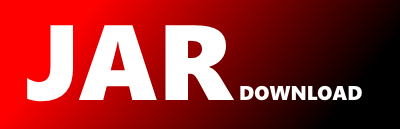
org.jaitools.jiffle.parser.CheckAssignments Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/CheckAssignments.g 2012-10-10 10:44:32
package org.jaitools.jiffle.parser;
import java.util.Set;
import org.jaitools.CollectionFactory;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
/**
* Checks for valid use of image variables.
*
* @author Michael Bedward
*/
public class CheckAssignments extends TreeParser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ABS_POS", "BAND_REF", "BLOCK", "CON_CALL", "DECL", "DECLARED_LIST", "EXPR_LIST", "FUNC_CALL", "IMAGE_POS", "JIFFLE_OPTION", "PAR", "PIXEL_REF", "POSTFIX", "PREFIX", "REL_POS", "SEQUENCE", "VAR_DEST", "VAR_IMAGE_SCOPE", "VAR_SOURCE", "CONSTANT", "IMAGE_WRITE", "LIST_NEW", "VAR_IMAGE", "VAR_PIXEL_SCOPE", "VAR_PROVIDED", "VAR_LOOP", "VAR_LIST", "OPTIONS", "LCURLY", "RCURLY", "ID", "EQ", "SEMI", "IMAGES", "READ", "WRITE", "INIT", "WHILE", "LPAR", "RPAR", "UNTIL", "FOREACH", "IN", "IF", "ELSE", "BREAKIF", "BREAK", "COMMA", "COLON", "CON", "APPEND", "TIMESEQ", "DIVEQ", "MODEQ", "PLUSEQ", "MINUSEQ", "QUESTION", "OR", "XOR", "AND", "LOGICALEQ", "NE", "GT", "GE", "LE", "LT", "PLUS", "MINUS", "TIMES", "DIV", "MOD", "NOT", "INCR", "DECR", "POW", "LSQUARE", "RSQUARE", "ABS_POS_PREFIX", "INT_LITERAL", "FLOAT_LITERAL", "TRUE", "FALSE", "NULL", "COMMENT", "INT_TYPE", "FLOAT_TYPE", "DOUBLE_TYPE", "BOOLEAN_TYPE", "Letter", "UNDERSCORE", "Digit", "Dot", "NonZeroDigit", "FloatExp", "WS", "ESC_SEQ", "CHAR", "HEX_DIGIT", "UNICODE_ESC", "OCTAL_ESC"
};
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public CheckAssignments(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public CheckAssignments(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return CheckAssignments.tokenNames; }
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/CheckAssignments.g"; }
private MessageTable msgTable;
private SymbolScopeStack varScope;
public CheckAssignments(TreeNodeStream input, MessageTable msgTable) {
this(input);
if (msgTable == null) {
throw new IllegalArgumentException( "msgTable should not be null" );
}
this.msgTable = msgTable;
varScope = new SymbolScopeStack();
}
// $ANTLR start "start"
// org/jaitools/jiffle/parser/CheckAssignments.g:59:1: start : ( jiffleOption )* ( varDeclaration )* ( statement )+ ;
public final void start() throws RecognitionException {
varScope.addLevel("top");
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:62:17: ( ( jiffleOption )* ( varDeclaration )* ( statement )+ )
// org/jaitools/jiffle/parser/CheckAssignments.g:62:19: ( jiffleOption )* ( varDeclaration )* ( statement )+
{
// org/jaitools/jiffle/parser/CheckAssignments.g:62:19: ( jiffleOption )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case JIFFLE_OPTION:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:62:19: jiffleOption
{
pushFollow(FOLLOW_jiffleOption_in_start77);
jiffleOption();
state._fsp--;
}
break;
default :
break loop1;
}
} while (true);
// org/jaitools/jiffle/parser/CheckAssignments.g:62:33: ( varDeclaration )*
loop2:
do {
int alt2=2;
switch ( input.LA(1) ) {
case DECL:
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:62:33: varDeclaration
{
pushFollow(FOLLOW_varDeclaration_in_start80);
varDeclaration();
state._fsp--;
}
break;
default :
break loop2;
}
} while (true);
// org/jaitools/jiffle/parser/CheckAssignments.g:62:49: ( statement )+
int cnt3=0;
loop3:
do {
int alt3=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt3=1;
}
break;
}
switch (alt3) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:62:49: statement
{
pushFollow(FOLLOW_statement_in_start83);
statement();
state._fsp--;
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee =
new EarlyExitException(3, input);
throw eee;
}
cnt3++;
} while (true);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "start"
// $ANTLR start "jiffleOption"
// org/jaitools/jiffle/parser/CheckAssignments.g:66:1: jiffleOption : ^( JIFFLE_OPTION ID . ) ;
public final void jiffleOption() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:66:17: ( ^( JIFFLE_OPTION ID . ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:66:19: ^( JIFFLE_OPTION ID . )
{
match(input,JIFFLE_OPTION,FOLLOW_JIFFLE_OPTION_in_jiffleOption114);
match(input, Token.DOWN, null);
match(input,ID,FOLLOW_ID_in_jiffleOption116);
matchAny(input);
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "jiffleOption"
// $ANTLR start "varDeclaration"
// org/jaitools/jiffle/parser/CheckAssignments.g:70:1: varDeclaration : ( ^( DECL VAR_DEST ID ) | ^( DECL VAR_SOURCE ID ) | ^( DECL VAR_IMAGE_SCOPE ( . )? ) );
public final void varDeclaration() throws RecognitionException {
CommonTree VAR_IMAGE_SCOPE1=null;
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:70:17: ( ^( DECL VAR_DEST ID ) | ^( DECL VAR_SOURCE ID ) | ^( DECL VAR_IMAGE_SCOPE ( . )? ) )
int alt5=3;
switch ( input.LA(1) ) {
case DECL:
{
switch ( input.LA(2) ) {
case DOWN:
{
switch ( input.LA(3) ) {
case VAR_DEST:
{
alt5=1;
}
break;
case VAR_SOURCE:
{
alt5=2;
}
break;
case VAR_IMAGE_SCOPE:
{
alt5=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 5, 2, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 5, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:70:19: ^( DECL VAR_DEST ID )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration147);
match(input, Token.DOWN, null);
match(input,VAR_DEST,FOLLOW_VAR_DEST_in_varDeclaration149);
match(input,ID,FOLLOW_ID_in_varDeclaration151);
match(input, Token.UP, null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:71:19: ^( DECL VAR_SOURCE ID )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration173);
match(input, Token.DOWN, null);
match(input,VAR_SOURCE,FOLLOW_VAR_SOURCE_in_varDeclaration175);
match(input,ID,FOLLOW_ID_in_varDeclaration177);
match(input, Token.UP, null);
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:73:19: ^( DECL VAR_IMAGE_SCOPE ( . )? )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration200);
match(input, Token.DOWN, null);
VAR_IMAGE_SCOPE1=(CommonTree)match(input,VAR_IMAGE_SCOPE,FOLLOW_VAR_IMAGE_SCOPE_in_varDeclaration202);
// org/jaitools/jiffle/parser/CheckAssignments.g:73:42: ( . )?
int alt4=2;
switch ( input.LA(1) ) {
case ABS_POS:
case BAND_REF:
case BLOCK:
case CON_CALL:
case DECL:
case DECLARED_LIST:
case EXPR_LIST:
case FUNC_CALL:
case IMAGE_POS:
case JIFFLE_OPTION:
case PAR:
case PIXEL_REF:
case POSTFIX:
case PREFIX:
case REL_POS:
case SEQUENCE:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case LIST_NEW:
case VAR_IMAGE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case OPTIONS:
case LCURLY:
case RCURLY:
case ID:
case EQ:
case SEMI:
case IMAGES:
case READ:
case WRITE:
case INIT:
case WHILE:
case LPAR:
case RPAR:
case UNTIL:
case FOREACH:
case IN:
case IF:
case ELSE:
case BREAKIF:
case BREAK:
case COMMA:
case COLON:
case CON:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case NOT:
case INCR:
case DECR:
case POW:
case LSQUARE:
case RSQUARE:
case ABS_POS_PREFIX:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
case COMMENT:
case INT_TYPE:
case FLOAT_TYPE:
case DOUBLE_TYPE:
case BOOLEAN_TYPE:
case Letter:
case UNDERSCORE:
case Digit:
case Dot:
case NonZeroDigit:
case FloatExp:
case WS:
case ESC_SEQ:
case CHAR:
case HEX_DIGIT:
case UNICODE_ESC:
case OCTAL_ESC:
{
alt4=1;
}
break;
}
switch (alt4) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:73:42: .
{
matchAny(input);
}
break;
}
match(input, Token.UP, null);
varScope.addSymbol((VAR_IMAGE_SCOPE1!=null?VAR_IMAGE_SCOPE1.getText():null), SymbolType.SCALAR, ScopeType.IMAGE);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "varDeclaration"
// $ANTLR start "block"
// org/jaitools/jiffle/parser/CheckAssignments.g:80:1: block : ^( BLOCK ( statement )* ) ;
public final void block() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:80:17: ( ^( BLOCK ( statement )* ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:80:19: ^( BLOCK ( statement )* )
{
match(input,BLOCK,FOLLOW_BLOCK_in_block261);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/jaitools/jiffle/parser/CheckAssignments.g:80:27: ( statement )*
loop6:
do {
int alt6=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt6=1;
}
break;
}
switch (alt6) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:80:27: statement
{
pushFollow(FOLLOW_statement_in_block263);
statement();
state._fsp--;
}
break;
default :
break loop6;
}
} while (true);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "block"
// $ANTLR start "statement"
// org/jaitools/jiffle/parser/CheckAssignments.g:84:1: statement : ( block | ifCall | assignmentExpression | ^( WHILE loopCondition statement ) | ^( UNTIL loopCondition statement ) | foreachLoop | ^( BREAKIF expression ) | BREAK | expression );
public final void statement() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:84:17: ( block | ifCall | assignmentExpression | ^( WHILE loopCondition statement ) | ^( UNTIL loopCondition statement ) | foreachLoop | ^( BREAKIF expression ) | BREAK | expression )
int alt7=9;
switch ( input.LA(1) ) {
case BLOCK:
{
alt7=1;
}
break;
case IF:
{
alt7=2;
}
break;
case EQ:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
{
alt7=3;
}
break;
case WHILE:
{
alt7=4;
}
break;
case UNTIL:
{
alt7=5;
}
break;
case FOREACH:
{
alt7=6;
}
break;
case BREAKIF:
{
alt7=7;
}
break;
case BREAK:
{
alt7=8;
}
break;
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
case APPEND:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt7=9;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:84:19: block
{
pushFollow(FOLLOW_block_in_statement297);
block();
state._fsp--;
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:85:19: ifCall
{
pushFollow(FOLLOW_ifCall_in_statement317);
ifCall();
state._fsp--;
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:86:19: assignmentExpression
{
pushFollow(FOLLOW_assignmentExpression_in_statement337);
assignmentExpression();
state._fsp--;
}
break;
case 4 :
// org/jaitools/jiffle/parser/CheckAssignments.g:87:19: ^( WHILE loopCondition statement )
{
match(input,WHILE,FOLLOW_WHILE_in_statement358);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_loopCondition_in_statement360);
loopCondition();
state._fsp--;
pushFollow(FOLLOW_statement_in_statement362);
statement();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 5 :
// org/jaitools/jiffle/parser/CheckAssignments.g:88:19: ^( UNTIL loopCondition statement )
{
match(input,UNTIL,FOLLOW_UNTIL_in_statement384);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_loopCondition_in_statement386);
loopCondition();
state._fsp--;
pushFollow(FOLLOW_statement_in_statement388);
statement();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 6 :
// org/jaitools/jiffle/parser/CheckAssignments.g:89:19: foreachLoop
{
pushFollow(FOLLOW_foreachLoop_in_statement409);
foreachLoop();
state._fsp--;
}
break;
case 7 :
// org/jaitools/jiffle/parser/CheckAssignments.g:90:19: ^( BREAKIF expression )
{
match(input,BREAKIF,FOLLOW_BREAKIF_in_statement430);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_statement432);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 8 :
// org/jaitools/jiffle/parser/CheckAssignments.g:91:19: BREAK
{
match(input,BREAK,FOLLOW_BREAK_in_statement453);
}
break;
case 9 :
// org/jaitools/jiffle/parser/CheckAssignments.g:92:19: expression
{
pushFollow(FOLLOW_expression_in_statement473);
expression();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "statement"
// $ANTLR start "ifCall"
// org/jaitools/jiffle/parser/CheckAssignments.g:96:1: ifCall : ^( IF expression statement ( statement )? ) ;
public final void ifCall() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:96:17: ( ^( IF expression statement ( statement )? ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:96:19: ^( IF expression statement ( statement )? )
{
match(input,IF,FOLLOW_IF_in_ifCall509);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_ifCall511);
expression();
state._fsp--;
pushFollow(FOLLOW_statement_in_ifCall513);
statement();
state._fsp--;
// org/jaitools/jiffle/parser/CheckAssignments.g:96:45: ( statement )?
int alt8=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:96:45: statement
{
pushFollow(FOLLOW_statement_in_ifCall515);
statement();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "ifCall"
// $ANTLR start "foreachLoop"
// org/jaitools/jiffle/parser/CheckAssignments.g:100:1: foreachLoop : ^( FOREACH ID loopSet statement ) ;
public final void foreachLoop() throws RecognitionException {
CommonTree ID2=null;
varScope.addLevel("foreach");
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:107:17: ( ^( FOREACH ID loopSet statement ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:107:19: ^( FOREACH ID loopSet statement )
{
match(input,FOREACH,FOLLOW_FOREACH_in_foreachLoop570);
match(input, Token.DOWN, null);
ID2=(CommonTree)match(input,ID,FOLLOW_ID_in_foreachLoop572);
varScope.addSymbol((ID2!=null?ID2.getText():null), SymbolType.LOOP_VAR, ScopeType.PIXEL);
pushFollow(FOLLOW_loopSet_in_foreachLoop576);
loopSet();
state._fsp--;
pushFollow(FOLLOW_statement_in_foreachLoop578);
statement();
state._fsp--;
match(input, Token.UP, null);
}
varScope.dropLevel();
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "foreachLoop"
// $ANTLR start "loopCondition"
// org/jaitools/jiffle/parser/CheckAssignments.g:111:1: loopCondition : expression ;
public final void loopCondition() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:111:17: ( expression )
// org/jaitools/jiffle/parser/CheckAssignments.g:111:19: expression
{
pushFollow(FOLLOW_expression_in_loopCondition607);
expression();
state._fsp--;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "loopCondition"
// $ANTLR start "loopSet"
// org/jaitools/jiffle/parser/CheckAssignments.g:115:1: loopSet : ( ^( SEQUENCE expression expression ) | listLiteral | VAR_LIST );
public final void loopSet() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:115:17: ( ^( SEQUENCE expression expression ) | listLiteral | VAR_LIST )
int alt9=3;
switch ( input.LA(1) ) {
case SEQUENCE:
{
alt9=1;
}
break;
case DECLARED_LIST:
{
alt9=2;
}
break;
case VAR_LIST:
{
alt9=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:115:19: ^( SEQUENCE expression expression )
{
match(input,SEQUENCE,FOLLOW_SEQUENCE_in_loopSet642);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_loopSet644);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_loopSet646);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:116:19: listLiteral
{
pushFollow(FOLLOW_listLiteral_in_loopSet667);
listLiteral();
state._fsp--;
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:117:19: VAR_LIST
{
match(input,VAR_LIST,FOLLOW_VAR_LIST_in_loopSet687);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "loopSet"
// $ANTLR start "expressionList"
// org/jaitools/jiffle/parser/CheckAssignments.g:121:1: expressionList : ^( EXPR_LIST ( expression )* ) ;
public final void expressionList() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:121:17: ( ^( EXPR_LIST ( expression )* ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:121:19: ^( EXPR_LIST ( expression )* )
{
match(input,EXPR_LIST,FOLLOW_EXPR_LIST_in_expressionList715);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/jaitools/jiffle/parser/CheckAssignments.g:121:31: ( expression )*
loop10:
do {
int alt10=2;
switch ( input.LA(1) ) {
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
case APPEND:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt10=1;
}
break;
}
switch (alt10) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:121:31: expression
{
pushFollow(FOLLOW_expression_in_expressionList717);
expression();
state._fsp--;
}
break;
default :
break loop10;
}
} while (true);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "expressionList"
// $ANTLR start "assignmentExpression"
// org/jaitools/jiffle/parser/CheckAssignments.g:125:1: assignmentExpression : ^( assignmentOp identifier expression ) ;
public final void assignmentExpression() throws RecognitionException {
CheckAssignments.identifier_return identifier3 = null;
CheckAssignments.assignmentOp_return assignmentOp4 = null;
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:126:17: ( ^( assignmentOp identifier expression ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:126:19: ^( assignmentOp identifier expression )
{
pushFollow(FOLLOW_assignmentOp_in_assignmentExpression762);
assignmentOp4=assignmentOp();
state._fsp--;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_identifier_in_assignmentExpression764);
identifier3=identifier();
state._fsp--;
pushFollow(FOLLOW_expression_in_assignmentExpression766);
expression();
state._fsp--;
match(input, Token.UP, null);
String varName = (identifier3!=null?((CommonTree)identifier3.start):null).getText();
int idtype = (identifier3!=null?((CommonTree)identifier3.start):null).getType();
switch (idtype) {
case CONSTANT:
msgTable.add(varName, Message.CONSTANT_LHS);
break;
case VAR_SOURCE:
msgTable.add(varName, Message.ASSIGNMENT_TO_SRC_IMAGE);
break;
default:
if ((assignmentOp4!=null?((CommonTree)assignmentOp4.start):null).getType() == EQ) {
if (!varScope.isDefined(varName)) {
varScope.addSymbol(varName, SymbolType.SCALAR, ScopeType.PIXEL);
}
} else {
switch (idtype) {
case VAR_DEST:
msgTable.add(varName, Message.INVALID_ASSIGNMENT_OP_WITH_DEST_IMAGE);
break;
default:
if (!varScope.isDefined(varName)) {
msgTable.add(varName, Message.UNINIT_VAR);
}
}
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "assignmentExpression"
public static class assignmentOp_return extends TreeRuleReturnScope {
};
// $ANTLR start "assignmentOp"
// org/jaitools/jiffle/parser/CheckAssignments.g:162:1: assignmentOp : ( EQ | TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ );
public final CheckAssignments.assignmentOp_return assignmentOp() throws RecognitionException {
CheckAssignments.assignmentOp_return retval = new CheckAssignments.assignmentOp_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:162:17: ( EQ | TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( input.LA(1)==EQ||(input.LA(1)>=TIMESEQ && input.LA(1)<=MINUSEQ) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "assignmentOp"
public static class identifier_return extends TreeRuleReturnScope {
};
// $ANTLR start "identifier"
// org/jaitools/jiffle/parser/CheckAssignments.g:171:1: identifier : ( imageVar | userVar | CONSTANT );
public final CheckAssignments.identifier_return identifier() throws RecognitionException {
CheckAssignments.identifier_return retval = new CheckAssignments.identifier_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:171:17: ( imageVar | userVar | CONSTANT )
int alt11=3;
switch ( input.LA(1) ) {
case VAR_DEST:
case VAR_SOURCE:
{
alt11=1;
}
break;
case VAR_IMAGE_SCOPE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
{
alt11=2;
}
break;
case CONSTANT:
{
alt11=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:171:19: imageVar
{
pushFollow(FOLLOW_imageVar_in_identifier945);
imageVar();
state._fsp--;
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:172:19: userVar
{
pushFollow(FOLLOW_userVar_in_identifier965);
userVar();
state._fsp--;
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:173:19: CONSTANT
{
match(input,CONSTANT,FOLLOW_CONSTANT_in_identifier985);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "identifier"
// $ANTLR start "imageVar"
// org/jaitools/jiffle/parser/CheckAssignments.g:177:1: imageVar : ( VAR_SOURCE | VAR_DEST );
public final void imageVar() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:177:17: ( VAR_SOURCE | VAR_DEST )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( input.LA(1)==VAR_DEST||input.LA(1)==VAR_SOURCE ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "imageVar"
public static class userVar_return extends TreeRuleReturnScope {
};
// $ANTLR start "userVar"
// org/jaitools/jiffle/parser/CheckAssignments.g:181:1: userVar : ( VAR_IMAGE_SCOPE | VAR_PIXEL_SCOPE | VAR_LOOP | VAR_LIST );
public final CheckAssignments.userVar_return userVar() throws RecognitionException {
CheckAssignments.userVar_return retval = new CheckAssignments.userVar_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:181:17: ( VAR_IMAGE_SCOPE | VAR_PIXEL_SCOPE | VAR_LOOP | VAR_LIST )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( input.LA(1)==VAR_IMAGE_SCOPE||input.LA(1)==VAR_PIXEL_SCOPE||(input.LA(1)>=VAR_LOOP && input.LA(1)<=VAR_LIST) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "userVar"
// $ANTLR start "expression"
// org/jaitools/jiffle/parser/CheckAssignments.g:188:1: expression : ( ^( FUNC_CALL ID expressionList ) | ^( CON_CALL expressionList ) | ^( QUESTION expression expression expression ) | ^( IMAGE_WRITE . expression ) | ^( IMAGE_POS . ( bandSpecifier )? ( pixelSpecifier )? ) | ^( logicalOp expression expression ) | ^( arithmeticOp expression expression ) | ^( POW expression expression ) | ^( PREFIX prefixOp expression ) | ^( POSTFIX incdecOp expression ) | ^( PAR expression ) | listOperation | listLiteral | literal | imageVar | CONSTANT | userVar );
public final void expression() throws RecognitionException {
CheckAssignments.userVar_return userVar5 = null;
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:188:17: ( ^( FUNC_CALL ID expressionList ) | ^( CON_CALL expressionList ) | ^( QUESTION expression expression expression ) | ^( IMAGE_WRITE . expression ) | ^( IMAGE_POS . ( bandSpecifier )? ( pixelSpecifier )? ) | ^( logicalOp expression expression ) | ^( arithmeticOp expression expression ) | ^( POW expression expression ) | ^( PREFIX prefixOp expression ) | ^( POSTFIX incdecOp expression ) | ^( PAR expression ) | listOperation | listLiteral | literal | imageVar | CONSTANT | userVar )
int alt14=17;
switch ( input.LA(1) ) {
case FUNC_CALL:
{
alt14=1;
}
break;
case CON_CALL:
{
alt14=2;
}
break;
case QUESTION:
{
alt14=3;
}
break;
case IMAGE_WRITE:
{
alt14=4;
}
break;
case IMAGE_POS:
{
alt14=5;
}
break;
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
{
alt14=6;
}
break;
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
{
alt14=7;
}
break;
case POW:
{
alt14=8;
}
break;
case PREFIX:
{
alt14=9;
}
break;
case POSTFIX:
{
alt14=10;
}
break;
case PAR:
{
alt14=11;
}
break;
case APPEND:
{
alt14=12;
}
break;
case DECLARED_LIST:
{
alt14=13;
}
break;
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt14=14;
}
break;
case VAR_DEST:
case VAR_SOURCE:
{
alt14=15;
}
break;
case CONSTANT:
{
alt14=16;
}
break;
case VAR_IMAGE_SCOPE:
case VAR_PIXEL_SCOPE:
case VAR_LOOP:
case VAR_LIST:
{
alt14=17;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:188:19: ^( FUNC_CALL ID expressionList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_expression1163);
match(input, Token.DOWN, null);
match(input,ID,FOLLOW_ID_in_expression1165);
pushFollow(FOLLOW_expressionList_in_expression1167);
expressionList();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:189:19: ^( CON_CALL expressionList )
{
match(input,CON_CALL,FOLLOW_CON_CALL_in_expression1189);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expressionList_in_expression1191);
expressionList();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:190:19: ^( QUESTION expression expression expression )
{
match(input,QUESTION,FOLLOW_QUESTION_in_expression1213);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression1215);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1217);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1219);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 4 :
// org/jaitools/jiffle/parser/CheckAssignments.g:191:19: ^( IMAGE_WRITE . expression )
{
match(input,IMAGE_WRITE,FOLLOW_IMAGE_WRITE_in_expression1241);
match(input, Token.DOWN, null);
matchAny(input);
pushFollow(FOLLOW_expression_in_expression1245);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 5 :
// org/jaitools/jiffle/parser/CheckAssignments.g:192:19: ^( IMAGE_POS . ( bandSpecifier )? ( pixelSpecifier )? )
{
match(input,IMAGE_POS,FOLLOW_IMAGE_POS_in_expression1267);
match(input, Token.DOWN, null);
matchAny(input);
// org/jaitools/jiffle/parser/CheckAssignments.g:192:33: ( bandSpecifier )?
int alt12=2;
switch ( input.LA(1) ) {
case BAND_REF:
{
alt12=1;
}
break;
}
switch (alt12) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:192:33: bandSpecifier
{
pushFollow(FOLLOW_bandSpecifier_in_expression1271);
bandSpecifier();
state._fsp--;
}
break;
}
// org/jaitools/jiffle/parser/CheckAssignments.g:192:48: ( pixelSpecifier )?
int alt13=2;
switch ( input.LA(1) ) {
case PIXEL_REF:
{
alt13=1;
}
break;
}
switch (alt13) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:192:48: pixelSpecifier
{
pushFollow(FOLLOW_pixelSpecifier_in_expression1274);
pixelSpecifier();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
}
break;
case 6 :
// org/jaitools/jiffle/parser/CheckAssignments.g:193:19: ^( logicalOp expression expression )
{
pushFollow(FOLLOW_logicalOp_in_expression1297);
logicalOp();
state._fsp--;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression1299);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1301);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 7 :
// org/jaitools/jiffle/parser/CheckAssignments.g:194:19: ^( arithmeticOp expression expression )
{
pushFollow(FOLLOW_arithmeticOp_in_expression1323);
arithmeticOp();
state._fsp--;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression1325);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1327);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 8 :
// org/jaitools/jiffle/parser/CheckAssignments.g:195:19: ^( POW expression expression )
{
match(input,POW,FOLLOW_POW_in_expression1349);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression1351);
expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1353);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 9 :
// org/jaitools/jiffle/parser/CheckAssignments.g:196:19: ^( PREFIX prefixOp expression )
{
match(input,PREFIX,FOLLOW_PREFIX_in_expression1375);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_prefixOp_in_expression1377);
prefixOp();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1379);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 10 :
// org/jaitools/jiffle/parser/CheckAssignments.g:197:19: ^( POSTFIX incdecOp expression )
{
match(input,POSTFIX,FOLLOW_POSTFIX_in_expression1401);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_incdecOp_in_expression1403);
incdecOp();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression1405);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 11 :
// org/jaitools/jiffle/parser/CheckAssignments.g:198:19: ^( PAR expression )
{
match(input,PAR,FOLLOW_PAR_in_expression1427);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression1429);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 12 :
// org/jaitools/jiffle/parser/CheckAssignments.g:199:19: listOperation
{
pushFollow(FOLLOW_listOperation_in_expression1450);
listOperation();
state._fsp--;
}
break;
case 13 :
// org/jaitools/jiffle/parser/CheckAssignments.g:200:19: listLiteral
{
pushFollow(FOLLOW_listLiteral_in_expression1470);
listLiteral();
state._fsp--;
}
break;
case 14 :
// org/jaitools/jiffle/parser/CheckAssignments.g:201:19: literal
{
pushFollow(FOLLOW_literal_in_expression1490);
literal();
state._fsp--;
}
break;
case 15 :
// org/jaitools/jiffle/parser/CheckAssignments.g:202:19: imageVar
{
pushFollow(FOLLOW_imageVar_in_expression1510);
imageVar();
state._fsp--;
}
break;
case 16 :
// org/jaitools/jiffle/parser/CheckAssignments.g:203:19: CONSTANT
{
match(input,CONSTANT,FOLLOW_CONSTANT_in_expression1530);
}
break;
case 17 :
// org/jaitools/jiffle/parser/CheckAssignments.g:205:19: userVar
{
pushFollow(FOLLOW_userVar_in_expression1551);
userVar5=userVar();
state._fsp--;
String varName = (userVar5!=null?((CommonTree)userVar5.start):null).getText();
int varType = (userVar5!=null?((CommonTree)userVar5.start):null).getType();
if (!varScope.isDefined(varName)) {
msgTable.add(varName, Message.UNINIT_VAR);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "expression"
// $ANTLR start "listOperation"
// org/jaitools/jiffle/parser/CheckAssignments.g:216:1: listOperation : ^( APPEND VAR_LIST expression ) ;
public final void listOperation() throws RecognitionException {
CommonTree VAR_LIST6=null;
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:216:17: ( ^( APPEND VAR_LIST expression ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:216:19: ^( APPEND VAR_LIST expression )
{
match(input,APPEND,FOLLOW_APPEND_in_listOperation1598);
match(input, Token.DOWN, null);
VAR_LIST6=(CommonTree)match(input,VAR_LIST,FOLLOW_VAR_LIST_in_listOperation1600);
pushFollow(FOLLOW_expression_in_listOperation1602);
expression();
state._fsp--;
match(input, Token.UP, null);
if (!varScope.isDefined((VAR_LIST6!=null?VAR_LIST6.getText():null))) {
msgTable.add((VAR_LIST6!=null?VAR_LIST6.getText():null), Message.UNDECLARED_LIST_VAR);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "listOperation"
// $ANTLR start "listLiteral"
// org/jaitools/jiffle/parser/CheckAssignments.g:225:1: listLiteral : ^( DECLARED_LIST expressionList ) ;
public final void listLiteral() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:225:17: ( ^( DECLARED_LIST expressionList ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:225:19: ^( DECLARED_LIST expressionList )
{
match(input,DECLARED_LIST,FOLLOW_DECLARED_LIST_in_listLiteral1652);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expressionList_in_listLiteral1654);
expressionList();
state._fsp--;
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "listLiteral"
// $ANTLR start "logicalOp"
// org/jaitools/jiffle/parser/CheckAssignments.g:229:1: logicalOp : ( OR | XOR | AND | LOGICALEQ | NE | GT | GE | LT | LE );
public final void logicalOp() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:229:17: ( OR | XOR | AND | LOGICALEQ | NE | GT | GE | LT | LE )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( (input.LA(1)>=OR && input.LA(1)<=LT) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "logicalOp"
// $ANTLR start "arithmeticOp"
// org/jaitools/jiffle/parser/CheckAssignments.g:241:1: arithmeticOp : ( PLUS | MINUS | TIMES | DIV | MOD );
public final void arithmeticOp() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:241:17: ( PLUS | MINUS | TIMES | DIV | MOD )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( (input.LA(1)>=PLUS && input.LA(1)<=MOD) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "arithmeticOp"
// $ANTLR start "prefixOp"
// org/jaitools/jiffle/parser/CheckAssignments.g:249:1: prefixOp : ( PLUS | MINUS | NOT | incdecOp );
public final void prefixOp() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:249:17: ( PLUS | MINUS | NOT | incdecOp )
int alt15=4;
switch ( input.LA(1) ) {
case PLUS:
{
alt15=1;
}
break;
case MINUS:
{
alt15=2;
}
break;
case NOT:
{
alt15=3;
}
break;
case INCR:
case DECR:
{
alt15=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 0, input);
throw nvae;
}
switch (alt15) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:249:19: PLUS
{
match(input,PLUS,FOLLOW_PLUS_in_prefixOp1989);
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:250:19: MINUS
{
match(input,MINUS,FOLLOW_MINUS_in_prefixOp2009);
}
break;
case 3 :
// org/jaitools/jiffle/parser/CheckAssignments.g:251:19: NOT
{
match(input,NOT,FOLLOW_NOT_in_prefixOp2029);
}
break;
case 4 :
// org/jaitools/jiffle/parser/CheckAssignments.g:252:19: incdecOp
{
pushFollow(FOLLOW_incdecOp_in_prefixOp2049);
incdecOp();
state._fsp--;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "prefixOp"
// $ANTLR start "incdecOp"
// org/jaitools/jiffle/parser/CheckAssignments.g:256:1: incdecOp : ( INCR | DECR );
public final void incdecOp() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:256:17: ( INCR | DECR )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( (input.LA(1)>=INCR && input.LA(1)<=DECR) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "incdecOp"
// $ANTLR start "pixelSpecifier"
// org/jaitools/jiffle/parser/CheckAssignments.g:261:1: pixelSpecifier : ^( PIXEL_REF pixelPos pixelPos ) ;
public final void pixelSpecifier() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:261:17: ( ^( PIXEL_REF pixelPos pixelPos ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:261:19: ^( PIXEL_REF pixelPos pixelPos )
{
match(input,PIXEL_REF,FOLLOW_PIXEL_REF_in_pixelSpecifier2130);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier2132);
pixelPos();
state._fsp--;
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier2134);
pixelPos();
state._fsp--;
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "pixelSpecifier"
// $ANTLR start "bandSpecifier"
// org/jaitools/jiffle/parser/CheckAssignments.g:265:1: bandSpecifier : ^( BAND_REF expression ) ;
public final void bandSpecifier() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:265:17: ( ^( BAND_REF expression ) )
// org/jaitools/jiffle/parser/CheckAssignments.g:265:19: ^( BAND_REF expression )
{
match(input,BAND_REF,FOLLOW_BAND_REF_in_bandSpecifier2164);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_bandSpecifier2166);
expression();
state._fsp--;
match(input, Token.UP, null);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "bandSpecifier"
// $ANTLR start "pixelPos"
// org/jaitools/jiffle/parser/CheckAssignments.g:269:1: pixelPos : ( ^( ABS_POS expression ) | ^( REL_POS expression ) );
public final void pixelPos() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:269:17: ( ^( ABS_POS expression ) | ^( REL_POS expression ) )
int alt16=2;
switch ( input.LA(1) ) {
case ABS_POS:
{
alt16=1;
}
break;
case REL_POS:
{
alt16=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 16, 0, input);
throw nvae;
}
switch (alt16) {
case 1 :
// org/jaitools/jiffle/parser/CheckAssignments.g:269:19: ^( ABS_POS expression )
{
match(input,ABS_POS,FOLLOW_ABS_POS_in_pixelPos2201);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_pixelPos2203);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/CheckAssignments.g:270:19: ^( REL_POS expression )
{
match(input,REL_POS,FOLLOW_REL_POS_in_pixelPos2225);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_pixelPos2227);
expression();
state._fsp--;
match(input, Token.UP, null);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "pixelPos"
// $ANTLR start "literal"
// org/jaitools/jiffle/parser/CheckAssignments.g:274:1: literal : ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL );
public final void literal() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckAssignments.g:274:17: ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL )
// org/jaitools/jiffle/parser/CheckAssignments.g:
{
if ( (input.LA(1)>=INT_LITERAL && input.LA(1)<=NULL) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "literal"
// Delegated rules
public static final BitSet FOLLOW_jiffleOption_in_start77 = new BitSet(new long[]{0xFFC6B20869F37BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_varDeclaration_in_start80 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_start83 = new BitSet(new long[]{0xFFC6B20869F35BC2L,0x00000000007C47FFL});
public static final BitSet FOLLOW_JIFFLE_OPTION_in_jiffleOption114 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_jiffleOption116 = new BitSet(new long[]{0xFFFFFFFFFFFFFFF0L,0x000000FFFFFFFFFFL});
public static final BitSet FOLLOW_DECL_in_varDeclaration147 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_DEST_in_varDeclaration149 = new BitSet(new long[]{0x0000000400000000L});
public static final BitSet FOLLOW_ID_in_varDeclaration151 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECL_in_varDeclaration173 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_SOURCE_in_varDeclaration175 = new BitSet(new long[]{0x0000000400000000L});
public static final BitSet FOLLOW_ID_in_varDeclaration177 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECL_in_varDeclaration200 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_IMAGE_SCOPE_in_varDeclaration202 = new BitSet(new long[]{0xFFFFFFFFFFFFFFF8L,0x000000FFFFFFFFFFL});
public static final BitSet FOLLOW_BLOCK_in_block261 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_statement_in_block263 = new BitSet(new long[]{0xFFC6B20869F35BC8L,0x00000000007C47FFL});
public static final BitSet FOLLOW_block_in_statement297 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ifCall_in_statement317 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assignmentExpression_in_statement337 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WHILE_in_statement358 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_loopCondition_in_statement360 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_statement362 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_UNTIL_in_statement384 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_loopCondition_in_statement386 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_statement388 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_foreachLoop_in_statement409 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_BREAKIF_in_statement430 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_statement432 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BREAK_in_statement453 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_statement473 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IF_in_ifCall509 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_ifCall511 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_ifCall513 = new BitSet(new long[]{0xFFC6B20869F35BC8L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_ifCall515 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FOREACH_in_foreachLoop570 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_foreachLoop572 = new BitSet(new long[]{0x0000000040080200L});
public static final BitSet FOLLOW_loopSet_in_foreachLoop576 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_statement_in_foreachLoop578 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_expression_in_loopCondition607 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEQUENCE_in_loopSet642 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_loopSet644 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_loopSet646 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_listLiteral_in_loopSet667 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_LIST_in_loopSet687 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EXPR_LIST_in_expressionList715 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expressionList717 = new BitSet(new long[]{0xFFC6B20869F35BC8L,0x00000000007C47FFL});
public static final BitSet FOLLOW_assignmentOp_in_assignmentExpression762 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_identifier_in_assignmentExpression764 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_assignmentExpression766 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_set_in_assignmentOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_imageVar_in_identifier945 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_userVar_in_identifier965 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONSTANT_in_identifier985 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_imageVar0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_userVar0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FUNC_CALL_in_expression1163 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_expression1165 = new BitSet(new long[]{0x0000000000000400L});
public static final BitSet FOLLOW_expressionList_in_expression1167 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_CON_CALL_in_expression1189 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expressionList_in_expression1191 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_QUESTION_in_expression1213 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1215 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1217 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1219 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_IMAGE_WRITE_in_expression1241 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1245 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_IMAGE_POS_in_expression1267 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_bandSpecifier_in_expression1271 = new BitSet(new long[]{0x0000000000008008L});
public static final BitSet FOLLOW_pixelSpecifier_in_expression1274 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_logicalOp_in_expression1297 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1299 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1301 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_arithmeticOp_in_expression1323 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1325 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1327 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_POW_in_expression1349 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1351 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1353 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PREFIX_in_expression1375 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_prefixOp_in_expression1377 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1379 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_POSTFIX_in_expression1401 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_incdecOp_in_expression1403 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_expression1405 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PAR_in_expression1427 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression1429 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_listOperation_in_expression1450 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listLiteral_in_expression1470 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_expression1490 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_imageVar_in_expression1510 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONSTANT_in_expression1530 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_userVar_in_expression1551 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_APPEND_in_listOperation1598 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_LIST_in_listOperation1600 = new BitSet(new long[]{0xFFC6B20869F35BC0L,0x00000000007C47FFL});
public static final BitSet FOLLOW_expression_in_listOperation1602 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECLARED_LIST_in_listLiteral1652 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expressionList_in_listLiteral1654 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_set_in_logicalOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_arithmeticOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PLUS_in_prefixOp1989 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MINUS_in_prefixOp2009 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_in_prefixOp2029 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_incdecOp_in_prefixOp2049 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_incdecOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PIXEL_REF_in_pixelSpecifier2130 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier2132 = new BitSet(new long[]{0x0000000000040010L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier2134 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BAND_REF_in_bandSpecifier2164 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_bandSpecifier2166 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ABS_POS_in_pixelPos2201 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_pixelPos2203 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_REL_POS_in_pixelPos2225 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_pixelPos2227 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_set_in_literal0 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy