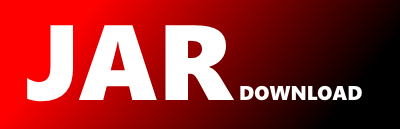
org.jaitools.jiffle.parser.JiffleParser Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/Jiffle.g 2012-10-10 10:44:30
package org.jaitools.jiffle.parser;
import java.util.Map;
import org.jaitools.CollectionFactory;
import org.jaitools.jiffle.Jiffle;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import org.antlr.runtime.tree.*;
/**
* Jiffle language parser grammar. Generates the primary AST
* from an input Jiffle script.
*
* @author Michael Bedward
*/
public class JiffleParser extends Parser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ABS_POS", "BAND_REF", "BLOCK", "CON_CALL", "DECL", "DECLARED_LIST", "EXPR_LIST", "FUNC_CALL", "IMAGE_POS", "JIFFLE_OPTION", "PAR", "PIXEL_REF", "POSTFIX", "PREFIX", "REL_POS", "SEQUENCE", "VAR_DEST", "VAR_IMAGE_SCOPE", "VAR_SOURCE", "CONSTANT", "IMAGE_WRITE", "LIST_NEW", "VAR_IMAGE", "VAR_PIXEL_SCOPE", "VAR_PROVIDED", "VAR_LOOP", "VAR_LIST", "OPTIONS", "LCURLY", "RCURLY", "ID", "EQ", "SEMI", "IMAGES", "READ", "WRITE", "INIT", "WHILE", "LPAR", "RPAR", "UNTIL", "FOREACH", "IN", "IF", "ELSE", "BREAKIF", "BREAK", "COMMA", "COLON", "CON", "APPEND", "TIMESEQ", "DIVEQ", "MODEQ", "PLUSEQ", "MINUSEQ", "QUESTION", "OR", "XOR", "AND", "LOGICALEQ", "NE", "GT", "GE", "LE", "LT", "PLUS", "MINUS", "TIMES", "DIV", "MOD", "NOT", "INCR", "DECR", "POW", "LSQUARE", "RSQUARE", "ABS_POS_PREFIX", "INT_LITERAL", "FLOAT_LITERAL", "TRUE", "FALSE", "NULL", "COMMENT", "INT_TYPE", "FLOAT_TYPE", "DOUBLE_TYPE", "BOOLEAN_TYPE", "Letter", "UNDERSCORE", "Digit", "Dot", "NonZeroDigit", "FloatExp", "WS", "ESC_SEQ", "CHAR", "HEX_DIGIT", "UNICODE_ESC", "OCTAL_ESC"
};
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public JiffleParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public JiffleParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
this.state.ruleMemo = new HashMap[110+1];
}
protected TreeAdaptor adaptor = new CommonTreeAdaptor();
public void setTreeAdaptor(TreeAdaptor adaptor) {
this.adaptor = adaptor;
}
public TreeAdaptor getTreeAdaptor() {
return adaptor;
}
public String[] getTokenNames() { return JiffleParser.tokenNames; }
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/Jiffle.g"; }
@Override
protected Object recoverFromMismatchedToken(IntStream input, int ttype, BitSet follow) throws RecognitionException {
if (ttype == Token.EOF) {
throw new UnexpectedInputException("Invalid statement before end of file");
}
return super.recoverFromMismatchedToken(input, ttype, follow);
}
private List blocksFound = CollectionFactory.list();
private void checkBlock(Token blockToken) {
if ( blocksFound.contains(blockToken.getType()) ) {
throw new JiffleParserException("Duplicate " + blockToken.getText() + " block");
}
blocksFound.add(blockToken.getType());
}
private Map imageParams = CollectionFactory.map();
private void setImageVar(String varName, int type) {
Jiffle.ImageRole role = null;
switch (type) {
case READ:
role = Jiffle.ImageRole.SOURCE;
break;
case WRITE:
role = Jiffle.ImageRole.DEST;
break;
default:
throw new IllegalArgumentException("type must be READ or WRITE");
}
imageParams.put(varName, role);
}
public Map getImageParams() { return imageParams; }
public static class prog_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "prog"
// org/jaitools/jiffle/parser/Jiffle.g:126:1: prog : (blk= specialBlock )* ( statement )+ EOF ;
public final JiffleParser.prog_return prog() throws RecognitionException {
JiffleParser.prog_return retval = new JiffleParser.prog_return();
retval.start = input.LT(1);
int prog_StartIndex = input.index();
CommonTree root_0 = null;
Token EOF2=null;
JiffleParser.specialBlock_return blk = null;
JiffleParser.statement_return statement1 = null;
CommonTree EOF2_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 1) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:126:17: ( (blk= specialBlock )* ( statement )+ EOF )
// org/jaitools/jiffle/parser/Jiffle.g:126:19: (blk= specialBlock )* ( statement )+ EOF
{
root_0 = (CommonTree)adaptor.nil();
// org/jaitools/jiffle/parser/Jiffle.g:126:19: (blk= specialBlock )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case OPTIONS:
case IMAGES:
case INIT:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:126:20: blk= specialBlock
{
pushFollow(FOLLOW_specialBlock_in_prog299);
blk=specialBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, blk.getTree());
if ( state.backtracking==0 ) {
checkBlock((blk!=null?((Token)blk.start):null));
}
}
break;
default :
break loop1;
}
} while (true);
// org/jaitools/jiffle/parser/Jiffle.g:126:66: ( statement )+
int cnt2=0;
loop2:
do {
int alt2=2;
switch ( input.LA(1) ) {
case LCURLY:
case ID:
case SEMI:
case WHILE:
case LPAR:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:0:0: statement
{
pushFollow(FOLLOW_statement_in_prog306);
statement1=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, statement1.getTree());
}
break;
default :
if ( cnt2 >= 1 ) break loop2;
if (state.backtracking>0) {state.failed=true; return retval;}
EarlyExitException eee =
new EarlyExitException(2, input);
throw eee;
}
cnt2++;
} while (true);
EOF2=(Token)match(input,EOF,FOLLOW_EOF_in_prog309); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (UnexpectedInputException ex) {
throw new JiffleParserException(ex);
}
catch (EarlyExitException ex) {
throw new JiffleParserException("Unexpected input at line " + ex.line);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 1, prog_StartIndex); }
}
return retval;
}
// $ANTLR end "prog"
public static class specialBlock_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "specialBlock"
// org/jaitools/jiffle/parser/Jiffle.g:136:1: specialBlock : ( optionsBlock | imagesBlock | initBlock );
public final JiffleParser.specialBlock_return specialBlock() throws RecognitionException {
JiffleParser.specialBlock_return retval = new JiffleParser.specialBlock_return();
retval.start = input.LT(1);
int specialBlock_StartIndex = input.index();
CommonTree root_0 = null;
JiffleParser.optionsBlock_return optionsBlock3 = null;
JiffleParser.imagesBlock_return imagesBlock4 = null;
JiffleParser.initBlock_return initBlock5 = null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 2) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:136:17: ( optionsBlock | imagesBlock | initBlock )
int alt3=3;
switch ( input.LA(1) ) {
case OPTIONS:
{
alt3=1;
}
break;
case IMAGES:
{
alt3=2;
}
break;
case INIT:
{
alt3=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:136:19: optionsBlock
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_optionsBlock_in_specialBlock383);
optionsBlock3=optionsBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, optionsBlock3.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:137:19: imagesBlock
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_imagesBlock_in_specialBlock403);
imagesBlock4=imagesBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, imagesBlock4.getTree());
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:138:19: initBlock
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_initBlock_in_specialBlock423);
initBlock5=initBlock();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, initBlock5.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 2, specialBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "specialBlock"
public static class optionsBlock_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "optionsBlock"
// org/jaitools/jiffle/parser/Jiffle.g:142:1: optionsBlock : OPTIONS LCURLY ( option )* RCURLY -> ( option )* ;
public final JiffleParser.optionsBlock_return optionsBlock() throws RecognitionException {
JiffleParser.optionsBlock_return retval = new JiffleParser.optionsBlock_return();
retval.start = input.LT(1);
int optionsBlock_StartIndex = input.index();
CommonTree root_0 = null;
Token OPTIONS6=null;
Token LCURLY7=null;
Token RCURLY9=null;
JiffleParser.option_return option8 = null;
CommonTree OPTIONS6_tree=null;
CommonTree LCURLY7_tree=null;
CommonTree RCURLY9_tree=null;
RewriteRuleTokenStream stream_LCURLY=new RewriteRuleTokenStream(adaptor,"token LCURLY");
RewriteRuleTokenStream stream_OPTIONS=new RewriteRuleTokenStream(adaptor,"token OPTIONS");
RewriteRuleTokenStream stream_RCURLY=new RewriteRuleTokenStream(adaptor,"token RCURLY");
RewriteRuleSubtreeStream stream_option=new RewriteRuleSubtreeStream(adaptor,"rule option");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 3) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:142:17: ( OPTIONS LCURLY ( option )* RCURLY -> ( option )* )
// org/jaitools/jiffle/parser/Jiffle.g:142:19: OPTIONS LCURLY ( option )* RCURLY
{
OPTIONS6=(Token)match(input,OPTIONS,FOLLOW_OPTIONS_in_optionsBlock452); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_OPTIONS.add(OPTIONS6);
LCURLY7=(Token)match(input,LCURLY,FOLLOW_LCURLY_in_optionsBlock454); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LCURLY.add(LCURLY7);
// org/jaitools/jiffle/parser/Jiffle.g:142:34: ( option )*
loop4:
do {
int alt4=2;
switch ( input.LA(1) ) {
case ID:
{
alt4=1;
}
break;
}
switch (alt4) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:0:0: option
{
pushFollow(FOLLOW_option_in_optionsBlock456);
option8=option();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_option.add(option8.getTree());
}
break;
default :
break loop4;
}
} while (true);
RCURLY9=(Token)match(input,RCURLY,FOLLOW_RCURLY_in_optionsBlock459); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RCURLY.add(RCURLY9);
// AST REWRITE
// elements: option
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 142:49: -> ( option )*
{
// org/jaitools/jiffle/parser/Jiffle.g:142:52: ( option )*
while ( stream_option.hasNext() ) {
adaptor.addChild(root_0, stream_option.nextTree());
}
stream_option.reset();
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 3, optionsBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "optionsBlock"
public static class option_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "option"
// org/jaitools/jiffle/parser/Jiffle.g:145:1: option : ID EQ optionValue SEMI -> ^( JIFFLE_OPTION ID optionValue ) ;
public final JiffleParser.option_return option() throws RecognitionException {
JiffleParser.option_return retval = new JiffleParser.option_return();
retval.start = input.LT(1);
int option_StartIndex = input.index();
CommonTree root_0 = null;
Token ID10=null;
Token EQ11=null;
Token SEMI13=null;
JiffleParser.optionValue_return optionValue12 = null;
CommonTree ID10_tree=null;
CommonTree EQ11_tree=null;
CommonTree SEMI13_tree=null;
RewriteRuleTokenStream stream_EQ=new RewriteRuleTokenStream(adaptor,"token EQ");
RewriteRuleTokenStream stream_ID=new RewriteRuleTokenStream(adaptor,"token ID");
RewriteRuleTokenStream stream_SEMI=new RewriteRuleTokenStream(adaptor,"token SEMI");
RewriteRuleSubtreeStream stream_optionValue=new RewriteRuleSubtreeStream(adaptor,"rule optionValue");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 4) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:145:17: ( ID EQ optionValue SEMI -> ^( JIFFLE_OPTION ID optionValue ) )
// org/jaitools/jiffle/parser/Jiffle.g:145:19: ID EQ optionValue SEMI
{
ID10=(Token)match(input,ID,FOLLOW_ID_in_option498); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ID.add(ID10);
EQ11=(Token)match(input,EQ,FOLLOW_EQ_in_option500); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQ.add(EQ11);
pushFollow(FOLLOW_optionValue_in_option502);
optionValue12=optionValue();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_optionValue.add(optionValue12.getTree());
SEMI13=(Token)match(input,SEMI,FOLLOW_SEMI_in_option504); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMI.add(SEMI13);
// AST REWRITE
// elements: optionValue, ID
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 145:42: -> ^( JIFFLE_OPTION ID optionValue )
{
// org/jaitools/jiffle/parser/Jiffle.g:145:45: ^( JIFFLE_OPTION ID optionValue )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(JIFFLE_OPTION, "JIFFLE_OPTION"), root_1);
adaptor.addChild(root_1, stream_ID.nextNode());
adaptor.addChild(root_1, stream_optionValue.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 4, option_StartIndex); }
}
return retval;
}
// $ANTLR end "option"
public static class optionValue_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "optionValue"
// org/jaitools/jiffle/parser/Jiffle.g:149:1: optionValue : ( ID | literal );
public final JiffleParser.optionValue_return optionValue() throws RecognitionException {
JiffleParser.optionValue_return retval = new JiffleParser.optionValue_return();
retval.start = input.LT(1);
int optionValue_StartIndex = input.index();
CommonTree root_0 = null;
Token ID14=null;
JiffleParser.literal_return literal15 = null;
CommonTree ID14_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 5) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:149:17: ( ID | literal )
int alt5=2;
switch ( input.LA(1) ) {
case ID:
{
alt5=1;
}
break;
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt5=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:149:19: ID
{
root_0 = (CommonTree)adaptor.nil();
ID14=(Token)match(input,ID,FOLLOW_ID_in_optionValue544); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID14_tree = (CommonTree)adaptor.create(ID14);
adaptor.addChild(root_0, ID14_tree);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:150:19: literal
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_literal_in_optionValue564);
literal15=literal();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, literal15.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 5, optionValue_StartIndex); }
}
return retval;
}
// $ANTLR end "optionValue"
public static class imagesBlock_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "imagesBlock"
// org/jaitools/jiffle/parser/Jiffle.g:155:1: imagesBlock : IMAGES LCURLY ( imageVarDeclaration )* RCURLY ->;
public final JiffleParser.imagesBlock_return imagesBlock() throws RecognitionException {
JiffleParser.imagesBlock_return retval = new JiffleParser.imagesBlock_return();
retval.start = input.LT(1);
int imagesBlock_StartIndex = input.index();
CommonTree root_0 = null;
Token IMAGES16=null;
Token LCURLY17=null;
Token RCURLY19=null;
JiffleParser.imageVarDeclaration_return imageVarDeclaration18 = null;
CommonTree IMAGES16_tree=null;
CommonTree LCURLY17_tree=null;
CommonTree RCURLY19_tree=null;
RewriteRuleTokenStream stream_LCURLY=new RewriteRuleTokenStream(adaptor,"token LCURLY");
RewriteRuleTokenStream stream_IMAGES=new RewriteRuleTokenStream(adaptor,"token IMAGES");
RewriteRuleTokenStream stream_RCURLY=new RewriteRuleTokenStream(adaptor,"token RCURLY");
RewriteRuleSubtreeStream stream_imageVarDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule imageVarDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 6) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:155:17: ( IMAGES LCURLY ( imageVarDeclaration )* RCURLY ->)
// org/jaitools/jiffle/parser/Jiffle.g:155:19: IMAGES LCURLY ( imageVarDeclaration )* RCURLY
{
IMAGES16=(Token)match(input,IMAGES,FOLLOW_IMAGES_in_imagesBlock595); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_IMAGES.add(IMAGES16);
LCURLY17=(Token)match(input,LCURLY,FOLLOW_LCURLY_in_imagesBlock597); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LCURLY.add(LCURLY17);
// org/jaitools/jiffle/parser/Jiffle.g:155:33: ( imageVarDeclaration )*
loop6:
do {
int alt6=2;
switch ( input.LA(1) ) {
case ID:
{
alt6=1;
}
break;
}
switch (alt6) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:0:0: imageVarDeclaration
{
pushFollow(FOLLOW_imageVarDeclaration_in_imagesBlock599);
imageVarDeclaration18=imageVarDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_imageVarDeclaration.add(imageVarDeclaration18.getTree());
}
break;
default :
break loop6;
}
} while (true);
RCURLY19=(Token)match(input,RCURLY,FOLLOW_RCURLY_in_imagesBlock602); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RCURLY.add(RCURLY19);
// AST REWRITE
// elements:
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 155:61: ->
{
root_0 = null;
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 6, imagesBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "imagesBlock"
public static class imageVarDeclaration_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "imageVarDeclaration"
// org/jaitools/jiffle/parser/Jiffle.g:159:1: imageVarDeclaration : ID EQ role SEMI ;
public final JiffleParser.imageVarDeclaration_return imageVarDeclaration() throws RecognitionException {
JiffleParser.imageVarDeclaration_return retval = new JiffleParser.imageVarDeclaration_return();
retval.start = input.LT(1);
int imageVarDeclaration_StartIndex = input.index();
CommonTree root_0 = null;
Token ID20=null;
Token EQ21=null;
Token SEMI23=null;
JiffleParser.role_return role22 = null;
CommonTree ID20_tree=null;
CommonTree EQ21_tree=null;
CommonTree SEMI23_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 7) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:160:17: ( ID EQ role SEMI )
// org/jaitools/jiffle/parser/Jiffle.g:160:19: ID EQ role SEMI
{
root_0 = (CommonTree)adaptor.nil();
ID20=(Token)match(input,ID,FOLLOW_ID_in_imageVarDeclaration647); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID20_tree = (CommonTree)adaptor.create(ID20);
adaptor.addChild(root_0, ID20_tree);
}
EQ21=(Token)match(input,EQ,FOLLOW_EQ_in_imageVarDeclaration649); if (state.failed) return retval;
if ( state.backtracking==0 ) {
EQ21_tree = (CommonTree)adaptor.create(EQ21);
adaptor.addChild(root_0, EQ21_tree);
}
pushFollow(FOLLOW_role_in_imageVarDeclaration651);
role22=role();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, role22.getTree());
SEMI23=(Token)match(input,SEMI,FOLLOW_SEMI_in_imageVarDeclaration653); if (state.failed) return retval;
if ( state.backtracking==0 ) {
SEMI23_tree = (CommonTree)adaptor.create(SEMI23);
adaptor.addChild(root_0, SEMI23_tree);
}
if ( state.backtracking==0 ) {
setImageVar((ID20!=null?ID20.getText():null), (role22!=null?((Token)role22.start):null).getType());
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 7, imageVarDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "imageVarDeclaration"
public static class role_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "role"
// org/jaitools/jiffle/parser/Jiffle.g:165:1: role : ( READ | WRITE );
public final JiffleParser.role_return role() throws RecognitionException {
JiffleParser.role_return retval = new JiffleParser.role_return();
retval.start = input.LT(1);
int role_StartIndex = input.index();
CommonTree root_0 = null;
Token set24=null;
CommonTree set24_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 8) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:165:17: ( READ | WRITE )
// org/jaitools/jiffle/parser/Jiffle.g:
{
root_0 = (CommonTree)adaptor.nil();
set24=(Token)input.LT(1);
if ( (input.LA(1)>=READ && input.LA(1)<=WRITE) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (CommonTree)adaptor.create(set24));
state.errorRecovery=false;state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 8, role_StartIndex); }
}
return retval;
}
// $ANTLR end "role"
public static class initBlock_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "initBlock"
// org/jaitools/jiffle/parser/Jiffle.g:170:1: initBlock : INIT LCURLY ( varDeclaration )* RCURLY -> ( varDeclaration )* ;
public final JiffleParser.initBlock_return initBlock() throws RecognitionException {
JiffleParser.initBlock_return retval = new JiffleParser.initBlock_return();
retval.start = input.LT(1);
int initBlock_StartIndex = input.index();
CommonTree root_0 = null;
Token INIT25=null;
Token LCURLY26=null;
Token RCURLY28=null;
JiffleParser.varDeclaration_return varDeclaration27 = null;
CommonTree INIT25_tree=null;
CommonTree LCURLY26_tree=null;
CommonTree RCURLY28_tree=null;
RewriteRuleTokenStream stream_LCURLY=new RewriteRuleTokenStream(adaptor,"token LCURLY");
RewriteRuleTokenStream stream_INIT=new RewriteRuleTokenStream(adaptor,"token INIT");
RewriteRuleTokenStream stream_RCURLY=new RewriteRuleTokenStream(adaptor,"token RCURLY");
RewriteRuleSubtreeStream stream_varDeclaration=new RewriteRuleSubtreeStream(adaptor,"rule varDeclaration");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 9) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:170:17: ( INIT LCURLY ( varDeclaration )* RCURLY -> ( varDeclaration )* )
// org/jaitools/jiffle/parser/Jiffle.g:170:19: INIT LCURLY ( varDeclaration )* RCURLY
{
INIT25=(Token)match(input,INIT,FOLLOW_INIT_in_initBlock760); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_INIT.add(INIT25);
LCURLY26=(Token)match(input,LCURLY,FOLLOW_LCURLY_in_initBlock762); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LCURLY.add(LCURLY26);
// org/jaitools/jiffle/parser/Jiffle.g:170:31: ( varDeclaration )*
loop7:
do {
int alt7=2;
switch ( input.LA(1) ) {
case ID:
{
alt7=1;
}
break;
}
switch (alt7) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:0:0: varDeclaration
{
pushFollow(FOLLOW_varDeclaration_in_initBlock764);
varDeclaration27=varDeclaration();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_varDeclaration.add(varDeclaration27.getTree());
}
break;
default :
break loop7;
}
} while (true);
RCURLY28=(Token)match(input,RCURLY,FOLLOW_RCURLY_in_initBlock767); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RCURLY.add(RCURLY28);
// AST REWRITE
// elements: varDeclaration
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 170:54: -> ( varDeclaration )*
{
// org/jaitools/jiffle/parser/Jiffle.g:170:57: ( varDeclaration )*
while ( stream_varDeclaration.hasNext() ) {
adaptor.addChild(root_0, stream_varDeclaration.nextTree());
}
stream_varDeclaration.reset();
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 9, initBlock_StartIndex); }
}
return retval;
}
// $ANTLR end "initBlock"
public static class varDeclaration_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "varDeclaration"
// org/jaitools/jiffle/parser/Jiffle.g:174:1: varDeclaration : ID ( EQ expression )? SEMI -> ^( DECL VAR_IMAGE_SCOPE ID ( expression )? ) ;
public final JiffleParser.varDeclaration_return varDeclaration() throws RecognitionException {
JiffleParser.varDeclaration_return retval = new JiffleParser.varDeclaration_return();
retval.start = input.LT(1);
int varDeclaration_StartIndex = input.index();
CommonTree root_0 = null;
Token ID29=null;
Token EQ30=null;
Token SEMI32=null;
JiffleParser.expression_return expression31 = null;
CommonTree ID29_tree=null;
CommonTree EQ30_tree=null;
CommonTree SEMI32_tree=null;
RewriteRuleTokenStream stream_EQ=new RewriteRuleTokenStream(adaptor,"token EQ");
RewriteRuleTokenStream stream_ID=new RewriteRuleTokenStream(adaptor,"token ID");
RewriteRuleTokenStream stream_SEMI=new RewriteRuleTokenStream(adaptor,"token SEMI");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 10) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:174:17: ( ID ( EQ expression )? SEMI -> ^( DECL VAR_IMAGE_SCOPE ID ( expression )? ) )
// org/jaitools/jiffle/parser/Jiffle.g:174:19: ID ( EQ expression )? SEMI
{
ID29=(Token)match(input,ID,FOLLOW_ID_in_varDeclaration799); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ID.add(ID29);
// org/jaitools/jiffle/parser/Jiffle.g:174:22: ( EQ expression )?
int alt8=2;
switch ( input.LA(1) ) {
case EQ:
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:174:23: EQ expression
{
EQ30=(Token)match(input,EQ,FOLLOW_EQ_in_varDeclaration802); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_EQ.add(EQ30);
pushFollow(FOLLOW_expression_in_varDeclaration804);
expression31=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression31.getTree());
}
break;
}
SEMI32=(Token)match(input,SEMI,FOLLOW_SEMI_in_varDeclaration808); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_SEMI.add(SEMI32);
// AST REWRITE
// elements: expression, ID
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 174:44: -> ^( DECL VAR_IMAGE_SCOPE ID ( expression )? )
{
// org/jaitools/jiffle/parser/Jiffle.g:174:47: ^( DECL VAR_IMAGE_SCOPE ID ( expression )? )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(DECL, "DECL"), root_1);
adaptor.addChild(root_1, (CommonTree)adaptor.create(VAR_IMAGE_SCOPE, "VAR_IMAGE_SCOPE"));
adaptor.addChild(root_1, stream_ID.nextNode());
// org/jaitools/jiffle/parser/Jiffle.g:174:73: ( expression )?
if ( stream_expression.hasNext() ) {
adaptor.addChild(root_1, stream_expression.nextTree());
}
stream_expression.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 10, varDeclaration_StartIndex); }
}
return retval;
}
// $ANTLR end "varDeclaration"
public static class block_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "block"
// org/jaitools/jiffle/parser/Jiffle.g:178:1: block : LCURLY ( statement )* RCURLY -> ^( BLOCK ( statement )* ) ;
public final JiffleParser.block_return block() throws RecognitionException {
JiffleParser.block_return retval = new JiffleParser.block_return();
retval.start = input.LT(1);
int block_StartIndex = input.index();
CommonTree root_0 = null;
Token LCURLY33=null;
Token RCURLY35=null;
JiffleParser.statement_return statement34 = null;
CommonTree LCURLY33_tree=null;
CommonTree RCURLY35_tree=null;
RewriteRuleTokenStream stream_LCURLY=new RewriteRuleTokenStream(adaptor,"token LCURLY");
RewriteRuleTokenStream stream_RCURLY=new RewriteRuleTokenStream(adaptor,"token RCURLY");
RewriteRuleSubtreeStream stream_statement=new RewriteRuleSubtreeStream(adaptor,"rule statement");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 11) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:178:17: ( LCURLY ( statement )* RCURLY -> ^( BLOCK ( statement )* ) )
// org/jaitools/jiffle/parser/Jiffle.g:178:19: LCURLY ( statement )* RCURLY
{
LCURLY33=(Token)match(input,LCURLY,FOLLOW_LCURLY_in_block857); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LCURLY.add(LCURLY33);
// org/jaitools/jiffle/parser/Jiffle.g:178:26: ( statement )*
loop9:
do {
int alt9=2;
switch ( input.LA(1) ) {
case LCURLY:
case ID:
case SEMI:
case WHILE:
case LPAR:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt9=1;
}
break;
}
switch (alt9) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:0:0: statement
{
pushFollow(FOLLOW_statement_in_block859);
statement34=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement34.getTree());
}
break;
default :
break loop9;
}
} while (true);
RCURLY35=(Token)match(input,RCURLY,FOLLOW_RCURLY_in_block862); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RCURLY.add(RCURLY35);
// AST REWRITE
// elements: statement
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 178:44: -> ^( BLOCK ( statement )* )
{
// org/jaitools/jiffle/parser/Jiffle.g:178:47: ^( BLOCK ( statement )* )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(BLOCK, "BLOCK"), root_1);
// org/jaitools/jiffle/parser/Jiffle.g:178:55: ( statement )*
while ( stream_statement.hasNext() ) {
adaptor.addChild(root_1, stream_statement.nextTree());
}
stream_statement.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 11, block_StartIndex); }
}
return retval;
}
// $ANTLR end "block"
public static class statement_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "statement"
// org/jaitools/jiffle/parser/Jiffle.g:182:1: statement : ( ifCall | block | delimitedStatement SEMI | assignmentExpression SEMI | WHILE LPAR loopCondition RPAR statement -> ^( WHILE loopCondition statement ) | UNTIL LPAR loopCondition RPAR statement -> ^( UNTIL loopCondition statement ) | FOREACH LPAR ID IN loopSet RPAR statement -> ^( FOREACH ID loopSet statement ) | SEMI );
public final JiffleParser.statement_return statement() throws RecognitionException {
JiffleParser.statement_return retval = new JiffleParser.statement_return();
retval.start = input.LT(1);
int statement_StartIndex = input.index();
CommonTree root_0 = null;
Token SEMI39=null;
Token SEMI41=null;
Token WHILE42=null;
Token LPAR43=null;
Token RPAR45=null;
Token UNTIL47=null;
Token LPAR48=null;
Token RPAR50=null;
Token FOREACH52=null;
Token LPAR53=null;
Token ID54=null;
Token IN55=null;
Token RPAR57=null;
Token SEMI59=null;
JiffleParser.ifCall_return ifCall36 = null;
JiffleParser.block_return block37 = null;
JiffleParser.delimitedStatement_return delimitedStatement38 = null;
JiffleParser.assignmentExpression_return assignmentExpression40 = null;
JiffleParser.loopCondition_return loopCondition44 = null;
JiffleParser.statement_return statement46 = null;
JiffleParser.loopCondition_return loopCondition49 = null;
JiffleParser.statement_return statement51 = null;
JiffleParser.loopSet_return loopSet56 = null;
JiffleParser.statement_return statement58 = null;
CommonTree SEMI39_tree=null;
CommonTree SEMI41_tree=null;
CommonTree WHILE42_tree=null;
CommonTree LPAR43_tree=null;
CommonTree RPAR45_tree=null;
CommonTree UNTIL47_tree=null;
CommonTree LPAR48_tree=null;
CommonTree RPAR50_tree=null;
CommonTree FOREACH52_tree=null;
CommonTree LPAR53_tree=null;
CommonTree ID54_tree=null;
CommonTree IN55_tree=null;
CommonTree RPAR57_tree=null;
CommonTree SEMI59_tree=null;
RewriteRuleTokenStream stream_RPAR=new RewriteRuleTokenStream(adaptor,"token RPAR");
RewriteRuleTokenStream stream_FOREACH=new RewriteRuleTokenStream(adaptor,"token FOREACH");
RewriteRuleTokenStream stream_IN=new RewriteRuleTokenStream(adaptor,"token IN");
RewriteRuleTokenStream stream_LPAR=new RewriteRuleTokenStream(adaptor,"token LPAR");
RewriteRuleTokenStream stream_WHILE=new RewriteRuleTokenStream(adaptor,"token WHILE");
RewriteRuleTokenStream stream_ID=new RewriteRuleTokenStream(adaptor,"token ID");
RewriteRuleTokenStream stream_UNTIL=new RewriteRuleTokenStream(adaptor,"token UNTIL");
RewriteRuleSubtreeStream stream_statement=new RewriteRuleSubtreeStream(adaptor,"rule statement");
RewriteRuleSubtreeStream stream_loopSet=new RewriteRuleSubtreeStream(adaptor,"rule loopSet");
RewriteRuleSubtreeStream stream_loopCondition=new RewriteRuleSubtreeStream(adaptor,"rule loopCondition");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 12) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:182:17: ( ifCall | block | delimitedStatement SEMI | assignmentExpression SEMI | WHILE LPAR loopCondition RPAR statement -> ^( WHILE loopCondition statement ) | UNTIL LPAR loopCondition RPAR statement -> ^( UNTIL loopCondition statement ) | FOREACH LPAR ID IN loopSet RPAR statement -> ^( FOREACH ID loopSet statement ) | SEMI )
int alt10=8;
alt10 = dfa10.predict(input);
switch (alt10) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:182:19: ifCall
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_ifCall_in_statement903);
ifCall36=ifCall();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, ifCall36.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:183:19: block
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_block_in_statement923);
block37=block();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, block37.getTree());
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:184:19: delimitedStatement SEMI
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_delimitedStatement_in_statement943);
delimitedStatement38=delimitedStatement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, delimitedStatement38.getTree());
SEMI39=(Token)match(input,SEMI,FOLLOW_SEMI_in_statement945); if (state.failed) return retval;
}
break;
case 4 :
// org/jaitools/jiffle/parser/Jiffle.g:185:19: assignmentExpression SEMI
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_assignmentExpression_in_statement966);
assignmentExpression40=assignmentExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, assignmentExpression40.getTree());
SEMI41=(Token)match(input,SEMI,FOLLOW_SEMI_in_statement968); if (state.failed) return retval;
}
break;
case 5 :
// org/jaitools/jiffle/parser/Jiffle.g:186:19: WHILE LPAR loopCondition RPAR statement
{
WHILE42=(Token)match(input,WHILE,FOLLOW_WHILE_in_statement989); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_WHILE.add(WHILE42);
LPAR43=(Token)match(input,LPAR,FOLLOW_LPAR_in_statement991); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR43);
pushFollow(FOLLOW_loopCondition_in_statement993);
loopCondition44=loopCondition();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_loopCondition.add(loopCondition44.getTree());
RPAR45=(Token)match(input,RPAR,FOLLOW_RPAR_in_statement995); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR45);
pushFollow(FOLLOW_statement_in_statement997);
statement46=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement46.getTree());
// AST REWRITE
// elements: statement, loopCondition, WHILE
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 186:59: -> ^( WHILE loopCondition statement )
{
// org/jaitools/jiffle/parser/Jiffle.g:186:62: ^( WHILE loopCondition statement )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_WHILE.nextNode(), root_1);
adaptor.addChild(root_1, stream_loopCondition.nextTree());
adaptor.addChild(root_1, stream_statement.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 6 :
// org/jaitools/jiffle/parser/Jiffle.g:187:19: UNTIL LPAR loopCondition RPAR statement
{
UNTIL47=(Token)match(input,UNTIL,FOLLOW_UNTIL_in_statement1027); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_UNTIL.add(UNTIL47);
LPAR48=(Token)match(input,LPAR,FOLLOW_LPAR_in_statement1029); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR48);
pushFollow(FOLLOW_loopCondition_in_statement1031);
loopCondition49=loopCondition();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_loopCondition.add(loopCondition49.getTree());
RPAR50=(Token)match(input,RPAR,FOLLOW_RPAR_in_statement1033); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR50);
pushFollow(FOLLOW_statement_in_statement1035);
statement51=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement51.getTree());
// AST REWRITE
// elements: UNTIL, statement, loopCondition
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 187:59: -> ^( UNTIL loopCondition statement )
{
// org/jaitools/jiffle/parser/Jiffle.g:187:62: ^( UNTIL loopCondition statement )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_UNTIL.nextNode(), root_1);
adaptor.addChild(root_1, stream_loopCondition.nextTree());
adaptor.addChild(root_1, stream_statement.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 7 :
// org/jaitools/jiffle/parser/Jiffle.g:188:19: FOREACH LPAR ID IN loopSet RPAR statement
{
FOREACH52=(Token)match(input,FOREACH,FOLLOW_FOREACH_in_statement1065); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_FOREACH.add(FOREACH52);
LPAR53=(Token)match(input,LPAR,FOLLOW_LPAR_in_statement1067); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR53);
ID54=(Token)match(input,ID,FOLLOW_ID_in_statement1069); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ID.add(ID54);
IN55=(Token)match(input,IN,FOLLOW_IN_in_statement1071); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_IN.add(IN55);
pushFollow(FOLLOW_loopSet_in_statement1073);
loopSet56=loopSet();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_loopSet.add(loopSet56.getTree());
RPAR57=(Token)match(input,RPAR,FOLLOW_RPAR_in_statement1075); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR57);
pushFollow(FOLLOW_statement_in_statement1077);
statement58=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(statement58.getTree());
// AST REWRITE
// elements: statement, FOREACH, loopSet, ID
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 188:61: -> ^( FOREACH ID loopSet statement )
{
// org/jaitools/jiffle/parser/Jiffle.g:188:64: ^( FOREACH ID loopSet statement )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_FOREACH.nextNode(), root_1);
adaptor.addChild(root_1, stream_ID.nextNode());
adaptor.addChild(root_1, stream_loopSet.nextTree());
adaptor.addChild(root_1, stream_statement.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 8 :
// org/jaitools/jiffle/parser/Jiffle.g:189:19: SEMI
{
root_0 = (CommonTree)adaptor.nil();
SEMI59=(Token)match(input,SEMI,FOLLOW_SEMI_in_statement1109); if (state.failed) return retval;
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 12, statement_StartIndex); }
}
return retval;
}
// $ANTLR end "statement"
public static class ifCall_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "ifCall"
// org/jaitools/jiffle/parser/Jiffle.g:193:1: ifCall : IF LPAR orExpression RPAR s1= statement ( ELSE s2= statement -> ^( IF orExpression $s1 $s2) | -> ^( IF orExpression $s1) ) ;
public final JiffleParser.ifCall_return ifCall() throws RecognitionException {
JiffleParser.ifCall_return retval = new JiffleParser.ifCall_return();
retval.start = input.LT(1);
int ifCall_StartIndex = input.index();
CommonTree root_0 = null;
Token IF60=null;
Token LPAR61=null;
Token RPAR63=null;
Token ELSE64=null;
JiffleParser.statement_return s1 = null;
JiffleParser.statement_return s2 = null;
JiffleParser.orExpression_return orExpression62 = null;
CommonTree IF60_tree=null;
CommonTree LPAR61_tree=null;
CommonTree RPAR63_tree=null;
CommonTree ELSE64_tree=null;
RewriteRuleTokenStream stream_RPAR=new RewriteRuleTokenStream(adaptor,"token RPAR");
RewriteRuleTokenStream stream_LPAR=new RewriteRuleTokenStream(adaptor,"token LPAR");
RewriteRuleTokenStream stream_IF=new RewriteRuleTokenStream(adaptor,"token IF");
RewriteRuleTokenStream stream_ELSE=new RewriteRuleTokenStream(adaptor,"token ELSE");
RewriteRuleSubtreeStream stream_statement=new RewriteRuleSubtreeStream(adaptor,"rule statement");
RewriteRuleSubtreeStream stream_orExpression=new RewriteRuleSubtreeStream(adaptor,"rule orExpression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 13) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:193:17: ( IF LPAR orExpression RPAR s1= statement ( ELSE s2= statement -> ^( IF orExpression $s1 $s2) | -> ^( IF orExpression $s1) ) )
// org/jaitools/jiffle/parser/Jiffle.g:193:19: IF LPAR orExpression RPAR s1= statement ( ELSE s2= statement -> ^( IF orExpression $s1 $s2) | -> ^( IF orExpression $s1) )
{
IF60=(Token)match(input,IF,FOLLOW_IF_in_ifCall1145); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_IF.add(IF60);
LPAR61=(Token)match(input,LPAR,FOLLOW_LPAR_in_ifCall1147); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR61);
pushFollow(FOLLOW_orExpression_in_ifCall1149);
orExpression62=orExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_orExpression.add(orExpression62.getTree());
RPAR63=(Token)match(input,RPAR,FOLLOW_RPAR_in_ifCall1151); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR63);
pushFollow(FOLLOW_statement_in_ifCall1155);
s1=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(s1.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:194:19: ( ELSE s2= statement -> ^( IF orExpression $s1 $s2) | -> ^( IF orExpression $s1) )
int alt11=2;
switch ( input.LA(1) ) {
case ELSE:
{
int LA11_1 = input.LA(2);
if ( (synpred19_Jiffle()) ) {
alt11=1;
}
else if ( (true) ) {
alt11=2;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 11, 1, input);
throw nvae;
}
}
break;
case EOF:
case LCURLY:
case RCURLY:
case ID:
case SEMI:
case WHILE:
case LPAR:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt11=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:194:21: ELSE s2= statement
{
ELSE64=(Token)match(input,ELSE,FOLLOW_ELSE_in_ifCall1177); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ELSE.add(ELSE64);
pushFollow(FOLLOW_statement_in_ifCall1181);
s2=statement();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_statement.add(s2.getTree());
// AST REWRITE
// elements: s2, IF, orExpression, s1
// token labels:
// rule labels: retval, s2, s1
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
RewriteRuleSubtreeStream stream_s2=new RewriteRuleSubtreeStream(adaptor,"rule s2",s2!=null?s2.tree:null);
RewriteRuleSubtreeStream stream_s1=new RewriteRuleSubtreeStream(adaptor,"rule s1",s1!=null?s1.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 194:39: -> ^( IF orExpression $s1 $s2)
{
// org/jaitools/jiffle/parser/Jiffle.g:194:42: ^( IF orExpression $s1 $s2)
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_IF.nextNode(), root_1);
adaptor.addChild(root_1, stream_orExpression.nextTree());
adaptor.addChild(root_1, stream_s1.nextTree());
adaptor.addChild(root_1, stream_s2.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:195:21:
{
// AST REWRITE
// elements: s1, IF, orExpression
// token labels:
// rule labels: retval, s1
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
RewriteRuleSubtreeStream stream_s1=new RewriteRuleSubtreeStream(adaptor,"rule s1",s1!=null?s1.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 195:21: -> ^( IF orExpression $s1)
{
// org/jaitools/jiffle/parser/Jiffle.g:195:24: ^( IF orExpression $s1)
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_IF.nextNode(), root_1);
adaptor.addChild(root_1, stream_orExpression.nextTree());
adaptor.addChild(root_1, stream_s1.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 13, ifCall_StartIndex); }
}
return retval;
}
// $ANTLR end "ifCall"
public static class delimitedStatement_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "delimitedStatement"
// org/jaitools/jiffle/parser/Jiffle.g:200:1: delimitedStatement : ( expression | BREAKIF LPAR expression RPAR -> ^( BREAKIF expression ) | BREAK );
public final JiffleParser.delimitedStatement_return delimitedStatement() throws RecognitionException {
JiffleParser.delimitedStatement_return retval = new JiffleParser.delimitedStatement_return();
retval.start = input.LT(1);
int delimitedStatement_StartIndex = input.index();
CommonTree root_0 = null;
Token BREAKIF66=null;
Token LPAR67=null;
Token RPAR69=null;
Token BREAK70=null;
JiffleParser.expression_return expression65 = null;
JiffleParser.expression_return expression68 = null;
CommonTree BREAKIF66_tree=null;
CommonTree LPAR67_tree=null;
CommonTree RPAR69_tree=null;
CommonTree BREAK70_tree=null;
RewriteRuleTokenStream stream_RPAR=new RewriteRuleTokenStream(adaptor,"token RPAR");
RewriteRuleTokenStream stream_LPAR=new RewriteRuleTokenStream(adaptor,"token LPAR");
RewriteRuleTokenStream stream_BREAKIF=new RewriteRuleTokenStream(adaptor,"token BREAKIF");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 14) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:201:17: ( expression | BREAKIF LPAR expression RPAR -> ^( BREAKIF expression ) | BREAK )
int alt12=3;
switch ( input.LA(1) ) {
case ID:
case LPAR:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt12=1;
}
break;
case BREAKIF:
{
alt12=2;
}
break;
case BREAK:
{
alt12=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 12, 0, input);
throw nvae;
}
switch (alt12) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:201:19: expression
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_expression_in_delimitedStatement1288);
expression65=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression65.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:202:19: BREAKIF LPAR expression RPAR
{
BREAKIF66=(Token)match(input,BREAKIF,FOLLOW_BREAKIF_in_delimitedStatement1308); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_BREAKIF.add(BREAKIF66);
LPAR67=(Token)match(input,LPAR,FOLLOW_LPAR_in_delimitedStatement1310); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR67);
pushFollow(FOLLOW_expression_in_delimitedStatement1312);
expression68=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression68.getTree());
RPAR69=(Token)match(input,RPAR,FOLLOW_RPAR_in_delimitedStatement1314); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR69);
// AST REWRITE
// elements: expression, BREAKIF
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 202:48: -> ^( BREAKIF expression )
{
// org/jaitools/jiffle/parser/Jiffle.g:202:51: ^( BREAKIF expression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot(stream_BREAKIF.nextNode(), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:203:19: BREAK
{
root_0 = (CommonTree)adaptor.nil();
BREAK70=(Token)match(input,BREAK,FOLLOW_BREAK_in_delimitedStatement1342); if (state.failed) return retval;
if ( state.backtracking==0 ) {
BREAK70_tree = (CommonTree)adaptor.create(BREAK70);
adaptor.addChild(root_0, BREAK70_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 14, delimitedStatement_StartIndex); }
}
return retval;
}
// $ANTLR end "delimitedStatement"
public static class loopCondition_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "loopCondition"
// org/jaitools/jiffle/parser/Jiffle.g:207:1: loopCondition : orExpression ;
public final JiffleParser.loopCondition_return loopCondition() throws RecognitionException {
JiffleParser.loopCondition_return retval = new JiffleParser.loopCondition_return();
retval.start = input.LT(1);
int loopCondition_StartIndex = input.index();
CommonTree root_0 = null;
JiffleParser.orExpression_return orExpression71 = null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 15) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:207:17: ( orExpression )
// org/jaitools/jiffle/parser/Jiffle.g:207:19: orExpression
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_orExpression_in_loopCondition1370);
orExpression71=orExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, orExpression71.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 15, loopCondition_StartIndex); }
}
return retval;
}
// $ANTLR end "loopCondition"
public static class loopSet_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "loopSet"
// org/jaitools/jiffle/parser/Jiffle.g:211:1: loopSet : ( listLiteral | sequence | ID );
public final JiffleParser.loopSet_return loopSet() throws RecognitionException {
JiffleParser.loopSet_return retval = new JiffleParser.loopSet_return();
retval.start = input.LT(1);
int loopSet_StartIndex = input.index();
CommonTree root_0 = null;
Token ID74=null;
JiffleParser.listLiteral_return listLiteral72 = null;
JiffleParser.sequence_return sequence73 = null;
CommonTree ID74_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 16) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:211:17: ( listLiteral | sequence | ID )
int alt13=3;
alt13 = dfa13.predict(input);
switch (alt13) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:211:19: listLiteral
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_listLiteral_in_loopSet1404);
listLiteral72=listLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, listLiteral72.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:212:19: sequence
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_sequence_in_loopSet1424);
sequence73=sequence();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, sequence73.getTree());
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:213:19: ID
{
root_0 = (CommonTree)adaptor.nil();
ID74=(Token)match(input,ID,FOLLOW_ID_in_loopSet1444); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID74_tree = (CommonTree)adaptor.create(ID74);
adaptor.addChild(root_0, ID74_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 16, loopSet_StartIndex); }
}
return retval;
}
// $ANTLR end "loopSet"
public static class expressionList_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "expressionList"
// org/jaitools/jiffle/parser/Jiffle.g:217:1: expressionList : ( expression ( COMMA expression )* )? -> ^( EXPR_LIST ( expression )* ) ;
public final JiffleParser.expressionList_return expressionList() throws RecognitionException {
JiffleParser.expressionList_return retval = new JiffleParser.expressionList_return();
retval.start = input.LT(1);
int expressionList_StartIndex = input.index();
CommonTree root_0 = null;
Token COMMA76=null;
JiffleParser.expression_return expression75 = null;
JiffleParser.expression_return expression77 = null;
CommonTree COMMA76_tree=null;
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 17) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:217:17: ( ( expression ( COMMA expression )* )? -> ^( EXPR_LIST ( expression )* ) )
// org/jaitools/jiffle/parser/Jiffle.g:217:19: ( expression ( COMMA expression )* )?
{
// org/jaitools/jiffle/parser/Jiffle.g:217:19: ( expression ( COMMA expression )* )?
int alt15=2;
switch ( input.LA(1) ) {
case ID:
case LPAR:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt15=1;
}
break;
}
switch (alt15) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:217:20: expression ( COMMA expression )*
{
pushFollow(FOLLOW_expression_in_expressionList1472);
expression75=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression75.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:217:31: ( COMMA expression )*
loop14:
do {
int alt14=2;
switch ( input.LA(1) ) {
case COMMA:
{
alt14=1;
}
break;
}
switch (alt14) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:217:32: COMMA expression
{
COMMA76=(Token)match(input,COMMA,FOLLOW_COMMA_in_expressionList1475); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(COMMA76);
pushFollow(FOLLOW_expression_in_expressionList1477);
expression77=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression77.getTree());
}
break;
default :
break loop14;
}
} while (true);
}
break;
}
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 217:54: -> ^( EXPR_LIST ( expression )* )
{
// org/jaitools/jiffle/parser/Jiffle.g:217:57: ^( EXPR_LIST ( expression )* )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(EXPR_LIST, "EXPR_LIST"), root_1);
// org/jaitools/jiffle/parser/Jiffle.g:217:69: ( expression )*
while ( stream_expression.hasNext() ) {
adaptor.addChild(root_1, stream_expression.nextTree());
}
stream_expression.reset();
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 17, expressionList_StartIndex); }
}
return retval;
}
// $ANTLR end "expressionList"
public static class sequence_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "sequence"
// org/jaitools/jiffle/parser/Jiffle.g:221:1: sequence : lo= expression COLON hi= expression -> ^( SEQUENCE $lo $hi) ;
public final JiffleParser.sequence_return sequence() throws RecognitionException {
JiffleParser.sequence_return retval = new JiffleParser.sequence_return();
retval.start = input.LT(1);
int sequence_StartIndex = input.index();
CommonTree root_0 = null;
Token COLON78=null;
JiffleParser.expression_return lo = null;
JiffleParser.expression_return hi = null;
CommonTree COLON78_tree=null;
RewriteRuleTokenStream stream_COLON=new RewriteRuleTokenStream(adaptor,"token COLON");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 18) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:221:17: (lo= expression COLON hi= expression -> ^( SEQUENCE $lo $hi) )
// org/jaitools/jiffle/parser/Jiffle.g:221:19: lo= expression COLON hi= expression
{
pushFollow(FOLLOW_expression_in_sequence1526);
lo=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(lo.getTree());
COLON78=(Token)match(input,COLON,FOLLOW_COLON_in_sequence1528); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COLON.add(COLON78);
pushFollow(FOLLOW_expression_in_sequence1532);
hi=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(hi.getTree());
// AST REWRITE
// elements: lo, hi
// token labels:
// rule labels: lo, retval, hi
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_lo=new RewriteRuleSubtreeStream(adaptor,"rule lo",lo!=null?lo.tree:null);
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
RewriteRuleSubtreeStream stream_hi=new RewriteRuleSubtreeStream(adaptor,"rule hi",hi!=null?hi.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 221:53: -> ^( SEQUENCE $lo $hi)
{
// org/jaitools/jiffle/parser/Jiffle.g:221:56: ^( SEQUENCE $lo $hi)
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(SEQUENCE, "SEQUENCE"), root_1);
adaptor.addChild(root_1, stream_lo.nextTree());
adaptor.addChild(root_1, stream_hi.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 18, sequence_StartIndex); }
}
return retval;
}
// $ANTLR end "sequence"
public static class conCall_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "conCall"
// org/jaitools/jiffle/parser/Jiffle.g:230:1: conCall : CON LPAR expressionList RPAR -> ^( CON_CALL expressionList ) ;
public final JiffleParser.conCall_return conCall() throws RecognitionException {
JiffleParser.conCall_return retval = new JiffleParser.conCall_return();
retval.start = input.LT(1);
int conCall_StartIndex = input.index();
CommonTree root_0 = null;
Token CON79=null;
Token LPAR80=null;
Token RPAR82=null;
JiffleParser.expressionList_return expressionList81 = null;
CommonTree CON79_tree=null;
CommonTree LPAR80_tree=null;
CommonTree RPAR82_tree=null;
RewriteRuleTokenStream stream_RPAR=new RewriteRuleTokenStream(adaptor,"token RPAR");
RewriteRuleTokenStream stream_LPAR=new RewriteRuleTokenStream(adaptor,"token LPAR");
RewriteRuleTokenStream stream_CON=new RewriteRuleTokenStream(adaptor,"token CON");
RewriteRuleSubtreeStream stream_expressionList=new RewriteRuleSubtreeStream(adaptor,"rule expressionList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 19) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:230:17: ( CON LPAR expressionList RPAR -> ^( CON_CALL expressionList ) )
// org/jaitools/jiffle/parser/Jiffle.g:230:19: CON LPAR expressionList RPAR
{
CON79=(Token)match(input,CON,FOLLOW_CON_in_conCall1580); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_CON.add(CON79);
LPAR80=(Token)match(input,LPAR,FOLLOW_LPAR_in_conCall1582); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR80);
pushFollow(FOLLOW_expressionList_in_conCall1584);
expressionList81=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionList.add(expressionList81.getTree());
RPAR82=(Token)match(input,RPAR,FOLLOW_RPAR_in_conCall1586); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR82);
// AST REWRITE
// elements: expressionList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 230:48: -> ^( CON_CALL expressionList )
{
// org/jaitools/jiffle/parser/Jiffle.g:230:51: ^( CON_CALL expressionList )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(CON_CALL, "CON_CALL"), root_1);
adaptor.addChild(root_1, stream_expressionList.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 19, conCall_StartIndex); }
}
return retval;
}
// $ANTLR end "conCall"
public static class assignmentExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "assignmentExpression"
// org/jaitools/jiffle/parser/Jiffle.g:239:1: assignmentExpression : ID assignmentOp expression ;
public final JiffleParser.assignmentExpression_return assignmentExpression() throws RecognitionException {
JiffleParser.assignmentExpression_return retval = new JiffleParser.assignmentExpression_return();
retval.start = input.LT(1);
int assignmentExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token ID83=null;
JiffleParser.assignmentOp_return assignmentOp84 = null;
JiffleParser.expression_return expression85 = null;
CommonTree ID83_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 20) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:240:17: ( ID assignmentOp expression )
// org/jaitools/jiffle/parser/Jiffle.g:240:19: ID assignmentOp expression
{
root_0 = (CommonTree)adaptor.nil();
ID83=(Token)match(input,ID,FOLLOW_ID_in_assignmentExpression1638); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID83_tree = (CommonTree)adaptor.create(ID83);
adaptor.addChild(root_0, ID83_tree);
}
pushFollow(FOLLOW_assignmentOp_in_assignmentExpression1640);
assignmentOp84=assignmentOp();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) root_0 = (CommonTree)adaptor.becomeRoot(assignmentOp84.getTree(), root_0);
pushFollow(FOLLOW_expression_in_assignmentExpression1643);
expression85=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression85.getTree());
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 20, assignmentExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "assignmentExpression"
public static class expression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "expression"
// org/jaitools/jiffle/parser/Jiffle.g:243:1: expression : ( conditionalExpression | ID APPEND expression );
public final JiffleParser.expression_return expression() throws RecognitionException {
JiffleParser.expression_return retval = new JiffleParser.expression_return();
retval.start = input.LT(1);
int expression_StartIndex = input.index();
CommonTree root_0 = null;
Token ID87=null;
Token APPEND88=null;
JiffleParser.conditionalExpression_return conditionalExpression86 = null;
JiffleParser.expression_return expression89 = null;
CommonTree ID87_tree=null;
CommonTree APPEND88_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 21) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:243:17: ( conditionalExpression | ID APPEND expression )
int alt16=2;
switch ( input.LA(1) ) {
case LPAR:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt16=1;
}
break;
case ID:
{
switch ( input.LA(2) ) {
case APPEND:
{
alt16=2;
}
break;
case EOF:
case SEMI:
case LPAR:
case RPAR:
case COMMA:
case COLON:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case INCR:
case DECR:
case POW:
case LSQUARE:
case RSQUARE:
{
alt16=1;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 16, 2, input);
throw nvae;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 16, 0, input);
throw nvae;
}
switch (alt16) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:243:19: conditionalExpression
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_conditionalExpression_in_expression1673);
conditionalExpression86=conditionalExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, conditionalExpression86.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:244:19: ID APPEND expression
{
root_0 = (CommonTree)adaptor.nil();
ID87=(Token)match(input,ID,FOLLOW_ID_in_expression1693); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID87_tree = (CommonTree)adaptor.create(ID87);
adaptor.addChild(root_0, ID87_tree);
}
APPEND88=(Token)match(input,APPEND,FOLLOW_APPEND_in_expression1695); if (state.failed) return retval;
if ( state.backtracking==0 ) {
APPEND88_tree = (CommonTree)adaptor.create(APPEND88);
root_0 = (CommonTree)adaptor.becomeRoot(APPEND88_tree, root_0);
}
pushFollow(FOLLOW_expression_in_expression1698);
expression89=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression89.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 21, expression_StartIndex); }
}
return retval;
}
// $ANTLR end "expression"
public static class assignmentOp_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "assignmentOp"
// org/jaitools/jiffle/parser/Jiffle.g:248:1: assignmentOp : ( EQ | TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ );
public final JiffleParser.assignmentOp_return assignmentOp() throws RecognitionException {
JiffleParser.assignmentOp_return retval = new JiffleParser.assignmentOp_return();
retval.start = input.LT(1);
int assignmentOp_StartIndex = input.index();
CommonTree root_0 = null;
Token set90=null;
CommonTree set90_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 22) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:248:17: ( EQ | TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ )
// org/jaitools/jiffle/parser/Jiffle.g:
{
root_0 = (CommonTree)adaptor.nil();
set90=(Token)input.LT(1);
if ( input.LA(1)==EQ||(input.LA(1)>=TIMESEQ && input.LA(1)<=MINUSEQ) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (CommonTree)adaptor.create(set90));
state.errorRecovery=false;state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 22, assignmentOp_StartIndex); }
}
return retval;
}
// $ANTLR end "assignmentOp"
public static class conditionalExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "conditionalExpression"
// org/jaitools/jiffle/parser/Jiffle.g:257:1: conditionalExpression : orExpression ( QUESTION expression COLON expression )? ;
public final JiffleParser.conditionalExpression_return conditionalExpression() throws RecognitionException {
JiffleParser.conditionalExpression_return retval = new JiffleParser.conditionalExpression_return();
retval.start = input.LT(1);
int conditionalExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token QUESTION92=null;
Token COLON94=null;
JiffleParser.orExpression_return orExpression91 = null;
JiffleParser.expression_return expression93 = null;
JiffleParser.expression_return expression95 = null;
CommonTree QUESTION92_tree=null;
CommonTree COLON94_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 23) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:258:17: ( orExpression ( QUESTION expression COLON expression )? )
// org/jaitools/jiffle/parser/Jiffle.g:258:19: orExpression ( QUESTION expression COLON expression )?
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_orExpression_in_conditionalExpression1869);
orExpression91=orExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, orExpression91.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:258:32: ( QUESTION expression COLON expression )?
int alt17=2;
switch ( input.LA(1) ) {
case QUESTION:
{
alt17=1;
}
break;
}
switch (alt17) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:258:33: QUESTION expression COLON expression
{
QUESTION92=(Token)match(input,QUESTION,FOLLOW_QUESTION_in_conditionalExpression1872); if (state.failed) return retval;
if ( state.backtracking==0 ) {
QUESTION92_tree = (CommonTree)adaptor.create(QUESTION92);
root_0 = (CommonTree)adaptor.becomeRoot(QUESTION92_tree, root_0);
}
pushFollow(FOLLOW_expression_in_conditionalExpression1875);
expression93=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression93.getTree());
COLON94=(Token)match(input,COLON,FOLLOW_COLON_in_conditionalExpression1877); if (state.failed) return retval;
pushFollow(FOLLOW_expression_in_conditionalExpression1880);
expression95=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expression95.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 23, conditionalExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "conditionalExpression"
public static class orExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "orExpression"
// org/jaitools/jiffle/parser/Jiffle.g:262:1: orExpression : xorExpression ( OR xorExpression )* ;
public final JiffleParser.orExpression_return orExpression() throws RecognitionException {
JiffleParser.orExpression_return retval = new JiffleParser.orExpression_return();
retval.start = input.LT(1);
int orExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token OR97=null;
JiffleParser.xorExpression_return xorExpression96 = null;
JiffleParser.xorExpression_return xorExpression98 = null;
CommonTree OR97_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 24) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:262:17: ( xorExpression ( OR xorExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:262:19: xorExpression ( OR xorExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_xorExpression_in_orExpression1911);
xorExpression96=xorExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, xorExpression96.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:262:33: ( OR xorExpression )*
loop18:
do {
int alt18=2;
switch ( input.LA(1) ) {
case OR:
{
alt18=1;
}
break;
}
switch (alt18) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:262:34: OR xorExpression
{
OR97=(Token)match(input,OR,FOLLOW_OR_in_orExpression1914); if (state.failed) return retval;
if ( state.backtracking==0 ) {
OR97_tree = (CommonTree)adaptor.create(OR97);
root_0 = (CommonTree)adaptor.becomeRoot(OR97_tree, root_0);
}
pushFollow(FOLLOW_xorExpression_in_orExpression1917);
xorExpression98=xorExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, xorExpression98.getTree());
}
break;
default :
break loop18;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 24, orExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "orExpression"
public static class xorExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "xorExpression"
// org/jaitools/jiffle/parser/Jiffle.g:266:1: xorExpression : andExpression ( XOR andExpression )* ;
public final JiffleParser.xorExpression_return xorExpression() throws RecognitionException {
JiffleParser.xorExpression_return retval = new JiffleParser.xorExpression_return();
retval.start = input.LT(1);
int xorExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token XOR100=null;
JiffleParser.andExpression_return andExpression99 = null;
JiffleParser.andExpression_return andExpression101 = null;
CommonTree XOR100_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 25) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:266:17: ( andExpression ( XOR andExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:266:19: andExpression ( XOR andExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_andExpression_in_xorExpression1947);
andExpression99=andExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, andExpression99.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:266:33: ( XOR andExpression )*
loop19:
do {
int alt19=2;
switch ( input.LA(1) ) {
case XOR:
{
alt19=1;
}
break;
}
switch (alt19) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:266:34: XOR andExpression
{
XOR100=(Token)match(input,XOR,FOLLOW_XOR_in_xorExpression1950); if (state.failed) return retval;
if ( state.backtracking==0 ) {
XOR100_tree = (CommonTree)adaptor.create(XOR100);
root_0 = (CommonTree)adaptor.becomeRoot(XOR100_tree, root_0);
}
pushFollow(FOLLOW_andExpression_in_xorExpression1953);
andExpression101=andExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, andExpression101.getTree());
}
break;
default :
break loop19;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 25, xorExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "xorExpression"
public static class andExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "andExpression"
// org/jaitools/jiffle/parser/Jiffle.g:270:1: andExpression : eqExpression ( AND eqExpression )* ;
public final JiffleParser.andExpression_return andExpression() throws RecognitionException {
JiffleParser.andExpression_return retval = new JiffleParser.andExpression_return();
retval.start = input.LT(1);
int andExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token AND103=null;
JiffleParser.eqExpression_return eqExpression102 = null;
JiffleParser.eqExpression_return eqExpression104 = null;
CommonTree AND103_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 26) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:270:17: ( eqExpression ( AND eqExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:270:19: eqExpression ( AND eqExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_eqExpression_in_andExpression1983);
eqExpression102=eqExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, eqExpression102.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:270:32: ( AND eqExpression )*
loop20:
do {
int alt20=2;
switch ( input.LA(1) ) {
case AND:
{
alt20=1;
}
break;
}
switch (alt20) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:270:33: AND eqExpression
{
AND103=(Token)match(input,AND,FOLLOW_AND_in_andExpression1986); if (state.failed) return retval;
if ( state.backtracking==0 ) {
AND103_tree = (CommonTree)adaptor.create(AND103);
root_0 = (CommonTree)adaptor.becomeRoot(AND103_tree, root_0);
}
pushFollow(FOLLOW_eqExpression_in_andExpression1989);
eqExpression104=eqExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, eqExpression104.getTree());
}
break;
default :
break loop20;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 26, andExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "andExpression"
public static class eqExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "eqExpression"
// org/jaitools/jiffle/parser/Jiffle.g:274:1: eqExpression : compExpression ( ( LOGICALEQ | NE ) compExpression )? ;
public final JiffleParser.eqExpression_return eqExpression() throws RecognitionException {
JiffleParser.eqExpression_return retval = new JiffleParser.eqExpression_return();
retval.start = input.LT(1);
int eqExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token LOGICALEQ106=null;
Token NE107=null;
JiffleParser.compExpression_return compExpression105 = null;
JiffleParser.compExpression_return compExpression108 = null;
CommonTree LOGICALEQ106_tree=null;
CommonTree NE107_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 27) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:274:17: ( compExpression ( ( LOGICALEQ | NE ) compExpression )? )
// org/jaitools/jiffle/parser/Jiffle.g:274:19: compExpression ( ( LOGICALEQ | NE ) compExpression )?
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_compExpression_in_eqExpression2020);
compExpression105=compExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compExpression105.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:274:34: ( ( LOGICALEQ | NE ) compExpression )?
int alt22=2;
switch ( input.LA(1) ) {
case LOGICALEQ:
case NE:
{
alt22=1;
}
break;
}
switch (alt22) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:274:35: ( LOGICALEQ | NE ) compExpression
{
// org/jaitools/jiffle/parser/Jiffle.g:274:35: ( LOGICALEQ | NE )
int alt21=2;
switch ( input.LA(1) ) {
case LOGICALEQ:
{
alt21=1;
}
break;
case NE:
{
alt21=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 21, 0, input);
throw nvae;
}
switch (alt21) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:274:36: LOGICALEQ
{
LOGICALEQ106=(Token)match(input,LOGICALEQ,FOLLOW_LOGICALEQ_in_eqExpression2024); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LOGICALEQ106_tree = (CommonTree)adaptor.create(LOGICALEQ106);
root_0 = (CommonTree)adaptor.becomeRoot(LOGICALEQ106_tree, root_0);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:274:49: NE
{
NE107=(Token)match(input,NE,FOLLOW_NE_in_eqExpression2029); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NE107_tree = (CommonTree)adaptor.create(NE107);
root_0 = (CommonTree)adaptor.becomeRoot(NE107_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_compExpression_in_eqExpression2033);
compExpression108=compExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, compExpression108.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 27, eqExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "eqExpression"
public static class compExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "compExpression"
// org/jaitools/jiffle/parser/Jiffle.g:278:1: compExpression : addExpression ( ( GT | GE | LE | LT ) addExpression )? ;
public final JiffleParser.compExpression_return compExpression() throws RecognitionException {
JiffleParser.compExpression_return retval = new JiffleParser.compExpression_return();
retval.start = input.LT(1);
int compExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token GT110=null;
Token GE111=null;
Token LE112=null;
Token LT113=null;
JiffleParser.addExpression_return addExpression109 = null;
JiffleParser.addExpression_return addExpression114 = null;
CommonTree GT110_tree=null;
CommonTree GE111_tree=null;
CommonTree LE112_tree=null;
CommonTree LT113_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 28) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:278:17: ( addExpression ( ( GT | GE | LE | LT ) addExpression )? )
// org/jaitools/jiffle/parser/Jiffle.g:278:19: addExpression ( ( GT | GE | LE | LT ) addExpression )?
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_addExpression_in_compExpression2062);
addExpression109=addExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, addExpression109.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:278:33: ( ( GT | GE | LE | LT ) addExpression )?
int alt24=2;
switch ( input.LA(1) ) {
case GT:
case GE:
case LE:
case LT:
{
alt24=1;
}
break;
}
switch (alt24) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:278:34: ( GT | GE | LE | LT ) addExpression
{
// org/jaitools/jiffle/parser/Jiffle.g:278:34: ( GT | GE | LE | LT )
int alt23=4;
switch ( input.LA(1) ) {
case GT:
{
alt23=1;
}
break;
case GE:
{
alt23=2;
}
break;
case LE:
{
alt23=3;
}
break;
case LT:
{
alt23=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 23, 0, input);
throw nvae;
}
switch (alt23) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:278:35: GT
{
GT110=(Token)match(input,GT,FOLLOW_GT_in_compExpression2066); if (state.failed) return retval;
if ( state.backtracking==0 ) {
GT110_tree = (CommonTree)adaptor.create(GT110);
root_0 = (CommonTree)adaptor.becomeRoot(GT110_tree, root_0);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:278:41: GE
{
GE111=(Token)match(input,GE,FOLLOW_GE_in_compExpression2071); if (state.failed) return retval;
if ( state.backtracking==0 ) {
GE111_tree = (CommonTree)adaptor.create(GE111);
root_0 = (CommonTree)adaptor.becomeRoot(GE111_tree, root_0);
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:278:47: LE
{
LE112=(Token)match(input,LE,FOLLOW_LE_in_compExpression2076); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LE112_tree = (CommonTree)adaptor.create(LE112);
root_0 = (CommonTree)adaptor.becomeRoot(LE112_tree, root_0);
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/Jiffle.g:278:53: LT
{
LT113=(Token)match(input,LT,FOLLOW_LT_in_compExpression2081); if (state.failed) return retval;
if ( state.backtracking==0 ) {
LT113_tree = (CommonTree)adaptor.create(LT113);
root_0 = (CommonTree)adaptor.becomeRoot(LT113_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_addExpression_in_compExpression2085);
addExpression114=addExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, addExpression114.getTree());
}
break;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 28, compExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "compExpression"
public static class addExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "addExpression"
// org/jaitools/jiffle/parser/Jiffle.g:282:1: addExpression : multExpression ( ( PLUS | MINUS ) multExpression )* ;
public final JiffleParser.addExpression_return addExpression() throws RecognitionException {
JiffleParser.addExpression_return retval = new JiffleParser.addExpression_return();
retval.start = input.LT(1);
int addExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token PLUS116=null;
Token MINUS117=null;
JiffleParser.multExpression_return multExpression115 = null;
JiffleParser.multExpression_return multExpression118 = null;
CommonTree PLUS116_tree=null;
CommonTree MINUS117_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 29) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:282:17: ( multExpression ( ( PLUS | MINUS ) multExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:282:19: multExpression ( ( PLUS | MINUS ) multExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_multExpression_in_addExpression2115);
multExpression115=multExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, multExpression115.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:282:34: ( ( PLUS | MINUS ) multExpression )*
loop26:
do {
int alt26=2;
switch ( input.LA(1) ) {
case PLUS:
case MINUS:
{
alt26=1;
}
break;
}
switch (alt26) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:282:35: ( PLUS | MINUS ) multExpression
{
// org/jaitools/jiffle/parser/Jiffle.g:282:35: ( PLUS | MINUS )
int alt25=2;
switch ( input.LA(1) ) {
case PLUS:
{
alt25=1;
}
break;
case MINUS:
{
alt25=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 25, 0, input);
throw nvae;
}
switch (alt25) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:282:36: PLUS
{
PLUS116=(Token)match(input,PLUS,FOLLOW_PLUS_in_addExpression2119); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PLUS116_tree = (CommonTree)adaptor.create(PLUS116);
root_0 = (CommonTree)adaptor.becomeRoot(PLUS116_tree, root_0);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:282:44: MINUS
{
MINUS117=(Token)match(input,MINUS,FOLLOW_MINUS_in_addExpression2124); if (state.failed) return retval;
if ( state.backtracking==0 ) {
MINUS117_tree = (CommonTree)adaptor.create(MINUS117);
root_0 = (CommonTree)adaptor.becomeRoot(MINUS117_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_multExpression_in_addExpression2128);
multExpression118=multExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, multExpression118.getTree());
}
break;
default :
break loop26;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 29, addExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "addExpression"
public static class multExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "multExpression"
// org/jaitools/jiffle/parser/Jiffle.g:286:1: multExpression : unaryExpression ( ( TIMES | DIV | MOD ) unaryExpression )* ;
public final JiffleParser.multExpression_return multExpression() throws RecognitionException {
JiffleParser.multExpression_return retval = new JiffleParser.multExpression_return();
retval.start = input.LT(1);
int multExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token TIMES120=null;
Token DIV121=null;
Token MOD122=null;
JiffleParser.unaryExpression_return unaryExpression119 = null;
JiffleParser.unaryExpression_return unaryExpression123 = null;
CommonTree TIMES120_tree=null;
CommonTree DIV121_tree=null;
CommonTree MOD122_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 30) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:286:17: ( unaryExpression ( ( TIMES | DIV | MOD ) unaryExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:286:19: unaryExpression ( ( TIMES | DIV | MOD ) unaryExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_unaryExpression_in_multExpression2157);
unaryExpression119=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unaryExpression119.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:286:35: ( ( TIMES | DIV | MOD ) unaryExpression )*
loop28:
do {
int alt28=2;
switch ( input.LA(1) ) {
case TIMES:
case DIV:
case MOD:
{
alt28=1;
}
break;
}
switch (alt28) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:286:36: ( TIMES | DIV | MOD ) unaryExpression
{
// org/jaitools/jiffle/parser/Jiffle.g:286:36: ( TIMES | DIV | MOD )
int alt27=3;
switch ( input.LA(1) ) {
case TIMES:
{
alt27=1;
}
break;
case DIV:
{
alt27=2;
}
break;
case MOD:
{
alt27=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 27, 0, input);
throw nvae;
}
switch (alt27) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:286:37: TIMES
{
TIMES120=(Token)match(input,TIMES,FOLLOW_TIMES_in_multExpression2161); if (state.failed) return retval;
if ( state.backtracking==0 ) {
TIMES120_tree = (CommonTree)adaptor.create(TIMES120);
root_0 = (CommonTree)adaptor.becomeRoot(TIMES120_tree, root_0);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:286:46: DIV
{
DIV121=(Token)match(input,DIV,FOLLOW_DIV_in_multExpression2166); if (state.failed) return retval;
if ( state.backtracking==0 ) {
DIV121_tree = (CommonTree)adaptor.create(DIV121);
root_0 = (CommonTree)adaptor.becomeRoot(DIV121_tree, root_0);
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:286:53: MOD
{
MOD122=(Token)match(input,MOD,FOLLOW_MOD_in_multExpression2171); if (state.failed) return retval;
if ( state.backtracking==0 ) {
MOD122_tree = (CommonTree)adaptor.create(MOD122);
root_0 = (CommonTree)adaptor.becomeRoot(MOD122_tree, root_0);
}
}
break;
}
pushFollow(FOLLOW_unaryExpression_in_multExpression2175);
unaryExpression123=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, unaryExpression123.getTree());
}
break;
default :
break loop28;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 30, multExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "multExpression"
public static class unaryExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "unaryExpression"
// org/jaitools/jiffle/parser/Jiffle.g:290:1: unaryExpression : ( prefixOp unaryExpression -> ^( PREFIX prefixOp unaryExpression ) | powerExpression );
public final JiffleParser.unaryExpression_return unaryExpression() throws RecognitionException {
JiffleParser.unaryExpression_return retval = new JiffleParser.unaryExpression_return();
retval.start = input.LT(1);
int unaryExpression_StartIndex = input.index();
CommonTree root_0 = null;
JiffleParser.prefixOp_return prefixOp124 = null;
JiffleParser.unaryExpression_return unaryExpression125 = null;
JiffleParser.powerExpression_return powerExpression126 = null;
RewriteRuleSubtreeStream stream_prefixOp=new RewriteRuleSubtreeStream(adaptor,"rule prefixOp");
RewriteRuleSubtreeStream stream_unaryExpression=new RewriteRuleSubtreeStream(adaptor,"rule unaryExpression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 31) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:290:17: ( prefixOp unaryExpression -> ^( PREFIX prefixOp unaryExpression ) | powerExpression )
int alt29=2;
switch ( input.LA(1) ) {
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
{
alt29=1;
}
break;
case ID:
case LPAR:
case CON:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt29=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 29, 0, input);
throw nvae;
}
switch (alt29) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:290:19: prefixOp unaryExpression
{
pushFollow(FOLLOW_prefixOp_in_unaryExpression2203);
prefixOp124=prefixOp();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_prefixOp.add(prefixOp124.getTree());
pushFollow(FOLLOW_unaryExpression_in_unaryExpression2205);
unaryExpression125=unaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_unaryExpression.add(unaryExpression125.getTree());
// AST REWRITE
// elements: prefixOp, unaryExpression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 290:44: -> ^( PREFIX prefixOp unaryExpression )
{
// org/jaitools/jiffle/parser/Jiffle.g:290:47: ^( PREFIX prefixOp unaryExpression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(PREFIX, "PREFIX"), root_1);
adaptor.addChild(root_1, stream_prefixOp.nextTree());
adaptor.addChild(root_1, stream_unaryExpression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:291:19: powerExpression
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_powerExpression_in_unaryExpression2235);
powerExpression126=powerExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, powerExpression126.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 31, unaryExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "unaryExpression"
public static class prefixOp_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "prefixOp"
// org/jaitools/jiffle/parser/Jiffle.g:295:1: prefixOp : ( PLUS | MINUS | NOT | incdecOp );
public final JiffleParser.prefixOp_return prefixOp() throws RecognitionException {
JiffleParser.prefixOp_return retval = new JiffleParser.prefixOp_return();
retval.start = input.LT(1);
int prefixOp_StartIndex = input.index();
CommonTree root_0 = null;
Token PLUS127=null;
Token MINUS128=null;
Token NOT129=null;
JiffleParser.incdecOp_return incdecOp130 = null;
CommonTree PLUS127_tree=null;
CommonTree MINUS128_tree=null;
CommonTree NOT129_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 32) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:295:17: ( PLUS | MINUS | NOT | incdecOp )
int alt30=4;
switch ( input.LA(1) ) {
case PLUS:
{
alt30=1;
}
break;
case MINUS:
{
alt30=2;
}
break;
case NOT:
{
alt30=3;
}
break;
case INCR:
case DECR:
{
alt30=4;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 30, 0, input);
throw nvae;
}
switch (alt30) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:295:19: PLUS
{
root_0 = (CommonTree)adaptor.nil();
PLUS127=(Token)match(input,PLUS,FOLLOW_PLUS_in_prefixOp2268); if (state.failed) return retval;
if ( state.backtracking==0 ) {
PLUS127_tree = (CommonTree)adaptor.create(PLUS127);
adaptor.addChild(root_0, PLUS127_tree);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:296:19: MINUS
{
root_0 = (CommonTree)adaptor.nil();
MINUS128=(Token)match(input,MINUS,FOLLOW_MINUS_in_prefixOp2288); if (state.failed) return retval;
if ( state.backtracking==0 ) {
MINUS128_tree = (CommonTree)adaptor.create(MINUS128);
adaptor.addChild(root_0, MINUS128_tree);
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:297:19: NOT
{
root_0 = (CommonTree)adaptor.nil();
NOT129=(Token)match(input,NOT,FOLLOW_NOT_in_prefixOp2308); if (state.failed) return retval;
if ( state.backtracking==0 ) {
NOT129_tree = (CommonTree)adaptor.create(NOT129);
adaptor.addChild(root_0, NOT129_tree);
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/Jiffle.g:298:19: incdecOp
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_incdecOp_in_prefixOp2328);
incdecOp130=incdecOp();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, incdecOp130.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 32, prefixOp_StartIndex); }
}
return retval;
}
// $ANTLR end "prefixOp"
public static class incdecOp_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "incdecOp"
// org/jaitools/jiffle/parser/Jiffle.g:302:1: incdecOp : ( INCR | DECR );
public final JiffleParser.incdecOp_return incdecOp() throws RecognitionException {
JiffleParser.incdecOp_return retval = new JiffleParser.incdecOp_return();
retval.start = input.LT(1);
int incdecOp_StartIndex = input.index();
CommonTree root_0 = null;
Token set131=null;
CommonTree set131_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 33) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:302:17: ( INCR | DECR )
// org/jaitools/jiffle/parser/Jiffle.g:
{
root_0 = (CommonTree)adaptor.nil();
set131=(Token)input.LT(1);
if ( (input.LA(1)>=INCR && input.LA(1)<=DECR) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (CommonTree)adaptor.create(set131));
state.errorRecovery=false;state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 33, incdecOp_StartIndex); }
}
return retval;
}
// $ANTLR end "incdecOp"
public static class powerExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "powerExpression"
// org/jaitools/jiffle/parser/Jiffle.g:307:1: powerExpression : primaryExpression ( POW primaryExpression )* ;
public final JiffleParser.powerExpression_return powerExpression() throws RecognitionException {
JiffleParser.powerExpression_return retval = new JiffleParser.powerExpression_return();
retval.start = input.LT(1);
int powerExpression_StartIndex = input.index();
CommonTree root_0 = null;
Token POW133=null;
JiffleParser.primaryExpression_return primaryExpression132 = null;
JiffleParser.primaryExpression_return primaryExpression134 = null;
CommonTree POW133_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 34) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:307:17: ( primaryExpression ( POW primaryExpression )* )
// org/jaitools/jiffle/parser/Jiffle.g:307:19: primaryExpression ( POW primaryExpression )*
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_primaryExpression_in_powerExpression2407);
primaryExpression132=primaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, primaryExpression132.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:307:37: ( POW primaryExpression )*
loop31:
do {
int alt31=2;
switch ( input.LA(1) ) {
case POW:
{
alt31=1;
}
break;
}
switch (alt31) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:307:38: POW primaryExpression
{
POW133=(Token)match(input,POW,FOLLOW_POW_in_powerExpression2410); if (state.failed) return retval;
if ( state.backtracking==0 ) {
POW133_tree = (CommonTree)adaptor.create(POW133);
root_0 = (CommonTree)adaptor.becomeRoot(POW133_tree, root_0);
}
pushFollow(FOLLOW_primaryExpression_in_powerExpression2413);
primaryExpression134=primaryExpression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, primaryExpression134.getTree());
}
break;
default :
break loop31;
}
} while (true);
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 34, powerExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "powerExpression"
public static class primaryExpression_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "primaryExpression"
// org/jaitools/jiffle/parser/Jiffle.g:311:1: primaryExpression : atom ( incdecOp )? -> {postfix}? ^( POSTFIX incdecOp atom ) -> atom ;
public final JiffleParser.primaryExpression_return primaryExpression() throws RecognitionException {
JiffleParser.primaryExpression_return retval = new JiffleParser.primaryExpression_return();
retval.start = input.LT(1);
int primaryExpression_StartIndex = input.index();
CommonTree root_0 = null;
JiffleParser.atom_return atom135 = null;
JiffleParser.incdecOp_return incdecOp136 = null;
RewriteRuleSubtreeStream stream_atom=new RewriteRuleSubtreeStream(adaptor,"rule atom");
RewriteRuleSubtreeStream stream_incdecOp=new RewriteRuleSubtreeStream(adaptor,"rule incdecOp");
boolean postfix = false;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 35) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:313:17: ( atom ( incdecOp )? -> {postfix}? ^( POSTFIX incdecOp atom ) -> atom )
// org/jaitools/jiffle/parser/Jiffle.g:313:19: atom ( incdecOp )?
{
pushFollow(FOLLOW_atom_in_primaryExpression2462);
atom135=atom();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_atom.add(atom135.getTree());
// org/jaitools/jiffle/parser/Jiffle.g:313:24: ( incdecOp )?
int alt32=2;
switch ( input.LA(1) ) {
case INCR:
case DECR:
{
alt32=1;
}
break;
}
switch (alt32) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:313:25: incdecOp
{
pushFollow(FOLLOW_incdecOp_in_primaryExpression2465);
incdecOp136=incdecOp();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_incdecOp.add(incdecOp136.getTree());
if ( state.backtracking==0 ) {
postfix = true;
}
}
break;
}
// AST REWRITE
// elements: atom, incdecOp, atom
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 314:19: -> {postfix}? ^( POSTFIX incdecOp atom )
if (postfix) {
// org/jaitools/jiffle/parser/Jiffle.g:314:33: ^( POSTFIX incdecOp atom )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(POSTFIX, "POSTFIX"), root_1);
adaptor.addChild(root_1, stream_incdecOp.nextTree());
adaptor.addChild(root_1, stream_atom.nextTree());
adaptor.addChild(root_0, root_1);
}
}
else // 315:19: -> atom
{
adaptor.addChild(root_0, stream_atom.nextTree());
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 35, primaryExpression_StartIndex); }
}
return retval;
}
// $ANTLR end "primaryExpression"
public static class atom_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "atom"
// org/jaitools/jiffle/parser/Jiffle.g:319:1: atom : ( LPAR expression RPAR -> ^( PAR expression ) | literal | listLiteral | conCall | identifiedAtom );
public final JiffleParser.atom_return atom() throws RecognitionException {
JiffleParser.atom_return retval = new JiffleParser.atom_return();
retval.start = input.LT(1);
int atom_StartIndex = input.index();
CommonTree root_0 = null;
Token LPAR137=null;
Token RPAR139=null;
JiffleParser.expression_return expression138 = null;
JiffleParser.literal_return literal140 = null;
JiffleParser.listLiteral_return listLiteral141 = null;
JiffleParser.conCall_return conCall142 = null;
JiffleParser.identifiedAtom_return identifiedAtom143 = null;
CommonTree LPAR137_tree=null;
CommonTree RPAR139_tree=null;
RewriteRuleTokenStream stream_RPAR=new RewriteRuleTokenStream(adaptor,"token RPAR");
RewriteRuleTokenStream stream_LPAR=new RewriteRuleTokenStream(adaptor,"token LPAR");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 36) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:319:17: ( LPAR expression RPAR -> ^( PAR expression ) | literal | listLiteral | conCall | identifiedAtom )
int alt33=5;
switch ( input.LA(1) ) {
case LPAR:
{
alt33=1;
}
break;
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt33=2;
}
break;
case LSQUARE:
{
alt33=3;
}
break;
case CON:
{
alt33=4;
}
break;
case ID:
{
alt33=5;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 33, 0, input);
throw nvae;
}
switch (alt33) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:319:19: LPAR expression RPAR
{
LPAR137=(Token)match(input,LPAR,FOLLOW_LPAR_in_atom2559); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LPAR.add(LPAR137);
pushFollow(FOLLOW_expression_in_atom2561);
expression138=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression138.getTree());
RPAR139=(Token)match(input,RPAR,FOLLOW_RPAR_in_atom2563); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RPAR.add(RPAR139);
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 319:40: -> ^( PAR expression )
{
// org/jaitools/jiffle/parser/Jiffle.g:319:43: ^( PAR expression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(PAR, "PAR"), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:320:19: literal
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_literal_in_atom2591);
literal140=literal();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, literal140.getTree());
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:321:19: listLiteral
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_listLiteral_in_atom2611);
listLiteral141=listLiteral();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, listLiteral141.getTree());
}
break;
case 4 :
// org/jaitools/jiffle/parser/Jiffle.g:322:19: conCall
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_conCall_in_atom2631);
conCall142=conCall();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, conCall142.getTree());
}
break;
case 5 :
// org/jaitools/jiffle/parser/Jiffle.g:323:19: identifiedAtom
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_identifiedAtom_in_atom2651);
identifiedAtom143=identifiedAtom();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, identifiedAtom143.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 36, atom_StartIndex); }
}
return retval;
}
// $ANTLR end "atom"
public static class identifiedAtom_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "identifiedAtom"
// org/jaitools/jiffle/parser/Jiffle.g:327:1: identifiedAtom : ( ID arguments -> ^( FUNC_CALL ID arguments ) | ID imagePos -> ^( IMAGE_POS ID imagePos ) | ID );
public final JiffleParser.identifiedAtom_return identifiedAtom() throws RecognitionException {
JiffleParser.identifiedAtom_return retval = new JiffleParser.identifiedAtom_return();
retval.start = input.LT(1);
int identifiedAtom_StartIndex = input.index();
CommonTree root_0 = null;
Token ID144=null;
Token ID146=null;
Token ID148=null;
JiffleParser.arguments_return arguments145 = null;
JiffleParser.imagePos_return imagePos147 = null;
CommonTree ID144_tree=null;
CommonTree ID146_tree=null;
CommonTree ID148_tree=null;
RewriteRuleTokenStream stream_ID=new RewriteRuleTokenStream(adaptor,"token ID");
RewriteRuleSubtreeStream stream_arguments=new RewriteRuleSubtreeStream(adaptor,"rule arguments");
RewriteRuleSubtreeStream stream_imagePos=new RewriteRuleSubtreeStream(adaptor,"rule imagePos");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 37) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:327:17: ( ID arguments -> ^( FUNC_CALL ID arguments ) | ID imagePos -> ^( IMAGE_POS ID imagePos ) | ID )
int alt34=3;
switch ( input.LA(1) ) {
case ID:
{
switch ( input.LA(2) ) {
case LPAR:
{
alt34=1;
}
break;
case LSQUARE:
{
alt34=2;
}
break;
case EOF:
case SEMI:
case RPAR:
case COMMA:
case COLON:
case QUESTION:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case INCR:
case DECR:
case POW:
case RSQUARE:
{
alt34=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 34, 1, input);
throw nvae;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 34, 0, input);
throw nvae;
}
switch (alt34) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:327:19: ID arguments
{
ID144=(Token)match(input,ID,FOLLOW_ID_in_identifiedAtom2678); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ID.add(ID144);
pushFollow(FOLLOW_arguments_in_identifiedAtom2680);
arguments145=arguments();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_arguments.add(arguments145.getTree());
// AST REWRITE
// elements: arguments, ID
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 327:32: -> ^( FUNC_CALL ID arguments )
{
// org/jaitools/jiffle/parser/Jiffle.g:327:35: ^( FUNC_CALL ID arguments )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(FUNC_CALL, "FUNC_CALL"), root_1);
adaptor.addChild(root_1, stream_ID.nextNode());
adaptor.addChild(root_1, stream_arguments.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:328:19: ID imagePos
{
ID146=(Token)match(input,ID,FOLLOW_ID_in_identifiedAtom2710); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ID.add(ID146);
pushFollow(FOLLOW_imagePos_in_identifiedAtom2712);
imagePos147=imagePos();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_imagePos.add(imagePos147.getTree());
// AST REWRITE
// elements: imagePos, ID
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 328:31: -> ^( IMAGE_POS ID imagePos )
{
// org/jaitools/jiffle/parser/Jiffle.g:328:34: ^( IMAGE_POS ID imagePos )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(IMAGE_POS, "IMAGE_POS"), root_1);
adaptor.addChild(root_1, stream_ID.nextNode());
adaptor.addChild(root_1, stream_imagePos.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:329:19: ID
{
root_0 = (CommonTree)adaptor.nil();
ID148=(Token)match(input,ID,FOLLOW_ID_in_identifiedAtom2742); if (state.failed) return retval;
if ( state.backtracking==0 ) {
ID148_tree = (CommonTree)adaptor.create(ID148);
adaptor.addChild(root_0, ID148_tree);
}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 37, identifiedAtom_StartIndex); }
}
return retval;
}
// $ANTLR end "identifiedAtom"
public static class arguments_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "arguments"
// org/jaitools/jiffle/parser/Jiffle.g:333:1: arguments : LPAR expressionList RPAR ;
public final JiffleParser.arguments_return arguments() throws RecognitionException {
JiffleParser.arguments_return retval = new JiffleParser.arguments_return();
retval.start = input.LT(1);
int arguments_StartIndex = input.index();
CommonTree root_0 = null;
Token LPAR149=null;
Token RPAR151=null;
JiffleParser.expressionList_return expressionList150 = null;
CommonTree LPAR149_tree=null;
CommonTree RPAR151_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 38) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:333:17: ( LPAR expressionList RPAR )
// org/jaitools/jiffle/parser/Jiffle.g:333:19: LPAR expressionList RPAR
{
root_0 = (CommonTree)adaptor.nil();
LPAR149=(Token)match(input,LPAR,FOLLOW_LPAR_in_arguments2774); if (state.failed) return retval;
pushFollow(FOLLOW_expressionList_in_arguments2777);
expressionList150=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, expressionList150.getTree());
RPAR151=(Token)match(input,RPAR,FOLLOW_RPAR_in_arguments2779); if (state.failed) return retval;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 38, arguments_StartIndex); }
}
return retval;
}
// $ANTLR end "arguments"
public static class imagePos_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "imagePos"
// org/jaitools/jiffle/parser/Jiffle.g:337:1: imagePos : ( bandSpecifier pixelSpecifier | pixelSpecifier | bandSpecifier );
public final JiffleParser.imagePos_return imagePos() throws RecognitionException {
JiffleParser.imagePos_return retval = new JiffleParser.imagePos_return();
retval.start = input.LT(1);
int imagePos_StartIndex = input.index();
CommonTree root_0 = null;
JiffleParser.bandSpecifier_return bandSpecifier152 = null;
JiffleParser.pixelSpecifier_return pixelSpecifier153 = null;
JiffleParser.pixelSpecifier_return pixelSpecifier154 = null;
JiffleParser.bandSpecifier_return bandSpecifier155 = null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 39) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:337:17: ( bandSpecifier pixelSpecifier | pixelSpecifier | bandSpecifier )
int alt35=3;
switch ( input.LA(1) ) {
case LSQUARE:
{
int LA35_1 = input.LA(2);
if ( (synpred60_Jiffle()) ) {
alt35=1;
}
else if ( (synpred61_Jiffle()) ) {
alt35=2;
}
else if ( (true) ) {
alt35=3;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 35, 1, input);
throw nvae;
}
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 35, 0, input);
throw nvae;
}
switch (alt35) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:337:19: bandSpecifier pixelSpecifier
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_bandSpecifier_in_imagePos2813);
bandSpecifier152=bandSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, bandSpecifier152.getTree());
pushFollow(FOLLOW_pixelSpecifier_in_imagePos2815);
pixelSpecifier153=pixelSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, pixelSpecifier153.getTree());
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:338:19: pixelSpecifier
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_pixelSpecifier_in_imagePos2835);
pixelSpecifier154=pixelSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, pixelSpecifier154.getTree());
}
break;
case 3 :
// org/jaitools/jiffle/parser/Jiffle.g:339:19: bandSpecifier
{
root_0 = (CommonTree)adaptor.nil();
pushFollow(FOLLOW_bandSpecifier_in_imagePos2855);
bandSpecifier155=bandSpecifier();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) adaptor.addChild(root_0, bandSpecifier155.getTree());
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 39, imagePos_StartIndex); }
}
return retval;
}
// $ANTLR end "imagePos"
public static class pixelSpecifier_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "pixelSpecifier"
// org/jaitools/jiffle/parser/Jiffle.g:343:1: pixelSpecifier : LSQUARE pixelPos COMMA pixelPos RSQUARE -> ^( PIXEL_REF pixelPos pixelPos ) ;
public final JiffleParser.pixelSpecifier_return pixelSpecifier() throws RecognitionException {
JiffleParser.pixelSpecifier_return retval = new JiffleParser.pixelSpecifier_return();
retval.start = input.LT(1);
int pixelSpecifier_StartIndex = input.index();
CommonTree root_0 = null;
Token LSQUARE156=null;
Token COMMA158=null;
Token RSQUARE160=null;
JiffleParser.pixelPos_return pixelPos157 = null;
JiffleParser.pixelPos_return pixelPos159 = null;
CommonTree LSQUARE156_tree=null;
CommonTree COMMA158_tree=null;
CommonTree RSQUARE160_tree=null;
RewriteRuleTokenStream stream_LSQUARE=new RewriteRuleTokenStream(adaptor,"token LSQUARE");
RewriteRuleTokenStream stream_RSQUARE=new RewriteRuleTokenStream(adaptor,"token RSQUARE");
RewriteRuleTokenStream stream_COMMA=new RewriteRuleTokenStream(adaptor,"token COMMA");
RewriteRuleSubtreeStream stream_pixelPos=new RewriteRuleSubtreeStream(adaptor,"rule pixelPos");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 40) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:343:17: ( LSQUARE pixelPos COMMA pixelPos RSQUARE -> ^( PIXEL_REF pixelPos pixelPos ) )
// org/jaitools/jiffle/parser/Jiffle.g:343:19: LSQUARE pixelPos COMMA pixelPos RSQUARE
{
LSQUARE156=(Token)match(input,LSQUARE,FOLLOW_LSQUARE_in_pixelSpecifier2882); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LSQUARE.add(LSQUARE156);
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier2884);
pixelPos157=pixelPos();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_pixelPos.add(pixelPos157.getTree());
COMMA158=(Token)match(input,COMMA,FOLLOW_COMMA_in_pixelSpecifier2886); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_COMMA.add(COMMA158);
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier2888);
pixelPos159=pixelPos();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_pixelPos.add(pixelPos159.getTree());
RSQUARE160=(Token)match(input,RSQUARE,FOLLOW_RSQUARE_in_pixelSpecifier2890); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RSQUARE.add(RSQUARE160);
// AST REWRITE
// elements: pixelPos, pixelPos
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 343:59: -> ^( PIXEL_REF pixelPos pixelPos )
{
// org/jaitools/jiffle/parser/Jiffle.g:343:62: ^( PIXEL_REF pixelPos pixelPos )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(PIXEL_REF, "PIXEL_REF"), root_1);
adaptor.addChild(root_1, stream_pixelPos.nextTree());
adaptor.addChild(root_1, stream_pixelPos.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 40, pixelSpecifier_StartIndex); }
}
return retval;
}
// $ANTLR end "pixelSpecifier"
public static class bandSpecifier_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "bandSpecifier"
// org/jaitools/jiffle/parser/Jiffle.g:347:1: bandSpecifier : LSQUARE expression RSQUARE -> ^( BAND_REF expression ) ;
public final JiffleParser.bandSpecifier_return bandSpecifier() throws RecognitionException {
JiffleParser.bandSpecifier_return retval = new JiffleParser.bandSpecifier_return();
retval.start = input.LT(1);
int bandSpecifier_StartIndex = input.index();
CommonTree root_0 = null;
Token LSQUARE161=null;
Token RSQUARE163=null;
JiffleParser.expression_return expression162 = null;
CommonTree LSQUARE161_tree=null;
CommonTree RSQUARE163_tree=null;
RewriteRuleTokenStream stream_LSQUARE=new RewriteRuleTokenStream(adaptor,"token LSQUARE");
RewriteRuleTokenStream stream_RSQUARE=new RewriteRuleTokenStream(adaptor,"token RSQUARE");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 41) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:347:17: ( LSQUARE expression RSQUARE -> ^( BAND_REF expression ) )
// org/jaitools/jiffle/parser/Jiffle.g:347:19: LSQUARE expression RSQUARE
{
LSQUARE161=(Token)match(input,LSQUARE,FOLLOW_LSQUARE_in_bandSpecifier2928); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LSQUARE.add(LSQUARE161);
pushFollow(FOLLOW_expression_in_bandSpecifier2930);
expression162=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression162.getTree());
RSQUARE163=(Token)match(input,RSQUARE,FOLLOW_RSQUARE_in_bandSpecifier2932); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RSQUARE.add(RSQUARE163);
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 347:46: -> ^( BAND_REF expression )
{
// org/jaitools/jiffle/parser/Jiffle.g:347:49: ^( BAND_REF expression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(BAND_REF, "BAND_REF"), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 41, bandSpecifier_StartIndex); }
}
return retval;
}
// $ANTLR end "bandSpecifier"
public static class pixelPos_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "pixelPos"
// org/jaitools/jiffle/parser/Jiffle.g:351:1: pixelPos : ( ABS_POS_PREFIX expression -> ^( ABS_POS expression ) | expression -> ^( REL_POS expression ) );
public final JiffleParser.pixelPos_return pixelPos() throws RecognitionException {
JiffleParser.pixelPos_return retval = new JiffleParser.pixelPos_return();
retval.start = input.LT(1);
int pixelPos_StartIndex = input.index();
CommonTree root_0 = null;
Token ABS_POS_PREFIX164=null;
JiffleParser.expression_return expression165 = null;
JiffleParser.expression_return expression166 = null;
CommonTree ABS_POS_PREFIX164_tree=null;
RewriteRuleTokenStream stream_ABS_POS_PREFIX=new RewriteRuleTokenStream(adaptor,"token ABS_POS_PREFIX");
RewriteRuleSubtreeStream stream_expression=new RewriteRuleSubtreeStream(adaptor,"rule expression");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 42) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:351:17: ( ABS_POS_PREFIX expression -> ^( ABS_POS expression ) | expression -> ^( REL_POS expression ) )
int alt36=2;
switch ( input.LA(1) ) {
case ABS_POS_PREFIX:
{
alt36=1;
}
break;
case ID:
case LPAR:
case CON:
case PLUS:
case MINUS:
case NOT:
case INCR:
case DECR:
case LSQUARE:
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt36=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 36, 0, input);
throw nvae;
}
switch (alt36) {
case 1 :
// org/jaitools/jiffle/parser/Jiffle.g:351:19: ABS_POS_PREFIX expression
{
ABS_POS_PREFIX164=(Token)match(input,ABS_POS_PREFIX,FOLLOW_ABS_POS_PREFIX_in_pixelPos2973); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_ABS_POS_PREFIX.add(ABS_POS_PREFIX164);
pushFollow(FOLLOW_expression_in_pixelPos2975);
expression165=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression165.getTree());
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 351:45: -> ^( ABS_POS expression )
{
// org/jaitools/jiffle/parser/Jiffle.g:351:48: ^( ABS_POS expression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(ABS_POS, "ABS_POS"), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
case 2 :
// org/jaitools/jiffle/parser/Jiffle.g:352:19: expression
{
pushFollow(FOLLOW_expression_in_pixelPos3003);
expression166=expression();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expression.add(expression166.getTree());
// AST REWRITE
// elements: expression
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 352:30: -> ^( REL_POS expression )
{
// org/jaitools/jiffle/parser/Jiffle.g:352:33: ^( REL_POS expression )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(REL_POS, "REL_POS"), root_1);
adaptor.addChild(root_1, stream_expression.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
break;
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 42, pixelPos_StartIndex); }
}
return retval;
}
// $ANTLR end "pixelPos"
public static class literal_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "literal"
// org/jaitools/jiffle/parser/Jiffle.g:356:1: literal : ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL );
public final JiffleParser.literal_return literal() throws RecognitionException {
JiffleParser.literal_return retval = new JiffleParser.literal_return();
retval.start = input.LT(1);
int literal_StartIndex = input.index();
CommonTree root_0 = null;
Token set167=null;
CommonTree set167_tree=null;
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 43) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:356:17: ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL )
// org/jaitools/jiffle/parser/Jiffle.g:
{
root_0 = (CommonTree)adaptor.nil();
set167=(Token)input.LT(1);
if ( (input.LA(1)>=INT_LITERAL && input.LA(1)<=NULL) ) {
input.consume();
if ( state.backtracking==0 ) adaptor.addChild(root_0, (CommonTree)adaptor.create(set167));
state.errorRecovery=false;state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 43, literal_StartIndex); }
}
return retval;
}
// $ANTLR end "literal"
public static class listLiteral_return extends ParserRuleReturnScope {
CommonTree tree;
public Object getTree() { return tree; }
};
// $ANTLR start "listLiteral"
// org/jaitools/jiffle/parser/Jiffle.g:364:1: listLiteral : LSQUARE expressionList RSQUARE -> ^( DECLARED_LIST expressionList ) ;
public final JiffleParser.listLiteral_return listLiteral() throws RecognitionException {
JiffleParser.listLiteral_return retval = new JiffleParser.listLiteral_return();
retval.start = input.LT(1);
int listLiteral_StartIndex = input.index();
CommonTree root_0 = null;
Token LSQUARE168=null;
Token RSQUARE170=null;
JiffleParser.expressionList_return expressionList169 = null;
CommonTree LSQUARE168_tree=null;
CommonTree RSQUARE170_tree=null;
RewriteRuleTokenStream stream_LSQUARE=new RewriteRuleTokenStream(adaptor,"token LSQUARE");
RewriteRuleTokenStream stream_RSQUARE=new RewriteRuleTokenStream(adaptor,"token RSQUARE");
RewriteRuleSubtreeStream stream_expressionList=new RewriteRuleSubtreeStream(adaptor,"rule expressionList");
try {
if ( state.backtracking>0 && alreadyParsedRule(input, 44) ) { return retval; }
// org/jaitools/jiffle/parser/Jiffle.g:364:17: ( LSQUARE expressionList RSQUARE -> ^( DECLARED_LIST expressionList ) )
// org/jaitools/jiffle/parser/Jiffle.g:364:19: LSQUARE expressionList RSQUARE
{
LSQUARE168=(Token)match(input,LSQUARE,FOLLOW_LSQUARE_in_listLiteral3155); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_LSQUARE.add(LSQUARE168);
pushFollow(FOLLOW_expressionList_in_listLiteral3157);
expressionList169=expressionList();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==0 ) stream_expressionList.add(expressionList169.getTree());
RSQUARE170=(Token)match(input,RSQUARE,FOLLOW_RSQUARE_in_listLiteral3159); if (state.failed) return retval;
if ( state.backtracking==0 ) stream_RSQUARE.add(RSQUARE170);
// AST REWRITE
// elements: expressionList
// token labels:
// rule labels: retval
// token list labels:
// rule list labels:
// wildcard labels:
if ( state.backtracking==0 ) {
retval.tree = root_0;
RewriteRuleSubtreeStream stream_retval=new RewriteRuleSubtreeStream(adaptor,"rule retval",retval!=null?retval.tree:null);
root_0 = (CommonTree)adaptor.nil();
// 364:50: -> ^( DECLARED_LIST expressionList )
{
// org/jaitools/jiffle/parser/Jiffle.g:364:53: ^( DECLARED_LIST expressionList )
{
CommonTree root_1 = (CommonTree)adaptor.nil();
root_1 = (CommonTree)adaptor.becomeRoot((CommonTree)adaptor.create(DECLARED_LIST, "DECLARED_LIST"), root_1);
adaptor.addChild(root_1, stream_expressionList.nextTree());
adaptor.addChild(root_0, root_1);
}
}
retval.tree = root_0;}
}
retval.stop = input.LT(-1);
if ( state.backtracking==0 ) {
retval.tree = (CommonTree)adaptor.rulePostProcessing(root_0);
adaptor.setTokenBoundaries(retval.tree, retval.start, retval.stop);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
retval.tree = (CommonTree)adaptor.errorNode(input, retval.start, input.LT(-1), re);
}
finally {
if ( state.backtracking>0 ) { memoize(input, 44, listLiteral_StartIndex); }
}
return retval;
}
// $ANTLR end "listLiteral"
// $ANTLR start synpred19_Jiffle
public final void synpred19_Jiffle_fragment() throws RecognitionException {
JiffleParser.statement_return s2 = null;
// org/jaitools/jiffle/parser/Jiffle.g:194:21: ( ELSE s2= statement )
// org/jaitools/jiffle/parser/Jiffle.g:194:21: ELSE s2= statement
{
match(input,ELSE,FOLLOW_ELSE_in_synpred19_Jiffle1177); if (state.failed) return ;
pushFollow(FOLLOW_statement_in_synpred19_Jiffle1181);
s2=statement();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred19_Jiffle
// $ANTLR start synpred22_Jiffle
public final void synpred22_Jiffle_fragment() throws RecognitionException {
// org/jaitools/jiffle/parser/Jiffle.g:211:19: ( listLiteral )
// org/jaitools/jiffle/parser/Jiffle.g:211:19: listLiteral
{
pushFollow(FOLLOW_listLiteral_in_synpred22_Jiffle1404);
listLiteral();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred22_Jiffle
// $ANTLR start synpred23_Jiffle
public final void synpred23_Jiffle_fragment() throws RecognitionException {
// org/jaitools/jiffle/parser/Jiffle.g:212:19: ( sequence )
// org/jaitools/jiffle/parser/Jiffle.g:212:19: sequence
{
pushFollow(FOLLOW_sequence_in_synpred23_Jiffle1424);
sequence();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred23_Jiffle
// $ANTLR start synpred60_Jiffle
public final void synpred60_Jiffle_fragment() throws RecognitionException {
// org/jaitools/jiffle/parser/Jiffle.g:337:19: ( bandSpecifier pixelSpecifier )
// org/jaitools/jiffle/parser/Jiffle.g:337:19: bandSpecifier pixelSpecifier
{
pushFollow(FOLLOW_bandSpecifier_in_synpred60_Jiffle2813);
bandSpecifier();
state._fsp--;
if (state.failed) return ;
pushFollow(FOLLOW_pixelSpecifier_in_synpred60_Jiffle2815);
pixelSpecifier();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred60_Jiffle
// $ANTLR start synpred61_Jiffle
public final void synpred61_Jiffle_fragment() throws RecognitionException {
// org/jaitools/jiffle/parser/Jiffle.g:338:19: ( pixelSpecifier )
// org/jaitools/jiffle/parser/Jiffle.g:338:19: pixelSpecifier
{
pushFollow(FOLLOW_pixelSpecifier_in_synpred61_Jiffle2835);
pixelSpecifier();
state._fsp--;
if (state.failed) return ;
}
}
// $ANTLR end synpred61_Jiffle
// Delegated rules
public final boolean synpred23_Jiffle() {
state.backtracking++;
int start = input.mark();
try {
synpred23_Jiffle_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred60_Jiffle() {
state.backtracking++;
int start = input.mark();
try {
synpred60_Jiffle_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred19_Jiffle() {
state.backtracking++;
int start = input.mark();
try {
synpred19_Jiffle_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred22_Jiffle() {
state.backtracking++;
int start = input.mark();
try {
synpred22_Jiffle_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred61_Jiffle() {
state.backtracking++;
int start = input.mark();
try {
synpred61_Jiffle_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
protected DFA10 dfa10 = new DFA10(this);
protected DFA13 dfa13 = new DFA13(this);
static final String DFA10_eotS =
"\12\uffff";
static final String DFA10_eofS =
"\12\uffff";
static final String DFA10_minS =
"\1\40\3\uffff\1\43\5\uffff";
static final String DFA10_maxS =
"\1\126\3\uffff\1\117\5\uffff";
static final String DFA10_acceptS =
"\1\uffff\1\1\1\2\1\3\1\uffff\1\5\1\6\1\7\1\10\1\4";
static final String DFA10_specialS =
"\12\uffff}>";
static final String[] DFA10_transitionS = {
"\1\2\1\uffff\1\4\1\uffff\1\10\4\uffff\1\5\1\3\1\uffff\1\6\1"+
"\7\1\uffff\1\1\1\uffff\2\3\2\uffff\1\3\20\uffff\2\3\3\uffff"+
"\3\3\1\uffff\1\3\2\uffff\5\3",
"",
"",
"",
"\1\11\1\3\5\uffff\1\3\13\uffff\1\3\5\11\17\3\1\uffff\4\3",
"",
"",
"",
"",
""
};
static final short[] DFA10_eot = DFA.unpackEncodedString(DFA10_eotS);
static final short[] DFA10_eof = DFA.unpackEncodedString(DFA10_eofS);
static final char[] DFA10_min = DFA.unpackEncodedStringToUnsignedChars(DFA10_minS);
static final char[] DFA10_max = DFA.unpackEncodedStringToUnsignedChars(DFA10_maxS);
static final short[] DFA10_accept = DFA.unpackEncodedString(DFA10_acceptS);
static final short[] DFA10_special = DFA.unpackEncodedString(DFA10_specialS);
static final short[][] DFA10_transition;
static {
int numStates = DFA10_transitionS.length;
DFA10_transition = new short[numStates][];
for (int i=0; i ^( WHILE loopCondition statement ) | UNTIL LPAR loopCondition RPAR statement -> ^( UNTIL loopCondition statement ) | FOREACH LPAR ID IN loopSet RPAR statement -> ^( FOREACH ID loopSet statement ) | SEMI );";
}
}
static final String DFA13_eotS =
"\14\uffff";
static final String DFA13_eofS =
"\14\uffff";
static final String DFA13_minS =
"\1\42\1\0\7\uffff\1\0\2\uffff";
static final String DFA13_maxS =
"\1\126\1\0\7\uffff\1\0\2\uffff";
static final String DFA13_acceptS =
"\2\uffff\1\2\7\uffff\1\1\1\3";
static final String DFA13_specialS =
"\1\uffff\1\0\7\uffff\1\1\2\uffff}>";
static final String[] DFA13_transitionS = {
"\1\11\7\uffff\1\2\12\uffff\1\2\20\uffff\2\2\3\uffff\3\2\1\uffff"+
"\1\1\2\uffff\5\2",
"\1\uffff",
"",
"",
"",
"",
"",
"",
"",
"\1\uffff",
"",
""
};
static final short[] DFA13_eot = DFA.unpackEncodedString(DFA13_eotS);
static final short[] DFA13_eof = DFA.unpackEncodedString(DFA13_eofS);
static final char[] DFA13_min = DFA.unpackEncodedStringToUnsignedChars(DFA13_minS);
static final char[] DFA13_max = DFA.unpackEncodedStringToUnsignedChars(DFA13_maxS);
static final short[] DFA13_accept = DFA.unpackEncodedString(DFA13_acceptS);
static final short[] DFA13_special = DFA.unpackEncodedString(DFA13_specialS);
static final short[][] DFA13_transition;
static {
int numStates = DFA13_transitionS.length;
DFA13_transition = new short[numStates][];
for (int i=0; i=0 ) return s;
break;
case 1 :
int LA13_9 = input.LA(1);
int index13_9 = input.index();
input.rewind();
s = -1;
if ( (synpred23_Jiffle()) ) {s = 2;}
else if ( (true) ) {s = 11;}
input.seek(index13_9);
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 13, _s, input);
error(nvae);
throw nvae;
}
}
public static final BitSet FOLLOW_specialBlock_in_prog299 = new BitSet(new long[]{0x0026B73580000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_prog306 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_EOF_in_prog309 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_optionsBlock_in_specialBlock383 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_imagesBlock_in_specialBlock403 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_initBlock_in_specialBlock423 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OPTIONS_in_optionsBlock452 = new BitSet(new long[]{0x0000000100000000L});
public static final BitSet FOLLOW_LCURLY_in_optionsBlock454 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_option_in_optionsBlock456 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_RCURLY_in_optionsBlock459 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_option498 = new BitSet(new long[]{0x0000000800000000L});
public static final BitSet FOLLOW_EQ_in_option500 = new BitSet(new long[]{0x0000000400000000L,0x00000000007C0000L});
public static final BitSet FOLLOW_optionValue_in_option502 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_SEMI_in_option504 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_optionValue544 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_optionValue564 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IMAGES_in_imagesBlock595 = new BitSet(new long[]{0x0000000100000000L});
public static final BitSet FOLLOW_LCURLY_in_imagesBlock597 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_imageVarDeclaration_in_imagesBlock599 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_RCURLY_in_imagesBlock602 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_imageVarDeclaration647 = new BitSet(new long[]{0x0000000800000000L});
public static final BitSet FOLLOW_EQ_in_imageVarDeclaration649 = new BitSet(new long[]{0x000000C000000000L});
public static final BitSet FOLLOW_role_in_imageVarDeclaration651 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_SEMI_in_imageVarDeclaration653 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_role0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INIT_in_initBlock760 = new BitSet(new long[]{0x0000000100000000L});
public static final BitSet FOLLOW_LCURLY_in_initBlock762 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_varDeclaration_in_initBlock764 = new BitSet(new long[]{0x0000000600000000L});
public static final BitSet FOLLOW_RCURLY_in_initBlock767 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_varDeclaration799 = new BitSet(new long[]{0x0000001800000000L});
public static final BitSet FOLLOW_EQ_in_varDeclaration802 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_varDeclaration804 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_SEMI_in_varDeclaration808 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LCURLY_in_block857 = new BitSet(new long[]{0x0026B61700000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_block859 = new BitSet(new long[]{0x0026B61700000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_RCURLY_in_block862 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ifCall_in_statement903 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_block_in_statement923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_delimitedStatement_in_statement943 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_SEMI_in_statement945 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_assignmentExpression_in_statement966 = new BitSet(new long[]{0x0000001000000000L});
public static final BitSet FOLLOW_SEMI_in_statement968 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WHILE_in_statement989 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_statement991 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_loopCondition_in_statement993 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_statement995 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_statement997 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_UNTIL_in_statement1027 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_statement1029 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_loopCondition_in_statement1031 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_statement1033 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_statement1035 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FOREACH_in_statement1065 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_statement1067 = new BitSet(new long[]{0x0000000400000000L});
public static final BitSet FOLLOW_ID_in_statement1069 = new BitSet(new long[]{0x0000400000000000L});
public static final BitSet FOLLOW_IN_in_statement1071 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_loopSet_in_statement1073 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_statement1075 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_statement1077 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_SEMI_in_statement1109 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IF_in_ifCall1145 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_ifCall1147 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_orExpression_in_ifCall1149 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_ifCall1151 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_ifCall1155 = new BitSet(new long[]{0x0001000000000002L});
public static final BitSet FOLLOW_ELSE_in_ifCall1177 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_ifCall1181 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_delimitedStatement1288 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_BREAKIF_in_delimitedStatement1308 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_delimitedStatement1310 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_delimitedStatement1312 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_delimitedStatement1314 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_BREAK_in_delimitedStatement1342 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_orExpression_in_loopCondition1370 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listLiteral_in_loopSet1404 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_sequence_in_loopSet1424 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_loopSet1444 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_expressionList1472 = new BitSet(new long[]{0x0008000000000002L});
public static final BitSet FOLLOW_COMMA_in_expressionList1475 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_expressionList1477 = new BitSet(new long[]{0x0008000000000002L});
public static final BitSet FOLLOW_expression_in_sequence1526 = new BitSet(new long[]{0x0010000000000000L});
public static final BitSet FOLLOW_COLON_in_sequence1528 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_sequence1532 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CON_in_conCall1580 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_LPAR_in_conCall1582 = new BitSet(new long[]{0x00200C0400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expressionList_in_conCall1584 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_conCall1586 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_assignmentExpression1638 = new BitSet(new long[]{0x0F80000800000000L});
public static final BitSet FOLLOW_assignmentOp_in_assignmentExpression1640 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_assignmentExpression1643 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_conditionalExpression_in_expression1673 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_expression1693 = new BitSet(new long[]{0x0040000000000000L});
public static final BitSet FOLLOW_APPEND_in_expression1695 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_expression1698 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_assignmentOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_orExpression_in_conditionalExpression1869 = new BitSet(new long[]{0x1000000000000002L});
public static final BitSet FOLLOW_QUESTION_in_conditionalExpression1872 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_conditionalExpression1875 = new BitSet(new long[]{0x0010000000000000L});
public static final BitSet FOLLOW_COLON_in_conditionalExpression1877 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_conditionalExpression1880 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_xorExpression_in_orExpression1911 = new BitSet(new long[]{0x2000000000000002L});
public static final BitSet FOLLOW_OR_in_orExpression1914 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_xorExpression_in_orExpression1917 = new BitSet(new long[]{0x2000000000000002L});
public static final BitSet FOLLOW_andExpression_in_xorExpression1947 = new BitSet(new long[]{0x4000000000000002L});
public static final BitSet FOLLOW_XOR_in_xorExpression1950 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_andExpression_in_xorExpression1953 = new BitSet(new long[]{0x4000000000000002L});
public static final BitSet FOLLOW_eqExpression_in_andExpression1983 = new BitSet(new long[]{0x8000000000000002L});
public static final BitSet FOLLOW_AND_in_andExpression1986 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_eqExpression_in_andExpression1989 = new BitSet(new long[]{0x8000000000000002L});
public static final BitSet FOLLOW_compExpression_in_eqExpression2020 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000003L});
public static final BitSet FOLLOW_LOGICALEQ_in_eqExpression2024 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_NE_in_eqExpression2029 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_compExpression_in_eqExpression2033 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_addExpression_in_compExpression2062 = new BitSet(new long[]{0x0000000000000002L,0x000000000000003CL});
public static final BitSet FOLLOW_GT_in_compExpression2066 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_GE_in_compExpression2071 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_LE_in_compExpression2076 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_LT_in_compExpression2081 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_addExpression_in_compExpression2085 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_multExpression_in_addExpression2115 = new BitSet(new long[]{0x0000000000000002L,0x00000000000000C0L});
public static final BitSet FOLLOW_PLUS_in_addExpression2119 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_MINUS_in_addExpression2124 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_multExpression_in_addExpression2128 = new BitSet(new long[]{0x0000000000000002L,0x00000000000000C0L});
public static final BitSet FOLLOW_unaryExpression_in_multExpression2157 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000700L});
public static final BitSet FOLLOW_TIMES_in_multExpression2161 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_DIV_in_multExpression2166 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_MOD_in_multExpression2171 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_unaryExpression_in_multExpression2175 = new BitSet(new long[]{0x0000000000000002L,0x0000000000000700L});
public static final BitSet FOLLOW_prefixOp_in_unaryExpression2203 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_unaryExpression_in_unaryExpression2205 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_powerExpression_in_unaryExpression2235 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PLUS_in_prefixOp2268 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MINUS_in_prefixOp2288 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_in_prefixOp2308 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_incdecOp_in_prefixOp2328 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_incdecOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_primaryExpression_in_powerExpression2407 = new BitSet(new long[]{0x0000000000000002L,0x0000000000004000L});
public static final BitSet FOLLOW_POW_in_powerExpression2410 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_primaryExpression_in_powerExpression2413 = new BitSet(new long[]{0x0000000000000002L,0x0000000000004000L});
public static final BitSet FOLLOW_atom_in_primaryExpression2462 = new BitSet(new long[]{0x0000000000000002L,0x00000000000038C0L});
public static final BitSet FOLLOW_incdecOp_in_primaryExpression2465 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LPAR_in_atom2559 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_atom2561 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_atom2563 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_atom2591 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listLiteral_in_atom2611 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_conCall_in_atom2631 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_identifiedAtom_in_atom2651 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_identifiedAtom2678 = new BitSet(new long[]{0x0000040000000000L});
public static final BitSet FOLLOW_arguments_in_identifiedAtom2680 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_identifiedAtom2710 = new BitSet(new long[]{0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_imagePos_in_identifiedAtom2712 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ID_in_identifiedAtom2742 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LPAR_in_arguments2774 = new BitSet(new long[]{0x00200C0400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expressionList_in_arguments2777 = new BitSet(new long[]{0x0000080000000000L});
public static final BitSet FOLLOW_RPAR_in_arguments2779 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_bandSpecifier_in_imagePos2813 = new BitSet(new long[]{0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_pixelSpecifier_in_imagePos2815 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pixelSpecifier_in_imagePos2835 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_bandSpecifier_in_imagePos2855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LSQUARE_in_pixelSpecifier2882 = new BitSet(new long[]{0x0020040400000000L,0x00000000007EB8C0L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier2884 = new BitSet(new long[]{0x0008000000000000L});
public static final BitSet FOLLOW_COMMA_in_pixelSpecifier2886 = new BitSet(new long[]{0x0020040400000000L,0x00000000007EB8C0L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier2888 = new BitSet(new long[]{0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_RSQUARE_in_pixelSpecifier2890 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LSQUARE_in_bandSpecifier2928 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_bandSpecifier2930 = new BitSet(new long[]{0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_RSQUARE_in_bandSpecifier2932 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ABS_POS_PREFIX_in_pixelPos2973 = new BitSet(new long[]{0x0020040400000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_expression_in_pixelPos2975 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_pixelPos3003 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_literal0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_LSQUARE_in_listLiteral3155 = new BitSet(new long[]{0x0020040400000000L,0x00000000007DB8C0L});
public static final BitSet FOLLOW_expressionList_in_listLiteral3157 = new BitSet(new long[]{0x0000000000000000L,0x0000000000010000L});
public static final BitSet FOLLOW_RSQUARE_in_listLiteral3159 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ELSE_in_synpred19_Jiffle1177 = new BitSet(new long[]{0x0026B61500000000L,0x00000000007CB8C0L});
public static final BitSet FOLLOW_statement_in_synpred19_Jiffle1181 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listLiteral_in_synpred22_Jiffle1404 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_sequence_in_synpred23_Jiffle1424 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_bandSpecifier_in_synpred60_Jiffle2813 = new BitSet(new long[]{0x0000000000000000L,0x0000000000008000L});
public static final BitSet FOLLOW_pixelSpecifier_in_synpred60_Jiffle2815 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_pixelSpecifier_in_synpred61_Jiffle2835 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy