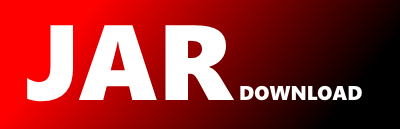
org.jamesii.mlrules.observation.visualization.BufferedSimulationDataHandler Maven / Gradle / Ivy
package org.jamesii.mlrules.observation.visualization;
import org.jamesii.mlrules.model.species.Species;
import java.io.*;
import java.util.*;
/**
* Created by Florian Thonig on 31.01.2017.
*
* This Class is for a "performant" use of a temporary file but it is not used so far
*/
public class BufferedSimulationDataHandler extends ASimulationDataHandler {
private File tmpFile;
private ObjectOutputStream oout;
private FileOutputStream fout;
private ObjectInputStream oin;
private FileInputStream fin;
private Vector bout;
private Thread outputBufferThread;
public BufferedSimulationDataHandler() {
super();
try {
// get temporarary file
tmpFile = File.createTempFile("sim_data", null);
fout = new FileOutputStream(tmpFile);
oout = new ObjectOutputStream(fout);
// setting up buffers
bout = new Vector<>(1000);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Addind data as Simulation point to the DataHandler
*
* @param time
* @param root
*/
@Override
public void addData(double time, Species root) {
setNumSimPoints(getNumSimPoints() + 1);
bout.add(new SimulationPoint(time, root.copy()));
if (getNumSimPoints()%100 == 0) {
try {
for (SimulationPoint i : bout)
oout.writeObject(i);
bout.clear();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* function to get all Data
*
* @return Simulation point
*/
public SimulationPoint get_next() {
try {
if (fin == null)
fin = new FileInputStream(tmpFile);
if (oin == null)
oin = new ObjectInputStream(fin);
return (SimulationPoint) oin.readObject();
} catch (EOFException e) {
return null;
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* function to reset the file input
*/
@Override
public void reset() {
try {
if (fin != null)
fin.close();
fin = new FileInputStream(tmpFile);
if (oin != null)
oin.close();
oin = new ObjectInputStream(fin);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* function called, when simulation is over and all data is collected
*/
@Override
public void finished() {
try {
for (SimulationPoint i : bout)
oout.writeObject(i);
bout.clear();
oout.close();
fout.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* function called when simulation is closed, to remove the temporary file
*/
@Override
public void clean() {
try {
if (fin != null)
fin.close();
if (oin != null)
oin.close();
} catch (IOException e) {
e.printStackTrace();
}
tmpFile.delete();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy