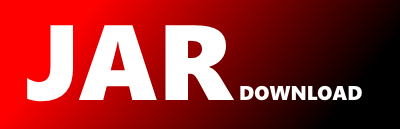
org.jaitools.jiffle.parser.CheckFunctionCalls Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/CheckFunctionCalls.g 2012-10-10 10:44:33
package org.jaitools.jiffle.parser;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
/**
* Checks function calls in the primary AST.
*
* @author Michael Bedward
*/
public class CheckFunctionCalls extends TreeFilter {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ABS_POS", "BAND_REF", "BLOCK", "CON_CALL", "DECL", "DECLARED_LIST", "EXPR_LIST", "FUNC_CALL", "IMAGE_POS", "JIFFLE_OPTION", "PAR", "PIXEL_REF", "POSTFIX", "PREFIX", "REL_POS", "SEQUENCE", "VAR_DEST", "VAR_IMAGE_SCOPE", "VAR_SOURCE", "CONSTANT", "IMAGE_WRITE", "LIST_NEW", "VAR_IMAGE", "VAR_PIXEL_SCOPE", "VAR_PROVIDED", "VAR_LOOP", "VAR_LIST", "OPTIONS", "LCURLY", "RCURLY", "ID", "EQ", "SEMI", "IMAGES", "READ", "WRITE", "INIT", "WHILE", "LPAR", "RPAR", "UNTIL", "FOREACH", "IN", "IF", "ELSE", "BREAKIF", "BREAK", "COMMA", "COLON", "CON", "APPEND", "TIMESEQ", "DIVEQ", "MODEQ", "PLUSEQ", "MINUSEQ", "QUESTION", "OR", "XOR", "AND", "LOGICALEQ", "NE", "GT", "GE", "LE", "LT", "PLUS", "MINUS", "TIMES", "DIV", "MOD", "NOT", "INCR", "DECR", "POW", "LSQUARE", "RSQUARE", "ABS_POS_PREFIX", "INT_LITERAL", "FLOAT_LITERAL", "TRUE", "FALSE", "NULL", "COMMENT", "INT_TYPE", "FLOAT_TYPE", "DOUBLE_TYPE", "BOOLEAN_TYPE", "Letter", "UNDERSCORE", "Digit", "Dot", "NonZeroDigit", "FloatExp", "WS", "ESC_SEQ", "CHAR", "HEX_DIGIT", "UNICODE_ESC", "OCTAL_ESC"
};
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public CheckFunctionCalls(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public CheckFunctionCalls(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return CheckFunctionCalls.tokenNames; }
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/CheckFunctionCalls.g"; }
private MessageTable msgTable;
public CheckFunctionCalls(TreeNodeStream input, MessageTable msgTable) {
this(input);
if (msgTable == null) {
throw new IllegalArgumentException( "msgTable should not be null" );
}
this.msgTable = msgTable;
}
// $ANTLR start "topdown"
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:53:1: topdown : functionCall ;
public final void topdown() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:53:9: ( functionCall )
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:53:11: functionCall
{
pushFollow(FOLLOW_functionCall_in_topdown71);
functionCall();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "topdown"
// $ANTLR start "functionCall"
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:57:1: functionCall : ^( FUNC_CALL ID expressionList ) ;
public final void functionCall() throws RecognitionException {
CommonTree ID1=null;
List expressionList2 = null;
try {
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:57:17: ( ^( FUNC_CALL ID expressionList ) )
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:57:19: ^( FUNC_CALL ID expressionList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_functionCall93); if (state.failed) return ;
match(input, Token.DOWN, null); if (state.failed) return ;
ID1=(CommonTree)match(input,ID,FOLLOW_ID_in_functionCall95); if (state.failed) return ;
pushFollow(FOLLOW_expressionList_in_functionCall97);
expressionList2=expressionList();
state._fsp--;
if (state.failed) return ;
match(input, Token.UP, null); if (state.failed) return ;
if ( state.backtracking==1 ) {
if (!FunctionLookup.isDefined((ID1!=null?ID1.getText():null), expressionList2)) {
StringBuilder sb = new StringBuilder();
sb.append((ID1!=null?ID1.getText():null));
sb.append("(");
int k = 0;
for (String s : expressionList2) {
sb.append(s);
if (++k < expressionList2.size()) {
sb.append(", ");
}
}
sb.append(")");
msgTable.add(sb.toString(), Message.UNDEFINED_FUNCTION);
}
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "functionCall"
// $ANTLR start "expressionList"
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:77:1: expressionList returns [List argTypes] : ^( EXPR_LIST (e= . )* ) ;
public final List expressionList() throws RecognitionException {
List argTypes = null;
CommonTree e=null;
argTypes = new ArrayList();
try {
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:79:17: ( ^( EXPR_LIST (e= . )* ) )
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:79:19: ^( EXPR_LIST (e= . )* )
{
match(input,EXPR_LIST,FOLLOW_EXPR_LIST_in_expressionList167); if (state.failed) return argTypes;
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null); if (state.failed) return argTypes;
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:79:31: (e= . )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case ABS_POS:
case BAND_REF:
case BLOCK:
case CON_CALL:
case DECL:
case DECLARED_LIST:
case EXPR_LIST:
case FUNC_CALL:
case IMAGE_POS:
case JIFFLE_OPTION:
case PAR:
case PIXEL_REF:
case POSTFIX:
case PREFIX:
case REL_POS:
case SEQUENCE:
case VAR_DEST:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case LIST_NEW:
case VAR_IMAGE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case OPTIONS:
case LCURLY:
case RCURLY:
case ID:
case EQ:
case SEMI:
case IMAGES:
case READ:
case WRITE:
case INIT:
case WHILE:
case LPAR:
case RPAR:
case UNTIL:
case FOREACH:
case IN:
case IF:
case ELSE:
case BREAKIF:
case BREAK:
case COMMA:
case COLON:
case CON:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case NOT:
case INCR:
case DECR:
case POW:
case LSQUARE:
case RSQUARE:
case ABS_POS_PREFIX:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
case COMMENT:
case INT_TYPE:
case FLOAT_TYPE:
case DOUBLE_TYPE:
case BOOLEAN_TYPE:
case Letter:
case UNDERSCORE:
case Digit:
case Dot:
case NonZeroDigit:
case FloatExp:
case WS:
case ESC_SEQ:
case CHAR:
case HEX_DIGIT:
case UNICODE_ESC:
case OCTAL_ESC:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/CheckFunctionCalls.g:79:32: e= .
{
e=(CommonTree)input.LT(1);
matchAny(input); if (state.failed) return argTypes;
if ( state.backtracking==1 ) {
int ttype = e.getToken().getType();
argTypes.add(ttype == VAR_LIST || ttype == DECLARED_LIST ? "List" : "D");
}
}
break;
default :
break loop1;
}
} while (true);
match(input, Token.UP, null); if (state.failed) return argTypes;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return argTypes;
}
// $ANTLR end "expressionList"
// Delegated rules
public static final BitSet FOLLOW_functionCall_in_topdown71 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FUNC_CALL_in_functionCall93 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_functionCall95 = new BitSet(new long[]{0x0000000000000400L});
public static final BitSet FOLLOW_expressionList_in_functionCall97 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_EXPR_LIST_in_expressionList167 = new BitSet(new long[]{0x0000000000000004L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy