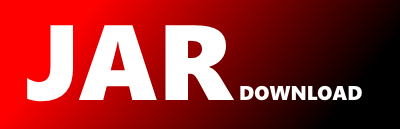
org.jaitools.jiffle.parser.OptionsBlockReader Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/OptionsBlockReader.g 2012-10-10 10:44:32
package org.jaitools.jiffle.parser;
import java.util.Map;
import org.jaitools.CollectionFactory;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
/**
* Reads the options block, if present.
*
* @author Michael Bedward
*/
public class OptionsBlockReader extends TreeFilter {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ABS_POS", "BAND_REF", "BLOCK", "CON_CALL", "DECL", "DECLARED_LIST", "EXPR_LIST", "FUNC_CALL", "IMAGE_POS", "JIFFLE_OPTION", "PAR", "PIXEL_REF", "POSTFIX", "PREFIX", "REL_POS", "SEQUENCE", "VAR_DEST", "VAR_IMAGE_SCOPE", "VAR_SOURCE", "CONSTANT", "IMAGE_WRITE", "LIST_NEW", "VAR_IMAGE", "VAR_PIXEL_SCOPE", "VAR_PROVIDED", "VAR_LOOP", "VAR_LIST", "OPTIONS", "LCURLY", "RCURLY", "ID", "EQ", "SEMI", "IMAGES", "READ", "WRITE", "INIT", "WHILE", "LPAR", "RPAR", "UNTIL", "FOREACH", "IN", "IF", "ELSE", "BREAKIF", "BREAK", "COMMA", "COLON", "CON", "APPEND", "TIMESEQ", "DIVEQ", "MODEQ", "PLUSEQ", "MINUSEQ", "QUESTION", "OR", "XOR", "AND", "LOGICALEQ", "NE", "GT", "GE", "LE", "LT", "PLUS", "MINUS", "TIMES", "DIV", "MOD", "NOT", "INCR", "DECR", "POW", "LSQUARE", "RSQUARE", "ABS_POS_PREFIX", "INT_LITERAL", "FLOAT_LITERAL", "TRUE", "FALSE", "NULL", "COMMENT", "INT_TYPE", "FLOAT_TYPE", "DOUBLE_TYPE", "BOOLEAN_TYPE", "Letter", "UNDERSCORE", "Digit", "Dot", "NonZeroDigit", "FloatExp", "WS", "ESC_SEQ", "CHAR", "HEX_DIGIT", "UNICODE_ESC", "OCTAL_ESC"
};
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public OptionsBlockReader(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public OptionsBlockReader(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return OptionsBlockReader.tokenNames; }
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/OptionsBlockReader.g"; }
private MessageTable msgTable;
private Map options = CollectionFactory.map();
public OptionsBlockReader( TreeNodeStream nodes, MessageTable msgTable ) {
this(nodes);
if (msgTable == null) {
throw new IllegalArgumentException( "msgTable should not be null" );
}
this.msgTable = msgTable;
}
public Map getOptions() {
return options;
}
public void addOption(String name, String value) {
try {
if (OptionLookup.isValidValue(name, value)) {
options.put(name, value);
} else {
msgTable.add(value, Message.INVALID_OPTION_VALUE);
}
} catch (UndefinedOptionException ex) {
msgTable.add(name, Message.INVALID_OPTION);
}
}
// $ANTLR start "topdown"
// org/jaitools/jiffle/parser/OptionsBlockReader.g:76:1: topdown : jiffleOption ;
public final void topdown() throws RecognitionException {
try {
// org/jaitools/jiffle/parser/OptionsBlockReader.g:76:17: ( jiffleOption )
// org/jaitools/jiffle/parser/OptionsBlockReader.g:76:19: jiffleOption
{
pushFollow(FOLLOW_jiffleOption_in_topdown82);
jiffleOption();
state._fsp--;
if (state.failed) return ;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "topdown"
// $ANTLR start "jiffleOption"
// org/jaitools/jiffle/parser/OptionsBlockReader.g:80:1: jiffleOption : ^( JIFFLE_OPTION ID optionValue ) ;
public final void jiffleOption() throws RecognitionException {
CommonTree ID1=null;
OptionsBlockReader.optionValue_return optionValue2 = null;
try {
// org/jaitools/jiffle/parser/OptionsBlockReader.g:80:17: ( ^( JIFFLE_OPTION ID optionValue ) )
// org/jaitools/jiffle/parser/OptionsBlockReader.g:80:19: ^( JIFFLE_OPTION ID optionValue )
{
match(input,JIFFLE_OPTION,FOLLOW_JIFFLE_OPTION_in_jiffleOption112); if (state.failed) return ;
match(input, Token.DOWN, null); if (state.failed) return ;
ID1=(CommonTree)match(input,ID,FOLLOW_ID_in_jiffleOption114); if (state.failed) return ;
pushFollow(FOLLOW_optionValue_in_jiffleOption116);
optionValue2=optionValue();
state._fsp--;
if (state.failed) return ;
match(input, Token.UP, null); if (state.failed) return ;
if ( state.backtracking==1 ) {
addOption((ID1!=null?ID1.getText():null), (optionValue2!=null?((CommonTree)optionValue2.start):null).getText());
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return ;
}
// $ANTLR end "jiffleOption"
public static class optionValue_return extends TreeRuleReturnScope {
public String str;
};
// $ANTLR start "optionValue"
// org/jaitools/jiffle/parser/OptionsBlockReader.g:85:1: optionValue returns [String str] : ( ID | literal );
public final OptionsBlockReader.optionValue_return optionValue() throws RecognitionException {
OptionsBlockReader.optionValue_return retval = new OptionsBlockReader.optionValue_return();
retval.start = input.LT(1);
CommonTree ID3=null;
OptionsBlockReader.literal_return literal4 = null;
try {
// org/jaitools/jiffle/parser/OptionsBlockReader.g:86:17: ( ID | literal )
int alt1=2;
switch ( input.LA(1) ) {
case ID:
{
alt1=1;
}
break;
case INT_LITERAL:
case FLOAT_LITERAL:
case TRUE:
case FALSE:
case NULL:
{
alt1=2;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return retval;}
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/OptionsBlockReader.g:86:19: ID
{
ID3=(CommonTree)match(input,ID,FOLLOW_ID_in_optionValue181); if (state.failed) return retval;
if ( state.backtracking==1 ) {
retval.str = (ID3!=null?ID3.getText():null);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/OptionsBlockReader.g:87:19: literal
{
pushFollow(FOLLOW_literal_in_optionValue203);
literal4=literal();
state._fsp--;
if (state.failed) return retval;
if ( state.backtracking==1 ) {
retval.str = (literal4!=null?((CommonTree)literal4.start):null).getText();
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "optionValue"
public static class literal_return extends TreeRuleReturnScope {
};
// $ANTLR start "literal"
// org/jaitools/jiffle/parser/OptionsBlockReader.g:91:1: literal : ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL );
public final OptionsBlockReader.literal_return literal() throws RecognitionException {
OptionsBlockReader.literal_return retval = new OptionsBlockReader.literal_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/OptionsBlockReader.g:91:17: ( INT_LITERAL | FLOAT_LITERAL | TRUE | FALSE | NULL )
// org/jaitools/jiffle/parser/OptionsBlockReader.g:
{
if ( (input.LA(1)>=INT_LITERAL && input.LA(1)<=NULL) ) {
input.consume();
state.errorRecovery=false;state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return retval;}
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "literal"
// Delegated rules
public static final BitSet FOLLOW_jiffleOption_in_topdown82 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_JIFFLE_OPTION_in_jiffleOption112 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_jiffleOption114 = new BitSet(new long[]{0x0000000400000000L,0x00000000007C0000L});
public static final BitSet FOLLOW_optionValue_in_jiffleOption116 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ID_in_optionValue181 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_optionValue203 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_set_in_literal0 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy