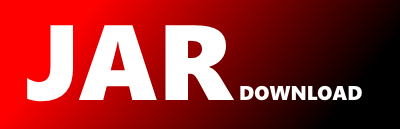
org.iworkz.habitat.dao.Context Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of habitat Show documentation
Show all versions of habitat Show documentation
A lightweight Java library for simple database access.
package org.iworkz.habitat.dao;
import java.sql.Connection;
import java.sql.SQLException;
import javax.sql.DataSource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Context implements ConnectionProvider {
private static final Logger logger = LoggerFactory.getLogger(Context.class);
private boolean started;
private Connection connection;
private DataSource dataSource;
public Context(DataSource dataSource) {
setDataSource(dataSource);
}
protected void setDataSource(DataSource dataSource) {
this.dataSource = dataSource;
}
@Override
public Connection get() {
checkState();
if (this.connection == null) {
try {
this.connection = this.dataSource.getConnection();
} catch (SQLException e) {
throw new RuntimeException("Can not get connection", e);
}
}
return this.connection;
}
protected void checkState() {
if (!this.started) {
throw new IllegalStateException("The context has not been started.");
}
}
public void start() {
if (this.started) {
throw new IllegalStateException("The context is already started.");
}
logger.debug("Start context");
this.started = true;
}
public void close() {
if (this.connection != null) {
try {
logger.debug("Close connection");
this.connection.close();
} catch (SQLException e) {
logger.error("Connection can not be closed", e);
} finally {
this.connection = null;
}
} else {
logger.debug("No connection to close");
}
this.started = false;
}
public boolean isStarted() {
return this.started;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy