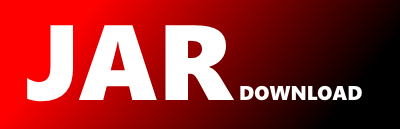
org.jamesii.mlrules.observation.Observer Maven / Gradle / Ivy
/*
* Copyright 2015 University of Rostock
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jamesii.mlrules.observation;
import org.jamesii.mlrules.simulator.Simulator;
import java.util.HashSet;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
/**
* An observer observes the simulator, collects data and informs listener if
* necessary. The observer must decide when to extract data (e.g., depending on
* the number of steps) and how to format data (e.g., aggregate and filter
* species). The observer is not doing something with the data (e.g., save the
* data). This is the task of a listener.
*
* @author Tobias Helms
*
*/
public abstract class Observer {
private Set listeners = new HashSet<>();
public void register(Listener l) {
listeners.add(l);
}
public boolean remove(Listener l) {
return listeners.remove(l);
}
/**
* Notify all listener about the current observation. Remove all inactive
* listener from the listener set.
*/
public void notifyListener() {
removeInactiveListeners();
listeners.forEach(l -> l.notify(this));
}
/**
* Removes all inactive listeners from the listener set.
*/
private void removeInactiveListeners(){
this.listeners = this.listeners.stream().filter( l -> l.isActive()).collect(Collectors.toSet());
}
/**
* Extract data from the simulator to update the current observation data.
*/
public abstract void update(Simulator simulator);
/**
* If available, return the simulation time of the next observation.
*/
public abstract Optional nextObservationPoint();
public Set getListener() {
return listeners;
}
public boolean hasListener() {
return !listeners.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy