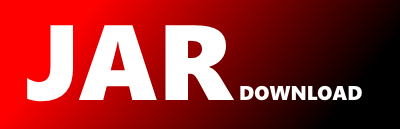
org.jacodb.api.Api.kt Maven / Gradle / Ivy
Show all versions of jacodb-api Show documentation
/*
* Copyright 2022 UnitTestBot contributors (utbot.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jacodb.api
import kotlinx.coroutines.GlobalScope
import kotlinx.coroutines.future.future
import org.jooq.DSLContext
import java.io.Closeable
import java.io.File
import java.sql.Connection
enum class LocationType {
RUNTIME,
APP
}
interface ClassSource {
val className: String
val byteCode: ByteArray
val location: RegisteredLocation
}
/**
* field usage mode
*/
enum class FieldUsageMode {
/** search for reads */
READ,
/** search for writes */
WRITE
}
interface JavaVersion {
val majorVersion: Int
}
/**
* Compilation database
*
* `close` method should be called when database is not needed anymore
*/
@JvmDefaultWithoutCompatibility
interface JcDatabase : Closeable {
val locations: List
val persistence: JcDatabasePersistence
val runtimeVersion: JavaVersion
/**
* create classpath instance
*
* @param dirOrJars list of byte-code resources to be processed and included in classpath
* @return new classpath instance associated with specified byte-code locations
*/
suspend fun classpath(dirOrJars: List, features: List?): JcClasspath
suspend fun classpath(dirOrJars: List): JcClasspath = classpath(dirOrJars, null)
fun asyncClasspath(dirOrJars: List) = GlobalScope.future { classpath(dirOrJars) }
fun asyncClasspath(dirOrJars: List, features: List?) =
GlobalScope.future { classpath(dirOrJars, features) }
fun classpathOf(locations: List, features: List?): JcClasspath
/**
* process and index single byte-code resource
* @param dirOrJar build folder or jar file
* @return current database instance
*/
suspend fun load(dirOrJar: File): JcDatabase
fun asyncLoad(dirOrJar: File) = GlobalScope.future { load(dirOrJar) }
/**
* process and index byte-code resources
* @param dirOrJars list of build folder or jar file
* @return current database instance
*/
suspend fun load(dirOrJars: List): JcDatabase
fun asyncLoad(dirOrJars: List) = GlobalScope.future { load(dirOrJars) }
/**
* load locations
* @param locations locations to load
* @return current database instance
*/
suspend fun loadLocations(locations: List): JcDatabase
fun asyncLocations(locations: List) = GlobalScope.future { loadLocations(locations) }
/**
* explicitly refreshes the state of resources from file-system.
* That means that any new classpath created after refresh is done will
* reference fresh byte-code from file-system. While any classpath created
* before `refresh` will still reference byte-code which is outdated
* according to file-system
*/
suspend fun refresh()
fun asyncRefresh() = GlobalScope.future { refresh() }
/**
* rebuilds features data (indexes)
*/
suspend fun rebuildFeatures()
fun asyncRebuildFeatures() = GlobalScope.future { rebuildFeatures() }
/**
* watch file system for changes and refreshes the state of database in case loaded resources and resources from
* file systems are different.
*
* @return current database instance
*/
fun watchFileSystemChanges(): JcDatabase
/**
* await background jobs
*/
suspend fun awaitBackgroundJobs()
fun asyncAwaitBackgroundJobs() = GlobalScope.future { awaitBackgroundJobs() }
fun isInstalled(feature: JcFeature<*, *>): Boolean
}
interface JcDatabasePersistence : Closeable {
val locations: List
fun setup()
fun write(action: (DSLContext) -> T): T
fun read(action: (DSLContext) -> T): T
fun persist(location: RegisteredLocation, classes: List)
fun findSymbolId(symbol: String): Long?
fun findSymbolName(symbolId: Long): String
fun findLocation(locationId: Long): RegisteredLocation
val symbolInterner: JCDBSymbolsInterner
fun findBytecode(classId: Long): ByteArray
fun findClassSourceByName(cp: JcClasspath, fullName: String): ClassSource?
fun findClassSources(db: JcDatabase, location: RegisteredLocation): List
fun findClassSources(cp: JcClasspath, fullName: String): List
fun createIndexes() {}
}
interface RegisteredLocation {
val jcLocation: JcByteCodeLocation?
val id: Long
val path: String
val isRuntime: Boolean
}
interface JCDBSymbolsInterner {
val jooq: DSLContext
fun findOrNew(symbol: String): Long
fun flush(conn: Connection)
}