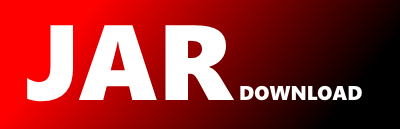
org.isisaddons.module.excel.dom.ExcelService Maven / Gradle / Ivy
Show all versions of isis-module-excel-dom Show documentation
/*
* Copyright 2014-2016 Dan Haywood
*
* Licensed under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.isisaddons.module.excel.dom;
import java.util.List;
import java.util.Map;
import javax.annotation.PostConstruct;
import org.apache.isis.applib.DomainObjectContainer;
import org.apache.isis.applib.RecoverableException;
import org.apache.isis.applib.annotation.DomainService;
import org.apache.isis.applib.annotation.NatureOfService;
import org.apache.isis.applib.annotation.Programmatic;
import org.apache.isis.applib.services.bookmark.BookmarkService;
import org.apache.isis.applib.services.registry.ServiceRegistry;
import org.apache.isis.applib.value.Blob;
import org.isisaddons.module.excel.dom.util.ExcelServiceImpl;
@DomainService(
nature = NatureOfService.DOMAIN
)
public class ExcelService {
public static class Exception extends RecoverableException {
private static final long serialVersionUID = 1L;
public Exception(final String msg, final Throwable ex) {
super(msg, ex);
}
public Exception(final Throwable ex) {
super(ex);
}
}
public static final String XSLX_MIME_TYPE = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
private ExcelServiceImpl excelServiceImpl;
public ExcelService() {
}
@Programmatic
@PostConstruct
public void init(final Map properties) {
excelServiceImpl = new ExcelServiceImpl();
serviceRegistry.injectServicesInto(excelServiceImpl);
}
// //////////////////////////////////////
/**
* Creates a Blob holding a spreadsheet of the domain objects.
*
*
* There are no specific restrictions on the domain objects; they can be either persistable entities or
* view models. Do be aware though that if imported back using {@link #fromExcel(Blob, Class)},
* then new instances are always created. It is generally better therefore to work with view models than to
* work with entities. This also makes it easier to maintain backward compatibility in the future if the
* persistence model changes; using view models represents a stable API for import/export.
*
*/
@Programmatic
public Blob toExcel(
final List domainObjects,
final Class cls,
final String fileName) throws ExcelService.Exception {
return excelServiceImpl.toExcel(domainObjects, cls, fileName);
}
public Blob toExcel(
final List domainObjects,
final Class cls,
final String sheetName,
final String fileName) throws ExcelService.Exception {
return excelServiceImpl.toExcel(domainObjects, cls, sheetName, fileName);
}
@Programmatic
public Blob toExcel(
final WorksheetContent worksheetContent,
final String fileName) throws ExcelService.Exception {
return excelServiceImpl.toExcel(worksheetContent, fileName);
}
@Programmatic
public Blob toExcel(
final List worksheetContents,
final String fileName) throws ExcelService.Exception {
return excelServiceImpl.toExcel(worksheetContents, fileName);
}
/**
* Returns a list of objects for each line in the spreadsheet, of the specified type.
*
*
* If the class is a view model then the objects will be properly instantiated (that is, using
* {@link DomainObjectContainer#newViewModelInstance(Class, String)}, with the correct
* view model memento); otherwise the objects will be simple transient objects (that is, using
* {@link DomainObjectContainer#newTransientInstance(Class)}).
*
*/
@Programmatic
public List fromExcel(
final Blob excelBlob,
final Class cls) throws ExcelService.Exception {
return excelServiceImpl.fromExcel(excelBlob, cls);
}
@Programmatic
public List fromExcel(
final Blob excelBlob,
final Class cls,
final String sheetName) throws ExcelService.Exception {
return excelServiceImpl.fromExcel(excelBlob, cls, sheetName);
}
@Programmatic
public List fromExcel(
final Blob excelBlob,
final WorksheetSpec worksheetSpec) throws ExcelService.Exception {
return excelServiceImpl.fromExcel(excelBlob, worksheetSpec);
}
@Programmatic
public List> fromExcel(
final Blob excelBlob,
final List worksheetSpecs) throws ExcelService.Exception {
return excelServiceImpl.fromExcel(excelBlob, worksheetSpecs);
}
@javax.inject.Inject
private DomainObjectContainer container;
@javax.inject.Inject
private BookmarkService bookmarkService;
@javax.inject.Inject
private ServiceRegistry serviceRegistry;
}