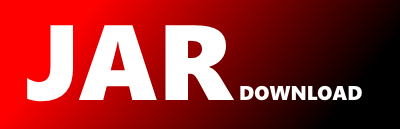
org.jamesii.mlrules.model.species.LeafSpecies Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mlrules Show documentation
Show all versions of mlrules Show documentation
The main project for the external domain-specific modeling
language ML-Rules, which is used to model hierarchical biochemical
reaction networks with nested and attributed species
/*
* Copyright 2015 University of Rostock
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jamesii.mlrules.model.species;
import java.io.Serializable;
/**
* A {@link LeafSpecies} is a species that cannot contain other species, i.e.,
* it is always a leaf is a species tree. {@link LeafSpecies} are treated
* population-based.
*
* @author Tobias Helms
*
*/
public class LeafSpecies extends Species implements Serializable {
/**
* The amount of a species saved as a floating point number. Floating point
* numbers are needed in case a simulation is computed deterministically with
* numerical integration methods.
*/
private double amount;
public LeafSpecies(SpeciesType type, Object[] attributes, Compartment context, double amount) {
super(type, attributes, context);
this.amount = amount;
}
@Override
public double getAmount() {
return amount;
}
private void checkAmount(double amount) {
if (amount < 0) {
throw new IllegalArgumentException(
String.format("Negative amount %s for %s is not allowed.", amount, toString()));
}
}
public void setAmount(double amount) {
checkAmount(amount);
this.amount = amount;
}
public void changeAmount(double change) {
double result = amount + change;
checkAmount(result);
this.amount = result;
}
@Override
public Species copy() {
Object[] attributes = new Object[getType().getAttributesSize()];
for (int i = 0; i < getType().getAttributesSize(); ++i) {
attributes[i] = getAttribute(i);
}
Species result = new LeafSpecies(getType(), attributes, getContext(), amount);
return result;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof LeafSpecies) {
LeafSpecies s = (LeafSpecies) o;
if (!s.getType().equals(getType())) {
return false;
}
for (int i = 0; i < getType().getAttributesSize(); ++i) {
if (!getAttribute(i).equals(s.getAttribute(i))) {
return false;
}
}
return true;
}
return false;
}
@Override
public String toString() {
return amount + " " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy