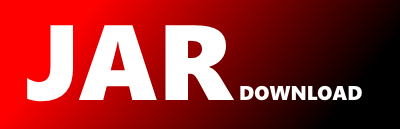
org.jamesii.mlrules.parser.nodes.FunctionCallNode Maven / Gradle / Ivy
/*
* Copyright 2015 University of Rostock
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jamesii.mlrules.parser.nodes;
import org.jamesii.core.math.parsetree.INode;
import org.jamesii.core.math.parsetree.Node;
import org.jamesii.core.math.parsetree.ValueNode;
import org.jamesii.core.math.parsetree.variables.IEnvironment;
import org.jamesii.mlrules.parser.functions.Function;
import org.jamesii.mlrules.parser.functions.FunctionDefinition;
import org.jamesii.mlrules.parser.types.Tuple;
import org.jamesii.mlrules.util.Assignment;
import org.jamesii.mlrules.util.LazyInitialization;
import org.jamesii.mlrules.util.MLEnvironment;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* The function call node takes a set of arguments and a function node and
* calculates the values of the arguments and decides which arguments match
* which function alternative. Function alternatives are processed top-down.
*
* @author Tobias Helms
*
*/
public class FunctionCallNode extends Node {
private static final long serialVersionUID = 1L;
private Node functionNode;
private final List arguments;
private FunctionDefinition matched(Function usedFunction, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy