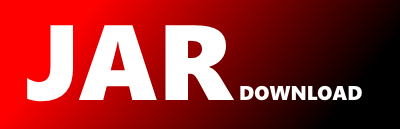
org.interledger.stream.StreamConnectionId Maven / Gradle / Ivy
Show all versions of stream-core Show documentation
package org.interledger.stream;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Ids._StreamConnectionId}.
*
* Use the static factory method to create immutable instances:
* {@code StreamConnectionId.of()}.
*/
@Generated(from = "Ids._StreamConnectionId", generator = "Immutables")
@SuppressWarnings({"checkstyle:TypeName", "all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class StreamConnectionId extends Ids._StreamConnectionId {
private final String value;
private StreamConnectionId(String value) {
this.value = Objects.requireNonNull(value, "value");
}
/**
* @return The value of the {@code value} attribute
*/
@Override
public String value() {
return value;
}
/**
* Construct a new immutable {@code StreamConnectionId} instance.
* @param value The value for the {@code value} attribute
* @return An immutable StreamConnectionId instance
*/
public static StreamConnectionId of(String value) {
return new StreamConnectionId(value);
}
}