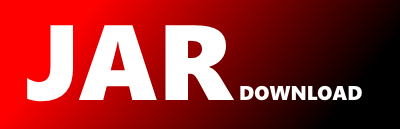
org.jaitools.jiffle.parser.Runtime.stg Maven / Gradle / Ivy
/*
* Copyright 2011 Michael Bedward
*
* This file is part of jai-tools.
*
* jai-tools is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* jai-tools is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with jai-tools. If not, see .
*
*/
group Runtime;
/*
* This file contains templates used for both direct and indirect runtime class
* source code generation.
*
* The templates are formatted for the ANTLR StringTemplate libary and are
* called from the source generator class built from the RuntimeSourceCreator.g
* grammar file.
*
* @author Michael Bedward
* @since 1.1
* @version $Id$
*/
////////////////////////////////////////////////////////////
// Class javadocs
javadocs(script) ::= <<
<{/**}>
* Java runtime class generated from the following Jiffle script:
*
}; separator="\n">
<{ */}>
>>
////////////////////////////////////////////////////////////
// Constructor
//
// The fields arg holds templates for image-scope variables
ctor(classname, fields) ::= <<
public () {
}
>>
ctorbody(fields) ::= <<
>>
registervars(name, fields) ::= <<
", truefalse);}; separator="\n">
>>
////////////////////////////////////////////////////////////
// Field declaration
//
// This template defines no output. It is used as a
// data structure by other templates.
field(name, type, mods, init) ::= << >>
////////////////////////////////////////////////////////////
// Script option
//
// This template defines no output. It is used as a
// data structure by other templates.
scriptoption(name, value) ::= << >>
////////////////////////////////////////////////////////////
// Helper templates for the runtime template
//
// Creates an int index field for each image-scope variable
fieldindices(vars) ::= <<
= ;}; separator="\n">
>>
// Formats initializing expressions for option-related fields.
initoptions(opts) ::= <<
protected void initOptionVars() {
;}; separator="\n">
}
>>
// Getter function for image-scope variable default values
defaultvaluegetter(fields) ::= <<
protected Double getDefaultValue(int index) {
switch (index) {
}, field=field)>}; separator="\n">
default:
return null;
}
}
>>
// Creates a case statement for a variable default value
defaultvaluecase(index, field) ::= <<
case : //
return null;
>>
////////////////////////////////////////////////////////////
// Read from source image
getsourcevalue(var, pixel, band) ::= <<
readFromImage("", _x, _y, (int)()0)
>>
pixel(x, y) ::= <<
,
>>
////////////////////////////////////////////////////////////
// Code block
block(stmts) ::= <<
{
}
>>
////////////////////////////////////////////////////////////
// Delimited statement
delimstmt(stmt) ::= ";"
////////////////////////////////////////////////////////////
// Loop: while
while(cond, stmt) ::= <<
while (true) {
if (_FN.sign() != 1) break;
}
>>
////////////////////////////////////////////////////////////
// Loop: until
until(cond, stmt) ::= <<
while (true) {
if (_FN.sign() == 1) break;
}
>>
////////////////////////////////////////////////////////////
// Loop: foreach with list literal
foreachlist(n, var, list, stmt) ::= <<
Double[] _loopset = {
};
int _index=0;
while (_index \< _loopset.length) {
double = _loopset[_index];
_index++ ;
}
>>
////////////////////////////////////////////////////////////
// Loop: foreach with list variable
foreachlistvar(n, var, listvar, stmt) ::= <<
Iterator _iter = .iterator();
while (_iter.hasNext()) {
double = ((Number) _iter.next()).doubleValue();
}
>>
////////////////////////////////////////////////////////////
// Loop: foreach with sequence
foreachseq(n, var, lo, hi, stmt) ::= <<
int _lo = (int)();
int _hi = (int)();
for (int = _lo; \<= _hi; ++)
>>
////////////////////////////////////////////////////////////
// Loop exit - breakif
breakif(cond) ::= <<
if (_FN.sign() == 1) break;
>>
////////////////////////////////////////////////////////////
// con calls
//
// These are treated separately from general functions to
// ensure lazy evaluation of the alternatives
// This template is called from the grammar
concall(args) ::= <<
<({con})(args)>
>>
////////////////////////////////////////////////////////////
// Helper templates called by concall
con1(cond) ::= <<
(_stk.push(_FN.sign()) == null ? Double.NaN :
_stk.peek() != 0 ? 1.0 : 0.0)
>>
con2(args) ::= <<
(_stk.push(_FN.sign()) == null ? Double.NaN :
_stk.peek() != 0 ? : 0.0)
>>
con3(args) ::= <<
(_stk.push(_FN.sign()) == null ? Double.NaN :
_stk.peek() != 0 ? : )
>>
con4(args) ::= <<
(_stk.push(_FN.sign()) == null ? Double.NaN :
_stk.peek() == 1 ? :
_stk.peek() == 0 ? : )
>>
////////////////////////////////////////////////////////////
// If statement
ifcall(cond, case) ::= <<
if (_stk.push(_FN.sign()) != null && _stk.peek() != 0)
>>
ifelsecall(cond, case1, case2) ::= <<
if (_stk.push(_FN.sign()) != null && _stk.peek() != 0)
else
>>
////////////////////////////////////////////////////////////
// General function call
call(name, args) ::= <<
()
>>
////////////////////////////////////////////////////////////
// Simple binary expressions
binaryexpr(lhs, op, rhs) ::= <<
>>
pow(x, y) ::= <<
Math.pow(, )
>>
// this template is a work-around for Janino 2.5.16 being unable
// to compile come expressions with "+=" etc compound ops
compoundassignment(lhs, op, rhs) ::= <<
=
>>
////////////////////////////////////////////////////////////
// Parenthesised expression
par(expr) ::= "()"
////////////////////////////////////////////////////////////
// Prefix operator
preop(op, expr) ::= <<
>>
////////////////////////////////////////////////////////////
// Postfix operator
postop(op, expr) ::= <<
>>
////////////////////////////////////////////////////////////
// List expressions
listappend(var, expr) ::= <<
.add()
>>
listassign(isnew, var, expr) ::= <<
List =
>>
listliteral(exprs) ::= <<
new ArrayList()
>>
// helper for listliteral
listinits(exprs) ::= <<
>>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy