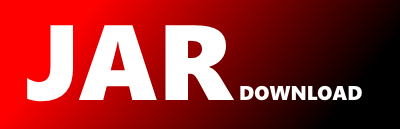
org.intocps.fmichecker.Orch Maven / Gradle / Ivy
package org.intocps.fmichecker;
import java.util.*;
import org.overture.codegen.runtime.*;
@SuppressWarnings("all")
final public class Orch
{
private Orch()
{
}
public static Tuple TypeOk( final Type ty)
{
final Type recordPattern_1 = Utils.copy(ty);
Boolean success_1 = true;
Object t = null;
Object s = null;
t = recordPattern_1.type;
s = recordPattern_1.startValue;
if ( !(success_1) )
{
throw new RuntimeException("Record pattern match failed");
}
Tuple ternaryIfExp_1 = null;
Boolean orResult_1 = false;
if ( Utils.equals(s, null) )
{
orResult_1 = true;
}
else
{
Boolean orResult_2 = false;
Boolean andResult_1 = false;
if ( Utils.equals(t, org.intocps.fmichecker.quotes.RealQuote.getInstance()) )
{
if ( Utils.is_real(s) )
{
andResult_1 = true;
}
}
if ( andResult_1 )
{
orResult_2 = true;
}
else
{
Boolean orResult_3 = false;
Boolean andResult_2 = false;
if ( Utils.equals(t, org.intocps.fmichecker.quotes.IntegerQuote.getInstance()) )
{
if ( Utils.is_int(s) )
{
andResult_2 = true;
}
}
if ( andResult_2 )
{
orResult_3 = true;
}
else
{
Boolean orResult_4 = false;
Boolean andResult_3 = false;
if ( Utils.equals(t, org.intocps.fmichecker.quotes.BooleanQuote.getInstance()) )
{
Boolean orResult_5 = false;
if ( Utils.is_bool(s) )
{
orResult_5 = true;
}
else
{
Boolean orResult_6 = false;
if ( Utils.equals(s, 0L) )
{
orResult_6 = true;
}
else
{
orResult_6 = Utils.equals(s, 1L);
}
orResult_5 = orResult_6;
}
if ( orResult_5 )
{
andResult_3 = true;
}
}
if ( andResult_3 )
{
orResult_4 = true;
}
else
{
Boolean orResult_7 = false;
Boolean andResult_4 = false;
if ( Utils.equals(t, org.intocps.fmichecker.quotes.StringQuote.getInstance()) )
{
Boolean orResult_8 = false;
if ( Utils.is_(s, String.class)
)
{
orResult_8 = true;
}
else
{
orResult_8 = Utils.equals( ((VDMSeq) s).size(), 0L);
}
if ( orResult_8 )
{
andResult_4 = true;
}
}
if ( andResult_4 )
{
orResult_7 = true;
}
else
{
Boolean andResult_5 = false;
if ( Utils.equals(t, org.intocps.fmichecker.quotes.EnumerationQuote.getInstance()) )
{
if ( Utils.is_int(s) )
{
andResult_5 = true;
}
}
orResult_7 = andResult_5;
}
orResult_4 = orResult_7;
}
orResult_3 = orResult_4;
}
orResult_2 = orResult_3;
}
orResult_1 = orResult_2;
}
if ( orResult_1 )
{
ternaryIfExp_1 = Tuple.mk_(true, "Combination OK");
}
else
{
ternaryIfExp_1 = Tuple.mk_(false, "Type and startvalue incompatible. Got: type " + VDMUtil.val2seq_of_char( ((Object) t))
+ " and start value "
+ VDMUtil.val2seq_of_char( ((Object) s))
);
}
return Utils.copy(ternaryIfExp_1);
}
public static Object CalcInitial( final Object c, final Object v)
{
Object casesExpResult_1 = null;
Object quotePattern_1 = c;
Boolean success_2 = Utils.equals(quotePattern_1, org.intocps.fmichecker.quotes.parameterQuote.getInstance());
if ( !(success_2) )
{
Object quotePattern_2 = c;
success_2 = Utils.equals(quotePattern_2, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance());
if ( !(success_2) )
{
Object quotePattern_3 = c;
success_2 = Utils.equals(quotePattern_3, org.intocps.fmichecker.quotes.inputQuote.getInstance());
if ( !(success_2) )
{
Object quotePattern_4 = c;
success_2 = Utils.equals(quotePattern_4, org.intocps.fmichecker.quotes.outputQuote.getInstance());
if ( !(success_2) )
{
Object quotePattern_5 = c;
success_2 = Utils.equals(quotePattern_5, org.intocps.fmichecker.quotes.localQuote.getInstance());
if ( !(success_2) )
{
Object quotePattern_6 = c;
success_2 = Utils.equals(quotePattern_6, org.intocps.fmichecker.quotes.independentQuote.getInstance());
if ( success_2 )
{
casesExpResult_1 = null;
}
}
else
{
Object ternaryIfExp_6 = null;
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
ternaryIfExp_6 = org.intocps.fmichecker.quotes.exactQuote.getInstance();
}
else
{
Object ternaryIfExp_7 = null;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
ternaryIfExp_7 = org.intocps.fmichecker.quotes.calculatedQuote.getInstance();
}
else
{
ternaryIfExp_7 = null;
}
ternaryIfExp_6 = ternaryIfExp_7;
}
casesExpResult_1 = ternaryIfExp_6;
}
}
else
{
Object ternaryIfExp_4 = null;
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
ternaryIfExp_4 = org.intocps.fmichecker.quotes.exactQuote.getInstance();
}
else
{
Object ternaryIfExp_5 = null;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
ternaryIfExp_5 = org.intocps.fmichecker.quotes.calculatedQuote.getInstance();
}
else
{
ternaryIfExp_5 = null;
}
ternaryIfExp_4 = ternaryIfExp_5;
}
casesExpResult_1 = ternaryIfExp_4;
}
}
else
{
casesExpResult_1 = null;
}
}
else
{
Object ternaryIfExp_3 = null;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance())) )
{
ternaryIfExp_3 = org.intocps.fmichecker.quotes.calculatedQuote.getInstance();
}
else
{
ternaryIfExp_3 = null;
}
casesExpResult_1 = ternaryIfExp_3;
}
}
else
{
Object ternaryIfExp_2 = null;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance())) )
{
ternaryIfExp_2 = org.intocps.fmichecker.quotes.exactQuote.getInstance();
}
else
{
ternaryIfExp_2 = null;
}
casesExpResult_1 = ternaryIfExp_2;
}
return casesExpResult_1;
}
public static SV_X_ InitSV( final SV sv)
{
final SV recordPattern_2 = Utils.copy(sv);
Boolean success_3 = true;
Object c = null;
Object v = null;
Object i = null;
Type t = null;
c = recordPattern_2.causality;
v = recordPattern_2.variability;
i = recordPattern_2.initial;
t = Utils.copy(recordPattern_2.type);
if ( !(success_3) )
{
throw new RuntimeException("Record pattern match failed");
}
Object ternaryIfExp_10 = null;
if ( Utils.equals(c, null) )
{
ternaryIfExp_10 = org.intocps.fmichecker.quotes.localQuote.getInstance();
}
else
{
ternaryIfExp_10 = c;
}
final Object c_X_ = ternaryIfExp_10;
Object ternaryIfExp_11 = null;
if ( Utils.equals(v, null) )
{
ternaryIfExp_11 = org.intocps.fmichecker.quotes.continuousQuote.getInstance();
}
else
{
ternaryIfExp_11 = v;
}
final Object v_X_ = ternaryIfExp_11;
Object ternaryIfExp_12 = null;
if ( Utils.equals(i, null) )
{
ternaryIfExp_12 = CalcInitial( ((Object) c_X_), ((Object) v_X_));
}
else
{
ternaryIfExp_12 = i;
}
final Object i_X_ = ternaryIfExp_12;
return new SV_X_( ((Object) c_X_), ((Object) v_X_), ((Object) i_X_), t);
}
public static Tuple TypeVariability( final Object v, final Type t)
{
Boolean orResult_12 = false;
if ( !(Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
orResult_12 = true;
}
else
{
orResult_12 = Utils.equals( t.type, org.intocps.fmichecker.quotes.RealQuote.getInstance());
}
if ( orResult_12 )
{
return Tuple.mk_(true, "Combination OK");
}
else
{
return Tuple.mk_(false, "For a continuous variable the type has to be Real");
}
}
public static String ValidSettingsToStr( final Object c, final Object v)
{
Boolean andResult_9 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.parameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_9 = true;
}
}
if ( andResult_9 )
{
return "fixed parameter";
}
else
{
Boolean andResult_10 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.parameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_10 = true;
}
}
if ( andResult_10 )
{
return "tunable parameter";
}
else
{
Boolean andResult_11 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_11 = true;
}
}
if ( andResult_11 )
{
return "fixed dependent parameter";
}
else
{
Boolean andResult_12 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_12 = true;
}
}
if ( andResult_12 )
{
return "tunable dependent parameter";
}
else
{
Boolean andResult_13 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_13 = true;
}
}
if ( andResult_13 )
{
return "discrete input";
}
else
{
Boolean andResult_14 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_14 = true;
}
}
if ( andResult_14 )
{
return "continuous input";
}
else
{
Boolean andResult_15 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
andResult_15 = true;
}
}
if ( andResult_15 )
{
return "constant output";
}
else
{
Boolean andResult_16 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_16 = true;
}
}
if ( andResult_16 )
{
return "discrete output";
}
else
{
Boolean andResult_17 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_17 = true;
}
}
if ( andResult_17 )
{
return "continuous output";
}
else
{
Boolean andResult_18 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
andResult_18 = true;
}
}
if ( andResult_18 )
{
return "constant local";
}
else
{
Boolean andResult_19 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_19 = true;
}
}
if ( andResult_19 )
{
return "fixed local";
}
else
{
Boolean andResult_20 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_20 = true;
}
}
if ( andResult_20 )
{
return "tunable local";
}
else
{
Boolean andResult_21 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_21 = true;
}
}
if ( andResult_21 )
{
return "discrete local";
}
else
{
Boolean andResult_22 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_22 = true;
}
}
if ( andResult_22 )
{
return "continuous local";
}
else
{
Boolean andResult_23 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.independentQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_23 = true;
}
}
if ( andResult_23 )
{
return "continuous independent";
}
else
{
return null;
}
}
}
}
}
}
}
}
}
}
}
}
}
}
}
}
public static Tuple CausalityVariabilityOk( final Object c, final Object v)
{
Boolean andResult_24 = false;
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.parameterQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance(), org.intocps.fmichecker.quotes.inputQuote.getInstance())) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
andResult_24 = true;
}
}
if ( andResult_24 )
{
return Tuple.mk_(false, ErrorMsg( ((Object) c), ((Object) v)));
}
else
{
Boolean andResult_25 = false;
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.parameterQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance())) )
{
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
andResult_25 = true;
}
}
if ( andResult_25 )
{
return Tuple.mk_(false, ErrorMsg( ((Object) c), ((Object) v)));
}
else
{
Boolean andResult_26 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.independentQuote.getInstance()) )
{
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.constantQuote.getInstance(), org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance(), org.intocps.fmichecker.quotes.discreteQuote.getInstance())) )
{
andResult_26 = true;
}
}
if ( andResult_26 )
{
return Tuple.mk_(false, ErrorMsg( ((Object) c), ((Object) v)));
}
else
{
Boolean andResult_27 = false;
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.inputQuote.getInstance(), org.intocps.fmichecker.quotes.outputQuote.getInstance())) )
{
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance())) )
{
andResult_27 = true;
}
}
if ( andResult_27 )
{
return Tuple.mk_(false, ErrorMsg( ((Object) c), ((Object) v)));
}
else
{
return Tuple.mk_(true, "Valid combination of Causality and Variability.");
}
}
}
}
}
public static Boolean ValidCV( final Object c, final Object v)
{
Boolean orResult_14 = false;
Boolean andResult_28 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.parameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_28 = true;
}
}
if ( andResult_28 )
{
orResult_14 = true;
}
else
{
Boolean orResult_15 = false;
Boolean andResult_29 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.parameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_29 = true;
}
}
if ( andResult_29 )
{
orResult_15 = true;
}
else
{
Boolean orResult_16 = false;
Boolean andResult_30 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_30 = true;
}
}
if ( andResult_30 )
{
orResult_16 = true;
}
else
{
Boolean orResult_17 = false;
Boolean andResult_31 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_31 = true;
}
}
if ( andResult_31 )
{
orResult_17 = true;
}
else
{
Boolean orResult_18 = false;
Boolean andResult_32 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_32 = true;
}
}
if ( andResult_32 )
{
orResult_18 = true;
}
else
{
Boolean orResult_19 = false;
Boolean andResult_33 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_33 = true;
}
}
if ( andResult_33 )
{
orResult_19 = true;
}
else
{
Boolean orResult_20 = false;
Boolean andResult_34 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
andResult_34 = true;
}
}
if ( andResult_34 )
{
orResult_20 = true;
}
else
{
Boolean orResult_21 = false;
Boolean andResult_35 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_35 = true;
}
}
if ( andResult_35 )
{
orResult_21 = true;
}
else
{
Boolean orResult_22 = false;
Boolean andResult_36 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.outputQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_36 = true;
}
}
if ( andResult_36 )
{
orResult_22 = true;
}
else
{
Boolean orResult_23 = false;
Boolean andResult_37 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
andResult_37 = true;
}
}
if ( andResult_37 )
{
orResult_23 = true;
}
else
{
Boolean orResult_24 = false;
Boolean andResult_38 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.fixedQuote.getInstance()) )
{
andResult_38 = true;
}
}
if ( andResult_38 )
{
orResult_24 = true;
}
else
{
Boolean orResult_25 = false;
Boolean andResult_39 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.tunableQuote.getInstance()) )
{
andResult_39 = true;
}
}
if ( andResult_39 )
{
orResult_25 = true;
}
else
{
Boolean orResult_26 = false;
Boolean andResult_40 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.discreteQuote.getInstance()) )
{
andResult_40 = true;
}
}
if ( andResult_40 )
{
orResult_26 = true;
}
else
{
Boolean orResult_27 = false;
Boolean andResult_41 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.localQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_41 = true;
}
}
if ( andResult_41 )
{
orResult_27 = true;
}
else
{
Boolean andResult_42 = false;
if ( Utils.equals(c, org.intocps.fmichecker.quotes.independentQuote.getInstance()) )
{
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
andResult_42 = true;
}
}
orResult_27 = andResult_42;
}
orResult_26 = orResult_27;
}
orResult_25 = orResult_26;
}
orResult_24 = orResult_25;
}
orResult_23 = orResult_24;
}
orResult_22 = orResult_23;
}
orResult_21 = orResult_22;
}
orResult_20 = orResult_21;
}
orResult_19 = orResult_20;
}
orResult_18 = orResult_19;
}
orResult_17 = orResult_18;
}
orResult_16 = orResult_17;
}
orResult_15 = orResult_16;
}
orResult_14 = orResult_15;
}
return orResult_14;
}
public static String ErrorMsg( final Object c, final Object v)
{
return IO.sprintf("Invalid combination of causality and variability. For causality '%s' the expected variabilities are '%s' but got: '%s'", SeqUtil.seq(c, ValidVariabilities( ((Object) c)), v));
}
public static VDMSet ValidVariabilities( final Object c)
{
VDMSet casesExpResult_2 = null;
Object quotePattern_7 = c;
Boolean success_4 = Utils.equals(quotePattern_7, org.intocps.fmichecker.quotes.parameterQuote.getInstance());
if ( !(success_4) )
{
Object quotePattern_8 = c;
success_4 = Utils.equals(quotePattern_8, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance());
if ( !(success_4) )
{
Object quotePattern_9 = c;
success_4 = Utils.equals(quotePattern_9, org.intocps.fmichecker.quotes.inputQuote.getInstance());
if ( !(success_4) )
{
Object quotePattern_10 = c;
success_4 = Utils.equals(quotePattern_10, org.intocps.fmichecker.quotes.outputQuote.getInstance());
if ( !(success_4) )
{
Object quotePattern_11 = c;
success_4 = Utils.equals(quotePattern_11, org.intocps.fmichecker.quotes.localQuote.getInstance());
if ( !(success_4) )
{
Object quotePattern_12 = c;
success_4 = Utils.equals(quotePattern_12, org.intocps.fmichecker.quotes.independentQuote.getInstance());
if ( success_4 )
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.continuousQuote.getInstance());
}
}
else
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.constantQuote.getInstance(), org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance());
}
}
else
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance(), org.intocps.fmichecker.quotes.constantQuote.getInstance());
}
}
else
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance());
}
}
else
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance());
}
}
else
{
casesExpResult_2 = SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance());
}
return Utils.copy(casesExpResult_2);
}
public static Tuple InitialOk( final Object c, final Object v, final Object i)
{
final String EXPECT_SPECIFIC_VAL = "An invalid value of Initial. Actual: %s, but expected: %s " + "for the combination of causality: %s and variability: %s"
;
final String EXPECT_INITIAL_NOT_SET = "An invalid value of Initial. Actual: %s, but expected " + "inital not to be set for the combination of causality: %s and variability: %s"
;
final String INITIAL_NOT_DEFINED = "An invalid value of Initial. Actual: Initial not defined, " + "but expected: %s for the combination of causality: %s and variability: %s"
;
final String COMB_OK = "Initial OK";
Tuple casesExpResult_3 = null;
Object quotePattern_13 = c;
Boolean success_5 = Utils.equals(quotePattern_13, org.intocps.fmichecker.quotes.parameterQuote.getInstance());
if ( !(success_5) )
{
Object quotePattern_14 = c;
success_5 = Utils.equals(quotePattern_14, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance());
if ( !(success_5) )
{
Object quotePattern_15 = c;
success_5 = Utils.equals(quotePattern_15, org.intocps.fmichecker.quotes.inputQuote.getInstance());
if ( !(success_5) )
{
Object quotePattern_16 = c;
success_5 = Utils.equals(quotePattern_16, org.intocps.fmichecker.quotes.outputQuote.getInstance());
if ( !(success_5) )
{
Object quotePattern_17 = c;
success_5 = Utils.equals(quotePattern_17, org.intocps.fmichecker.quotes.localQuote.getInstance());
if ( !(success_5) )
{
Object quotePattern_18 = c;
success_5 = Utils.equals(quotePattern_18, org.intocps.fmichecker.quotes.independentQuote.getInstance());
if ( success_5 )
{
casesExpResult_3 = Tuple.mk_(Utils.equals(i, null), IO.sprintf(EXPECT_INITIAL_NOT_SET, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
}
else
{
Tuple ternaryIfExp_15 = null;
Boolean orResult_30 = false;
if ( !(Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance())) )
{
orResult_30 = true;
}
else
{
orResult_30 = Utils.equals(i, org.intocps.fmichecker.quotes.exactQuote.getInstance());
}
if ( !(orResult_30) )
{
ternaryIfExp_15 = Tuple.mk_(false, IO.sprintf(INITIAL_NOT_DEFINED, SeqUtil.seq(i, org.intocps.fmichecker.quotes.exactQuote.getInstance(), c, v)));
}
else
{
Tuple ternaryIfExp_16 = null;
Boolean orResult_31 = false;
if ( !(SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance()))) )
{
orResult_31 = true;
}
else
{
orResult_31 = SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()));
}
if ( !(orResult_31) )
{
ternaryIfExp_16 = Tuple.mk_(false, IO.sprintf(INITIAL_NOT_DEFINED, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
else
{
Tuple ternaryIfExp_17 = null;
Boolean orResult_32 = false;
if ( !(SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance()))) )
{
orResult_32 = true;
}
else
{
orResult_32 = SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()));
}
if ( !(orResult_32) )
{
ternaryIfExp_17 = Tuple.mk_(false, IO.sprintf(INITIAL_NOT_DEFINED, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
else
{
ternaryIfExp_17 = Tuple.mk_(true, COMB_OK);
}
ternaryIfExp_16 = Utils.copy(ternaryIfExp_17);
}
ternaryIfExp_15 = Utils.copy(ternaryIfExp_16);
}
casesExpResult_3 = Utils.copy(ternaryIfExp_15);
}
}
else
{
Tuple ternaryIfExp_13 = null;
Boolean orResult_28 = false;
if ( !(Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance())) )
{
orResult_28 = true;
}
else
{
orResult_28 = Utils.equals(i, org.intocps.fmichecker.quotes.exactQuote.getInstance());
}
if ( !(orResult_28) )
{
ternaryIfExp_13 = Tuple.mk_(false, IO.sprintf(EXPECT_SPECIFIC_VAL, SeqUtil.seq(i, org.intocps.fmichecker.quotes.exactQuote.getInstance(), c, v)));
}
else
{
Tuple ternaryIfExp_14 = null;
Boolean orResult_29 = false;
if ( !(SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.continuousQuote.getInstance(), org.intocps.fmichecker.quotes.discreteQuote.getInstance()))) )
{
orResult_29 = true;
}
else
{
orResult_29 = SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()));
}
if ( !(orResult_29) )
{
ternaryIfExp_14 = Tuple.mk_(false, IO.sprintf(INITIAL_NOT_DEFINED, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
else
{
ternaryIfExp_14 = Tuple.mk_(true, COMB_OK);
}
ternaryIfExp_13 = Utils.copy(ternaryIfExp_14);
}
casesExpResult_3 = Utils.copy(ternaryIfExp_13);
}
}
else
{
casesExpResult_3 = Tuple.mk_(Utils.equals(i, null), IO.sprintf(EXPECT_INITIAL_NOT_SET, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
}
else
{
casesExpResult_3 = Tuple.mk_(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance())), IO.sprintf(EXPECT_SPECIFIC_VAL, SeqUtil.seq(i, SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance()), c, v)));
}
}
else
{
casesExpResult_3 = Tuple.mk_(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance())), IO.sprintf(EXPECT_SPECIFIC_VAL, SeqUtil.seq(i, org.intocps.fmichecker.quotes.exactQuote.getInstance(), c, v)));
}
return Utils.copy(casesExpResult_3);
}
public static Boolean ValidCVI( final Object c, final Object v, final Object i)
{
Boolean orResult_33 = false;
Boolean andResult_43 = false;
if ( Utils.equals(i, org.intocps.fmichecker.quotes.exactQuote.getInstance()) )
{
Boolean orResult_34 = false;
Boolean andResult_44 = false;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance())) )
{
if ( Utils.equals(c, org.intocps.fmichecker.quotes.parameterQuote.getInstance()) )
{
andResult_44 = true;
}
}
if ( andResult_44 )
{
orResult_34 = true;
}
else
{
Boolean andResult_45 = false;
if ( Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance()) )
{
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.outputQuote.getInstance(), org.intocps.fmichecker.quotes.localQuote.getInstance())) )
{
andResult_45 = true;
}
}
orResult_34 = andResult_45;
}
if ( orResult_34 )
{
andResult_43 = true;
}
}
if ( andResult_43 )
{
orResult_33 = true;
}
else
{
Boolean orResult_35 = false;
Boolean andResult_46 = false;
if ( SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance())) )
{
Boolean andResult_47 = false;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance())) )
{
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance(), org.intocps.fmichecker.quotes.localQuote.getInstance())) )
{
andResult_47 = true;
}
}
if ( andResult_47 )
{
andResult_46 = true;
}
}
if ( andResult_46 )
{
orResult_35 = true;
}
else
{
Boolean orResult_36 = false;
Boolean andResult_48 = false;
if ( SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance())) )
{
Boolean andResult_49 = false;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.outputQuote.getInstance(), org.intocps.fmichecker.quotes.localQuote.getInstance())) )
{
andResult_49 = true;
}
}
if ( andResult_49 )
{
andResult_48 = true;
}
}
if ( andResult_48 )
{
orResult_36 = true;
}
else
{
Boolean orResult_37 = false;
Boolean andResult_50 = false;
if ( Utils.equals(i, null) )
{
Boolean andResult_51 = false;
if ( SetUtil.inSet(v,SetUtil.set(org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance())) )
{
if ( Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance()) )
{
andResult_51 = true;
}
}
if ( andResult_51 )
{
andResult_50 = true;
}
}
if ( andResult_50 )
{
orResult_37 = true;
}
else
{
Boolean andResult_52 = false;
if ( Utils.equals(i, null) )
{
Boolean andResult_53 = false;
if ( Utils.equals(v, org.intocps.fmichecker.quotes.continuousQuote.getInstance()) )
{
if ( Utils.equals(c, org.intocps.fmichecker.quotes.independentQuote.getInstance()) )
{
andResult_53 = true;
}
}
if ( andResult_53 )
{
andResult_52 = true;
}
}
orResult_37 = andResult_52;
}
orResult_36 = orResult_37;
}
orResult_35 = orResult_36;
}
orResult_33 = orResult_35;
}
return orResult_33;
}
public static Tuple StartValueOk( final Object c, final Object v, final Object i, final Type t)
{
final Boolean startValReq = true;
final String combOk = "Combination OK";
Tuple casesExpResult_4 = null;
Object quotePattern_19 = c;
Boolean success_6 = Utils.equals(quotePattern_19, org.intocps.fmichecker.quotes.parameterQuote.getInstance());
if ( !(success_6) )
{
Object quotePattern_20 = c;
success_6 = Utils.equals(quotePattern_20, org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance());
if ( !(success_6) )
{
Object quotePattern_21 = c;
success_6 = Utils.equals(quotePattern_21, org.intocps.fmichecker.quotes.inputQuote.getInstance());
if ( !(success_6) )
{
Object quotePattern_22 = c;
success_6 = Utils.equals(quotePattern_22, org.intocps.fmichecker.quotes.outputQuote.getInstance());
if ( !(success_6) )
{
Object quotePattern_23 = c;
success_6 = Utils.equals(quotePattern_23, org.intocps.fmichecker.quotes.localQuote.getInstance());
if ( !(success_6) )
{
Object quotePattern_24 = c;
success_6 = Utils.equals(quotePattern_24, org.intocps.fmichecker.quotes.independentQuote.getInstance());
if ( success_6 )
{
Tuple ternaryIfExp_26 = null;
if ( !(Utils.equals( t.startValue, null)) )
{
ternaryIfExp_26 = Tuple.mk_(false, InvalidStartValueToStr(!(startValReq), ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_26 = Tuple.mk_(true, combOk);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_26);
}
}
else
{
Tuple ternaryIfExp_24 = null;
Boolean orResult_43 = false;
if ( !(Utils.equals(i, org.intocps.fmichecker.quotes.calculatedQuote.getInstance())) )
{
orResult_43 = true;
}
else
{
orResult_43 = Utils.equals( t.startValue, null);
}
if ( !(orResult_43) )
{
ternaryIfExp_24 = Tuple.mk_(false, InvalidStartValueToStr(!(startValReq), ((Object) c), ((Object) v), ((Object) i)));
}
else
{
Tuple ternaryIfExp_25 = null;
Boolean orResult_44 = false;
if ( !(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance()))) )
{
orResult_44 = true;
}
else
{
orResult_44 = !(Utils.equals( t.startValue, null));
}
if ( !(orResult_44) )
{
ternaryIfExp_25 = Tuple.mk_(false, InvalidStartValueToStr(startValReq, ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_25 = Tuple.mk_(true, combOk);
}
ternaryIfExp_24 = Utils.copy(ternaryIfExp_25);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_24);
}
}
else
{
Tuple ternaryIfExp_22 = null;
Boolean orResult_41 = false;
if ( !(Utils.equals(i, org.intocps.fmichecker.quotes.calculatedQuote.getInstance())) )
{
orResult_41 = true;
}
else
{
orResult_41 = Utils.equals( t.startValue, null);
}
if ( !(orResult_41) )
{
ternaryIfExp_22 = Tuple.mk_(false, InvalidStartValueToStr(!(startValReq), ((Object) c), ((Object) v), ((Object) i)));
}
else
{
Tuple ternaryIfExp_23 = null;
Boolean orResult_42 = false;
if ( !(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance()))) )
{
orResult_42 = true;
}
else
{
orResult_42 = !(Utils.equals( t.startValue, null));
}
if ( !(orResult_42) )
{
ternaryIfExp_23 = Tuple.mk_(false, InvalidStartValueToStr(startValReq, ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_23 = Tuple.mk_(true, combOk);
}
ternaryIfExp_22 = Utils.copy(ternaryIfExp_23);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_22);
}
}
else
{
Tuple ternaryIfExp_21 = null;
if ( !(!(Utils.equals( t.startValue, null))) )
{
ternaryIfExp_21 = Tuple.mk_(false, InvalidStartValueToStr(startValReq, ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_21 = Tuple.mk_(true, combOk);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_21);
}
}
else
{
Tuple ternaryIfExp_19 = null;
Boolean orResult_39 = false;
if ( !(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance()))) )
{
orResult_39 = true;
}
else
{
orResult_39 = !(Utils.equals( t.startValue, null));
}
if ( !(orResult_39) )
{
ternaryIfExp_19 = Tuple.mk_(false, InvalidStartValueToStr(startValReq, ((Object) c), ((Object) v), ((Object) i)));
}
else
{
Tuple ternaryIfExp_20 = null;
Boolean orResult_40 = false;
if ( !(Utils.equals(i, org.intocps.fmichecker.quotes.calculatedQuote.getInstance())) )
{
orResult_40 = true;
}
else
{
orResult_40 = Utils.equals( t.startValue, null);
}
if ( !(orResult_40) )
{
ternaryIfExp_20 = Tuple.mk_(false, InvalidStartValueToStr(!(startValReq), ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_20 = Tuple.mk_(true, combOk);
}
ternaryIfExp_19 = Utils.copy(ternaryIfExp_20);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_19);
}
}
else
{
Tuple ternaryIfExp_18 = null;
Boolean orResult_38 = false;
if ( !(SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance()))) )
{
orResult_38 = true;
}
else
{
orResult_38 = !(Utils.equals( t.startValue, null));
}
if ( !(orResult_38) )
{
ternaryIfExp_18 = Tuple.mk_(false, InvalidStartValueToStr(startValReq, ((Object) c), ((Object) v), ((Object) i)));
}
else
{
ternaryIfExp_18 = Tuple.mk_(true, combOk);
}
casesExpResult_4 = Utils.copy(ternaryIfExp_18);
}
return Utils.copy(casesExpResult_4);
}
public static Boolean ValidStart( final Object c, final Object v, final Object i, final Type t)
{
Boolean orResult_45 = false;
if ( SetUtil.inSet(i,SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance())) )
{
orResult_45 = true;
}
else
{
orResult_45 = Utils.equals(c, org.intocps.fmichecker.quotes.inputQuote.getInstance());
}
if ( orResult_45 )
{
return !(Utils.equals( t.startValue, null));
}
else
{
Boolean orResult_46 = false;
if ( Utils.equals(i, org.intocps.fmichecker.quotes.calculatedQuote.getInstance()) )
{
orResult_46 = true;
}
else
{
orResult_46 = Utils.equals(c, org.intocps.fmichecker.quotes.independentQuote.getInstance());
}
if ( orResult_46 )
{
return Utils.equals( t.startValue, null);
}
else
{
Boolean orResult_47 = false;
if ( SetUtil.inSet(c,SetUtil.set(org.intocps.fmichecker.quotes.parameterQuote.getInstance(), org.intocps.fmichecker.quotes.inputQuote.getInstance())) )
{
orResult_47 = true;
}
else
{
orResult_47 = Utils.equals(v, org.intocps.fmichecker.quotes.constantQuote.getInstance());
}
if ( orResult_47 )
{
return !(Utils.equals( t.startValue, null));
}
else
{
return false;
}
}
}
}
public static String InvalidStartValueToStr( final Boolean b, final Object c, final Object v, final Object i)
{
if ( b )
{
return IO.sprintf( "A start value has to be set for a combination of " + "Causality: %s, Variability: %s and Initial: %s"
, SeqUtil.seq(c, v, i));
}
else
{
return IO.sprintf( "A start value is not allowed to be set for a combination " + "of Causality: %s, Variability: %s and Initial: %s"
, SeqUtil.seq(c, v, i));
}
}
public static Tuple Validate( final SV_X_ sv_X_)
{
final Tuple tuplePattern_1 = TypeOk( Utils.copy(sv_X_.type));
Boolean success_7 = tuplePattern_1.compatible(Boolean.class, String.class);
Boolean tc_ok = null;
String tc_msg = null;
if ( success_7 )
{
tc_ok = ((Boolean) tuplePattern_1.get(0)) ;
tc_msg = SeqUtil.toStr( tuplePattern_1.get(1));
}
if ( !(success_7) )
{
throw new RuntimeException("Tuple pattern match failed");
}
Tuple ternaryIfExp_27 = null;
if ( !(tc_ok) )
{
ternaryIfExp_27 = Tuple.mk_(false, tc_msg);
}
else
{
final Tuple tuplePattern_2 = TypeVariability( ((Object) sv_X_.variability), Utils.copy(sv_X_.type));
Boolean success_8 = tuplePattern_2.compatible(Boolean.class, String.class);
Boolean vt_ok = null;
String vt_msg = null;
if ( success_8 )
{
vt_ok = ((Boolean) tuplePattern_2.get(0)) ;
vt_msg = SeqUtil.toStr( tuplePattern_2.get(1));
}
if ( !(success_8) )
{
throw new RuntimeException("Tuple pattern match failed");
}
Tuple ternaryIfExp_28 = null;
if ( !(vt_ok) )
{
ternaryIfExp_28 = Tuple.mk_(false, vt_msg);
}
else
{
final Tuple tuplePattern_3 = CausalityVariabilityOk( ((Object) sv_X_.causality), ((Object) sv_X_.variability));
Boolean success_9 = tuplePattern_3.compatible(Boolean.class, String.class);
Boolean cv_ok = null;
String cv_msg = null;
if ( success_9 )
{
cv_ok = ((Boolean) tuplePattern_3.get(0)) ;
cv_msg = SeqUtil.toStr( tuplePattern_3.get(1));
}
if ( !(success_9) )
{
throw new RuntimeException("Tuple pattern match failed");
}
Tuple ternaryIfExp_29 = null;
if ( !(cv_ok) )
{
ternaryIfExp_29 = Tuple.mk_(false, cv_msg);
}
else
{
final Tuple tuplePattern_4 = InitialOk( ((Object) sv_X_.causality), ((Object) sv_X_.variability), ((Object) sv_X_.initial));
Boolean success_10 = tuplePattern_4.compatible(Boolean.class, String.class);
Boolean ini_ok = null;
String ini_msg = null;
if ( success_10 )
{
ini_ok = ((Boolean) tuplePattern_4.get(0)) ;
ini_msg = SeqUtil.toStr( tuplePattern_4.get(1));
}
if ( !(success_10) )
{
throw new RuntimeException("Tuple pattern match failed");
}
Tuple ternaryIfExp_30 = null;
if ( !(ini_ok) )
{
ternaryIfExp_30 = Tuple.mk_(false, ini_msg);
}
else
{
final Tuple tuplePattern_5 = StartValueOk( ((Object) sv_X_.causality), ((Object) sv_X_.variability), ((Object) sv_X_.initial), Utils.copy(sv_X_.type));
Boolean success_11 = tuplePattern_5.compatible(Boolean.class, String.class);
Boolean start_ok = null;
String start_msg = null;
if ( success_11 )
{
start_ok = ((Boolean) tuplePattern_5.get(0)) ;
start_msg = SeqUtil.toStr( tuplePattern_5.get(1));
}
if ( !(success_11) )
{
throw new RuntimeException("Tuple pattern match failed");
}
Tuple ternaryIfExp_31 = null;
if ( !(start_ok) )
{
ternaryIfExp_31 = Tuple.mk_(false, start_msg);
}
else
{
ternaryIfExp_31 = Tuple.mk_(true, "ScalarVariable is valid");
}
ternaryIfExp_30 = Utils.copy(ternaryIfExp_31);
}
ternaryIfExp_29 = Utils.copy(ternaryIfExp_30);
}
ternaryIfExp_28 = Utils.copy(ternaryIfExp_29);
}
ternaryIfExp_27 = Utils.copy(ternaryIfExp_28);
}
return Utils.copy(ternaryIfExp_27);
}
public static VDMSet allSv()
{
VDMSet setCompResult_1 = SetUtil.set();
VDMSet set_2 = SetUtil.set(org.intocps.fmichecker.quotes.constantQuote.getInstance(), org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance(), null);
for( Iterator iterator_2 = set_2.iterator(); iterator_2.hasNext(); )
{
Object v = ((Object) iterator_2.next());
VDMSet set_3 = SetUtil.set(org.intocps.fmichecker.quotes.parameterQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance(), org.intocps.fmichecker.quotes.inputQuote.getInstance(), org.intocps.fmichecker.quotes.outputQuote.getInstance(), org.intocps.fmichecker.quotes.localQuote.getInstance(), org.intocps.fmichecker.quotes.independentQuote.getInstance(), null);
for( Iterator iterator_3 = set_3.iterator(); iterator_3.hasNext(); )
{
Object c = ((Object) iterator_3.next());
VDMSet set_4 = SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance(), null);
for( Iterator iterator_4 = set_4.iterator(); iterator_4.hasNext(); )
{
Object i = ((Object) iterator_4.next());
VDMSet set_5 = SetUtil.set(org.intocps.fmichecker.quotes.RealQuote.getInstance(), org.intocps.fmichecker.quotes.IntegerQuote.getInstance(), org.intocps.fmichecker.quotes.BooleanQuote.getInstance(), org.intocps.fmichecker.quotes.StringQuote.getInstance(), org.intocps.fmichecker.quotes.EnumerationQuote.getInstance());
for( Iterator iterator_5 = set_5.iterator(); iterator_5.hasNext(); )
{
Object t = ((Object) iterator_5.next());
VDMSet set_6 = SetUtil.set(2L, null);
for( Iterator iterator_6 = set_6.iterator(); iterator_6.hasNext(); )
{
Object s = ((Object) iterator_6.next());
setCompResult_1.add(new SV( ((Object) c), ((Object) v), ((Object) i), new Type( ((Object) t), ((Object) s))));
}
}
}
}
}
return Utils.copy(setCompResult_1);
}
public static VDMSet allC()
{
return SetUtil.set(org.intocps.fmichecker.quotes.parameterQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedParameterQuote.getInstance(), org.intocps.fmichecker.quotes.inputQuote.getInstance(), org.intocps.fmichecker.quotes.outputQuote.getInstance(), org.intocps.fmichecker.quotes.localQuote.getInstance(), org.intocps.fmichecker.quotes.independentQuote.getInstance());
}
public static VDMSet allV()
{
return SetUtil.set(org.intocps.fmichecker.quotes.constantQuote.getInstance(), org.intocps.fmichecker.quotes.discreteQuote.getInstance(), org.intocps.fmichecker.quotes.fixedQuote.getInstance(), org.intocps.fmichecker.quotes.tunableQuote.getInstance(), org.intocps.fmichecker.quotes.continuousQuote.getInstance());
}
public static VDMSet allI()
{
return SetUtil.set(org.intocps.fmichecker.quotes.exactQuote.getInstance(), org.intocps.fmichecker.quotes.approxQuote.getInstance(), org.intocps.fmichecker.quotes.calculatedQuote.getInstance(), null);
}
public static VDMSet allT()
{
VDMSet setCompResult_2 = SetUtil.set();
VDMSet set_7 = SetUtil.set(org.intocps.fmichecker.quotes.RealQuote.getInstance(), org.intocps.fmichecker.quotes.IntegerQuote.getInstance(), org.intocps.fmichecker.quotes.BooleanQuote.getInstance(), org.intocps.fmichecker.quotes.StringQuote.getInstance(), org.intocps.fmichecker.quotes.EnumerationQuote.getInstance());
for( Iterator iterator_7 = set_7.iterator(); iterator_7.hasNext(); )
{
Object t = ((Object) iterator_7.next());
VDMSet set_8 = SetUtil.set(2.2, 2L, true, "INTO", new Token("INTO"), null);
for( Iterator iterator_8 = set_8.iterator(); iterator_8.hasNext(); )
{
Object s = ((Object) iterator_8.next());
setCompResult_2.add(new Type( ((Object) t), ((Object) s)));
}
}
return Utils.copy(setCompResult_2);
}
public static VDMSet allCV()
{
VDMSet setCompResult_3 = SetUtil.set();
VDMSet set_9 = allV();
for( Iterator iterator_9 = set_9.iterator(); iterator_9.hasNext(); )
{
Object v = ((Object) iterator_9.next());
VDMSet set_10 = allC();
for( Iterator iterator_10 = set_10.iterator(); iterator_10.hasNext(); )
{
Object c = ((Object) iterator_10.next());
setCompResult_3.add(Tuple.mk_(c, v));
}
}
return Utils.copy(setCompResult_3);
}
public static VDMSet allCVI()
{
VDMSet setCompResult_4 = SetUtil.set();
VDMSet set_11 = allV();
for( Iterator iterator_11 = set_11.iterator(); iterator_11.hasNext(); )
{
Object v = ((Object) iterator_11.next());
VDMSet set_12 = allC();
for( Iterator iterator_12 = set_12.iterator(); iterator_12.hasNext(); )
{
Object c = ((Object) iterator_12.next());
VDMSet set_13 = allI();
for( Iterator iterator_13 = set_13.iterator(); iterator_13.hasNext(); )
{
Object i = ((Object) iterator_13.next());
setCompResult_4.add(Tuple.mk_(c, v, i));
}
}
}
return Utils.copy(setCompResult_4);
}
public static VDMSet allCVIT()
{
VDMSet setCompResult_5 = SetUtil.set();
VDMSet set_14 = allV();
for( Iterator iterator_14 = set_14.iterator(); iterator_14.hasNext(); )
{
Object v = ((Object) iterator_14.next());
VDMSet set_15 = allC();
for( Iterator iterator_15 = set_15.iterator(); iterator_15.hasNext(); )
{
Object c = ((Object) iterator_15.next());
VDMSet set_16 = allI();
for( Iterator iterator_16 = set_16.iterator(); iterator_16.hasNext(); )
{
Object i = ((Object) iterator_16.next());
VDMSet set_17 = allT();
for( Iterator iterator_17 = set_17.iterator(); iterator_17.hasNext(); )
{
Type t = ((Type) iterator_17.next());
setCompResult_5.add(Tuple.mk_(c, v, i, Utils.copy(t)));
}
}
}
}
return Utils.copy(setCompResult_5);
}
public static VDMSet allSV_X_()
{
VDMSet setCompResult_6 = SetUtil.set();
VDMSet set_18 = allC();
for( Iterator iterator_18 = set_18.iterator(); iterator_18.hasNext(); )
{
Object c = ((Object) iterator_18.next());
VDMSet set_19 = allV();
for( Iterator iterator_19 = set_19.iterator(); iterator_19.hasNext(); )
{
Object v = ((Object) iterator_19.next());
VDMSet set_20 = allI();
for( Iterator iterator_20 = set_20.iterator(); iterator_20.hasNext(); )
{
Object i = ((Object) iterator_20.next());
VDMSet set_21 = allT();
for( Iterator iterator_21 = set_21.iterator(); iterator_21.hasNext(); )
{
Type t = ((Type) iterator_21.next());
setCompResult_6.add(new SV_X_( ((Object) c), ((Object) v), ((Object) i), t));
}
}
}
}
return Utils.copy(setCompResult_6);
}
public String toString()
{
return "Orch{}";
}
public static class Type implements Record
{
public Object type ;
public Object startValue ;
public Type( final Object _type, final Object _startValue)
{
type = _type != null ? _type : null;
startValue = _startValue != null ? _startValue : null;
}
public boolean equals( final Object obj)
{
if ( !(obj instanceof Type) )
{
return false;
}
Type other = ((Type) obj);
return (Utils.equals(type, other.type)) && (Utils.equals(startValue, other.startValue));
}
public int hashCode()
{
return Utils.hashCode(type, startValue);
}
public Type copy()
{
return new Type(type, startValue);
}
public String toString()
{
return "mk_Orch`Type" + Utils.formatFields(type, startValue)
;
}
}
public static class SV implements Record
{
public Object causality ;
public Object variability ;
public Object initial ;
public Type type ;
public SV( final Object _causality, final Object _variability, final Object _initial, final Type _type)
{
causality = _causality != null ? _causality : null;
variability = _variability != null ? _variability : null;
initial = _initial != null ? _initial : null;
type = _type != null ? Utils.copy(_type) : null;
}
public boolean equals( final Object obj)
{
if ( !(obj instanceof SV) )
{
return false;
}
SV other = ((SV) obj);
return (Utils.equals(causality, other.causality)) && (Utils.equals(variability, other.variability)) && (Utils.equals(initial, other.initial)) && (Utils.equals(type, other.type));
}
public int hashCode()
{
return Utils.hashCode(causality, variability, initial, type);
}
public SV copy()
{
return new SV(causality, variability, initial, type);
}
public String toString()
{
return "mk_Orch`SV" + Utils.formatFields(causality, variability, initial, type)
;
}
}
public static class SV_X_ implements Record
{
public Object causality ;
public Object variability ;
public Object initial ;
public Type type ;
public SV_X_( final Object _causality, final Object _variability, final Object _initial, final Type _type)
{
causality = _causality != null ? _causality : null;
variability = _variability != null ? _variability : null;
initial = _initial != null ? _initial : null;
type = _type != null ? Utils.copy(_type) : null;
}
public boolean equals( final Object obj)
{
if ( !(obj instanceof SV_X_) )
{
return false;
}
SV_X_ other = ((SV_X_) obj);
return (Utils.equals(causality, other.causality)) && (Utils.equals(variability, other.variability)) && (Utils.equals(initial, other.initial)) && (Utils.equals(type, other.type));
}
public int hashCode()
{
return Utils.hashCode(causality, variability, initial, type);
}
public SV_X_ copy()
{
return new SV_X_(causality, variability, initial, type);
}
public String toString()
{
return "mk_Orch`SV_X_" + Utils.formatFields(causality, variability, initial, type)
;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy