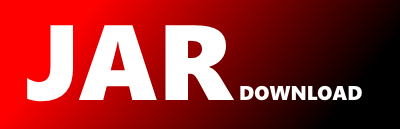
org.jaitools.jiffle.parser.JiffleLexer Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/Jiffle.g 2012-10-10 10:44:31
package org.jaitools.jiffle.parser;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
public class JiffleLexer extends Lexer {
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public JiffleLexer() {;}
public JiffleLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public JiffleLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/Jiffle.g"; }
// $ANTLR start "COMMENT"
public final void mCOMMENT() throws RecognitionException {
try {
int _type = COMMENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:373:5: ( '//' (~ ( '\\n' | '\\r' ) )* ( '\\r' )? '\\n' | '/*' ( options {greedy=false; } : . )* '*/' )
int alt4=2;
switch ( input.LA(1) ) {
case '/':
{
switch ( input.LA(2) ) {
case '/':
{
alt4=1;
}
break;
case '*':
{
alt4=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:373:9: '//' (~ ( '\\n' | '\\r' ) )* ( '\\r' )? '\\n'
{
match("//");
// org/jaitools/jiffle/parser/Jiffle.g:373:14: (~ ( '\\n' | '\\r' ) )*
loop1:
do {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0>='\u0000' && LA1_0<='\t')||(LA1_0>='\u000B' && LA1_0<='\f')||(LA1_0>='\u000E' && LA1_0<='\uFFFF')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:373:14: ~ ( '\\n' | '\\r' )
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='\t')||(input.LA(1)>='\u000B' && input.LA(1)<='\f')||(input.LA(1)>='\u000E' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
break loop1;
}
} while (true);
// org/jaitools/jiffle/parser/Jiffle.g:373:28: ( '\\r' )?
int alt2=2;
switch ( input.LA(1) ) {
case '\r':
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:373:28: '\\r'
{
match('\r');
}
break;
}
match('\n');
_channel=HIDDEN;
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:374:9: '/*' ( options {greedy=false; } : . )* '*/'
{
match("/*");
// org/jaitools/jiffle/parser/Jiffle.g:374:14: ( options {greedy=false; } : . )*
loop3:
do {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0=='*') ) {
int LA3_1 = input.LA(2);
if ( (LA3_1=='/') ) {
alt3=2;
}
else if ( ((LA3_1>='\u0000' && LA3_1<='.')||(LA3_1>='0' && LA3_1<='\uFFFF')) ) {
alt3=1;
}
}
else if ( ((LA3_0>='\u0000' && LA3_0<=')')||(LA3_0>='+' && LA3_0<='\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:374:42: .
{
matchAny();
}
break;
default :
break loop3;
}
} while (true);
match("*/");
_channel=HIDDEN;
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "COMMENT"
// $ANTLR start "TRUE"
public final void mTRUE() throws RecognitionException {
try {
int _type = TRUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:378:9: ( 'TRUE' | 'true' )
int alt5=2;
switch ( input.LA(1) ) {
case 'T':
{
alt5=1;
}
break;
case 't':
{
alt5=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:378:11: 'TRUE'
{
match("TRUE");
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:378:20: 'true'
{
match("true");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "TRUE"
// $ANTLR start "FALSE"
public final void mFALSE() throws RecognitionException {
try {
int _type = FALSE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:379:9: ( 'FALSE' | 'false' )
int alt6=2;
switch ( input.LA(1) ) {
case 'F':
{
alt6=1;
}
break;
case 'f':
{
alt6=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:379:11: 'FALSE'
{
match("FALSE");
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:379:21: 'false'
{
match("false");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "FALSE"
// $ANTLR start "NULL"
public final void mNULL() throws RecognitionException {
try {
int _type = NULL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:380:9: ( 'NULL' | 'null' )
int alt7=2;
switch ( input.LA(1) ) {
case 'N':
{
alt7=1;
}
break;
case 'n':
{
alt7=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:380:11: 'NULL'
{
match("NULL");
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:380:20: 'null'
{
match("null");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "NULL"
// $ANTLR start "INT_TYPE"
public final void mINT_TYPE() throws RecognitionException {
try {
int _type = INT_TYPE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:383:17: ( 'int' )
// org/jaitools/jiffle/parser/Jiffle.g:383:19: 'int'
{
match("int");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "INT_TYPE"
// $ANTLR start "FLOAT_TYPE"
public final void mFLOAT_TYPE() throws RecognitionException {
try {
int _type = FLOAT_TYPE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:384:17: ( 'float' )
// org/jaitools/jiffle/parser/Jiffle.g:384:19: 'float'
{
match("float");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "FLOAT_TYPE"
// $ANTLR start "DOUBLE_TYPE"
public final void mDOUBLE_TYPE() throws RecognitionException {
try {
int _type = DOUBLE_TYPE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:385:17: ( 'double' )
// org/jaitools/jiffle/parser/Jiffle.g:385:19: 'double'
{
match("double");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "DOUBLE_TYPE"
// $ANTLR start "BOOLEAN_TYPE"
public final void mBOOLEAN_TYPE() throws RecognitionException {
try {
int _type = BOOLEAN_TYPE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:386:17: ( 'boolean' )
// org/jaitools/jiffle/parser/Jiffle.g:386:19: 'boolean'
{
match("boolean");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "BOOLEAN_TYPE"
// $ANTLR start "OPTIONS"
public final void mOPTIONS() throws RecognitionException {
try {
int _type = OPTIONS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:388:9: ( 'options' )
// org/jaitools/jiffle/parser/Jiffle.g:388:11: 'options'
{
match("options");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "OPTIONS"
// $ANTLR start "IMAGES"
public final void mIMAGES() throws RecognitionException {
try {
int _type = IMAGES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:389:9: ( 'images' )
// org/jaitools/jiffle/parser/Jiffle.g:389:11: 'images'
{
match("images");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "IMAGES"
// $ANTLR start "INIT"
public final void mINIT() throws RecognitionException {
try {
int _type = INIT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:390:9: ( 'init' )
// org/jaitools/jiffle/parser/Jiffle.g:390:11: 'init'
{
match("init");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "INIT"
// $ANTLR start "READ"
public final void mREAD() throws RecognitionException {
try {
int _type = READ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:391:9: ( 'read' )
// org/jaitools/jiffle/parser/Jiffle.g:391:11: 'read'
{
match("read");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "READ"
// $ANTLR start "WRITE"
public final void mWRITE() throws RecognitionException {
try {
int _type = WRITE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:392:9: ( 'write' )
// org/jaitools/jiffle/parser/Jiffle.g:392:11: 'write'
{
match("write");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "WRITE"
// $ANTLR start "CON"
public final void mCON() throws RecognitionException {
try {
int _type = CON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:394:9: ( 'con' )
// org/jaitools/jiffle/parser/Jiffle.g:394:11: 'con'
{
match("con");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "CON"
// $ANTLR start "IF"
public final void mIF() throws RecognitionException {
try {
int _type = IF;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:395:9: ( 'if' )
// org/jaitools/jiffle/parser/Jiffle.g:395:11: 'if'
{
match("if");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "IF"
// $ANTLR start "ELSE"
public final void mELSE() throws RecognitionException {
try {
int _type = ELSE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:396:9: ( 'else' )
// org/jaitools/jiffle/parser/Jiffle.g:396:11: 'else'
{
match("else");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "ELSE"
// $ANTLR start "WHILE"
public final void mWHILE() throws RecognitionException {
try {
int _type = WHILE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:397:9: ( 'while' )
// org/jaitools/jiffle/parser/Jiffle.g:397:11: 'while'
{
match("while");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "WHILE"
// $ANTLR start "UNTIL"
public final void mUNTIL() throws RecognitionException {
try {
int _type = UNTIL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:398:9: ( 'until' )
// org/jaitools/jiffle/parser/Jiffle.g:398:11: 'until'
{
match("until");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "UNTIL"
// $ANTLR start "FOREACH"
public final void mFOREACH() throws RecognitionException {
try {
int _type = FOREACH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:399:9: ( 'foreach' )
// org/jaitools/jiffle/parser/Jiffle.g:399:11: 'foreach'
{
match("foreach");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "FOREACH"
// $ANTLR start "IN"
public final void mIN() throws RecognitionException {
try {
int _type = IN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:400:9: ( 'in' )
// org/jaitools/jiffle/parser/Jiffle.g:400:11: 'in'
{
match("in");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "IN"
// $ANTLR start "BREAKIF"
public final void mBREAKIF() throws RecognitionException {
try {
int _type = BREAKIF;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:401:9: ( 'breakif' )
// org/jaitools/jiffle/parser/Jiffle.g:401:11: 'breakif'
{
match("breakif");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "BREAKIF"
// $ANTLR start "BREAK"
public final void mBREAK() throws RecognitionException {
try {
int _type = BREAK;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:402:9: ( 'break' )
// org/jaitools/jiffle/parser/Jiffle.g:402:11: 'break'
{
match("break");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "BREAK"
// $ANTLR start "ABS_POS_PREFIX"
public final void mABS_POS_PREFIX() throws RecognitionException {
try {
int _type = ABS_POS_PREFIX;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:407:9: ( '$' )
// org/jaitools/jiffle/parser/Jiffle.g:407:11: '$'
{
match('$');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "ABS_POS_PREFIX"
// $ANTLR start "APPEND"
public final void mAPPEND() throws RecognitionException {
try {
int _type = APPEND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:409:9: ( '<<' )
// org/jaitools/jiffle/parser/Jiffle.g:409:11: '<<'
{
match("<<");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "APPEND"
// $ANTLR start "INCR"
public final void mINCR() throws RecognitionException {
try {
int _type = INCR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:411:9: ( '++' )
// org/jaitools/jiffle/parser/Jiffle.g:411:11: '++'
{
match("++");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "INCR"
// $ANTLR start "DECR"
public final void mDECR() throws RecognitionException {
try {
int _type = DECR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:412:9: ( '--' )
// org/jaitools/jiffle/parser/Jiffle.g:412:11: '--'
{
match("--");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "DECR"
// $ANTLR start "NOT"
public final void mNOT() throws RecognitionException {
try {
int _type = NOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:414:9: ( '!' )
// org/jaitools/jiffle/parser/Jiffle.g:414:11: '!'
{
match('!');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "NOT"
// $ANTLR start "POW"
public final void mPOW() throws RecognitionException {
try {
int _type = POW;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:415:9: ( '^' )
// org/jaitools/jiffle/parser/Jiffle.g:415:11: '^'
{
match('^');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "POW"
// $ANTLR start "TIMES"
public final void mTIMES() throws RecognitionException {
try {
int _type = TIMES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:416:9: ( '*' )
// org/jaitools/jiffle/parser/Jiffle.g:416:11: '*'
{
match('*');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "TIMES"
// $ANTLR start "DIV"
public final void mDIV() throws RecognitionException {
try {
int _type = DIV;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:417:9: ( '/' )
// org/jaitools/jiffle/parser/Jiffle.g:417:11: '/'
{
match('/');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "DIV"
// $ANTLR start "MOD"
public final void mMOD() throws RecognitionException {
try {
int _type = MOD;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:418:9: ( '%' )
// org/jaitools/jiffle/parser/Jiffle.g:418:11: '%'
{
match('%');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "MOD"
// $ANTLR start "PLUS"
public final void mPLUS() throws RecognitionException {
try {
int _type = PLUS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:419:9: ( '+' )
// org/jaitools/jiffle/parser/Jiffle.g:419:11: '+'
{
match('+');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "PLUS"
// $ANTLR start "MINUS"
public final void mMINUS() throws RecognitionException {
try {
int _type = MINUS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:420:9: ( '-' )
// org/jaitools/jiffle/parser/Jiffle.g:420:11: '-'
{
match('-');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "MINUS"
// $ANTLR start "GT"
public final void mGT() throws RecognitionException {
try {
int _type = GT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:421:9: ( '>' )
// org/jaitools/jiffle/parser/Jiffle.g:421:11: '>'
{
match('>');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "GT"
// $ANTLR start "GE"
public final void mGE() throws RecognitionException {
try {
int _type = GE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:422:9: ( '>=' )
// org/jaitools/jiffle/parser/Jiffle.g:422:11: '>='
{
match(">=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "GE"
// $ANTLR start "LE"
public final void mLE() throws RecognitionException {
try {
int _type = LE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:423:9: ( '<=' )
// org/jaitools/jiffle/parser/Jiffle.g:423:11: '<='
{
match("<=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LE"
// $ANTLR start "LT"
public final void mLT() throws RecognitionException {
try {
int _type = LT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:424:9: ( '<' )
// org/jaitools/jiffle/parser/Jiffle.g:424:11: '<'
{
match('<');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LT"
// $ANTLR start "LOGICALEQ"
public final void mLOGICALEQ() throws RecognitionException {
try {
int _type = LOGICALEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:425:11: ( '==' )
// org/jaitools/jiffle/parser/Jiffle.g:425:13: '=='
{
match("==");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LOGICALEQ"
// $ANTLR start "NE"
public final void mNE() throws RecognitionException {
try {
int _type = NE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:426:9: ( '!=' )
// org/jaitools/jiffle/parser/Jiffle.g:426:11: '!='
{
match("!=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "NE"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:427:9: ( '&&' )
// org/jaitools/jiffle/parser/Jiffle.g:427:11: '&&'
{
match("&&");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "AND"
// $ANTLR start "OR"
public final void mOR() throws RecognitionException {
try {
int _type = OR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:428:9: ( '||' )
// org/jaitools/jiffle/parser/Jiffle.g:428:11: '||'
{
match("||");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "OR"
// $ANTLR start "XOR"
public final void mXOR() throws RecognitionException {
try {
int _type = XOR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:429:9: ( '^|' )
// org/jaitools/jiffle/parser/Jiffle.g:429:11: '^|'
{
match("^|");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "XOR"
// $ANTLR start "QUESTION"
public final void mQUESTION() throws RecognitionException {
try {
int _type = QUESTION;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:430:9: ( '?' )
// org/jaitools/jiffle/parser/Jiffle.g:430:11: '?'
{
match('?');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "QUESTION"
// $ANTLR start "TIMESEQ"
public final void mTIMESEQ() throws RecognitionException {
try {
int _type = TIMESEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:431:9: ( '*=' )
// org/jaitools/jiffle/parser/Jiffle.g:431:11: '*='
{
match("*=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "TIMESEQ"
// $ANTLR start "DIVEQ"
public final void mDIVEQ() throws RecognitionException {
try {
int _type = DIVEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:432:9: ( '/=' )
// org/jaitools/jiffle/parser/Jiffle.g:432:11: '/='
{
match("/=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "DIVEQ"
// $ANTLR start "MODEQ"
public final void mMODEQ() throws RecognitionException {
try {
int _type = MODEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:433:9: ( '%=' )
// org/jaitools/jiffle/parser/Jiffle.g:433:11: '%='
{
match("%=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "MODEQ"
// $ANTLR start "PLUSEQ"
public final void mPLUSEQ() throws RecognitionException {
try {
int _type = PLUSEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:434:9: ( '+=' )
// org/jaitools/jiffle/parser/Jiffle.g:434:11: '+='
{
match("+=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "PLUSEQ"
// $ANTLR start "MINUSEQ"
public final void mMINUSEQ() throws RecognitionException {
try {
int _type = MINUSEQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:435:9: ( '-=' )
// org/jaitools/jiffle/parser/Jiffle.g:435:11: '-='
{
match("-=");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "MINUSEQ"
// $ANTLR start "EQ"
public final void mEQ() throws RecognitionException {
try {
int _type = EQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:436:9: ( '=' )
// org/jaitools/jiffle/parser/Jiffle.g:436:11: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "EQ"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:439:9: ( ',' )
// org/jaitools/jiffle/parser/Jiffle.g:439:11: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "COMMA"
// $ANTLR start "SEMI"
public final void mSEMI() throws RecognitionException {
try {
int _type = SEMI;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:440:9: ( ';' )
// org/jaitools/jiffle/parser/Jiffle.g:440:11: ';'
{
match(';');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "SEMI"
// $ANTLR start "COLON"
public final void mCOLON() throws RecognitionException {
try {
int _type = COLON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:441:9: ( ':' )
// org/jaitools/jiffle/parser/Jiffle.g:441:11: ':'
{
match(':');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "COLON"
// $ANTLR start "LPAR"
public final void mLPAR() throws RecognitionException {
try {
int _type = LPAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:442:9: ( '(' )
// org/jaitools/jiffle/parser/Jiffle.g:442:11: '('
{
match('(');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LPAR"
// $ANTLR start "RPAR"
public final void mRPAR() throws RecognitionException {
try {
int _type = RPAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:443:9: ( ')' )
// org/jaitools/jiffle/parser/Jiffle.g:443:11: ')'
{
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "RPAR"
// $ANTLR start "LSQUARE"
public final void mLSQUARE() throws RecognitionException {
try {
int _type = LSQUARE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:444:9: ( '[' )
// org/jaitools/jiffle/parser/Jiffle.g:444:11: '['
{
match('[');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LSQUARE"
// $ANTLR start "RSQUARE"
public final void mRSQUARE() throws RecognitionException {
try {
int _type = RSQUARE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:445:9: ( ']' )
// org/jaitools/jiffle/parser/Jiffle.g:445:11: ']'
{
match(']');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "RSQUARE"
// $ANTLR start "LCURLY"
public final void mLCURLY() throws RecognitionException {
try {
int _type = LCURLY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:446:9: ( '{' )
// org/jaitools/jiffle/parser/Jiffle.g:446:11: '{'
{
match('{');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LCURLY"
// $ANTLR start "RCURLY"
public final void mRCURLY() throws RecognitionException {
try {
int _type = RCURLY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:447:9: ( '}' )
// org/jaitools/jiffle/parser/Jiffle.g:447:11: '}'
{
match('}');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "RCURLY"
// $ANTLR start "ID"
public final void mID() throws RecognitionException {
try {
int _type = ID;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:449:9: ( ( Letter ) ( Letter | UNDERSCORE | Digit | Dot )* )
// org/jaitools/jiffle/parser/Jiffle.g:449:11: ( Letter ) ( Letter | UNDERSCORE | Digit | Dot )*
{
// org/jaitools/jiffle/parser/Jiffle.g:449:11: ( Letter )
// org/jaitools/jiffle/parser/Jiffle.g:449:12: Letter
{
mLetter();
}
// org/jaitools/jiffle/parser/Jiffle.g:449:20: ( Letter | UNDERSCORE | Digit | Dot )*
loop8:
do {
int alt8=2;
switch ( input.LA(1) ) {
case '.':
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
case '_':
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'o':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'u':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:
{
if ( input.LA(1)=='.'||(input.LA(1)>='0' && input.LA(1)<='9')||(input.LA(1)>='A' && input.LA(1)<='Z')||input.LA(1)=='_'||(input.LA(1)>='a' && input.LA(1)<='z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
break loop8;
}
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "ID"
// $ANTLR start "Letter"
public final void mLetter() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:454:9: ( 'a' .. 'z' | 'A' .. 'Z' )
// org/jaitools/jiffle/parser/Jiffle.g:
{
if ( (input.LA(1)>='A' && input.LA(1)<='Z')||(input.LA(1)>='a' && input.LA(1)<='z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
}
finally {
}
}
// $ANTLR end "Letter"
// $ANTLR start "UNDERSCORE"
public final void mUNDERSCORE() throws RecognitionException {
try {
int _type = UNDERSCORE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:458:9: ( '_' )
// org/jaitools/jiffle/parser/Jiffle.g:458:11: '_'
{
match('_');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "UNDERSCORE"
// $ANTLR start "INT_LITERAL"
public final void mINT_LITERAL() throws RecognitionException {
try {
int _type = INT_LITERAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:461:9: ( '0' | NonZeroDigit ( Digit )* )
int alt10=2;
switch ( input.LA(1) ) {
case '0':
{
alt10=1;
}
break;
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt10=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 10, 0, input);
throw nvae;
}
switch (alt10) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:461:11: '0'
{
match('0');
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:461:17: NonZeroDigit ( Digit )*
{
mNonZeroDigit();
// org/jaitools/jiffle/parser/Jiffle.g:461:30: ( Digit )*
loop9:
do {
int alt9=2;
switch ( input.LA(1) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt9=1;
}
break;
}
switch (alt9) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:461:30: Digit
{
mDigit();
}
break;
default :
break loop9;
}
} while (true);
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "INT_LITERAL"
// $ANTLR start "FLOAT_LITERAL"
public final void mFLOAT_LITERAL() throws RecognitionException {
try {
int _type = FLOAT_LITERAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:465:9: ( ( '0' | NonZeroDigit ( Digit )* )? Dot ( Digit )* ( FloatExp )? )
// org/jaitools/jiffle/parser/Jiffle.g:465:11: ( '0' | NonZeroDigit ( Digit )* )? Dot ( Digit )* ( FloatExp )?
{
// org/jaitools/jiffle/parser/Jiffle.g:465:11: ( '0' | NonZeroDigit ( Digit )* )?
int alt12=3;
switch ( input.LA(1) ) {
case '0':
{
alt12=1;
}
break;
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt12=2;
}
break;
}
switch (alt12) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:465:12: '0'
{
match('0');
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:465:18: NonZeroDigit ( Digit )*
{
mNonZeroDigit();
// org/jaitools/jiffle/parser/Jiffle.g:465:31: ( Digit )*
loop11:
do {
int alt11=2;
switch ( input.LA(1) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt11=1;
}
break;
}
switch (alt11) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:465:31: Digit
{
mDigit();
}
break;
default :
break loop11;
}
} while (true);
}
break;
}
mDot();
// org/jaitools/jiffle/parser/Jiffle.g:465:44: ( Digit )*
loop13:
do {
int alt13=2;
switch ( input.LA(1) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt13=1;
}
break;
}
switch (alt13) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:465:44: Digit
{
mDigit();
}
break;
default :
break loop13;
}
} while (true);
// org/jaitools/jiffle/parser/Jiffle.g:465:51: ( FloatExp )?
int alt14=2;
switch ( input.LA(1) ) {
case 'E':
case 'e':
{
alt14=1;
}
break;
}
switch (alt14) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:465:51: FloatExp
{
mFloatExp();
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "FLOAT_LITERAL"
// $ANTLR start "Digit"
public final void mDigit() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:469:9: ( '0' .. '9' )
// org/jaitools/jiffle/parser/Jiffle.g:469:11: '0' .. '9'
{
matchRange('0','9');
}
}
finally {
}
}
// $ANTLR end "Digit"
// $ANTLR start "Dot"
public final void mDot() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:472:9: ( '.' )
// org/jaitools/jiffle/parser/Jiffle.g:472:11: '.'
{
match('.');
}
}
finally {
}
}
// $ANTLR end "Dot"
// $ANTLR start "NonZeroDigit"
public final void mNonZeroDigit() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:476:9: ( '1' .. '9' )
// org/jaitools/jiffle/parser/Jiffle.g:476:11: '1' .. '9'
{
matchRange('1','9');
}
}
finally {
}
}
// $ANTLR end "NonZeroDigit"
// $ANTLR start "FloatExp"
public final void mFloatExp() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:481:9: ( ( 'e' | 'E' ( PLUS | MINUS )? ( '0' .. '9' )+ ) )
// org/jaitools/jiffle/parser/Jiffle.g:481:11: ( 'e' | 'E' ( PLUS | MINUS )? ( '0' .. '9' )+ )
{
// org/jaitools/jiffle/parser/Jiffle.g:481:11: ( 'e' | 'E' ( PLUS | MINUS )? ( '0' .. '9' )+ )
int alt17=2;
switch ( input.LA(1) ) {
case 'e':
{
alt17=1;
}
break;
case 'E':
{
alt17=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 17, 0, input);
throw nvae;
}
switch (alt17) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:481:12: 'e'
{
match('e');
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:481:16: 'E' ( PLUS | MINUS )? ( '0' .. '9' )+
{
match('E');
// org/jaitools/jiffle/parser/Jiffle.g:481:20: ( PLUS | MINUS )?
int alt15=2;
switch ( input.LA(1) ) {
case '+':
case '-':
{
alt15=1;
}
break;
}
switch (alt15) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
}
// org/jaitools/jiffle/parser/Jiffle.g:481:34: ( '0' .. '9' )+
int cnt16=0;
loop16:
do {
int alt16=2;
switch ( input.LA(1) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
{
alt16=1;
}
break;
}
switch (alt16) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:481:34: '0' .. '9'
{
matchRange('0','9');
}
break;
default :
if ( cnt16 >= 1 ) break loop16;
EarlyExitException eee =
new EarlyExitException(16, input);
throw eee;
}
cnt16++;
} while (true);
}
break;
}
}
}
finally {
}
}
// $ANTLR end "FloatExp"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:484:5: ( ( ' ' | '\\t' | '\\r' | '\\n' | '\\u000C' ) )
// org/jaitools/jiffle/parser/Jiffle.g:484:9: ( ' ' | '\\t' | '\\r' | '\\n' | '\\u000C' )
{
if ( (input.LA(1)>='\t' && input.LA(1)<='\n')||(input.LA(1)>='\f' && input.LA(1)<='\r')||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
_channel=HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "WS"
// $ANTLR start "CHAR"
public final void mCHAR() throws RecognitionException {
try {
int _type = CHAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/jaitools/jiffle/parser/Jiffle.g:497:5: ( '\\'' ( ESC_SEQ | ~ ( '\\'' | '\\\\' ) ) '\\'' )
// org/jaitools/jiffle/parser/Jiffle.g:497:8: '\\'' ( ESC_SEQ | ~ ( '\\'' | '\\\\' ) ) '\\''
{
match('\'');
// org/jaitools/jiffle/parser/Jiffle.g:497:13: ( ESC_SEQ | ~ ( '\\'' | '\\\\' ) )
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0=='\\') ) {
alt18=1;
}
else if ( ((LA18_0>='\u0000' && LA18_0<='&')||(LA18_0>='(' && LA18_0<='[')||(LA18_0>=']' && LA18_0<='\uFFFF')) ) {
alt18=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 18, 0, input);
throw nvae;
}
switch (alt18) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:497:15: ESC_SEQ
{
mESC_SEQ();
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:497:25: ~ ( '\\'' | '\\\\' )
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='&')||(input.LA(1)>='(' && input.LA(1)<='[')||(input.LA(1)>=']' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
}
match('\'');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "CHAR"
// $ANTLR start "HEX_DIGIT"
public final void mHEX_DIGIT() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:501:11: ( ( '0' .. '9' | 'a' .. 'f' | 'A' .. 'F' ) )
// org/jaitools/jiffle/parser/Jiffle.g:501:13: ( '0' .. '9' | 'a' .. 'f' | 'A' .. 'F' )
{
if ( (input.LA(1)>='0' && input.LA(1)<='9')||(input.LA(1)>='A' && input.LA(1)<='F')||(input.LA(1)>='a' && input.LA(1)<='f') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
}
finally {
}
}
// $ANTLR end "HEX_DIGIT"
// $ANTLR start "ESC_SEQ"
public final void mESC_SEQ() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:505:5: ( '\\\\' ( 'b' | 't' | 'n' | 'f' | 'r' | '\\\"' | '\\'' | '\\\\' ) | UNICODE_ESC | OCTAL_ESC )
int alt19=3;
switch ( input.LA(1) ) {
case '\\':
{
switch ( input.LA(2) ) {
case '\"':
case '\'':
case '\\':
case 'b':
case 'f':
case 'n':
case 'r':
case 't':
{
alt19=1;
}
break;
case 'u':
{
alt19=2;
}
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
{
alt19=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 19, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 19, 0, input);
throw nvae;
}
switch (alt19) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:505:9: '\\\\' ( 'b' | 't' | 'n' | 'f' | 'r' | '\\\"' | '\\'' | '\\\\' )
{
match('\\');
if ( input.LA(1)=='\"'||input.LA(1)=='\''||input.LA(1)=='\\'||input.LA(1)=='b'||input.LA(1)=='f'||input.LA(1)=='n'||input.LA(1)=='r'||input.LA(1)=='t' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:506:9: UNICODE_ESC
{
mUNICODE_ESC();
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:507:9: OCTAL_ESC
{
mOCTAL_ESC();
}
break;
}
}
finally {
}
}
// $ANTLR end "ESC_SEQ"
// $ANTLR start "OCTAL_ESC"
public final void mOCTAL_ESC() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:512:5: ( '\\\\' ( '0' .. '3' ) ( '0' .. '7' ) ( '0' .. '7' ) | '\\\\' ( '0' .. '7' ) ( '0' .. '7' ) | '\\\\' ( '0' .. '7' ) )
int alt20=3;
switch ( input.LA(1) ) {
case '\\':
{
switch ( input.LA(2) ) {
case '0':
case '1':
case '2':
case '3':
{
switch ( input.LA(3) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
{
switch ( input.LA(4) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
{
alt20=1;
}
break;
default:
alt20=2;}
}
break;
default:
alt20=3;}
}
break;
case '4':
case '5':
case '6':
case '7':
{
switch ( input.LA(3) ) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
{
alt20=2;
}
break;
default:
alt20=3;}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 20, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 20, 0, input);
throw nvae;
}
switch (alt20) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:512:9: '\\\\' ( '0' .. '3' ) ( '0' .. '7' ) ( '0' .. '7' )
{
match('\\');
// org/jaitools/jiffle/parser/Jiffle.g:512:14: ( '0' .. '3' )
// org/jaitools/jiffle/parser/Jiffle.g:512:15: '0' .. '3'
{
matchRange('0','3');
}
// org/jaitools/jiffle/parser/Jiffle.g:512:25: ( '0' .. '7' )
// org/jaitools/jiffle/parser/Jiffle.g:512:26: '0' .. '7'
{
matchRange('0','7');
}
// org/jaitools/jiffle/parser/Jiffle.g:512:36: ( '0' .. '7' )
// org/jaitools/jiffle/parser/Jiffle.g:512:37: '0' .. '7'
{
matchRange('0','7');
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:513:9: '\\\\' ( '0' .. '7' ) ( '0' .. '7' )
{
match('\\');
// org/jaitools/jiffle/parser/Jiffle.g:513:14: ( '0' .. '7' )
// org/jaitools/jiffle/parser/Jiffle.g:513:15: '0' .. '7'
{
matchRange('0','7');
}
// org/jaitools/jiffle/parser/Jiffle.g:513:25: ( '0' .. '7' )
// org/jaitools/jiffle/parser/Jiffle.g:513:26: '0' .. '7'
{
matchRange('0','7');
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:514:9: '\\\\' ( '0' .. '7' )
{
match('\\');
// org/jaitools/jiffle/parser/Jiffle.g:514:14: ( '0' .. '7' )
// org/jaitools/jiffle/parser/Jiffle.g:514:15: '0' .. '7'
{
matchRange('0','7');
}
}
break;
}
}
finally {
}
}
// $ANTLR end "OCTAL_ESC"
// $ANTLR start "UNICODE_ESC"
public final void mUNICODE_ESC() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/Jiffle.g:519:5: ( '\\\\' 'u' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT )
// org/jaitools/jiffle/parser/Jiffle.g:519:9: '\\\\' 'u' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT
{
match('\\');
match('u');
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
}
}
finally {
}
}
// $ANTLR end "UNICODE_ESC"
public void mTokens() throws RecognitionException {
// org/jaitools/jiffle/parser/Jiffle.g:1:8: ( COMMENT | TRUE | FALSE | NULL | INT_TYPE | FLOAT_TYPE | DOUBLE_TYPE | BOOLEAN_TYPE | OPTIONS | IMAGES | INIT | READ | WRITE | CON | IF | ELSE | WHILE | UNTIL | FOREACH | IN | BREAKIF | BREAK | ABS_POS_PREFIX | APPEND | INCR | DECR | NOT | POW | TIMES | DIV | MOD | PLUS | MINUS | GT | GE | LE | LT | LOGICALEQ | NE | AND | OR | XOR | QUESTION | TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ | EQ | COMMA | SEMI | COLON | LPAR | RPAR | LSQUARE | RSQUARE | LCURLY | RCURLY | ID | UNDERSCORE | INT_LITERAL | FLOAT_LITERAL | WS | CHAR )
int alt21=64;
alt21 = dfa21.predict(input);
switch (alt21) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:1:10: COMMENT
{
mCOMMENT();
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:1:18: TRUE
{
mTRUE();
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:1:23: FALSE
{
mFALSE();
}
break;
case 4 :
// org/jaitools/jiffle/parser/Jiffle.g:1:29: NULL
{
mNULL();
}
break;
case 5 :
// org/jaitools/jiffle/parser/Jiffle.g:1:34: INT_TYPE
{
mINT_TYPE();
}
break;
case 6 :
// org/jaitools/jiffle/parser/Jiffle.g:1:43: FLOAT_TYPE
{
mFLOAT_TYPE();
}
break;
case 7 :
// org/jaitools/jiffle/parser/Jiffle.g:1:54: DOUBLE_TYPE
{
mDOUBLE_TYPE();
}
break;
case 8 :
// org/jaitools/jiffle/parser/Jiffle.g:1:66: BOOLEAN_TYPE
{
mBOOLEAN_TYPE();
}
break;
case 9 :
// org/jaitools/jiffle/parser/Jiffle.g:1:79: OPTIONS
{
mOPTIONS();
}
break;
case 10 :
// org/jaitools/jiffle/parser/Jiffle.g:1:87: IMAGES
{
mIMAGES();
}
break;
case 11 :
// org/jaitools/jiffle/parser/Jiffle.g:1:94: INIT
{
mINIT();
}
break;
case 12 :
// org/jaitools/jiffle/parser/Jiffle.g:1:99: READ
{
mREAD();
}
break;
case 13 :
// org/jaitools/jiffle/parser/Jiffle.g:1:104: WRITE
{
mWRITE();
}
break;
case 14 :
// org/jaitools/jiffle/parser/Jiffle.g:1:110: CON
{
mCON();
}
break;
case 15 :
// org/jaitools/jiffle/parser/Jiffle.g:1:114: IF
{
mIF();
}
break;
case 16 :
// org/jaitools/jiffle/parser/Jiffle.g:1:117: ELSE
{
mELSE();
}
break;
case 17 :
// org/jaitools/jiffle/parser/Jiffle.g:1:122: WHILE
{
mWHILE();
}
break;
case 18 :
// org/jaitools/jiffle/parser/Jiffle.g:1:128: UNTIL
{
mUNTIL();
}
break;
case 19 :
// org/jaitools/jiffle/parser/Jiffle.g:1:134: FOREACH
{
mFOREACH();
}
break;
case 20 :
// org/jaitools/jiffle/parser/Jiffle.g:1:142: IN
{
mIN();
}
break;
case 21 :
// org/jaitools/jiffle/parser/Jiffle.g:1:145: BREAKIF
{
mBREAKIF();
}
break;
case 22 :
// org/jaitools/jiffle/parser/Jiffle.g:1:153: BREAK
{
mBREAK();
}
break;
case 23 :
// org/jaitools/jiffle/parser/Jiffle.g:1:159: ABS_POS_PREFIX
{
mABS_POS_PREFIX();
}
break;
case 24 :
// org/jaitools/jiffle/parser/Jiffle.g:1:174: APPEND
{
mAPPEND();
}
break;
case 25 :
// org/jaitools/jiffle/parser/Jiffle.g:1:181: INCR
{
mINCR();
}
break;
case 26 :
// org/jaitools/jiffle/parser/Jiffle.g:1:186: DECR
{
mDECR();
}
break;
case 27 :
// org/jaitools/jiffle/parser/Jiffle.g:1:191: NOT
{
mNOT();
}
break;
case 28 :
// org/jaitools/jiffle/parser/Jiffle.g:1:195: POW
{
mPOW();
}
break;
case 29 :
// org/jaitools/jiffle/parser/Jiffle.g:1:199: TIMES
{
mTIMES();
}
break;
case 30 :
// org/jaitools/jiffle/parser/Jiffle.g:1:205: DIV
{
mDIV();
}
break;
case 31 :
// org/jaitools/jiffle/parser/Jiffle.g:1:209: MOD
{
mMOD();
}
break;
case 32 :
// org/jaitools/jiffle/parser/Jiffle.g:1:213: PLUS
{
mPLUS();
}
break;
case 33 :
// org/jaitools/jiffle/parser/Jiffle.g:1:218: MINUS
{
mMINUS();
}
break;
case 34 :
// org/jaitools/jiffle/parser/Jiffle.g:1:224: GT
{
mGT();
}
break;
case 35 :
// org/jaitools/jiffle/parser/Jiffle.g:1:227: GE
{
mGE();
}
break;
case 36 :
// org/jaitools/jiffle/parser/Jiffle.g:1:230: LE
{
mLE();
}
break;
case 37 :
// org/jaitools/jiffle/parser/Jiffle.g:1:233: LT
{
mLT();
}
break;
case 38 :
// org/jaitools/jiffle/parser/Jiffle.g:1:236: LOGICALEQ
{
mLOGICALEQ();
}
break;
case 39 :
// org/jaitools/jiffle/parser/Jiffle.g:1:246: NE
{
mNE();
}
break;
case 40 :
// org/jaitools/jiffle/parser/Jiffle.g:1:249: AND
{
mAND();
}
break;
case 41 :
// org/jaitools/jiffle/parser/Jiffle.g:1:253: OR
{
mOR();
}
break;
case 42 :
// org/jaitools/jiffle/parser/Jiffle.g:1:256: XOR
{
mXOR();
}
break;
case 43 :
// org/jaitools/jiffle/parser/Jiffle.g:1:260: QUESTION
{
mQUESTION();
}
break;
case 44 :
// org/jaitools/jiffle/parser/Jiffle.g:1:269: TIMESEQ
{
mTIMESEQ();
}
break;
case 45 :
// org/jaitools/jiffle/parser/Jiffle.g:1:277: DIVEQ
{
mDIVEQ();
}
break;
case 46 :
// org/jaitools/jiffle/parser/Jiffle.g:1:283: MODEQ
{
mMODEQ();
}
break;
case 47 :
// org/jaitools/jiffle/parser/Jiffle.g:1:289: PLUSEQ
{
mPLUSEQ();
}
break;
case 48 :
// org/jaitools/jiffle/parser/Jiffle.g:1:296: MINUSEQ
{
mMINUSEQ();
}
break;
case 49 :
// org/jaitools/jiffle/parser/Jiffle.g:1:304: EQ
{
mEQ();
}
break;
case 50 :
// org/jaitools/jiffle/parser/Jiffle.g:1:307: COMMA
{
mCOMMA();
}
break;
case 51 :
// org/jaitools/jiffle/parser/Jiffle.g:1:313: SEMI
{
mSEMI();
}
break;
case 52 :
// org/jaitools/jiffle/parser/Jiffle.g:1:318: COLON
{
mCOLON();
}
break;
case 53 :
// org/jaitools/jiffle/parser/Jiffle.g:1:324: LPAR
{
mLPAR();
}
break;
case 54 :
// org/jaitools/jiffle/parser/Jiffle.g:1:329: RPAR
{
mRPAR();
}
break;
case 55 :
// org/jaitools/jiffle/parser/Jiffle.g:1:334: LSQUARE
{
mLSQUARE();
}
break;
case 56 :
// org/jaitools/jiffle/parser/Jiffle.g:1:342: RSQUARE
{
mRSQUARE();
}
break;
case 57 :
// org/jaitools/jiffle/parser/Jiffle.g:1:350: LCURLY
{
mLCURLY();
}
break;
case 58 :
// org/jaitools/jiffle/parser/Jiffle.g:1:357: RCURLY
{
mRCURLY();
}
break;
case 59 :
// org/jaitools/jiffle/parser/Jiffle.g:1:364: ID
{
mID();
}
break;
case 60 :
// org/jaitools/jiffle/parser/Jiffle.g:1:367: UNDERSCORE
{
mUNDERSCORE();
}
break;
case 61 :
// org/jaitools/jiffle/parser/Jiffle.g:1:378: INT_LITERAL
{
mINT_LITERAL();
}
break;
case 62 :
// org/jaitools/jiffle/parser/Jiffle.g:1:390: FLOAT_LITERAL
{
mFLOAT_LITERAL();
}
break;
case 63 :
// org/jaitools/jiffle/parser/Jiffle.g:1:404: WS
{
mWS();
}
break;
case 64 :
// org/jaitools/jiffle/parser/Jiffle.g:1:407: CHAR
{
mCHAR();
}
break;
}
}
protected DFA21 dfa21 = new DFA21(this);
static final String DFA21_eotS =
"\1\uffff\1\60\17\47\1\uffff\1\110\1\113\1\116\1\120\1\122\1\124"+
"\1\126\1\130\1\132\16\uffff\2\133\6\uffff\10\47\1\147\1\47\1\151"+
"\12\47\26\uffff\1\133\10\47\1\174\1\47\1\uffff\1\47\1\uffff\7\47"+
"\1\u0086\2\47\2\u0089\4\47\2\u008e\1\uffff\1\u008f\5\47\1\u0095"+
"\2\47\1\uffff\1\u0098\1\47\1\uffff\2\u009a\1\u009b\1\47\2\uffff"+
"\3\47\1\u00a1\1\47\1\uffff\1\u00a3\1\u00a4\1\uffff\1\u00a5\2\uffff"+
"\1\47\1\u00a7\1\u00a8\2\47\1\uffff\1\47\3\uffff\1\u00ac\2\uffff"+
"\1\u00ad\1\u00ae\1\u00af\4\uffff";
static final String DFA21_eofS =
"\u00b0\uffff";
static final String DFA21_minS =
"\1\11\1\52\1\122\1\162\1\101\1\141\1\125\1\165\1\146\2\157\1\160"+
"\1\145\1\150\1\157\1\154\1\156\1\uffff\1\74\1\53\1\55\1\75\1\174"+
"\4\75\16\uffff\2\56\6\uffff\1\125\1\165\1\114\1\154\1\157\1\162"+
"\1\114\1\154\1\56\1\141\1\56\1\165\1\157\1\145\1\164\1\141\2\151"+
"\1\156\1\163\1\164\26\uffff\1\56\1\105\1\145\1\123\1\163\1\141\1"+
"\145\1\114\1\154\1\56\1\164\1\uffff\1\147\1\uffff\1\142\1\154\1"+
"\141\1\151\1\144\1\164\1\154\1\56\1\145\1\151\2\56\1\105\1\145\1"+
"\164\1\141\2\56\1\uffff\1\56\1\145\1\154\1\145\1\153\1\157\1\56"+
"\2\145\1\uffff\1\56\1\154\1\uffff\3\56\1\143\2\uffff\1\163\1\145"+
"\1\141\1\56\1\156\1\uffff\2\56\1\uffff\1\56\2\uffff\1\150\2\56\1"+
"\156\1\146\1\uffff\1\163\3\uffff\1\56\2\uffff\3\56\4\uffff";
static final String DFA21_maxS =
"\1\175\1\75\1\122\1\162\1\101\1\157\1\125\1\165\1\156\1\157\1\162"+
"\1\160\1\145\1\162\1\157\1\154\1\156\1\uffff\4\75\1\174\4\75\16"+
"\uffff\1\56\1\71\6\uffff\1\125\1\165\1\114\1\154\1\157\1\162\1\114"+
"\1\154\1\172\1\141\1\172\1\165\1\157\1\145\1\164\1\141\2\151\1\156"+
"\1\163\1\164\26\uffff\1\71\1\105\1\145\1\123\1\163\1\141\1\145\1"+
"\114\1\154\1\172\1\164\1\uffff\1\147\1\uffff\1\142\1\154\1\141\1"+
"\151\1\144\1\164\1\154\1\172\1\145\1\151\2\172\1\105\1\145\1\164"+
"\1\141\2\172\1\uffff\1\172\1\145\1\154\1\145\1\153\1\157\1\172\2"+
"\145\1\uffff\1\172\1\154\1\uffff\3\172\1\143\2\uffff\1\163\1\145"+
"\1\141\1\172\1\156\1\uffff\2\172\1\uffff\1\172\2\uffff\1\150\2\172"+
"\1\156\1\146\1\uffff\1\163\3\uffff\1\172\2\uffff\3\172\4\uffff";
static final String DFA21_acceptS =
"\21\uffff\1\27\11\uffff\1\50\1\51\1\53\1\62\1\63\1\64\1\65\1\66"+
"\1\67\1\70\1\71\1\72\1\73\1\74\2\uffff\1\76\1\77\1\100\1\1\1\55"+
"\1\36\25\uffff\1\30\1\44\1\45\1\31\1\57\1\40\1\32\1\60\1\41\1\47"+
"\1\33\1\52\1\34\1\54\1\35\1\56\1\37\1\43\1\42\1\46\1\61\1\75\13"+
"\uffff\1\24\1\uffff\1\17\22\uffff\1\5\11\uffff\1\16\2\uffff\1\2"+
"\4\uffff\1\4\1\13\5\uffff\1\14\2\uffff\1\20\1\uffff\1\3\1\6\5\uffff"+
"\1\26\1\uffff\1\15\1\21\1\22\1\uffff\1\12\1\7\3\uffff\1\23\1\10"+
"\1\25\1\11";
static final String DFA21_specialS =
"\u00b0\uffff}>";
static final String[] DFA21_transitionS = {
"\2\54\1\uffff\2\54\22\uffff\1\54\1\25\2\uffff\1\21\1\30\1\33"+
"\1\55\1\41\1\42\1\27\1\23\1\36\1\24\1\53\1\1\1\51\11\52\1\40"+
"\1\37\1\22\1\32\1\31\1\35\1\uffff\5\47\1\4\7\47\1\6\5\47\1\2"+
"\6\47\1\43\1\uffff\1\44\1\26\1\50\1\uffff\1\47\1\12\1\16\1\11"+
"\1\17\1\5\2\47\1\10\4\47\1\7\1\13\2\47\1\14\1\47\1\3\1\20\1"+
"\47\1\15\3\47\1\45\1\34\1\46",
"\1\56\4\uffff\1\56\15\uffff\1\57",
"\1\61",
"\1\62",
"\1\63",
"\1\64\12\uffff\1\65\2\uffff\1\66",
"\1\67",
"\1\70",
"\1\73\6\uffff\1\72\1\71",
"\1\74",
"\1\75\2\uffff\1\76",
"\1\77",
"\1\100",
"\1\102\11\uffff\1\101",
"\1\103",
"\1\104",
"\1\105",
"",
"\1\106\1\107",
"\1\111\21\uffff\1\112",
"\1\114\17\uffff\1\115",
"\1\117",
"\1\121",
"\1\123",
"\1\125",
"\1\127",
"\1\131",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\53",
"\1\53\1\uffff\12\134",
"",
"",
"",
"",
"",
"",
"\1\135",
"\1\136",
"\1\137",
"\1\140",
"\1\141",
"\1\142",
"\1\143",
"\1\144",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\10\47"+
"\1\146\12\47\1\145\6\47",
"\1\150",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\152",
"\1\153",
"\1\154",
"\1\155",
"\1\156",
"\1\157",
"\1\160",
"\1\161",
"\1\162",
"\1\163",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\53\1\uffff\12\134",
"\1\164",
"\1\165",
"\1\166",
"\1\167",
"\1\170",
"\1\171",
"\1\172",
"\1\173",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\175",
"",
"\1\176",
"",
"\1\177",
"\1\u0080",
"\1\u0081",
"\1\u0082",
"\1\u0083",
"\1\u0084",
"\1\u0085",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u0087",
"\1\u0088",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u008a",
"\1\u008b",
"\1\u008c",
"\1\u008d",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u0090",
"\1\u0091",
"\1\u0092",
"\1\u0093",
"\1\u0094",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u0096",
"\1\u0097",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u0099",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u009c",
"",
"",
"\1\u009d",
"\1\u009e",
"\1\u009f",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\10\47"+
"\1\u00a0\21\47",
"\1\u00a2",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"",
"",
"\1\u00a6",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\u00a9",
"\1\u00aa",
"",
"\1\u00ab",
"",
"",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"",
"",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"\1\47\1\uffff\12\47\7\uffff\32\47\4\uffff\1\47\1\uffff\32\47",
"",
"",
"",
""
};
static final short[] DFA21_eot = DFA.unpackEncodedString(DFA21_eotS);
static final short[] DFA21_eof = DFA.unpackEncodedString(DFA21_eofS);
static final char[] DFA21_min = DFA.unpackEncodedStringToUnsignedChars(DFA21_minS);
static final char[] DFA21_max = DFA.unpackEncodedStringToUnsignedChars(DFA21_maxS);
static final short[] DFA21_accept = DFA.unpackEncodedString(DFA21_acceptS);
static final short[] DFA21_special = DFA.unpackEncodedString(DFA21_specialS);
static final short[][] DFA21_transition;
static {
int numStates = DFA21_transitionS.length;
DFA21_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy