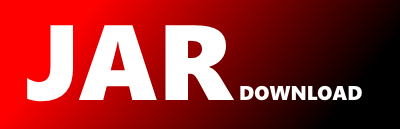
org.iworkz.habitat.dialect.DatabaseDialect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of habitat Show documentation
Show all versions of habitat Show documentation
A lightweight Java library for simple database access.
package org.iworkz.habitat.dialect;
import java.lang.reflect.Type;
import javax.inject.Singleton;
import org.iworkz.habitat.entity.EntityDefinition;
import org.iworkz.habitat.entity.EntityFieldDefinition;
import org.iworkz.habitat.entity.FieldValueStrategy;
@Singleton
public class DatabaseDialect {
public String getTypeName(EntityFieldDefinition entityFieldDefinition) {
switch (entityFieldDefinition.getType()) {
case NUMBER:
return "NUMBER";
case VARCHAR:
return "VARCHAR";
case DATE:
return "DATE";
case BLOB:
return "BLOB";
}
throw new RuntimeException("Type name not defined for type " + entityFieldDefinition.getType());
}
public String getConstraintsSql(EntityFieldDefinition entityFieldDefinition) {
Integer constraint1 = entityFieldDefinition.getConstraint1();
Integer constraint2 = entityFieldDefinition.getConstraint2();
if (constraint1 != null && constraint1 > 0) {
if (constraint2 != null && constraint2 > 0) {
return "(" + constraint1 + "," + constraint2 + ")";
} else {
return "(" + constraint1 + ")";
}
}
return "";
}
public String buildDropTableIfExistsStatement(EntityDefinition table) {
return "DROP TABLE IF EXISTS " + table.getName() + ";";
}
public String buildCreateTableIfExistsStatement(EntityDefinition table, boolean onlyIfNotExists) {
StringBuilder sql = new StringBuilder();
sql.append("CREATE TABLE ");
if (onlyIfNotExists) {
sql.append("IF NOT EXISTS ");
}
sql.append(table.getName() + "(");
table.appendFieldDefinitionsSql(sql);
table.appendPrimaryKeySqL(sql);
sql.append(")");
return sql.toString();
}
public void appendFieldDefinitionsSql(StringBuilder sql, EntityDefinition table) {
boolean first = true;
for (EntityFieldDefinition f : table.getFields()) {
StringBuilder command = new StringBuilder();
if (!first) {
command.append(", ");
} else {
first = false;
}
command.append(f.getName());
command.append(" ");
command.append(f.getTypeName());
command.append(f.getConstraintsSql());
if (f.getValueStrategy() == FieldValueStrategy.AUTO) {
command.append(" auto_increment");
}
command.append(f.getNotNullSql());
sql.append(command.toString());
}
if (!table.getPrimaryKey().isEmpty()) {
sql.append(", ");
}
}
public void appendPrimaryKeySqL(StringBuilder sql, EntityDefinition table) {
if (!table.getPrimaryKey().isEmpty()) {
sql.append("PRIMARY KEY (");
for (EntityFieldDefinition p : table.getPrimaryKey()) {
sql.append(p.getName());
}
sql.append(")");
}
}
public Integer getDefaultPrecision(Type javaType) {
return null;
}
public Integer getDefaultScale(Type javaType) {
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy