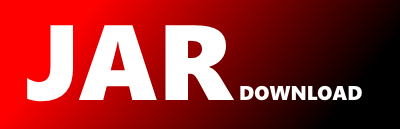
org.interledger.stream.SendMoneyRequestBuilder Maven / Gradle / Ivy
Show all versions of stream-core Show documentation
package org.interledger.stream;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.UnsignedLong;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.interledger.core.InterledgerAddress;
import org.interledger.core.SharedSecret;
/**
* Builds instances of type {@link SendMoneyRequest SendMoneyRequest}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code SendMoneyRequestBuilder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SendMoneyRequest", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@NotThreadSafe
public final class SendMoneyRequestBuilder {
private static final long INIT_BIT_SHARED_SECRET = 0x1L;
private static final long INIT_BIT_SOURCE_ADDRESS = 0x2L;
private static final long INIT_BIT_DESTINATION_ADDRESS = 0x4L;
private static final long INIT_BIT_AMOUNT = 0x8L;
private static final long INIT_BIT_SENDER_AMOUNT_MODE = 0x10L;
private static final long INIT_BIT_DENOMINATION = 0x20L;
private static final long INIT_BIT_PAYMENT_TRACKER = 0x40L;
private long initBits = 0x7fL;
private @Nullable SharedSecret sharedSecret;
private @Nullable InterledgerAddress sourceAddress;
private @Nullable InterledgerAddress destinationAddress;
private @Nullable UnsignedLong amount;
private @Nullable SenderAmountMode senderAmountMode;
private @Nullable Denomination denomination;
private @Nullable Duration timeout;
private @Nullable PaymentTracker paymentTracker;
/**
* Creates a builder for {@link SendMoneyRequest SendMoneyRequest} instances.
*
* new SendMoneyRequestBuilder()
* .sharedSecret(org.interledger.core.SharedSecret) // required {@link SendMoneyRequest#sharedSecret() sharedSecret}
* .sourceAddress(org.interledger.core.InterledgerAddress) // required {@link SendMoneyRequest#sourceAddress() sourceAddress}
* .destinationAddress(org.interledger.core.InterledgerAddress) // required {@link SendMoneyRequest#destinationAddress() destinationAddress}
* .amount(com.google.common.primitives.UnsignedLong) // required {@link SendMoneyRequest#amount() amount}
* .senderAmountMode(org.interledger.stream.SenderAmountMode) // required {@link SendMoneyRequest#getSenderAmountMode() senderAmountMode}
* .denomination(org.interledger.stream.Denomination) // required {@link SendMoneyRequest#denomination() denomination}
* .timeout(java.time.Duration) // optional {@link SendMoneyRequest#timeout() timeout}
* .paymentTracker(org.interledger.stream.PaymentTracker<org.interledger.stream.SenderAmountMode>) // required {@link SendMoneyRequest#paymentTracker() paymentTracker}
* .build();
*
*/
public SendMoneyRequestBuilder() {
}
/**
* Fill a builder with attribute values from the provided {@code SendMoneyRequest} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder from(SendMoneyRequest instance) {
Objects.requireNonNull(instance, "instance");
sharedSecret(instance.sharedSecret());
sourceAddress(instance.sourceAddress());
destinationAddress(instance.destinationAddress());
amount(instance.amount());
senderAmountMode(instance.getSenderAmountMode());
denomination(instance.denomination());
Optional timeoutOptional = instance.timeout();
if (timeoutOptional.isPresent()) {
timeout(timeoutOptional);
}
paymentTracker(instance.paymentTracker());
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#sharedSecret() sharedSecret} attribute.
* @param sharedSecret The value for sharedSecret
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder sharedSecret(SharedSecret sharedSecret) {
this.sharedSecret = Objects.requireNonNull(sharedSecret, "sharedSecret");
initBits &= ~INIT_BIT_SHARED_SECRET;
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#sourceAddress() sourceAddress} attribute.
* @param sourceAddress The value for sourceAddress
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder sourceAddress(InterledgerAddress sourceAddress) {
this.sourceAddress = Objects.requireNonNull(sourceAddress, "sourceAddress");
initBits &= ~INIT_BIT_SOURCE_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#destinationAddress() destinationAddress} attribute.
* @param destinationAddress The value for destinationAddress
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder destinationAddress(InterledgerAddress destinationAddress) {
this.destinationAddress = Objects.requireNonNull(destinationAddress, "destinationAddress");
initBits &= ~INIT_BIT_DESTINATION_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#amount() amount} attribute.
* @param amount The value for amount
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder amount(UnsignedLong amount) {
this.amount = Objects.requireNonNull(amount, "amount");
initBits &= ~INIT_BIT_AMOUNT;
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#getSenderAmountMode() senderAmountMode} attribute.
* @param senderAmountMode The value for senderAmountMode
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder senderAmountMode(SenderAmountMode senderAmountMode) {
this.senderAmountMode = Objects.requireNonNull(senderAmountMode, "senderAmountMode");
initBits &= ~INIT_BIT_SENDER_AMOUNT_MODE;
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#denomination() denomination} attribute.
* @param denomination The value for denomination
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder denomination(Denomination denomination) {
this.denomination = Objects.requireNonNull(denomination, "denomination");
initBits &= ~INIT_BIT_DENOMINATION;
return this;
}
/**
* Initializes the optional value {@link SendMoneyRequest#timeout() timeout} to timeout.
* @param timeout The value for timeout
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder timeout(Duration timeout) {
this.timeout = Objects.requireNonNull(timeout, "timeout");
return this;
}
/**
* Initializes the optional value {@link SendMoneyRequest#timeout() timeout} to timeout.
* @param timeout The value for timeout
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder timeout(Optional extends Duration> timeout) {
this.timeout = timeout.orElse(null);
return this;
}
/**
* Initializes the value for the {@link SendMoneyRequest#paymentTracker() paymentTracker} attribute.
* @param paymentTracker The value for paymentTracker
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final SendMoneyRequestBuilder paymentTracker(PaymentTracker paymentTracker) {
this.paymentTracker = Objects.requireNonNull(paymentTracker, "paymentTracker");
initBits &= ~INIT_BIT_PAYMENT_TRACKER;
return this;
}
/**
* Builds a new {@link SendMoneyRequest SendMoneyRequest}.
* @return An immutable instance of SendMoneyRequest
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public SendMoneyRequest build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return SendMoneyRequestBuilder.ImmutableSendMoneyRequest.validate(new SendMoneyRequestBuilder.ImmutableSendMoneyRequest(this));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_SHARED_SECRET) != 0) attributes.add("sharedSecret");
if ((initBits & INIT_BIT_SOURCE_ADDRESS) != 0) attributes.add("sourceAddress");
if ((initBits & INIT_BIT_DESTINATION_ADDRESS) != 0) attributes.add("destinationAddress");
if ((initBits & INIT_BIT_AMOUNT) != 0) attributes.add("amount");
if ((initBits & INIT_BIT_SENDER_AMOUNT_MODE) != 0) attributes.add("senderAmountMode");
if ((initBits & INIT_BIT_DENOMINATION) != 0) attributes.add("denomination");
if ((initBits & INIT_BIT_PAYMENT_TRACKER) != 0) attributes.add("paymentTracker");
return "Cannot build SendMoneyRequest, some of required attributes are not set " + attributes;
}
/**
* Immutable implementation of {@link SendMoneyRequest}.
*
* Use the builder to create immutable instances:
* {@code new SendMoneyRequestBuilder()}.
*/
@Generated(from = "SendMoneyRequest", generator = "Immutables")
@Immutable
@CheckReturnValue
private static final class ImmutableSendMoneyRequest implements SendMoneyRequest {
private final SharedSecret sharedSecret;
private final InterledgerAddress sourceAddress;
private final InterledgerAddress destinationAddress;
private final UnsignedLong amount;
private final SenderAmountMode senderAmountMode;
private final Denomination denomination;
private final @Nullable Duration timeout;
private final PaymentTracker paymentTracker;
private ImmutableSendMoneyRequest(SendMoneyRequestBuilder builder) {
this.sharedSecret = builder.sharedSecret;
this.sourceAddress = builder.sourceAddress;
this.destinationAddress = builder.destinationAddress;
this.amount = builder.amount;
this.senderAmountMode = builder.senderAmountMode;
this.denomination = builder.denomination;
this.timeout = builder.timeout;
this.paymentTracker = builder.paymentTracker;
}
/**
* @return The value of the {@code sharedSecret} attribute
*/
@Override
public SharedSecret sharedSecret() {
return sharedSecret;
}
/**
* @return The value of the {@code sourceAddress} attribute
*/
@Override
public InterledgerAddress sourceAddress() {
return sourceAddress;
}
/**
* @return The value of the {@code destinationAddress} attribute
*/
@Override
public InterledgerAddress destinationAddress() {
return destinationAddress;
}
/**
* @return The value of the {@code amount} attribute
*/
@Override
public UnsignedLong amount() {
return amount;
}
/**
* @return The value of the {@code senderAmountMode} attribute
*/
@Override
public SenderAmountMode getSenderAmountMode() {
return senderAmountMode;
}
/**
* @return The value of the {@code denomination} attribute
*/
@Override
public Denomination denomination() {
return denomination;
}
/**
* @return The value of the {@code timeout} attribute
*/
@Override
public Optional timeout() {
return Optional.ofNullable(timeout);
}
/**
* @return The value of the {@code paymentTracker} attribute
*/
@Override
public PaymentTracker paymentTracker() {
return paymentTracker;
}
/**
* This instance is equal to all instances of {@code ImmutableSendMoneyRequest} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SendMoneyRequestBuilder.ImmutableSendMoneyRequest
&& equalTo((SendMoneyRequestBuilder.ImmutableSendMoneyRequest) another);
}
private boolean equalTo(SendMoneyRequestBuilder.ImmutableSendMoneyRequest another) {
return sharedSecret.equals(another.sharedSecret)
&& sourceAddress.equals(another.sourceAddress)
&& destinationAddress.equals(another.destinationAddress)
&& amount.equals(another.amount)
&& senderAmountMode.equals(another.senderAmountMode)
&& denomination.equals(another.denomination)
&& Objects.equals(timeout, another.timeout)
&& paymentTracker.equals(another.paymentTracker);
}
/**
* Computes a hash code from attributes: {@code sharedSecret}, {@code sourceAddress}, {@code destinationAddress}, {@code amount}, {@code senderAmountMode}, {@code denomination}, {@code timeout}, {@code paymentTracker}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + sharedSecret.hashCode();
h += (h << 5) + sourceAddress.hashCode();
h += (h << 5) + destinationAddress.hashCode();
h += (h << 5) + amount.hashCode();
h += (h << 5) + senderAmountMode.hashCode();
h += (h << 5) + denomination.hashCode();
h += (h << 5) + Objects.hashCode(timeout);
h += (h << 5) + paymentTracker.hashCode();
return h;
}
/**
* Prints the immutable value {@code SendMoneyRequest} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("SendMoneyRequest")
.omitNullValues()
.add("sharedSecret", sharedSecret)
.add("sourceAddress", sourceAddress)
.add("destinationAddress", destinationAddress)
.add("amount", amount)
.add("senderAmountMode", senderAmountMode)
.add("denomination", denomination)
.add("timeout", timeout)
.add("paymentTracker", paymentTracker)
.toString();
}
private static SendMoneyRequestBuilder.ImmutableSendMoneyRequest validate(SendMoneyRequestBuilder.ImmutableSendMoneyRequest instance) {
instance = (SendMoneyRequestBuilder.ImmutableSendMoneyRequest) instance.check();
return instance;
}
}
}