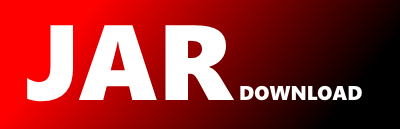
org.interledger.spsp.server.model.ModifiableParentAccountSettings Maven / Gradle / Ivy
Show all versions of spsp-server Show documentation
package org.interledger.spsp.server.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.UnsignedLong;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import java.time.Instant;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.interledger.connector.accounts.AccountBalanceSettings;
import org.interledger.connector.accounts.AccountId;
import org.interledger.connector.accounts.ImmutableAccountBalanceSettings;
import org.interledger.connector.accounts.SettlementEngineDetails;
import org.interledger.link.LinkType;
/**
* A modifiable implementation of the {@link ParentAccountSettings.AbstractParentAccountSettings AbstractParentAccountSettings} type.
* Use the {@link #create()} static factory methods to create new instances.
* Use the {@link #toImmutable()} method to convert to canonical immutable instances.
*
ModifiableParentAccountSettings is not thread-safe
* @see ImmutableParentAccountSettings
*/
@Generated(from = "ParentAccountSettings.AbstractParentAccountSettings", generator = "Modifiables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated({"Modifiables.generator", "ParentAccountSettings.AbstractParentAccountSettings"})
@NotThreadSafe
public final class ModifiableParentAccountSettings
extends ParentAccountSettings.AbstractParentAccountSettings {
private static final long INIT_BIT_ASSET_CODE = 0x1L;
private static final long INIT_BIT_ASSET_SCALE = 0x2L;
private static final long INIT_BIT_ACCOUNT_ID = 0x4L;
private static final long INIT_BIT_LINK_TYPE = 0x8L;
private long initBits = 0xfL;
private String assetCode;
private int assetScale;
private Optional maximumPacketAmount = Optional.empty();
private final Map customSettings = new LinkedHashMap();
private AccountId accountId;
private Instant createdAt;
private Instant modifiedAt;
private LinkType linkType;
private AccountBalanceSettings balanceSettings;
private Optional settlementEngineDetails = Optional.empty();
private ModifiableParentAccountSettings() {}
/**
* Construct a modifiable instance of {@code AbstractParentAccountSettings}.
* @return A new modifiable instance
*/
public static ModifiableParentAccountSettings create() {
return new ModifiableParentAccountSettings();
}
/**
* Currency code or other asset identifier that will be used to select the correct rate for this account.
*/
@JsonProperty("assetCode")
@Override
public final String assetCode() {
if (!assetCodeIsSet()) {
checkRequiredAttributes();
}
return assetCode;
}
/**
* Interledger amounts are integers, but most currencies are typically represented as # fractional units, e.g. cents.
* This property defines how many Interledger units make # up one regular unit. For dollars, this would usually be set
* to 9, so that Interledger # amounts are expressed in nano-dollars.
* @return an int representing this account's asset scale.
*/
@JsonProperty("assetScale")
@Override
public final int assetScale() {
if (!assetScaleIsSet()) {
checkRequiredAttributes();
}
return assetScale;
}
/**
* The maximum amount per-packet for incoming prepare packets. The connector will reject any incoming prepare packets
* from this account with a higher amount.
* @return The maximum packet amount allowed by this account.
*/
@JsonProperty("maximumPacketAmount")
@Override
public final Optional maximumPacketAmount() {
return maximumPacketAmount;
}
/**
* Additional, custom settings that any plugin can define.
*/
@JsonProperty("customSettings")
@Override
public final Map customSettings() {
return customSettings;
}
/**
* @return value of {@code accountId} attribute
*/
@JsonProperty("accountId")
@Override
public final AccountId accountId() {
if (!accountIdIsSet()) {
checkRequiredAttributes();
}
return accountId;
}
/**
* @return assigned or, otherwise, newly computed, not cached value of {@code createdAt} attribute
*/
@JsonProperty("createdAt")
@Override
public final Instant createdAt() {
return createdAtIsSet()
? createdAt
: super.createdAt();
}
/**
* @return assigned or, otherwise, newly computed, not cached value of {@code modifiedAt} attribute
*/
@JsonProperty("modifiedAt")
@Override
public final Instant modifiedAt() {
return modifiedAtIsSet()
? modifiedAt
: super.modifiedAt();
}
/**
* @return value of {@code linkType} attribute
*/
@JsonProperty("linkType")
@Override
public final LinkType linkType() {
if (!linkTypeIsSet()) {
checkRequiredAttributes();
}
return linkType;
}
/**
* @return assigned or, otherwise, newly computed, not cached value of {@code balanceSettings} attribute
*/
@JsonProperty("balanceSettings")
@JsonSerialize(as = ImmutableAccountBalanceSettings.class)
@JsonDeserialize(as = ImmutableAccountBalanceSettings.class)
@Override
public final AccountBalanceSettings balanceSettings() {
return balanceSettingsIsSet()
? balanceSettings
: super.balanceSettings();
}
/**
* @return value of {@code settlementEngineDetails} attribute
*/
@JsonProperty("settlementEngineDetails")
@Override
public final Optional settlementEngineDetails() {
return settlementEngineDetails;
}
/**
* Clears the object by setting all attributes to their initial values.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings clear() {
initBits = 0xfL;
assetCode = null;
assetScale = 0;
maximumPacketAmount = Optional.empty();
customSettings.clear();
accountId = null;
createdAt = null;
modifiedAt = null;
linkType = null;
balanceSettings = null;
settlementEngineDetails = Optional.empty();
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link org.interledger.spsp.server.model.ParentAccountSettings.AbstractParentAccountSettings} instance.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings from(ParentAccountSettings.AbstractParentAccountSettings instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link org.interledger.spsp.server.model.ParentAccountSettings} instance.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings from(ParentAccountSettings instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link ParentAccountSettings.AbstractParentAccountSettings} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableParentAccountSettings from(ModifiableParentAccountSettings instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof ModifiableParentAccountSettings) {
ModifiableParentAccountSettings instance = (ModifiableParentAccountSettings) object;
if (instance.assetCodeIsSet()) {
setAssetCode(instance.assetCode());
}
if (instance.assetScaleIsSet()) {
setAssetScale(instance.assetScale());
}
Optional maximumPacketAmountOptional = instance.maximumPacketAmount();
if (maximumPacketAmountOptional.isPresent()) {
setMaximumPacketAmount(maximumPacketAmountOptional);
}
putAllCustomSettings(instance.customSettings());
if (instance.accountIdIsSet()) {
setAccountId(instance.accountId());
}
setCreatedAt(instance.createdAt());
setModifiedAt(instance.modifiedAt());
if (instance.linkTypeIsSet()) {
setLinkType(instance.linkType());
}
setBalanceSettings(instance.balanceSettings());
Optional settlementEngineDetailsOptional = instance.settlementEngineDetails();
if (settlementEngineDetailsOptional.isPresent()) {
setSettlementEngineDetails(settlementEngineDetailsOptional);
}
return;
}
long bits = 0;
if (object instanceof ParentAccountSettings.AbstractParentAccountSettings) {
ParentAccountSettings.AbstractParentAccountSettings instance = (ParentAccountSettings.AbstractParentAccountSettings) object;
if ((bits & 0x1L) == 0) {
setAccountId(instance.accountId());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
setCreatedAt(instance.createdAt());
bits |= 0x2L;
}
if ((bits & 0x8L) == 0) {
setBalanceSettings(instance.balanceSettings());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional settlementEngineDetailsOptional = instance.settlementEngineDetails();
if (settlementEngineDetailsOptional.isPresent()) {
setSettlementEngineDetails(settlementEngineDetailsOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
setLinkType(instance.linkType());
bits |= 0x20L;
}
if ((bits & 0x4L) == 0) {
setModifiedAt(instance.modifiedAt());
bits |= 0x4L;
}
}
if (object instanceof ParentAccountSettings) {
ParentAccountSettings instance = (ParentAccountSettings) object;
if ((bits & 0x1L) == 0) {
setAccountId(instance.accountId());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
setCreatedAt(instance.createdAt());
bits |= 0x2L;
}
setAssetCode(instance.assetCode());
if ((bits & 0x4L) == 0) {
setModifiedAt(instance.modifiedAt());
bits |= 0x4L;
}
putAllCustomSettings(instance.customSettings());
if ((bits & 0x8L) == 0) {
setBalanceSettings(instance.balanceSettings());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional settlementEngineDetailsOptional = instance.settlementEngineDetails();
if (settlementEngineDetailsOptional.isPresent()) {
setSettlementEngineDetails(settlementEngineDetailsOptional);
}
bits |= 0x10L;
}
setAssetScale(instance.assetScale());
if ((bits & 0x20L) == 0) {
setLinkType(instance.linkType());
bits |= 0x20L;
}
Optional maximumPacketAmountOptional = instance.maximumPacketAmount();
if (maximumPacketAmountOptional.isPresent()) {
setMaximumPacketAmount(maximumPacketAmountOptional);
}
}
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#assetCode() assetCode} attribute.
* @param assetCode The value for assetCode
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setAssetCode(String assetCode) {
this.assetCode = Objects.requireNonNull(assetCode, "assetCode");
initBits &= ~INIT_BIT_ASSET_CODE;
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#assetScale() assetScale} attribute.
* @param assetScale The value for assetScale
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setAssetScale(int assetScale) {
this.assetScale = assetScale;
initBits &= ~INIT_BIT_ASSET_SCALE;
return this;
}
/**
* Assigns a present value for the optional {@link ParentAccountSettings.AbstractParentAccountSettings#maximumPacketAmount() maximumPacketAmount} attribute.
* @param maximumPacketAmount A value for maximumPacketAmount
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setMaximumPacketAmount(UnsignedLong maximumPacketAmount) {
this.maximumPacketAmount = Optional.of(maximumPacketAmount);
return this;
}
/**
* Assigns an optional value for {@link ParentAccountSettings.AbstractParentAccountSettings#maximumPacketAmount() maximumPacketAmount}.
* @param maximumPacketAmount A value for maximumPacketAmount
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setMaximumPacketAmount(Optional maximumPacketAmount) {
this.maximumPacketAmount = Objects.requireNonNull(maximumPacketAmount, "maximumPacketAmount");
return this;
}
/**
* Put one entry to the {@link ParentAccountSettings.AbstractParentAccountSettings#customSettings() customSettings} map.
* @param key The key in customSettings map
* @param value The associated value in the customSettings map
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings putCustomSettings(String key, java.lang.Object value) {
this.customSettings.put(
Objects.requireNonNull(key, "customSettings key"),
Objects.requireNonNull(value, "customSettings value"));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ParentAccountSettings.AbstractParentAccountSettings#customSettings() customSettings} map.
* Nulls are not permitted as keys or values.
* @param entries The entries that will be added to the customSettings map
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setCustomSettings(Map entries) {
this.customSettings.clear();
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
java.lang.Object v = e.getValue();
this.customSettings.put(
Objects.requireNonNull(k, "customSettings key"),
Objects.requireNonNull(v, "customSettings value"));
}
return this;
}
/**
* Put all mappings from the specified map as entries to the {@link ParentAccountSettings.AbstractParentAccountSettings#customSettings() customSettings} map.
* Nulls are not permitted as keys or values.
* @param entries to be added to customSettings map
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings putAllCustomSettings(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
java.lang.Object v = e.getValue();
this.customSettings.put(
Objects.requireNonNull(k, "customSettings key"),
Objects.requireNonNull(v, "customSettings value"));
}
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#accountId() accountId} attribute.
* @param accountId The value for accountId
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setAccountId(AccountId accountId) {
this.accountId = Objects.requireNonNull(accountId, "accountId");
initBits &= ~INIT_BIT_ACCOUNT_ID;
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#createdAt() createdAt} attribute.
* If not set, this attribute will have a default value returned by the initializer of {@link ParentAccountSettings.AbstractParentAccountSettings#createdAt() createdAt}.
* @param createdAt The value for createdAt
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setCreatedAt(Instant createdAt) {
this.createdAt = Objects.requireNonNull(createdAt, "createdAt");
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#modifiedAt() modifiedAt} attribute.
*
If not set, this attribute will have a default value returned by the initializer of {@link ParentAccountSettings.AbstractParentAccountSettings#modifiedAt() modifiedAt}.
* @param modifiedAt The value for modifiedAt
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setModifiedAt(Instant modifiedAt) {
this.modifiedAt = Objects.requireNonNull(modifiedAt, "modifiedAt");
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#linkType() linkType} attribute.
* @param linkType The value for linkType
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setLinkType(LinkType linkType) {
this.linkType = Objects.requireNonNull(linkType, "linkType");
initBits &= ~INIT_BIT_LINK_TYPE;
return this;
}
/**
* Assigns a value to the {@link ParentAccountSettings.AbstractParentAccountSettings#balanceSettings() balanceSettings} attribute.
*
If not set, this attribute will have a default value returned by the initializer of {@link ParentAccountSettings.AbstractParentAccountSettings#balanceSettings() balanceSettings}.
* @param balanceSettings The value for balanceSettings
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setBalanceSettings(AccountBalanceSettings balanceSettings) {
this.balanceSettings = Objects.requireNonNull(balanceSettings, "balanceSettings");
return this;
}
/**
* Assigns a present value for the optional {@link ParentAccountSettings.AbstractParentAccountSettings#settlementEngineDetails() settlementEngineDetails} attribute.
* @param settlementEngineDetails A value for settlementEngineDetails
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setSettlementEngineDetails(SettlementEngineDetails settlementEngineDetails) {
this.settlementEngineDetails = Optional.of(settlementEngineDetails);
return this;
}
/**
* Assigns an optional value for {@link ParentAccountSettings.AbstractParentAccountSettings#settlementEngineDetails() settlementEngineDetails}.
* @param settlementEngineDetails A value for settlementEngineDetails
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableParentAccountSettings setSettlementEngineDetails(Optional settlementEngineDetails) {
this.settlementEngineDetails = Objects.requireNonNull(settlementEngineDetails, "settlementEngineDetails");
return this;
}
/**
* Returns {@code true} if the required attribute {@link ParentAccountSettings.AbstractParentAccountSettings#assetCode() assetCode} is set.
* @return {@code true} if set
*/
public final boolean assetCodeIsSet() {
return (initBits & INIT_BIT_ASSET_CODE) == 0;
}
/**
* Returns {@code true} if the required attribute {@link ParentAccountSettings.AbstractParentAccountSettings#assetScale() assetScale} is set.
* @return {@code true} if set
*/
public final boolean assetScaleIsSet() {
return (initBits & INIT_BIT_ASSET_SCALE) == 0;
}
/**
* Returns {@code true} if the required attribute {@link ParentAccountSettings.AbstractParentAccountSettings#accountId() accountId} is set.
* @return {@code true} if set
*/
public final boolean accountIdIsSet() {
return (initBits & INIT_BIT_ACCOUNT_ID) == 0;
}
/**
* Returns {@code true} if the required attribute {@link ParentAccountSettings.AbstractParentAccountSettings#linkType() linkType} is set.
* @return {@code true} if set
*/
public final boolean linkTypeIsSet() {
return (initBits & INIT_BIT_LINK_TYPE) == 0;
}
/**
* Returns {@code true} if the default attribute {@link ParentAccountSettings.AbstractParentAccountSettings#createdAt() createdAt} is set.
* @return {@code true} if set
*/
public final boolean createdAtIsSet() {
return createdAt != null;
}
/**
* Returns {@code true} if the default attribute {@link ParentAccountSettings.AbstractParentAccountSettings#modifiedAt() modifiedAt} is set.
* @return {@code true} if set
*/
public final boolean modifiedAtIsSet() {
return modifiedAt != null;
}
/**
* Returns {@code true} if the default attribute {@link ParentAccountSettings.AbstractParentAccountSettings#balanceSettings() balanceSettings} is set.
* @return {@code true} if set
*/
public final boolean balanceSettingsIsSet() {
return balanceSettings != null;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableParentAccountSettings unsetAssetCode() {
initBits |= INIT_BIT_ASSET_CODE;
assetCode = null;
return this;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableParentAccountSettings unsetAssetScale() {
initBits |= INIT_BIT_ASSET_SCALE;
assetScale = 0;
return this;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableParentAccountSettings unsetAccountId() {
initBits |= INIT_BIT_ACCOUNT_ID;
accountId = null;
return this;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableParentAccountSettings unsetLinkType() {
initBits |= INIT_BIT_LINK_TYPE;
linkType = null;
return this;
}
/**
* Returns {@code true} if all required attributes are set, indicating that the object is initialized.
* @return {@code true} if set
*/
public final boolean isInitialized() {
return initBits == 0;
}
private void checkRequiredAttributes() {
if (!isInitialized()) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!assetCodeIsSet()) attributes.add("assetCode");
if (!assetScaleIsSet()) attributes.add("assetScale");
if (!accountIdIsSet()) attributes.add("accountId");
if (!linkTypeIsSet()) attributes.add("linkType");
return "ParentAccountSettings is not initialized, some of the required attributes are not set " + attributes;
}
/**
* Converts to {@link ImmutableParentAccountSettings ImmutableParentAccountSettings}.
* @return An immutable instance of ParentAccountSettings
*/
public final ImmutableParentAccountSettings toImmutable() {
checkRequiredAttributes();
return ImmutableParentAccountSettings.copyOf(this);
}
/**
* This instance is equal to all instances of {@code ModifiableParentAccountSettings} that have equal attribute values.
* An uninitialized instance is equal only to itself.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
if (!(another instanceof ModifiableParentAccountSettings)) return false;
ModifiableParentAccountSettings other = (ModifiableParentAccountSettings) another;
if (!isInitialized() || !other.isInitialized()) {
return false;
}
return equalTo(other);
}
private boolean equalTo(ModifiableParentAccountSettings another) {
return super.equals(another);
}
public final int hashCode() {
if (!isInitialized()) return 329984524;
return super.hashCode();
}
/**
* Generates a string representation of this {@code AbstractParentAccountSettings}.
* If uninitialized, some attribute values may appear as question marks.
* @return A string representation
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ModifiableParentAccountSettings")
.add("assetCode", assetCodeIsSet() ? assetCode() : "?")
.add("assetScale", assetScaleIsSet() ? assetScale() : "?")
.add("maximumPacketAmount", maximumPacketAmount())
.add("customSettings", customSettings())
.add("accountId", accountIdIsSet() ? accountId() : "?")
.add("createdAt", createdAt())
.add("modifiedAt", modifiedAt())
.add("linkType", linkTypeIsSet() ? linkType() : "?")
.add("balanceSettings", balanceSettings())
.add("settlementEngineDetails", settlementEngineDetails())
.toString();
}
}