall cDefineDefineStatementParserRuleCall_1_6_0_0 = (RuleCall)cDefineAssignment_1_6_0.eContents().get(0);
private final Keyword cSemicolonKeyword_1_6_1 = (Keyword)cGroup_1_6.eContents().get(1);
/// **
// * TODO enable annotations to go with the namespace (those would be the ones before the
// * namespace statement or at the end when there is no object to attach them to). This
// * can be done by making an annotation a statement.
// * /
//Statement:
// annotations+=Annotation* (concept=ConceptStatement ';' | concept=ContextualRedeclaration ';' |
// contextualization=ContextualizeStatement ';' | property=PropertyStatement ';' | model=ModelStatement ';' |
// observe=ObserveStatement ';' | define=DefineStatement ';');
@Override public ParserRule getRule() { return rule; }
//annotations+=Annotation* (concept=ConceptStatement ';' | concept=ContextualRedeclaration ';' |
//contextualization=ContextualizeStatement ';' | property=PropertyStatement ';' | model=ModelStatement ';' |
//observe=ObserveStatement ';' | define=DefineStatement ';')
public Group getGroup() { return cGroup; }
//annotations+=Annotation*
public Assignment getAnnotationsAssignment_0() { return cAnnotationsAssignment_0; }
//Annotation
public RuleCall getAnnotationsAnnotationParserRuleCall_0_0() { return cAnnotationsAnnotationParserRuleCall_0_0; }
//(concept=ConceptStatement ';' | concept=ContextualRedeclaration ';' | contextualization=ContextualizeStatement ';' |
//property=PropertyStatement ';' | model=ModelStatement ';' | observe=ObserveStatement ';' | define=DefineStatement ';')
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//concept=ConceptStatement ';'
public Group getGroup_1_0() { return cGroup_1_0; }
//concept=ConceptStatement
public Assignment getConceptAssignment_1_0_0() { return cConceptAssignment_1_0_0; }
//ConceptStatement
public RuleCall getConceptConceptStatementParserRuleCall_1_0_0_0() { return cConceptConceptStatementParserRuleCall_1_0_0_0; }
//';'
public Keyword getSemicolonKeyword_1_0_1() { return cSemicolonKeyword_1_0_1; }
//concept=ContextualRedeclaration ';'
public Group getGroup_1_1() { return cGroup_1_1; }
//concept=ContextualRedeclaration
public Assignment getConceptAssignment_1_1_0() { return cConceptAssignment_1_1_0; }
//ContextualRedeclaration
public RuleCall getConceptContextualRedeclarationParserRuleCall_1_1_0_0() { return cConceptContextualRedeclarationParserRuleCall_1_1_0_0; }
//';'
public Keyword getSemicolonKeyword_1_1_1() { return cSemicolonKeyword_1_1_1; }
//contextualization=ContextualizeStatement ';'
public Group getGroup_1_2() { return cGroup_1_2; }
//contextualization=ContextualizeStatement
public Assignment getContextualizationAssignment_1_2_0() { return cContextualizationAssignment_1_2_0; }
//ContextualizeStatement
public RuleCall getContextualizationContextualizeStatementParserRuleCall_1_2_0_0() { return cContextualizationContextualizeStatementParserRuleCall_1_2_0_0; }
//';'
public Keyword getSemicolonKeyword_1_2_1() { return cSemicolonKeyword_1_2_1; }
//property=PropertyStatement ';'
public Group getGroup_1_3() { return cGroup_1_3; }
//property=PropertyStatement
public Assignment getPropertyAssignment_1_3_0() { return cPropertyAssignment_1_3_0; }
//PropertyStatement
public RuleCall getPropertyPropertyStatementParserRuleCall_1_3_0_0() { return cPropertyPropertyStatementParserRuleCall_1_3_0_0; }
//';'
public Keyword getSemicolonKeyword_1_3_1() { return cSemicolonKeyword_1_3_1; }
//model=ModelStatement ';'
public Group getGroup_1_4() { return cGroup_1_4; }
//model=ModelStatement
public Assignment getModelAssignment_1_4_0() { return cModelAssignment_1_4_0; }
//ModelStatement
public RuleCall getModelModelStatementParserRuleCall_1_4_0_0() { return cModelModelStatementParserRuleCall_1_4_0_0; }
//';'
public Keyword getSemicolonKeyword_1_4_1() { return cSemicolonKeyword_1_4_1; }
//observe=ObserveStatement ';'
public Group getGroup_1_5() { return cGroup_1_5; }
//observe=ObserveStatement
public Assignment getObserveAssignment_1_5_0() { return cObserveAssignment_1_5_0; }
//ObserveStatement
public RuleCall getObserveObserveStatementParserRuleCall_1_5_0_0() { return cObserveObserveStatementParserRuleCall_1_5_0_0; }
//';'
public Keyword getSemicolonKeyword_1_5_1() { return cSemicolonKeyword_1_5_1; }
//define=DefineStatement ';'
public Group getGroup_1_6() { return cGroup_1_6; }
//define=DefineStatement
public Assignment getDefineAssignment_1_6_0() { return cDefineAssignment_1_6_0; }
//DefineStatement
public RuleCall getDefineDefineStatementParserRuleCall_1_6_0_0() { return cDefineDefineStatementParserRuleCall_1_6_0_0; }
//';'
public Keyword getSemicolonKeyword_1_6_1() { return cSemicolonKeyword_1_6_1; }
}
public class ModelStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ModelStatement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_0_0 = (UnorderedGroup)cGroup_0.eContents().get(0);
private final Assignment cPrivateAssignment_0_0_0 = (Assignment)cUnorderedGroup_0_0.eContents().get(0);
private final Keyword cPrivatePrivateKeyword_0_0_0_0 = (Keyword)cPrivateAssignment_0_0_0.eContents().get(0);
private final Assignment cInactiveAssignment_0_0_1 = (Assignment)cUnorderedGroup_0_0.eContents().get(1);
private final Keyword cInactiveVoidKeyword_0_0_1_0 = (Keyword)cInactiveAssignment_0_0_1.eContents().get(0);
private final Keyword cModelKeyword_0_1 = (Keyword)cGroup_0.eContents().get(1);
private final Group cGroup_0_2 = (Group)cGroup_0.eContents().get(2);
private final Assignment cObservablesAssignment_0_2_0 = (Assignment)cGroup_0_2.eContents().get(0);
private final RuleCall cObservablesModelObservableParserRuleCall_0_2_0_0 = (RuleCall)cObservablesAssignment_0_2_0.eContents().get(0);
private final Group cGroup_0_2_1 = (Group)cGroup_0_2.eContents().get(1);
private final Keyword cNamedKeyword_0_2_1_0 = (Keyword)cGroup_0_2_1.eContents().get(0);
private final Assignment cNameAssignment_0_2_1_1 = (Assignment)cGroup_0_2_1.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_0_2_1_1_0 = (RuleCall)cNameAssignment_0_2_1_1.eContents().get(0);
private final Group cGroup_0_2_2 = (Group)cGroup_0_2.eContents().get(2);
private final Keyword cCommaKeyword_0_2_2_0 = (Keyword)cGroup_0_2_2.eContents().get(0);
private final Assignment cObservablesAssignment_0_2_2_1 = (Assignment)cGroup_0_2_2.eContents().get(1);
private final RuleCall cObservablesModelObservableAdditionalParserRuleCall_0_2_2_1_0 = (RuleCall)cObservablesAssignment_0_2_2_1.eContents().get(0);
private final Group cGroup_0_3 = (Group)cGroup_0.eContents().get(3);
private final Keyword cObservingKeyword_0_3_0 = (Keyword)cGroup_0_3.eContents().get(0);
private final Assignment cDependenciesAssignment_0_3_1 = (Assignment)cGroup_0_3.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_0_3_1_0 = (RuleCall)cDependenciesAssignment_0_3_1.eContents().get(0);
private final Group cGroup_0_3_2 = (Group)cGroup_0_3.eContents().get(2);
private final Keyword cCommaKeyword_0_3_2_0 = (Keyword)cGroup_0_3_2.eContents().get(0);
private final Assignment cDependenciesAssignment_0_3_2_1 = (Assignment)cGroup_0_3_2.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_0_3_2_1_0 = (RuleCall)cDependenciesAssignment_0_3_2_1.eContents().get(0);
private final Alternatives cAlternatives_0_4 = (Alternatives)cGroup_0.eContents().get(4);
private final Group cGroup_0_4_0 = (Group)cAlternatives_0_4.eContents().get(0);
private final Keyword cAsKeyword_0_4_0_0 = (Keyword)cGroup_0_4_0.eContents().get(0);
private final Assignment cObserversAssignment_0_4_0_1 = (Assignment)cGroup_0_4_0.eContents().get(1);
private final RuleCall cObserversObservationGeneratorSwitchParserRuleCall_0_4_0_1_0 = (RuleCall)cObserversAssignment_0_4_0_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_0_4_1 = (UnorderedGroup)cAlternatives_0_4.eContents().get(1);
private final Group cGroup_0_4_1_0 = (Group)cUnorderedGroup_0_4_1.eContents().get(0);
private final Keyword cUsingKeyword_0_4_1_0_0 = (Keyword)cGroup_0_4_1_0.eContents().get(0);
private final Alternatives cAlternatives_0_4_1_0_1 = (Alternatives)cGroup_0_4_1_0.eContents().get(1);
private final Assignment cAccessorAssignment_0_4_1_0_1_0 = (Assignment)cAlternatives_0_4_1_0_1.eContents().get(0);
private final RuleCall cAccessorFunctionParserRuleCall_0_4_1_0_1_0_0 = (RuleCall)cAccessorAssignment_0_4_1_0_1_0.eContents().get(0);
private final Assignment cLookupAssignment_0_4_1_0_1_1 = (Assignment)cAlternatives_0_4_1_0_1.eContents().get(1);
private final RuleCall cLookupLookupFunctionParserRuleCall_0_4_1_0_1_1_0 = (RuleCall)cLookupAssignment_0_4_1_0_1_1.eContents().get(0);
private final Group cGroup_0_4_1_1 = (Group)cUnorderedGroup_0_4_1.eContents().get(1);
private final Assignment cContextualizersAssignment_0_4_1_1_0 = (Assignment)cGroup_0_4_1_1.eContents().get(0);
private final RuleCall cContextualizersContextualizationParserRuleCall_0_4_1_1_0_0 = (RuleCall)cContextualizersAssignment_0_4_1_1_0.eContents().get(0);
private final Assignment cContextualizersAssignment_0_4_1_1_1 = (Assignment)cGroup_0_4_1_1.eContents().get(1);
private final RuleCall cContextualizersContextualizationParserRuleCall_0_4_1_1_1_0 = (RuleCall)cContextualizersAssignment_0_4_1_1_1.eContents().get(0);
private final Group cGroup_0_5 = (Group)cGroup_0.eContents().get(5);
private final Keyword cWithKeyword_0_5_0 = (Keyword)cGroup_0_5.eContents().get(0);
private final Keyword cMetadataKeyword_0_5_1 = (Keyword)cGroup_0_5.eContents().get(1);
private final Assignment cMetadataAssignment_0_5_2 = (Assignment)cGroup_0_5.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_0_5_2_0 = (RuleCall)cMetadataAssignment_0_5_2.eContents().get(0);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final UnorderedGroup cUnorderedGroup_1_0 = (UnorderedGroup)cGroup_1.eContents().get(0);
private final Assignment cPrivateAssignment_1_0_0 = (Assignment)cUnorderedGroup_1_0.eContents().get(0);
private final Keyword cPrivatePrivateKeyword_1_0_0_0 = (Keyword)cPrivateAssignment_1_0_0.eContents().get(0);
private final Assignment cInactiveAssignment_1_0_1 = (Assignment)cUnorderedGroup_1_0.eContents().get(1);
private final Keyword cInactiveVoidKeyword_1_0_1_0 = (Keyword)cInactiveAssignment_1_0_1.eContents().get(0);
private final Assignment cInterpreterAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final Keyword cInterpreterModelKeyword_1_1_0 = (Keyword)cInterpreterAssignment_1_1.eContents().get(0);
private final Group cGroup_1_2 = (Group)cGroup_1.eContents().get(2);
private final Keyword cNamedKeyword_1_2_0 = (Keyword)cGroup_1_2.eContents().get(0);
private final Assignment cNameAssignment_1_2_1 = (Assignment)cGroup_1_2.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_1_2_1_0 = (RuleCall)cNameAssignment_1_2_1.eContents().get(0);
private final Assignment cObserversAssignment_1_3 = (Assignment)cGroup_1.eContents().get(3);
private final RuleCall cObserversObservationGeneratorSwitchParserRuleCall_1_3_0 = (RuleCall)cObserversAssignment_1_3.eContents().get(0);
private final Group cGroup_1_4 = (Group)cGroup_1.eContents().get(4);
private final Keyword cNamedKeyword_1_4_0 = (Keyword)cGroup_1_4.eContents().get(0);
private final Assignment cName2Assignment_1_4_1 = (Assignment)cGroup_1_4.eContents().get(1);
private final RuleCall cName2ValidIDParserRuleCall_1_4_1_0 = (RuleCall)cName2Assignment_1_4_1.eContents().get(0);
private final Group cGroup_1_5 = (Group)cGroup_1.eContents().get(5);
private final Keyword cWithKeyword_1_5_0 = (Keyword)cGroup_1_5.eContents().get(0);
private final Keyword cMetadataKeyword_1_5_1 = (Keyword)cGroup_1_5.eContents().get(1);
private final Assignment cMetadataAssignment_1_5_2 = (Assignment)cGroup_1_5.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_1_5_2_0 = (RuleCall)cMetadataAssignment_1_5_2.eContents().get(0);
private final Group cGroup_2 = (Group)cAlternatives.eContents().get(2);
private final UnorderedGroup cUnorderedGroup_2_0 = (UnorderedGroup)cGroup_2.eContents().get(0);
private final Assignment cPrivateAssignment_2_0_0 = (Assignment)cUnorderedGroup_2_0.eContents().get(0);
private final Keyword cPrivatePrivateKeyword_2_0_0_0 = (Keyword)cPrivateAssignment_2_0_0.eContents().get(0);
private final Assignment cInactiveAssignment_2_0_1 = (Assignment)cUnorderedGroup_2_0.eContents().get(1);
private final Keyword cInactiveVoidKeyword_2_0_1_0 = (Keyword)cInactiveAssignment_2_0_1.eContents().get(0);
private final Keyword cModelKeyword_2_1 = (Keyword)cGroup_2.eContents().get(1);
private final Assignment cReificationAssignment_2_2 = (Assignment)cGroup_2.eContents().get(2);
private final Keyword cReificationEachKeyword_2_2_0 = (Keyword)cReificationAssignment_2_2.eContents().get(0);
private final Alternatives cAlternatives_2_3 = (Alternatives)cGroup_2.eContents().get(3);
private final Group cGroup_2_3_0 = (Group)cAlternatives_2_3.eContents().get(0);
private final Assignment cAgentSourceAssignment_2_3_0_0 = (Assignment)cGroup_2_3_0.eContents().get(0);
private final RuleCall cAgentSourceFunctionParserRuleCall_2_3_0_0_0 = (RuleCall)cAgentSourceAssignment_2_3_0_0.eContents().get(0);
private final Group cGroup_2_3_0_1 = (Group)cGroup_2_3_0.eContents().get(1);
private final Keyword cNamedKeyword_2_3_0_1_0 = (Keyword)cGroup_2_3_0_1.eContents().get(0);
private final Assignment cNameAssignment_2_3_0_1_1 = (Assignment)cGroup_2_3_0_1.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_2_3_0_1_1_0 = (RuleCall)cNameAssignment_2_3_0_1_1.eContents().get(0);
private final Keyword cAsKeyword_2_3_0_2 = (Keyword)cGroup_2_3_0.eContents().get(2);
private final Assignment cObservableAssignment_2_3_0_3 = (Assignment)cGroup_2_3_0.eContents().get(3);
private final RuleCall cObservableConceptDeclarationParserRuleCall_2_3_0_3_0 = (RuleCall)cObservableAssignment_2_3_0_3.eContents().get(0);
private final Group cGroup_2_3_1 = (Group)cAlternatives_2_3.eContents().get(1);
private final Assignment cObservableAssignment_2_3_1_0 = (Assignment)cGroup_2_3_1.eContents().get(0);
private final RuleCall cObservableConceptDeclarationParserRuleCall_2_3_1_0_0 = (RuleCall)cObservableAssignment_2_3_1_0.eContents().get(0);
private final Group cGroup_2_3_1_1 = (Group)cGroup_2_3_1.eContents().get(1);
private final Keyword cNamedKeyword_2_3_1_1_0 = (Keyword)cGroup_2_3_1_1.eContents().get(0);
private final Assignment cNameAssignment_2_3_1_1_1 = (Assignment)cGroup_2_3_1_1.eContents().get(1);
private final RuleCall cNameValidIDParserRuleCall_2_3_1_1_1_0 = (RuleCall)cNameAssignment_2_3_1_1_1.eContents().get(0);
private final Group cGroup_2_4 = (Group)cGroup_2.eContents().get(4);
private final Keyword cObservingKeyword_2_4_0 = (Keyword)cGroup_2_4.eContents().get(0);
private final Assignment cDependenciesAssignment_2_4_1 = (Assignment)cGroup_2_4.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_2_4_1_0 = (RuleCall)cDependenciesAssignment_2_4_1.eContents().get(0);
private final Group cGroup_2_4_2 = (Group)cGroup_2_4.eContents().get(2);
private final Keyword cCommaKeyword_2_4_2_0 = (Keyword)cGroup_2_4_2.eContents().get(0);
private final Assignment cDependenciesAssignment_2_4_2_1 = (Assignment)cGroup_2_4_2.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_2_4_2_1_0 = (RuleCall)cDependenciesAssignment_2_4_2_1.eContents().get(0);
private final Group cGroup_2_5 = (Group)cGroup_2.eContents().get(5);
private final Keyword cInterpretKeyword_2_5_0 = (Keyword)cGroup_2_5.eContents().get(0);
private final Assignment cAttributeTranslatorsAssignment_2_5_1 = (Assignment)cGroup_2_5.eContents().get(1);
private final RuleCall cAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_1_0 = (RuleCall)cAttributeTranslatorsAssignment_2_5_1.eContents().get(0);
private final Group cGroup_2_5_2 = (Group)cGroup_2_5.eContents().get(2);
private final Keyword cCommaKeyword_2_5_2_0 = (Keyword)cGroup_2_5_2.eContents().get(0);
private final Assignment cAttributeTranslatorsAssignment_2_5_2_1 = (Assignment)cGroup_2_5_2.eContents().get(1);
private final RuleCall cAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_2_1_0 = (RuleCall)cAttributeTranslatorsAssignment_2_5_2_1.eContents().get(0);
private final Group cGroup_2_6 = (Group)cGroup_2.eContents().get(6);
private final Assignment cContextualizersAssignment_2_6_0 = (Assignment)cGroup_2_6.eContents().get(0);
private final RuleCall cContextualizersContextualizationParserRuleCall_2_6_0_0 = (RuleCall)cContextualizersAssignment_2_6_0.eContents().get(0);
private final Assignment cContextualizersAssignment_2_6_1 = (Assignment)cGroup_2_6.eContents().get(1);
private final RuleCall cContextualizersContextualizationParserRuleCall_2_6_1_0 = (RuleCall)cContextualizersAssignment_2_6_1.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_2_7 = (UnorderedGroup)cGroup_2.eContents().get(7);
private final Group cGroup_2_7_0 = (Group)cUnorderedGroup_2_7.eContents().get(0);
private final Keyword cWithKeyword_2_7_0_0 = (Keyword)cGroup_2_7_0.eContents().get(0);
private final Keyword cMetadataKeyword_2_7_0_1 = (Keyword)cGroup_2_7_0.eContents().get(1);
private final Assignment cMetadataAssignment_2_7_0_2 = (Assignment)cGroup_2_7_0.eContents().get(2);
private final RuleCall cMetadataMetadataParserRuleCall_2_7_0_2_0 = (RuleCall)cMetadataAssignment_2_7_0_2.eContents().get(0);
private final Group cGroup_2_7_1 = (Group)cUnorderedGroup_2_7.eContents().get(1);
private final Keyword cUsingKeyword_2_7_1_0 = (Keyword)cGroup_2_7_1.eContents().get(0);
private final Alternatives cAlternatives_2_7_1_1 = (Alternatives)cGroup_2_7_1.eContents().get(1);
private final Assignment cAccessorAssignment_2_7_1_1_0 = (Assignment)cAlternatives_2_7_1_1.eContents().get(0);
private final RuleCall cAccessorFunctionParserRuleCall_2_7_1_1_0_0 = (RuleCall)cAccessorAssignment_2_7_1_1_0.eContents().get(0);
private final Assignment cLookupAssignment_2_7_1_1_1 = (Assignment)cAlternatives_2_7_1_1.eContents().get(1);
private final RuleCall cLookupLookupFunctionParserRuleCall_2_7_1_1_1_0 = (RuleCall)cLookupAssignment_2_7_1_1_1.eContents().get(0);
/// **
// * The models in 'using' are used as a context model to choose the
// * generator contextually. There is no other use for dependencies in a model; observers
// * use those as actual dependencies.
// *
// * 'as' is optional (if not there, define an agent (subject) with the dependencies; in that
// * case, using should be like in observation generator.)
// *
// * If the observable is *, this is used as the top-level obs and the observable is generated
// * as an instance of the union of the observable for the observers, which must be capable of
// * producing the observables.
// *
// * /
//ModelStatement:
// (private?='private'? & inactive?='void'?) 'model' (observables+=ModelObservable ('named' name=ValidID)? (=> ','
// observables+=ModelObservableAdditional)*) ('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
// ('as' observers=ObservationGeneratorSwitch | ('using' (accessor=Function | lookup=LookupFunction))? &
// (contextualizers+=Contextualization contextualizers+=Contextualization*)?) ('with' 'metadata' metadata=Metadata)? |
// (private?='private'? & inactive?='void'?) interpreter?='model' ('named' name=ValidID)?
// observers=ObservationGeneratorSwitch ('named' name2=ValidID)? ('with' 'metadata' metadata=Metadata)? |
// (private?='private'? & inactive?='void'?) 'model' reification?='each' (agentSource=Function ('named' name=ValidID)?
// 'as' observable+=ConceptDeclaration | observable+=ConceptDeclaration ('named' name=ValidID)?) ('observing'
// dependencies+=Dependency (=> ',' dependencies+=Dependency)*)? (=> 'interpret'
// attributeTranslators+=AttributeTranslator (',' attributeTranslators+=AttributeTranslator)*)?
// (contextualizers+=Contextualization contextualizers+=Contextualization*)? (('with' 'metadata' metadata=Metadata)? &
// ('using' (accessor=Function | lookup=LookupFunction))?);
@Override public ParserRule getRule() { return rule; }
//(private?='private'? & inactive?='void'?) 'model' (observables+=ModelObservable ('named' name=ValidID)? (=> ','
//observables+=ModelObservableAdditional)*) ('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
//('as' observers=ObservationGeneratorSwitch | ('using' (accessor=Function | lookup=LookupFunction))? &
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?) ('with' 'metadata' metadata=Metadata)? |
//(private?='private'? & inactive?='void'?) interpreter?='model' ('named' name=ValidID)?
//observers=ObservationGeneratorSwitch ('named' name2=ValidID)? ('with' 'metadata' metadata=Metadata)? |
//(private?='private'? & inactive?='void'?) 'model' reification?='each' (agentSource=Function ('named' name=ValidID)?
//'as' observable+=ConceptDeclaration | observable+=ConceptDeclaration ('named' name=ValidID)?) ('observing'
//dependencies+=Dependency (=> ',' dependencies+=Dependency)*)? (=> 'interpret'
//attributeTranslators+=AttributeTranslator (',' attributeTranslators+=AttributeTranslator)*)?
//(contextualizers+=Contextualization contextualizers+=Contextualization*)? (('with' 'metadata' metadata=Metadata)? &
//('using' (accessor=Function | lookup=LookupFunction))?)
public Alternatives getAlternatives() { return cAlternatives; }
//(private?='private'? & inactive?='void'?) 'model' (observables+=ModelObservable ('named' name=ValidID)? (=> ','
//observables+=ModelObservableAdditional)*) ('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
//('as' observers=ObservationGeneratorSwitch | ('using' (accessor=Function | lookup=LookupFunction))? &
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?) ('with' 'metadata' metadata=Metadata)?
public Group getGroup_0() { return cGroup_0; }
//(private?='private'? & inactive?='void'?)
public UnorderedGroup getUnorderedGroup_0_0() { return cUnorderedGroup_0_0; }
//private?='private'?
public Assignment getPrivateAssignment_0_0_0() { return cPrivateAssignment_0_0_0; }
//'private'
public Keyword getPrivatePrivateKeyword_0_0_0_0() { return cPrivatePrivateKeyword_0_0_0_0; }
//inactive?='void'?
public Assignment getInactiveAssignment_0_0_1() { return cInactiveAssignment_0_0_1; }
//'void'
public Keyword getInactiveVoidKeyword_0_0_1_0() { return cInactiveVoidKeyword_0_0_1_0; }
//'model'
public Keyword getModelKeyword_0_1() { return cModelKeyword_0_1; }
//(observables+=ModelObservable ('named' name=ValidID)? (=> ',' observables+=ModelObservableAdditional)*)
public Group getGroup_0_2() { return cGroup_0_2; }
//observables+=ModelObservable
public Assignment getObservablesAssignment_0_2_0() { return cObservablesAssignment_0_2_0; }
//ModelObservable
public RuleCall getObservablesModelObservableParserRuleCall_0_2_0_0() { return cObservablesModelObservableParserRuleCall_0_2_0_0; }
//('named' name=ValidID)?
public Group getGroup_0_2_1() { return cGroup_0_2_1; }
//'named'
public Keyword getNamedKeyword_0_2_1_0() { return cNamedKeyword_0_2_1_0; }
//name=ValidID
public Assignment getNameAssignment_0_2_1_1() { return cNameAssignment_0_2_1_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_0_2_1_1_0() { return cNameValidIDParserRuleCall_0_2_1_1_0; }
//(=> ',' observables+=ModelObservableAdditional)*
public Group getGroup_0_2_2() { return cGroup_0_2_2; }
//=> ','
public Keyword getCommaKeyword_0_2_2_0() { return cCommaKeyword_0_2_2_0; }
//observables+=ModelObservableAdditional
public Assignment getObservablesAssignment_0_2_2_1() { return cObservablesAssignment_0_2_2_1; }
//ModelObservableAdditional
public RuleCall getObservablesModelObservableAdditionalParserRuleCall_0_2_2_1_0() { return cObservablesModelObservableAdditionalParserRuleCall_0_2_2_1_0; }
//('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
public Group getGroup_0_3() { return cGroup_0_3; }
//'observing'
public Keyword getObservingKeyword_0_3_0() { return cObservingKeyword_0_3_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_0_3_1() { return cDependenciesAssignment_0_3_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_0_3_1_0() { return cDependenciesDependencyParserRuleCall_0_3_1_0; }
//(=> ',' dependencies+=Dependency)*
public Group getGroup_0_3_2() { return cGroup_0_3_2; }
//=> ','
public Keyword getCommaKeyword_0_3_2_0() { return cCommaKeyword_0_3_2_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_0_3_2_1() { return cDependenciesAssignment_0_3_2_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_0_3_2_1_0() { return cDependenciesDependencyParserRuleCall_0_3_2_1_0; }
//('as' observers=ObservationGeneratorSwitch | ('using' (accessor=Function | lookup=LookupFunction))? &
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?)
public Alternatives getAlternatives_0_4() { return cAlternatives_0_4; }
//'as' observers=ObservationGeneratorSwitch
public Group getGroup_0_4_0() { return cGroup_0_4_0; }
//'as'
public Keyword getAsKeyword_0_4_0_0() { return cAsKeyword_0_4_0_0; }
//observers=ObservationGeneratorSwitch
public Assignment getObserversAssignment_0_4_0_1() { return cObserversAssignment_0_4_0_1; }
//ObservationGeneratorSwitch
public RuleCall getObserversObservationGeneratorSwitchParserRuleCall_0_4_0_1_0() { return cObserversObservationGeneratorSwitchParserRuleCall_0_4_0_1_0; }
//('using' (accessor=Function | lookup=LookupFunction))? & (contextualizers+=Contextualization
//contextualizers+=Contextualization*)?
public UnorderedGroup getUnorderedGroup_0_4_1() { return cUnorderedGroup_0_4_1; }
//('using' (accessor=Function | lookup=LookupFunction))?
public Group getGroup_0_4_1_0() { return cGroup_0_4_1_0; }
//'using'
public Keyword getUsingKeyword_0_4_1_0_0() { return cUsingKeyword_0_4_1_0_0; }
//(accessor=Function | lookup=LookupFunction)
public Alternatives getAlternatives_0_4_1_0_1() { return cAlternatives_0_4_1_0_1; }
//accessor=Function
public Assignment getAccessorAssignment_0_4_1_0_1_0() { return cAccessorAssignment_0_4_1_0_1_0; }
//Function
public RuleCall getAccessorFunctionParserRuleCall_0_4_1_0_1_0_0() { return cAccessorFunctionParserRuleCall_0_4_1_0_1_0_0; }
//lookup=LookupFunction
public Assignment getLookupAssignment_0_4_1_0_1_1() { return cLookupAssignment_0_4_1_0_1_1; }
//LookupFunction
public RuleCall getLookupLookupFunctionParserRuleCall_0_4_1_0_1_1_0() { return cLookupLookupFunctionParserRuleCall_0_4_1_0_1_1_0; }
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?
public Group getGroup_0_4_1_1() { return cGroup_0_4_1_1; }
//contextualizers+=Contextualization
public Assignment getContextualizersAssignment_0_4_1_1_0() { return cContextualizersAssignment_0_4_1_1_0; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_0_4_1_1_0_0() { return cContextualizersContextualizationParserRuleCall_0_4_1_1_0_0; }
//contextualizers+=Contextualization*
public Assignment getContextualizersAssignment_0_4_1_1_1() { return cContextualizersAssignment_0_4_1_1_1; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_0_4_1_1_1_0() { return cContextualizersContextualizationParserRuleCall_0_4_1_1_1_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_0_5() { return cGroup_0_5; }
//'with'
public Keyword getWithKeyword_0_5_0() { return cWithKeyword_0_5_0; }
//'metadata'
public Keyword getMetadataKeyword_0_5_1() { return cMetadataKeyword_0_5_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_0_5_2() { return cMetadataAssignment_0_5_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_0_5_2_0() { return cMetadataMetadataParserRuleCall_0_5_2_0; }
//(private?='private'? & inactive?='void'?) interpreter?='model' ('named' name=ValidID)?
//observers=ObservationGeneratorSwitch ('named' name2=ValidID)? ('with' 'metadata' metadata=Metadata)?
public Group getGroup_1() { return cGroup_1; }
//(private?='private'? & inactive?='void'?)
public UnorderedGroup getUnorderedGroup_1_0() { return cUnorderedGroup_1_0; }
//private?='private'?
public Assignment getPrivateAssignment_1_0_0() { return cPrivateAssignment_1_0_0; }
//'private'
public Keyword getPrivatePrivateKeyword_1_0_0_0() { return cPrivatePrivateKeyword_1_0_0_0; }
//inactive?='void'?
public Assignment getInactiveAssignment_1_0_1() { return cInactiveAssignment_1_0_1; }
//'void'
public Keyword getInactiveVoidKeyword_1_0_1_0() { return cInactiveVoidKeyword_1_0_1_0; }
//interpreter?='model'
public Assignment getInterpreterAssignment_1_1() { return cInterpreterAssignment_1_1; }
//'model'
public Keyword getInterpreterModelKeyword_1_1_0() { return cInterpreterModelKeyword_1_1_0; }
//('named' name=ValidID)?
public Group getGroup_1_2() { return cGroup_1_2; }
//'named'
public Keyword getNamedKeyword_1_2_0() { return cNamedKeyword_1_2_0; }
//name=ValidID
public Assignment getNameAssignment_1_2_1() { return cNameAssignment_1_2_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_1_2_1_0() { return cNameValidIDParserRuleCall_1_2_1_0; }
//observers=ObservationGeneratorSwitch
public Assignment getObserversAssignment_1_3() { return cObserversAssignment_1_3; }
//ObservationGeneratorSwitch
public RuleCall getObserversObservationGeneratorSwitchParserRuleCall_1_3_0() { return cObserversObservationGeneratorSwitchParserRuleCall_1_3_0; }
//('named' name2=ValidID)?
public Group getGroup_1_4() { return cGroup_1_4; }
//'named'
public Keyword getNamedKeyword_1_4_0() { return cNamedKeyword_1_4_0; }
//name2=ValidID
public Assignment getName2Assignment_1_4_1() { return cName2Assignment_1_4_1; }
//ValidID
public RuleCall getName2ValidIDParserRuleCall_1_4_1_0() { return cName2ValidIDParserRuleCall_1_4_1_0; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_1_5() { return cGroup_1_5; }
//'with'
public Keyword getWithKeyword_1_5_0() { return cWithKeyword_1_5_0; }
//'metadata'
public Keyword getMetadataKeyword_1_5_1() { return cMetadataKeyword_1_5_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_1_5_2() { return cMetadataAssignment_1_5_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_1_5_2_0() { return cMetadataMetadataParserRuleCall_1_5_2_0; }
//(private?='private'? & inactive?='void'?) 'model' reification?='each' (agentSource=Function ('named' name=ValidID)? 'as'
//observable+=ConceptDeclaration | observable+=ConceptDeclaration ('named' name=ValidID)?) ('observing'
//dependencies+=Dependency (=> ',' dependencies+=Dependency)*)? (=> 'interpret'
//attributeTranslators+=AttributeTranslator (',' attributeTranslators+=AttributeTranslator)*)?
//(contextualizers+=Contextualization contextualizers+=Contextualization*)? (('with' 'metadata' metadata=Metadata)? &
//('using' (accessor=Function | lookup=LookupFunction))?)
public Group getGroup_2() { return cGroup_2; }
//(private?='private'? & inactive?='void'?)
public UnorderedGroup getUnorderedGroup_2_0() { return cUnorderedGroup_2_0; }
//private?='private'?
public Assignment getPrivateAssignment_2_0_0() { return cPrivateAssignment_2_0_0; }
//'private'
public Keyword getPrivatePrivateKeyword_2_0_0_0() { return cPrivatePrivateKeyword_2_0_0_0; }
//inactive?='void'?
public Assignment getInactiveAssignment_2_0_1() { return cInactiveAssignment_2_0_1; }
//'void'
public Keyword getInactiveVoidKeyword_2_0_1_0() { return cInactiveVoidKeyword_2_0_1_0; }
//'model'
public Keyword getModelKeyword_2_1() { return cModelKeyword_2_1; }
//reification?='each'
public Assignment getReificationAssignment_2_2() { return cReificationAssignment_2_2; }
//'each'
public Keyword getReificationEachKeyword_2_2_0() { return cReificationEachKeyword_2_2_0; }
//(agentSource=Function ('named' name=ValidID)? 'as' observable+=ConceptDeclaration | observable+=ConceptDeclaration
//('named' name=ValidID)?)
public Alternatives getAlternatives_2_3() { return cAlternatives_2_3; }
//agentSource=Function ('named' name=ValidID)? 'as' observable+=ConceptDeclaration
public Group getGroup_2_3_0() { return cGroup_2_3_0; }
//agentSource=Function
public Assignment getAgentSourceAssignment_2_3_0_0() { return cAgentSourceAssignment_2_3_0_0; }
//Function
public RuleCall getAgentSourceFunctionParserRuleCall_2_3_0_0_0() { return cAgentSourceFunctionParserRuleCall_2_3_0_0_0; }
//('named' name=ValidID)?
public Group getGroup_2_3_0_1() { return cGroup_2_3_0_1; }
//'named'
public Keyword getNamedKeyword_2_3_0_1_0() { return cNamedKeyword_2_3_0_1_0; }
//name=ValidID
public Assignment getNameAssignment_2_3_0_1_1() { return cNameAssignment_2_3_0_1_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_3_0_1_1_0() { return cNameValidIDParserRuleCall_2_3_0_1_1_0; }
//'as'
public Keyword getAsKeyword_2_3_0_2() { return cAsKeyword_2_3_0_2; }
//observable+=ConceptDeclaration
public Assignment getObservableAssignment_2_3_0_3() { return cObservableAssignment_2_3_0_3; }
//ConceptDeclaration
public RuleCall getObservableConceptDeclarationParserRuleCall_2_3_0_3_0() { return cObservableConceptDeclarationParserRuleCall_2_3_0_3_0; }
//observable+=ConceptDeclaration ('named' name=ValidID)?
public Group getGroup_2_3_1() { return cGroup_2_3_1; }
//observable+=ConceptDeclaration
public Assignment getObservableAssignment_2_3_1_0() { return cObservableAssignment_2_3_1_0; }
//ConceptDeclaration
public RuleCall getObservableConceptDeclarationParserRuleCall_2_3_1_0_0() { return cObservableConceptDeclarationParserRuleCall_2_3_1_0_0; }
//('named' name=ValidID)?
public Group getGroup_2_3_1_1() { return cGroup_2_3_1_1; }
//'named'
public Keyword getNamedKeyword_2_3_1_1_0() { return cNamedKeyword_2_3_1_1_0; }
//name=ValidID
public Assignment getNameAssignment_2_3_1_1_1() { return cNameAssignment_2_3_1_1_1; }
//ValidID
public RuleCall getNameValidIDParserRuleCall_2_3_1_1_1_0() { return cNameValidIDParserRuleCall_2_3_1_1_1_0; }
//('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
public Group getGroup_2_4() { return cGroup_2_4; }
//'observing'
public Keyword getObservingKeyword_2_4_0() { return cObservingKeyword_2_4_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_2_4_1() { return cDependenciesAssignment_2_4_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_2_4_1_0() { return cDependenciesDependencyParserRuleCall_2_4_1_0; }
//(=> ',' dependencies+=Dependency)*
public Group getGroup_2_4_2() { return cGroup_2_4_2; }
//=> ','
public Keyword getCommaKeyword_2_4_2_0() { return cCommaKeyword_2_4_2_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_2_4_2_1() { return cDependenciesAssignment_2_4_2_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_2_4_2_1_0() { return cDependenciesDependencyParserRuleCall_2_4_2_1_0; }
//(=> 'interpret' attributeTranslators+=AttributeTranslator (',' attributeTranslators+=AttributeTranslator)*)?
public Group getGroup_2_5() { return cGroup_2_5; }
//=> 'interpret'
public Keyword getInterpretKeyword_2_5_0() { return cInterpretKeyword_2_5_0; }
//attributeTranslators+=AttributeTranslator
public Assignment getAttributeTranslatorsAssignment_2_5_1() { return cAttributeTranslatorsAssignment_2_5_1; }
//AttributeTranslator
public RuleCall getAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_1_0() { return cAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_1_0; }
//(',' attributeTranslators+=AttributeTranslator)*
public Group getGroup_2_5_2() { return cGroup_2_5_2; }
//','
public Keyword getCommaKeyword_2_5_2_0() { return cCommaKeyword_2_5_2_0; }
//attributeTranslators+=AttributeTranslator
public Assignment getAttributeTranslatorsAssignment_2_5_2_1() { return cAttributeTranslatorsAssignment_2_5_2_1; }
//AttributeTranslator
public RuleCall getAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_2_1_0() { return cAttributeTranslatorsAttributeTranslatorParserRuleCall_2_5_2_1_0; }
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?
public Group getGroup_2_6() { return cGroup_2_6; }
//contextualizers+=Contextualization
public Assignment getContextualizersAssignment_2_6_0() { return cContextualizersAssignment_2_6_0; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_2_6_0_0() { return cContextualizersContextualizationParserRuleCall_2_6_0_0; }
//contextualizers+=Contextualization*
public Assignment getContextualizersAssignment_2_6_1() { return cContextualizersAssignment_2_6_1; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_2_6_1_0() { return cContextualizersContextualizationParserRuleCall_2_6_1_0; }
//(('with' 'metadata' metadata=Metadata)? & ('using' (accessor=Function | lookup=LookupFunction))?)
public UnorderedGroup getUnorderedGroup_2_7() { return cUnorderedGroup_2_7; }
//('with' 'metadata' metadata=Metadata)?
public Group getGroup_2_7_0() { return cGroup_2_7_0; }
//'with'
public Keyword getWithKeyword_2_7_0_0() { return cWithKeyword_2_7_0_0; }
//'metadata'
public Keyword getMetadataKeyword_2_7_0_1() { return cMetadataKeyword_2_7_0_1; }
//metadata=Metadata
public Assignment getMetadataAssignment_2_7_0_2() { return cMetadataAssignment_2_7_0_2; }
//Metadata
public RuleCall getMetadataMetadataParserRuleCall_2_7_0_2_0() { return cMetadataMetadataParserRuleCall_2_7_0_2_0; }
//('using' (accessor=Function | lookup=LookupFunction))?
public Group getGroup_2_7_1() { return cGroup_2_7_1; }
//'using'
public Keyword getUsingKeyword_2_7_1_0() { return cUsingKeyword_2_7_1_0; }
//(accessor=Function | lookup=LookupFunction)
public Alternatives getAlternatives_2_7_1_1() { return cAlternatives_2_7_1_1; }
//accessor=Function
public Assignment getAccessorAssignment_2_7_1_1_0() { return cAccessorAssignment_2_7_1_1_0; }
//Function
public RuleCall getAccessorFunctionParserRuleCall_2_7_1_1_0_0() { return cAccessorFunctionParserRuleCall_2_7_1_1_0_0; }
//lookup=LookupFunction
public Assignment getLookupAssignment_2_7_1_1_1() { return cLookupAssignment_2_7_1_1_1; }
//LookupFunction
public RuleCall getLookupLookupFunctionParserRuleCall_2_7_1_1_1_0() { return cLookupLookupFunctionParserRuleCall_2_7_1_1_1_0; }
}
public class ContextualizeStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ContextualizeStatement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final UnorderedGroup cUnorderedGroup_0 = (UnorderedGroup)cGroup.eContents().get(0);
private final Assignment cPrivateAssignment_0_0 = (Assignment)cUnorderedGroup_0.eContents().get(0);
private final Keyword cPrivatePrivateKeyword_0_0_0 = (Keyword)cPrivateAssignment_0_0.eContents().get(0);
private final Assignment cInactiveAssignment_0_1 = (Assignment)cUnorderedGroup_0.eContents().get(1);
private final Keyword cInactiveVoidKeyword_0_1_0 = (Keyword)cInactiveAssignment_0_1.eContents().get(0);
private final Keyword cContextualizeKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cConceptAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cConceptDerivedConceptDeclarationParserRuleCall_2_0 = (RuleCall)cConceptAssignment_2.eContents().get(0);
private final UnorderedGroup cUnorderedGroup_3 = (UnorderedGroup)cGroup.eContents().get(3);
private final Assignment cRoleStatementAssignment_3_0 = (Assignment)cUnorderedGroup_3.eContents().get(0);
private final RuleCall cRoleStatementRoleStatementParserRuleCall_3_0_0 = (RuleCall)cRoleStatementAssignment_3_0.eContents().get(0);
private final Assignment cResolutionStatementAssignment_3_1 = (Assignment)cUnorderedGroup_3.eContents().get(1);
private final RuleCall cResolutionStatementResolutionStatementParserRuleCall_3_1_0 = (RuleCall)cResolutionStatementAssignment_3_1.eContents().get(0);
//ContextualizeStatement:
// (private?='private'? & inactive?='void'?) 'contextualize' concept=DerivedConceptDeclaration
// (roleStatement+=RoleStatement* & resolutionStatement+=ResolutionStatement*);
@Override public ParserRule getRule() { return rule; }
//(private?='private'? & inactive?='void'?) 'contextualize' concept=DerivedConceptDeclaration
//(roleStatement+=RoleStatement* & resolutionStatement+=ResolutionStatement*)
public Group getGroup() { return cGroup; }
//(private?='private'? & inactive?='void'?)
public UnorderedGroup getUnorderedGroup_0() { return cUnorderedGroup_0; }
//private?='private'?
public Assignment getPrivateAssignment_0_0() { return cPrivateAssignment_0_0; }
//'private'
public Keyword getPrivatePrivateKeyword_0_0_0() { return cPrivatePrivateKeyword_0_0_0; }
//inactive?='void'?
public Assignment getInactiveAssignment_0_1() { return cInactiveAssignment_0_1; }
//'void'
public Keyword getInactiveVoidKeyword_0_1_0() { return cInactiveVoidKeyword_0_1_0; }
//'contextualize'
public Keyword getContextualizeKeyword_1() { return cContextualizeKeyword_1; }
//concept=DerivedConceptDeclaration
public Assignment getConceptAssignment_2() { return cConceptAssignment_2; }
//DerivedConceptDeclaration
public RuleCall getConceptDerivedConceptDeclarationParserRuleCall_2_0() { return cConceptDerivedConceptDeclarationParserRuleCall_2_0; }
//(roleStatement+=RoleStatement* & resolutionStatement+=ResolutionStatement*)
public UnorderedGroup getUnorderedGroup_3() { return cUnorderedGroup_3; }
//roleStatement+=RoleStatement*
public Assignment getRoleStatementAssignment_3_0() { return cRoleStatementAssignment_3_0; }
//RoleStatement
public RuleCall getRoleStatementRoleStatementParserRuleCall_3_0_0() { return cRoleStatementRoleStatementParserRuleCall_3_0_0; }
//resolutionStatement+=ResolutionStatement*
public Assignment getResolutionStatementAssignment_3_1() { return cResolutionStatementAssignment_3_1; }
//ResolutionStatement
public RuleCall getResolutionStatementResolutionStatementParserRuleCall_3_1_0() { return cResolutionStatementResolutionStatementParserRuleCall_3_1_0; }
}
public class ResolutionStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ResolutionStatement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cResolveKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Keyword cToKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cResolutionsAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cResolutionsConditionalResolutionParserRuleCall_2_0 = (RuleCall)cResolutionsAssignment_2.eContents().get(0);
private final Group cGroup_3 = (Group)cGroup.eContents().get(3);
private final Keyword cCommaKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cResolutionsAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cResolutionsConditionalResolutionParserRuleCall_3_1_0 = (RuleCall)cResolutionsAssignment_3_1.eContents().get(0);
private final Group cGroup_4 = (Group)cGroup.eContents().get(4);
private final Keyword cObservingKeyword_4_0 = (Keyword)cGroup_4.eContents().get(0);
private final Assignment cDependenciesAssignment_4_1 = (Assignment)cGroup_4.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_4_1_0 = (RuleCall)cDependenciesAssignment_4_1.eContents().get(0);
private final Group cGroup_4_2 = (Group)cGroup_4.eContents().get(2);
private final Keyword cCommaKeyword_4_2_0 = (Keyword)cGroup_4_2.eContents().get(0);
private final Assignment cDependenciesAssignment_4_2_1 = (Assignment)cGroup_4_2.eContents().get(1);
private final RuleCall cDependenciesDependencyParserRuleCall_4_2_1_0 = (RuleCall)cDependenciesAssignment_4_2_1.eContents().get(0);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Assignment cContextualizersAssignment_5_0 = (Assignment)cGroup_5.eContents().get(0);
private final RuleCall cContextualizersContextualizationParserRuleCall_5_0_0 = (RuleCall)cContextualizersAssignment_5_0.eContents().get(0);
private final Assignment cContextualizersAssignment_5_1 = (Assignment)cGroup_5.eContents().get(1);
private final RuleCall cContextualizersContextualizationParserRuleCall_5_1_0 = (RuleCall)cContextualizersAssignment_5_1.eContents().get(0);
private final Group cGroup_6 = (Group)cGroup.eContents().get(6);
private final Keyword cUsingKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Alternatives cAlternatives_6_1 = (Alternatives)cGroup_6.eContents().get(1);
private final Assignment cAccessorAssignment_6_1_0 = (Assignment)cAlternatives_6_1.eContents().get(0);
private final RuleCall cAccessorFunctionParserRuleCall_6_1_0_0 = (RuleCall)cAccessorAssignment_6_1_0.eContents().get(0);
private final Assignment cLookupAssignment_6_1_1 = (Assignment)cAlternatives_6_1.eContents().get(1);
private final RuleCall cLookupLookupFunctionParserRuleCall_6_1_1_0 = (RuleCall)cLookupAssignment_6_1_1.eContents().get(0);
//ResolutionStatement:
// 'resolve' 'to' resolutions+=ConditionalResolution (=> ',' resolutions+=ConditionalResolution) ('observing'
// dependencies+=Dependency (=> ',' dependencies+=Dependency)*)? (contextualizers+=Contextualization
// contextualizers+=Contextualization*)? ('using' (accessor=Function | lookup=LookupFunction))?;
@Override public ParserRule getRule() { return rule; }
//'resolve' 'to' resolutions+=ConditionalResolution (=> ',' resolutions+=ConditionalResolution) ('observing'
//dependencies+=Dependency (=> ',' dependencies+=Dependency)*)? (contextualizers+=Contextualization
//contextualizers+=Contextualization*)? ('using' (accessor=Function | lookup=LookupFunction))?
public Group getGroup() { return cGroup; }
//'resolve'
public Keyword getResolveKeyword_0() { return cResolveKeyword_0; }
//'to'
public Keyword getToKeyword_1() { return cToKeyword_1; }
//resolutions+=ConditionalResolution
public Assignment getResolutionsAssignment_2() { return cResolutionsAssignment_2; }
//ConditionalResolution
public RuleCall getResolutionsConditionalResolutionParserRuleCall_2_0() { return cResolutionsConditionalResolutionParserRuleCall_2_0; }
//(=> ',' resolutions+=ConditionalResolution)
public Group getGroup_3() { return cGroup_3; }
//=> ','
public Keyword getCommaKeyword_3_0() { return cCommaKeyword_3_0; }
//resolutions+=ConditionalResolution
public Assignment getResolutionsAssignment_3_1() { return cResolutionsAssignment_3_1; }
//ConditionalResolution
public RuleCall getResolutionsConditionalResolutionParserRuleCall_3_1_0() { return cResolutionsConditionalResolutionParserRuleCall_3_1_0; }
//('observing' dependencies+=Dependency (=> ',' dependencies+=Dependency)*)?
public Group getGroup_4() { return cGroup_4; }
//'observing'
public Keyword getObservingKeyword_4_0() { return cObservingKeyword_4_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_4_1() { return cDependenciesAssignment_4_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_4_1_0() { return cDependenciesDependencyParserRuleCall_4_1_0; }
//(=> ',' dependencies+=Dependency)*
public Group getGroup_4_2() { return cGroup_4_2; }
//=> ','
public Keyword getCommaKeyword_4_2_0() { return cCommaKeyword_4_2_0; }
//dependencies+=Dependency
public Assignment getDependenciesAssignment_4_2_1() { return cDependenciesAssignment_4_2_1; }
//Dependency
public RuleCall getDependenciesDependencyParserRuleCall_4_2_1_0() { return cDependenciesDependencyParserRuleCall_4_2_1_0; }
//(contextualizers+=Contextualization contextualizers+=Contextualization*)?
public Group getGroup_5() { return cGroup_5; }
//contextualizers+=Contextualization
public Assignment getContextualizersAssignment_5_0() { return cContextualizersAssignment_5_0; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_5_0_0() { return cContextualizersContextualizationParserRuleCall_5_0_0; }
//contextualizers+=Contextualization*
public Assignment getContextualizersAssignment_5_1() { return cContextualizersAssignment_5_1; }
//Contextualization
public RuleCall getContextualizersContextualizationParserRuleCall_5_1_0() { return cContextualizersContextualizationParserRuleCall_5_1_0; }
//('using' (accessor=Function | lookup=LookupFunction))?
public Group getGroup_6() { return cGroup_6; }
//'using'
public Keyword getUsingKeyword_6_0() { return cUsingKeyword_6_0; }
//(accessor=Function | lookup=LookupFunction)
public Alternatives getAlternatives_6_1() { return cAlternatives_6_1; }
//accessor=Function
public Assignment getAccessorAssignment_6_1_0() { return cAccessorAssignment_6_1_0; }
//Function
public RuleCall getAccessorFunctionParserRuleCall_6_1_0_0() { return cAccessorFunctionParserRuleCall_6_1_0_0; }
//lookup=LookupFunction
public Assignment getLookupAssignment_6_1_1() { return cLookupAssignment_6_1_1; }
//LookupFunction
public RuleCall getLookupLookupFunctionParserRuleCall_6_1_1_0() { return cLookupLookupFunctionParserRuleCall_6_1_1_0; }
}
public class ConditionalResolutionElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.ConditionalResolution");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cConcreteConceptAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cConcreteConceptConceptDeclarationParserRuleCall_0_0 = (RuleCall)cConcreteConceptAssignment_0.eContents().get(0);
private final Alternatives cAlternatives_1 = (Alternatives)cGroup.eContents().get(1);
private final Group cGroup_1_0 = (Group)cAlternatives_1.eContents().get(0);
private final Keyword cIfKeyword_1_0_0 = (Keyword)cGroup_1_0.eContents().get(0);
private final Assignment cExprAssignment_1_0_1 = (Assignment)cGroup_1_0.eContents().get(1);
private final RuleCall cExprEXPRTerminalRuleCall_1_0_1_0 = (RuleCall)cExprAssignment_1_0_1.eContents().get(0);
private final Assignment cOtherwiseAssignment_1_1 = (Assignment)cAlternatives_1.eContents().get(1);
private final Keyword cOtherwiseOtherwiseKeyword_1_1_0 = (Keyword)cOtherwiseAssignment_1_1.eContents().get(0);
//ConditionalResolution:
// concreteConcept=ConceptDeclaration ('if' expr=EXPR | otherwise?='otherwise')?;
@Override public ParserRule getRule() { return rule; }
//concreteConcept=ConceptDeclaration ('if' expr=EXPR | otherwise?='otherwise')?
public Group getGroup() { return cGroup; }
//concreteConcept=ConceptDeclaration
public Assignment getConcreteConceptAssignment_0() { return cConcreteConceptAssignment_0; }
//ConceptDeclaration
public RuleCall getConcreteConceptConceptDeclarationParserRuleCall_0_0() { return cConcreteConceptConceptDeclarationParserRuleCall_0_0; }
//('if' expr=EXPR | otherwise?='otherwise')?
public Alternatives getAlternatives_1() { return cAlternatives_1; }
//'if' expr=EXPR
public Group getGroup_1_0() { return cGroup_1_0; }
//'if'
public Keyword getIfKeyword_1_0_0() { return cIfKeyword_1_0_0; }
//expr=EXPR
public Assignment getExprAssignment_1_0_1() { return cExprAssignment_1_0_1; }
//EXPR
public RuleCall getExprEXPRTerminalRuleCall_1_0_1_0() { return cExprEXPRTerminalRuleCall_1_0_1_0; }
//otherwise?='otherwise'
public Assignment getOtherwiseAssignment_1_1() { return cOtherwiseAssignment_1_1; }
//'otherwise'
public Keyword getOtherwiseOtherwiseKeyword_1_1_0() { return cOtherwiseOtherwiseKeyword_1_1_0; }
}
public class RoleStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.RoleStatement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cWithKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Keyword cRoleKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cRoleAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cRoleConceptListParserRuleCall_2_0 = (RuleCall)cRoleAssignment_2.eContents().get(0);
private final Group cGroup_3 = (Group)cGroup.eContents().get(3);
private final Keyword cForKeyword_3_0 = (Keyword)cGroup_3.eContents().get(0);
private final Assignment cTargetObservableAssignment_3_1 = (Assignment)cGroup_3.eContents().get(1);
private final RuleCall cTargetObservableConceptListParserRuleCall_3_1_0 = (RuleCall)cTargetObservableAssignment_3_1.eContents().get(0);
private final Keyword cInKeyword_4 = (Keyword)cGroup.eContents().get(4);
private final Assignment cRestrictedObservableAssignment_5 = (Assignment)cGroup.eContents().get(5);
private final RuleCall cRestrictedObservableConceptListParserRuleCall_5_0 = (RuleCall)cRestrictedObservableAssignment_5.eContents().get(0);
//RoleStatement:
// 'with' 'role' role=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList;
@Override public ParserRule getRule() { return rule; }
//'with' 'role' role=ConceptList ('for' targetObservable=ConceptList)? 'in' restrictedObservable=ConceptList
public Group getGroup() { return cGroup; }
//'with'
public Keyword getWithKeyword_0() { return cWithKeyword_0; }
//'role'
public Keyword getRoleKeyword_1() { return cRoleKeyword_1; }
//role=ConceptList
public Assignment getRoleAssignment_2() { return cRoleAssignment_2; }
//ConceptList
public RuleCall getRoleConceptListParserRuleCall_2_0() { return cRoleConceptListParserRuleCall_2_0; }
//('for' targetObservable=ConceptList)?
public Group getGroup_3() { return cGroup_3; }
//'for'
public Keyword getForKeyword_3_0() { return cForKeyword_3_0; }
//targetObservable=ConceptList
public Assignment getTargetObservableAssignment_3_1() { return cTargetObservableAssignment_3_1; }
//ConceptList
public RuleCall getTargetObservableConceptListParserRuleCall_3_1_0() { return cTargetObservableConceptListParserRuleCall_3_1_0; }
//'in'
public Keyword getInKeyword_4() { return cInKeyword_4; }
//restrictedObservable=ConceptList
public Assignment getRestrictedObservableAssignment_5() { return cRestrictedObservableAssignment_5; }
//ConceptList
public RuleCall getRestrictedObservableConceptListParserRuleCall_5_0() { return cRestrictedObservableConceptListParserRuleCall_5_0; }
}
public class AttributeTranslatorElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.AttributeTranslator");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cAttributeIdAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cAttributeIdValidIDParserRuleCall_0_0 = (RuleCall)cAttributeIdAssignment_0.eContents().get(0);
private final Keyword cAsKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Alternatives cAlternatives_2 = (Alternatives)cGroup.eContents().get(2);
private final Assignment cObserversAssignment_2_0 = (Assignment)cAlternatives_2.eContents().get(0);
private final RuleCall cObserversObservationGeneratorParserRuleCall_2_0_0 = (RuleCall)cObserversAssignment_2_0.eContents().get(0);
private final Assignment cPropertyAssignment_2_1 = (Assignment)cAlternatives_2.eContents().get(1);
private final RuleCall cPropertyValidIDParserRuleCall_2_1_0 = (RuleCall)cPropertyAssignment_2_1.eContents().get(0);
/// *
// * attribute is either a quality observer for de-reification or a property to define an annotation
// * for the objects.
// *
// * /
//AttributeTranslator:
// attributeId=ValidID 'as' (observers=ObservationGenerator | property=ValidID);
@Override public ParserRule getRule() { return rule; }
//attributeId=ValidID 'as' (observers=ObservationGenerator | property=ValidID)
public Group getGroup() { return cGroup; }
//attributeId=ValidID
public Assignment getAttributeIdAssignment_0() { return cAttributeIdAssignment_0; }
//ValidID
public RuleCall getAttributeIdValidIDParserRuleCall_0_0() { return cAttributeIdValidIDParserRuleCall_0_0; }
//'as'
public Keyword getAsKeyword_1() { return cAsKeyword_1; }
//(observers=ObservationGenerator | property=ValidID)
public Alternatives getAlternatives_2() { return cAlternatives_2; }
//observers=ObservationGenerator
public Assignment getObserversAssignment_2_0() { return cObserversAssignment_2_0; }
//ObservationGenerator
public RuleCall getObserversObservationGeneratorParserRuleCall_2_0_0() { return cObserversObservationGeneratorParserRuleCall_2_0_0; }
//property=ValidID
public Assignment getPropertyAssignment_2_1() { return cPropertyAssignment_2_1; }
//ValidID
public RuleCall getPropertyValidIDParserRuleCall_2_1_0() { return cPropertyValidIDParserRuleCall_2_1_0; }
}
public class ObservationElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.Observation");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Assignment cFunctionAssignment_0 = (Assignment)cAlternatives.eContents().get(0);
private final RuleCall cFunctionFunctionParserRuleCall_0_0 = (RuleCall)cFunctionAssignment_0.eContents().get(0);
private final Assignment cObserverAssignment_1 = (Assignment)cAlternatives.eContents().get(1);
private final RuleCall cObserverObservationGeneratorParserRuleCall_1_0 = (RuleCall)cObserverAssignment_1.eContents().get(0);
private final Assignment cIdAssignment_2 = (Assignment)cAlternatives.eContents().get(2);
private final RuleCall cIdValidIDParserRuleCall_2_0 = (RuleCall)cIdAssignment_2.eContents().get(0);
//Observation:
// function=Function | observer=ObservationGenerator | id=ValidID;
@Override public ParserRule getRule() { return rule; }
//function=Function | observer=ObservationGenerator | id=ValidID
public Alternatives getAlternatives() { return cAlternatives; }
//function=Function
public Assignment getFunctionAssignment_0() { return cFunctionAssignment_0; }
//Function
public RuleCall getFunctionFunctionParserRuleCall_0_0() { return cFunctionFunctionParserRuleCall_0_0; }
//observer=ObservationGenerator
public Assignment getObserverAssignment_1() { return cObserverAssignment_1; }
//ObservationGenerator
public RuleCall getObserverObservationGeneratorParserRuleCall_1_0() { return cObserverObservationGeneratorParserRuleCall_1_0; }
//id=ValidID
public Assignment getIdAssignment_2() { return cIdAssignment_2; }
//ValidID
public RuleCall getIdValidIDParserRuleCall_2_0() { return cIdValidIDParserRuleCall_2_0; }
}
public class DefineStatementElements extends AbstractParserRuleElementFinder {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "org.integratedmodelling.kim.Kim.DefineStatement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cDefineKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Assignment cNameAssignment_1_0 = (Assignment)cGroup_1.eContents().get(0);
private final RuleCall cNameValidIDParserRuleCall_1_0_0 = (RuleCall)cNameAssignment_1_0.eContents().get(0);
private final Keyword cAsKeyword_1_1 = (Keyword)cGroup_1.eContents().get(1);
private final Alternatives cAlternatives_1_2 = (Alternatives)cGroup_1.eContents().get(2);
private final Assignment cValueAssignment_1_2_0 = (Assignment)cAlternatives_1_2.eContents().get(0);
private final RuleCall cValueValueParserRuleCall_1_2_0_0 = (RuleCall)cValueAssignment_1_2_0.eContents().get(0);
private final Assignment cTableAssignment_1_2_1 = (Assignment)cAlternatives_1_2.eContents().get(1);
private final RuleCall cTableTableParserRuleCall_1_2_1_0 = (RuleCall)cTableAssignment_1_2_1.eContents().get(0);
/// **
// * define
© 2015 - 2025 Weber Informatics LLC | Privacy Policy