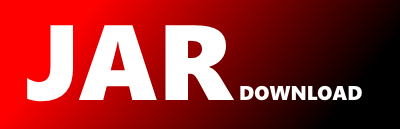
org.jaitools.jiffle.parser.RuntimeSourceGenerator Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 org/jaitools/jiffle/parser/RuntimeSourceGenerator.g 2012-10-10 10:44:31
package org.jaitools.jiffle.parser;
import org.jaitools.jiffle.Jiffle;
import org.jaitools.jiffle.runtime.AbstractJiffleRuntime;
import org.antlr.runtime.*;
import org.antlr.runtime.tree.*;import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import org.antlr.stringtemplate.*;
import org.antlr.stringtemplate.language.*;
import java.util.HashMap;
/**
* Generates Java sources for the runtime class from the final AST.
*
* @author Michael Bedward
*/
public class RuntimeSourceGenerator extends AbstractSourceGenerator {
public static final String[] tokenNames = new String[] {
"", "", "", "", "ABS_POS", "BAND_REF", "BLOCK", "CON_CALL", "DECL", "DECLARED_LIST", "EXPR_LIST", "FUNC_CALL", "IMAGE_POS", "JIFFLE_OPTION", "PAR", "PIXEL_REF", "POSTFIX", "PREFIX", "REL_POS", "SEQUENCE", "VAR_DEST", "VAR_IMAGE_SCOPE", "VAR_SOURCE", "CONSTANT", "IMAGE_WRITE", "LIST_NEW", "VAR_IMAGE", "VAR_PIXEL_SCOPE", "VAR_PROVIDED", "VAR_LOOP", "VAR_LIST", "OPTIONS", "LCURLY", "RCURLY", "ID", "EQ", "SEMI", "IMAGES", "READ", "WRITE", "INIT", "WHILE", "LPAR", "RPAR", "UNTIL", "FOREACH", "IN", "IF", "ELSE", "BREAKIF", "BREAK", "COMMA", "COLON", "CON", "APPEND", "TIMESEQ", "DIVEQ", "MODEQ", "PLUSEQ", "MINUSEQ", "QUESTION", "OR", "XOR", "AND", "LOGICALEQ", "NE", "GT", "GE", "LE", "LT", "PLUS", "MINUS", "TIMES", "DIV", "MOD", "NOT", "INCR", "DECR", "POW", "LSQUARE", "RSQUARE", "ABS_POS_PREFIX", "INT_LITERAL", "FLOAT_LITERAL", "TRUE", "FALSE", "NULL", "COMMENT", "INT_TYPE", "FLOAT_TYPE", "DOUBLE_TYPE", "BOOLEAN_TYPE", "Letter", "UNDERSCORE", "Digit", "Dot", "NonZeroDigit", "FloatExp", "WS", "ESC_SEQ", "CHAR", "HEX_DIGIT", "UNICODE_ESC", "OCTAL_ESC"
};
public static final int EOF=-1;
public static final int ABS_POS=4;
public static final int BAND_REF=5;
public static final int BLOCK=6;
public static final int CON_CALL=7;
public static final int DECL=8;
public static final int DECLARED_LIST=9;
public static final int EXPR_LIST=10;
public static final int FUNC_CALL=11;
public static final int IMAGE_POS=12;
public static final int JIFFLE_OPTION=13;
public static final int PAR=14;
public static final int PIXEL_REF=15;
public static final int POSTFIX=16;
public static final int PREFIX=17;
public static final int REL_POS=18;
public static final int SEQUENCE=19;
public static final int VAR_DEST=20;
public static final int VAR_IMAGE_SCOPE=21;
public static final int VAR_SOURCE=22;
public static final int CONSTANT=23;
public static final int IMAGE_WRITE=24;
public static final int LIST_NEW=25;
public static final int VAR_IMAGE=26;
public static final int VAR_PIXEL_SCOPE=27;
public static final int VAR_PROVIDED=28;
public static final int VAR_LOOP=29;
public static final int VAR_LIST=30;
public static final int OPTIONS=31;
public static final int LCURLY=32;
public static final int RCURLY=33;
public static final int ID=34;
public static final int EQ=35;
public static final int SEMI=36;
public static final int IMAGES=37;
public static final int READ=38;
public static final int WRITE=39;
public static final int INIT=40;
public static final int WHILE=41;
public static final int LPAR=42;
public static final int RPAR=43;
public static final int UNTIL=44;
public static final int FOREACH=45;
public static final int IN=46;
public static final int IF=47;
public static final int ELSE=48;
public static final int BREAKIF=49;
public static final int BREAK=50;
public static final int COMMA=51;
public static final int COLON=52;
public static final int CON=53;
public static final int APPEND=54;
public static final int TIMESEQ=55;
public static final int DIVEQ=56;
public static final int MODEQ=57;
public static final int PLUSEQ=58;
public static final int MINUSEQ=59;
public static final int QUESTION=60;
public static final int OR=61;
public static final int XOR=62;
public static final int AND=63;
public static final int LOGICALEQ=64;
public static final int NE=65;
public static final int GT=66;
public static final int GE=67;
public static final int LE=68;
public static final int LT=69;
public static final int PLUS=70;
public static final int MINUS=71;
public static final int TIMES=72;
public static final int DIV=73;
public static final int MOD=74;
public static final int NOT=75;
public static final int INCR=76;
public static final int DECR=77;
public static final int POW=78;
public static final int LSQUARE=79;
public static final int RSQUARE=80;
public static final int ABS_POS_PREFIX=81;
public static final int INT_LITERAL=82;
public static final int FLOAT_LITERAL=83;
public static final int TRUE=84;
public static final int FALSE=85;
public static final int NULL=86;
public static final int COMMENT=87;
public static final int INT_TYPE=88;
public static final int FLOAT_TYPE=89;
public static final int DOUBLE_TYPE=90;
public static final int BOOLEAN_TYPE=91;
public static final int Letter=92;
public static final int UNDERSCORE=93;
public static final int Digit=94;
public static final int Dot=95;
public static final int NonZeroDigit=96;
public static final int FloatExp=97;
public static final int WS=98;
public static final int ESC_SEQ=99;
public static final int CHAR=100;
public static final int HEX_DIGIT=101;
public static final int UNICODE_ESC=102;
public static final int OCTAL_ESC=103;
// delegates
// delegators
public RuntimeSourceGenerator(TreeNodeStream input) {
this(input, new RecognizerSharedState());
}
public RuntimeSourceGenerator(TreeNodeStream input, RecognizerSharedState state) {
super(input, state);
}
protected StringTemplateGroup templateLib =
new StringTemplateGroup("RuntimeSourceGeneratorTemplates", AngleBracketTemplateLexer.class);
public void setTemplateLib(StringTemplateGroup templateLib) {
this.templateLib = templateLib;
}
public StringTemplateGroup getTemplateLib() {
return templateLib;
}
/** allows convenient multi-value initialization:
* "new STAttrMap().put(...).put(...)"
*/
public static class STAttrMap extends HashMap {
public STAttrMap put(String attrName, Object value) {
super.put(attrName, value);
return this;
}
public STAttrMap put(String attrName, int value) {
super.put(attrName, new Integer(value));
return this;
}
}
public String[] getTokenNames() { return RuntimeSourceGenerator.tokenNames; }
public String getGrammarFileName() { return "org/jaitools/jiffle/parser/RuntimeSourceGenerator.g"; }
private SymbolScopeStack varScope = new SymbolScopeStack();
private String getConstantString(String name) {
String s = String.valueOf(ConstantLookup.getValue(name));
if ("NaN".equals(s)) {
return "Double.NaN";
}
return s;
}
private String getImageScopeVarExpr(String varName) {
return AbstractJiffleRuntime.VAR_STRING.replace("_VAR_", varName);
}
public static class generate_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "generate"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:63:1: generate[String script] : (o+= jiffleOption )* (v+= varDeclaration )* (s+= statement )+ -> runtime(script=scriptLinespkgname=pkgNameimports=importsname=classNamebase=baseClassNameopts=$ofields=$veval=$s);
public final RuntimeSourceGenerator.generate_return generate(String script) throws RecognitionException {
RuntimeSourceGenerator.generate_return retval = new RuntimeSourceGenerator.generate_return();
retval.start = input.LT(1);
List list_o=null;
List list_v=null;
List list_s=null;
RuleReturnScope o = null;
RuleReturnScope v = null;
RuleReturnScope s = null;
varScope.addLevel("top");
List scriptLines = null;
if (script != null && script.length() > 0) {
scriptLines = prepareScriptForComments(script);
}
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:17: ( (o+= jiffleOption )* (v+= varDeclaration )* (s+= statement )+ -> runtime(script=scriptLinespkgname=pkgNameimports=importsname=classNamebase=baseClassNameopts=$ofields=$veval=$s))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:19: (o+= jiffleOption )* (v+= varDeclaration )* (s+= statement )+
{
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:20: (o+= jiffleOption )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case JIFFLE_OPTION:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:20: o+= jiffleOption
{
pushFollow(FOLLOW_jiffleOption_in_generate107);
o=jiffleOption();
state._fsp--;
if (list_o==null) list_o=new ArrayList();
list_o.add(o.getTemplate());
}
break;
default :
break loop1;
}
} while (true);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:37: (v+= varDeclaration )*
loop2:
do {
int alt2=2;
switch ( input.LA(1) ) {
case DECL:
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:37: v+= varDeclaration
{
pushFollow(FOLLOW_varDeclaration_in_generate112);
v=varDeclaration();
state._fsp--;
if (list_v==null) list_v=new ArrayList();
list_v.add(v.getTemplate());
}
break;
default :
break loop2;
}
} while (true);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:56: (s+= statement )+
int cnt3=0;
loop3:
do {
int alt3=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt3=1;
}
break;
}
switch (alt3) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:71:56: s+= statement
{
pushFollow(FOLLOW_statement_in_generate117);
s=statement();
state._fsp--;
if (list_s==null) list_s=new ArrayList();
list_s.add(s.getTemplate());
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee =
new EarlyExitException(3, input);
throw eee;
}
cnt3++;
} while (true);
// TEMPLATE REWRITE
// 73:17: -> runtime(script=scriptLinespkgname=pkgNameimports=importsname=classNamebase=baseClassNameopts=$ofields=$veval=$s)
{
retval.st = templateLib.getInstanceOf("runtime",
new STAttrMap().put("script", scriptLines).put("pkgname", pkgName).put("imports", imports).put("name", className).put("base", baseClassName).put("opts", list_o).put("fields", list_v).put("eval", list_s));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "generate"
public static class jiffleOption_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "jiffleOption"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:79:1: jiffleOption : ^( JIFFLE_OPTION ID optionValue ) -> {%{getOptionExpr($ID.text, $optionValue.src)}};
public final RuntimeSourceGenerator.jiffleOption_return jiffleOption() throws RecognitionException {
RuntimeSourceGenerator.jiffleOption_return retval = new RuntimeSourceGenerator.jiffleOption_return();
retval.start = input.LT(1);
CommonTree ID1=null;
RuntimeSourceGenerator.optionValue_return optionValue2 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:79:17: ( ^( JIFFLE_OPTION ID optionValue ) -> {%{getOptionExpr($ID.text, $optionValue.src)}})
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:79:19: ^( JIFFLE_OPTION ID optionValue )
{
match(input,JIFFLE_OPTION,FOLLOW_JIFFLE_OPTION_in_jiffleOption264);
match(input, Token.DOWN, null);
ID1=(CommonTree)match(input,ID,FOLLOW_ID_in_jiffleOption266);
pushFollow(FOLLOW_optionValue_in_jiffleOption268);
optionValue2=optionValue();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 80:17: -> {%{getOptionExpr($ID.text, $optionValue.src)}}
{
retval.st = new StringTemplate(templateLib,getOptionExpr((ID1!=null?ID1.getText():null), (optionValue2!=null?optionValue2.src:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "jiffleOption"
public static class optionValue_return extends TreeRuleReturnScope {
public String src;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "optionValue"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:84:1: optionValue returns [String src] : ( ID | literal | CONSTANT );
public final RuntimeSourceGenerator.optionValue_return optionValue() throws RecognitionException {
RuntimeSourceGenerator.optionValue_return retval = new RuntimeSourceGenerator.optionValue_return();
retval.start = input.LT(1);
CommonTree ID3=null;
CommonTree CONSTANT5=null;
RuntimeSourceGenerator.literal_return literal4 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:85:17: ( ID | literal | CONSTANT )
int alt4=3;
switch ( input.LA(1) ) {
case ID:
{
alt4=1;
}
break;
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt4=2;
}
break;
case CONSTANT:
{
alt4=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:85:19: ID
{
ID3=(CommonTree)match(input,ID,FOLLOW_ID_in_optionValue335);
retval.src = (ID3!=null?ID3.getText():null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:86:19: literal
{
pushFollow(FOLLOW_literal_in_optionValue357);
literal4=literal();
state._fsp--;
retval.src = (literal4!=null?((CommonTree)literal4.start):null).getText();
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:87:19: CONSTANT
{
CONSTANT5=(CommonTree)match(input,CONSTANT,FOLLOW_CONSTANT_in_optionValue379);
retval.src = getConstantString((CONSTANT5!=null?CONSTANT5.getText():null));
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "optionValue"
public static class varDeclaration_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "varDeclaration"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:91:1: varDeclaration : ( ^( DECL VAR_DEST ID ) | ^( DECL VAR_SOURCE ID ) | ^( DECL VAR_IMAGE_SCOPE (e= expression )? ) -> field(name=$VAR_IMAGE_SCOPE.texttype=%{\"double\"}mods=%{\"private\"}init=$e.st));
public final RuntimeSourceGenerator.varDeclaration_return varDeclaration() throws RecognitionException {
RuntimeSourceGenerator.varDeclaration_return retval = new RuntimeSourceGenerator.varDeclaration_return();
retval.start = input.LT(1);
CommonTree VAR_IMAGE_SCOPE6=null;
RuntimeSourceGenerator.expression_return e = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:91:17: ( ^( DECL VAR_DEST ID ) | ^( DECL VAR_SOURCE ID ) | ^( DECL VAR_IMAGE_SCOPE (e= expression )? ) -> field(name=$VAR_IMAGE_SCOPE.texttype=%{\"double\"}mods=%{\"private\"}init=$e.st))
int alt6=3;
switch ( input.LA(1) ) {
case DECL:
{
switch ( input.LA(2) ) {
case DOWN:
{
switch ( input.LA(3) ) {
case VAR_DEST:
{
alt6=1;
}
break;
case VAR_SOURCE:
{
alt6=2;
}
break;
case VAR_IMAGE_SCOPE:
{
alt6=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 2, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:91:19: ^( DECL VAR_DEST ID )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration409);
match(input, Token.DOWN, null);
match(input,VAR_DEST,FOLLOW_VAR_DEST_in_varDeclaration411);
match(input,ID,FOLLOW_ID_in_varDeclaration413);
match(input, Token.UP, null);
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:92:19: ^( DECL VAR_SOURCE ID )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration435);
match(input, Token.DOWN, null);
match(input,VAR_SOURCE,FOLLOW_VAR_SOURCE_in_varDeclaration437);
match(input,ID,FOLLOW_ID_in_varDeclaration439);
match(input, Token.UP, null);
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:94:18: ^( DECL VAR_IMAGE_SCOPE (e= expression )? )
{
match(input,DECL,FOLLOW_DECL_in_varDeclaration461);
match(input, Token.DOWN, null);
VAR_IMAGE_SCOPE6=(CommonTree)match(input,VAR_IMAGE_SCOPE,FOLLOW_VAR_IMAGE_SCOPE_in_varDeclaration463);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:94:42: (e= expression )?
int alt5=2;
switch ( input.LA(1) ) {
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case APPEND:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt5=1;
}
break;
}
switch (alt5) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:94:42: e= expression
{
pushFollow(FOLLOW_expression_in_varDeclaration467);
e=expression();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
varScope.addSymbol((VAR_IMAGE_SCOPE6!=null?VAR_IMAGE_SCOPE6.getText():null), SymbolType.SCALAR, ScopeType.IMAGE);
StringTemplate exprST = (e == null ? null : (e!=null?e.st:null));
// TEMPLATE REWRITE
// 99:17: -> field(name=$VAR_IMAGE_SCOPE.texttype=%{\"double\"}mods=%{\"private\"}init=$e.st)
{
retval.st = templateLib.getInstanceOf("field",
new STAttrMap().put("name", (VAR_IMAGE_SCOPE6!=null?VAR_IMAGE_SCOPE6.getText():null)).put("type", new StringTemplate(templateLib,"double")).put("mods", new StringTemplate(templateLib,"private")).put("init", (e!=null?e.st:null)));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "varDeclaration"
public static class block_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "block"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:103:1: block : ^( BLOCK (s+= statement )* ) -> block(stmts=$s);
public final RuntimeSourceGenerator.block_return block() throws RecognitionException {
RuntimeSourceGenerator.block_return retval = new RuntimeSourceGenerator.block_return();
retval.start = input.LT(1);
List list_s=null;
RuleReturnScope s = null;
varScope.addLevel("block");
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:110:17: ( ^( BLOCK (s+= statement )* ) -> block(stmts=$s))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:110:19: ^( BLOCK (s+= statement )* )
{
match(input,BLOCK,FOLLOW_BLOCK_in_block580);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:110:28: (s+= statement )*
loop7:
do {
int alt7=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt7=1;
}
break;
}
switch (alt7) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:110:28: s+= statement
{
pushFollow(FOLLOW_statement_in_block584);
s=statement();
state._fsp--;
if (list_s==null) list_s=new ArrayList();
list_s.add(s.getTemplate());
}
break;
default :
break loop7;
}
} while (true);
match(input, Token.UP, null);
}
// TEMPLATE REWRITE
// 111:17: -> block(stmts=$s)
{
retval.st = templateLib.getInstanceOf("block",
new STAttrMap().put("stmts", list_s));
}
}
varScope.dropLevel();
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "block"
public static class statement_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "statement"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:115:1: statement : ( simpleStatement -> delimstmt(stmt=$simpleStatement.st) | block -> {$block.st});
public final RuntimeSourceGenerator.statement_return statement() throws RecognitionException {
RuntimeSourceGenerator.statement_return retval = new RuntimeSourceGenerator.statement_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.simpleStatement_return simpleStatement7 = null;
RuntimeSourceGenerator.block_return block8 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:115:17: ( simpleStatement -> delimstmt(stmt=$simpleStatement.st) | block -> {$block.st})
int alt8=2;
switch ( input.LA(1) ) {
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt8=1;
}
break;
case BLOCK:
{
alt8=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:115:19: simpleStatement
{
pushFollow(FOLLOW_simpleStatement_in_statement643);
simpleStatement7=simpleStatement();
state._fsp--;
// TEMPLATE REWRITE
// 115:35: -> delimstmt(stmt=$simpleStatement.st)
{
retval.st = templateLib.getInstanceOf("delimstmt",
new STAttrMap().put("stmt", (simpleStatement7!=null?simpleStatement7.st:null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:116:19: block
{
pushFollow(FOLLOW_block_in_statement672);
block8=block();
state._fsp--;
// TEMPLATE REWRITE
// 116:25: -> {$block.st}
{
retval.st = (block8!=null?block8.st:null);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "statement"
public static class simpleStatement_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "simpleStatement"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:120:1: simpleStatement : ( imageWrite -> {$imageWrite.st} | scalarAssignment -> {$scalarAssignment.st} | listAssignment -> {$listAssignment.st} | loop -> {$loop.st} | ^( BREAKIF expression ) -> breakif(cond=$expression.st) | BREAK -> {%{\"break\"}} | ifCall -> {$ifCall.st} | expression -> {$expression.st});
public final RuntimeSourceGenerator.simpleStatement_return simpleStatement() throws RecognitionException {
RuntimeSourceGenerator.simpleStatement_return retval = new RuntimeSourceGenerator.simpleStatement_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.imageWrite_return imageWrite9 = null;
RuntimeSourceGenerator.scalarAssignment_return scalarAssignment10 = null;
RuntimeSourceGenerator.listAssignment_return listAssignment11 = null;
RuntimeSourceGenerator.loop_return loop12 = null;
RuntimeSourceGenerator.expression_return expression13 = null;
RuntimeSourceGenerator.ifCall_return ifCall14 = null;
RuntimeSourceGenerator.expression_return expression15 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:120:17: ( imageWrite -> {$imageWrite.st} | scalarAssignment -> {$scalarAssignment.st} | listAssignment -> {$listAssignment.st} | loop -> {$loop.st} | ^( BREAKIF expression ) -> breakif(cond=$expression.st) | BREAK -> {%{\"break\"}} | ifCall -> {$ifCall.st} | expression -> {$expression.st})
int alt9=8;
alt9 = dfa9.predict(input);
switch (alt9) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:120:19: imageWrite
{
pushFollow(FOLLOW_imageWrite_in_simpleStatement702);
imageWrite9=imageWrite();
state._fsp--;
// TEMPLATE REWRITE
// 120:30: -> {$imageWrite.st}
{
retval.st = (imageWrite9!=null?imageWrite9.st:null);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:121:19: scalarAssignment
{
pushFollow(FOLLOW_scalarAssignment_in_simpleStatement726);
scalarAssignment10=scalarAssignment();
state._fsp--;
// TEMPLATE REWRITE
// 121:36: -> {$scalarAssignment.st}
{
retval.st = (scalarAssignment10!=null?scalarAssignment10.st:null);
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:122:19: listAssignment
{
pushFollow(FOLLOW_listAssignment_in_simpleStatement750);
listAssignment11=listAssignment();
state._fsp--;
// TEMPLATE REWRITE
// 122:34: -> {$listAssignment.st}
{
retval.st = (listAssignment11!=null?listAssignment11.st:null);
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:123:19: loop
{
pushFollow(FOLLOW_loop_in_simpleStatement774);
loop12=loop();
state._fsp--;
// TEMPLATE REWRITE
// 123:24: -> {$loop.st}
{
retval.st = (loop12!=null?loop12.st:null);
}
}
break;
case 5 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:124:19: ^( BREAKIF expression )
{
match(input,BREAKIF,FOLLOW_BREAKIF_in_simpleStatement799);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_simpleStatement801);
expression13=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 124:41: -> breakif(cond=$expression.st)
{
retval.st = templateLib.getInstanceOf("breakif",
new STAttrMap().put("cond", (expression13!=null?expression13.st:null)));
}
}
break;
case 6 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:125:19: BREAK
{
match(input,BREAK,FOLLOW_BREAK_in_simpleStatement831);
// TEMPLATE REWRITE
// 125:25: -> {%{\"break\"}}
{
retval.st = new StringTemplate(templateLib,"break");
}
}
break;
case 7 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:126:19: ifCall
{
pushFollow(FOLLOW_ifCall_in_simpleStatement855);
ifCall14=ifCall();
state._fsp--;
// TEMPLATE REWRITE
// 126:26: -> {$ifCall.st}
{
retval.st = (ifCall14!=null?ifCall14.st:null);
}
}
break;
case 8 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:127:19: expression
{
pushFollow(FOLLOW_expression_in_simpleStatement879);
expression15=expression();
state._fsp--;
// TEMPLATE REWRITE
// 127:30: -> {$expression.st}
{
retval.st = (expression15!=null?expression15.st:null);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "simpleStatement"
public static class imageWrite_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "imageWrite"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:131:1: imageWrite : ^( IMAGE_WRITE VAR_DEST expression ) -> setdestvalue(var=$VAR_DEST.textexpr=$expression.st);
public final RuntimeSourceGenerator.imageWrite_return imageWrite() throws RecognitionException {
RuntimeSourceGenerator.imageWrite_return retval = new RuntimeSourceGenerator.imageWrite_return();
retval.start = input.LT(1);
CommonTree VAR_DEST16=null;
RuntimeSourceGenerator.expression_return expression17 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:131:17: ( ^( IMAGE_WRITE VAR_DEST expression ) -> setdestvalue(var=$VAR_DEST.textexpr=$expression.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:131:19: ^( IMAGE_WRITE VAR_DEST expression )
{
match(input,IMAGE_WRITE,FOLLOW_IMAGE_WRITE_in_imageWrite915);
match(input, Token.DOWN, null);
VAR_DEST16=(CommonTree)match(input,VAR_DEST,FOLLOW_VAR_DEST_in_imageWrite917);
pushFollow(FOLLOW_expression_in_imageWrite919);
expression17=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 132:17: -> setdestvalue(var=$VAR_DEST.textexpr=$expression.st)
{
retval.st = templateLib.getInstanceOf("setdestvalue",
new STAttrMap().put("var", (VAR_DEST16!=null?VAR_DEST16.getText():null)).put("expr", (expression17!=null?expression17.st:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "imageWrite"
public static class expressionList_return extends TreeRuleReturnScope {
public List argTypes;
public List templates;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "expressionList"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:136:1: expressionList returns [List argTypes, List templates] : ^( EXPR_LIST ( expression )* ) ;
public final RuntimeSourceGenerator.expressionList_return expressionList() throws RecognitionException {
RuntimeSourceGenerator.expressionList_return retval = new RuntimeSourceGenerator.expressionList_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.expression_return expression18 = null;
retval.argTypes = new ArrayList();
retval.templates = new ArrayList();
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:141:17: ( ^( EXPR_LIST ( expression )* ) )
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:141:19: ^( EXPR_LIST ( expression )* )
{
match(input,EXPR_LIST,FOLLOW_EXPR_LIST_in_expressionList1002);
if ( input.LA(1)==Token.DOWN ) {
match(input, Token.DOWN, null);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:141:31: ( expression )*
loop10:
do {
int alt10=2;
switch ( input.LA(1) ) {
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case APPEND:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt10=1;
}
break;
}
switch (alt10) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:141:32: expression
{
pushFollow(FOLLOW_expression_in_expressionList1005);
expression18=expression();
state._fsp--;
int ttype = (expression18!=null?((CommonTree)expression18.start):null).getType();
retval.argTypes.add(ttype == VAR_LIST || ttype == DECLARED_LIST ? "List" : "D");
retval.templates.add((expression18!=null?expression18.st:null));
}
break;
default :
break loop10;
}
} while (true);
match(input, Token.UP, null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "expressionList"
public static class scalarAssignment_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "scalarAssignment"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:150:1: scalarAssignment : ( ^( EQ scalar expression ) -> binaryexpr(lhs=$scalar.stop=$EQ.textrhs=$expression.st) | ^( compoundAssignmentOp scalar expression ) -> compoundassignment(lhs=$scalar.stop=opChar1rhs=$expression.st));
public final RuntimeSourceGenerator.scalarAssignment_return scalarAssignment() throws RecognitionException {
RuntimeSourceGenerator.scalarAssignment_return retval = new RuntimeSourceGenerator.scalarAssignment_return();
retval.start = input.LT(1);
CommonTree EQ20=null;
RuntimeSourceGenerator.scalar_return scalar19 = null;
RuntimeSourceGenerator.expression_return expression21 = null;
RuntimeSourceGenerator.compoundAssignmentOp_return compoundAssignmentOp22 = null;
RuntimeSourceGenerator.scalar_return scalar23 = null;
RuntimeSourceGenerator.expression_return expression24 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:151:17: ( ^( EQ scalar expression ) -> binaryexpr(lhs=$scalar.stop=$EQ.textrhs=$expression.st) | ^( compoundAssignmentOp scalar expression ) -> compoundassignment(lhs=$scalar.stop=opChar1rhs=$expression.st))
int alt11=2;
switch ( input.LA(1) ) {
case EQ:
{
alt11=1;
}
break;
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
{
alt11=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:151:19: ^( EQ scalar expression )
{
EQ20=(CommonTree)match(input,EQ,FOLLOW_EQ_in_scalarAssignment1075);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_scalar_in_scalarAssignment1077);
scalar19=scalar();
state._fsp--;
pushFollow(FOLLOW_expression_in_scalarAssignment1079);
expression21=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 152:17: -> binaryexpr(lhs=$scalar.stop=$EQ.textrhs=$expression.st)
{
retval.st = templateLib.getInstanceOf("binaryexpr",
new STAttrMap().put("lhs", (scalar19!=null?scalar19.st:null)).put("op", (EQ20!=null?EQ20.getText():null)).put("rhs", (expression21!=null?expression21.st:null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:154:19: ^( compoundAssignmentOp scalar expression )
{
pushFollow(FOLLOW_compoundAssignmentOp_in_scalarAssignment1138);
compoundAssignmentOp22=compoundAssignmentOp();
state._fsp--;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_scalar_in_scalarAssignment1140);
scalar23=scalar();
state._fsp--;
pushFollow(FOLLOW_expression_in_scalarAssignment1142);
expression24=expression();
state._fsp--;
match(input, Token.UP, null);
String opChar1 = String.valueOf((compoundAssignmentOp22!=null?((CommonTree)compoundAssignmentOp22.start):null).getText().charAt(0));
// TEMPLATE REWRITE
// 158:17: -> compoundassignment(lhs=$scalar.stop=opChar1rhs=$expression.st)
{
retval.st = templateLib.getInstanceOf("compoundassignment",
new STAttrMap().put("lhs", (scalar23!=null?scalar23.st:null)).put("op", opChar1).put("rhs", (expression24!=null?expression24.st:null)));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "scalarAssignment"
public static class compoundAssignmentOp_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "compoundAssignmentOp"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:161:1: compoundAssignmentOp : ( TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ );
public final RuntimeSourceGenerator.compoundAssignmentOp_return compoundAssignmentOp() throws RecognitionException {
RuntimeSourceGenerator.compoundAssignmentOp_return retval = new RuntimeSourceGenerator.compoundAssignmentOp_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:162:17: ( TIMESEQ | DIVEQ | MODEQ | PLUSEQ | MINUSEQ )
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:
{
if ( (input.LA(1)>=TIMESEQ && input.LA(1)<=MINUSEQ) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "compoundAssignmentOp"
protected static class listAssignment_scope {
boolean isNew;
}
protected Stack listAssignment_stack = new Stack();
public static class listAssignment_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "listAssignment"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:170:1: listAssignment : ^( EQ VAR_LIST e= expression ) -> listassign(isnew=$listAssignment::isNewvar=$VAR_LIST.textexpr=$expression.st);
public final RuntimeSourceGenerator.listAssignment_return listAssignment() throws RecognitionException {
listAssignment_stack.push(new listAssignment_scope());
RuntimeSourceGenerator.listAssignment_return retval = new RuntimeSourceGenerator.listAssignment_return();
retval.start = input.LT(1);
CommonTree VAR_LIST25=null;
RuntimeSourceGenerator.expression_return e = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:172:17: ( ^( EQ VAR_LIST e= expression ) -> listassign(isnew=$listAssignment::isNewvar=$VAR_LIST.textexpr=$expression.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:172:19: ^( EQ VAR_LIST e= expression )
{
match(input,EQ,FOLLOW_EQ_in_listAssignment1364);
match(input, Token.DOWN, null);
VAR_LIST25=(CommonTree)match(input,VAR_LIST,FOLLOW_VAR_LIST_in_listAssignment1366);
pushFollow(FOLLOW_expression_in_listAssignment1370);
e=expression();
state._fsp--;
match(input, Token.UP, null);
((listAssignment_scope)listAssignment_stack.peek()).isNew = !varScope.isDefined((VAR_LIST25!=null?VAR_LIST25.getText():null), SymbolType.LIST);
if (((listAssignment_scope)listAssignment_stack.peek()).isNew) {
addImport("java.util.List", "java.util.ArrayList");
varScope.addSymbol((VAR_LIST25!=null?VAR_LIST25.getText():null), SymbolType.LIST, ScopeType.PIXEL);
}
// TEMPLATE REWRITE
// 181:17: -> listassign(isnew=$listAssignment::isNewvar=$VAR_LIST.textexpr=$expression.st)
{
retval.st = templateLib.getInstanceOf("listassign",
new STAttrMap().put("isnew", ((listAssignment_scope)listAssignment_stack.peek()).isNew).put("var", (VAR_LIST25!=null?VAR_LIST25.getText():null)).put("expr", (e!=null?e.st:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
listAssignment_stack.pop();
}
return retval;
}
// $ANTLR end "listAssignment"
public static class scalar_return extends TreeRuleReturnScope {
public boolean newVar;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "scalar"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:186:1: scalar returns [boolean newVar] : ( VAR_IMAGE_SCOPE | VAR_PIXEL_SCOPE );
public final RuntimeSourceGenerator.scalar_return scalar() throws RecognitionException {
RuntimeSourceGenerator.scalar_return retval = new RuntimeSourceGenerator.scalar_return();
retval.start = input.LT(1);
CommonTree VAR_PIXEL_SCOPE26=null;
retval.newVar = false;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:202:17: ( VAR_IMAGE_SCOPE | VAR_PIXEL_SCOPE )
int alt12=2;
switch ( input.LA(1) ) {
case VAR_IMAGE_SCOPE:
{
alt12=1;
}
break;
case VAR_PIXEL_SCOPE:
{
alt12=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 12, 0, input);
throw nvae;
}
switch (alt12) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:202:19: VAR_IMAGE_SCOPE
{
match(input,VAR_IMAGE_SCOPE,FOLLOW_VAR_IMAGE_SCOPE_in_scalar1482);
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:203:19: VAR_PIXEL_SCOPE
{
VAR_PIXEL_SCOPE26=(CommonTree)match(input,VAR_PIXEL_SCOPE,FOLLOW_VAR_PIXEL_SCOPE_in_scalar1503);
if (!varScope.isDefined((VAR_PIXEL_SCOPE26!=null?VAR_PIXEL_SCOPE26.getText():null))) {
varScope.addSymbol((VAR_PIXEL_SCOPE26!=null?VAR_PIXEL_SCOPE26.getText():null), SymbolType.SCALAR, ScopeType.PIXEL);
retval.newVar = true;
}
}
break;
}
String varName = ((CommonTree)retval.start).getText();
if (retval.newVar) {
retval.st = new StringTemplate(templateLib,"double " + varName);
} else if (((CommonTree)retval.start).getType() == VAR_IMAGE_SCOPE) {
retval.st = new StringTemplate(templateLib,getImageScopeVarExpr(varName));
} else {
retval.st = new StringTemplate(templateLib,varName);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "scalar"
public static class loop_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "loop"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:213:1: loop : ( conditionalLoop -> {$conditionalLoop.st} | foreachLoop -> {$foreachLoop.st});
public final RuntimeSourceGenerator.loop_return loop() throws RecognitionException {
RuntimeSourceGenerator.loop_return retval = new RuntimeSourceGenerator.loop_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.conditionalLoop_return conditionalLoop27 = null;
RuntimeSourceGenerator.foreachLoop_return foreachLoop28 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:213:17: ( conditionalLoop -> {$conditionalLoop.st} | foreachLoop -> {$foreachLoop.st})
int alt13=2;
switch ( input.LA(1) ) {
case WHILE:
case UNTIL:
{
alt13=1;
}
break;
case FOREACH:
{
alt13=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 13, 0, input);
throw nvae;
}
switch (alt13) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:213:19: conditionalLoop
{
pushFollow(FOLLOW_conditionalLoop_in_loop1559);
conditionalLoop27=conditionalLoop();
state._fsp--;
// TEMPLATE REWRITE
// 213:35: -> {$conditionalLoop.st}
{
retval.st = (conditionalLoop27!=null?conditionalLoop27.st:null);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:214:19: foreachLoop
{
pushFollow(FOLLOW_foreachLoop_in_loop1583);
foreachLoop28=foreachLoop();
state._fsp--;
// TEMPLATE REWRITE
// 214:31: -> {$foreachLoop.st}
{
retval.st = (foreachLoop28!=null?foreachLoop28.st:null);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "loop"
public static class conditionalLoop_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "conditionalLoop"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:218:1: conditionalLoop : ( ^( WHILE e= expression s= statement ) -> while(cond=$e.ststmt=$s.st) | ^( UNTIL e= expression s= statement ) -> until(cond=$e.ststmt=$s.st));
public final RuntimeSourceGenerator.conditionalLoop_return conditionalLoop() throws RecognitionException {
RuntimeSourceGenerator.conditionalLoop_return retval = new RuntimeSourceGenerator.conditionalLoop_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.expression_return e = null;
RuntimeSourceGenerator.statement_return s = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:219:17: ( ^( WHILE e= expression s= statement ) -> while(cond=$e.ststmt=$s.st) | ^( UNTIL e= expression s= statement ) -> until(cond=$e.ststmt=$s.st))
int alt14=2;
switch ( input.LA(1) ) {
case WHILE:
{
alt14=1;
}
break;
case UNTIL:
{
alt14=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:219:19: ^( WHILE e= expression s= statement )
{
match(input,WHILE,FOLLOW_WHILE_in_conditionalLoop1630);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_conditionalLoop1634);
e=expression();
state._fsp--;
pushFollow(FOLLOW_statement_in_conditionalLoop1638);
s=statement();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 219:53: -> while(cond=$e.ststmt=$s.st)
{
retval.st = templateLib.getInstanceOf("while",
new STAttrMap().put("cond", (e!=null?e.st:null)).put("stmt", (s!=null?s.st:null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:220:19: ^( UNTIL e= expression s= statement )
{
match(input,UNTIL,FOLLOW_UNTIL_in_conditionalLoop1674);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_conditionalLoop1678);
e=expression();
state._fsp--;
pushFollow(FOLLOW_statement_in_conditionalLoop1682);
s=statement();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 220:53: -> until(cond=$e.ststmt=$s.st)
{
retval.st = templateLib.getInstanceOf("until",
new STAttrMap().put("cond", (e!=null?e.st:null)).put("stmt", (s!=null?s.st:null)));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "conditionalLoop"
public static class foreachLoop_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "foreachLoop"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:223:1: foreachLoop : ( ^( FOREACH ID ^( DECLARED_LIST e= expressionList ) s= statement ) -> foreachlist(n=++varIndexvar=$ID.textlist=$e.templatesstmt=$s.st) | ^( FOREACH ID VAR_LIST s= statement ) -> foreachlistvar(n=++varIndexvar=$ID.textlistvar=%{$VAR_LIST.text}stmt=$s.st) | ^( FOREACH ID ^( SEQUENCE lo= expression hi= expression ) s= statement ) -> foreachseq(n=++varIndexvar=$ID.textlo=$lo.sthi=$hi.ststmt=$s.st));
public final RuntimeSourceGenerator.foreachLoop_return foreachLoop() throws RecognitionException {
RuntimeSourceGenerator.foreachLoop_return retval = new RuntimeSourceGenerator.foreachLoop_return();
retval.start = input.LT(1);
CommonTree ID29=null;
CommonTree ID30=null;
CommonTree VAR_LIST31=null;
CommonTree ID32=null;
RuntimeSourceGenerator.expressionList_return e = null;
RuntimeSourceGenerator.statement_return s = null;
RuntimeSourceGenerator.expression_return lo = null;
RuntimeSourceGenerator.expression_return hi = null;
varScope.addLevel("foreach");
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:230:17: ( ^( FOREACH ID ^( DECLARED_LIST e= expressionList ) s= statement ) -> foreachlist(n=++varIndexvar=$ID.textlist=$e.templatesstmt=$s.st) | ^( FOREACH ID VAR_LIST s= statement ) -> foreachlistvar(n=++varIndexvar=$ID.textlistvar=%{$VAR_LIST.text}stmt=$s.st) | ^( FOREACH ID ^( SEQUENCE lo= expression hi= expression ) s= statement ) -> foreachseq(n=++varIndexvar=$ID.textlo=$lo.sthi=$hi.ststmt=$s.st))
int alt15=3;
switch ( input.LA(1) ) {
case FOREACH:
{
switch ( input.LA(2) ) {
case DOWN:
{
switch ( input.LA(3) ) {
case ID:
{
switch ( input.LA(4) ) {
case DECLARED_LIST:
{
alt15=1;
}
break;
case VAR_LIST:
{
alt15=2;
}
break;
case SEQUENCE:
{
alt15=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 3, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 2, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 1, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 15, 0, input);
throw nvae;
}
switch (alt15) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:230:19: ^( FOREACH ID ^( DECLARED_LIST e= expressionList ) s= statement )
{
match(input,FOREACH,FOLLOW_FOREACH_in_foreachLoop1749);
match(input, Token.DOWN, null);
ID29=(CommonTree)match(input,ID,FOLLOW_ID_in_foreachLoop1751);
varScope.addSymbol((ID29!=null?ID29.getText():null), SymbolType.LOOP_VAR, ScopeType.PIXEL);
match(input,DECLARED_LIST,FOLLOW_DECLARED_LIST_in_foreachLoop1797);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expressionList_in_foreachLoop1801);
e=expressionList();
state._fsp--;
match(input, Token.UP, null);
pushFollow(FOLLOW_statement_in_foreachLoop1806);
s=statement();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 234:17: -> foreachlist(n=++varIndexvar=$ID.textlist=$e.templatesstmt=$s.st)
{
retval.st = templateLib.getInstanceOf("foreachlist",
new STAttrMap().put("n", ++varIndex).put("var", (ID29!=null?ID29.getText():null)).put("list", (e!=null?e.templates:null)).put("stmt", (s!=null?s.st:null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:236:19: ^( FOREACH ID VAR_LIST s= statement )
{
match(input,FOREACH,FOLLOW_FOREACH_in_foreachLoop1870);
match(input, Token.DOWN, null);
ID30=(CommonTree)match(input,ID,FOLLOW_ID_in_foreachLoop1872);
varScope.addSymbol((ID30!=null?ID30.getText():null), SymbolType.LOOP_VAR, ScopeType.PIXEL);
VAR_LIST31=(CommonTree)match(input,VAR_LIST,FOLLOW_VAR_LIST_in_foreachLoop1917);
pushFollow(FOLLOW_statement_in_foreachLoop1921);
s=statement();
state._fsp--;
match(input, Token.UP, null);
addImport("java.util.Iterator");
// TEMPLATE REWRITE
// 241:17: -> foreachlistvar(n=++varIndexvar=$ID.textlistvar=%{$VAR_LIST.text}stmt=$s.st)
{
retval.st = templateLib.getInstanceOf("foreachlistvar",
new STAttrMap().put("n", ++varIndex).put("var", (ID30!=null?ID30.getText():null)).put("listvar", new StringTemplate(templateLib,(VAR_LIST31!=null?VAR_LIST31.getText():null))).put("stmt", (s!=null?s.st:null)));
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:243:19: ^( FOREACH ID ^( SEQUENCE lo= expression hi= expression ) s= statement )
{
match(input,FOREACH,FOLLOW_FOREACH_in_foreachLoop2019);
match(input, Token.DOWN, null);
ID32=(CommonTree)match(input,ID,FOLLOW_ID_in_foreachLoop2021);
varScope.addSymbol((ID32!=null?ID32.getText():null), SymbolType.LOOP_VAR, ScopeType.PIXEL);
match(input,SEQUENCE,FOLLOW_SEQUENCE_in_foreachLoop2067);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_foreachLoop2071);
lo=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_foreachLoop2075);
hi=expression();
state._fsp--;
match(input, Token.UP, null);
pushFollow(FOLLOW_statement_in_foreachLoop2080);
s=statement();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 247:17: -> foreachseq(n=++varIndexvar=$ID.textlo=$lo.sthi=$hi.ststmt=$s.st)
{
retval.st = templateLib.getInstanceOf("foreachseq",
new STAttrMap().put("n", ++varIndex).put("var", (ID32!=null?ID32.getText():null)).put("lo", (lo!=null?lo.st:null)).put("hi", (hi!=null?hi.st:null)).put("stmt", (s!=null?s.st:null)));
}
}
break;
}
varScope.dropLevel();
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "foreachLoop"
public static class ifCall_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "ifCall"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:251:1: ifCall : ^( IF expression s1= statement (s2= statement )? ) -> {$s2.st == null}? ifcall(cond=$expression.stcase=$s1.st) -> ifelsecall(cond=$expression.stcase1=$s1.stcase2=$s2.st);
public final RuntimeSourceGenerator.ifCall_return ifCall() throws RecognitionException {
RuntimeSourceGenerator.ifCall_return retval = new RuntimeSourceGenerator.ifCall_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.statement_return s1 = null;
RuntimeSourceGenerator.statement_return s2 = null;
RuntimeSourceGenerator.expression_return expression33 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:251:17: ( ^( IF expression s1= statement (s2= statement )? ) -> {$s2.st == null}? ifcall(cond=$expression.stcase=$s1.st) -> ifelsecall(cond=$expression.stcase1=$s1.stcase2=$s2.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:251:19: ^( IF expression s1= statement (s2= statement )? )
{
match(input,IF,FOLLOW_IF_in_ifCall2163);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_ifCall2165);
expression33=expression();
state._fsp--;
pushFollow(FOLLOW_statement_in_ifCall2169);
s1=statement();
state._fsp--;
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:251:50: (s2= statement )?
int alt16=2;
switch ( input.LA(1) ) {
case BLOCK:
case CON_CALL:
case DECLARED_LIST:
case FUNC_CALL:
case IMAGE_POS:
case PAR:
case POSTFIX:
case PREFIX:
case VAR_IMAGE_SCOPE:
case VAR_SOURCE:
case CONSTANT:
case IMAGE_WRITE:
case VAR_PIXEL_SCOPE:
case VAR_PROVIDED:
case VAR_LOOP:
case VAR_LIST:
case EQ:
case WHILE:
case UNTIL:
case FOREACH:
case IF:
case BREAKIF:
case BREAK:
case APPEND:
case TIMESEQ:
case DIVEQ:
case MODEQ:
case PLUSEQ:
case MINUSEQ:
case OR:
case XOR:
case AND:
case LOGICALEQ:
case NE:
case GT:
case GE:
case LE:
case LT:
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
case POW:
case INT_LITERAL:
case FLOAT_LITERAL:
{
alt16=1;
}
break;
}
switch (alt16) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:251:50: s2= statement
{
pushFollow(FOLLOW_statement_in_ifCall2173);
s2=statement();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 252:17: -> {$s2.st == null}? ifcall(cond=$expression.stcase=$s1.st)
if ((s2!=null?s2.st:null) == null) {
retval.st = templateLib.getInstanceOf("ifcall",
new STAttrMap().put("cond", (expression33!=null?expression33.st:null)).put("case", (s1!=null?s1.st:null)));
}
else // 253:17: -> ifelsecall(cond=$expression.stcase1=$s1.stcase2=$s2.st)
{
retval.st = templateLib.getInstanceOf("ifelsecall",
new STAttrMap().put("cond", (expression33!=null?expression33.st:null)).put("case1", (s1!=null?s1.st:null)).put("case2", (s2!=null?s2.st:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "ifCall"
public static class expression_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "expression"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:257:1: expression : ( ^( FUNC_CALL ID el= expressionList ) -> call(name=getRuntimeExpr($ID.text, $el.argTypes)args=$el.templates) | ^( CON_CALL el= expressionList ) -> concall(args=$el.templates) | imagePos -> {$imagePos.st} | binaryExpression -> {$binaryExpression.st} | ^( PREFIX NOT e= expression ) -> call(name=getRuntimeExpr(\"NOT\", \"D\")args=$e.st) | ^( PREFIX prefixOp e= expression ) -> preop(op=$prefixOp.stexpr=$e.st) | ^( POSTFIX postfixOp e= expression ) -> postop(op=$postfixOp.stexpr=$e.st) | ^( PAR e= expression ) -> par(expr=$e.st) | listOperation -> {$listOperation.st} | listLiteral -> {$listLiteral.st} | var -> {$var.st} | VAR_SOURCE -> getsourcevalue(var=$VAR_SOURCE.text) | CONSTANT -> {%{getConstantString($CONSTANT.text)}} | literal -> {$literal.st});
public final RuntimeSourceGenerator.expression_return expression() throws RecognitionException {
RuntimeSourceGenerator.expression_return retval = new RuntimeSourceGenerator.expression_return();
retval.start = input.LT(1);
CommonTree ID34=null;
CommonTree VAR_SOURCE42=null;
CommonTree CONSTANT43=null;
RuntimeSourceGenerator.expressionList_return el = null;
RuntimeSourceGenerator.expression_return e = null;
RuntimeSourceGenerator.imagePos_return imagePos35 = null;
RuntimeSourceGenerator.binaryExpression_return binaryExpression36 = null;
RuntimeSourceGenerator.prefixOp_return prefixOp37 = null;
RuntimeSourceGenerator.postfixOp_return postfixOp38 = null;
RuntimeSourceGenerator.listOperation_return listOperation39 = null;
RuntimeSourceGenerator.listLiteral_return listLiteral40 = null;
RuntimeSourceGenerator.var_return var41 = null;
RuntimeSourceGenerator.literal_return literal44 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:257:17: ( ^( FUNC_CALL ID el= expressionList ) -> call(name=getRuntimeExpr($ID.text, $el.argTypes)args=$el.templates) | ^( CON_CALL el= expressionList ) -> concall(args=$el.templates) | imagePos -> {$imagePos.st} | binaryExpression -> {$binaryExpression.st} | ^( PREFIX NOT e= expression ) -> call(name=getRuntimeExpr(\"NOT\", \"D\")args=$e.st) | ^( PREFIX prefixOp e= expression ) -> preop(op=$prefixOp.stexpr=$e.st) | ^( POSTFIX postfixOp e= expression ) -> postop(op=$postfixOp.stexpr=$e.st) | ^( PAR e= expression ) -> par(expr=$e.st) | listOperation -> {$listOperation.st} | listLiteral -> {$listLiteral.st} | var -> {$var.st} | VAR_SOURCE -> getsourcevalue(var=$VAR_SOURCE.text) | CONSTANT -> {%{getConstantString($CONSTANT.text)}} | literal -> {$literal.st})
int alt17=14;
alt17 = dfa17.predict(input);
switch (alt17) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:257:19: ^( FUNC_CALL ID el= expressionList )
{
match(input,FUNC_CALL,FOLLOW_FUNC_CALL_in_expression2274);
match(input, Token.DOWN, null);
ID34=(CommonTree)match(input,ID,FOLLOW_ID_in_expression2276);
pushFollow(FOLLOW_expressionList_in_expression2280);
el=expressionList();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 258:17: -> call(name=getRuntimeExpr($ID.text, $el.argTypes)args=$el.templates)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", getRuntimeExpr((ID34!=null?ID34.getText():null), (el!=null?el.argTypes:null))).put("args", (el!=null?el.templates:null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:260:19: ^( CON_CALL el= expressionList )
{
match(input,CON_CALL,FOLLOW_CON_CALL_in_expression2334);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expressionList_in_expression2338);
el=expressionList();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 260:49: -> concall(args=$el.templates)
{
retval.st = templateLib.getInstanceOf("concall",
new STAttrMap().put("args", (el!=null?el.templates:null)));
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:262:19: imagePos
{
pushFollow(FOLLOW_imagePos_in_expression2369);
imagePos35=imagePos();
state._fsp--;
// TEMPLATE REWRITE
// 262:28: -> {$imagePos.st}
{
retval.st = (imagePos35!=null?imagePos35.st:null);
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:264:19: binaryExpression
{
pushFollow(FOLLOW_binaryExpression_in_expression2394);
binaryExpression36=binaryExpression();
state._fsp--;
// TEMPLATE REWRITE
// 264:36: -> {$binaryExpression.st}
{
retval.st = (binaryExpression36!=null?binaryExpression36.st:null);
}
}
break;
case 5 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:266:19: ^( PREFIX NOT e= expression )
{
match(input,PREFIX,FOLLOW_PREFIX_in_expression2420);
match(input, Token.DOWN, null);
match(input,NOT,FOLLOW_NOT_in_expression2422);
pushFollow(FOLLOW_expression_in_expression2426);
e=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 267:17: -> call(name=getRuntimeExpr(\"NOT\", \"D\")args=$e.st)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", getRuntimeExpr("NOT", "D")).put("args", (e!=null?e.st:null)));
}
}
break;
case 6 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:269:19: ^( PREFIX prefixOp e= expression )
{
match(input,PREFIX,FOLLOW_PREFIX_in_expression2480);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_prefixOp_in_expression2482);
prefixOp37=prefixOp();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2486);
e=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 269:51: -> preop(op=$prefixOp.stexpr=$e.st)
{
retval.st = templateLib.getInstanceOf("preop",
new STAttrMap().put("op", (prefixOp37!=null?prefixOp37.st:null)).put("expr", (e!=null?e.st:null)));
}
}
break;
case 7 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:271:19: ^( POSTFIX postfixOp e= expression )
{
match(input,POSTFIX,FOLLOW_POSTFIX_in_expression2523);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_postfixOp_in_expression2525);
postfixOp38=postfixOp();
state._fsp--;
pushFollow(FOLLOW_expression_in_expression2529);
e=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 271:53: -> postop(op=$postfixOp.stexpr=$e.st)
{
retval.st = templateLib.getInstanceOf("postop",
new STAttrMap().put("op", (postfixOp38!=null?postfixOp38.st:null)).put("expr", (e!=null?e.st:null)));
}
}
break;
case 8 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:273:19: ^( PAR e= expression )
{
match(input,PAR,FOLLOW_PAR_in_expression2566);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_expression2570);
e=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 273:39: -> par(expr=$e.st)
{
retval.st = templateLib.getInstanceOf("par",
new STAttrMap().put("expr", (e!=null?e.st:null)));
}
}
break;
case 9 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:275:19: listOperation
{
pushFollow(FOLLOW_listOperation_in_expression2601);
listOperation39=listOperation();
state._fsp--;
// TEMPLATE REWRITE
// 275:33: -> {$listOperation.st}
{
retval.st = (listOperation39!=null?listOperation39.st:null);
}
}
break;
case 10 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:277:19: listLiteral
{
pushFollow(FOLLOW_listLiteral_in_expression2626);
listLiteral40=listLiteral();
state._fsp--;
// TEMPLATE REWRITE
// 277:31: -> {$listLiteral.st}
{
retval.st = (listLiteral40!=null?listLiteral40.st:null);
}
}
break;
case 11 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:279:19: var
{
pushFollow(FOLLOW_var_in_expression2651);
var41=var();
state._fsp--;
// TEMPLATE REWRITE
// 279:23: -> {$var.st}
{
retval.st = (var41!=null?var41.st:null);
}
}
break;
case 12 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:281:19: VAR_SOURCE
{
VAR_SOURCE42=(CommonTree)match(input,VAR_SOURCE,FOLLOW_VAR_SOURCE_in_expression2676);
// TEMPLATE REWRITE
// 281:30: -> getsourcevalue(var=$VAR_SOURCE.text)
{
retval.st = templateLib.getInstanceOf("getsourcevalue",
new STAttrMap().put("var", (VAR_SOURCE42!=null?VAR_SOURCE42.getText():null)));
}
}
break;
case 13 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:283:19: CONSTANT
{
CONSTANT43=(CommonTree)match(input,CONSTANT,FOLLOW_CONSTANT_in_expression2706);
// TEMPLATE REWRITE
// 283:28: -> {%{getConstantString($CONSTANT.text)}}
{
retval.st = new StringTemplate(templateLib,getConstantString((CONSTANT43!=null?CONSTANT43.getText():null)));
}
}
break;
case 14 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:285:19: literal
{
pushFollow(FOLLOW_literal_in_expression2731);
literal44=literal();
state._fsp--;
// TEMPLATE REWRITE
// 285:27: -> {$literal.st}
{
retval.st = (literal44!=null?literal44.st:null);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "expression"
public static class listOperation_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "listOperation"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:289:1: listOperation : ^( APPEND VAR_LIST expression ) -> listappend(var=$VAR_LIST.textexpr=$expression.st);
public final RuntimeSourceGenerator.listOperation_return listOperation() throws RecognitionException {
RuntimeSourceGenerator.listOperation_return retval = new RuntimeSourceGenerator.listOperation_return();
retval.start = input.LT(1);
CommonTree VAR_LIST45=null;
RuntimeSourceGenerator.expression_return expression46 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:289:17: ( ^( APPEND VAR_LIST expression ) -> listappend(var=$VAR_LIST.textexpr=$expression.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:289:19: ^( APPEND VAR_LIST expression )
{
match(input,APPEND,FOLLOW_APPEND_in_listOperation2764);
match(input, Token.DOWN, null);
VAR_LIST45=(CommonTree)match(input,VAR_LIST,FOLLOW_VAR_LIST_in_listOperation2766);
pushFollow(FOLLOW_expression_in_listOperation2768);
expression46=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 290:17: -> listappend(var=$VAR_LIST.textexpr=$expression.st)
{
retval.st = templateLib.getInstanceOf("listappend",
new STAttrMap().put("var", (VAR_LIST45!=null?VAR_LIST45.getText():null)).put("expr", (expression46!=null?expression46.st:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "listOperation"
public static class listLiteral_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "listLiteral"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:294:1: listLiteral : ^( DECLARED_LIST e= expressionList ) -> listliteral(empty=$e.templates.isEmpty()exprs=$e.templates);
public final RuntimeSourceGenerator.listLiteral_return listLiteral() throws RecognitionException {
RuntimeSourceGenerator.listLiteral_return retval = new RuntimeSourceGenerator.listLiteral_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.expressionList_return e = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:294:17: ( ^( DECLARED_LIST e= expressionList ) -> listliteral(empty=$e.templates.isEmpty()exprs=$e.templates))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:294:19: ^( DECLARED_LIST e= expressionList )
{
match(input,DECLARED_LIST,FOLLOW_DECLARED_LIST_in_listLiteral2831);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expressionList_in_listLiteral2835);
e=expressionList();
state._fsp--;
match(input, Token.UP, null);
addImport("java.util.Arrays", "java.util.ArrayList");
// TEMPLATE REWRITE
// 296:17: -> listliteral(empty=$e.templates.isEmpty()exprs=$e.templates)
{
retval.st = templateLib.getInstanceOf("listliteral",
new STAttrMap().put("empty", (e!=null?e.templates:null).isEmpty()).put("exprs", (e!=null?e.templates:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "listLiteral"
public static class var_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "var"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:300:1: var : ( VAR_IMAGE_SCOPE -> {%{getImageScopeVarExpr($VAR_IMAGE_SCOPE.text)}} | VAR_PIXEL_SCOPE -> {%{$VAR_PIXEL_SCOPE.text}} | VAR_PROVIDED -> {%{$VAR_PROVIDED.text}} | VAR_LOOP -> {%{$VAR_LOOP.text}} | VAR_LIST -> {%{\"(List)\" + $VAR_LIST.text}});
public final RuntimeSourceGenerator.var_return var() throws RecognitionException {
RuntimeSourceGenerator.var_return retval = new RuntimeSourceGenerator.var_return();
retval.start = input.LT(1);
CommonTree VAR_IMAGE_SCOPE47=null;
CommonTree VAR_PIXEL_SCOPE48=null;
CommonTree VAR_PROVIDED49=null;
CommonTree VAR_LOOP50=null;
CommonTree VAR_LIST51=null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:300:17: ( VAR_IMAGE_SCOPE -> {%{getImageScopeVarExpr($VAR_IMAGE_SCOPE.text)}} | VAR_PIXEL_SCOPE -> {%{$VAR_PIXEL_SCOPE.text}} | VAR_PROVIDED -> {%{$VAR_PROVIDED.text}} | VAR_LOOP -> {%{$VAR_LOOP.text}} | VAR_LIST -> {%{\"(List)\" + $VAR_LIST.text}})
int alt18=5;
switch ( input.LA(1) ) {
case VAR_IMAGE_SCOPE:
{
alt18=1;
}
break;
case VAR_PIXEL_SCOPE:
{
alt18=2;
}
break;
case VAR_PROVIDED:
{
alt18=3;
}
break;
case VAR_LOOP:
{
alt18=4;
}
break;
case VAR_LIST:
{
alt18=5;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 18, 0, input);
throw nvae;
}
switch (alt18) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:300:19: VAR_IMAGE_SCOPE
{
VAR_IMAGE_SCOPE47=(CommonTree)match(input,VAR_IMAGE_SCOPE,FOLLOW_VAR_IMAGE_SCOPE_in_var2923);
// TEMPLATE REWRITE
// 300:35: -> {%{getImageScopeVarExpr($VAR_IMAGE_SCOPE.text)}}
{
retval.st = new StringTemplate(templateLib,getImageScopeVarExpr((VAR_IMAGE_SCOPE47!=null?VAR_IMAGE_SCOPE47.getText():null)));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:301:19: VAR_PIXEL_SCOPE
{
VAR_PIXEL_SCOPE48=(CommonTree)match(input,VAR_PIXEL_SCOPE,FOLLOW_VAR_PIXEL_SCOPE_in_var2947);
// TEMPLATE REWRITE
// 301:35: -> {%{$VAR_PIXEL_SCOPE.text}}
{
retval.st = new StringTemplate(templateLib,(VAR_PIXEL_SCOPE48!=null?VAR_PIXEL_SCOPE48.getText():null));
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:302:19: VAR_PROVIDED
{
VAR_PROVIDED49=(CommonTree)match(input,VAR_PROVIDED,FOLLOW_VAR_PROVIDED_in_var2971);
// TEMPLATE REWRITE
// 302:32: -> {%{$VAR_PROVIDED.text}}
{
retval.st = new StringTemplate(templateLib,(VAR_PROVIDED49!=null?VAR_PROVIDED49.getText():null));
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:303:19: VAR_LOOP
{
VAR_LOOP50=(CommonTree)match(input,VAR_LOOP,FOLLOW_VAR_LOOP_in_var2995);
// TEMPLATE REWRITE
// 303:28: -> {%{$VAR_LOOP.text}}
{
retval.st = new StringTemplate(templateLib,(VAR_LOOP50!=null?VAR_LOOP50.getText():null));
}
}
break;
case 5 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:304:19: VAR_LIST
{
VAR_LIST51=(CommonTree)match(input,VAR_LIST,FOLLOW_VAR_LIST_in_var3019);
// TEMPLATE REWRITE
// 304:28: -> {%{\"(List)\" + $VAR_LIST.text}}
{
retval.st = new StringTemplate(templateLib,"(List)" + (VAR_LIST51!=null?VAR_LIST51.getText():null));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "var"
public static class binaryExpression_return extends TreeRuleReturnScope {
public String src;
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "binaryExpression"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:308:1: binaryExpression returns [String src] : ( ^( POW x= expression y= expression ) -> pow(x=x.sty=y.st) | ^( OR e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( XOR e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( AND e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LOGICALEQ e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( NE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( GT e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( GE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LT e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( arithmeticOp x= expression y= expression ) -> binaryexpr(lhs=x.stop=$arithmeticOp.strhs=y.st));
public final RuntimeSourceGenerator.binaryExpression_return binaryExpression() throws RecognitionException {
RuntimeSourceGenerator.binaryExpression_return retval = new RuntimeSourceGenerator.binaryExpression_return();
retval.start = input.LT(1);
List list_e=null;
RuntimeSourceGenerator.expression_return x = null;
RuntimeSourceGenerator.expression_return y = null;
RuntimeSourceGenerator.arithmeticOp_return arithmeticOp52 = null;
RuleReturnScope e = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:309:17: ( ^( POW x= expression y= expression ) -> pow(x=x.sty=y.st) | ^( OR e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( XOR e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( AND e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LOGICALEQ e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( NE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( GT e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( GE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LT e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( LE e+= expression e+= expression ) -> call(name=$srcargs=$e) | ^( arithmeticOp x= expression y= expression ) -> binaryexpr(lhs=x.stop=$arithmeticOp.strhs=y.st))
int alt19=11;
switch ( input.LA(1) ) {
case POW:
{
alt19=1;
}
break;
case OR:
{
alt19=2;
}
break;
case XOR:
{
alt19=3;
}
break;
case AND:
{
alt19=4;
}
break;
case LOGICALEQ:
{
alt19=5;
}
break;
case NE:
{
alt19=6;
}
break;
case GT:
{
alt19=7;
}
break;
case GE:
{
alt19=8;
}
break;
case LT:
{
alt19=9;
}
break;
case LE:
{
alt19=10;
}
break;
case PLUS:
case MINUS:
case TIMES:
case DIV:
case MOD:
{
alt19=11;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 19, 0, input);
throw nvae;
}
switch (alt19) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:309:19: ^( POW x= expression y= expression )
{
match(input,POW,FOLLOW_POW_in_binaryExpression3070);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3074);
x=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_binaryExpression3078);
y=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 309:52: -> pow(x=x.sty=y.st)
{
retval.st = templateLib.getInstanceOf("pow",
new STAttrMap().put("x", x.st).put("y", y.st));
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:311:19: ^( OR e+= expression e+= expression )
{
match(input,OR,FOLLOW_OR_in_binaryExpression3115);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3119);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3123);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("OR", "D", "D");
// TEMPLATE REWRITE
// 312:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:314:19: ^( XOR e+= expression e+= expression )
{
match(input,XOR,FOLLOW_XOR_in_binaryExpression3179);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3183);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3187);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("XOR", "D", "D");
// TEMPLATE REWRITE
// 315:61: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 4 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:317:19: ^( AND e+= expression e+= expression )
{
match(input,AND,FOLLOW_AND_in_binaryExpression3243);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3247);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3251);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("AND", "D", "D");
// TEMPLATE REWRITE
// 318:61: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 5 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:320:19: ^( LOGICALEQ e+= expression e+= expression )
{
match(input,LOGICALEQ,FOLLOW_LOGICALEQ_in_binaryExpression3307);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3311);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3315);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("EQ", "D", "D");
// TEMPLATE REWRITE
// 321:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 6 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:323:19: ^( NE e+= expression e+= expression )
{
match(input,NE,FOLLOW_NE_in_binaryExpression3371);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3375);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3379);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("NE", "D", "D");
// TEMPLATE REWRITE
// 324:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 7 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:326:19: ^( GT e+= expression e+= expression )
{
match(input,GT,FOLLOW_GT_in_binaryExpression3435);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3439);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3443);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("GT", "D", "D");
// TEMPLATE REWRITE
// 327:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 8 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:329:19: ^( GE e+= expression e+= expression )
{
match(input,GE,FOLLOW_GE_in_binaryExpression3499);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3503);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3507);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("GE", "D", "D");
// TEMPLATE REWRITE
// 330:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 9 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:332:19: ^( LT e+= expression e+= expression )
{
match(input,LT,FOLLOW_LT_in_binaryExpression3563);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3567);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3571);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("LT", "D", "D");
// TEMPLATE REWRITE
// 333:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 10 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:335:19: ^( LE e+= expression e+= expression )
{
match(input,LE,FOLLOW_LE_in_binaryExpression3627);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3631);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
pushFollow(FOLLOW_expression_in_binaryExpression3635);
e=expression();
state._fsp--;
if (list_e==null) list_e=new ArrayList();
list_e.add(e.getTemplate());
match(input, Token.UP, null);
retval.src = getRuntimeExpr("LE", "D", "D");
// TEMPLATE REWRITE
// 336:60: -> call(name=$srcargs=$e)
{
retval.st = templateLib.getInstanceOf("call",
new STAttrMap().put("name", retval.src).put("args", list_e));
}
}
break;
case 11 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:338:19: ^( arithmeticOp x= expression y= expression )
{
pushFollow(FOLLOW_arithmeticOp_in_binaryExpression3691);
arithmeticOp52=arithmeticOp();
state._fsp--;
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_binaryExpression3695);
x=expression();
state._fsp--;
pushFollow(FOLLOW_expression_in_binaryExpression3699);
y=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 339:17: -> binaryexpr(lhs=x.stop=$arithmeticOp.strhs=y.st)
{
retval.st = templateLib.getInstanceOf("binaryexpr",
new STAttrMap().put("lhs", x.st).put("op", (arithmeticOp52!=null?arithmeticOp52.st:null)).put("rhs", y.st));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "binaryExpression"
public static class arithmeticOp_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "arithmeticOp"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:343:1: arithmeticOp : ( TIMES | DIV | MOD | PLUS | MINUS );
public final RuntimeSourceGenerator.arithmeticOp_return arithmeticOp() throws RecognitionException {
RuntimeSourceGenerator.arithmeticOp_return retval = new RuntimeSourceGenerator.arithmeticOp_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:345:17: ( TIMES | DIV | MOD | PLUS | MINUS )
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:
{
if ( (input.LA(1)>=PLUS && input.LA(1)<=MOD) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.st = new StringTemplate(templateLib,((CommonTree)retval.start).getText());
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "arithmeticOp"
public static class literal_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "literal"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:353:1: literal : ( INT_LITERAL -> {%{$INT_LITERAL.text + \".0\"}} | FLOAT_LITERAL -> {%{$FLOAT_LITERAL.text}});
public final RuntimeSourceGenerator.literal_return literal() throws RecognitionException {
RuntimeSourceGenerator.literal_return retval = new RuntimeSourceGenerator.literal_return();
retval.start = input.LT(1);
CommonTree INT_LITERAL53=null;
CommonTree FLOAT_LITERAL54=null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:353:17: ( INT_LITERAL -> {%{$INT_LITERAL.text + \".0\"}} | FLOAT_LITERAL -> {%{$FLOAT_LITERAL.text}})
int alt20=2;
switch ( input.LA(1) ) {
case INT_LITERAL:
{
alt20=1;
}
break;
case FLOAT_LITERAL:
{
alt20=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 20, 0, input);
throw nvae;
}
switch (alt20) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:353:19: INT_LITERAL
{
INT_LITERAL53=(CommonTree)match(input,INT_LITERAL,FOLLOW_INT_LITERAL_in_literal3897);
// TEMPLATE REWRITE
// 353:31: -> {%{$INT_LITERAL.text + \".0\"}}
{
retval.st = new StringTemplate(templateLib,(INT_LITERAL53!=null?INT_LITERAL53.getText():null) + ".0");
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:354:19: FLOAT_LITERAL
{
FLOAT_LITERAL54=(CommonTree)match(input,FLOAT_LITERAL,FOLLOW_FLOAT_LITERAL_in_literal3921);
// TEMPLATE REWRITE
// 354:33: -> {%{$FLOAT_LITERAL.text}}
{
retval.st = new StringTemplate(templateLib,(FLOAT_LITERAL54!=null?FLOAT_LITERAL54.getText():null));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "literal"
public static class imagePos_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "imagePos"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:1: imagePos : ^( IMAGE_POS VAR_SOURCE (b= bandSpecifier )? (p= pixelSpecifier )? ) -> getsourcevalue(var=$VAR_SOURCE.textpixel=$p.stband=$b.st);
public final RuntimeSourceGenerator.imagePos_return imagePos() throws RecognitionException {
RuntimeSourceGenerator.imagePos_return retval = new RuntimeSourceGenerator.imagePos_return();
retval.start = input.LT(1);
CommonTree VAR_SOURCE55=null;
RuntimeSourceGenerator.bandSpecifier_return b = null;
RuntimeSourceGenerator.pixelSpecifier_return p = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:17: ( ^( IMAGE_POS VAR_SOURCE (b= bandSpecifier )? (p= pixelSpecifier )? ) -> getsourcevalue(var=$VAR_SOURCE.textpixel=$p.stband=$b.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:19: ^( IMAGE_POS VAR_SOURCE (b= bandSpecifier )? (p= pixelSpecifier )? )
{
match(input,IMAGE_POS,FOLLOW_IMAGE_POS_in_imagePos3959);
match(input, Token.DOWN, null);
VAR_SOURCE55=(CommonTree)match(input,VAR_SOURCE,FOLLOW_VAR_SOURCE_in_imagePos3961);
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:43: (b= bandSpecifier )?
int alt21=2;
switch ( input.LA(1) ) {
case BAND_REF:
{
alt21=1;
}
break;
}
switch (alt21) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:43: b= bandSpecifier
{
pushFollow(FOLLOW_bandSpecifier_in_imagePos3965);
b=bandSpecifier();
state._fsp--;
}
break;
}
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:60: (p= pixelSpecifier )?
int alt22=2;
switch ( input.LA(1) ) {
case PIXEL_REF:
{
alt22=1;
}
break;
}
switch (alt22) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:358:60: p= pixelSpecifier
{
pushFollow(FOLLOW_pixelSpecifier_in_imagePos3970);
p=pixelSpecifier();
state._fsp--;
}
break;
}
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 359:17: -> getsourcevalue(var=$VAR_SOURCE.textpixel=$p.stband=$b.st)
{
retval.st = templateLib.getInstanceOf("getsourcevalue",
new STAttrMap().put("var", (VAR_SOURCE55!=null?VAR_SOURCE55.getText():null)).put("pixel", (p!=null?p.st:null)).put("band", (b!=null?b.st:null)));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "imagePos"
public static class bandSpecifier_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "bandSpecifier"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:363:1: bandSpecifier : ^( BAND_REF expression ) -> {$expression.st};
public final RuntimeSourceGenerator.bandSpecifier_return bandSpecifier() throws RecognitionException {
RuntimeSourceGenerator.bandSpecifier_return retval = new RuntimeSourceGenerator.bandSpecifier_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.expression_return expression56 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:363:17: ( ^( BAND_REF expression ) -> {$expression.st})
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:363:19: ^( BAND_REF expression )
{
match(input,BAND_REF,FOLLOW_BAND_REF_in_bandSpecifier4036);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_bandSpecifier4038);
expression56=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 363:42: -> {$expression.st}
{
retval.st = (expression56!=null?expression56.st:null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "bandSpecifier"
public static class pixelSpecifier_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "pixelSpecifier"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:367:1: pixelSpecifier : ^( PIXEL_REF xpos= pixelPos[\"_x\"] ypos= pixelPos[\"_y\"] ) -> pixel(x=xpos.sty=ypos.st);
public final RuntimeSourceGenerator.pixelSpecifier_return pixelSpecifier() throws RecognitionException {
RuntimeSourceGenerator.pixelSpecifier_return retval = new RuntimeSourceGenerator.pixelSpecifier_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.pixelPos_return xpos = null;
RuntimeSourceGenerator.pixelPos_return ypos = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:367:17: ( ^( PIXEL_REF xpos= pixelPos[\"_x\"] ypos= pixelPos[\"_y\"] ) -> pixel(x=xpos.sty=ypos.st))
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:367:19: ^( PIXEL_REF xpos= pixelPos[\"_x\"] ypos= pixelPos[\"_y\"] )
{
match(input,PIXEL_REF,FOLLOW_PIXEL_REF_in_pixelSpecifier4071);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier4075);
xpos=pixelPos("_x");
state._fsp--;
pushFollow(FOLLOW_pixelPos_in_pixelSpecifier4080);
ypos=pixelPos("_y");
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 367:72: -> pixel(x=xpos.sty=ypos.st)
{
retval.st = templateLib.getInstanceOf("pixel",
new STAttrMap().put("x", xpos.st).put("y", ypos.st));
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "pixelSpecifier"
public static class pixelPos_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "pixelPos"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:371:1: pixelPos[String var] : ( ^( ABS_POS expression ) -> {$expression.st} | ^( REL_POS expression ) -> binaryexpr(lhs=$varop=%{\"+\"}rhs=$expression.st));
public final RuntimeSourceGenerator.pixelPos_return pixelPos(String var) throws RecognitionException {
RuntimeSourceGenerator.pixelPos_return retval = new RuntimeSourceGenerator.pixelPos_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.expression_return expression57 = null;
RuntimeSourceGenerator.expression_return expression58 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:372:17: ( ^( ABS_POS expression ) -> {$expression.st} | ^( REL_POS expression ) -> binaryexpr(lhs=$varop=%{\"+\"}rhs=$expression.st))
int alt23=2;
switch ( input.LA(1) ) {
case ABS_POS:
{
alt23=1;
}
break;
case REL_POS:
{
alt23=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 23, 0, input);
throw nvae;
}
switch (alt23) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:372:19: ^( ABS_POS expression )
{
match(input,ABS_POS,FOLLOW_ABS_POS_in_pixelPos4140);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_pixelPos4142);
expression57=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 372:41: -> {$expression.st}
{
retval.st = (expression57!=null?expression57.st:null);
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:373:19: ^( REL_POS expression )
{
match(input,REL_POS,FOLLOW_REL_POS_in_pixelPos4168);
match(input, Token.DOWN, null);
pushFollow(FOLLOW_expression_in_pixelPos4170);
expression58=expression();
state._fsp--;
match(input, Token.UP, null);
// TEMPLATE REWRITE
// 373:41: -> binaryexpr(lhs=$varop=%{\"+\"}rhs=$expression.st)
{
retval.st = templateLib.getInstanceOf("binaryexpr",
new STAttrMap().put("lhs", var).put("op", new StringTemplate(templateLib,"+")).put("rhs", (expression58!=null?expression58.st:null)));
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "pixelPos"
public static class prefixOp_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "prefixOp"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:377:1: prefixOp : ( PLUS -> {%{\"+\"}} | MINUS -> {%{\"-\"}} | incdecOp -> {$incdecOp.st});
public final RuntimeSourceGenerator.prefixOp_return prefixOp() throws RecognitionException {
RuntimeSourceGenerator.prefixOp_return retval = new RuntimeSourceGenerator.prefixOp_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.incdecOp_return incdecOp59 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:377:17: ( PLUS -> {%{\"+\"}} | MINUS -> {%{\"-\"}} | incdecOp -> {$incdecOp.st})
int alt24=3;
switch ( input.LA(1) ) {
case PLUS:
{
alt24=1;
}
break;
case MINUS:
{
alt24=2;
}
break;
case INCR:
case DECR:
{
alt24=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 24, 0, input);
throw nvae;
}
switch (alt24) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:377:19: PLUS
{
match(input,PLUS,FOLLOW_PLUS_in_prefixOp4223);
// TEMPLATE REWRITE
// 377:24: -> {%{\"+\"}}
{
retval.st = new StringTemplate(templateLib,"+");
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:378:19: MINUS
{
match(input,MINUS,FOLLOW_MINUS_in_prefixOp4247);
// TEMPLATE REWRITE
// 378:25: -> {%{\"-\"}}
{
retval.st = new StringTemplate(templateLib,"-");
}
}
break;
case 3 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:379:19: incdecOp
{
pushFollow(FOLLOW_incdecOp_in_prefixOp4271);
incdecOp59=incdecOp();
state._fsp--;
// TEMPLATE REWRITE
// 379:29: -> {$incdecOp.st}
{
retval.st = (incdecOp59!=null?incdecOp59.st:null);
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "prefixOp"
public static class postfixOp_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "postfixOp"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:383:1: postfixOp : incdecOp -> {$incdecOp.st};
public final RuntimeSourceGenerator.postfixOp_return postfixOp() throws RecognitionException {
RuntimeSourceGenerator.postfixOp_return retval = new RuntimeSourceGenerator.postfixOp_return();
retval.start = input.LT(1);
RuntimeSourceGenerator.incdecOp_return incdecOp60 = null;
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:383:17: ( incdecOp -> {$incdecOp.st})
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:383:19: incdecOp
{
pushFollow(FOLLOW_incdecOp_in_postfixOp4308);
incdecOp60=incdecOp();
state._fsp--;
// TEMPLATE REWRITE
// 383:28: -> {$incdecOp.st}
{
retval.st = (incdecOp60!=null?incdecOp60.st:null);
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "postfixOp"
public static class incdecOp_return extends TreeRuleReturnScope {
public StringTemplate st;
public Object getTemplate() { return st; }
public String toString() { return st==null?null:st.toString(); }
};
// $ANTLR start "incdecOp"
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:387:1: incdecOp : ( INCR -> {%{\"++\"}} | DECR -> {%{\"--\"}});
public final RuntimeSourceGenerator.incdecOp_return incdecOp() throws RecognitionException {
RuntimeSourceGenerator.incdecOp_return retval = new RuntimeSourceGenerator.incdecOp_return();
retval.start = input.LT(1);
try {
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:387:17: ( INCR -> {%{\"++\"}} | DECR -> {%{\"--\"}})
int alt25=2;
switch ( input.LA(1) ) {
case INCR:
{
alt25=1;
}
break;
case DECR:
{
alt25=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 25, 0, input);
throw nvae;
}
switch (alt25) {
case 1 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:387:19: INCR
{
match(input,INCR,FOLLOW_INCR_in_incdecOp4345);
// TEMPLATE REWRITE
// 387:24: -> {%{\"++\"}}
{
retval.st = new StringTemplate(templateLib,"++");
}
}
break;
case 2 :
// org/jaitools/jiffle/parser/RuntimeSourceGenerator.g:388:19: DECR
{
match(input,DECR,FOLLOW_DECR_in_incdecOp4369);
// TEMPLATE REWRITE
// 388:24: -> {%{\"--\"}}
{
retval.st = new StringTemplate(templateLib,"--");
}
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
}
return retval;
}
// $ANTLR end "incdecOp"
// Delegated rules
protected DFA9 dfa9 = new DFA9(this);
protected DFA17 dfa17 = new DFA17(this);
static final String DFA9_eotS =
"\13\uffff";
static final String DFA9_eofS =
"\13\uffff";
static final String DFA9_minS =
"\1\7\1\uffff\1\2\6\uffff\1\25\1\uffff";
static final String DFA9_maxS =
"\1\123\1\uffff\1\2\6\uffff\1\36\1\uffff";
static final String DFA9_acceptS =
"\1\uffff\1\1\1\uffff\1\2\1\4\1\5\1\6\1\7\1\10\1\uffff\1\3";
static final String DFA9_specialS =
"\13\uffff}>";
static final String[] DFA9_transitionS = {
"\1\10\1\uffff\1\10\1\uffff\2\10\1\uffff\1\10\1\uffff\2\10\3"+
"\uffff\3\10\1\1\2\uffff\4\10\4\uffff\1\2\5\uffff\1\4\2\uffff"+
"\2\4\1\uffff\1\7\1\uffff\1\5\1\6\3\uffff\1\10\5\3\1\uffff\16"+
"\10\3\uffff\1\10\3\uffff\2\10",
"",
"\1\11",
"",
"",
"",
"",
"",
"",
"\1\3\5\uffff\1\3\2\uffff\1\12",
""
};
static final short[] DFA9_eot = DFA.unpackEncodedString(DFA9_eotS);
static final short[] DFA9_eof = DFA.unpackEncodedString(DFA9_eofS);
static final char[] DFA9_min = DFA.unpackEncodedStringToUnsignedChars(DFA9_minS);
static final char[] DFA9_max = DFA.unpackEncodedStringToUnsignedChars(DFA9_maxS);
static final short[] DFA9_accept = DFA.unpackEncodedString(DFA9_acceptS);
static final short[] DFA9_special = DFA.unpackEncodedString(DFA9_specialS);
static final short[][] DFA9_transition;
static {
int numStates = DFA9_transitionS.length;
DFA9_transition = new short[numStates][];
for (int i=0; i {$imageWrite.st} | scalarAssignment -> {$scalarAssignment.st} | listAssignment -> {$listAssignment.st} | loop -> {$loop.st} | ^( BREAKIF expression ) -> breakif(cond=$expression.st) | BREAK -> {%{\"break\"}} | ifCall -> {$ifCall.st} | expression -> {$expression.st});";
}
}
static final String DFA17_eotS =
"\21\uffff";
static final String DFA17_eofS =
"\21\uffff";
static final String DFA17_minS =
"\1\7\4\uffff\1\2\10\uffff\1\106\2\uffff";
static final String DFA17_maxS =
"\1\123\4\uffff\1\2\10\uffff\1\115\2\uffff";
static final String DFA17_acceptS =
"\1\uffff\1\1\1\2\1\3\1\4\1\uffff\1\7\1\10\1\11\1\12\1\13\1\14\1"+
"\15\1\16\1\uffff\1\5\1\6";
static final String DFA17_specialS =
"\21\uffff}>";
static final String[] DFA17_transitionS = {
"\1\2\1\uffff\1\11\1\uffff\1\1\1\3\1\uffff\1\7\1\uffff\1\6\1"+
"\5\3\uffff\1\12\1\13\1\14\3\uffff\4\12\27\uffff\1\10\6\uffff"+
"\16\4\3\uffff\1\4\3\uffff\2\15",
"",
"",
"",
"",
"\1\16",
"",
"",
"",
"",
"",
"",
"",
"",
"\2\20\3\uffff\1\17\2\20",
"",
""
};
static final short[] DFA17_eot = DFA.unpackEncodedString(DFA17_eotS);
static final short[] DFA17_eof = DFA.unpackEncodedString(DFA17_eofS);
static final char[] DFA17_min = DFA.unpackEncodedStringToUnsignedChars(DFA17_minS);
static final char[] DFA17_max = DFA.unpackEncodedStringToUnsignedChars(DFA17_maxS);
static final short[] DFA17_accept = DFA.unpackEncodedString(DFA17_acceptS);
static final short[] DFA17_special = DFA.unpackEncodedString(DFA17_specialS);
static final short[][] DFA17_transition;
static {
int numStates = DFA17_transitionS.length;
DFA17_transition = new short[numStates][];
for (int i=0; i call(name=getRuntimeExpr($ID.text, $el.argTypes)args=$el.templates) | ^( CON_CALL el= expressionList ) -> concall(args=$el.templates) | imagePos -> {$imagePos.st} | binaryExpression -> {$binaryExpression.st} | ^( PREFIX NOT e= expression ) -> call(name=getRuntimeExpr(\"NOT\", \"D\")args=$e.st) | ^( PREFIX prefixOp e= expression ) -> preop(op=$prefixOp.stexpr=$e.st) | ^( POSTFIX postfixOp e= expression ) -> postop(op=$postfixOp.stexpr=$e.st) | ^( PAR e= expression ) -> par(expr=$e.st) | listOperation -> {$listOperation.st} | listLiteral -> {$listLiteral.st} | var -> {$var.st} | VAR_SOURCE -> getsourcevalue(var=$VAR_SOURCE.text) | CONSTANT -> {%{getConstantString($CONSTANT.text)}} | literal -> {$literal.st});";
}
}
public static final BitSet FOLLOW_jiffleOption_in_generate107 = new BitSet(new long[]{0xEFC6B20879E37BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_varDeclaration_in_generate112 = new BitSet(new long[]{0xEFC6B20879E35BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_generate117 = new BitSet(new long[]{0xEFC6B20879E35BC2L,0x00000000000C47FFL});
public static final BitSet FOLLOW_JIFFLE_OPTION_in_jiffleOption264 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_jiffleOption266 = new BitSet(new long[]{0xEFC6B20C79E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_optionValue_in_jiffleOption268 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ID_in_optionValue335 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_optionValue357 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONSTANT_in_optionValue379 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DECL_in_varDeclaration409 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_DEST_in_varDeclaration411 = new BitSet(new long[]{0x0000000400000000L});
public static final BitSet FOLLOW_ID_in_varDeclaration413 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECL_in_varDeclaration435 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_SOURCE_in_varDeclaration437 = new BitSet(new long[]{0x0000000400000000L});
public static final BitSet FOLLOW_ID_in_varDeclaration439 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECL_in_varDeclaration461 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_IMAGE_SCOPE_in_varDeclaration463 = new BitSet(new long[]{0xEFC6B20879E35A88L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_varDeclaration467 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BLOCK_in_block580 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_statement_in_block584 = new BitSet(new long[]{0xEFC6B20879E35BC8L,0x00000000000C47FFL});
public static final BitSet FOLLOW_simpleStatement_in_statement643 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_block_in_statement672 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_imageWrite_in_simpleStatement702 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_scalarAssignment_in_simpleStatement726 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listAssignment_in_simpleStatement750 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_loop_in_simpleStatement774 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_BREAKIF_in_simpleStatement799 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_simpleStatement801 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BREAK_in_simpleStatement831 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_ifCall_in_simpleStatement855 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_expression_in_simpleStatement879 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IMAGE_WRITE_in_imageWrite915 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_DEST_in_imageWrite917 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_imageWrite919 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_EXPR_LIST_in_expressionList1002 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expressionList1005 = new BitSet(new long[]{0xEFC6B20879E35A88L,0x00000000000C47FFL});
public static final BitSet FOLLOW_EQ_in_scalarAssignment1075 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_scalar_in_scalarAssignment1077 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_scalarAssignment1079 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_compoundAssignmentOp_in_scalarAssignment1138 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_scalar_in_scalarAssignment1140 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_scalarAssignment1142 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_set_in_compoundAssignmentOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_EQ_in_listAssignment1364 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_LIST_in_listAssignment1366 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_listAssignment1370 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_VAR_IMAGE_SCOPE_in_scalar1482 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_PIXEL_SCOPE_in_scalar1503 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_conditionalLoop_in_loop1559 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_foreachLoop_in_loop1583 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WHILE_in_conditionalLoop1630 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_conditionalLoop1634 = new BitSet(new long[]{0xEFC6B20879E35BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_conditionalLoop1638 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_UNTIL_in_conditionalLoop1674 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_conditionalLoop1678 = new BitSet(new long[]{0xEFC6B20879E35BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_conditionalLoop1682 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FOREACH_in_foreachLoop1749 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_foreachLoop1751 = new BitSet(new long[]{0x0000000000000200L});
public static final BitSet FOLLOW_DECLARED_LIST_in_foreachLoop1797 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expressionList_in_foreachLoop1801 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_statement_in_foreachLoop1806 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FOREACH_in_foreachLoop1870 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_foreachLoop1872 = new BitSet(new long[]{0x0000000040000000L});
public static final BitSet FOLLOW_VAR_LIST_in_foreachLoop1917 = new BitSet(new long[]{0xEFC6B20879E35BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_foreachLoop1921 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FOREACH_in_foreachLoop2019 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_foreachLoop2021 = new BitSet(new long[]{0x0000000000080000L});
public static final BitSet FOLLOW_SEQUENCE_in_foreachLoop2067 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_foreachLoop2071 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_foreachLoop2075 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_statement_in_foreachLoop2080 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_IF_in_ifCall2163 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_ifCall2165 = new BitSet(new long[]{0xEFC6B20879E35BC0L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_ifCall2169 = new BitSet(new long[]{0xEFC6B20879E35BC8L,0x00000000000C47FFL});
public static final BitSet FOLLOW_statement_in_ifCall2173 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_FUNC_CALL_in_expression2274 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_ID_in_expression2276 = new BitSet(new long[]{0x0000000000000400L});
public static final BitSet FOLLOW_expressionList_in_expression2280 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_CON_CALL_in_expression2334 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expressionList_in_expression2338 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_imagePos_in_expression2369 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_binaryExpression_in_expression2394 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_PREFIX_in_expression2420 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_NOT_in_expression2422 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_expression2426 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PREFIX_in_expression2480 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_prefixOp_in_expression2482 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_expression2486 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_POSTFIX_in_expression2523 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_postfixOp_in_expression2525 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_expression2529 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PAR_in_expression2566 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_expression2570 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_listOperation_in_expression2601 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_listLiteral_in_expression2626 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_var_in_expression2651 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_SOURCE_in_expression2676 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CONSTANT_in_expression2706 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_literal_in_expression2731 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_APPEND_in_listOperation2764 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_LIST_in_listOperation2766 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_listOperation2768 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_DECLARED_LIST_in_listLiteral2831 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expressionList_in_listLiteral2835 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_VAR_IMAGE_SCOPE_in_var2923 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_PIXEL_SCOPE_in_var2947 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_PROVIDED_in_var2971 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_LOOP_in_var2995 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VAR_LIST_in_var3019 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_POW_in_binaryExpression3070 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3074 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3078 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_OR_in_binaryExpression3115 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3119 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3123 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_XOR_in_binaryExpression3179 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3183 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3187 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_AND_in_binaryExpression3243 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3247 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3251 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_LOGICALEQ_in_binaryExpression3307 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3311 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3315 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_NE_in_binaryExpression3371 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3375 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3379 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_GT_in_binaryExpression3435 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3439 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3443 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_GE_in_binaryExpression3499 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3503 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3507 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_LT_in_binaryExpression3563 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3567 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3571 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_LE_in_binaryExpression3627 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3631 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3635 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_arithmeticOp_in_binaryExpression3691 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_binaryExpression3695 = new BitSet(new long[]{0xEFC6B20879E35A80L,0x00000000000C47FFL});
public static final BitSet FOLLOW_expression_in_binaryExpression3699 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_set_in_arithmeticOp0 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INT_LITERAL_in_literal3897 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FLOAT_LITERAL_in_literal3921 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IMAGE_POS_in_imagePos3959 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_VAR_SOURCE_in_imagePos3961 = new BitSet(new long[]{0x0000000000008028L});
public static final BitSet FOLLOW_bandSpecifier_in_imagePos3965 = new BitSet(new long[]{0x0000000000008008L});
public static final BitSet FOLLOW_pixelSpecifier_in_imagePos3970 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_BAND_REF_in_bandSpecifier4036 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_bandSpecifier4038 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PIXEL_REF_in_pixelSpecifier4071 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier4075 = new BitSet(new long[]{0x0000000000040010L});
public static final BitSet FOLLOW_pixelPos_in_pixelSpecifier4080 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_ABS_POS_in_pixelPos4140 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_pixelPos4142 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_REL_POS_in_pixelPos4168 = new BitSet(new long[]{0x0000000000000004L});
public static final BitSet FOLLOW_expression_in_pixelPos4170 = new BitSet(new long[]{0x0000000000000008L});
public static final BitSet FOLLOW_PLUS_in_prefixOp4223 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_MINUS_in_prefixOp4247 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_incdecOp_in_prefixOp4271 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_incdecOp_in_postfixOp4308 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INCR_in_incdecOp4345 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DECR_in_incdecOp4369 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy