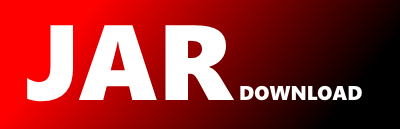
org.jasig.cas.web.flow.LogoutAction Maven / Gradle / Ivy
/*
* Licensed to Apereo under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Apereo licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a
* copy of the License at the following location:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.jasig.cas.web.flow;
import org.jasig.cas.authentication.principal.Service;
import org.jasig.cas.authentication.principal.SimpleWebApplicationServiceImpl;
import org.jasig.cas.logout.LogoutRequest;
import org.jasig.cas.logout.LogoutRequestStatus;
import org.jasig.cas.services.RegisteredService;
import org.jasig.cas.services.ServicesManager;
import org.jasig.cas.web.support.WebUtils;
import org.springframework.webflow.execution.Event;
import org.springframework.webflow.execution.RequestContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.validation.constraints.NotNull;
import java.util.List;
/**
* Action to delete the TGT and the appropriate cookies.
* It also performs the back-channel SLO on the services accessed by the user during its browsing.
* After this back-channel SLO, a front-channel SLO can be started if some services require it.
* The final logout page or a redirection url is also computed in this action.
*
* @author Scott Battaglia
* @author Jerome Leleu
* @since 3.0.0
*/
public final class LogoutAction extends AbstractLogoutAction {
/** The services manager. */
@NotNull
private ServicesManager servicesManager;
/**
* Boolean to determine if we will redirect to any url provided in the
* service request parameter.
*/
private boolean followServiceRedirects;
@Override
protected Event doInternalExecute(final HttpServletRequest request, final HttpServletResponse response,
final RequestContext context) throws Exception {
boolean needFrontSlo = false;
putLogoutIndex(context, 0);
final List logoutRequests = WebUtils.getLogoutRequests(context);
if (logoutRequests != null) {
for (final LogoutRequest logoutRequest : logoutRequests) {
// if some logout request must still be attempted
if (logoutRequest.getStatus() == LogoutRequestStatus.NOT_ATTEMPTED) {
needFrontSlo = true;
break;
}
}
}
final String service = request.getParameter("service");
if (this.followServiceRedirects && service != null) {
final Service webAppService = new SimpleWebApplicationServiceImpl(service);
final RegisteredService rService = this.servicesManager.findServiceBy(webAppService);
if (rService != null && rService.getAccessStrategy().isServiceAccessAllowed()) {
context.getFlowScope().put("logoutRedirectUrl", service);
}
}
// there are some front services to logout, perform front SLO
if (needFrontSlo) {
return new Event(this, FRONT_EVENT);
} else {
// otherwise, finish the logout process
return new Event(this, FINISH_EVENT);
}
}
public void setFollowServiceRedirects(final boolean followServiceRedirects) {
this.followServiceRedirects = followServiceRedirects;
}
public void setServicesManager(final ServicesManager servicesManager) {
this.servicesManager = servicesManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy