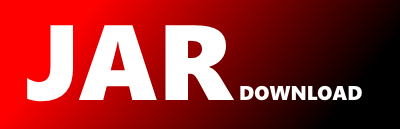
org.iq80.cli.ParserUtil Maven / Gradle / Ivy
package org.iq80.cli;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ListMultimap;
import org.iq80.cli.model.ArgumentsMetadata;
import org.iq80.cli.model.OptionMetadata;
import java.util.List;
import java.util.Map;
import static com.google.common.collect.Iterables.concat;
public class ParserUtil
{
public static T createInstance(Class type)
{
if (type != null) {
try {
return type.getConstructor().newInstance();
}
catch (Exception e) {
throw new ParseException("Unable to create instance %s", type.getName());
}
}
return null;
}
public static T createInstance(Class> type,
Iterable options,
ListMultimap parsedOptions,
ArgumentsMetadata arguments,
Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy