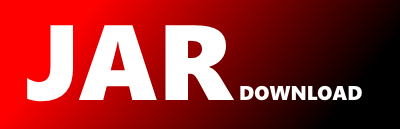
org.iworkz.habitat.dao.BlobHelper Maven / Gradle / Ivy
package org.iworkz.habitat.dao;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.Writer;
import java.sql.Blob;
import java.sql.Clob;
public class BlobHelper {
private static final int BUFFER_SIZE = 4096;
private BlobHelper() {
super();
}
public static void writeBlobToOutputStream(Blob blob, OutputStream outputStream) throws Exception {
InputStream inputStream = blob.getBinaryStream();
try {
writeInputStreamToOutputStream(inputStream,outputStream);
} finally {
inputStream.close();
}
}
public static void writeClobToOutputStream(Clob clob, OutputStream outputStream) throws Exception {
Reader reader = clob.getCharacterStream();
PrintWriter writer = new PrintWriter(outputStream);
try {
writeReaderToWriter(reader,writer);
} finally {
reader.close();
writer.close();
}
}
public static void writeReaderToWriter(Reader rd, Writer wr) throws Exception {
char[] buffer = new char[BUFFER_SIZE];
int bytesRead = -1;
while ((bytesRead = rd.read(buffer)) != -1) {
wr.write(buffer, 0, bytesRead);
}
}
public static void writeInputStreamToOutputStream(InputStream is, OutputStream outputStream) throws Exception {
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead = -1;
while ((bytesRead = is.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
}
public static byte[] getBytesFromInputStream(InputStream inputStream) throws Exception {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try {
writeInputStreamToOutputStream(inputStream,outputStream);
outputStream.flush();
return outputStream.toByteArray();
} finally {
outputStream.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy