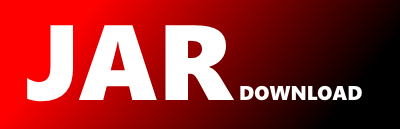
org.isisaddons.module.excel.dom.ExcelFixture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of isis-module-excel-dom Show documentation
Show all versions of isis-module-excel-dom Show documentation
A domain service for Apache Isis', allowing collections
of (view model) objects to be exported/imported to/from an
Excel spreadsheet.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.isisaddons.module.excel.dom;
import java.io.IOException;
import java.net.URL;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.io.Resources;
import org.datanucleus.enhancement.Persistable;
import org.apache.isis.applib.annotation.MemberOrder;
import org.apache.isis.applib.annotation.Programmatic;
import org.apache.isis.applib.fixturescripts.FixtureResultList;
import org.apache.isis.applib.fixturescripts.FixtureScript;
import org.apache.isis.applib.fixturescripts.FixtureScripts;
import org.apache.isis.applib.services.bookmark.BookmarkService;
import org.apache.isis.applib.services.registry.ServiceRegistry;
import org.apache.isis.applib.services.repository.RepositoryService;
import org.apache.isis.applib.value.Blob;
import org.isisaddons.module.excel.dom.util.ExcelServiceImpl;
import lombok.Getter;
import lombok.Setter;
/**
* This class should be executed using {@link FixtureScripts.MultipleExecutionStrategy#EXECUTE_ONCE_BY_VALUE} (it
* has value semantics).
*/
public class ExcelFixture extends FixtureScript {
private final List classes;
public ExcelFixture(final URL excelResource, final Class... classes) {
this(excelResource, Arrays.asList(classes));
}
public ExcelFixture(final URL excelResource, final List classes) {
this(classes);
setExcelResource(excelResource);
}
public ExcelFixture(final Blob upload, final Class... classes) {
this(upload, Arrays.asList(classes));
}
public ExcelFixture(final Blob blob, final List classes) {
this(classes);
setBlob(blob);
}
private ExcelFixture(final List classes) {
for (Class cls : classes) {
final boolean viewModel = ExcelFixtureRowHandler.class.isAssignableFrom(cls);
final boolean persistable = Persistable.class.isAssignableFrom(cls);
if (!viewModel && !persistable) {
throw new IllegalArgumentException(String.format(
"Class '%s' does not implement '%s', nor is it persistable",
cls.getSimpleName(), ExcelFixtureRowHandler.class.getSimpleName()));
}
}
this.classes = classes;
}
/**
* Input, optional: defines the name of the resource, used as a suffix to override {@link #getQualifiedName()}
* (disambiguate items when added to {@link FixtureResultList} if multiple instances of {@link ExcelFixture} are
* used with different excel spreadsheets).
*/
@Getter @Setter
@MemberOrder(sequence = "1.1")
private String excelResourceName;
/**
* Input, mandatory ... the Excel spreadsheet to read.
*/
@Getter @Setter
@MemberOrder(sequence = "1.2")
private URL excelResource;
/**
* Input, mandatory ... the Excel spreadsheet to read.
*/
@Getter @Setter
private Blob blob;
/**
* Output: the objects created by this fixture, for a specific persistable/row handler class.
*/
@Getter
private final Map> objectsByClass = Maps.newHashMap();
/**
* Output: all the objects created by this fixture.
*/
@Getter
private final List objects = Lists.newArrayList();
@Programmatic
@Override
public String getQualifiedName() {
return super.getQualifiedName() + (getExcelResourceName() != null ? "-" + getExcelResourceName() : "");
}
@Override
protected void execute(final ExecutionContext ec) {
final ExcelServiceImpl excelServiceImpl = new ExcelServiceImpl();
serviceRegistry.injectServicesInto(excelServiceImpl);
if (blob == null){
byte[] bytes = getBytes();
blob = new Blob("unused", ExcelService.XSLX_MIME_TYPE, bytes);
}
for (Class cls : classes) {
final List rowObjects = excelServiceImpl.fromExcel(blob, cls);
Object previousRow = null;
for (final Object rowObj : rowObjects) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy