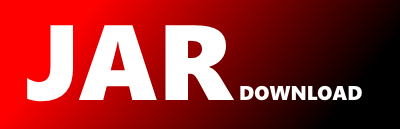
org.jaitools.jiffle.parser.CommentFinder Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/CommentFinder.g 2012-10-10 10:44:32
package org.jaitools.jiffle.parser;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
public class CommentFinder extends Lexer {
public static final int EOF=-1;
public static final int COMMENT=4;
private boolean lineComment = false;
// start : end pairs
private List commentIndices = new ArrayList();
public List getStartEndIndices() { return commentIndices; }
// delegates
// delegators
public CommentFinder() {;}
public CommentFinder(CharStream input) {
this(input, new RecognizerSharedState());
}
public CommentFinder(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/CommentFinder.g"; }
public Token nextToken() {
while (true) {
if ( input.LA(1)==CharStream.EOF ) {
Token eof = new CommonToken((CharStream)input,Token.EOF,
Token.DEFAULT_CHANNEL,
input.index(),input.index());
eof.setLine(getLine());
eof.setCharPositionInLine(getCharPositionInLine());
return eof;
}
state.token = null;
state.channel = Token.DEFAULT_CHANNEL;
state.tokenStartCharIndex = input.index();
state.tokenStartCharPositionInLine = input.getCharPositionInLine();
state.tokenStartLine = input.getLine();
state.text = null;
try {
int m = input.mark();
state.backtracking=1;
state.failed=false;
mTokens();
state.backtracking=0;
if ( state.failed ) {
input.rewind(m);
input.consume();
}
else {
emit();
return state.token;
}
}
catch (RecognitionException re) {
// shouldn't happen in backtracking mode, but...
reportError(re);
recover(re);
}
}
}
public void memoize(IntStream input,
int ruleIndex,
int ruleStartIndex)
{
if ( state.backtracking>1 ) super.memoize(input, ruleIndex, ruleStartIndex);
}
public boolean alreadyParsedRule(IntStream input, int ruleIndex) {
if ( state.backtracking>1 ) return super.alreadyParsedRule(input, ruleIndex);
return false;
}// $ANTLR start "COMMENT"
public final void mCOMMENT() throws RecognitionException {
try {
int _type = COMMENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/CommentFinder.g:30:5: ( '//' (~ ( '\\n' | '\\r' ) )* ( '\\r' )? '\\n' | '/*' ( options {greedy=false; } : . )* '*/' )
int alt4=2;
switch ( input.LA(1) ) {
case '/':
{
switch ( input.LA(2) ) {
case '/':
{
alt4=1;
}
break;
case '*':
{
alt4=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 4, 1, input);
throw nvae;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return ;}
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// org/jaitools/jiffle/parser/CommentFinder.g:30:9: '//' (~ ( '\\n' | '\\r' ) )* ( '\\r' )? '\\n'
{
match("//"); if (state.failed) return ;
// org/jaitools/jiffle/parser/CommentFinder.g:30:14: (~ ( '\\n' | '\\r' ) )*
loop1:
do {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0>='\u0000' && LA1_0<='\t')||(LA1_0>='\u000B' && LA1_0<='\f')||(LA1_0>='\u000E' && LA1_0<='\uFFFF')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/CommentFinder.g:30:14: ~ ( '\\n' | '\\r' )
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='\t')||(input.LA(1)>='\u000B' && input.LA(1)<='\f')||(input.LA(1)>='\u000E' && input.LA(1)<='\uFFFF') ) {
input.consume();
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return ;}
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
break loop1;
}
} while (true);
// org/jaitools/jiffle/parser/CommentFinder.g:30:28: ( '\\r' )?
int alt2=2;
switch ( input.LA(1) ) {
case '\r':
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// org/jaitools/jiffle/parser/CommentFinder.g:30:28: '\\r'
{
match('\r'); if (state.failed) return ;
}
break;
}
match('\n'); if (state.failed) return ;
if ( state.backtracking==1 ) {
lineComment = true;
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/CommentFinder.g:31:9: '/*' ( options {greedy=false; } : . )* '*/'
{
match("/*"); if (state.failed) return ;
// org/jaitools/jiffle/parser/CommentFinder.g:31:14: ( options {greedy=false; } : . )*
loop3:
do {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0=='*') ) {
int LA3_1 = input.LA(2);
if ( (LA3_1=='/') ) {
alt3=2;
}
else if ( ((LA3_1>='\u0000' && LA3_1<='.')||(LA3_1>='0' && LA3_1<='\uFFFF')) ) {
alt3=1;
}
}
else if ( ((LA3_0>='\u0000' && LA3_0<=')')||(LA3_0>='+' && LA3_0<='\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// org/jaitools/jiffle/parser/CommentFinder.g:31:42: .
{
matchAny(); if (state.failed) return ;
}
break;
default :
break loop3;
}
} while (true);
match("*/"); if (state.failed) return ;
if ( state.backtracking==1 ) {
lineComment = false;
}
}
break;
}
state.type = _type;
state.channel = _channel;
if ( state.backtracking==1 ) {
String s = getText();
int endIndex = getCharIndex();
int startIndex = endIndex - s.length();
if (lineComment) endIndex -= 1;
commentIndices.add(startIndex);
commentIndices.add(endIndex);
} }
finally {
}
}
// $ANTLR end "COMMENT"
public void mTokens() throws RecognitionException {
// org/jaitools/jiffle/parser/CommentFinder.g:1:39: ( COMMENT )
// org/jaitools/jiffle/parser/CommentFinder.g:1:41: COMMENT
{
mCOMMENT(); if (state.failed) return ;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy