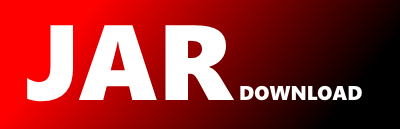
org.janusgraph.diskstorage.solr.SolrIndex Maven / Gradle / Ivy
// Copyright 2017 JanusGraph Authors
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package org.janusgraph.diskstorage.solr;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import org.apache.commons.lang3.StringUtils;
import org.apache.http.HttpEntity;
import org.apache.http.HttpEntityEnclosingRequest;
import org.apache.http.HttpRequestInterceptor;
import org.apache.http.client.HttpClient;
import org.apache.http.entity.BufferedHttpEntity;
import org.apache.http.impl.auth.KerberosScheme;
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.CachingTokenFilter;
import org.apache.lucene.analysis.Tokenizer;
import org.apache.lucene.analysis.tokenattributes.OffsetAttribute;
import org.apache.lucene.analysis.tokenattributes.TermToBytesRefAttribute;
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.SolrQuery;
import org.apache.solr.client.solrj.SolrServerException;
import org.apache.solr.client.solrj.impl.CloudSolrClient;
import org.apache.solr.client.solrj.impl.HttpClientUtil;
import org.apache.solr.client.solrj.impl.HttpSolrClient;
import org.apache.solr.client.solrj.impl.Krb5HttpClientBuilder;
import org.apache.solr.client.solrj.impl.LBHttpSolrClient;
import org.apache.solr.client.solrj.impl.PreemptiveAuth;
import org.apache.solr.client.solrj.impl.SolrHttpClientBuilder;
import org.apache.solr.client.solrj.request.CollectionAdminRequest;
import org.apache.solr.client.solrj.request.UpdateRequest;
import org.apache.solr.client.solrj.response.CollectionAdminResponse;
import org.apache.solr.client.solrj.response.QueryResponse;
import org.apache.solr.client.solrj.util.ClientUtils;
import org.apache.solr.common.SolrDocument;
import org.apache.solr.common.SolrInputDocument;
import org.apache.solr.common.cloud.ClusterState;
import org.apache.solr.common.cloud.DocCollection;
import org.apache.solr.common.cloud.Replica;
import org.apache.solr.common.cloud.Slice;
import org.apache.solr.common.cloud.ZkStateReader;
import org.apache.solr.common.params.CommonParams;
import org.apache.solr.common.params.ModifiableSolrParams;
import org.apache.zookeeper.KeeperException;
import org.janusgraph.core.Cardinality;
import org.janusgraph.core.JanusGraphElement;
import org.janusgraph.core.attribute.Cmp;
import org.janusgraph.core.attribute.Geo;
import org.janusgraph.core.attribute.Geoshape;
import org.janusgraph.core.attribute.Text;
import org.janusgraph.core.schema.Mapping;
import org.janusgraph.core.schema.Parameter;
import org.janusgraph.diskstorage.BackendException;
import org.janusgraph.diskstorage.BaseTransaction;
import org.janusgraph.diskstorage.BaseTransactionConfig;
import org.janusgraph.diskstorage.BaseTransactionConfigurable;
import org.janusgraph.diskstorage.PermanentBackendException;
import org.janusgraph.diskstorage.TemporaryBackendException;
import org.janusgraph.diskstorage.configuration.ConfigNamespace;
import org.janusgraph.diskstorage.configuration.ConfigOption;
import org.janusgraph.diskstorage.configuration.Configuration;
import org.janusgraph.diskstorage.indexing.IndexEntry;
import org.janusgraph.diskstorage.indexing.IndexFeatures;
import org.janusgraph.diskstorage.indexing.IndexMutation;
import org.janusgraph.diskstorage.indexing.IndexProvider;
import org.janusgraph.diskstorage.indexing.IndexQuery;
import org.janusgraph.diskstorage.indexing.KeyInformation;
import org.janusgraph.diskstorage.indexing.RawQuery;
import org.janusgraph.diskstorage.solr.transform.GeoToWktConverter;
import org.janusgraph.diskstorage.util.DefaultTransaction;
import org.janusgraph.graphdb.configuration.PreInitializeConfigOptions;
import org.janusgraph.graphdb.database.serialize.AttributeUtils;
import org.janusgraph.graphdb.internal.Order;
import org.janusgraph.graphdb.query.JanusGraphPredicate;
import org.janusgraph.graphdb.query.QueryUtil;
import org.janusgraph.graphdb.query.condition.And;
import org.janusgraph.graphdb.query.condition.Condition;
import org.janusgraph.graphdb.query.condition.Not;
import org.janusgraph.graphdb.query.condition.Or;
import org.janusgraph.graphdb.query.condition.PredicateCondition;
import org.janusgraph.graphdb.tinkerpop.optimize.step.Aggregation;
import org.janusgraph.graphdb.types.ParameterType;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.lang.reflect.Constructor;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.time.Instant;
import java.util.AbstractMap.SimpleEntry;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.TimeZone;
import java.util.UUID;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import static org.janusgraph.graphdb.configuration.GraphDatabaseConfiguration.INDEX_MAX_RESULT_SET_SIZE;
import static org.janusgraph.graphdb.configuration.GraphDatabaseConfiguration.INDEX_NS;
/**
* @author Jared Holmberg ([email protected]), Pavel Yaskevich ([email protected])
*/
@PreInitializeConfigOptions
public class SolrIndex implements IndexProvider {
private static final Logger logger = LoggerFactory.getLogger(SolrIndex.class);
private static final String DEFAULT_ID_FIELD = "id";
private static final String TEXT_SUFFIX = "_t";
private static final String TEXT_SUFFIX_MULTI = "_txt";
private static final String STRING_SUFFIX = "_s";
private static final String STRING_SUFFIX_MULTI = "_ss";
private enum Mode {
HTTP, CLOUD;
public static Mode parse(String mode) {
for (final Mode m : Mode.values()) {
if (m.toString().equalsIgnoreCase(mode)) return m;
}
throw new IllegalArgumentException("Unrecognized mode: "+mode);
}
}
public static final ConfigNamespace SOLR_NS =
new ConfigNamespace(INDEX_NS, "solr", "Solr index configuration");
public static final ConfigOption SOLR_MODE = new ConfigOption<>(SOLR_NS,"mode",
"The operation mode for Solr which is either via HTTP (`http`) or using SolrCloud (`cloud`)",
ConfigOption.Type.GLOBAL_OFFLINE, "cloud");
public static final ConfigOption DYNAMIC_FIELDS = new ConfigOption<>(SOLR_NS,"dyn-fields",
"Whether to use dynamic fields (which appends the data type to the field name). If dynamic fields is disabled, " +
"the user must map field names and define them explicitly in the schema.",
ConfigOption.Type.GLOBAL_OFFLINE, true);
public static final ConfigOption KEY_FIELD_NAMES = new ConfigOption<>(SOLR_NS,"key-field-names",
"Field name that uniquely identifies each document in Solr. Must be specified as a list of `collection=field`.",
ConfigOption.Type.GLOBAL, String[].class);
public static final ConfigOption TTL_FIELD = new ConfigOption<>(SOLR_NS,"ttl_field",
"Name of the TTL field for Solr collections.",
ConfigOption.Type.GLOBAL_OFFLINE, "ttl");
/** SolrCloud Configuration */
/*
* TODO Rename ZOOKEEPER_URL and "zookeeper-url" to ZOOKEEPER_URLS and
* "zookeeper-urls" in future major releases.
*/
public static final ConfigOption ZOOKEEPER_URL = new ConfigOption<>(SOLR_NS, "zookeeper-url",
"URL of the Zookeeper instance coordinating the SolrCloud cluster",
ConfigOption.Type.MASKABLE, new String[] { "localhost:2181" });
public static final ConfigOption NUM_SHARDS = new ConfigOption<>(SOLR_NS,"num-shards",
"Number of shards for a collection. This applies when creating a new collection which is only supported under the SolrCloud operation mode.",
ConfigOption.Type.GLOBAL_OFFLINE, 1);
public static final ConfigOption MAX_SHARDS_PER_NODE = new ConfigOption<>(SOLR_NS,"max-shards-per-node",
"Maximum number of shards per node. This applies when creating a new collection which is only supported under the SolrCloud operation mode.",
ConfigOption.Type.GLOBAL_OFFLINE, 1);
public static final ConfigOption REPLICATION_FACTOR = new ConfigOption<>(SOLR_NS,"replication-factor",
"Replication factor for a collection. This applies when creating a new collection which is only supported under the SolrCloud operation mode.",
ConfigOption.Type.GLOBAL_OFFLINE, 1);
public static final ConfigOption SOLR_DEFAULT_CONFIG = new ConfigOption<>(SOLR_NS,"configset",
"If specified, the same solr configSet can be reused for each new Collection that is created in SolrCloud.",
ConfigOption.Type.MASKABLE, String.class);
/** HTTP Configuration */
public static final ConfigOption HTTP_URLS = new ConfigOption<>(SOLR_NS,"http-urls",
"List of URLs to use to connect to Solr Servers (LBHttpSolrClient is used), don't add core or collection name to the URL.",
ConfigOption.Type.MASKABLE, new String[] { "http://localhost:8983/solr" });
public static final ConfigOption HTTP_CONNECTION_TIMEOUT = new ConfigOption<>(SOLR_NS,"http-connection-timeout",
"Solr HTTP connection timeout.",
ConfigOption.Type.MASKABLE, 5000);
public static final ConfigOption HTTP_ALLOW_COMPRESSION = new ConfigOption<>(SOLR_NS,"http-compression",
"Enable/disable compression on the HTTP connections made to Solr.",
ConfigOption.Type.MASKABLE, false);
public static final ConfigOption HTTP_MAX_CONNECTIONS_PER_HOST = new ConfigOption<>(SOLR_NS,"http-max-per-host",
"Maximum number of HTTP connections per Solr host.",
ConfigOption.Type.MASKABLE, 20);
public static final ConfigOption HTTP_GLOBAL_MAX_CONNECTIONS = new ConfigOption<>(SOLR_NS,"http-max",
"Maximum number of HTTP connections in total to all Solr servers.",
ConfigOption.Type.MASKABLE, 100);
public static final ConfigOption WAIT_SEARCHER = new ConfigOption<>(SOLR_NS, "wait-searcher",
"When mutating - wait for the index to reflect new mutations before returning. This can have a negative impact on performance.",
ConfigOption.Type.LOCAL, false);
/** Security Configuration */
public static final ConfigOption KERBEROS_ENABLED = new ConfigOption<>(SOLR_NS, "kerberos-enabled",
"Whether SOLR instance is Kerberized or not.",
ConfigOption.Type.MASKABLE, false);
private static final IndexFeatures SOLR_FEATURES = new IndexFeatures.Builder()
.supportsDocumentTTL()
.setDefaultStringMapping(Mapping.TEXT)
.supportedStringMappings(Mapping.TEXT, Mapping.STRING, Mapping.TEXTSTRING)
.supportsCardinality(Cardinality.SINGLE)
.supportsCardinality(Cardinality.LIST)
.supportsCardinality(Cardinality.SET)
.supportsCustomAnalyzer()
.supportsGeoContains()
.build();
private static final Map SPATIAL_PREDICATES = spatialPredicates();
private final SolrClient solrClient;
private final Configuration configuration;
private final Mode mode;
private final boolean dynFields;
private final Map keyFieldIds;
private final String ttlField;
private final int batchSize;
private final boolean waitSearcher;
private final boolean kerberosEnabled;
public SolrIndex(final Configuration config) throws BackendException {
Preconditions.checkArgument(config!=null);
configuration = config;
mode = Mode.parse(config.get(SOLR_MODE));
kerberosEnabled = config.get(KERBEROS_ENABLED);
dynFields = config.get(DYNAMIC_FIELDS);
keyFieldIds = parseKeyFieldsForCollections(config);
batchSize = config.get(INDEX_MAX_RESULT_SET_SIZE);
ttlField = config.get(TTL_FIELD);
waitSearcher = config.get(WAIT_SEARCHER);
if (kerberosEnabled) {
logger.debug("Kerberos is enabled. Configuring SOLR for Kerberos.");
configureSolrClientsForKerberos();
} else {
logger.debug("Kerberos is NOT enabled.");
logger.debug("KERBEROS_ENABLED name is " + KERBEROS_ENABLED.getName() + " and it is" + (KERBEROS_ENABLED.isOption() ? " " : " not") + " an option.");
logger.debug("KERBEROS_ENABLED type is " + KERBEROS_ENABLED.getType().name());
}
final ModifiableSolrParams clientParams = new ModifiableSolrParams();
switch (mode) {
case CLOUD:
final String[] zookeeperUrl = config.get(SolrIndex.ZOOKEEPER_URL);
// Process possible zookeeper chroot. e.g. localhost:2181/solr
// chroot has to be the same assuming one Zookeeper ensemble.
// Parse from the last string. If found, take it as the chroot.
Optional chroot = Optional.empty();
for (int i = zookeeperUrl.length - 1; i >= 0; i--) {
int chrootIndex = zookeeperUrl[i].indexOf("/");
if (chrootIndex != -1) {
String hostAndPort = zookeeperUrl[i].substring(0, chrootIndex);
if (!chroot.isPresent()) {
chroot = Optional.of(zookeeperUrl[i].substring(chrootIndex));
}
zookeeperUrl[i] = hostAndPort;
}
}
final CloudSolrClient.Builder builder = new CloudSolrClient
.Builder(Arrays.asList(zookeeperUrl), chroot)
.withLBHttpSolrClientBuilder(
new LBHttpSolrClient.Builder()
.withHttpSolrClientBuilder(new HttpSolrClient.Builder().withInvariantParams(clientParams))
.withBaseSolrUrls(config.get(HTTP_URLS))
)
.sendUpdatesOnlyToShardLeaders();
final CloudSolrClient cloudServer = builder.build();
cloudServer.connect();
solrClient = cloudServer;
break;
case HTTP:
clientParams.add(HttpClientUtil.PROP_ALLOW_COMPRESSION, config.get(HTTP_ALLOW_COMPRESSION).toString());
clientParams.add(HttpClientUtil.PROP_CONNECTION_TIMEOUT, config.get(HTTP_CONNECTION_TIMEOUT).toString());
clientParams.add(HttpClientUtil.PROP_MAX_CONNECTIONS_PER_HOST, config.get(HTTP_MAX_CONNECTIONS_PER_HOST).toString());
clientParams.add(HttpClientUtil.PROP_MAX_CONNECTIONS, config.get(HTTP_GLOBAL_MAX_CONNECTIONS).toString());
final HttpClient client = HttpClientUtil.createClient(clientParams);
solrClient = new LBHttpSolrClient.Builder()
.withHttpClient(client)
.withBaseSolrUrls(config.get(HTTP_URLS))
.build();
break;
default:
throw new IllegalArgumentException("Unsupported Solr operation mode: " + mode);
}
}
private void configureSolrClientsForKerberos() throws PermanentBackendException {
String kerberosConfig = System.getProperty("java.security.auth.login.config");
if(kerberosConfig == null) {
throw new PermanentBackendException("Unable to configure kerberos for solr client. System property 'java.security.auth.login.config' is not set.");
}
logger.debug("Using kerberos configuration file located at '{}'.", kerberosConfig);
try(Krb5HttpClientBuilder krbBuild = new Krb5HttpClientBuilder()) {
SolrHttpClientBuilder kb = krbBuild.getBuilder();
HttpClientUtil.setHttpClientBuilder(kb);
HttpRequestInterceptor bufferedEntityInterceptor = (request, context) -> {
if(request instanceof HttpEntityEnclosingRequest) {
HttpEntityEnclosingRequest enclosingRequest = ((HttpEntityEnclosingRequest) request);
HttpEntity requestEntity = enclosingRequest.getEntity();
enclosingRequest.setEntity(new BufferedHttpEntity(requestEntity));
}
};
HttpClientUtil.addRequestInterceptor(bufferedEntityInterceptor);
HttpRequestInterceptor preemptiveAuth = new PreemptiveAuth(new KerberosScheme());
HttpClientUtil.addRequestInterceptor(preemptiveAuth);
}
}
private Map parseKeyFieldsForCollections(Configuration config) throws BackendException {
final Map keyFieldNames = new HashMap<>();
final String[] collectionFieldStatements = config.has(KEY_FIELD_NAMES) ? config.get(KEY_FIELD_NAMES) : new String[0];
for (final String collectionFieldStatement : collectionFieldStatements) {
final String[] parts = collectionFieldStatement.trim().split("=");
if (parts.length != 2) {
throw new PermanentBackendException(
"Unable to parse the collection name / key field name pair. It should be of the format collection=field");
}
final String collectionName = parts[0];
final String keyFieldName = parts[1];
keyFieldNames.put(collectionName, keyFieldName);
}
return keyFieldNames;
}
private String getKeyFieldId(String collection) {
String field = keyFieldIds.get(collection);
if (field==null) field = DEFAULT_ID_FIELD;
return field;
}
/**
* Unlike the ElasticSearch Index, which is schema free, Solr requires a schema to
* support searching. This means that you will need to modify the solr schema with the
* appropriate field definitions in order to work properly. If you have a running instance
* of Solr and you modify its schema with new fields, don't forget to re-index!
* @param store Index store
* @param key New key to register
* @param information data type to register for the key
* @param tx enclosing transaction
* @throws org.janusgraph.diskstorage.BackendException in case an exception is thrown when
* creating a collection.
*/
@SuppressWarnings("unchecked")
@Override
public void register(String store, String key, KeyInformation information, BaseTransaction tx)
throws BackendException {
if (mode==Mode.CLOUD) {
final CloudSolrClient client = (CloudSolrClient) solrClient;
try {
createCollectionIfNotExists(client, configuration, store);
} catch (final IOException | SolrServerException | InterruptedException | KeeperException e) {
throw new PermanentBackendException(e);
}
}
//Since all data types must be defined in the schema.xml, pre-registering a type does not work
//But we check Analyse feature
String analyzer = ParameterType.STRING_ANALYZER.findParameter(information.getParameters(), null);
if (analyzer != null) {
//If the key have a tokenizer, we try to get it by reflection
try {
((Constructor) ClassLoader.getSystemClassLoader().loadClass(analyzer)
.getConstructor()).newInstance();
} catch (final ReflectiveOperationException e) {
throw new PermanentBackendException(e.getMessage(),e);
}
}
analyzer = ParameterType.TEXT_ANALYZER.findParameter(information.getParameters(), null);
if (analyzer != null) {
//If the key have a tokenizer, we try to get it by reflection
try {
((Constructor) ClassLoader.getSystemClassLoader().loadClass(analyzer)
.getConstructor()).newInstance();
} catch (final ReflectiveOperationException e) {
throw new PermanentBackendException(e.getMessage(),e);
}
}
}
@Override
public void mutate(Map> mutations, KeyInformation.IndexRetriever information,
BaseTransaction tx) throws BackendException {
logger.debug("Mutating SOLR");
try {
for (final Map.Entry> stores : mutations.entrySet()) {
final String collectionName = stores.getKey();
final String keyIdField = getKeyFieldId(collectionName);
final List deleteIds = new ArrayList<>();
final Collection changes = new ArrayList<>();
for (final Map.Entry entry : stores.getValue().entrySet()) {
final String docId = entry.getKey();
final IndexMutation mutation = entry.getValue();
Preconditions.checkArgument(!(mutation.isNew() && mutation.isDeleted()));
Preconditions.checkArgument(!mutation.isNew() || !mutation.hasDeletions());
Preconditions.checkArgument(!mutation.isDeleted() || !mutation.hasAdditions());
//Handle any deletions
if (mutation.hasDeletions()) {
if (mutation.isDeleted()) {
logger.trace("Deleting entire document {}", docId);
deleteIds.add(docId);
} else {
final List fieldDeletions = new ArrayList<>(mutation.getDeletions());
if (mutation.hasAdditions()) {
for (final IndexEntry indexEntry : mutation.getAdditions()) {
fieldDeletions.remove(indexEntry);
}
}
handleRemovalsFromIndex(collectionName, keyIdField, docId, fieldDeletions, information);
}
}
if (mutation.hasAdditions()) {
final int ttl = mutation.determineTTL();
final SolrInputDocument doc = new SolrInputDocument();
doc.setField(keyIdField, docId);
final boolean isNewDoc = mutation.isNew();
if (isNewDoc)
logger.trace("Adding new document {}", docId);
final Map adds = collectFieldValues(mutation.getAdditions(), collectionName,
information);
// If cardinality is not single then we should use the "add" operation to update
// the index so we don't overwrite existing values.
adds.forEach((k, v) -> {
final KeyInformation keyInformation = information.get(collectionName, k);
final String solrOp = keyInformation.getCardinality() == Cardinality.SINGLE ? "set" : "add";
doc.setField(k, isNewDoc ? v : Collections.singletonMap(solrOp, v));
getDualFieldName(k, keyInformation)
.ifPresent(dk -> doc.setField(dk, isNewDoc ? v : Collections.singletonMap(solrOp, v)));
});
if (ttl>0) {
Preconditions.checkArgument(isNewDoc,
"Solr only supports TTL on new documents [%s]", docId);
doc.setField(ttlField, String.format("+%dSECONDS", ttl));
}
changes.add(doc);
}
}
commitDeletes(collectionName, deleteIds);
commitChanges(collectionName, changes);
}
} catch (final IllegalArgumentException e) {
throw new PermanentBackendException("Unable to complete query on Solr.", e);
} catch (final Exception e) {
throw storageException(e);
}
}
private void handleRemovalsFromIndex(String collectionName, String keyIdField, String docId,
List fieldDeletions,
KeyInformation.IndexRetriever information) throws SolrServerException, IOException, BackendException {
final SolrInputDocument doc = new SolrInputDocument();
doc.addField(keyIdField, docId);
for (final IndexEntry indexEntry : fieldDeletions) {
final KeyInformation keyInformation = information.get(collectionName, indexEntry.field);
// If the cardinality is a Set or List, we just need to remove the individual value
// received in the mutation and not set the field to null, but we still consolidate the values
// in the event of multiple removals in one mutation.
final Map deletes = collectFieldValues(fieldDeletions, collectionName, information);
deletes.forEach((k, v) -> {
if (keyInformation.getCardinality() == Cardinality.SINGLE) {
doc.setField(k, Collections.singletonMap("set", null));
getDualFieldName(k, keyInformation)
.ifPresent(dk -> doc.setField(dk, Collections.singletonMap("set", null)));
} else {
doc.setField(k, Collections.singletonMap("remove", v));
getDualFieldName(k, keyInformation)
.ifPresent(dk -> doc.setField(dk, Collections.singletonMap("remove", v)));
}
});
}
final UpdateRequest singleDocument = newUpdateRequest();
singleDocument.add(doc);
solrClient.request(singleDocument, collectionName);
}
private Object convertValue(Object value) throws BackendException {
if (value instanceof Geoshape) {
return GeoToWktConverter.convertToWktString((Geoshape) value);
}
if (value instanceof UUID) {
return value.toString();
}
if(value instanceof Instant) {
if(Math.floorMod(((Instant) value).getNano(), 1000000) != 0) {
throw new IllegalArgumentException("Solr indexes do not support nanoseconds");
}
return new Date(((Instant) value).toEpochMilli());
}
return value;
}
@Override
public void restore(Map>> documents,
KeyInformation.IndexRetriever information, BaseTransaction tx) throws BackendException {
try {
for (final Map.Entry>> stores : documents.entrySet()) {
final String collectionName = stores.getKey();
final List deleteIds = new ArrayList<>();
final List newDocuments = new ArrayList<>();
for (final Map.Entry> entry : stores.getValue().entrySet()) {
final String docID = entry.getKey();
final List content = entry.getValue();
if (content == null || content.isEmpty()) {
if (logger.isTraceEnabled())
logger.trace("Deleting document [{}]", docID);
deleteIds.add(docID);
continue;
}
final SolrInputDocument doc = new SolrInputDocument();
doc.setField(getKeyFieldId(collectionName), docID);
final Map adds = collectFieldValues(content, collectionName, information);
adds.forEach((k, v) -> {
doc.setField(k, v);
getDualFieldName(k, information.get(collectionName, k))
.ifPresent(dk -> doc.setField(dk, v));
});
newDocuments.add(doc);
}
commitDeletes(collectionName, deleteIds);
commitChanges(collectionName, newDocuments);
}
} catch (final Exception e) {
throw new TemporaryBackendException("Could not restore Solr index", e);
}
}
// This method will create a map of field ids to values. In the case of multiValued fields,
// it will consolidate all the values into one List or Set so it can be updated with a single Solr operation
private Map collectFieldValues(List content, String collectionName,
KeyInformation.IndexRetriever information) throws BackendException {
final Map docs = new HashMap<>();
for (final IndexEntry addition: content) {
final KeyInformation keyInformation = information.get(collectionName, addition.field);
switch (keyInformation.getCardinality()) {
case SINGLE:
docs.put(addition.field, convertValue(addition.value));
break;
case SET:
if (!docs.containsKey(addition.field)) {
docs.put(addition.field, new HashSet<>());
}
((Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy