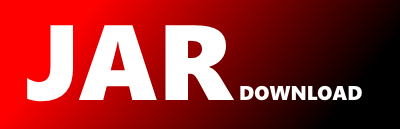
org.iq80.cli.Accessor Maven / Gradle / Ivy
package org.iq80.cli;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
public class Accessor
{
private final String name;
private final Class> javaType;
private final List path;
private boolean multiValued;
public Accessor(Field... path)
{
this(ImmutableList.copyOf(path));
}
public Accessor(Iterable path)
{
Preconditions.checkNotNull(path, "path is null");
Preconditions.checkArgument(!Iterables.isEmpty(path), "path is empty");
this.path = ImmutableList.copyOf(path);
this.name = this.path.get(0).getDeclaringClass().getSimpleName() + "." + Joiner.on('.').join(Iterables.transform(this.path, new Function()
{
public String apply(Field field)
{
return field.getName();
}
}));
Field field = this.path.get(this.path.size() - 1);
multiValued = Collection.class.isAssignableFrom(field.getType());
javaType = getItemType(name, field.getGenericType());
}
public String getName()
{
return name;
}
public Class> getJavaType()
{
return javaType;
}
public boolean isMultiValued()
{
return multiValued;
}
public Object getValue(Object instance)
{
StringBuilder pathName = new StringBuilder();
for (Field intermediateField : path.subList(0, path.size() - 1)) {
if (pathName.length() != 0) {
pathName.append(".");
}
pathName.append(intermediateField.getName());
try {
Object nextInstance = intermediateField.get(instance);
if (nextInstance == null) {
nextInstance = ParserUtil.createInstance(intermediateField.getType());
intermediateField.set(instance, nextInstance);
}
instance = nextInstance;
}
catch (Exception e) {
throw new ParseException(String.format("Error getting value of %s", pathName));
}
}
return instance;
}
public void addValues(Object commandInstance, Iterable> values)
{
if (Iterables.isEmpty(values)) {
return;
}
// get the actual instance
Object instance = getValue(commandInstance);
Field field = path.get(path.size() - 1);
field.setAccessible(true);
if (Collection.class.isAssignableFrom(field.getType())) {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy